In MATLAB, an array of arrays, often referred to as a cell array, allows you to store multiple arrays of varying sizes and types in a single variable for versatile data management.
Here’s a code snippet demonstrating how to create and access a cell array in MATLAB:
% Creating a cell array containing an array of different sizes
myCellArray = {1:5, [10 20 30], magic(3)};
% Accessing elements of the cell array
firstArray = myCellArray{1}; % Accesses the first array: [1 2 3 4 5]
secondArray = myCellArray{2}; % Accesses the second array: [10 20 30]
thirdArray = myCellArray{3}; % Accesses the third array: magic(3)
Understanding MATLAB Arrays
Basic Array Concepts
In MATLAB, an array is a fundamental data structure that can hold multiple values in a single variable. It is critical to understand both one-dimensional and two-dimensional arrays, as they serve as the building blocks for more complex data manipulation.
1D arrays, or vectors, are straightforward lists of values, while 2D arrays, often referred to as matrices, consist of rows and columns. This combination allows MATLAB to perform powerful mathematical computations and analyses.
Initialization of Arrays
Creating arrays in MATLAB is intuitive. You can initialize both one-dimensional and two-dimensional arrays with simple syntax. Here are a couple of basic examples:
% Create a 1D array
array1D = [1, 2, 3, 4, 5];
% Create a 2D array (matrix)
array2D = [1, 2; 3, 4; 5, 6];
In these examples, `array1D` holds five values, while `array2D` contains three rows and two columns. The flexibility to shape your data in various dimensions is one of MATLAB's powerful features.

What is an Array of Arrays?
Defining Array of Arrays
An array of arrays refers to a data structure where one array contains other arrays as its elements. This layered approach allows for nested arrays that can vary in size and shape.
In MATLAB, cell arrays are commonly used to construct an array of arrays. Unlike regular arrays, which require uniform data types, cell arrays allow for mixed types, adding to their versatility.
Creating an Array of Arrays
To create an array of arrays in MATLAB, you can utilize cell arrays. Here's how you can define a cell array containing individual arrays:
% Creating a cell array (array of arrays)
arrayOfArrays = { [1, 2, 3], [4, 5], [6, 7, 8, 9] };
In this code snippet, `arrayOfArrays` holds three separate arrays of varying lengths, demonstrating how cell arrays can encapsulate differing data structures.
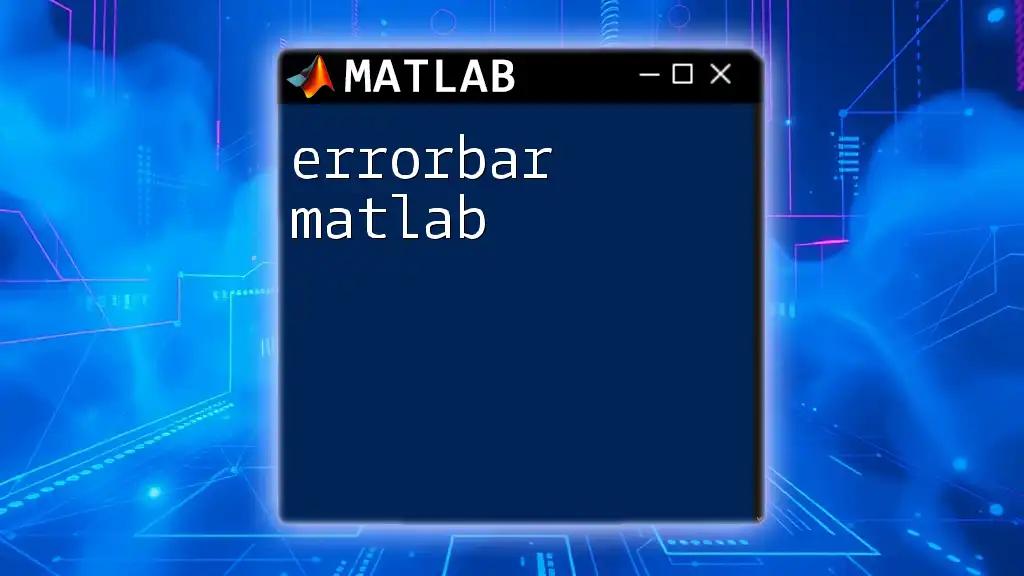
Manipulating Array of Arrays
Accessing Elements in an Array of Arrays
Accessing individual arrays within a cell array is straightforward. Use curly braces `{}` to reference the desired cell. For example:
% Accessing the second array
secondArray = arrayOfArrays{2};
This command assigns the second array, `[4, 5]`, to the variable `secondArray`, allowing for further manipulation or analysis.
Modifying Elements
Updating or modifying elements within an array of arrays using cell arrays is also simple. You can navigate to a specific array and change its contents easily. For instance:
% Modifying the first array
arrayOfArrays{1}(2) = 10; % Changes [1, 2, 3] to [1, 10, 3]
In this example, the second element of the first array is changed from `2` to `10`. This capability illustrates the dynamic nature of cell arrays.
Iterating Through an Array of Arrays
When you want to process every array within your cell array, a `for` loop is effective. Here’s how you can iterate through each element:
for i = 1:length(arrayOfArrays)
disp(arrayOfArrays{i});
end
This loop will display each of the nested arrays, illustrating the contents clearly. Iteration is essential for tasks like data processing and analysis when you need to handle multiple datasets.
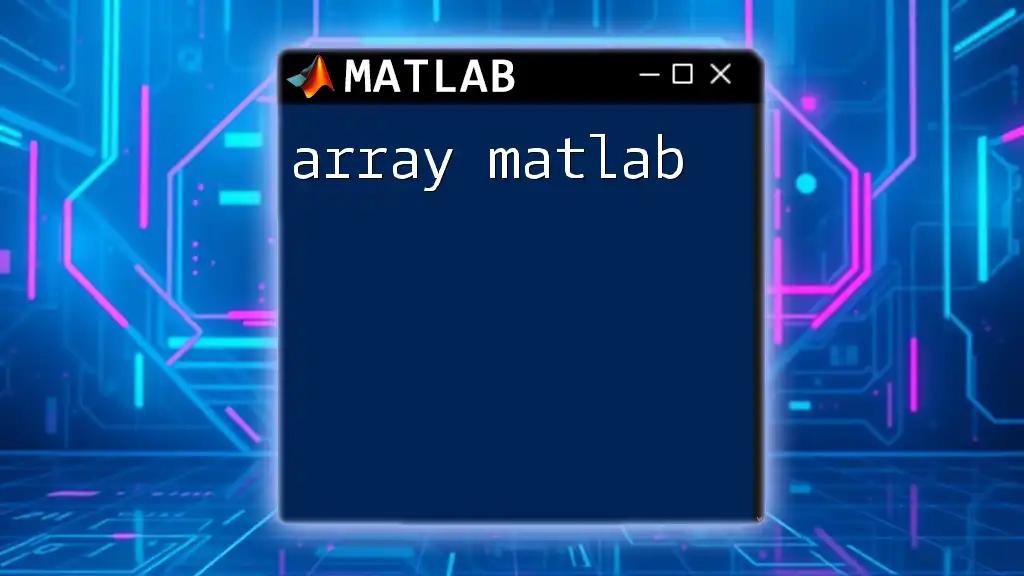
Applications of Array of Arrays in MATLAB
Use Cases in Data Manipulation
Arrays of arrays, particularly through cell arrays, can be beneficial when dealing with variable-length data. For example, if you are processing multiple datasets of differing sizes, an array of arrays allows you to store these efficiently without losing structure.
Examples in Data Analysis
Consider a scenario where you have collected scores from multiple tests, where each test has a variable number of participants. You can represent these scores as an array of arrays:
% Scores from different tests. Each test has different number of participants.
testScores = { [89, 92, 76], [88, 91], [85, 87, 90, 94] };
You could then perform operations such as calculating the average score for each test by traversing through `testScores`, using a similar iterating method as mentioned earlier.

Best Practices for Using Array of Arrays
Choosing Between Regular Arrays and Cell Arrays
Selecting the right array type hinges on the data structure you need. If your datasets are uniform and fixed in size, regular arrays might suffice. However, cell arrays shine when data lengths vary or when incorporating different data types.
Tips for Efficient Coding
When working with arrays of arrays in MATLAB, it’s crucial to avoid potential pitfalls for better performance and maintainability. For instance, always use cell arrays when your data elements differ in size or data type. This approach ensures your code remains clean and efficient.
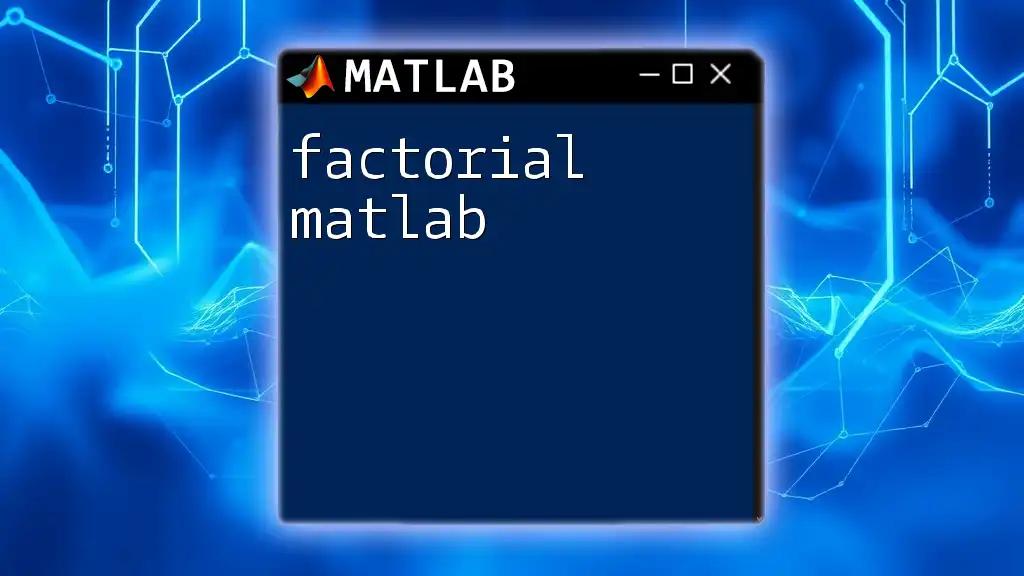
Conclusion
In summary, array of arrays in MATLAB presents a flexible method for managing complex data structures. By leveraging cell arrays, you can handle nested data efficiently, making MATLAB a powerful tool for numerical and data analysis tasks. Embrace the versatility they offer and explore their potential in your MATLAB projects for enhanced functionality.
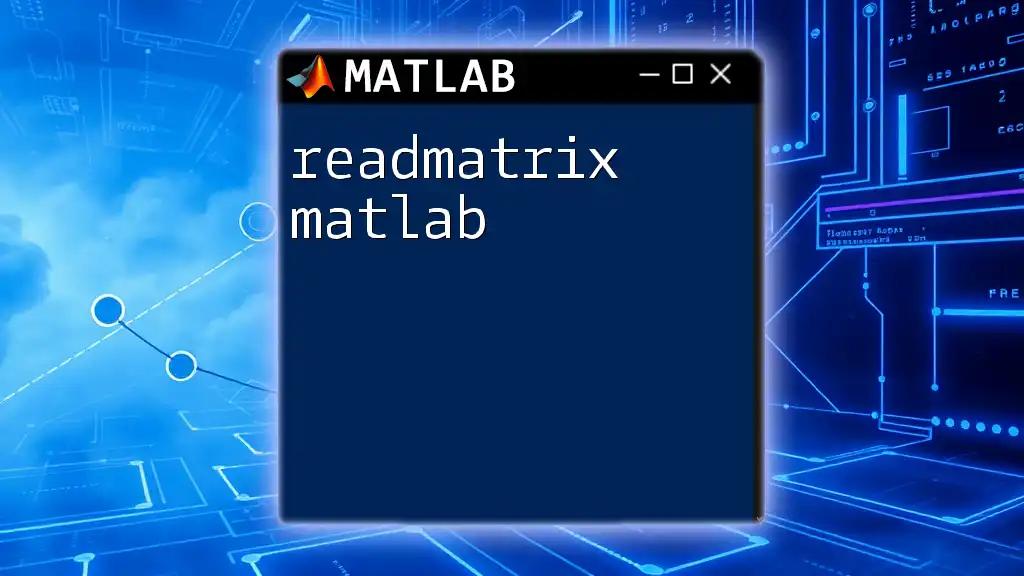
Additional Resources
For further learning about arrays and cell arrays in MATLAB, take advantage of the official MATLAB documentation and consider enrolling in MATLAB courses that delve deeper into data structuring techniques.