The `fft2` function in MATLAB computes the two-dimensional discrete Fourier transform of an input matrix, allowing for the analysis of signals in both the x and y dimensions.
Here's a code snippet that demonstrates how to use `fft2`:
% Example of using fft2 in MATLAB
A = rand(4, 4); % Create a 4x4 matrix of random numbers
B = fft2(A); % Compute the 2D Fourier transform of matrix A
What is `fft2`?
Definition of `fft2`
The `fft2` function in MATLAB is utilized to compute the two-dimensional Fast Fourier Transform (FFT) of a matrix. While `fft` performs the FFT on a one-dimensional array, `fft2` extends this functionality to two dimensions, making it indispensable for various applications, particularly in the realm of image processing and signal analysis.
Applications of `fft2`
`fft2` finds broad application across numerous fields:
- Image Analysis: In image processing, `fft2` helps analyze the frequency components of images, which can be instrumental for tasks like filtering and image compression.
- Physics and Engineering: Used in solving differential equations and modeling complex systems that require a frequency domain representation.
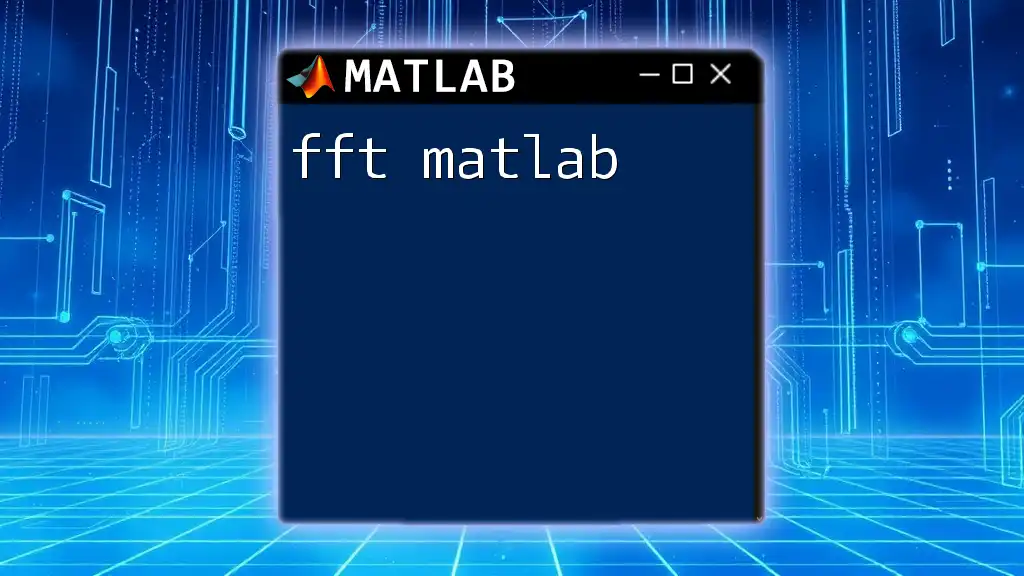
Syntax of `fft2`
Basic Syntax Overview
The basic syntax for using `fft2` in MATLAB is as follows:
Y = fft2(A)
Additionally, you can specify the dimensions of the output matrix with optional parameters:
Y = fft2(A, m, n)
Input Parameters
- A: This is the input matrix on which you wish to compute the 2D FFT.
- m: Specifies the number of rows in the output matrix.
- n: Specifies the number of columns in the output matrix.
Output
The output is a matrix `Y` of the same size as `A` (or the specified `m` and `n` if used). The elements of `Y` are complex numbers that represent frequency data derived from the input matrix.
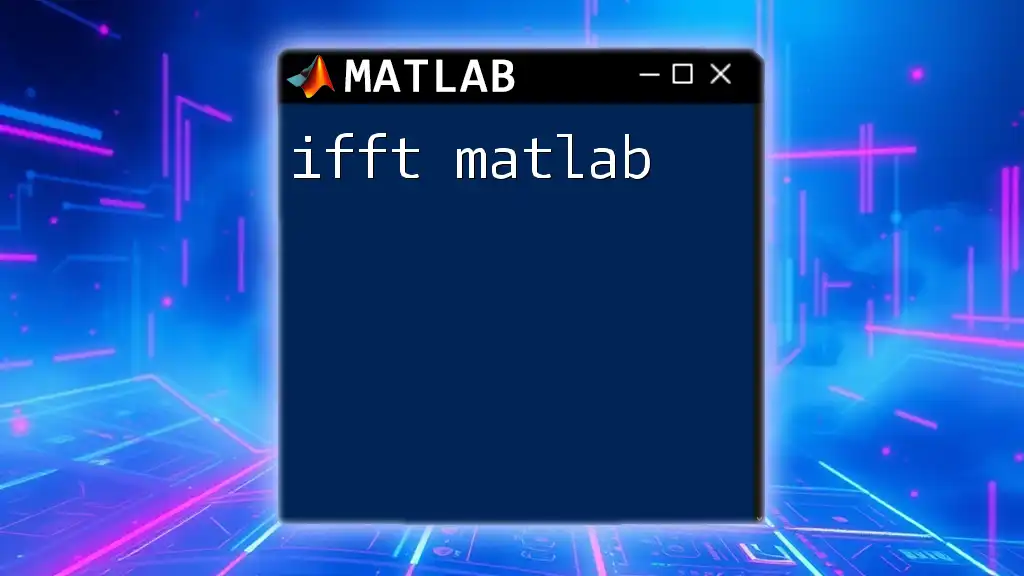
Working with `fft2`
Preparing Data
Before applying the `fft2` function, it's essential to prepare your data. For instance, here’s how you can generate a simple 2x2 sample matrix:
A = [1 2; 3 4];
Performing the 2D FFT
Now you can run the FFT on the prepared matrix:
Y = fft2(A);
disp(Y);
This command will calculate the two-dimensional FFT of matrix `A` and display the output.
Visualizing the Result
Interpreting the results of `fft2` can be taken a step further by visualizing the magnitude of the output. You can plot the magnitude as follows:
imshow(abs(Y), []);
title('Magnitude of FFT');
Here, `abs(Y)` calculates the magnitude of the complex numbers in the output matrix `Y`.
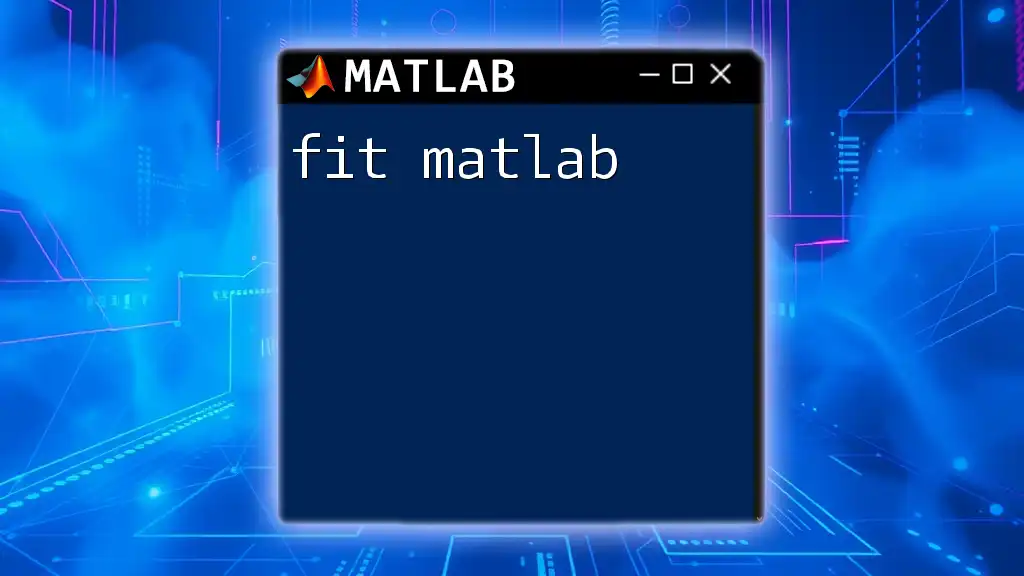
Understanding the Output of `fft2`
Magnitude and Phase
The output matrix produced by `fft2` consists of both magnitude and phase information:
- Magnitude can be calculated as `abs(Y)`, which provides insight into the strength of various frequency components.
- Phase is computed using `angle(Y)`, revealing information about the phase shifts in the signal.
Interpreting the Output
Analyzing the FFT output is paramount for understanding the frequency components. For example, if you applied `fft2` to an image, the output `Y` would reveal which frequency patterns dominate the image's pixel intensities.
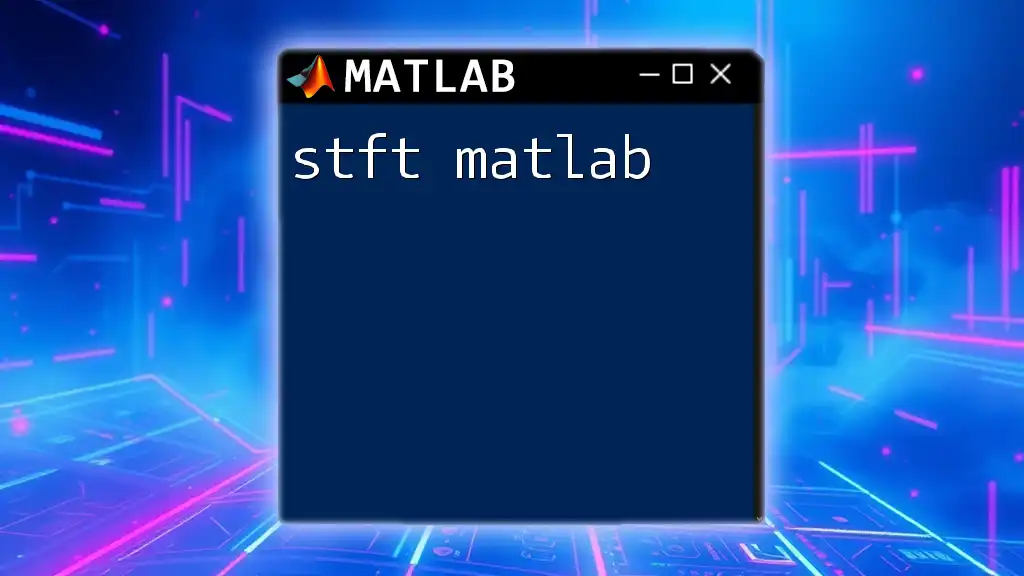
Advanced Usage of `fft2`
Using the Dimensions Arguments
The optional dimensions arguments `m` and `n` allow for tailored output sizes. For instance, if you want to pad the input matrix to a 4x4 zero-padded size, you can do so with:
Y = fft2(A, 4, 4); % Pad with zeros to 4x4
This can be advantageous in various scenarios, especially when performing operations that require matrices of uniform size.
Processing Images with `fft2`
If you want to apply `fft2` to a grayscale image, the typical workflow would be:
img = imread('image.png');
grayImg = rgb2gray(img);
Y = fft2(grayImg);
This conversion simplifies the complexity by reducing color channels and allows for effective frequency analysis.
Post-Processing the Output
After computing the FFT, you may want to filter certain frequencies. Techniques often involve masking high-frequency components and subsequently applying the inverse FFT with `ifft2` to recover the processed image or signal data.
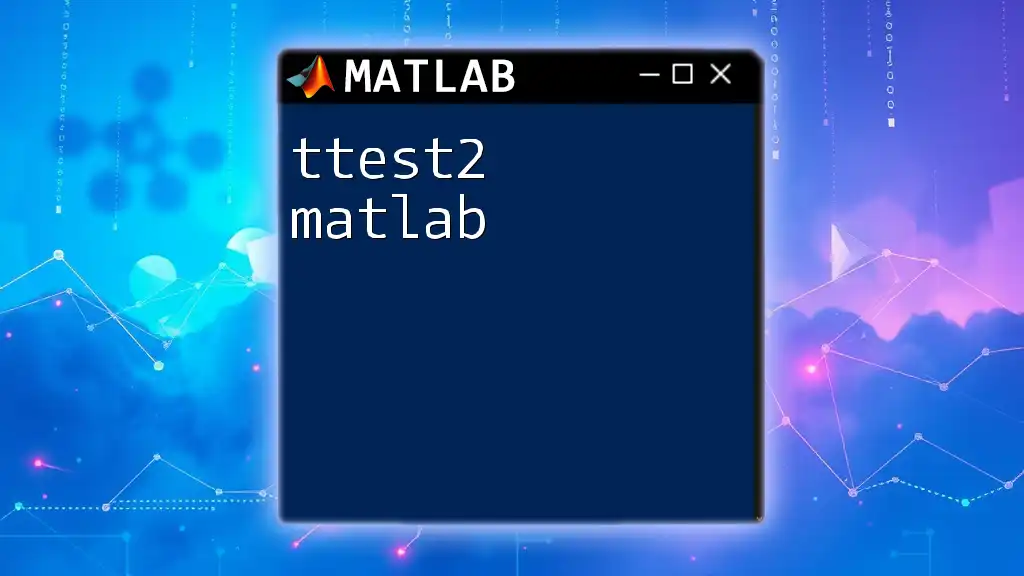
Common Errors and Debugging
Common Mistakes to Avoid
When using `fft2`, be cautious of common pitfalls:
- Input Data Types: Ensure that your matrix is properly formatted; using characters or strings will lead to errors.
- Matrix Dimensions: Be aware of the dimensions of your input matrix, especially if you are expecting a specific output shape.
Debugging Tips
To troubleshoot, you can utilize MATLAB functions like `size()` and `class()` to inspect the properties of your matrices:
disp(size(A)); % Check the dimensions of A
disp(class(A)); % Check the data type of A
These checks help ensure that your input is suitable for the `fft2` function.
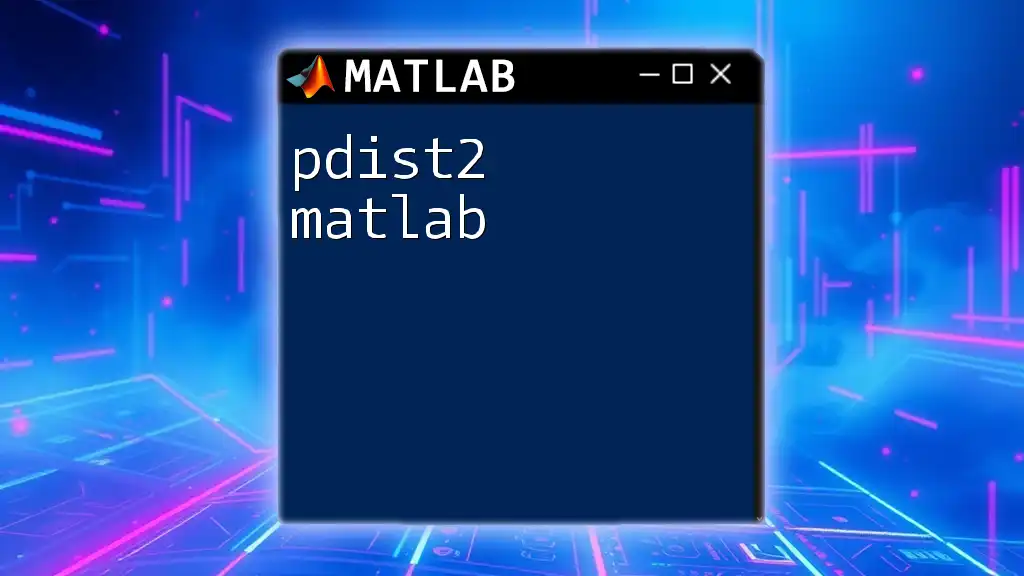
Conclusion
The `fft2` function in MATLAB serves as a crucial tool for conducting two-dimensional frequency analysis. Its versatility extends beyond the realm of image processing, solidifying its value across various disciplines. By practicing the concepts and experimenting with different datasets, you can unlock the full potential of `fft2`.
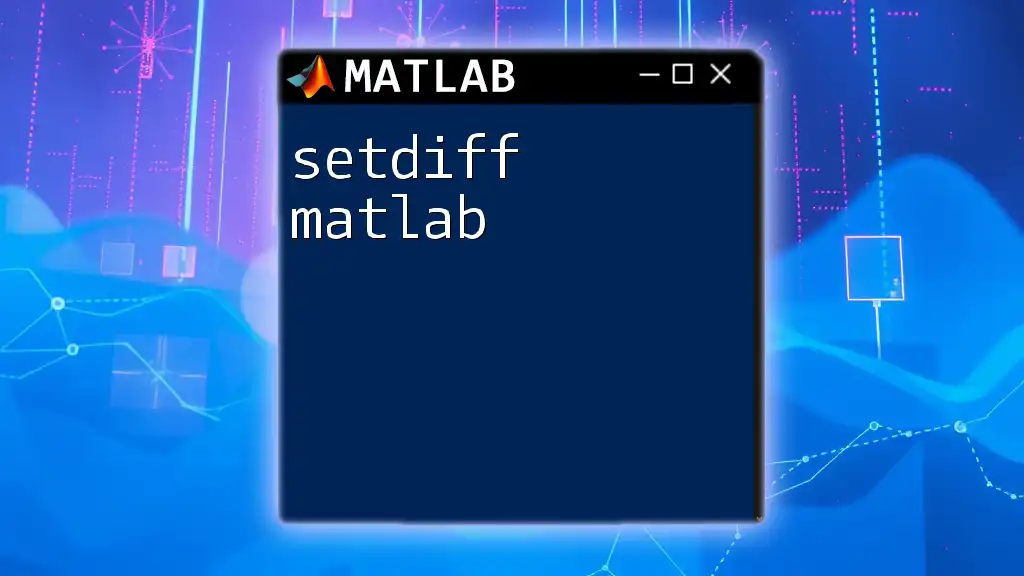
Further Resources
For more in-depth learning, consider exploring the MATLAB documentation, which provides comprehensive details on `fft2` and related functions. Additionally, several books and online courses focused on signal processing techniques may enhance your understanding and skills in this area.
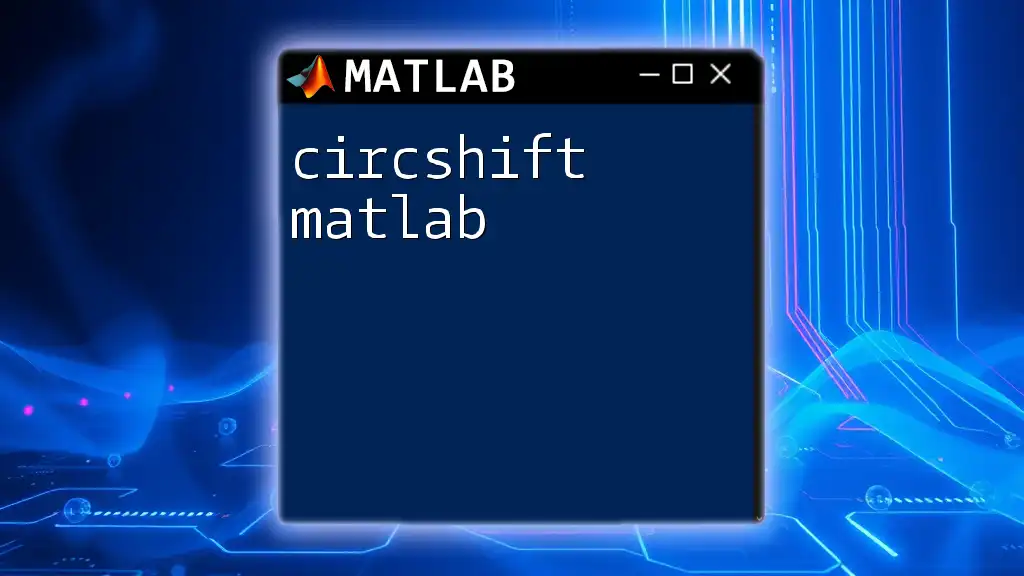
FAQs
Frequently Asked Questions about `fft2`
-
What are the computational complexities?
The computational complexity of `fft2` is generally O(N log N), where N is the number of elements in the input matrix. -
Can `fft2` be applied to non-image data?
Absolutely! Though commonly used for images, `fft2` can analyze any 2D data, making it a powerful tool for various applications.