The `atan2` function in MATLAB computes the four-quadrant inverse tangent of the given input values, allowing you to determine the angle from the X-axis to a point defined by its Y and X coordinates.
% Example of using atan2 to calculate the angle in radians
y = 5; % Y-coordinate
x = 3; % X-coordinate
theta = atan2(y, x); % Compute the angle
disp(theta); % Output the angle
Understanding `atan2`
What is `atan2`?
The `atan2` function is a two-argument function in MATLAB that computes the angle (or arctangent) between the positive x-axis and a point in the Cartesian plane defined by coordinates (x, y). This function is particularly useful for converting Cartesian coordinates to polar coordinates because it takes into account the signs of both arguments to determine the correct quadrant of the angle.
The Mathematical Background
Mathematically, `atan2(y, x)` returns the angle θ (in radians) such that:
- The tangent of θ is equal to \( \frac{y}{x} \)
- The angle θ lies in the correct quadrant based on the signs of x and y.
The advantage of using `atan2` over a simple arctangent function such as `atan(y/x)` is that `atan2` can correctly handle the ambiguity of angle values in different quadrants. The output ranges from -π to π, allowing for a full representation of the two-dimensional plane.
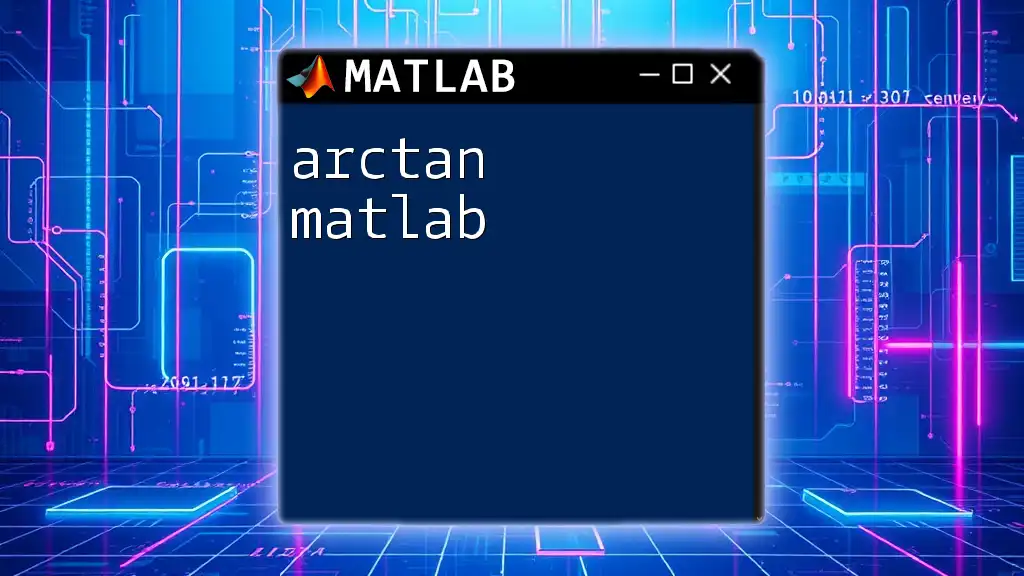
Syntax and Usage
The Syntax of `atan2`
The syntax for using the `atan2` function in MATLAB is straightforward:
theta = atan2(y, x)
In this syntax:
- `y`: The vertical coordinate (or opposite side) of the point.
- `x`: The horizontal coordinate (or adjacent side) of the point.
- `theta`: The output variable, storing the computed angle in radians.
Output Description
When you use `atan2`, you can expect the output to be an angle in radians that indicates the direction of the point (y, x) relative to the positive x-axis. It's important to note that `atan2` will automatically assess the values of y and x to provide the angle in the correct quadrant.
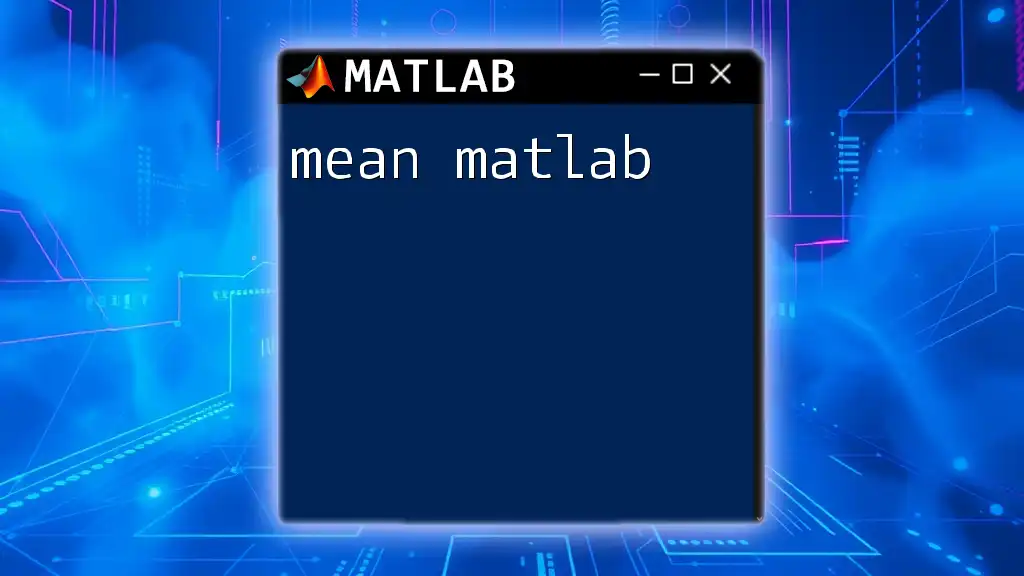
Working with `atan2` in MATLAB
Basic Example
Let’s start with a simple example. If you want to find the angle for the point (1, 1), you would write:
x = 1;
y = 1;
theta = atan2(y, x);
disp(theta); % Expecting π/4
This will output approximately 0.7854 radians or 45 degrees, indicating that the direction to the point (1, 1) is 45 degrees from the positive x-axis.
Advanced Examples
Example with Negative Values
For negative values, say you want to compute the angle for the point (-1, -1):
x = -1;
y = -1;
theta = atan2(y, x);
disp(theta); % Expecting -3π/4
This function will return approximately -2.3562 radians (or -135 degrees), reflecting that the point resides in the third quadrant.
Example with Complex Numbers
You can also utilize `atan2` with arrays of values. Consider an array of points:
x = [1, 0, -1];
y = [0, 1, 1];
theta = atan2(y, x);
disp(theta);
The output will provide an array of angles corresponding to each point. For example, (1, 0) yields 0, (0, 1) yields π/2, and (-1, 1) yields an angle that places it right in the second quadrant.
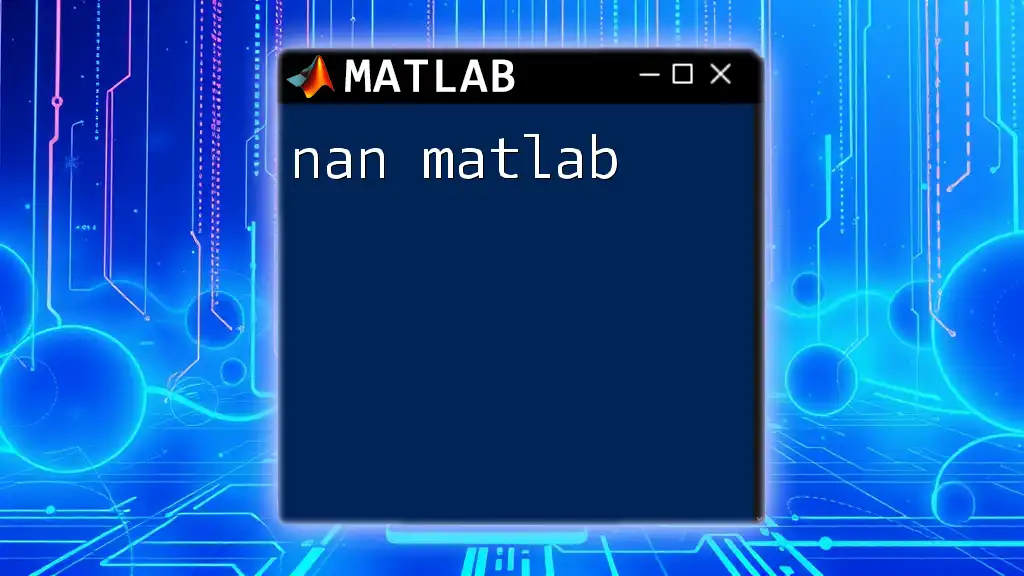
Practical Applications of `atan2`
Engineering Applications
In engineering, `atan2` is widely used for signal processing, particularly when analyzing phase relationships between signals. By converting signal vectors to polar coordinates, engineers can easily interpret phase differences and amplitudes, leading to more robust signal analysis.
Robotics and Navigation
In the field of robotics, determining the heading angle is crucial for path planning. The `atan2` function helps robots orient themselves by calculating the angle needed to navigate to a target location, maintaining accuracy in navigating through various terrains.
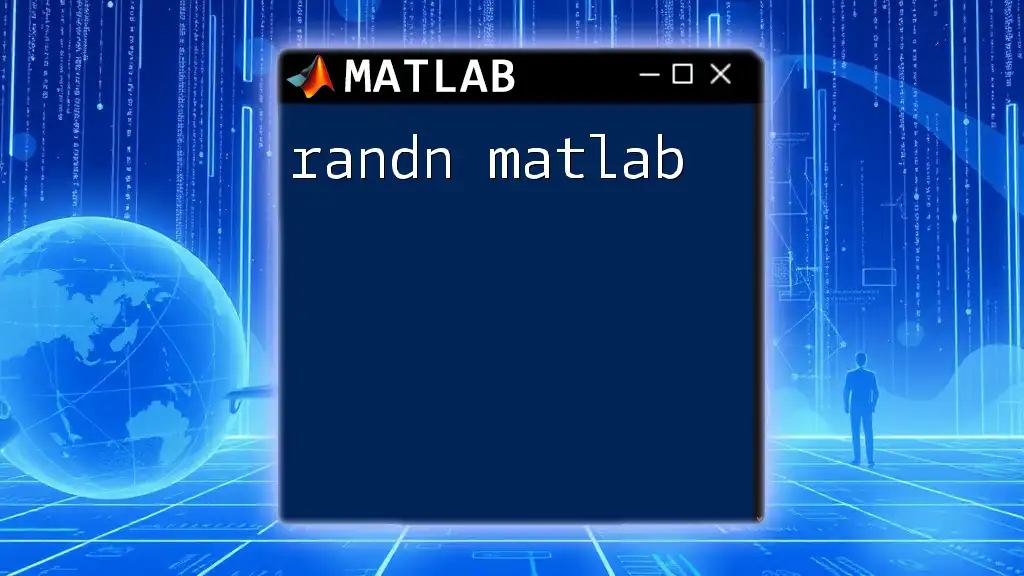
Common Pitfalls and Troubleshooting
Understanding Output Values
One common source of confusion arises from interpreting the output values of `atan2`. Remember that the output angle reflects the direction in the Cartesian plane and can be negative, indicating a clockwise rotation from the positive x-axis.
Error Handling
Ensure that you do not pass zero as both x and y. The inputs (0, 0) create an undefined scenario, resulting in an error. Always validate your inputs prior to calling `atan2`.
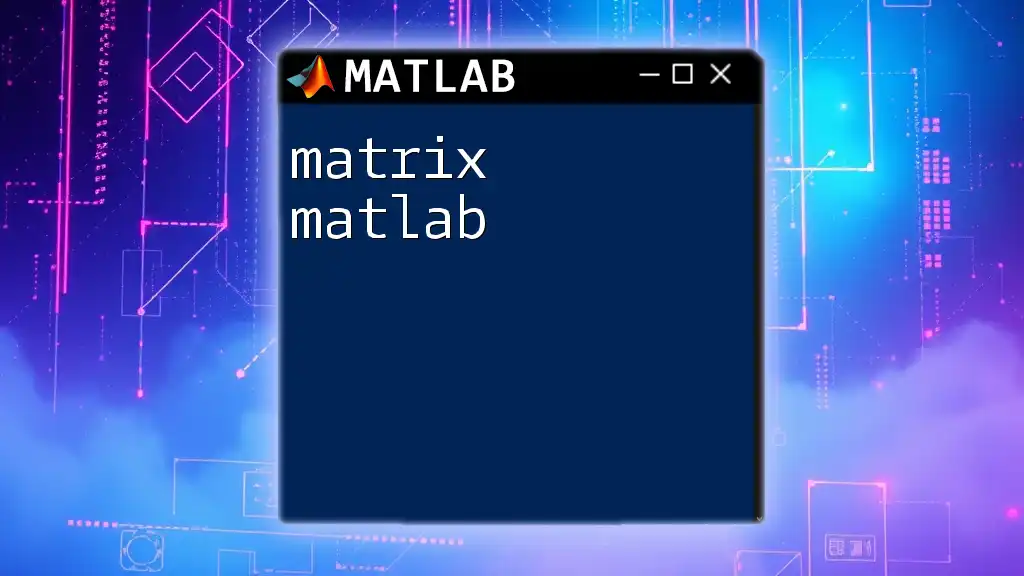
Visualization of `atan2` Outputs
Using Polar Coordinates
To help visualize angles generated by `atan2`, consider plotting values in a polar coordinate system:
theta = linspace(-pi, pi, 100);
r = ones(size(theta)); % radius
polarplot(theta, r);
This command generates a polar plot, showcasing the entire angular range defined by `atan2`.
Cartesian Plane Representation
To represent vectors visually in the Cartesian plane, you might use the following code snippet:
x = [1, -1, 0];
y = [1, -1, 1];
figure;
plot(x,y,'o');
hold on;
quiver(0, 0, x, y);
This will display points plotted on the Cartesian plane, with arrows showing the direction of each vector, allowing for an intuitive understanding of the angles involved.
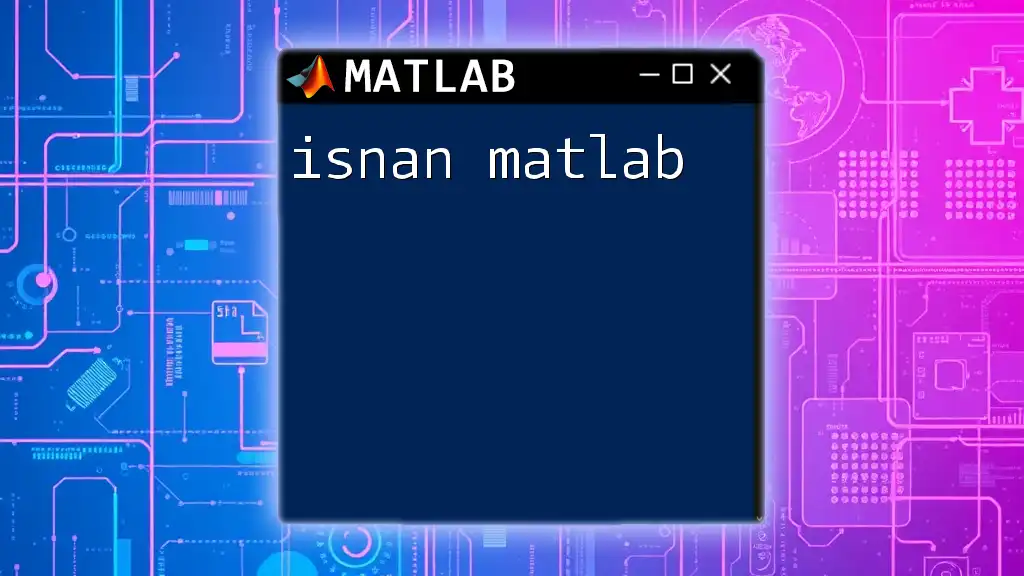
Conclusion
By mastering the `atan2` function in MATLAB, you gain a powerful tool for tackling problems related to angle calculation. The function's ability to handle inputs from all quadrants, along with its straightforward syntax, makes it an essential component of many mathematical, engineering, and navigational applications. Practice using `atan2` with various coordinates to build a solid understanding and leverage its capabilities for your projects.
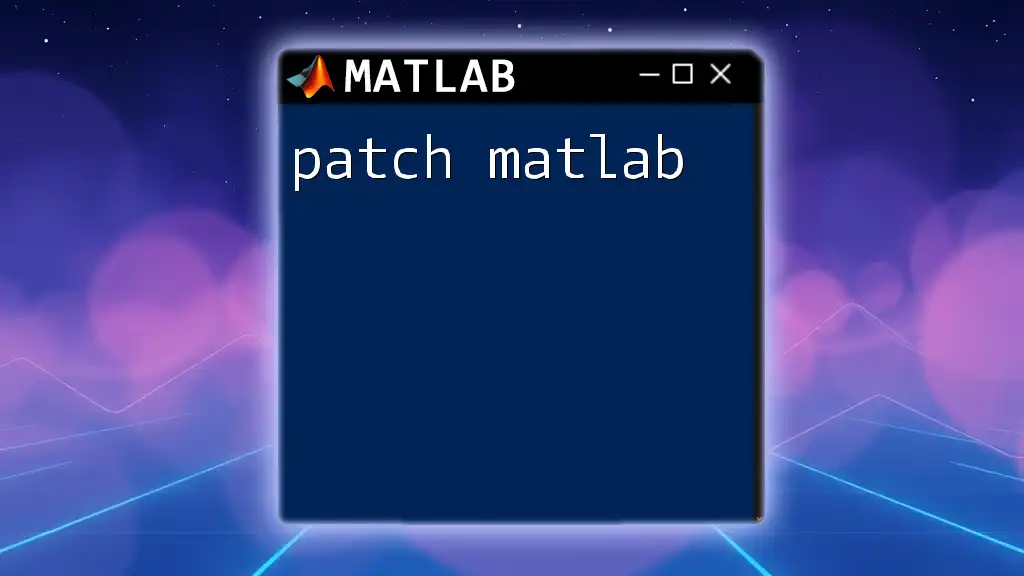
Further Readings and Resources
For more in-depth studies, consider checking MATLAB's official documentation, enrolling in online MATLAB courses, or engaging with community forums dedicated to MATLAB programming. Expanding your knowledge with these resources will further enhance your proficiency and application of the `atan2` function and MATLAB as a whole.