In MATLAB, strings are sequences of characters and can be created using either single quotes for character vectors or double quotes for string arrays, enabling efficient text manipulation and storage.
Here's a simple example of working with strings in MATLAB:
% Creating a string and displaying its length
str1 = 'Hello, MATLAB!';
strLength = strlength(str1);
disp(['The length of the string is: ' num2str(strLength)]);
Creating Strings in MATLAB
Using Single Quotes
In MATLAB, strings can be created using single quotes. This method creates a character vector, which is a sequence of characters. For instance:
greeting = 'Hello, World!';
In this example, the variable `greeting` now holds the string "Hello, World!". Character vectors work great when you need to manipulate text in a straightforward manner.
Using Double Quotes
MATLAB also allows the creation of string arrays using double quotes. This feature is particularly useful when dealing with multiple strings. For example:
greetings = ["Hello", "World"];
Here, `greetings` is now a string array containing two elements. Double-quoted strings provide more functionality, such as easier manipulation and built-in support for operations like joining strings.
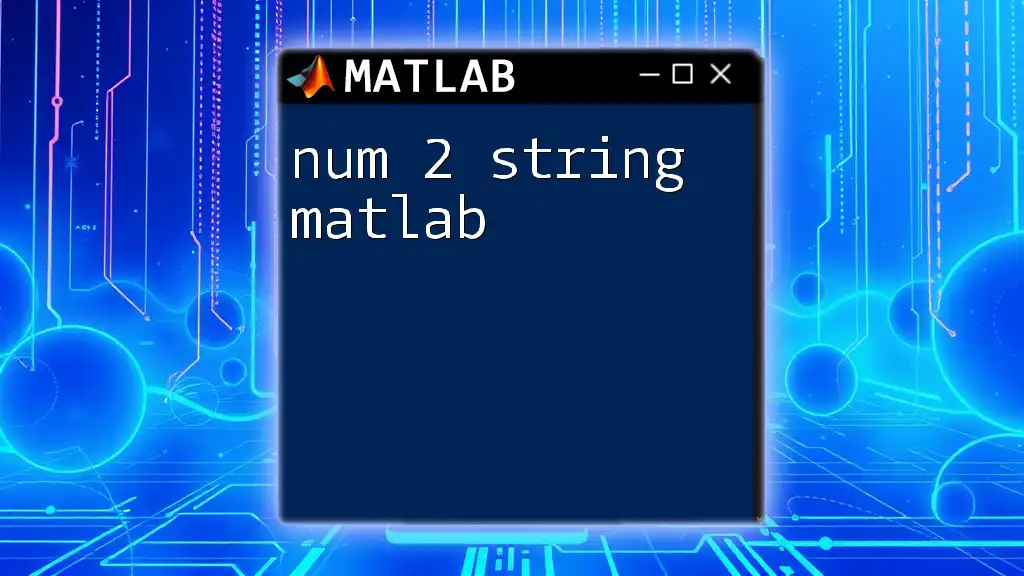
Working with Strings
Accessing Characters in Strings
One of the most fundamental operations when working with strings is indexing. You can access individual characters of a string using parentheses. For instance:
firstCharacter = greeting(1); % Returns 'H'
The variable `firstCharacter` now holds the first character of the `greeting`, which is 'H'. This ability to index into strings is essential for character manipulation.
Modifying Strings
Using Concatenation
Concatenating strings is a common operation when you need to combine text. In MATLAB, you can concatenate strings using the `+` operator:
fullGreeting = greeting + ' How are you?';
This will result in `fullGreeting` containing "Hello, World! How are you?".
Using Functions like `strcat`
Another way to concatenate strings is to use the built-in function `strcat`, which joins arrays of strings without adding any extra spaces:
fullGreeting = strcat(greeting, ' How are you?');
This method can be particularly useful when you're working with multiple strings.
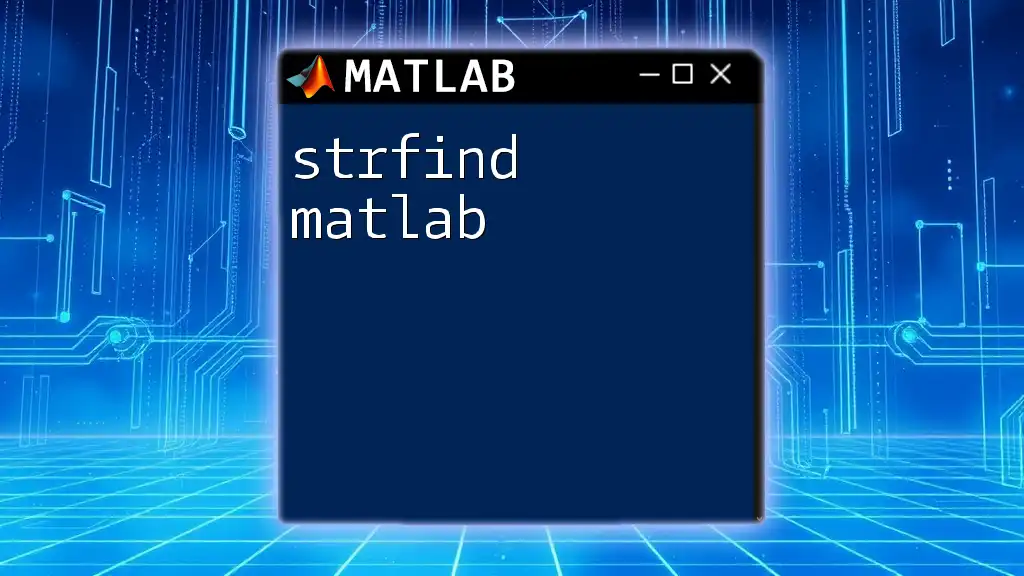
Common String Functions in MATLAB
String Length and Repetition
To find the length of a string, you can use the `strlength` function, which returns the number of characters in the string. For example:
lengthOfGreeting = strlength(greeting); % Returns 13
Furthermore, if you want to create a repeated string, you can use the `repmat` function:
repeatedGreeting = repmat(greeting, 2, 1); % Repeats the greeting
In this case, `repeatedGreeting` will contain "Hello, World!Hello, World!".
Searching and Replacing Strings
When working with strings, you often need to search for substrings or replace parts of strings. The `contains` function is handy for checking if a string contains a substring:
isContain = contains(greeting, 'World'); % Returns true
For more complex operations, the `replace` function is useful. If you want to replace a substring with another:
newGreeting = replace(greeting, 'World', 'MATLAB'); % Returns 'Hello, MATLAB!'
Comparing Strings
String comparison is another essential function in MATLAB. To check if two strings are identical, you can use the `strcmp` function:
isEqual = strcmp('hello', 'hello'); % Returns true
For case-insensitive comparison, utilize `strcmpi`:
isCaseInsensitiveEqual = strcmpi('Hello', 'hello'); % Returns true
These functions help you verify string identities seamlessly, which is crucial in many applications such as data validation.
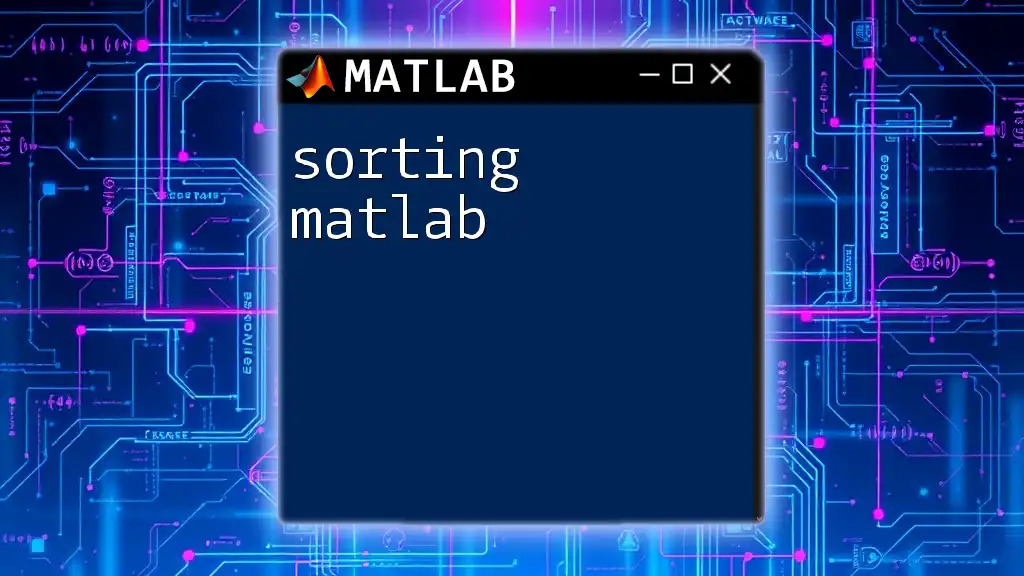
Formatting Strings
Customizing String Output
The `sprintf` and `fprintf` functions allow you to format strings for output. For example, when you need to create formatted strings, you can use:
formattedString = sprintf('The answer is %.2f', 42.0); % 'The answer is 42.00'
This approach is beneficial in cases where you need to present data in a specific format.
Joining Multiple Strings
When dealing with multiple strings, the `join` function can combine string arrays into single strings with specified delimiters. For instance:
combinedString = join(greetings, ', '); % 'Hello, World'
This function is particularly useful for creating readable outputs from collections of strings.
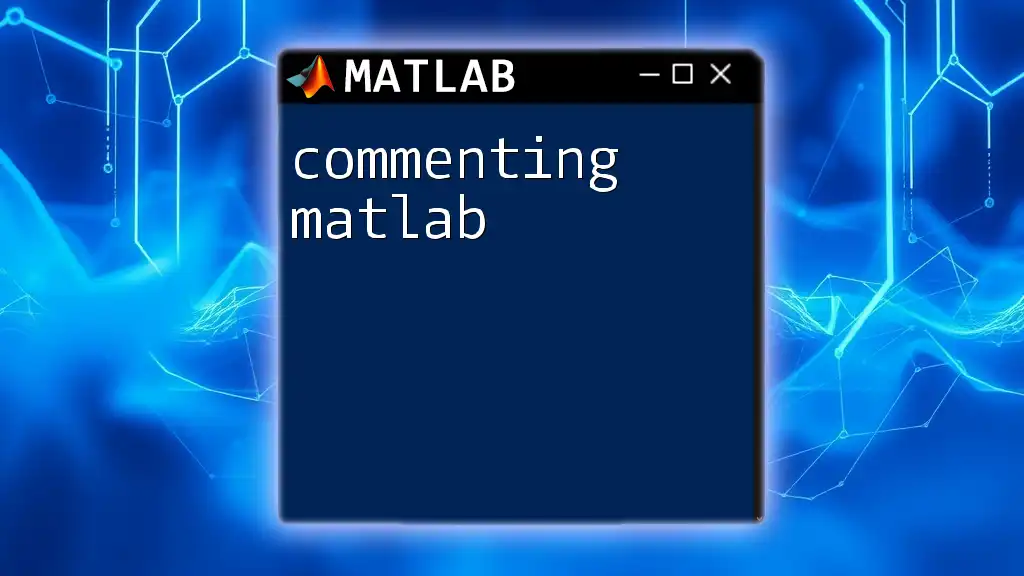
String Arrays vs. Character Vectors
Understanding the difference between string arrays and character vectors is critical for efficient programming in MATLAB. While character vectors are suitable for simple text manipulation, string arrays come with enhanced functionality and better performance characteristics.
When to Use Each Type?
- Character Vectors: Use these when performance is not a major issue and you are dealing with basic text.
- String Arrays: Prefer these for more complex string operations, especially when working with multiple strings or needing to apply vectorized operations.
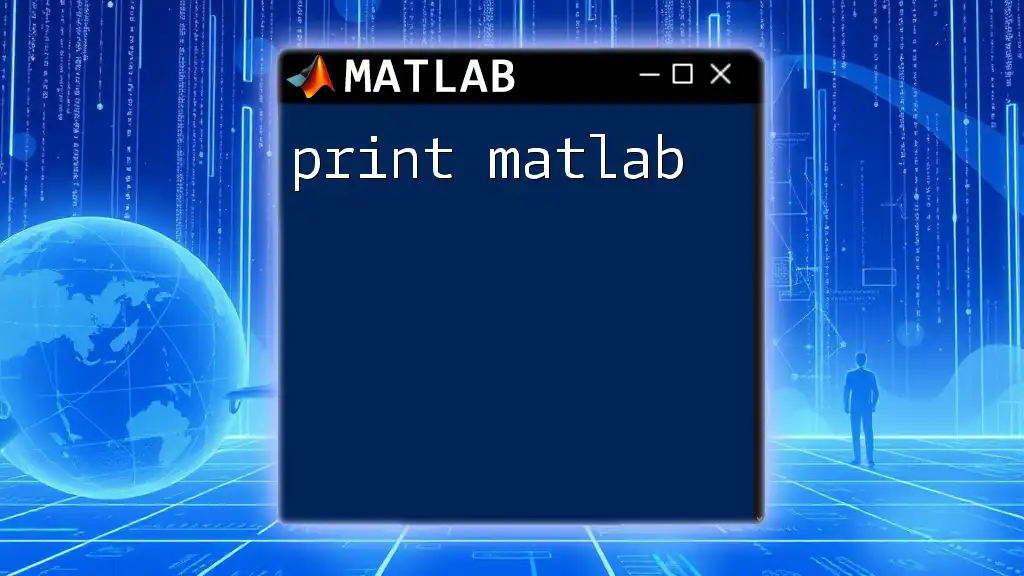
Error Handling with Strings
Working with strings does come with its set of common mistakes. Make sure to watch out for:
- Misspelling function names: MATLAB is case-sensitive.
- Indexing Errors: Accessing characters out of the bounds of the string.
- Using incompatible string types: Mixing character vectors with string arrays can lead to unexpected behavior.
Always validate your input and handle potential errors gracefully.
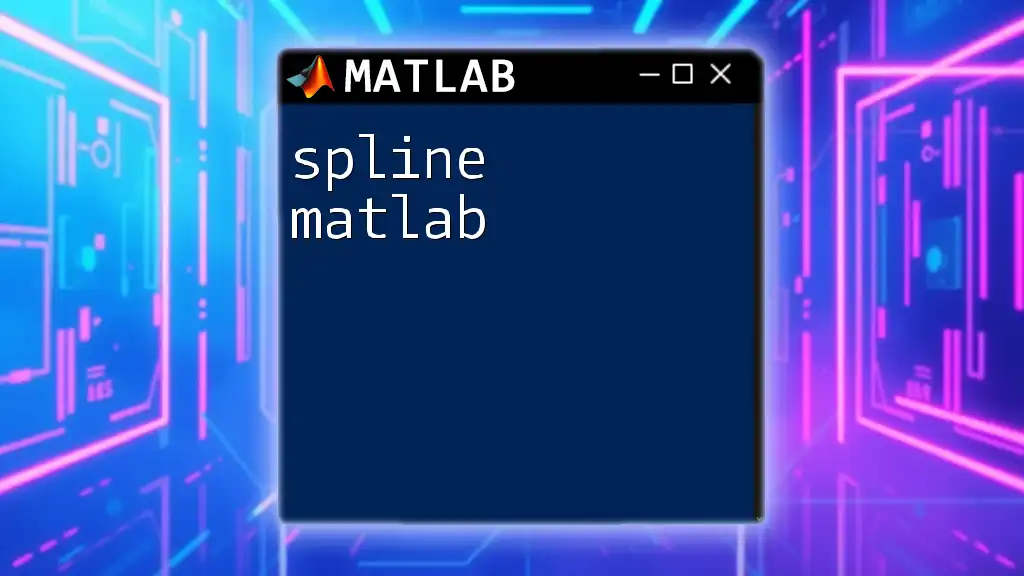
Conclusion
In working with strings in MATLAB, you have a robust set of tools at your disposal. From creating and modifying strings to performing complex searches and comparisons, understanding how to manipulate strings effectively is fundamental for any MATLAB user. Now, it's time to put this knowledge into practice and deepen your understanding of string manipulation in MATLAB. Happy coding!
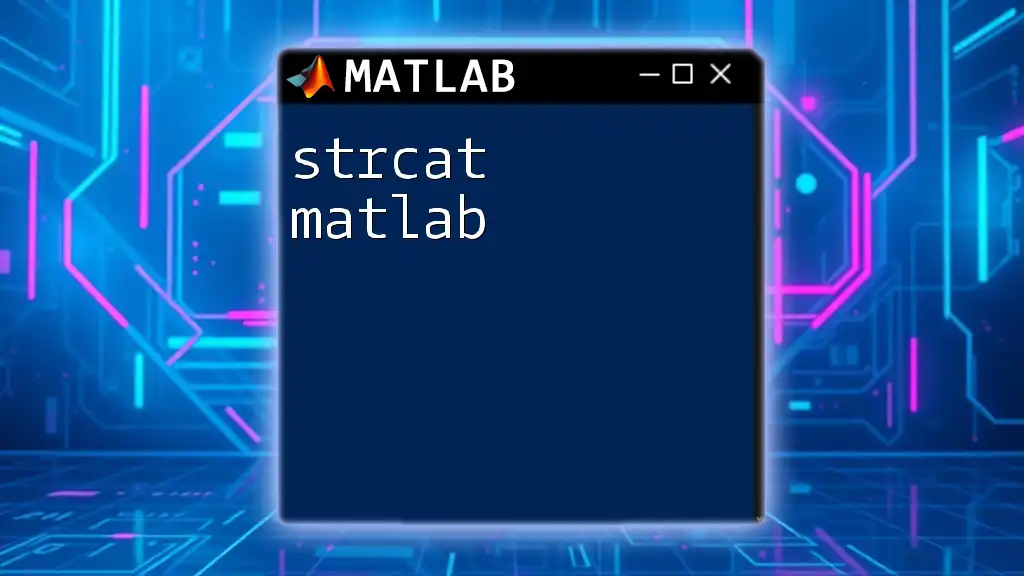
Further Reading and Resources
For a more in-depth understanding, consider consulting the official MATLAB documentation or exploring recommended books and tutorials that focus on string operations and their applications in various contexts.