In MATLAB, you can multiply two arrays element-wise using the `.*` operator to perform efficient mathematical operations on the corresponding elements of the arrays. Here's an example code snippet:
A = [1, 2, 3; 4, 5, 6];
B = [7, 8, 9; 10, 11, 12];
C = A .* B;
Understanding Arrays in MATLAB
What is an Array?
An array in MATLAB is a collection of elements organized in rows and columns. It's a fundamental data structure that allows users to perform numerical computations efficiently. Arrays can be classified into different types based on their dimensions:
- 1-D arrays (or vectors): One-dimensional arrays that can represent either a row or a column.
- 2-D arrays (or matrices): Two-dimensional arrays that represent data in rows and columns.
- Multi-dimensional arrays: Arrays that expand beyond two dimensions, often used in complex data analysis.
Arrays are central to MATLAB programming as they facilitate mathematical operations and data manipulations.
Creating Arrays
Creating arrays in MATLAB is straightforward. Here’s how you can create different types of arrays:
-
Row Arrays: Defined as a sequence of numbers enclosed in square brackets, separated by commas or spaces.
rowArray = [1, 2, 3];
-
Column Arrays: Defined with a semicolon separating the numbers.
colArray = [1; 2; 3];
-
Matrices: A two-dimensional grid of numbers also enclosed in square brackets, organized into rows and columns.
matrix = [1, 2; 3, 4];
It's beneficial to visualize arrays as they help in better understanding and debugging when programming in MATLAB.
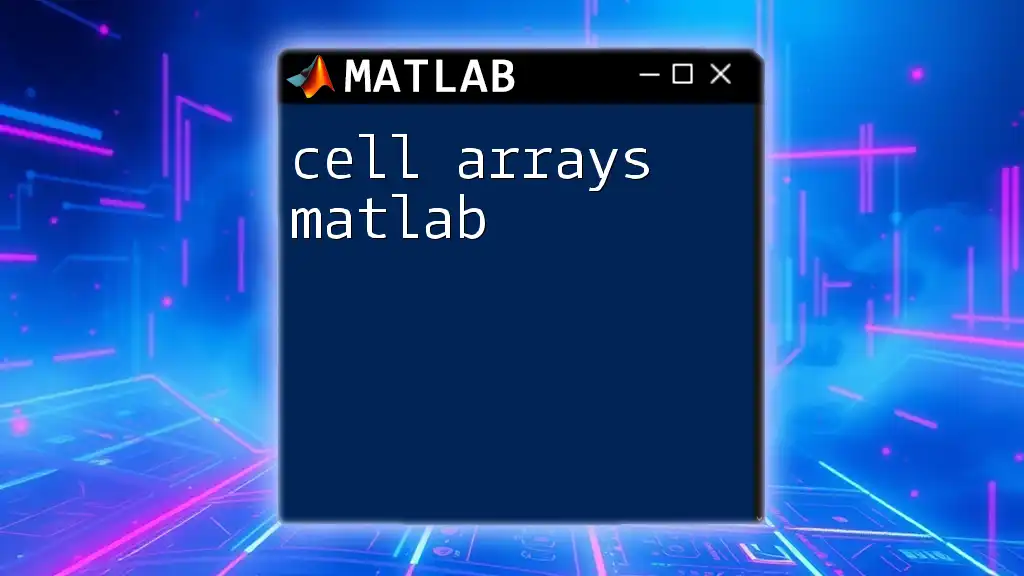
Methods of Multiplying Arrays in MATLAB
Element-wise Multiplication
Element-wise multiplication allows you to multiply corresponding elements of two arrays of the same size. This is performed using the `.*` operator.
Syntax:
C = A .* B;
Example:
A = [1, 2; 3, 4];
B = [5, 6; 7, 8];
C = A .* B; % C will be [5, 12; 21, 32]
In this case, each element of matrix A is multiplied with the corresponding element of matrix B. This method is particularly useful when you want to perform operations like scaling or modifying datasets.
Matrix Multiplication
Matrix multiplication, also known as linear algebra multiplication, is different from element-wise multiplication. Here, two matrices are multiplied according to specific algebraic rules involving the dot product of the rows and columns.
Syntax:
C = A * B;
Example:
A = [1, 2; 3, 4];
B = [5, 6; 7, 8];
C = A * B; % C will be [19, 22; 43, 50]
For matrix multiplication, it is essential that the number of columns in the first matrix (A) matches the number of rows in the second matrix (B). This multiplication is fundamental in solving systems of equations and transforming data sets.
Using the `dot` Function for Dot Products
The dot product of two vectors is another way to multiply arrays in MATLAB, yielding a single scalar value.
Syntax:
C = dot(A, B);
Example:
A = [1, 2, 3];
B = [4, 5, 6];
C = dot(A, B); % C will be 32
The dot product is particularly useful in many applications, including machine learning algorithms and computer graphics, where it provides a measure of similarity between two vectors.
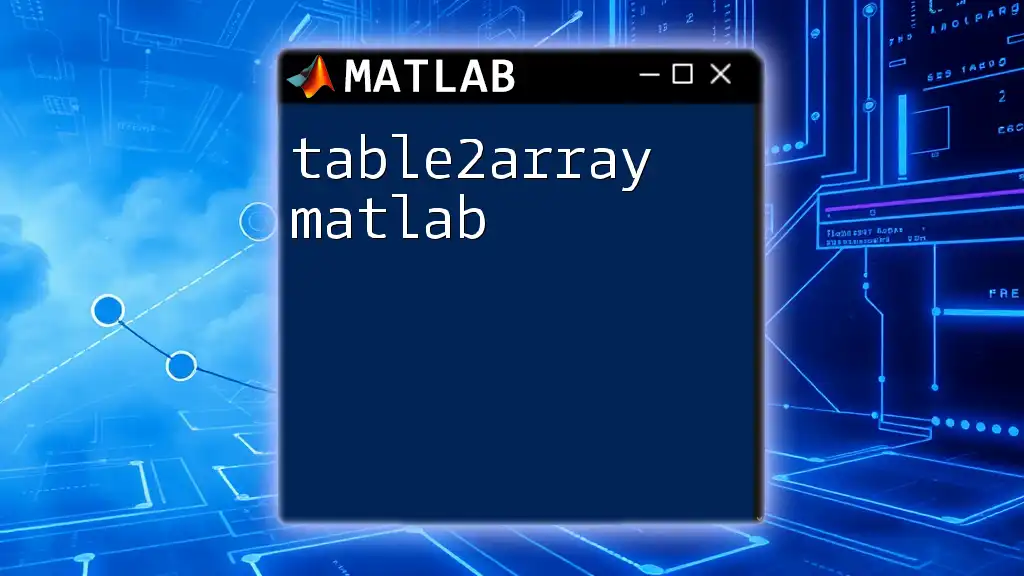
Special Cases in Array Multiplication
Multi-dimensional Arrays
In MATLAB, you can also multiply multi-dimensional arrays using either element-wise or matrix multiplication, depending on the context and dimensions of the arrays involved.
Example of element-wise multiplication for 3-D arrays:
A = rand(2, 3, 4);
B = rand(2, 3, 4);
C = A .* B; % Element-wise multiplication of 3-D arrays
This approach can be extended for any number of dimensions, enabling complex data manipulations needed in advanced computing techniques.
Handling Mismatched Dimensions
When working with arrays of different dimensions, MATLAB offers a feature called broadcasting. This allows you to perform operations efficiently even when the dimensions do not match.
Example:
A = [1; 2; 3];
B = [4, 5, 6];
C = A .* B; % Broadcasting applied
In this instance, MATLAB automatically expands the smaller array to match the size of the larger array during operations, facilitating seamless computations.
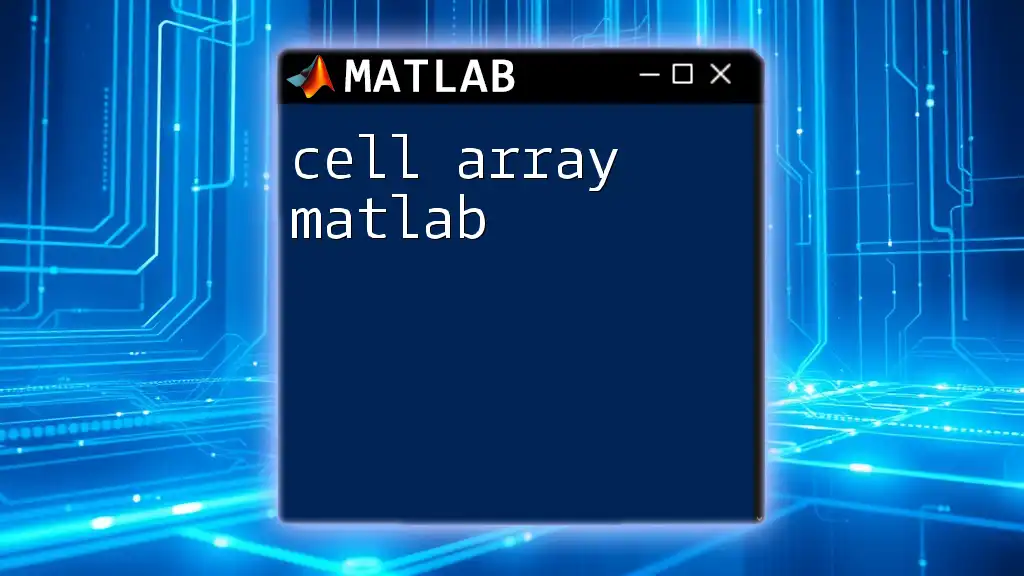
Best Practices for Array Multiplication in MATLAB
Performance Considerations
To ensure better performance during array multiplication, it is recommended to observe the following tips:
- Vectorization: Always prefer vectorized operations instead of using loops, as MATLAB is optimized for matrix and array operations.
- Preallocation: When creating large arrays, initialize them beforehand to save time and memory.
Debugging Common Errors
Common errors during array multiplication often arise from trying to multiply arrays with incompatible dimensions. When you encounter an error, check:
- The dimensions of the arrays using the `size` function.
- Ensure that the number of columns in the first array equals the number of rows in the second for matrix multiplication.
- Use element-wise multiplication when applicable to avoid dimension mismatch errors.
By following these guidelines, you can efficiently multiply arrays in MATLAB and enhance your programming skills.
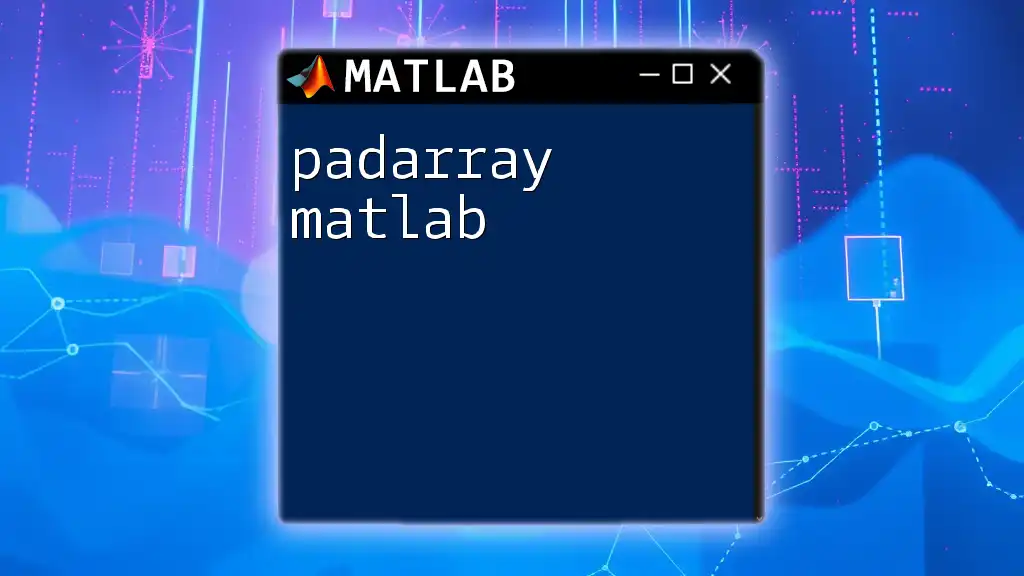
Conclusion
In summary, understanding how to multiply arrays in MATLAB is crucial for effective scientific computing and data analysis. By mastering element-wise multiplication, matrix multiplication, and using advanced operations like the dot product, you can greatly enhance the efficiency of your MATLAB programming. Practice these techniques in real-world scenarios to solidify your understanding and become proficient.
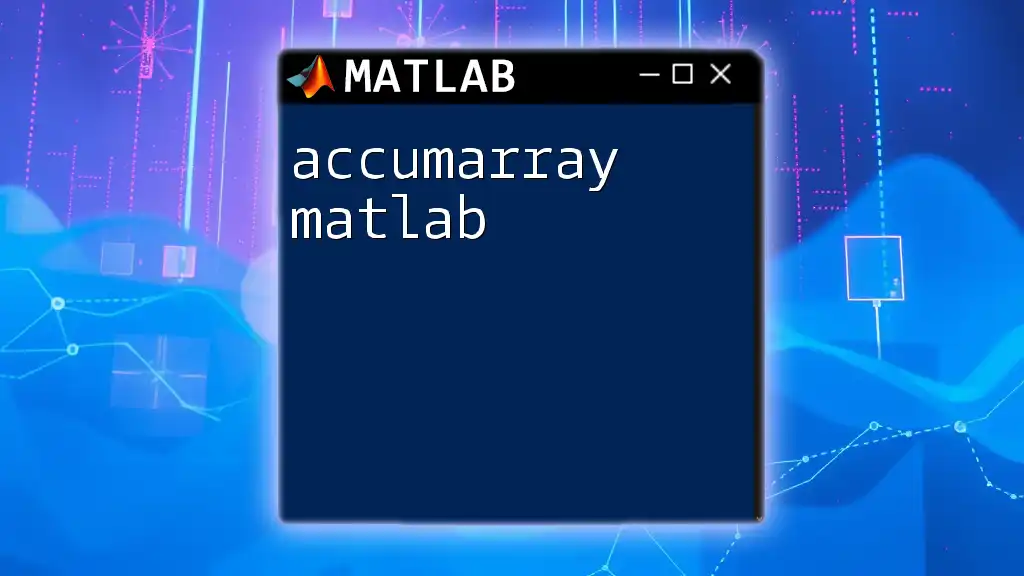
Additional Resources
For further reading and exploration, check out MATLAB's official documentation on array operations, join MATLAB user forums for community support, and consider exploring online courses that delve deeper into MATLAB programming and its applications.