The `gauss2` function in MATLAB can be used to perform Gaussian elimination with partial pivoting to solve a system of linear equations efficiently.
Here’s a simple code snippet demonstrating how to implement Gaussian elimination in MATLAB:
function x = gauss2(A, b)
n = length(b);
% Forward elimination
for k = 1:n-1
% Partial pivoting
[~, idx] = max(abs(A(k:n, k)));
idx = idx + k - 1;
if idx ~= k
A([k, idx], :) = A([idx, k], :);
b([k, idx]) = b([idx, k]);
end
for i = k+1:n
m = A(i, k) / A(k, k);
A(i, k:n) = A(i, k:n) - m * A(k, k:n);
b(i) = b(i) - m * b(k);
end
end
% Back substitution
x = zeros(n, 1);
for i = n:-1:1
x(i) = (b(i) - A(i, i+1:n) * x(i+1:n)) / A(i, i);
end
end
This code defines a function `gauss2` to solve the system of equations \(Ax = b\) using Gaussian elimination.
Understanding Gaussian Functions
What is a Gaussian Function?
A Gaussian function is a significant mathematical function characterized by its symmetric bell-shaped curve. The general form of a Gaussian function can be expressed as:
\[ f(x) = A \cdot e^{-\frac{(x - \mu)^2}{2\sigma^2}} \]
Where:
- \(A\) represents the amplitude (the peak value).
- \(\mu\) is the mean (the center of the curve).
- \(\sigma\) is the standard deviation (controls the width of the curve).
The Gaussian function is notable for its application in various fields, including statistics, probability theory, and signal processing.
Applications of Gaussian Functions
Gaussian functions are used in a variety of applications:
- Statistics: In probability distributions, the normal distribution is a Gaussian function. It is critical for various statistical tests and analyses.
- Image Processing: Gaussian functions serve as smoothing filters to reduce noise in images, facilitating better edge detection and object recognition.
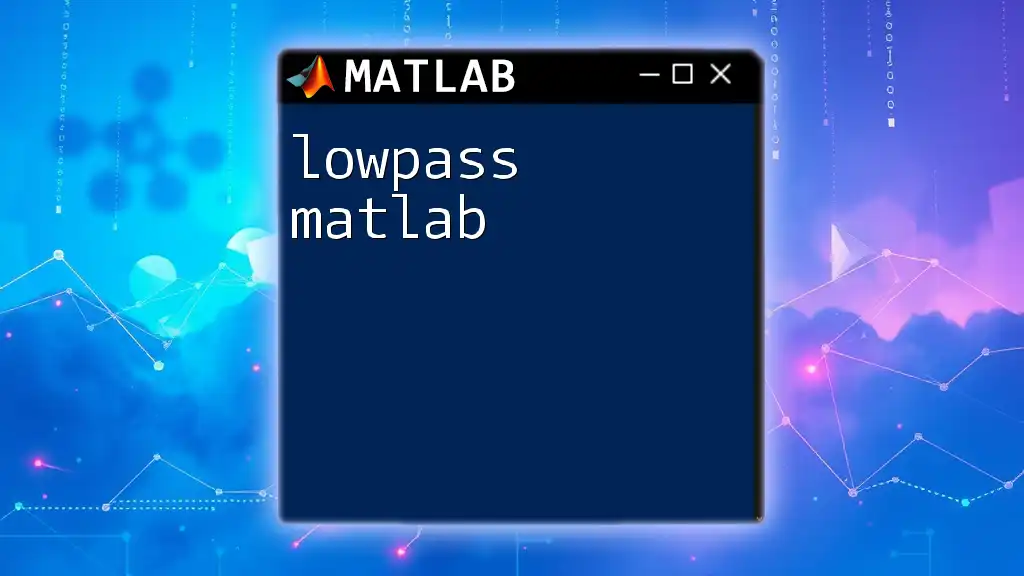
Getting Started with gauss2 in Matlab
Installing Matlab
To implement gauss2 in Matlab, you first need to ensure you have Matlab installed. You can download it from the MathWorks website. The installation is straightforward, but check that you have the necessary permissions and system requirements.
Workspace Setup for Using gauss2
Once installed, set up your Matlab workspace:
- Open Matlab and create a new script or function file.
- Ensure that you familiarize yourself with the interface, specifically the command window and the editor.
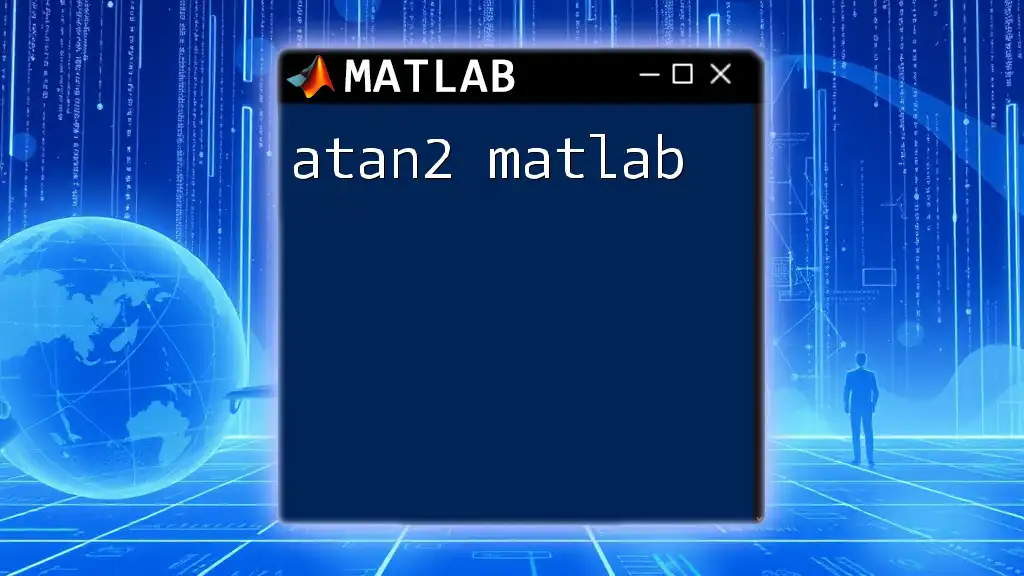
Syntax of gauss2 Function
Basic Syntax
The gauss2 function in Matlab is defined to create Gaussian-related outputs efficiently. The basic syntax is as follows:
y = gauss2(x, amplitude, mean, std_dev)
Where:
- x is the vector of input values for which you want to compute the Gaussian function.
- amplitude are the peak heights of the Gaussian curve.
- mean is the center of the distribution.
- std_dev is the standard deviation affecting the spread of the curve.
Optional Parameters
In addition to the basic parameters, gauss2 allows various optional parameters to customize the output:
- 'Option1', 'Option2', etc., where you can specify adjustments for particular needs. Each of these options typically alters the properties of the Gaussian function for more specialized situations.
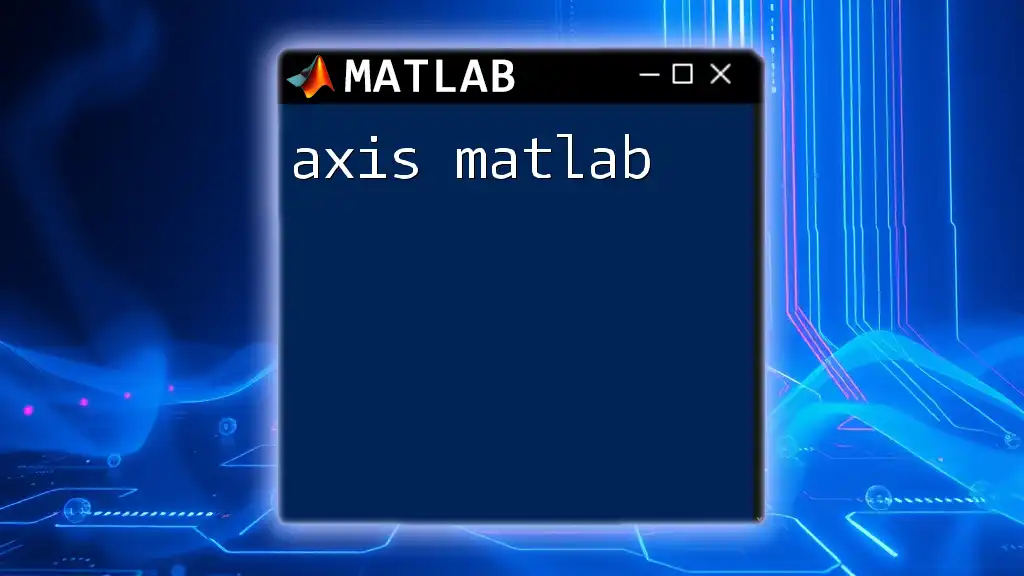
Implementing gauss2: Step-by-Step Example
Example Scenario
Consider a scenario where you want to model the height of a population. The mean height is 170 cm with a standard deviation of 10 cm. Using gauss2, we can visualize this distribution effectively.
Code Snippet 1: Basic Usage
To utilize the gauss2 function simply, you could implement the following code:
% Example of using gauss2 for a simple Gaussian curve
x = -5:0.1:5; % Creating an input range from -5 to 5
amplitude = 1; % Setting peak height
mean = 0; % Center of the Gaussian curve
std_dev = 1; % Spread of the curve
y = gauss2(x, amplitude, mean, std_dev);
plot(x, y);
title('Basic Gaussian Curve');
xlabel('X-axis');
ylabel('Y-axis');
In this code:
- We create an x vector ranging from -5 to 5.
- The function gauss2 computes the corresponding y values of the Gaussian function.
- A basic plot visualizes the output.
Code Snippet 2: Advanced Usage with Optional Parameters
You can enhance the functionality of gauss2 by utilizing optional parameters. Here’s an example:
% Example of using additional parameters in gauss2
y = gauss2(x, amplitude, mean, std_dev, 'Option1', value);
In this line, replace 'Option1' and value with actual parameter names and values applicable to your specific scenario. Adjusting these optional settings can yield variations in your output, adapting the Gaussian function to your dataset's requirements.
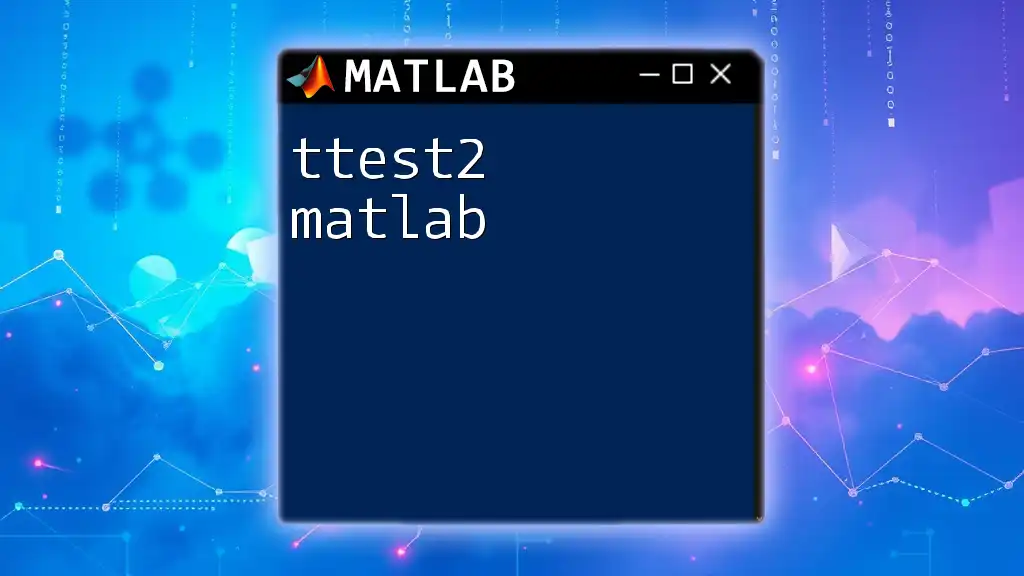
Visualizing the Output
Plotting Techniques in Matlab
Visualizing your data effectively is crucial for analysis. In Matlab, you can customize plots by adding grid lines, titles, axes labels, and legends to enhance readability.
Example of Enhanced Visuals
Here’s a way to create an enhanced visual representation of your Gaussian curve:
% Enhanced visualization for Gaussian curve
figure;
hold on;
grid on; % Adding a grid for better readability
plot(x, y, 'LineWidth', 2); % Plot with thicker lines
xlim([-6, 6]); % Set limits for x-axis
ylim([-0.1, 1.1]); % Set limits for y-axis
title('Enhanced Gaussian Curve Visualization');
xlabel('X-axis');
ylabel('Y-axis');
legend('Gaussian Curve'); % Adding a legend for clarity
This code creates a clear and effective visualization of the Gaussian function, making it easier to interpret and compare with other data.
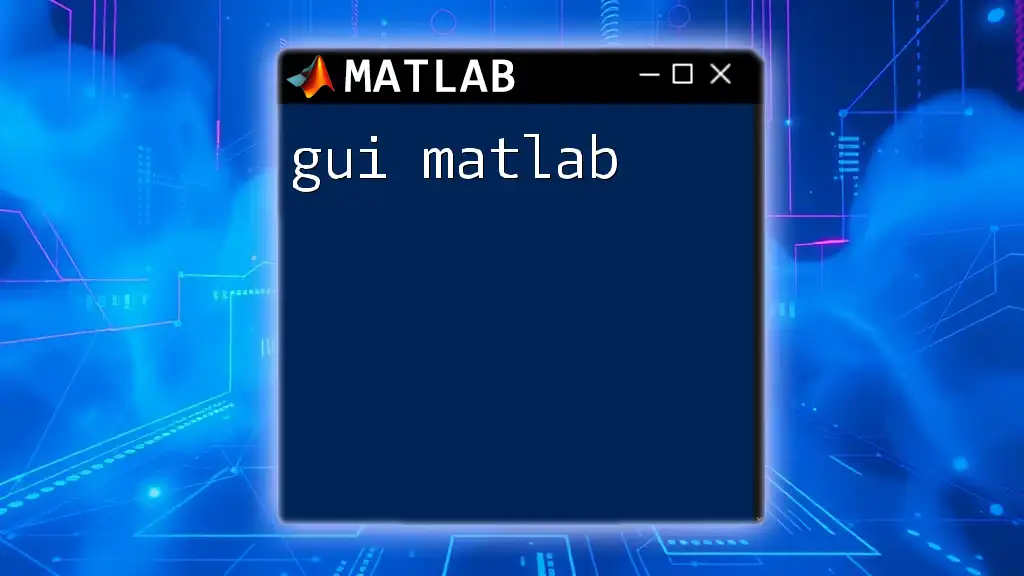
Troubleshooting Common Issues
Errors and Warnings
When using gauss2, you may encounter common errors, such as:
- Input size mismatch: Ensure your input vectors have the correct dimensions.
- Incorrect parameter values: Validate that all input and optional parameters are in suitable ranges.
Debugging Tips
To troubleshoot these issues, make effective use of Matlab's debugging tools:
- Use breakpoints within your script to pause execution and inspect variable values.
- Leverage the command window to test small pieces of code independently, allowing you to isolate problems.
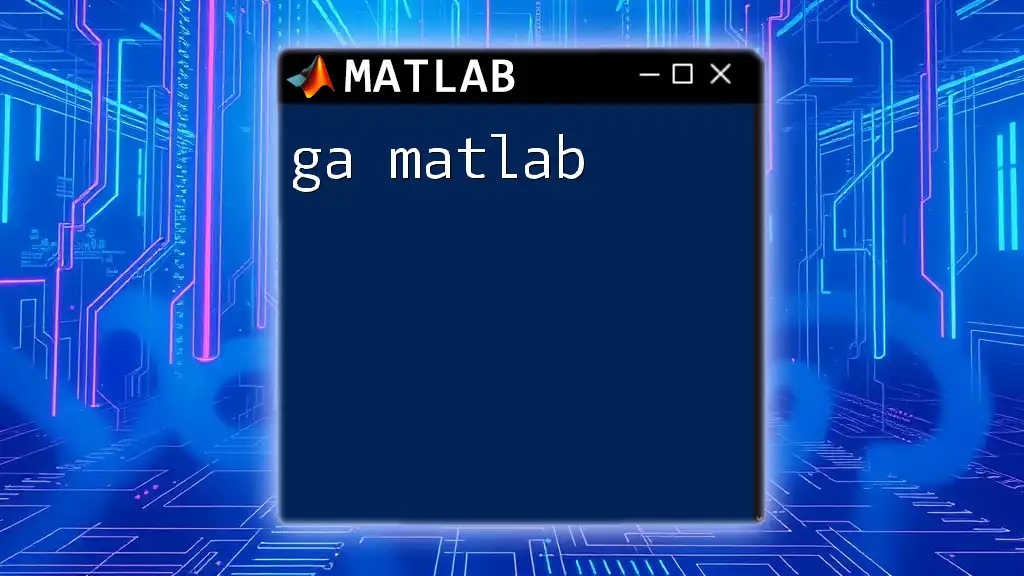
Advanced Concepts and Extensions
Customizing the gauss2 Function
With the knowledge of gauss2, you can create customized functions suited to your specific needs. For instance, writing your Gaussian function can provide additional flexibility.
Applications in Research and Industry
In research, Gaussian functions often model phenomena like stock price movements or analyze experimental results. They serve critical roles in various industries, such as data analysis, finance, and engineering, where accurate modeling is essential.
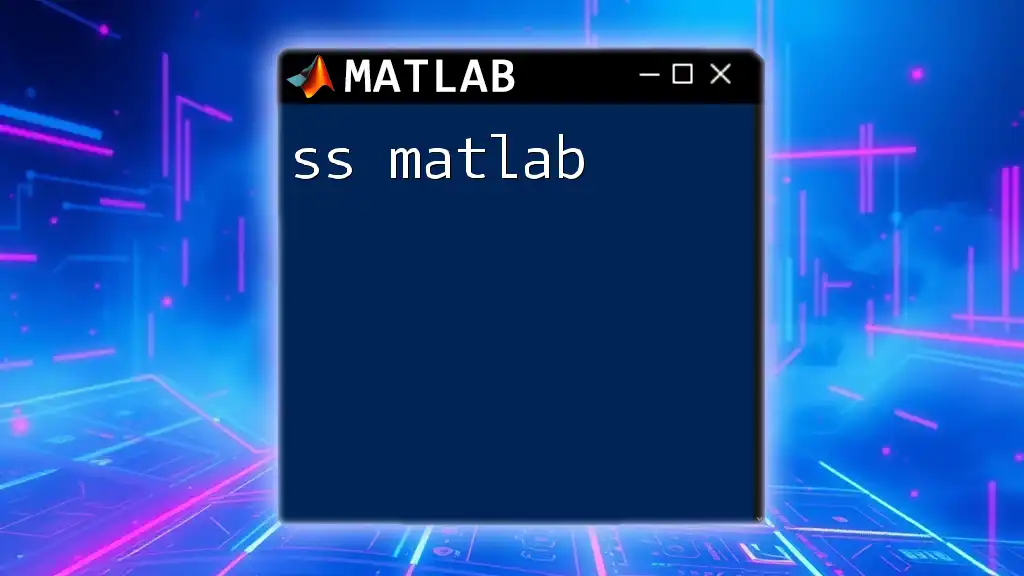
Conclusion
Mastering gauss2 matlab is vital for leveraging the full potential of Gaussian functions in your analysis endeavors. By understanding its syntax, practicing example scenarios, and learning to troubleshoot effectively, you're well on your way to utilizing this powerful function in practical applications.
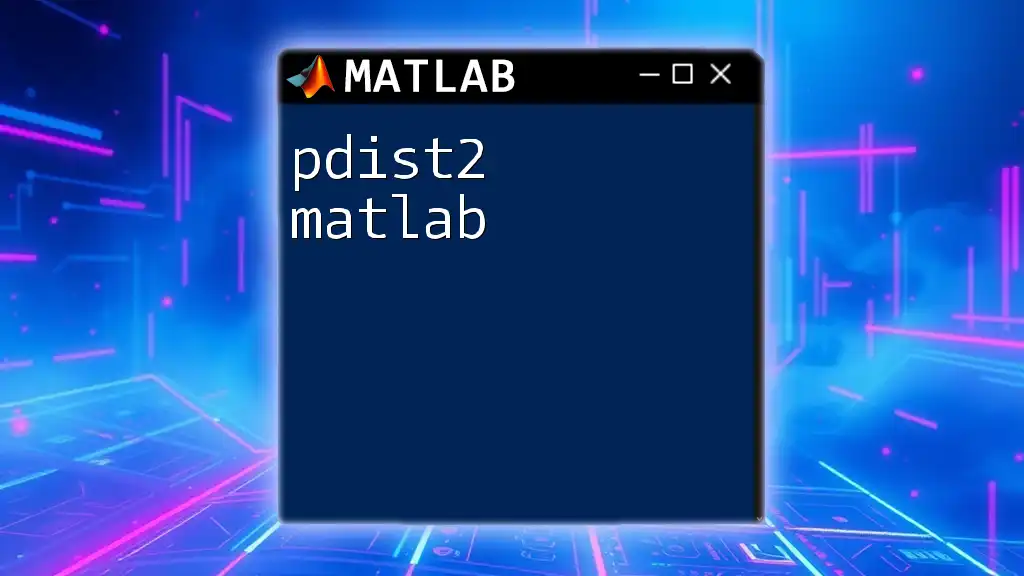
Call to Action
Explore further tutorials and resources on gauss2 and other Matlab functions to deepen your skills. Join our community or enroll in classes today to take your understanding of Matlab commands to the next level!