The `grad` function in MATLAB is typically used to compute the gradient of a matrix or function, providing insight into its rate of change in multi-dimensional space.
% Example of calculating the gradient of a 2D matrix
A = [1 2 3; 4 5 6; 7 8 9]; % Sample matrix
[FX,FY] = gradient(A); % Calculate gradient in x and y directions
disp('Gradient in x direction (FX):');
disp(FX);
disp('Gradient in y direction (FY):');
disp(FY);
Understanding the `grad` Function in MATLAB
What Does the `grad` Function Do?
The `grad` function in MATLAB plays a crucial role in calculating gradients, which are fundamental to numerous mathematical and engineering applications. A gradient essentially indicates the direction and rate of the fastest increase of a function. Understanding how to utilize the `grad` function is vital for tasks such as optimization and modeling, as it allows users to navigate function landscapes efficiently.
Syntax of `grad` Function
The syntax for the `grad` function can be described as follows:
G = grad(f, x)
Here, `f` represents the function for which you want to compute the gradient, while `x` is the point at which the gradient is evaluated. Mastering this syntax is essential to effectively apply the `grad` function in real-world scenarios.
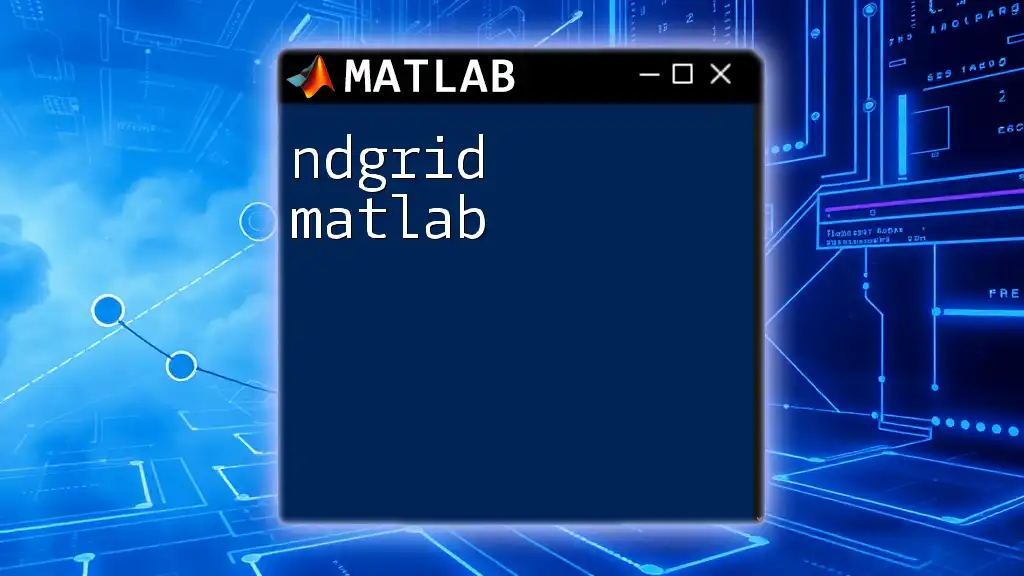
Implementing the `grad` Function
Setting Up MATLAB Environment
Before diving into using MATLAB, ensure you have the software installed on your machine. You can download it from the MathWorks official website. Following installation, familiarize yourself with the interface. It's advisable to explore the MATLAB documentation to learn about its various toolboxes, especially if you're working on specialized projects requiring additional features.
Creating Sample Functions
To illustrate the application of the `grad` function, let’s define a simple function:
Defining a Simple Function
For this example, we will create a quadratic function represented as:
f = @(x) x^2 + 3*x + 2; % Simple quadratic function
This function is chosen due to its straightforward structure, which makes it easy to compute the gradient.
Using `grad` to Calculate the Gradient
Next, we will compute the gradient at a specific point. Let's say we want to find the gradient at `x = 1`. Here’s how you can implement this:
x = 1; % Point at which gradient is calculated
G = grad(f, x); % Calculate the gradient
In this snippet, `G` will hold the value of the gradient, which is a measure of how steep the function is at `x = 1`.
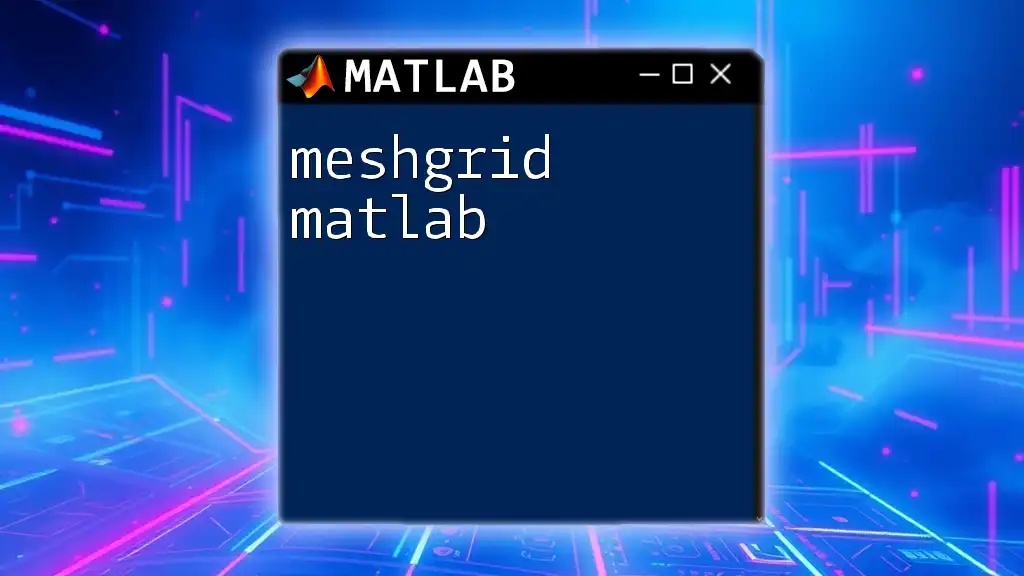
Applications of the `grad` Function
Optimization Problems
Gradients are essential in optimization techniques, particularly with algorithms like gradient descent. The idea behind gradient descent is to iteratively update your parameters in the direction of the steepest descent, minimizing your function effectively.
For instance, if you have a function `f` and want to minimize it, you may use the following approach:
alpha = 0.01; % Learning rate
x = 10; % Starting point
for i = 1:100
G = grad(f, x); % Calculate the gradient
x = x - alpha * G; % Update the parameter
end
In this case, `alpha` is the learning rate, which determines the size of the steps you take towards the minimum.
Physics and Engineering
Gradients also find extensive application in physics, especially in computing forces and potential energy. Consider a potential energy function of an object in a gravitational field, `U(x, y) = mgh`, where `m` represents mass, `g` represents gravitational acceleration, and `h` signifies height. The gradient of this function could help in predicting the motion of the object.
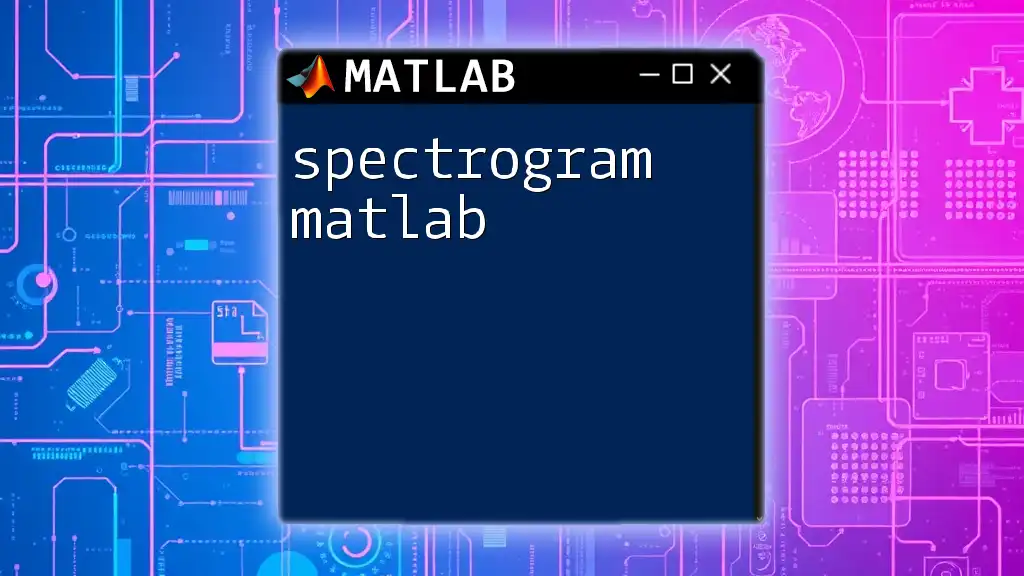
Advanced Topics
Working with Multivariable Functions
The `grad` function can also be extended to accommodate multivariable functions, enabling more complex analyses. For example, consider a function of two variables, defined as follows:
f = @(x,y) x.^2 + y.^2; % Function of two variables
When dealing with multiple variables, the gradient will produce a vector consisting of partial derivatives for each variable. This vector indicates the direction in which the function increases most rapidly.
Gradient Visualization
Visualizing gradients can greatly enhance understanding. A quiver plot is one way to illustrate this in MATLAB. Here’s a simple example to visualize the gradient field of the aforementioned multivariable function:
[X,Y] = meshgrid(-5:0.5:5, -5:0.5:5);
Z = f(X,Y);
[gx, gy] = gradient(Z);
quiver(X,Y,gx,gy); % Display gradient field
This code generates a 2D quiver plot representing the gradient field, allowing users to see the direction of steepest ascent visually.
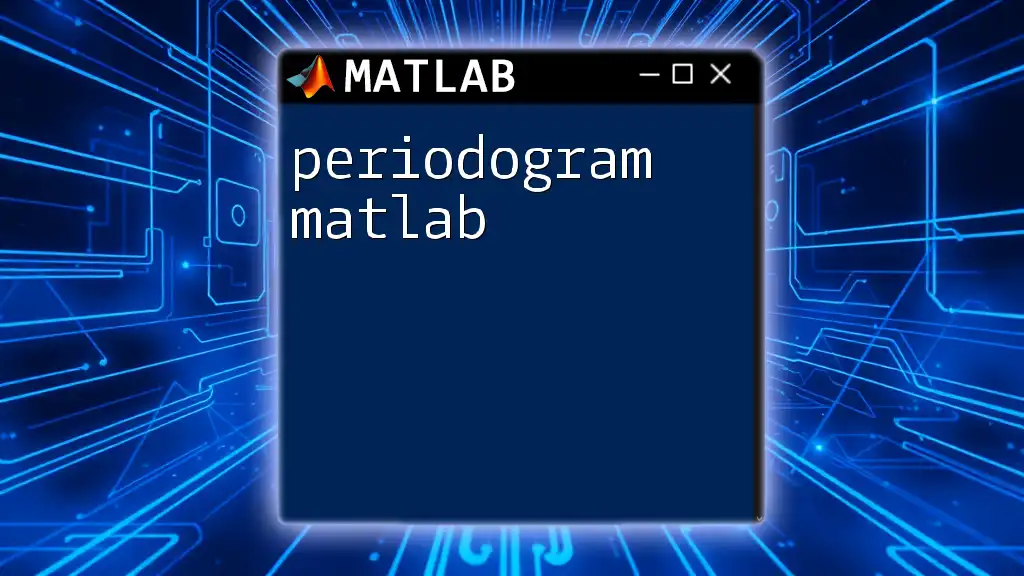
Common Errors and Troubleshooting
Common Mistakes When Using `grad`
When utilizing the `grad` function, some common mistakes can occur, such as forgetting to define the function properly or using incorrect dimensions for the input parameters. Ensuring that your function is defined as an anonymous function and that your input points are correctly specified is crucial.
Debugging Tips
Always review your function and input data if you encounter unexpected results. MATLAB offers debugging tools that can help trace errors in your code. Use simple test cases to ensure your `grad` function implementation is accurate before progressing to complex scenarios.
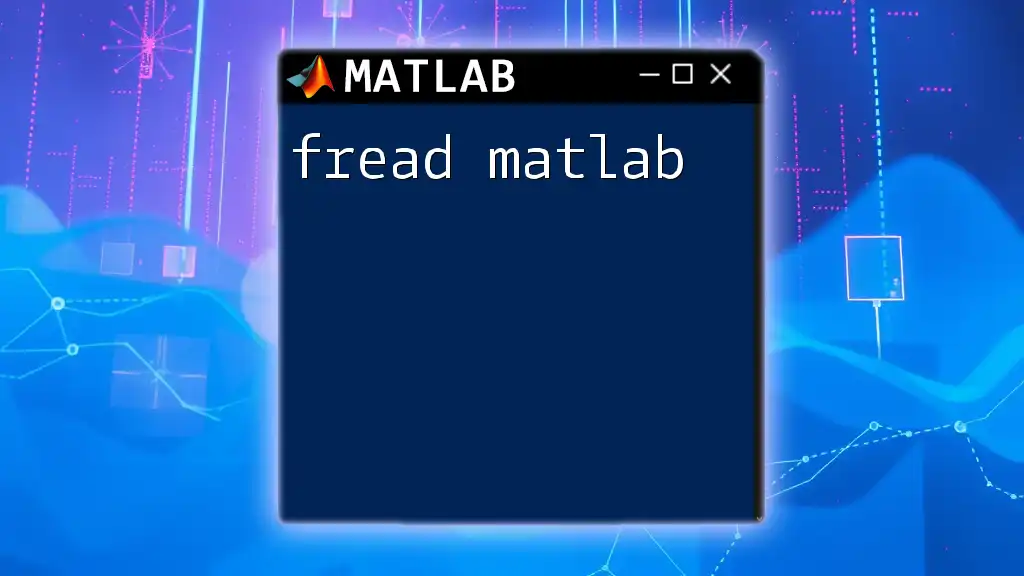
Conclusion
In this guide, we explored the `grad` function in MATLAB, covering its implementation in both single-variable and multivariable contexts. We also discussed its importance in optimization, physics, and engineering applications. By understanding how to compute and visualize gradients, you can enhance your MATLAB skills significantly.
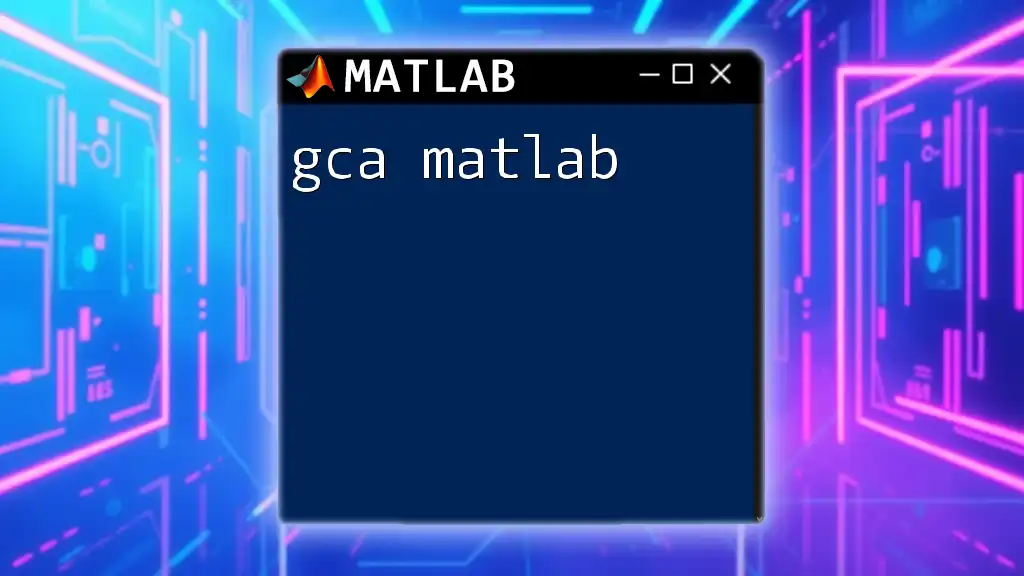
Additional Examples and Exercises
Practice Problems
- Define your own multivariable function and compute its gradient at various points.
- Implement a gradient descent algorithm to minimize a function of your choice.
Solutions to Practice Problems
Once you have attempted these exercises, refer to MATLAB's documentation or online forums to verify your solutions and broaden your understanding of gradient calculations.
By consistently practicing and experimenting, proficiency in using the `grad` function and MATLAB's computational capabilities will naturally follow.