The `std` function in MATLAB calculates the standard deviation of an array, providing a measure of the amount of variation or dispersion in the data set.
Here’s a basic code snippet showing how to use the `std` function:
data = [1, 2, 3, 4, 5];
standard_deviation = std(data);
disp(standard_deviation);
What is the `std` Function?
The `std` function in MATLAB is a vital statistical tool used to compute the standard deviation of a data set. Standard deviation measures the amount of variability or dispersion in a set of values, indicating how much individual data points deviate from the mean.
Understanding standard deviation is crucial in various fields, from quality control in manufacturing to risk assessment in finance. It provides insights into data spread, helping to interpret datasets meaningfully.
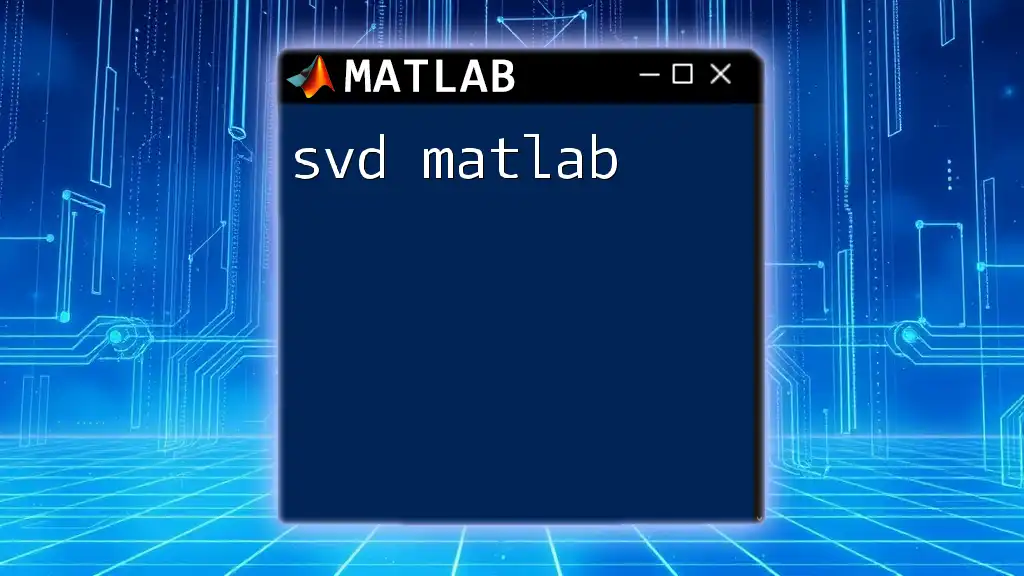
Syntax and Basic Usage
Syntax
The syntax for the `std` function is simple:
S = std(A)
Here, `A` can be a vector or matrix, while `S` returns the standard deviation. By default, MATLAB computes the sample standard deviation, which means it adjusts the formula for a sample rather than the entire population.
Basic Example
To illustrate its usage, consider the following example:
data = [1, 2, 3, 4, 5];
standard_deviation = std(data);
disp(standard_deviation);
When executed, this script returns 1.4142, signifying that, on average, the data points deviate from the mean by approximately 1.4142 units. This understanding of variability is fundamentally important for making data-driven decisions.
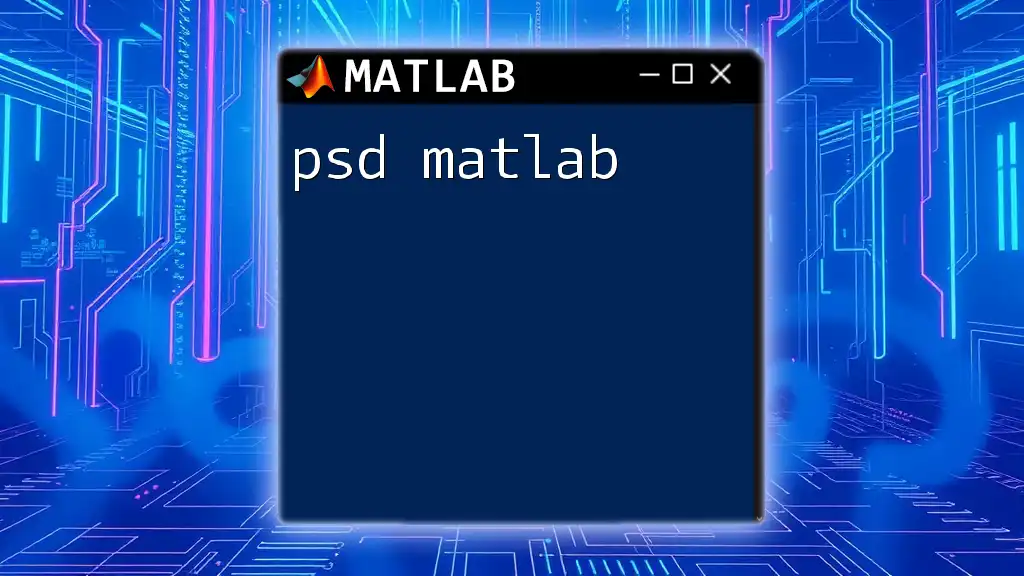
Computing Standard Deviation of Different Data Types
Vectors
The `std` function can be applied to both row and column vectors without any changes.
For instance:
row_vector = [1, 2, 3, 4, 5];
column_vector = [1; 2; 3; 4; 5];
std_row = std(row_vector); % Computes standard deviation of the row vector
std_column = std(column_vector); % Computes standard deviation of the column vector
Both `std_row` and `std_column` will return the same value, indicating the consistent functionality of the `std` command across data orientations.
Matrices
When applied to matrices, the `std` function allows you to specify the dimension along which to compute the standard deviation—either across rows or columns.
For example:
matrix_data = [1, 2, 3; 4, 5, 6];
std_dim1 = std(matrix_data, 0, 1); % calculates along columns
std_dim2 = std(matrix_data, 0, 2); % calculates along rows
- `std_dim1` will output an array reflecting the standard deviation of each column.
- `std_dim2` offers insight into the variance across each row.
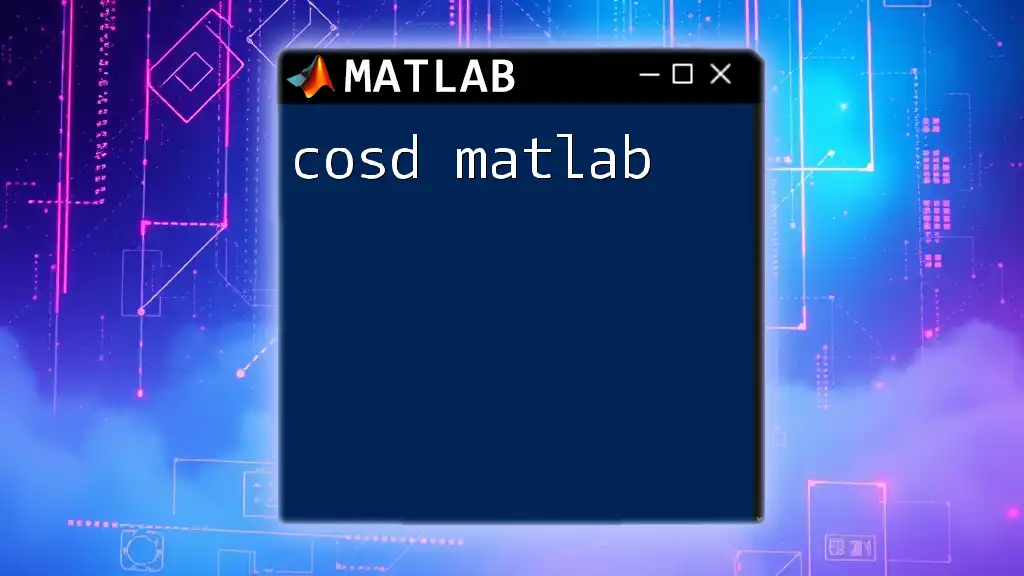
Normalization and Unbiased Estimation
Population vs Sample Standard Deviation
When working with datasets, it's crucial to determine whether you want the population standard deviation or the sample standard deviation. The `std` function allows this distinction through its second parameter.
To compute the population standard deviation, set the second argument to 1 as shown below:
population_std = std(data, 1); % Note the second param is 1
Here, setting the parameter to 1 transforms the calculation to reflect the entire population, utilizing the formula without adjusting for degrees of freedom.
Why It Matters: Unbiased Estimator
The choice between sample and population standard deviation affects the statistical analysis significantly. The sample standard deviation is adjusted by dividing by \( N-1 \) (degrees of freedom), rendering it an unbiased estimator. This adjustment is necessary to account for the variability within the sample, providing a more accurate representation of the whole population.
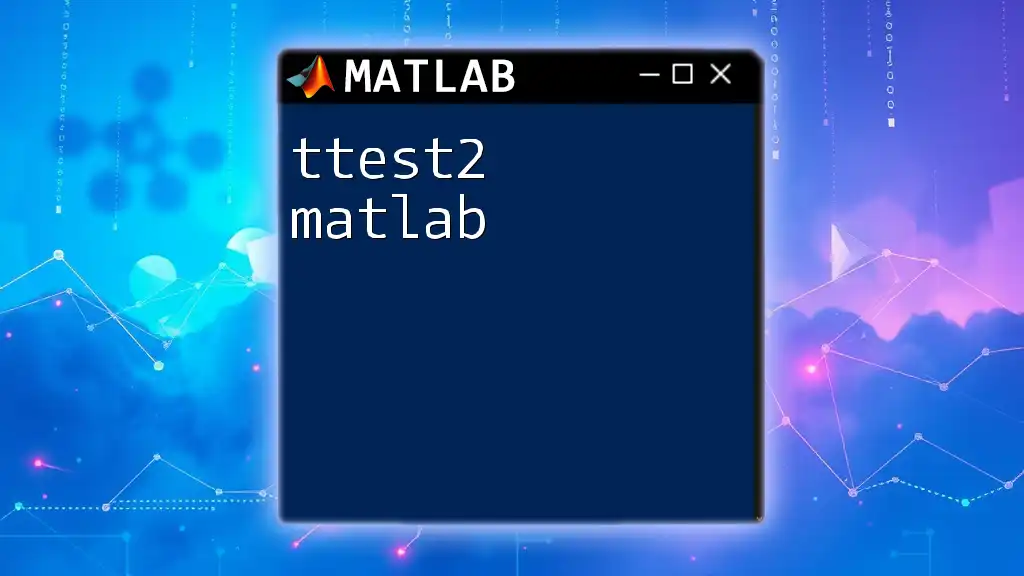
Handling NaN Values
Importance of Clean Data
In real-world applications, datasets often contain NaN (Not-a-Number) values that can disrupt calculations. Understanding how to manage these values is pivotal for accurate analysis.
Using `std` with NaN Values
MATLAB provides an option to ignore NaN values when calculating the standard deviation. The `omitnan` option allows you to effectively handle missing data.
For instance:
data_with_nan = [1, 2, NaN, 4];
std_with_nan_removal = std(data_with_nan, 'omitnan');
In this case, `std_with_nan_removal` will compute the standard deviation as if the NaN were not present, providing a more accurate representation of the remaining data.
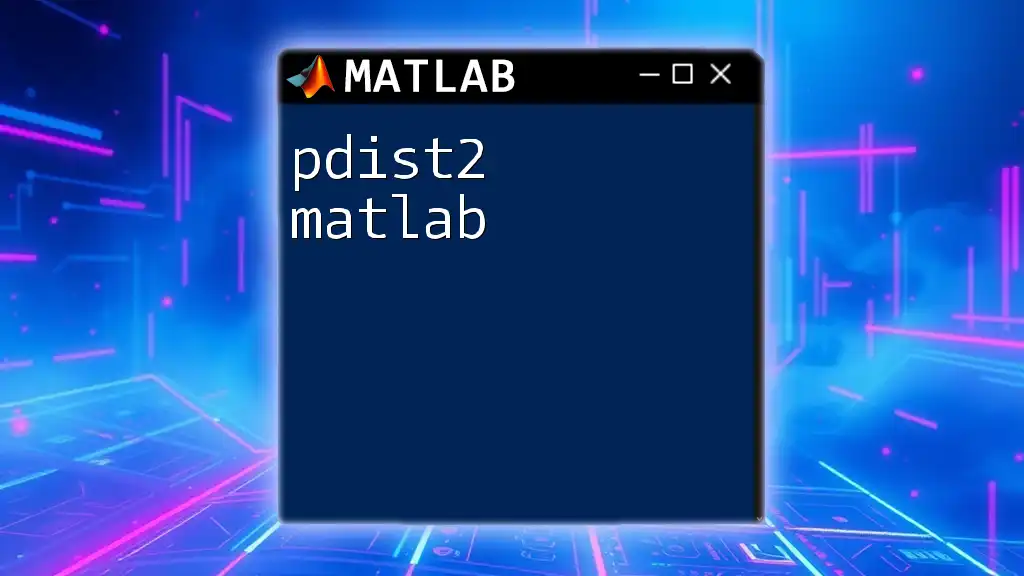
Applying `std` in Real-World Scenarios
Case Studies
Different fields rely on the `std` function to interpret variability in their datasets. For instance, in finance, analysts often use the standard deviation to evaluate the risk associated with assets.
Here's a simple application in stock price analysis:
stock_prices = [100, 102, 105, 107, 104, 109];
stock_std = std(stock_prices);
The output will give you the standard deviation of the stock prices, indicating the variation in prices over the evaluated period. A higher standard deviation reflects more volatility in stock prices.
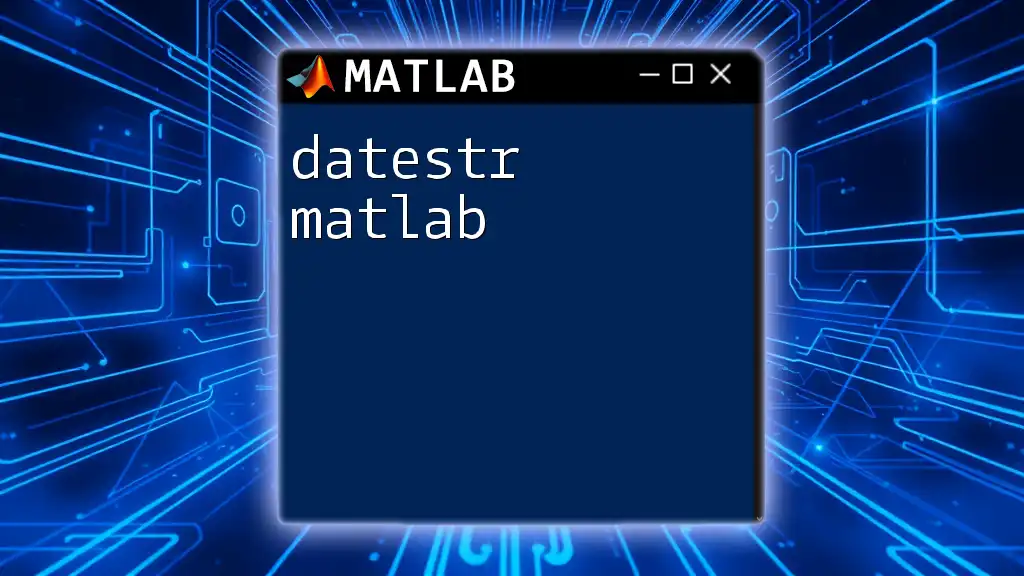
Visualization of Standard Deviation
Using Graphs to Understand Variability
Visualizing data and its variability through standard deviation can significantly enhance understanding. Irrespective of the field, interpretation becomes clearer when variability is displayed graphically.
Code Snippet for Visualization
Utilizing MATLAB’s `errorbar` function, you can illustrate standard deviation effectively:
mu = mean(stock_prices);
error = std(stock_prices);
x = 1:6; % Sample data points
errorbar(x, mu, error);
The resulting graph will show the mean stock price and its variability, allowing for intuitive insights into performance trends over time.
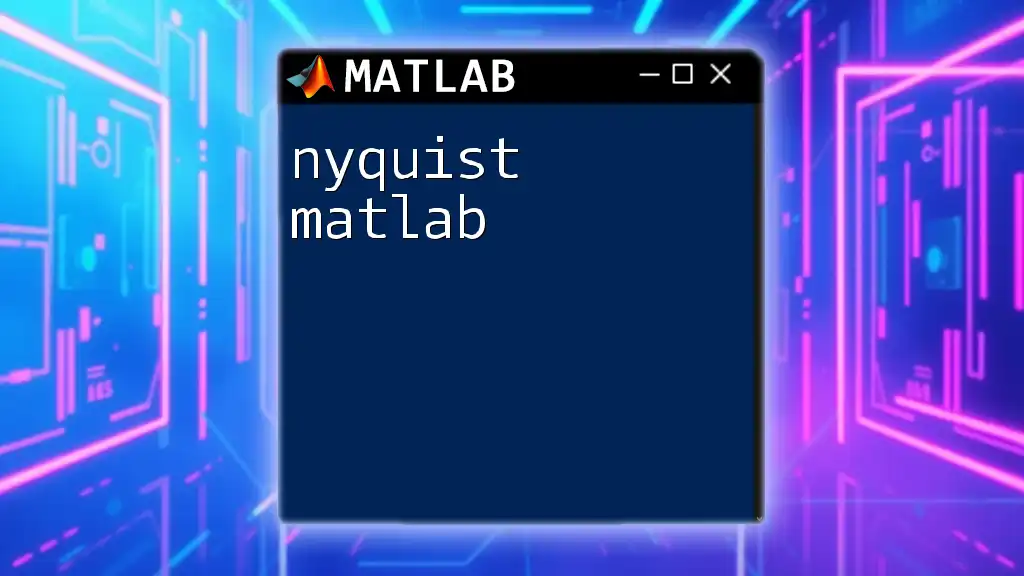
Advanced Usage of `std` with Custom Functions
Writing Custom Wrapper Functions
To streamline repeated calculations involving standard deviation, you might opt to create custom functions. These can encapsulate common settings and operations, making your code cleaner and more maintainable.
Sample Code
Here’s an example of a simple custom function:
function result = custom_std(data)
result = std(data, 'omitnan');
end
This function allows you to calculate the standard deviation while explicitly removing NaN values, ensuring cleaner outputs across various datasets.
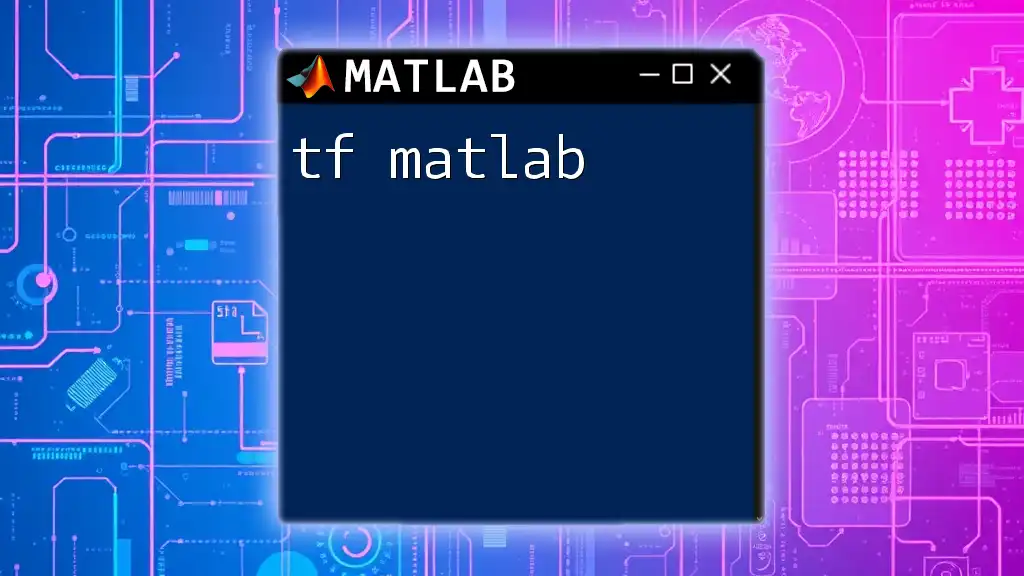
Conclusion
In conclusion, the `std` function in MATLAB serves as an invaluable tool for analyzing data variability. It empowers users to interpret datasets accurately, enabling informed decisions based on statistical analysis. Mastering the `std` function opens the door to enhanced data interpretation and effective utilization of MATLAB's vast capabilities.
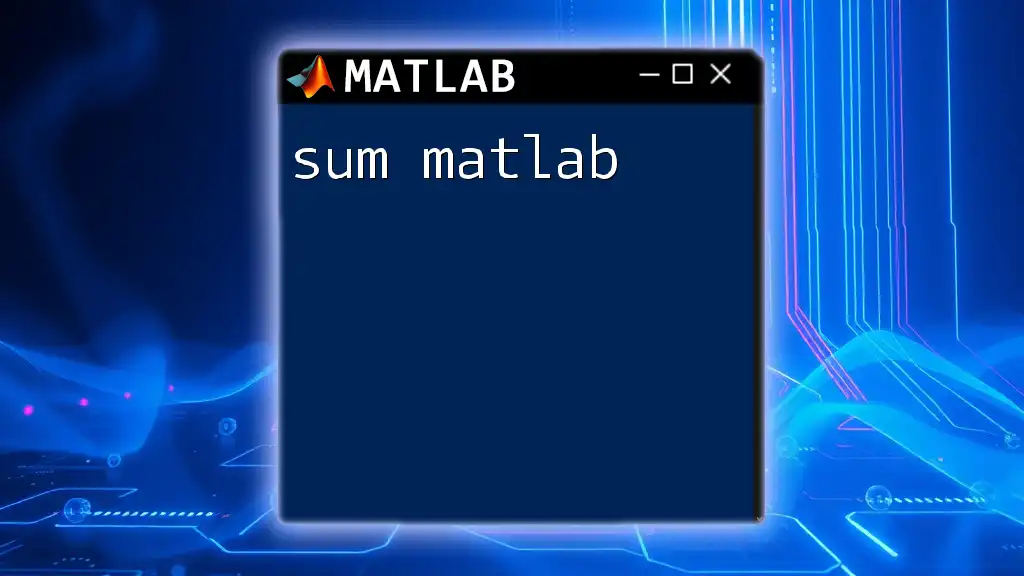
Frequently Asked Questions (FAQ)
What is the difference between `std` and variance?
While both measure data spread, they differ in calculation. Variance is the square of the standard deviation. Thus, knowing one allows you to calculate the other easily.
When should I use `std`?
Utilize the `std` function when you need to understand the variability of your data, especially in fields such as finance, engineering, or any area where data analysis is critical.
Can `std` be used on multidimensional arrays?
Yes, `std` can be applied to multidimensional arrays by specifying the dimension along which to compute the standard deviation, thereby enabling flexibility in data analysis.
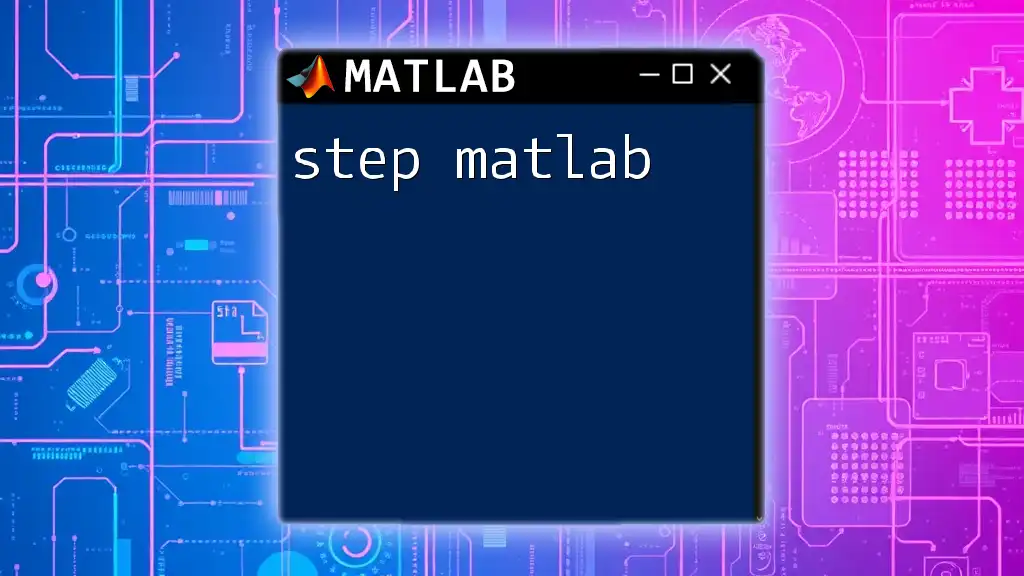
Additional Resources
For those looking to delve deeper, refer to MATLAB's official documentation and forums. They offer a vast array of tutorials and support from the community, enriching your learning journey with MATLAB and enhancing your understanding of its functions like `std`.