`fsolve` is a MATLAB function used to solve systems of nonlinear equations, allowing users to find the roots of a set of equations defined within a function.
Here’s a simple example of how to use `fsolve`:
% Define the system of equations
function F = myEquations(x)
F(1) = x(1)^2 + x(2)^2 - 4; % Example equation: x1^2 + x2^2 = 4
F(2) = x(1) - x(2); % Example equation: x1 - x2 = 0
end
% Initial guess
initialGuess = [1, 1];
% Solve the system of equations
solution = fsolve(@myEquations, initialGuess);
disp('Solution:');
disp(solution);
Understanding Nonlinear Equations
What are Nonlinear Equations?
Nonlinear equations are mathematical expressions where the variable(s) appear in a nonlinear form, such as squares, cubes, or other nonlinear operations. Unlike linear equations, where the relationship between variables is constant, nonlinear equations can represent a wide range of phenomena in fields like physics, engineering, and economics.
For example, a common nonlinear equation is the quadratic equation:
\[ ax^2 + bx + c = 0 \]
This equation can have zero, one, or two solutions depending on the values of \(a\), \(b\), and \(c\).
Why Use fsolve?
fsolve in MATLAB is a powerful tool for finding numerical solutions to systems of nonlinear equations. The advantages of using fsolve include its ability to handle complex problems that may not have analytical solutions and its capability to deal with multiple equations simultaneously. Compared to other MATLAB functions like `fzero` (which is used for finding roots of single equations), fsolve is specifically designed for systems of equations, making it a preferred choice for many applications.
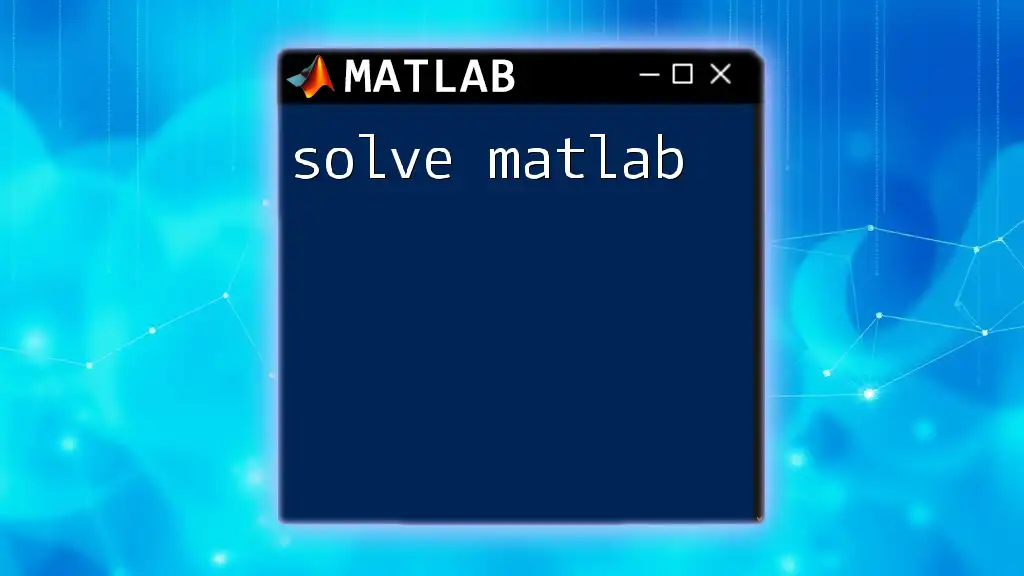
Getting Started with fsolve
Installation and Setup
To begin using fsolve, ensure you have MATLAB installed with the Optimization Toolbox, as this toolbox includes the fsolve function. Most standard MATLAB installations come with this toolbox, but it's always beneficial to check your version if you encounter any issues.
Basic Syntax of fsolve
The basic syntax of fsolve is straightforward:
x = fsolve(fun, x0)
Here:
- fun: This is the function handle that defines the system of equations to solve. It can be a single equation or a set of equations.
- x0: Your initial guess for the solution. It’s crucial to choose a good initial guess, as this can affect the convergence and the final solution.
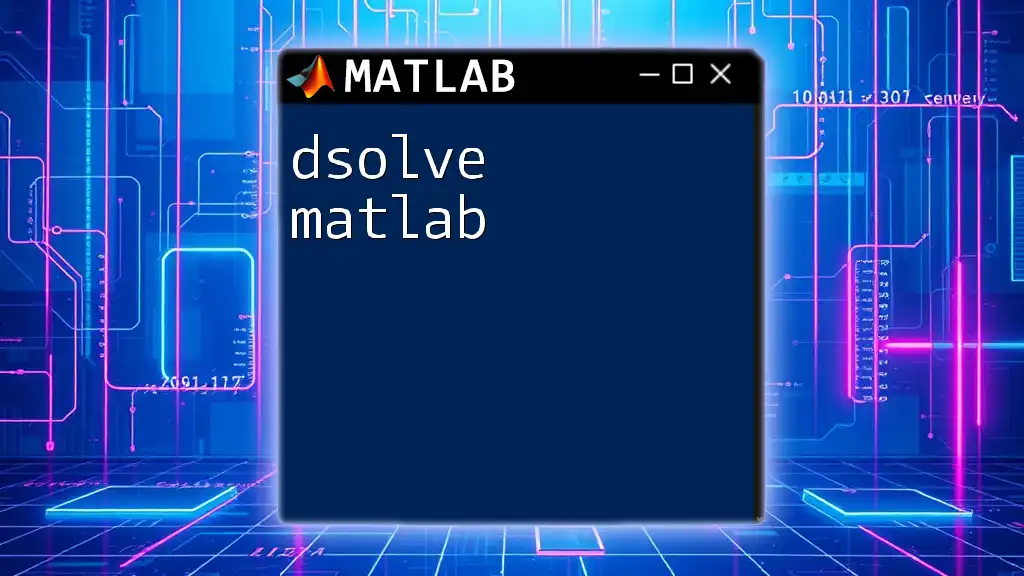
Defining Functions for fsolve
Creating Function Handles
Function handles are a way to call functions in MATLAB without explicitly having to write out the full function each time. To create a function handle for a simple nonlinear equation, you can use the `@` symbol. For example, to represent the equation \(x^2 - 4 = 0\), you would write:
fun = @(x) x.^2 - 4; % Example function
Writing System of Equations
When working with multiple equations, it’s useful to define them in a function. For instance, if we have the following system:
\[ \begin{align*} x_1^2 + x_2^2 &= 10 \\ x_1 - x_2 + 1 &= 0 \end{align*} \]
We can express this in MATLAB by creating a function:
function F = mySystem(x)
F(1) = x(1)^2 + x(2)^2 - 10; % First equation
F(2) = x(1) - x(2) + 1; % Second equation
end
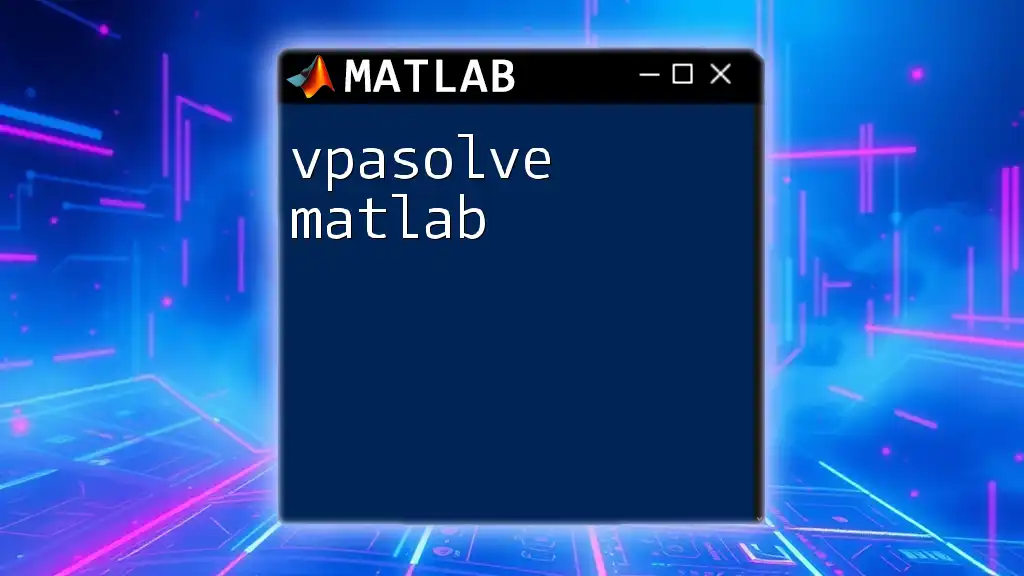
Using fsolve for Solving Equations
Step-by-Step Example
Example: Solving a Single Equation
Let’s break down an example of solving a simple nonlinear equation using fsolve. Assume we want to solve \(x^2 - 4 = 0\). Our approach will involve defining the equation, choosing an initial guess, and invoking fsolve.
Define the function first:
fun = @(x) x.^2 - 4; % The equation
Choose an initial guess (let's start with `1`):
x0 = 1; % Initial guess
Now, call fsolve to find the solution:
sol = fsolve(fun, x0);
disp(['The solution is: ', num2str(sol)]);
When you run this code, MATLAB will output the solution, which should ideally be close to `2` or `-2`, depending on the behavior of the function.
Example: Solving a System of Nonlinear Equations
Now, let’s apply fsolve to our system of equations defined earlier. We assume an initial guess for \(x_1\) and \(x_2\):
x0 = [1, 1]; % Initial guess
Next, we'll apply fsolve:
options = optimoptions('fsolve', 'Display', 'iter'); % Options for fsolve
sol = fsolve(@mySystem, x0, options);
disp(['The solution is: ', num2str(sol)]);
Analyzing the Results
Interpreting Output from fsolve
The output of fsolve includes the solution vector and information about the convergence. Typically, `sol` gives you the solution values for \(x_1\) and \(x_2\). The `exitflag` helps to determine if the solution converged successfully, while `output` provides details on the number of iterations and the algorithm used.
Common Issues and Solutions
When using fsolve, one might encounter issues like failure to converge. To troubleshoot:
- Refine your initial guess if the solution seems incorrect.
- Check the function definitions for errors, ensuring continuity and differentiability.
- Use different options with `optimoptions` to adjust tolerance levels or set the maximum number of iterations.
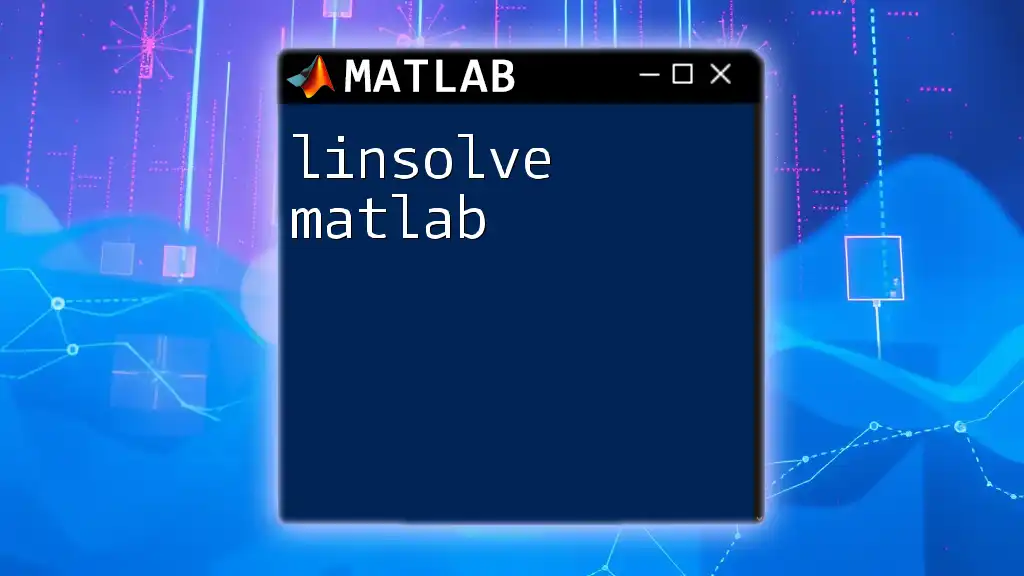
Advanced Features of fsolve
Setting Options
The `optimoptions` function allows you to customize your fsolve call further. Options can significantly affect performance and accuracy. For example:
options = optimoptions('fsolve', 'TolFun', 1e-6, 'MaxIter', 400, 'Display', 'final');
This sets the function to stop when the function value is less than `1e-6` and allows up to `400` iterations while displaying information at the end of the process.
Parallel Computing with fsolve
MATLAB also allows the use of parallel computing capabilities. If you have the Parallel Computing Toolbox, you can speed up your calculations. You can specify this in the options:
options = optimoptions('fsolve', 'UseParallel', true);
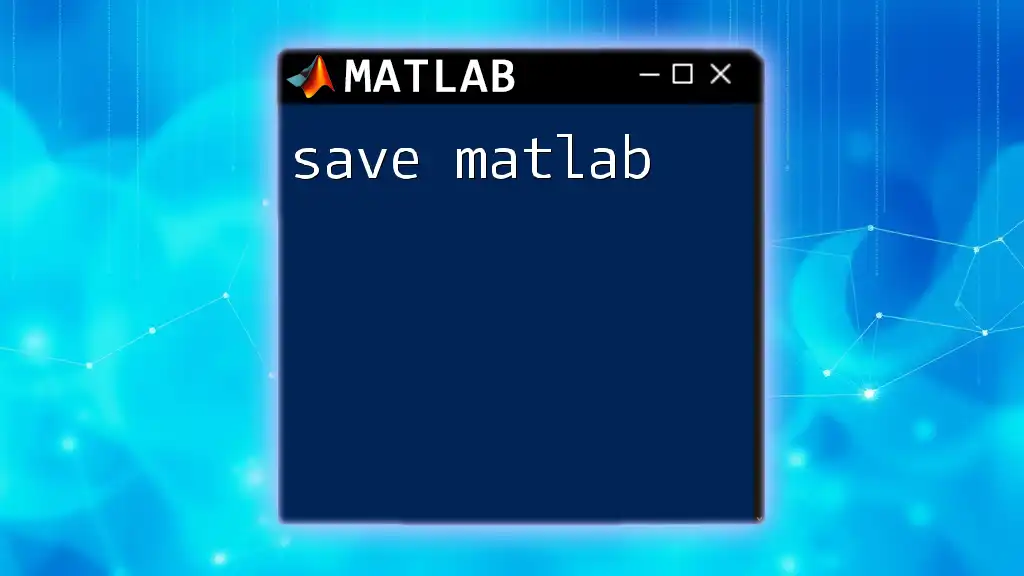
Applications of fsolve
Real-World Applications
fsolve has widespread applications across various fields:
- Engineering: For solving structural equilibrium equations.
- Finance: To find equilibrium prices in complex models.
- Physics: For solving equilibrium equations in various physical scenarios.
One hypothetical scenario might include calculating the intersection points of multiple curves in an optimization problem, such as maximizing profit in business, which could involve multiple nonlinear relationships.
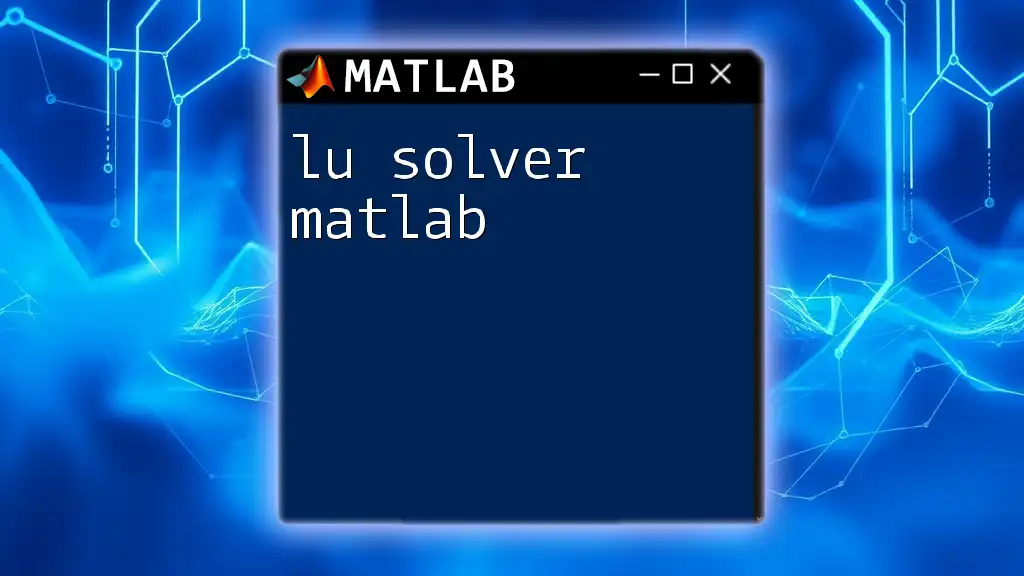
Conclusion
In summary, fsolve is an incredibly versatile function in MATLAB for finding numerical solutions to nonlinear equations. Its ability to work with both singular and system equations makes it a valuable tool for professionals in many technical fields. Regular practice with various problems will help solidify your understanding and enhance your problem-solving skills.
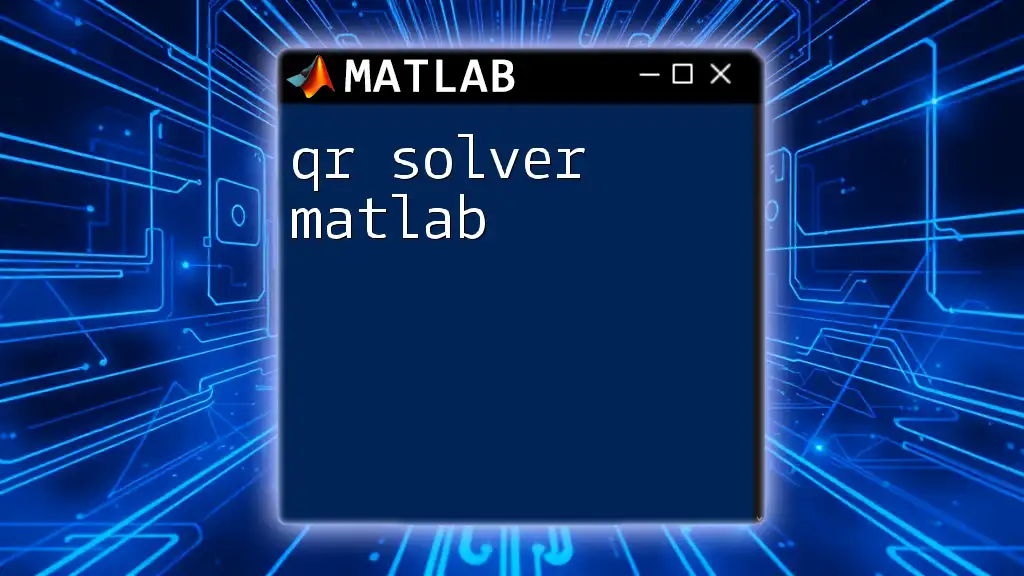
Additional Resources
Further Reading
For a deeper dive, refer to the official MATLAB documentation on fsolve and related functions. Online forums like MATLAB Central can also be valuable for encountering real-world problems and solutions.
Tutorials and Courses
Consider enrolling in specialized courses offered by our company that focus on using MATLAB effectively, particularly with functions like fsolve, to improve both your theoretical knowledge and practical skills.
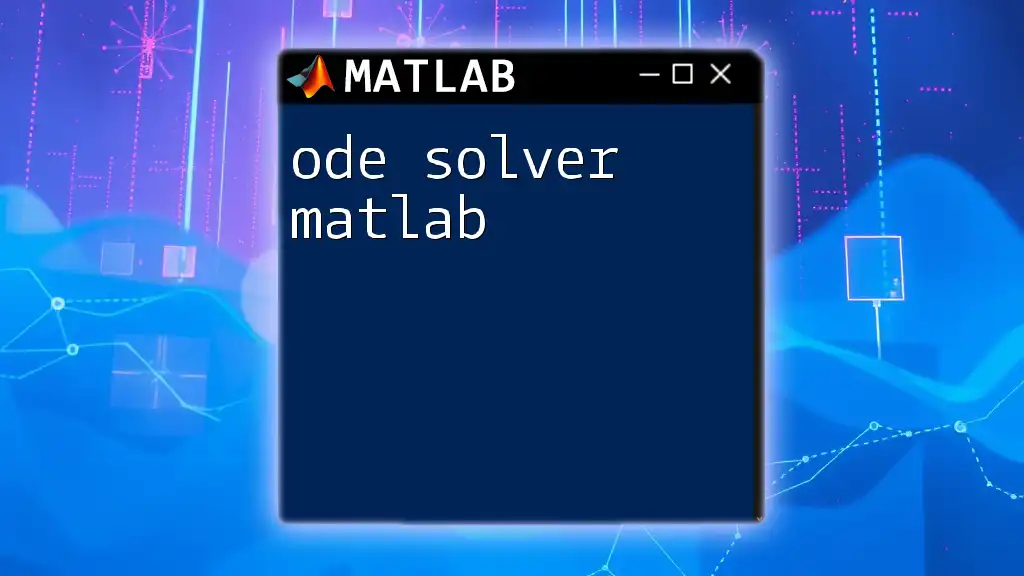
Call to Action
If you're interested in improving your MATLAB skills or exploring more advanced topics like fsolve, reach out to us for personalized tutoring or workshops designed to elevate your understanding and application of MATLAB in real-world scenarios.