The `errorbar` function in MATLAB is used to create a plot with error bars that represent the uncertainty or variability of data points.
Here is a code snippet demonstrating how to use the `errorbar` function:
x = 1:10; % x data points
y = rand(1,10); % y data points
err = 0.1 + 0.2*rand(1,10); % error values
errorbar(x, y, err, 'o'); % create error bar plot with circle markers
xlabel('X-axis');
ylabel('Y-axis');
title('Error Bar Example');
What is `errorbar` in MATLAB?
The `errorbar` function in MATLAB is a vital tool for visualizing uncertainty in experimental data. Error bars represent the range of variability for a data point, typically indicating a measurement's uncertainty or the standard deviation. By incorporating error bars into plots, researchers can better communicate the reliability and accuracy of their findings.
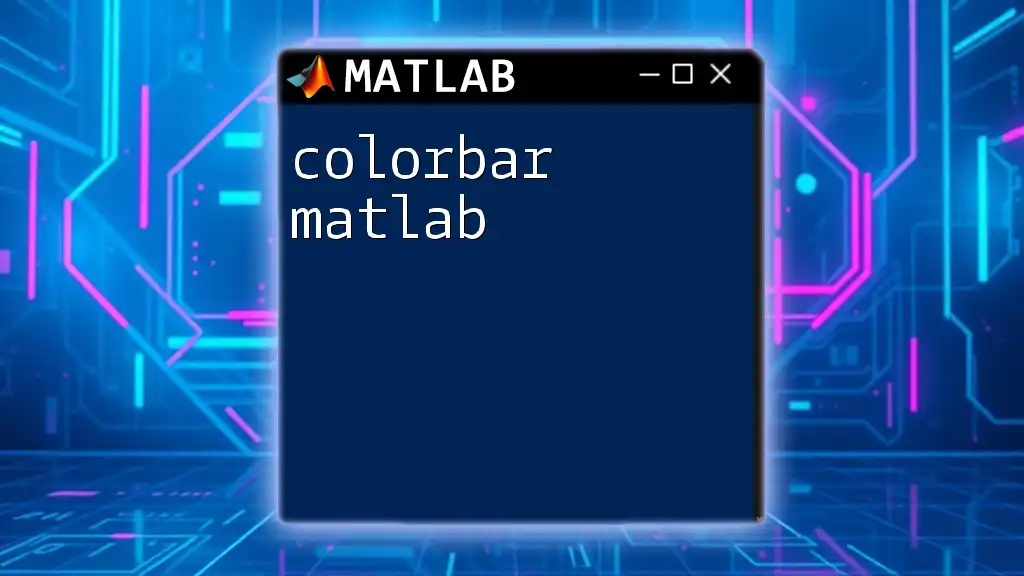
The Syntax of `errorbar`
To effectively use the `errorbar` function, it's essential to understand its syntax. The basic structure of the command is:
errorbar(x, y, e)
Basic Parameters
-
x: This parameter represents the data for the x-axis. It can be any numerical array that aligns with your dataset.
-
y: This parameter holds the data for the y-axis and, similarly to x, can be any numerical array.
-
e: Error values corresponding to the y-data, allowing for vertical error visualization. This array should be the same length as y, representing the uncertainty for each point.
Optional Parameters
`errorbar` also supports a variety of optional parameters that can enhance your plots. These include:
-
Line styles and marker types: You can customize the appearance using characters like `'-'` for solid lines or `'o'` for circles, among others.
-
Colors: You can specify colors using various naming conventions or RGB triplets.
-
Line width and marker size: Control visibility with parameters such as `'LineWidth', 1.5` and `'MarkerSize', 8`.
-
Labels and titles: Easily add clarity and emphasis to your plots using the MATLAB `xlabel`, `ylabel`, and `title` functions.
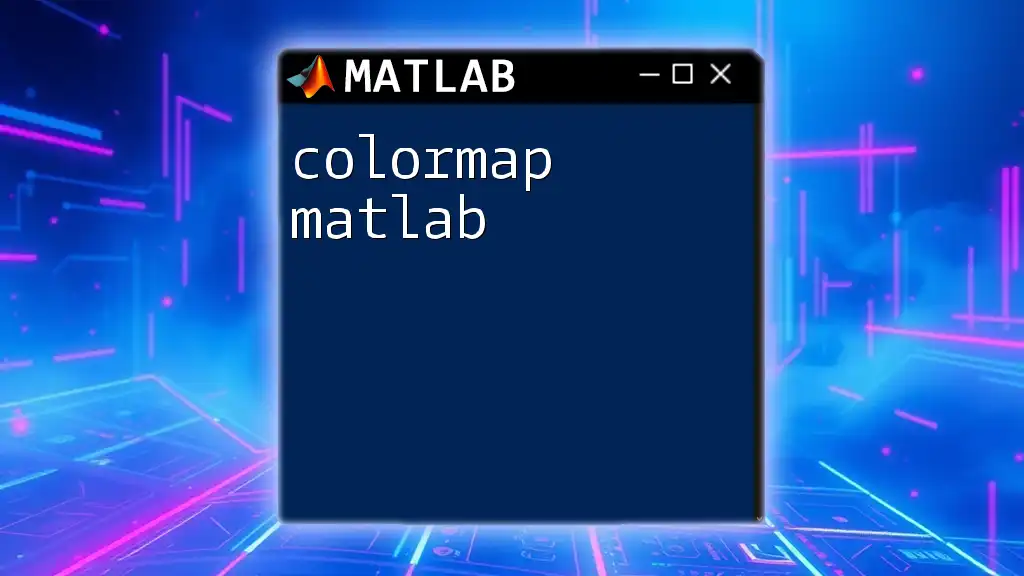
Creating a Simple `errorbar` Plot
Creating an error bar plot in MATLAB is straightforward. Below is a step-by-step guide with an example code snippet.
Sample Data Generation
First, let's generate some sample data. We use random values to simulate our y-values and corresponding errors:
x = 1:10; % X data
y = rand(1, 10); % Y data
e = rand(1, 10) * 0.1; % Error data
errorbar(x, y, e, 'o'); % Basic error bar plot
xlabel('X-axis');
ylabel('Y-axis');
title('Simple Error Bar Plot');
grid on;
In this example:
- `x` ranges from 1 to 10.
- `y` consists of random values between 0 and 1, representing the measured data points.
- `e` is also an array of random error values scaled by 0.1.
- The command `errorbar(x, y, e, 'o')` plots the data points with vertical error bars.
Explanation of the Code
In this code snippet, the `errorbar` function visually represents errors associated with each y value. Using `'o'` as an argument indicates that we want circular markers at each data point. This plot includes axes labels and a title, ensuring clarity.
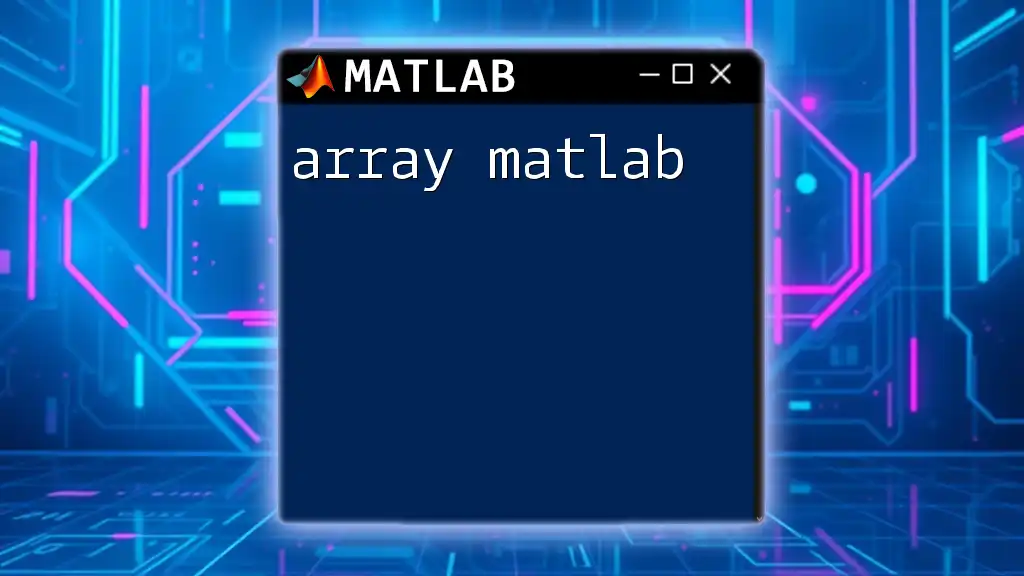
Customizing Your `errorbar` Plot
Customization is crucial for effectively communicating your data. You can easily change marker styles, colors, and other visual parameters. For instance:
errorbar(x, y, e, 'o--g', 'MarkerSize', 8, 'LineWidth', 1.5, 'DisplayName', 'Data with Error');
In this line:
- `'o--g'` specifies circular markers connected by a green dashed line for better visibility.
- The `MarkerSize` property increases the size of the markers, and `LineWidth` enhances line thickness.
- `DisplayName` adds a name to the plot, useful when displaying legends in multi-line graphs.
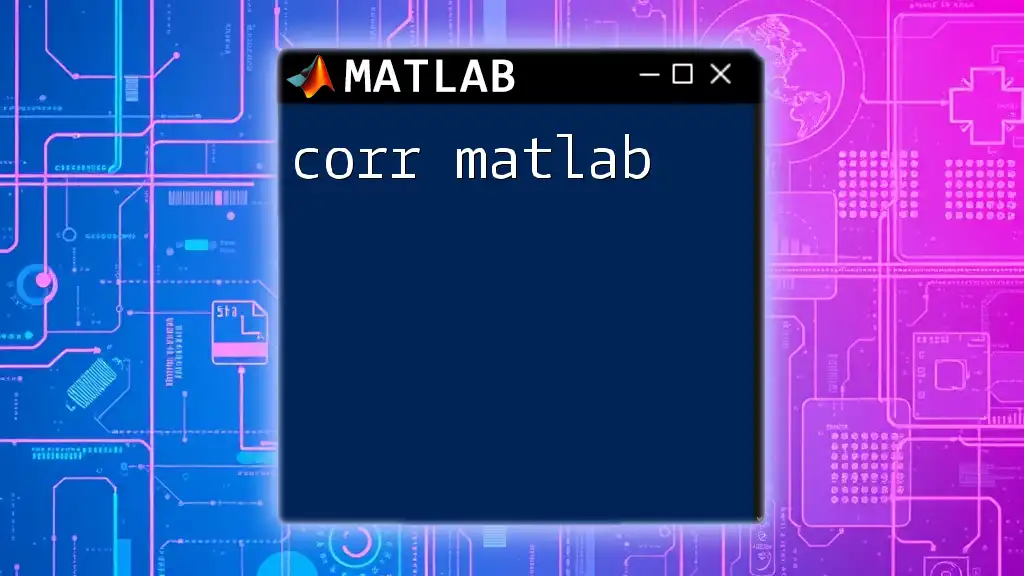
Adding Horizontal Error Bars
To incorporate horizontal error bars, you can modify the `errorbar` function to accept horizontal errors as an additional parameter. This allows you to represent uncertainty in both dimensions effectively:
e_h = rand(1, 10) * 0.1; % Horizontal error data
errorbar(x, y, e, e_h, 'o');
This example demonstrates how to plot errors in both the vertical and horizontal dimensions, giving a more detailed representation of data reliability.
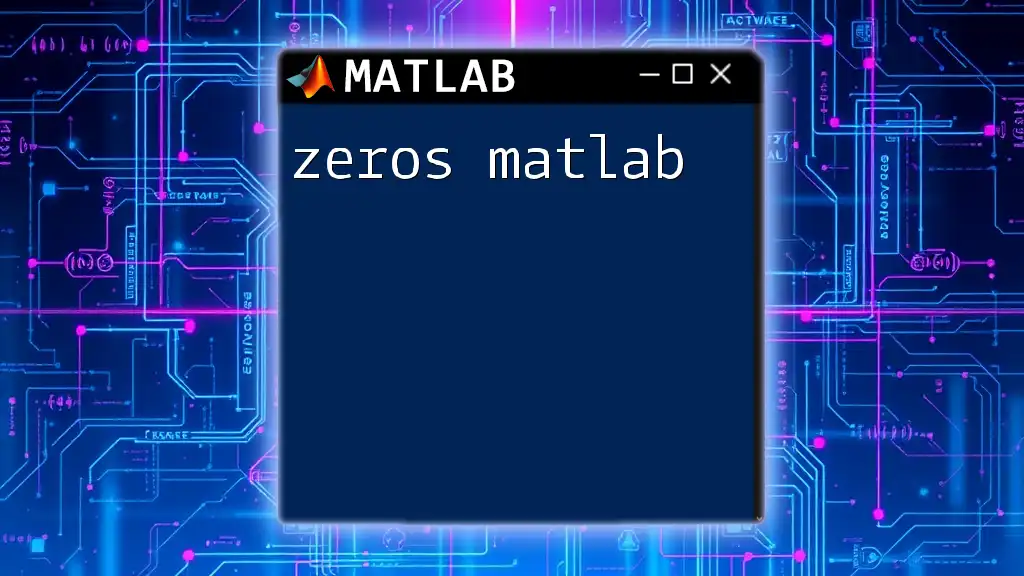
Handling Different Sizes of Error Data
When using the `errorbar` function, it’s crucial that your error data matches the dimensions of your dataset. If the lengths don’t match, MATLAB will return an error, such as "Dimensions of arrays being concatenated are not consistent." Always ensure that or reshape your data appropriately.
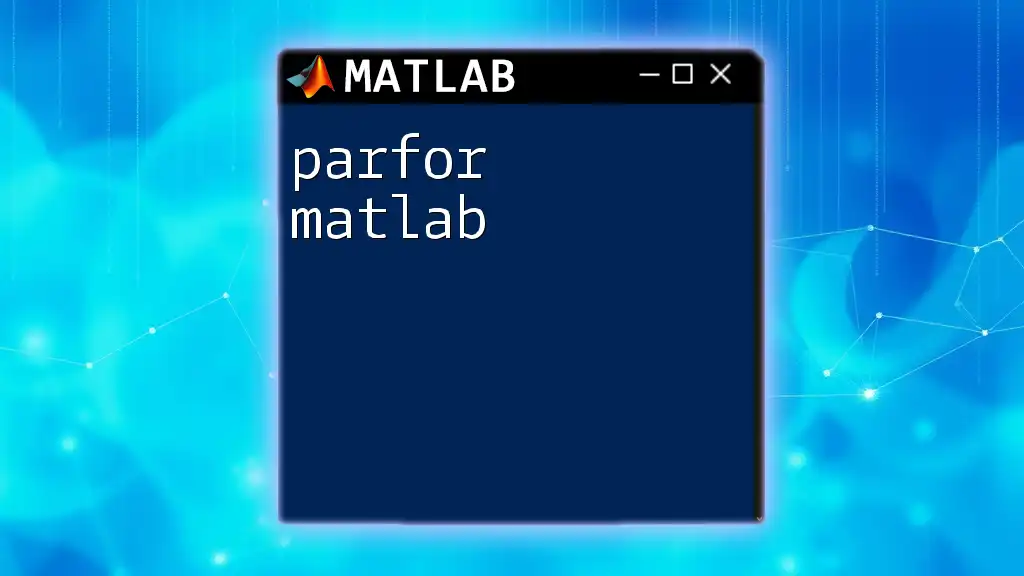
Practical Applications of `errorbar`
Error bars are commonly used in several practical scenarios, including:
-
Scientific research: Presenting experimental results with uncertainty information enhances the robustness of conclusions.
-
Engineering tests: In engineering fields, reporting tolerances and confidence intervals helps assess the reliability of materials and design processes.
-
Data analysis: Analysts often use error bars to visualize data variability during presentations and reports, enhancing interpretability for stakeholders.
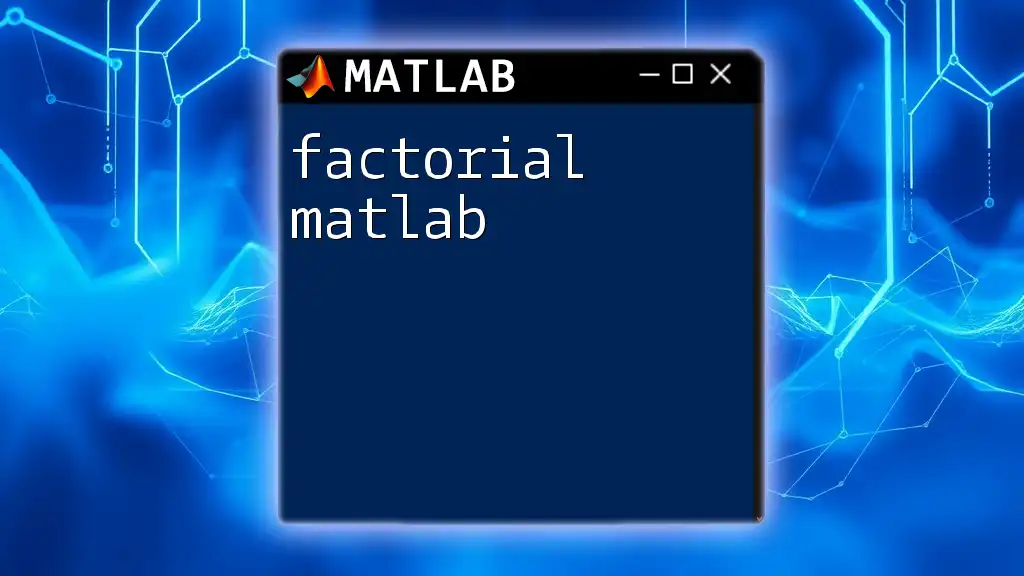
Troubleshooting Common Issues
While using the `errorbar` function, you may encounter typical pitfalls. Here are some common issues and solutions:
-
If you encounter dimension mismatch errors, verify that all input arrays (x, y, and e) are of compatible sizes. Ensure that error ranges are consistent with data points.
-
If your error bars are not displaying as expected, check your optional parameters and make sure they are specified correctly.
-
If the plot doesn’t appear, ensure that your current figure is not being overridden by subsequent plot commands. Use the `hold on` command to add multiple plots to the same figure without erasing previous ones.
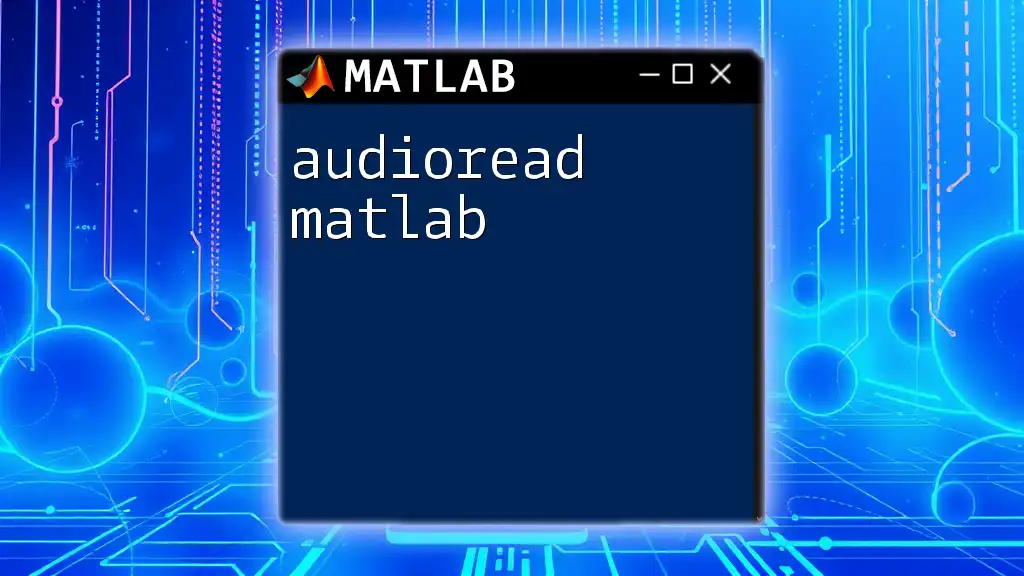
Conclusion
Utilizing the `errorbar` function in MATLAB is essential for visualizing the uncertainty associated with data. By incorporating error bars into your plots, you enhance the clarity of your research and enable better communication of results. Experiment with different parameters and customization options to find the most suitable representation of your data.
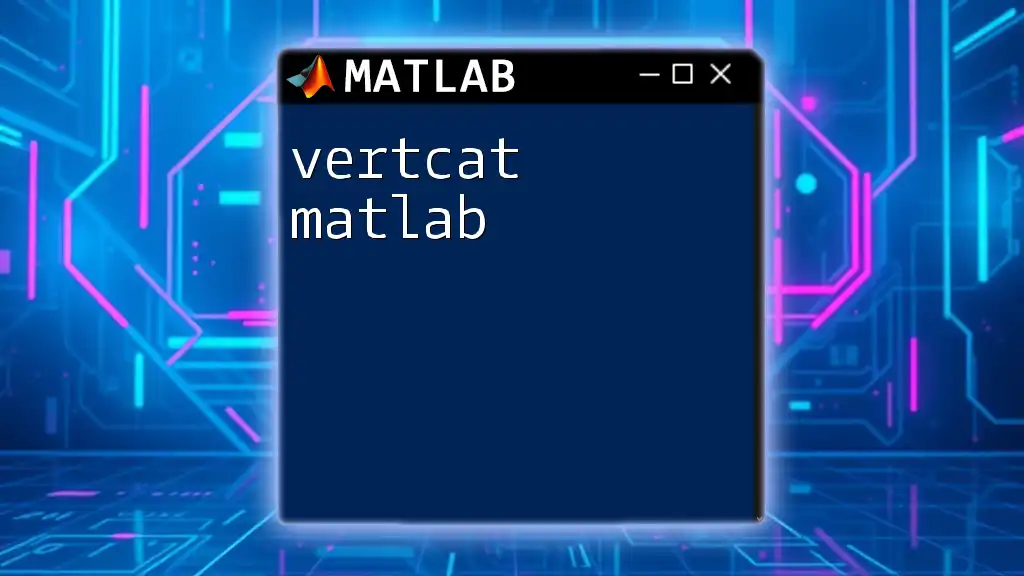
Additional Resources
For further exploration, consult MATLAB's official documentation on the `errorbar` function to discover additional features. Additionally, consider enrolling in online courses or tutorials focused on MATLAB for statistical analysis to deepen your understanding of this powerful tool.
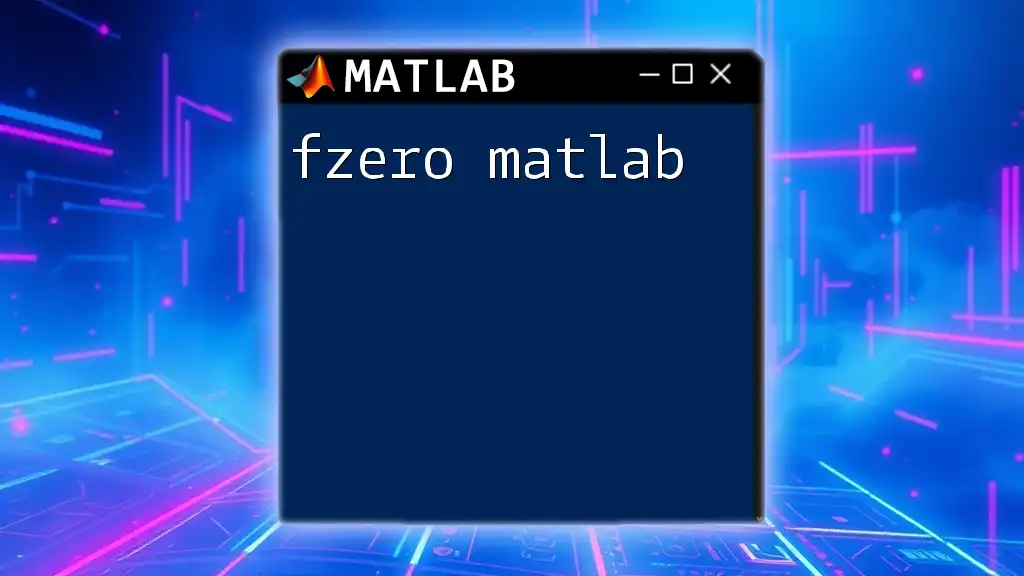
Call to Action
Explore more features in MATLAB to elevate your data visualization capabilities. Share your experiences, tips, or unique error bar designs created using MATLAB, and connect with the community to enhance collective learning!