The Singular Value Decomposition (SVD) in MATLAB is a powerful technique used for dimensionality reduction, noise reduction, and data compression, allowing you to decompose a matrix into its singular values and vectors.
Here’s a simple code snippet to perform SVD in MATLAB:
% Example of SVD in MATLAB
A = [1, 2; 3, 4; 5, 6]; % Input matrix
[U, S, V] = svd(A); % Perform SVD
What is SVD?
Singular Value Decomposition (SVD) is a powerful mathematical technique used in linear algebra. Essentially, it decomposes a matrix into three other matrices, simplifying complex calculations and transformations.
Definition and Mathematical Background
At its core, SVD helps express a matrix \( A \) as the product of three matrices:
\[ A = U \cdot S \cdot V^T \]
Where:
- U is an orthogonal matrix containing the left singular vectors.
- S is a diagonal matrix containing the singular values.
- V is an orthogonal matrix containing the right singular vectors.
SVD is tightly linked to concepts such as eigenvalues and eigenvectors, making it applicable to various fields including data analysis, statistics, and machine learning.
Applications of SVD
SVD finds numerous applications, including:
- Dimensionality Reduction: By reducing the number of attributes in data without losing essential information, SVD streamlines analysis in tasks like Principal Component Analysis (PCA).
- Image Compression: In image processing, SVD helps reduce the file size while retaining crucial visual quality. By retaining only the most significant singular values, an image can be stored more efficiently.
- Data Noise Reduction: SVD effectively filters out noise in data, helping to enhance the signal or meaningful patterns.
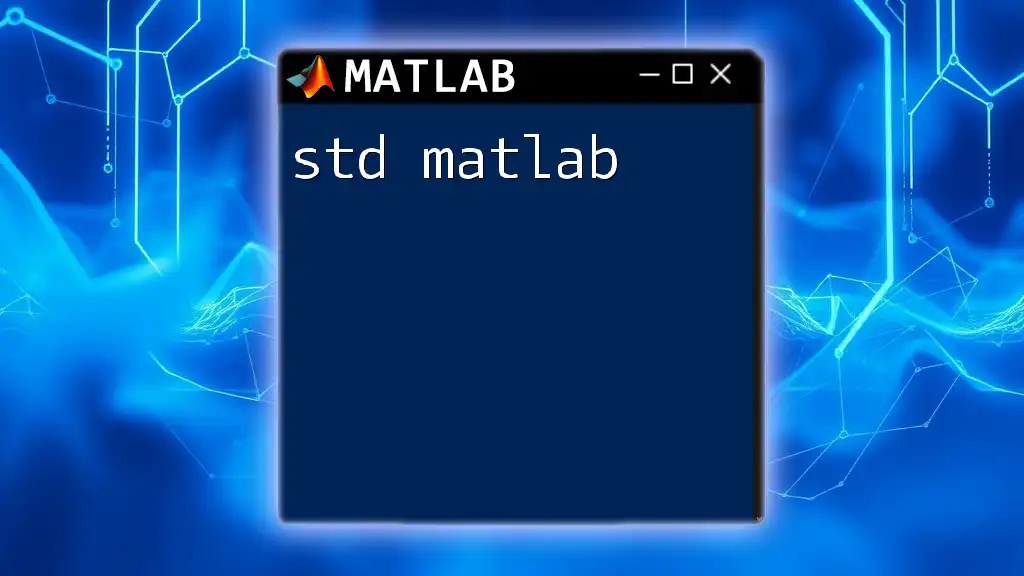
Understanding the SVD in MATLAB
Syntax of the SVD Function
In MATLAB, the basic command to perform SVD is:
[U, S, V] = svd(X)
Where `X` is your input matrix. The output will yield three matrices: U, S, and V, corresponding to the left singular vectors, singular values, and right singular vectors, respectively.
Components of SVD
- The U Matrix: Contains the left singular vectors. Each column of U represents an extracted pattern from the original dataset.
- The S Matrix: A diagonal matrix where the diagonal elements are the singular values, providing insight into the significance of corresponding singular vectors. The values are generally arranged in descending order.
- The V Matrix: Comprises the right singular vectors, representing another transformation of the original data.
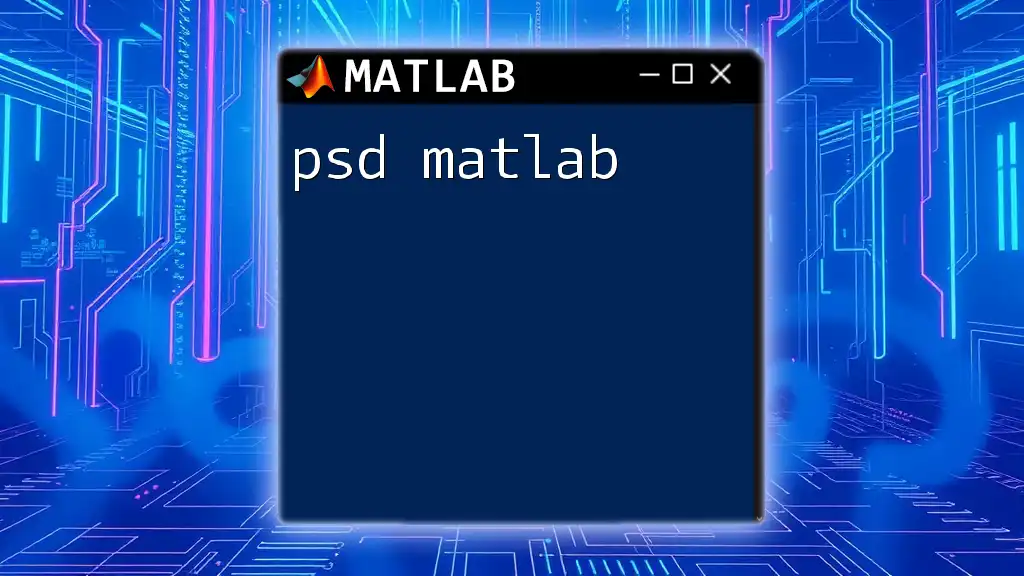
Steps to Perform SVD in MATLAB
Preparing Your Data
Before executing SVD, it's crucial to ensure your matrix is in the correct format. SVD can be applied to both square and rectangular matrices, allowing for flexibility in data analysis.
Example of Creating a Matrix in MATLAB
You can create a sample matrix in MATLAB like this:
A = [3 2; 1 4; 0 5];
Executing SVD
To execute SVD on your matrix A, simply run the command:
[U, S, V] = svd(A);
This command initiates the decomposition, yielding the matrices U, S, and V.
Explanation of Output Variables
After executing the SVD command:
- U gives the left singular vectors, providing the basis for the column space of your matrix.
- S contains the singular values, which reflect the importance of each vector in capturing the dataset's structure.
- V gives the right singular vectors, representing the basis for the row space.
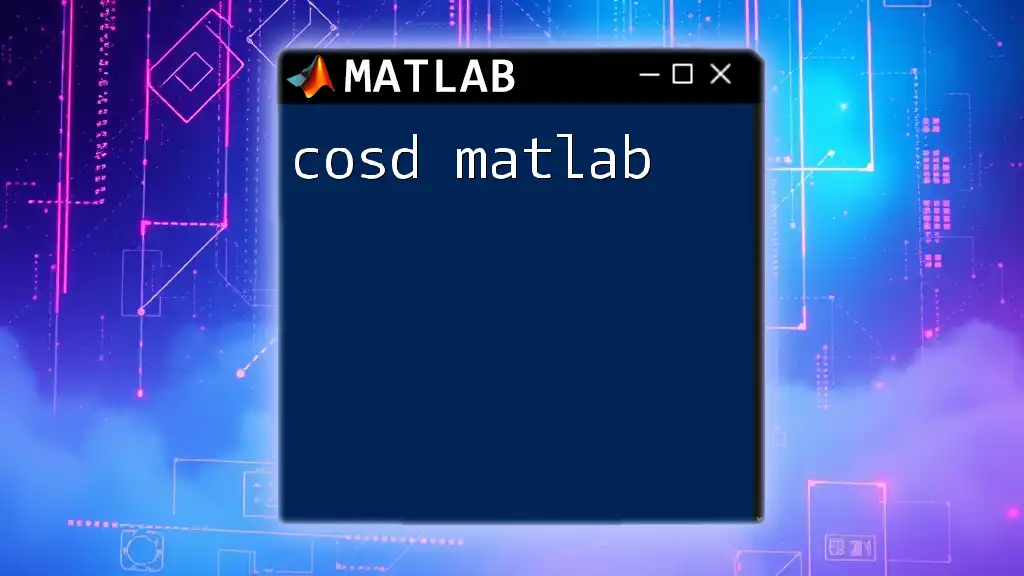
Interpreting SVD Results
Analyzing the U, S, and V Matrices
The resulting matrices hold significant interpretations:
- The U and V matrices are orthonormal, meaning their columns are orthogonal and normalized.
- The S matrix's size is determined by the smaller dimension of the original matrix, carrying valuable insights on data variance and relevance.
Visualizing Singular Values
Understanding the importance of singular values can be aided by visual representation. You can plot singular values using the following code:
singularValues = diag(S);
plot(singularValues);
title('Singular Values');
xlabel('Index');
ylabel('Value');
This visualization allows for a quick grasp of how many singular values are significantly contributing to the structure of your data.
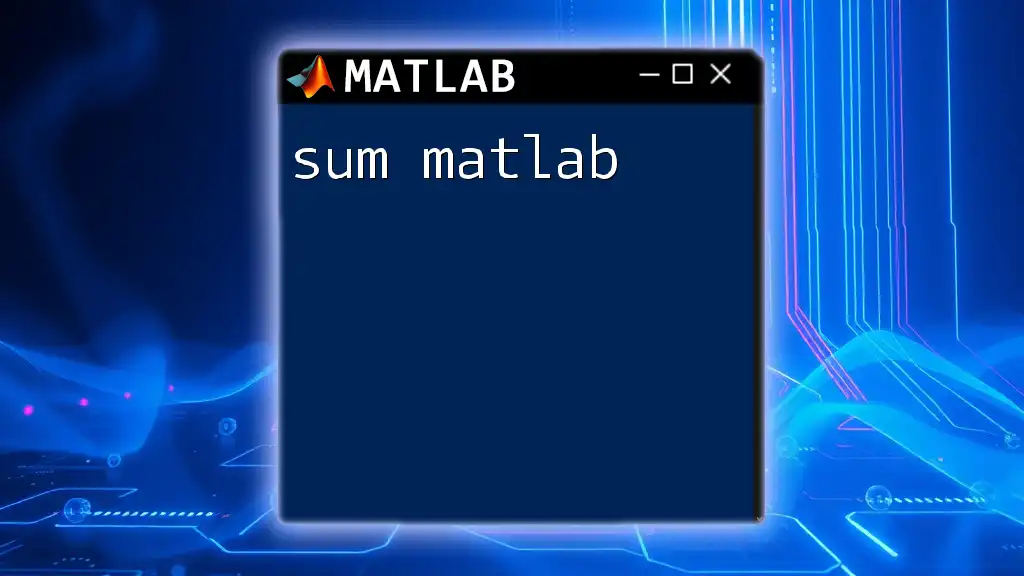
Applications of SVD in Real-Life Scenarios
Image Compression
SVD is particularly useful in image processing. By applying SVD, you can recognize patterns and store images more efficiently.
Example of Image Compression Using SVD
Here’s a quick illustration of how SVD can compress images in MATLAB:
img = imread('image.jpg');
img = double(rgb2gray(img)); % Converting an image to grayscale and double
[U, S, V] = svd(img);
In this example, the image is first converted to grayscale and then decomposed using SVD. You can then reconstruct the image using only a subset of singular values to achieve compression.
Dimensionality Reduction in Machine Learning
Reducing dimensions enhances the training process for models by cutting out noise and irrelevant features, leading to improved performance.
Code Example for Dimensionality Reduction
For instance, after obtaining the matrices, reducing the dimensions can be executed like so:
k = 2; % Number of dimensions to keep
reducedData = U(:,1:k) * S(1:k,1:k);
Here, we select the first k components from U and S, effectively reducing the dimensions of our data.
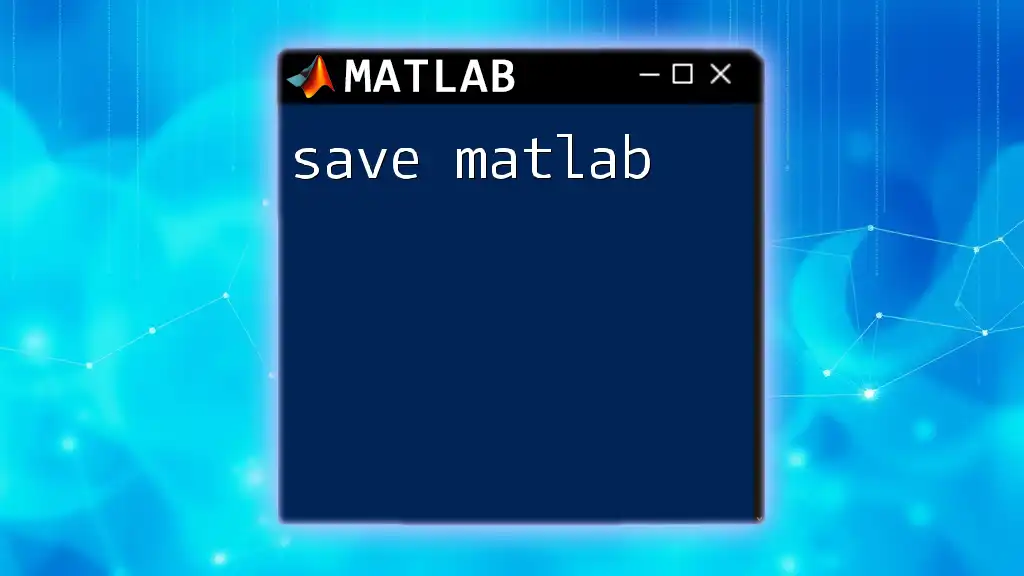
Troubleshooting Common Issues with SVD
Issues with Input Data
When working with SVD, it's crucial to ensure that your input data is numeric and structured correctly. Non-numeric data can lead to errors during decomposition.
Scaling and normalization of matrices might also be necessary to achieve optimal results, especially if the range of your data varies significantly.
Performance Tips
Working with large matrices can be computationally intensive. To enhance performance, consider using SVD's 'econ' option, which calculates the economy-sized decomposition to minimize resource usage:
[U, S, V] = svd(A, 'econ');
This command streamlines the process by returning smaller output matrices when possible, making it efficient for large datasets.
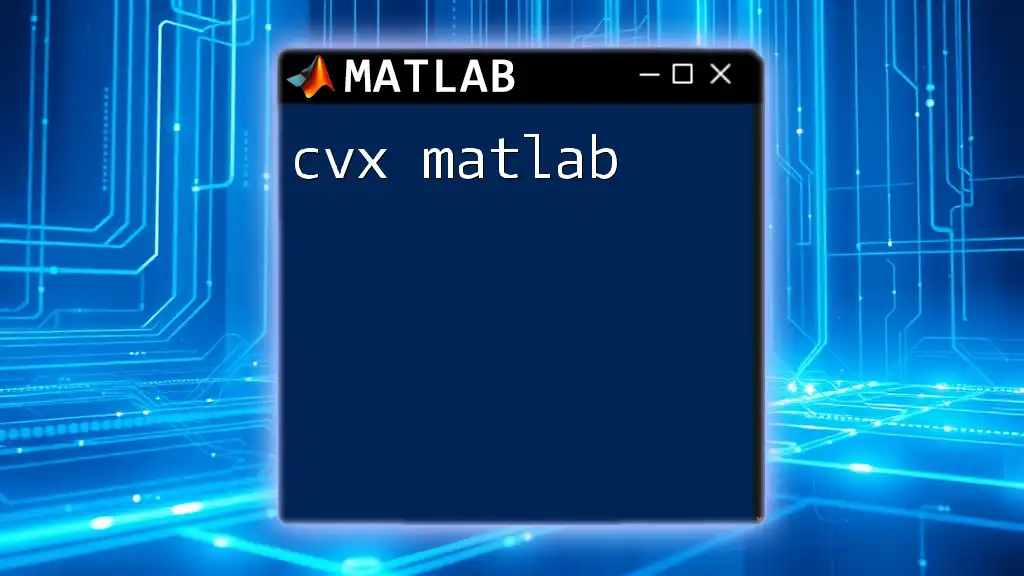
Conclusion
In summation, SVD in MATLAB is a robust tool that enhances data analysis capabilities. By understanding its components, applications, and execution, users can unlock rich insights from their data, whether it's for dimensional reduction or image compression. Embrace the power of SVD to improve your workflows in data science and machine learning.
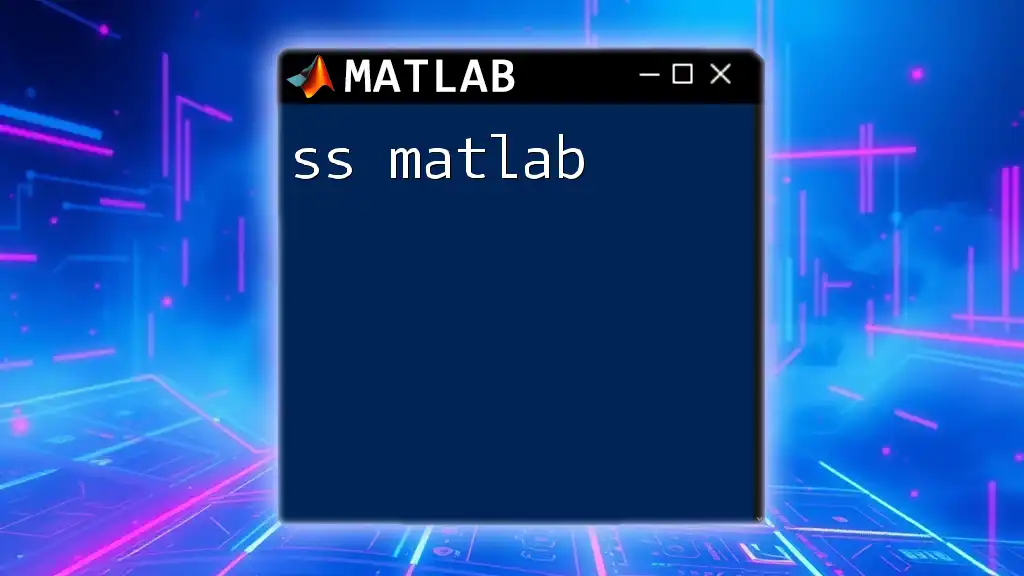
Additional Resources
For deepening your knowledge, explore the MATLAB documentation on SVD for a comprehensive understanding. Consider recommended books and online courses that delve into linear algebra, data analysis, and practical applications of SVD to elevate your skillset.