The `bsxfun` function in MATLAB applies a specified element-wise operation to arrays with singleton dimensions, allowing for more flexible and efficient computation without the need for explicit array expansion.
Here's a code snippet that demonstrates its usage:
A = [1; 2; 3]; % Column vector
B = [4, 5, 6]; % Row vector
C = bsxfun(@plus, A, B); % Add A and B using bsxfun
% C will be:
% 5 6 7
% 6 7 8
% 7 8 9
Understanding `bsxfun`
What is `bsxfun`?
`bsxfun` is a powerful function in MATLAB that stands for "Binary Singleton Expansion Function." Its primary purpose is to apply element-wise operations to arrays of different sizes in a memory-efficient manner. By automatically handling singleton dimensions to facilitate operations without needing explicit replication of data, `bsxfun` ensures that your code remains readable and efficient.
Syntax of `bsxfun`
The basic syntax of `bsxfun` looks like this:
C = bsxfun(fun, A, B)
Here:
- `fun` is a handle to the function you wish to apply (e.g., `@plus`, `@minus`, etc.).
- `A` and `B` are the input arrays you want to operate on.
- `C` is the output array resulting from the operation.
This function intelligently expands dimensions of the inputs as necessary, allowing for flexible calculations without manual resizing of the arrays.
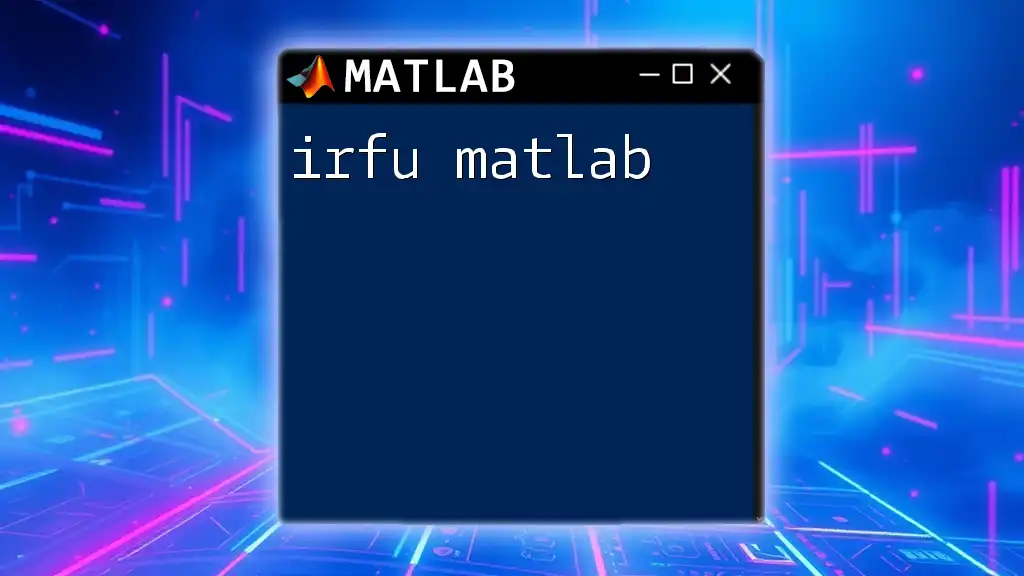
Why Use `bsxfun`?
Benefits of Using `bsxfun`
Using `bsxfun` can significantly enhance the performance of your MATLAB code. Here are some of the advantages:
- Performance advantages: `bsxfun` is optimized for speed, often outperforming traditional loops.
- Reduction of memory overhead: It avoids unnecessary memory allocation by not replicating matrices explicitly.
- Cleaner, more readable code: The syntax is direct and expressive, making the intention of the code clearer.
Comparison with Traditional Approaches
Consider a scenario where you want to add a vector to each row of a matrix. If you were to do this using loops, it would look something like this:
A = [1; 2; 3]; % Column vector
B = [10, 20, 30]; % Row vector
C = zeros(3, 3);
for i = 1:3
C(i, :) = A(i) + B;
end
In contrast, `bsxfun` simplifies this process:
C = bsxfun(@plus, A, B);
This single line achieves the same outcome, highlighting the efficiency and elegance of `bsxfun`.
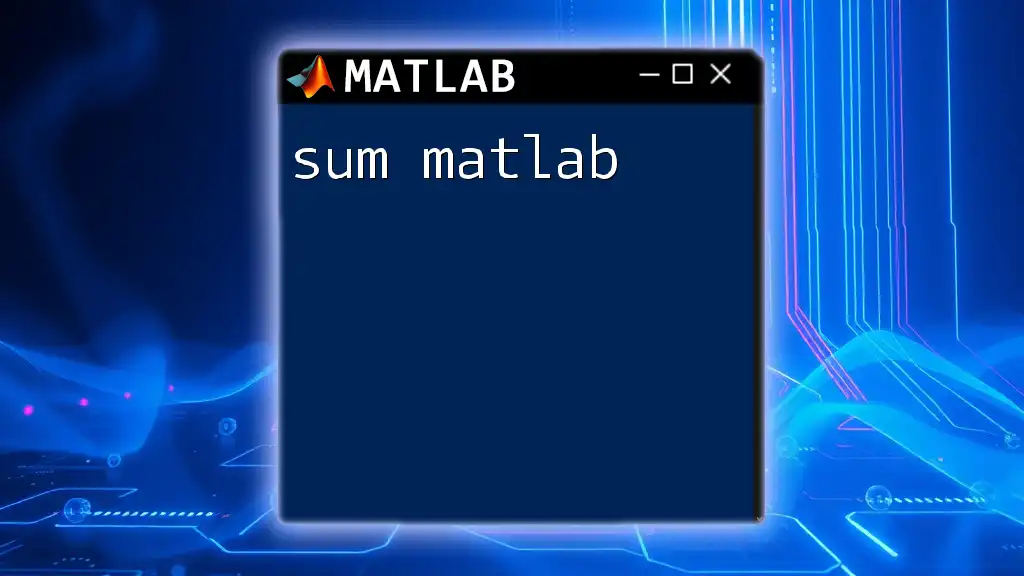
Common Use Cases for `bsxfun`
Mathematical Operations
`bsxfun` shines in performing various element-wise operations without additional overhead. For instance, consider element-wise addition:
A = [1; 2; 3];
B = [10, 20, 30];
C = bsxfun(@plus, A, B);
disp(C); % Output: [11, 21, 31; 12, 22, 32; 13, 23, 33]
In this example, each element of the column vector `A` is added to every element of the row vector `B`, producing a coherent matrix output.
Statistical Applications
`bsxfun` is also particularly useful for statistical operations. For instance, if you wish to subtract the mean from each column of a dataset:
data = rand(5, 3); % 5x3 matrix of random numbers
mean_data = bsxfun(@minus, data, mean(data, 1));
disp(mean_data);
This operation centers the data by removing the mean from each column, a common preprocessing step in many statistical analyses.
Logical Operations
You can also leverage `bsxfun` for logical comparisons, creating conditional statements with ease. For example, if you want to create a logical array based on a threshold:
threshold = 0.5;
logical_array = bsxfun(@gt, data, threshold);
disp(logical_array);
Here, `bsxfun` evaluates whether each element in the `data` matrix exceeds the `threshold` value, returning a logical array.
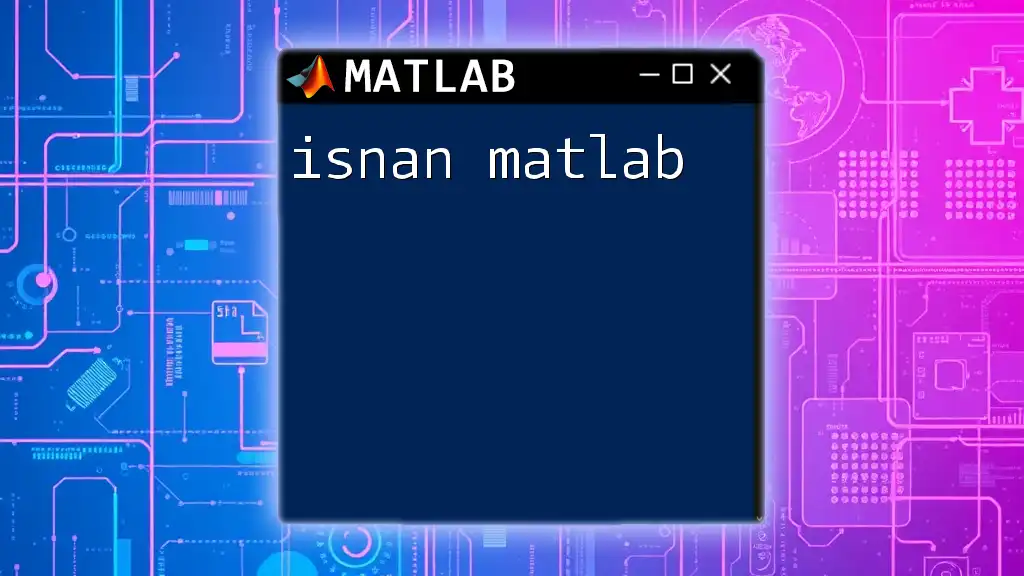
Tips and Best Practices
Efficient Use of `bsxfun`
To maximize performance and clarity in your code:
- Prefer `bsxfun` for large datasets: Use it when working with arrays of different sizes to enhance speed.
- Keep it readable: The intention behind using `bsxfun` should be clear to someone reviewing your code. Encountering it in context should immediately convey its purpose.
Transitioning to Implicit Expansion
Starting with MATLAB R2016b, implicit expansion has been introduced, allowing arrays to automatically expand in many situations. Here's a comparison of using `bsxfun` versus implicit expansion:
Using `bsxfun`:
C_bsxfun = bsxfun(@plus, A, B);
Using implicit expansion:
C_implicit = A + B;
While `bsxfun` is still useful, embrace implicit expansion when available for cleaner and more straightforward code.
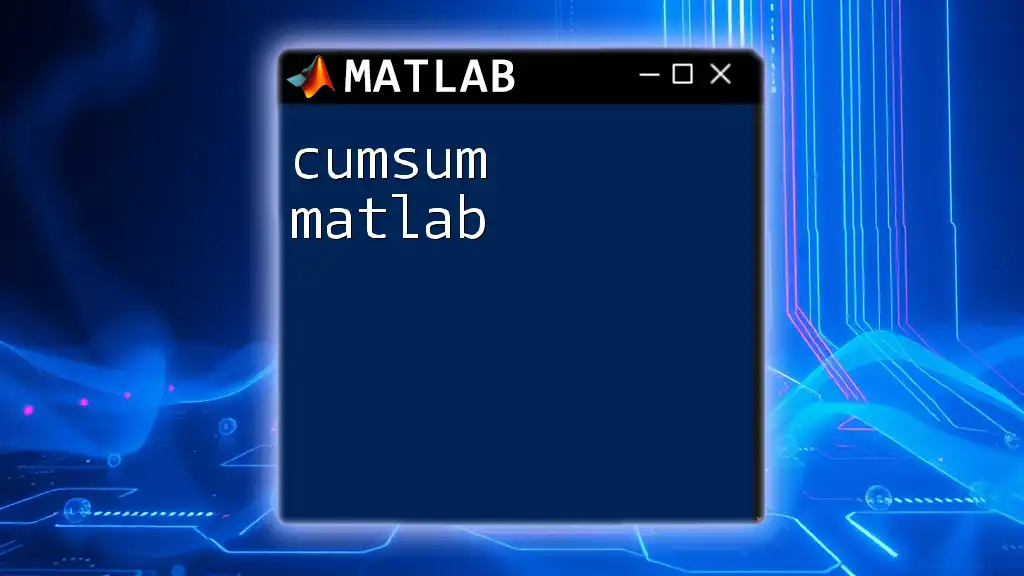
Common Pitfalls to Avoid
Mistakes to Watch Out For
Even seasoned MATLAB users can stumble upon issues with `bsxfun`. Here are some common pitfalls:
- Size mismatches: Ensure the arrays you’re working with are compatible for the operations you intend to perform. MATLAB will throw size mismatch errors when the dimensions aren’t compatible.
- Function compatibility: Not all functions are amenable to `bsxfun`. Check that the function you choose can accept scalar and non-scalar arguments as required.
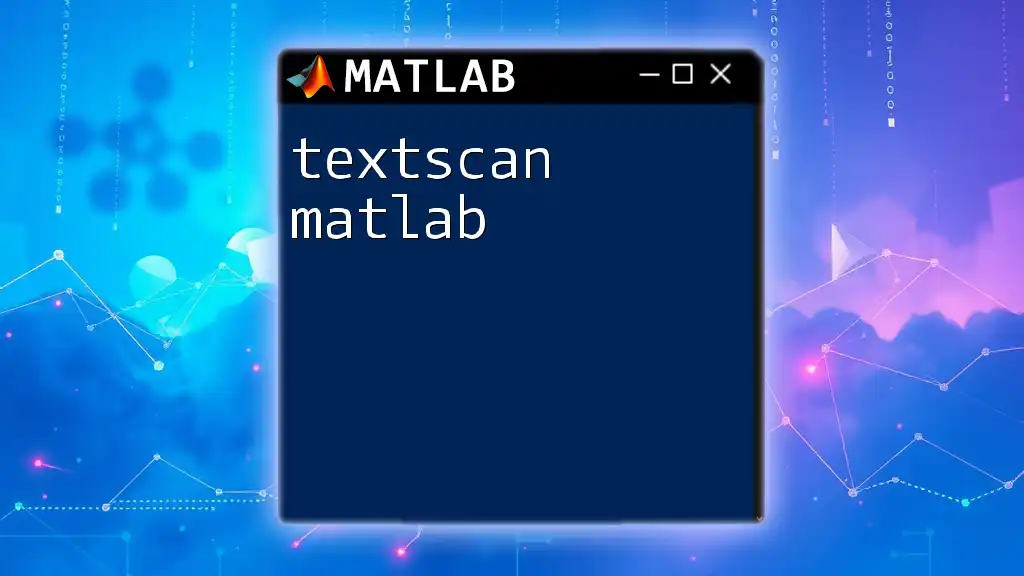
Conclusion
In summary, `bsxfun` is a versatile and powerful tool in MATLAB that allows for efficient and clean operations on arrays of different sizes. By leveraging the benefits of `bsxfun`, you can write faster, more maintainable code and streamline your data processing tasks.
As you continue your MATLAB journey, consider incorporating `bsxfun` into your coding practices. Its advantages in terms of performance and readability are too significant to overlook.
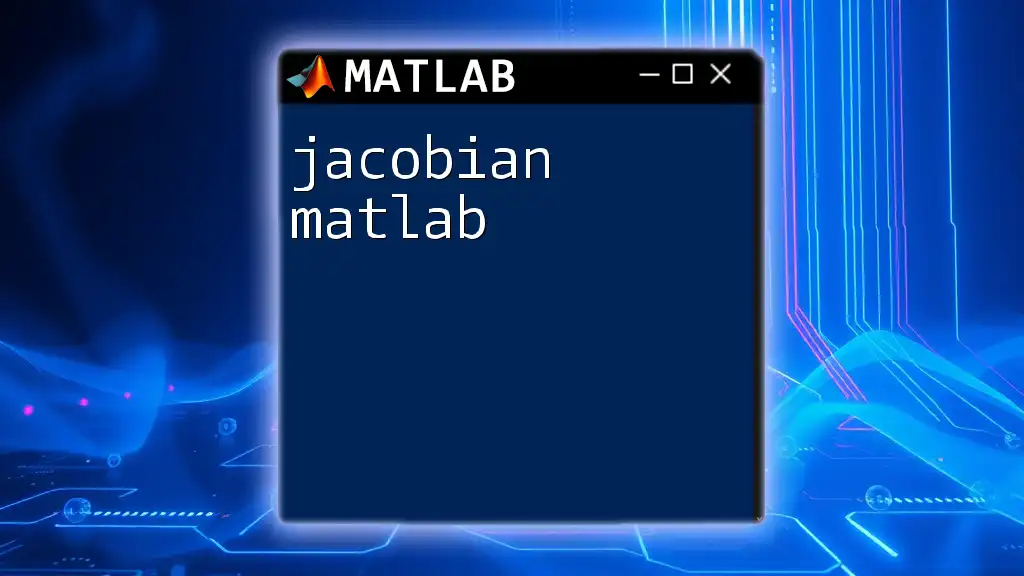
Additional Resources
To further explore `bsxfun`, check out the official MATLAB documentation and look for tutorials that dive deeper into matrix operations. Community forums and discussion boards can also offer valuable insights and examples shared by fellow MATLAB users.
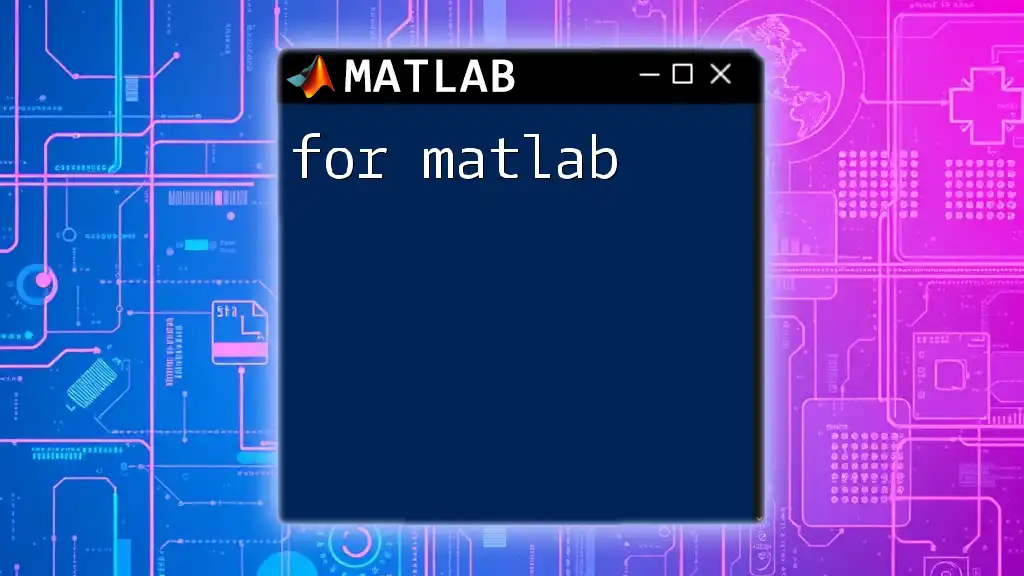
FAQs About `bsxfun`
-
What types of data can be processed with `bsxfun`?
`bsxfun` can process numeric arrays, logical arrays, and any other data types that support element-wise operations.
-
Is `bsxfun` deprecated in recent MATLAB versions?
While `bsxfun` is still available, MATLAB has introduced implicit expansion as an easier alternative, but it is not officially deprecated.
-
How does `bsxfun` compare to other vectorized functions?
`bsxfun` specifically addresses cases involving arrays of differing sizes, making it highly suitable for many applications. It's particularly beneficial when explicit replication of data would be inefficient.