Cell arrays in MATLAB are data types that allow you to store arrays of varying sizes and types, making them ideal for handling heterogeneous data.
Here's a simple example of creating and accessing elements in a cell array:
% Create a cell array
myCellArray = {1, 'Hello', [2, 3; 4, 5]};
% Accessing elements in the cell array
number = myCellArray{1}; % Access the first element (1)
text = myCellArray{2}; % Access the second element ('Hello')
matrix = myCellArray{3}; % Access the third element ([2, 3; 4, 5])
Introduction to Cell Arrays
What is a Cell Array?
A cell array in MATLAB is a data structure that allows you to store arrays of varying types and sizes. Unlike traditional arrays, which require all elements to be of the same data type and dimension, cell arrays provide flexibility. This makes them especially useful for situations where you need to store mixed data types, such as strings, numbers, or even other arrays.
Why Use Cell Arrays?
The primary advantage of using cell arrays over regular arrays is their versatility. They can hold different data types within the same array and can accommodate varying sizes of data. For instance, you might want to store a list of student names, their ages, and their grades—all in one structure.
Real-world applications of cell arrays include organizing data responses from surveys, handling varied datasets in machine learning, or managing multiple results from experiments.
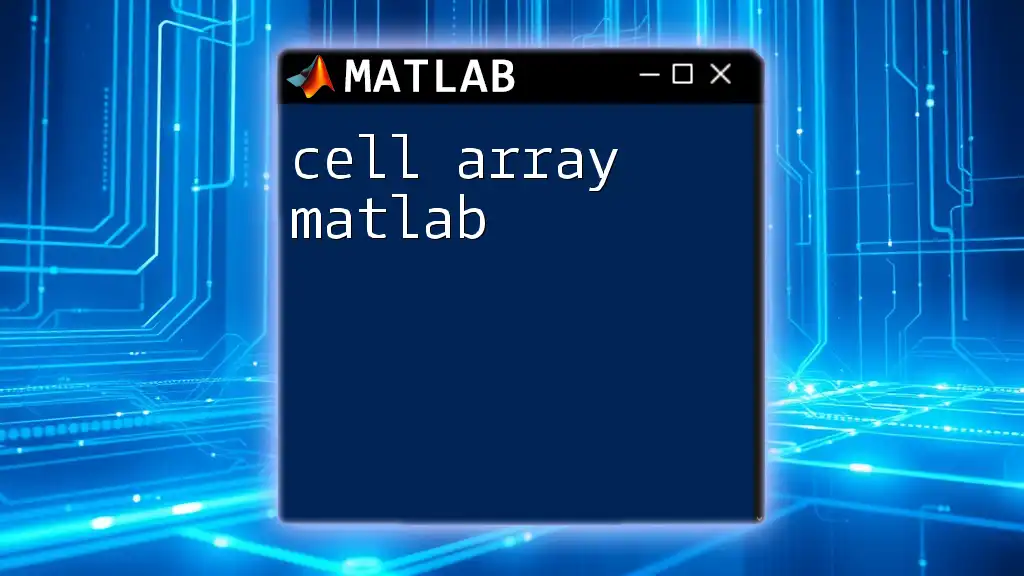
Structure of Cell Arrays
Creating Cell Arrays
Creating a cell array in MATLAB can be done in several ways. One common method is by using the `cell` function, which initializes a cell array of specified dimensions. However, you can also create a cell array by directly specifying its contents.
For instance:
C = {1, 'Hello', [1, 2, 3]};
In this example, `C` is a cell array containing an integer, a string, and another array.
Accessing Elements
Accessing elements within a cell array requires special notation. You can use curly braces `{}` to retrieve the content of a cell or parentheses `()` to obtain a sub-array.
For example:
singleElement = C{2}; % This accesses 'Hello' from the cell array
subArray = C(1:2); % This retrieves the first two cells as a sub-array
This distinction is crucial since using the wrong type of brackets can lead to unexpected results.
Modifying Elements
You can easily change an element in a cell array by using curly braces. Here’s how you can alter an element:
C{3} = [4, 5, 6]; % Modifying the third cell to contain a new array
This flexibility allows you to update specific contents without needing to recreate the entire array.
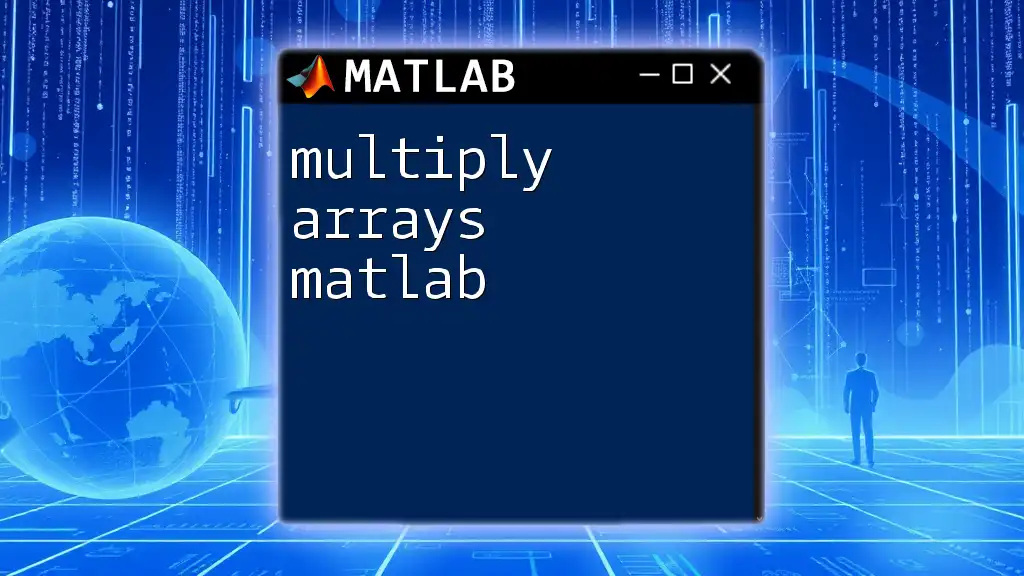
Manipulating Cell Arrays
Concatenating Cell Arrays
Cell arrays can be concatenated both horizontally and vertically. This means you can merge multiple cell arrays into one, either by side-by-side or top-down alignment.
For example:
C1 = {1, 2};
C2 = {'A', 'B'};
C_combined = [C1, C2]; % This performs a horizontal concatenation
The resulting cell array `C_combined` would now contain elements: `{1, 2, 'A', 'B'}`.
Cell Array Functions
MATLAB offers several built-in functions that enhance the manipulation of cell arrays. Some of the most useful functions include:
- `cellfun`: Applies a function to each cell of a cell array.
- `num2cell`: Converts a numeric array into a cell array.
- `struct2cell`: Converts a structure to a cell array.
For example, converting a numeric array to a cell array can be done as follows:
A = [1, 2, 3];
C = num2cell(A); % Converts the numeric array into a cell array
This allows further flexibility in handling diverse data types in your applications.
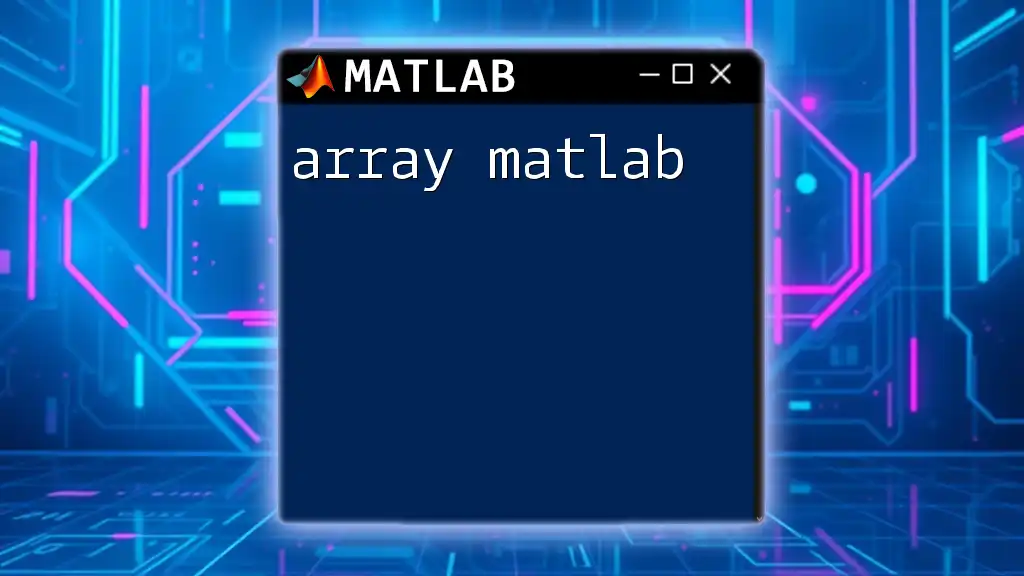
Advanced Topics
Nested Cell Arrays
A more complex structure is the nested cell array, where a cell can itself contain another cell array. This is particularly useful for multidimensional data.
Here’s how to create a nested cell array:
nestedC = {1, {2, 3}, 'Text'};
To access data within these nested structures, you can chain indexing:
innerValue = nestedC{2}{1}; % This retrieves '2' from the nested cell
Nested cell arrays enable you to model complex data relationships effectively.
Using Cell Arrays with Functions
Cell arrays can also be passed as arguments to functions. This allows for more flexible function designs that can handle various types of input.
For example:
function output = myFunction(inputCell)
output = inputCell{1} + inputCell{3}; % Summing first and third element of the cell array
end
In this case, `myFunction` takes a cell array and computes the sum of the first and third elements, showcasing how cell arrays facilitate diverse input handling.
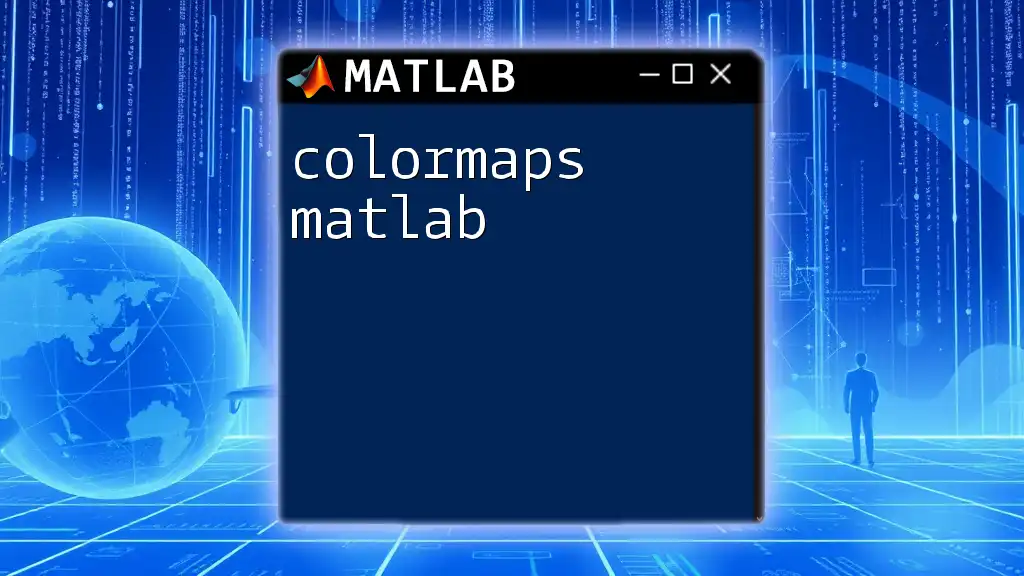
Common Pitfalls and Best Practices
Common Errors
When working with cell arrays, a common source of errors involves improper indexing. For example, attempting to access an element without using curly braces will return a cell instead of its content. This can lead to confusion, especially for those new to MATLAB.
Another error occurs with type mismatches. Be cautious of assumptions about the data types stored within the cells; you might run into unexpected results if the expected type doesn’t match.
Best Practices
When choosing between cell arrays and structures, consider the nature of your data. Use cell arrays for heterogeneous data types and structures when dealing with records or when a field-based approach is needed.
It's also essential to manage memory wisely, especially when dealing with large datasets. Cell arrays can consume more memory than traditional arrays, so assess performance implications before deciding on the data structure.
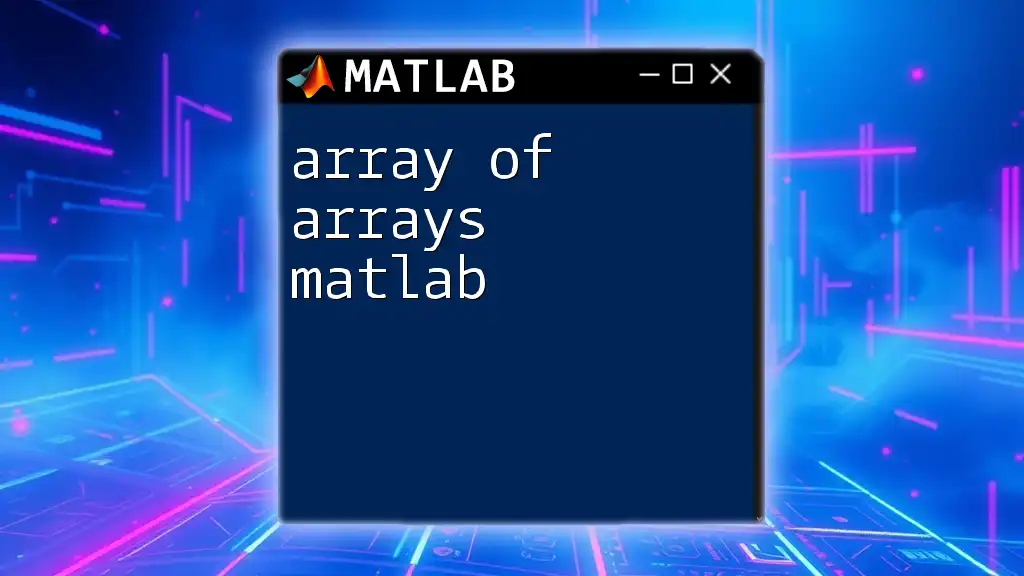
Conclusion
Cell arrays in MATLAB are a powerful tool when needing to manage diverse data types and sizes. Their flexibility allows you to address a range of programming challenges effectively. As you delve into MATLAB, mastering cell arrays will enable you to handle complex datasets and enhance your overall coding competency.
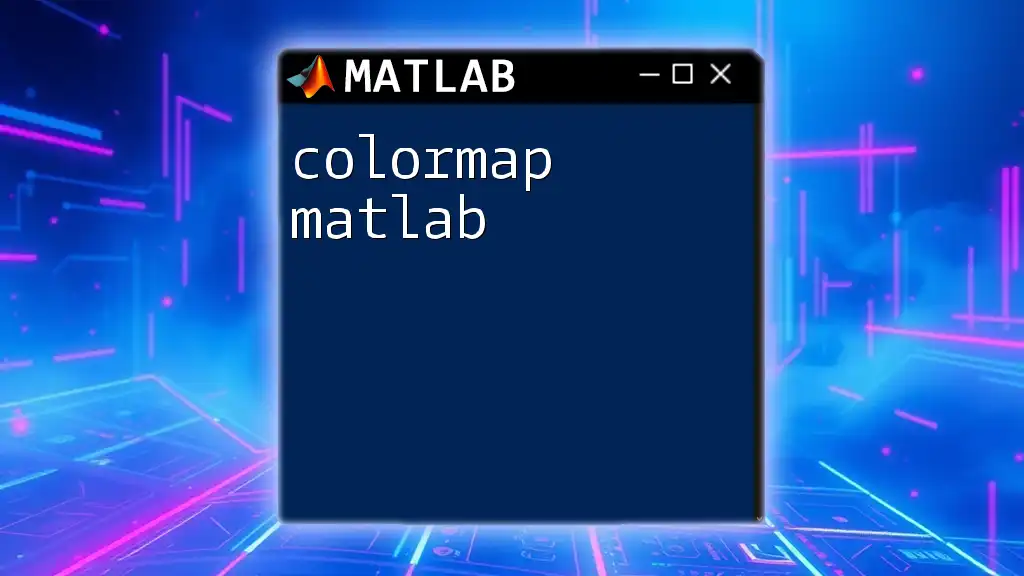
Frequently Asked Questions (FAQs)
What are the limitations of cell arrays?
While cell arrays offer significant advantages, they have limitations. They can lead to inefficient memory usage compared to numerical matrices and typically do not support vectorized operations, which can hinder performance in large-scale computations.
Can you apply mathematical operations to cell arrays directly?
Mathematical operations cannot be directly applied to cell arrays as you can with traditional numeric arrays. Instead, you'll first need to extract the required numeric data from the cells before performing calculations.
How do cell arrays compare to structures in MATLAB?
Cell arrays are ideal for storing heterogeneous data types, while structures are suited for labeled data that represents specific fields. Choose based on the use case: if data is organized by fields with names, use structures; if you're storing various data types together, prefer cell arrays.