The `strfind` function in MATLAB is used to find the starting indices of occurrences of a substring within a string.
str = 'Hello, welcome to MATLAB programming!';
substr = 'MATLAB';
indices = strfind(str, substr);
Understanding the Syntax of `strfind`
The `strfind` function is straightforward to use. The basic syntax for this function is:
strfind(haystack, needle)
Here, `haystack` is the string or array in which you want to search, and `needle` is the substring you are trying to find.
Parameters of `strfind`
In your usage of `strfind`, you will encounter two primary parameters:
- Haystack: This can be a single string or an array of characters where the search will take place.
- Needle: The substring that you are looking for within the haystack.
The function will return indices indicating the starting positions of needle within haystack. If the substring is not found, `strfind` will return an empty array.
Output of `strfind`
The function's output consists of indexes that show where the substring occurs in the main string. For instance, if your haystack is "Hello, MATLAB world!" and your needle is "MATLAB," the output will be 8, because "MATLAB" starts at the 8th character of the haystack.
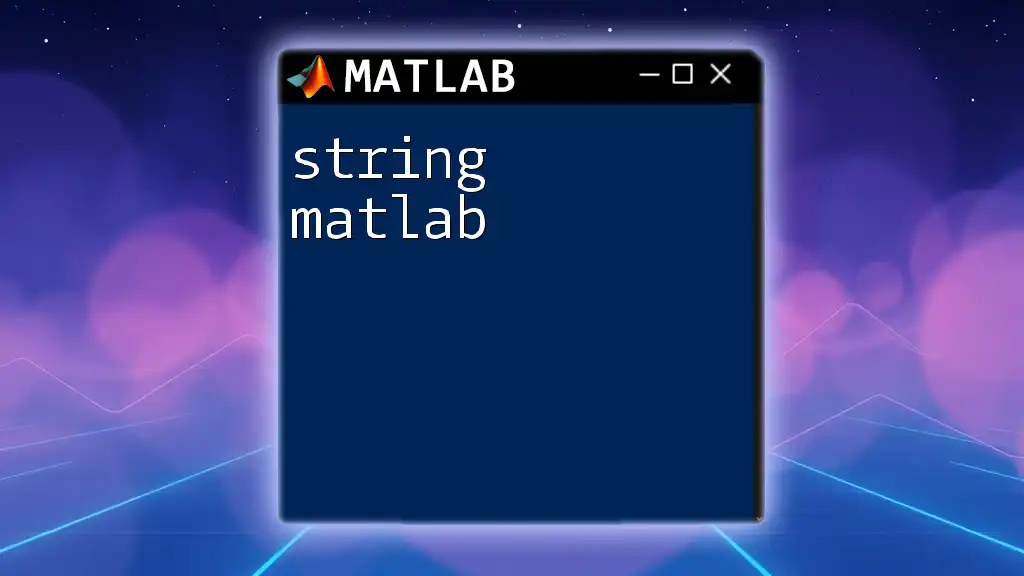
Practical Use Cases of `strfind`
Searching for Substrings
Using `strfind` for locating substrings is one of its most common applications. For example:
str = 'Hello, MATLAB world!';
subStr = 'MATLAB';
idx = strfind(str, subStr);
disp(idx); % Output: 8
In this example, we see that `strfind` correctly identifies that "MATLAB" starts at index 8. This is a crucial insight, especially for string analyses or text processing tasks.
Case Sensitivity in Searches
It's essential to note that `strfind` is case-sensitive, meaning that it differentiates between uppercase and lowercase letters. For example:
str = 'Hello, MATLAB World!';
subStr = 'matlab';
idx = strfind(str, subStr);
disp(idx); % Output: []
In this scenario, the output is an empty array because "matlab" in lowercase does not match "MATLAB" in uppercase. This distinction is vital to remember when performing searches that depend on specific letter casing.
Finding Multiple Occurrences
`strfind` is also capable of identifying multiple occurrences of a substring within a string. Consider the following example:
str = 'MATLAB is great. MATLAB is popular.';
subStr = 'MATLAB';
idx = strfind(str, subStr);
disp(idx); % Output: 1, 20
In this case, `strfind` returns an array with multiple indices, demonstrating its capability to find every instance of needle within haystack.
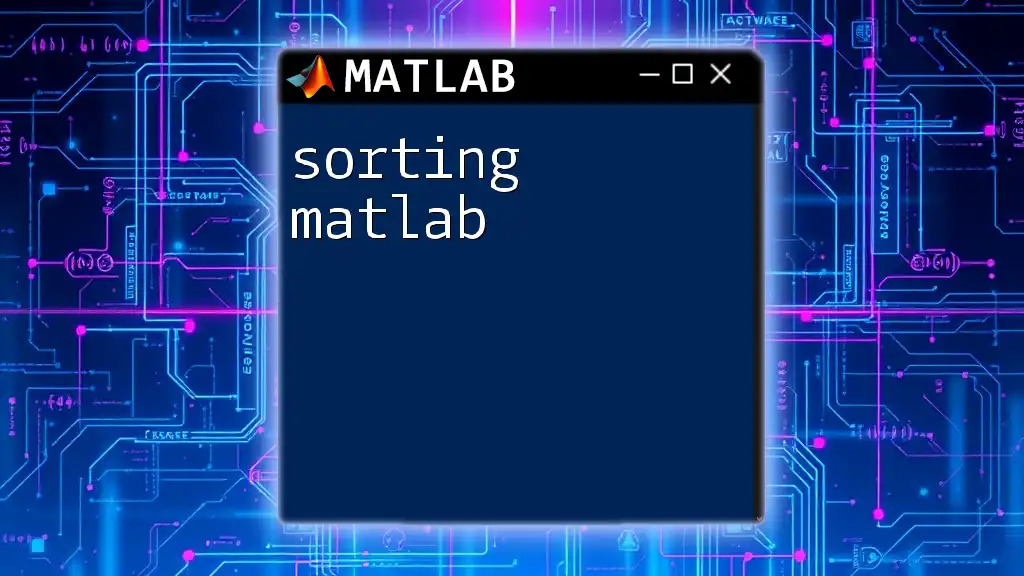
Advanced Features and Tips
Using `strfind` with Cell Arrays
You can also utilize `strfind` on cell arrays of strings. For example:
cellArray = {'Apple', 'MATLAB', 'Banana'};
idx = strfind(cellArray, 'MATLAB');
disp(idx); % Output: {[] 2 []}
Here, the output indicates that "MATLAB" was found in the second element of the cell array. The function returns indices in the form of a cell array, showcasing its flexibility with different data types.
Combining `strfind` with Other String Functions
You can often improve your use of `strfind` by combining it with other string functions in MATLAB. Two important functions to consider are `isempty` and `length`:
str = 'Learn MATLAB efficiently!';
subStr = 'Python';
idx = strfind(str, subStr);
if isempty(idx)
disp('Substring not found.');
else
disp('Substring found at index:');
disp(idx);
end
In this example, if the substring does not exist in the main string, the output will inform you accordingly. Combining these functions allows for a more robust and user-friendly approach to string searching.
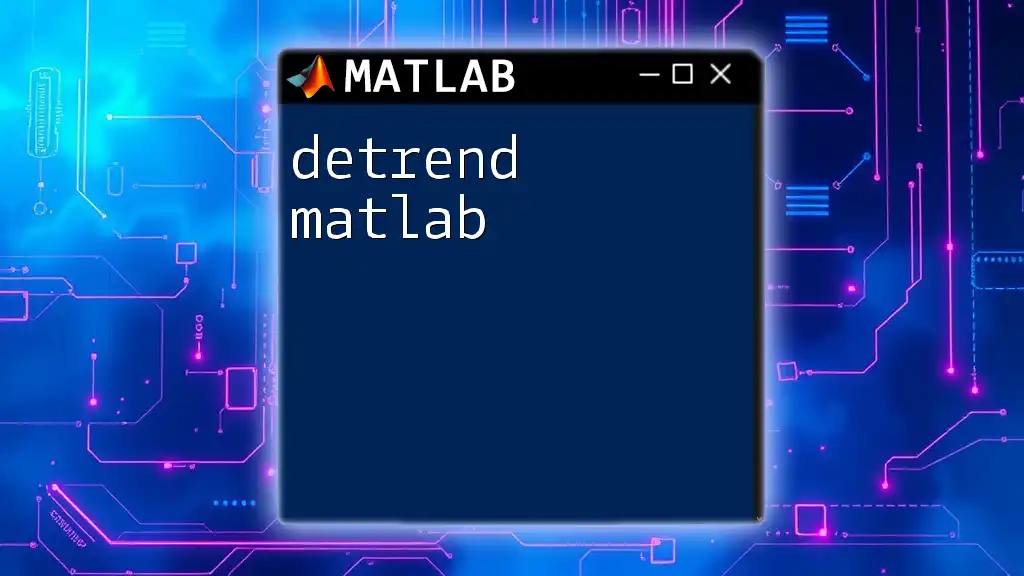
Performance Considerations
Efficiency of `strfind`
While `strfind` is generally efficient for small to moderate-sized strings, keep in mind that searching through very large texts may lead to longer processing times. It’s crucial to be aware of the potential performance implications, especially if your application requires frequent or large-scale searches.
Alternative Functions
MATLAB also offers related functions such as `contains`, `strmatch`, and `regexp`. Each of these commands has its own advantages:
- `contains`: This function allows for easier checks for the existence of substrings without returning indices.
- `strmatch`: Good for finding exact matches in a way that's less flexible than `strfind` but easier for certain applications.
- `regexp`: Useful for more complex pattern matching using regular expressions.
Choosing the right function depends on your specific needs. Understanding the differences can greatly enhance your string manipulation capabilities.
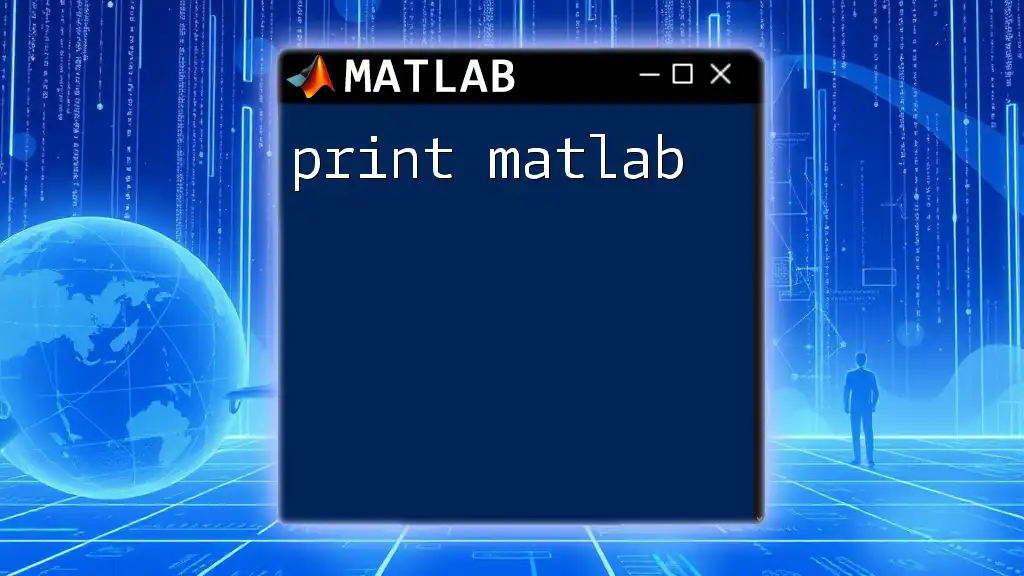
Common Errors and Troubleshooting
Common Mistakes with `strfind`
Users can encounter some typical errors when using `strfind`. Frequently, users might inadvertently search for substrings that are not present, resulting in an empty output array. Always double-check for typographical errors in your needle and consider the case sensitivity, as illustrated earlier.
Handling Unexpected Outputs
In cases where you receive unexpected results, implement debugging techniques such as printing the output of the indices before concluding the search. Remember that returning an empty array does not indicate failure; rather, it signifies that there were no matches found.
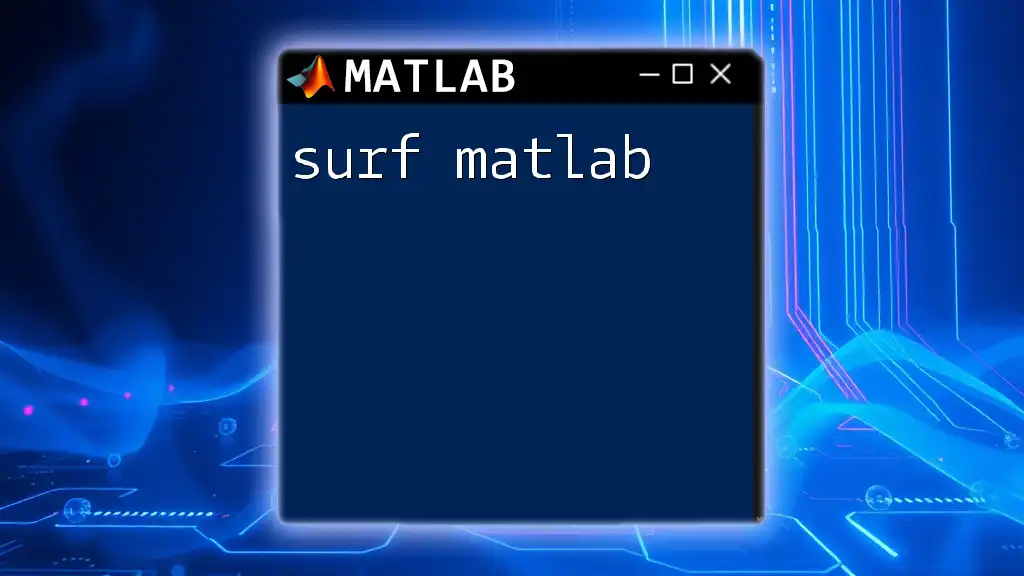
Conclusion
By mastering `strfind matlab`, you are equipped with a powerful tool for string searching and manipulation. Whether you are a beginner or an advanced user, integrating this function into your coding skill set will undoubtedly enhance your data processing capabilities. Be sure to practice with various examples and scenarios to truly harness the potential of `strfind` in MATLAB.