Sorting in MATLAB allows you to arrange elements of arrays in a specified order—either ascending or descending—with built-in functions that simplify the process.
Here's a basic example of sorting a numeric array in ascending order:
% Create an array
A = [5, 3, 8, 1, 2];
% Sort the array in ascending order
sortedA = sort(A);
Understanding MATLAB Sorting Functions
Built-in Sorting Functions
sort() Function
The `sort()` function in MATLAB is a fundamental tool for arranging elements of an array in ascending or descending order. The syntax is straightforward, allowing users to sort vectors, matrices, and even multidimensional arrays easily.
To use the `sort()` function, the basic format is `B = sort(A)`, where `A` is the array to be sorted and `B` is the resulting sorted array. This function defaults to sorting in ascending order.
Example and Code Snippet:
% Example of using sort() in MATLAB
A = [3, 1, 4, 1, 5, 9];
B = sort(A);
disp(B); % Output: 1 1 3 4 5 9
In this example, the array `A` is sorted, and the result, `B`, secures the elements in ascending order.
sortrows() Function
When dealing with matrices or tables, sorting specific rows can be essential. The `sortrows()` function sorts the rows of a matrix based on the values of one or more specified columns.
Example and Code Snippet:
% Example of using sortrows() in MATLAB
A = [3, 1; 4, 2; 1, 5; 9, 6];
B = sortrows(A, 1);
disp(B); % Output: 1 5
% 3 1
% 4 2
% 9 6
Here, the matrix `A` is sorted based on the values in its first column, resulting in a reordered matrix `B`.
Advanced Sorting Techniques
Using the 'descend' Option with sort()
Sometimes, you may need to sort data in descending order. The `sort()` function accommodates this with an additional parameter, allowing you to specify the order.
Example and Code Snippet:
% Sorting in descending order
A = [3, 1, 4, 1, 5, 9];
B = sort(A, 'descend');
disp(B); % Output: 9 5 4 3 1 1
In this instance, `sort()` arranges the array `A` in descending order, returning the sorted array `B`.
Sorting Based on Multiple Columns with sortrows()
When your matrix contains multiple columns, you can sort based on more than one column. The `sortrows()` function enables this functionality by accepting an array of column indices to sort by.
Example and Code Snippet:
% Sorting a matrix based on multiple columns
A = [3, 1, 2; 4, 1, 0; 1, 5, 6; 9, 6, 7];
B = sortrows(A, [1, 2]); % Sort by 1st then by 2nd column
disp(B);
With `sortrows()` analyzing both the first and second columns of matrix `A`, the output displays the rows sorted first by the 1st column, then the 2nd.
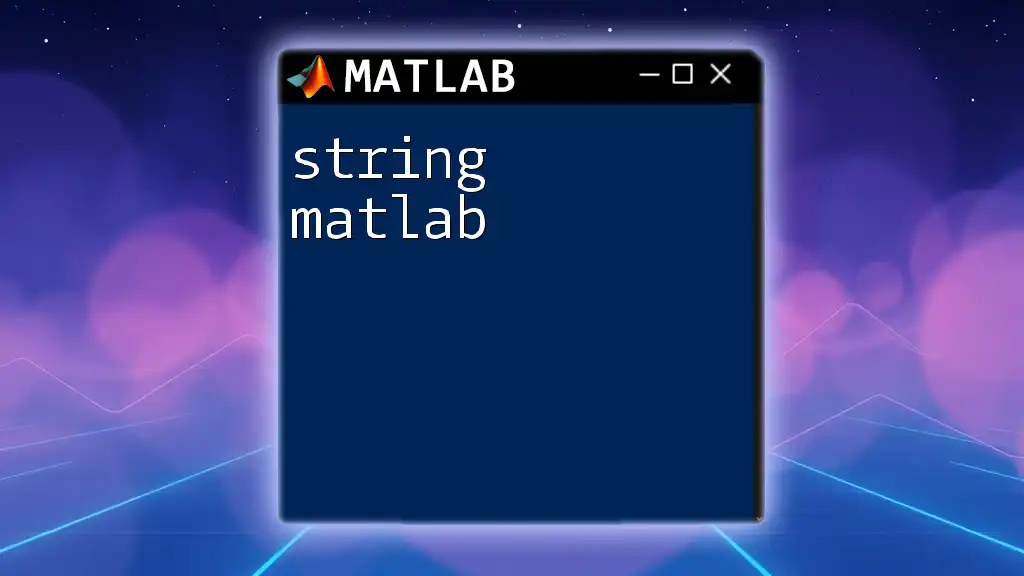
Creating Custom Sorting Functions
Writing Your Own Sorting Algorithm
While MATLAB's built-in functions are powerful, there may be instances where you need a custom sorting approach due to specific requirements or learning purposes. Designing your own sorting function can be an excellent way to enhance your understanding of algorithms.
Example: Implementing Bubble Sort in MATLAB
One of the simplest sorting algorithms is Bubble Sort, which repeatedly steps through the list, compares adjacent elements, and swaps them if they are in the wrong order.
Here's how you can implement Bubble Sort in MATLAB:
Code Snippet:
function sortedArray = bubbleSort(array)
n = length(array);
for i = 1:n
for j = 1:n-i
if array(j) > array(j+1)
% Swap elements
temp = array(j);
array(j) = array(j+1);
array(j+1) = temp;
end
end
end
sortedArray = array;
end
In this function, `bubbleSort()` takes an input array and processes it using the Bubble Sort algorithm, returning a newly sorted array.
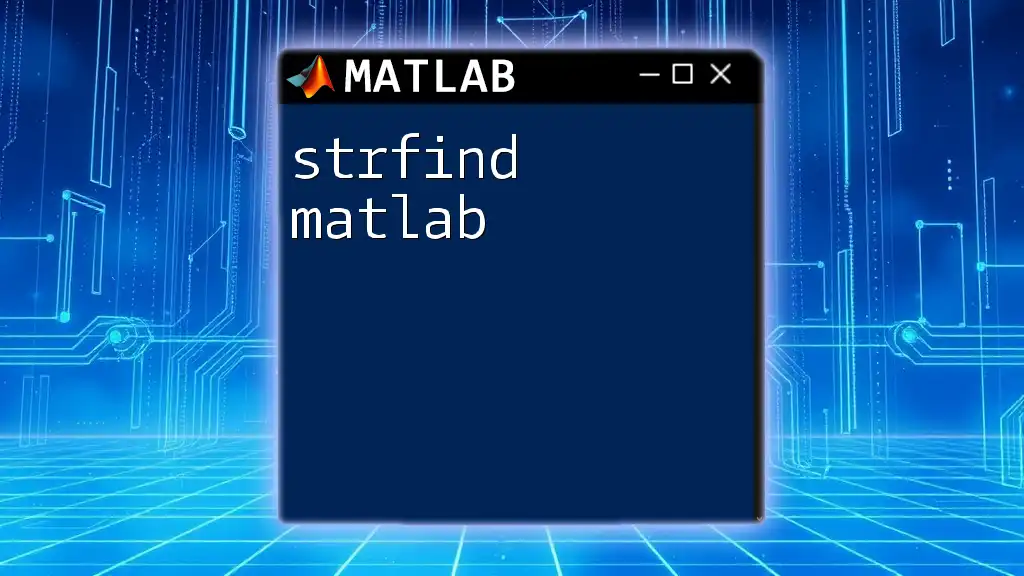
Real-World Applications of Sorting in MATLAB
Data Analysis and Visualization
In data analysis, sorting is often a crucial step, as it allows clearer and more insightful visualizations. When data is organized, it's far easier to spot trends, patterns, and outliers.
Example of Visualizing Sorted Data:
A = rand(1, 10);
sortedA = sort(A);
figure;
bar(sortedA);
title('Sorted Data Visualization');
xlabel('Index');
ylabel('Value');
In this snippet, a random array `A` is generated, sorted, and then displayed as a bar chart. This visualization helps to quickly grasp the data distribution.
Sorting Large Datasets
Handling large datasets can be a daunting task. MATLAB provides numerous features designed specifically for these scenarios, such as `tall` arrays, which allow users to perform operations on datasets too large to fit into memory. When sorting large datasets, consider these strategies to enhance performance:
- Optimize memory usage: Use data types that consume less memory.
- Leverage built-in functions: They are often optimized for speed and memory efficiency compared to developing custom solutions.
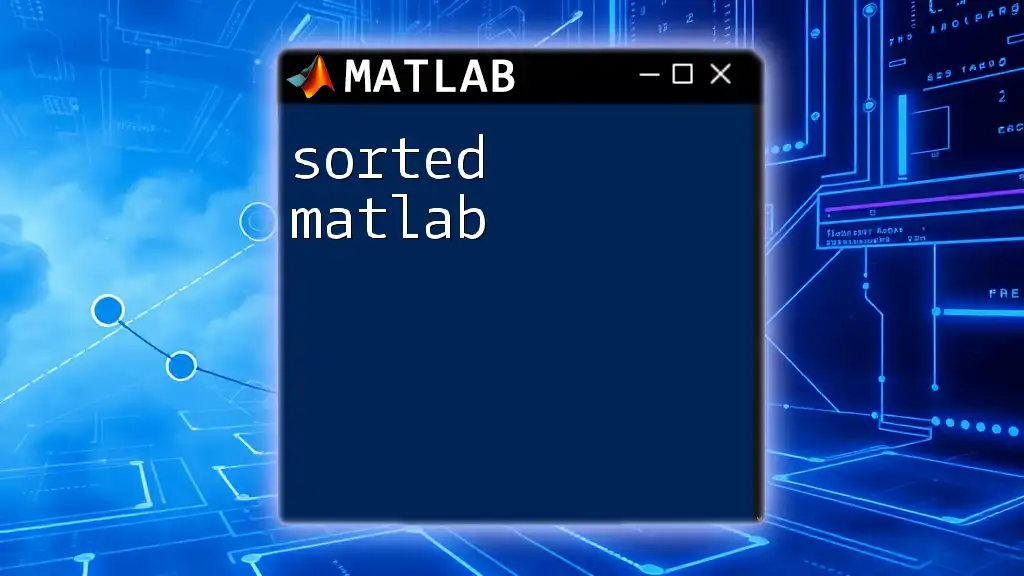
Conclusion
Sorting in MATLAB is an integral part of data processing that extends across both basic and advanced functionalities. With built-in functions like `sort()` and `sortrows()`, as well as the ability to create custom sorting algorithms, users can effectively manage and analyze their data. By practicing these techniques and utilizing sorting in your own projects, you'll unlock the potential of MATLAB in data analysis and visualization. Whether you're a beginner or an experienced user, mastering sorting will significantly enhance your capabilities within MATLAB.