The `end` command in MATLAB is used to reference the last element in arrays, matrices, or other data structures, simplifying operations when manipulating variable lengths. Here's a simple example:
A = [1, 2, 3; 4, 5, 6; 7, 8, 9];
lastElement = A(end); % This retrieves the last element of matrix A, which is 9
Understanding the `end` Keyword in MATLAB
What is the `end` Keyword?
The `end` keyword in MATLAB is a powerful tool that allows programmers to refer dynamically to the last element of arrays, matrices, cell arrays, and more. This keyword plays an essential role in helping users write more flexible and efficient code. Understanding how to utilize `end` can significantly improve your coding efficiency and make your scripts easier to read.
The Role of `end` in Different Contexts
In Indexing
One of the most common uses of `end` is in indexing. When working with arrays or vectors, `end` enables you to easily reference the last element without needing to know the exact size of the array.
For instance, consider the following example:
A = [1, 2, 3, 4, 5];
lastElement = A(end); % returns 5
In this code snippet, `A(end)` accesses the last element of the array `A`. This dynamic approach makes it unnecessary to hard-code the length of the array into your code, allowing for greater adaptability.
In Loop Constructs
The `end` keyword can also be effectively used in `for` and `while` loops to refer to the last index of an array during iteration. This can help prevent off-by-one errors.
For example:
A = [10, 20, 30, 40, 50];
for k = 1:end
disp(A(k));
end
In this loop, `end` allows the loop to run through every element in `A`, regardless of its length. However, note that you typically must specify the number of iterations explicitly if used this way, so adjust accordingly based on your loop structure.
In Functions
Within function definitions, `end` indicates the termination of the function body. Here’s a simple example demonstrating that:
function result = computeSquare(x)
result = x^2;
end
In this code, `end` clearly marks where the function `computeSquare` concludes. This clarity improves code readability, especially in scripts containing multiple functions.
Other Usage of `end` in Structures
In Cell Arrays
The `end` keyword also applies to cell arrays, providing a straightforward way to reference the last element. This is particularly useful since cell arrays can contain varying types of data.
Consider this example:
C = {10, 20, 30};
lastCell = C{end}; % returns 30
In this case, using `C{end}` allows you to easily retrieve the last value from the cell array `C` without needing to know its length.
In Matrices
When dealing with matrices, `end` can help you access entire rows or columns. This can simplify many common matrix operations.
For instance:
M = rand(4, 3); % 4x3 matrix
lastRow = M(end, :); % getting the last row
Here, `M(end, :)` gets the last row of the matrix `M`. This approach is essential for dynamic programming, especially in applications dealing with varying-size datasets.
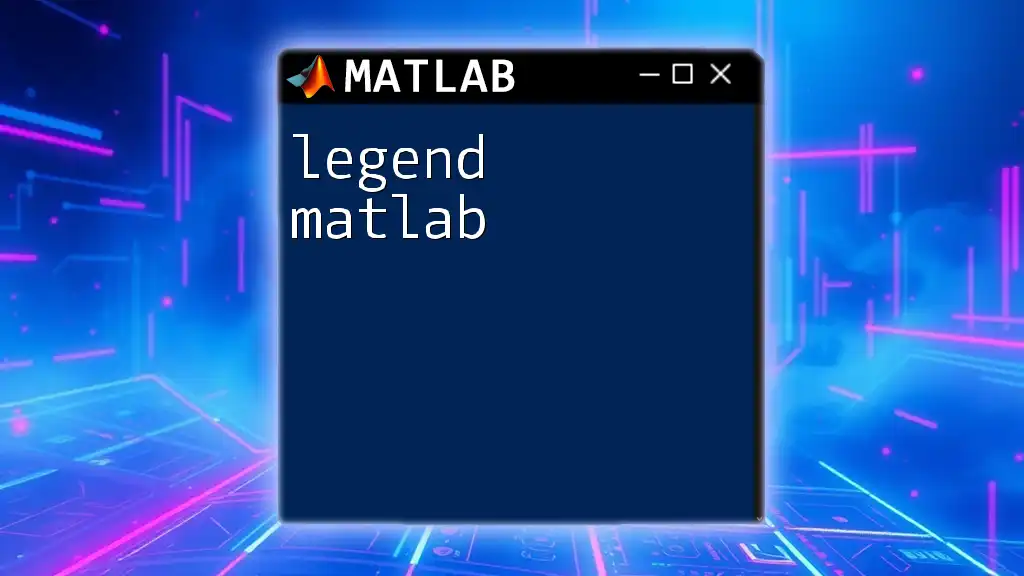
Benefits of Using `end`
Simplifying Code
Using `end` makes your code shorter and clearer. It reduces the need for manual indexing and dynamically adjusts when the size of the array or matrix changes. This way, it significantly streamlines code management, especially in larger scripts.
For example, if you use hard-coded indices, you risk running into errors if the data structure changes, whereas `end` automatically accommodates those changes.
Avoiding Hard-Coding
Leveraging `end` instead of hard-coded indices minimizes the risk of errors when the size of an array, matrix, or cell array varies. Hard-coded indices can lead to maintenance issues and errors down the line if your data structure changes.
For example, consider the difference:
% Hard-coded index (prone to errors)
lastElement = A(5);
% Using end (more flexible)
lastElement = A(end);
The second approach is not only more concise but also adaptable, ensuring your code runs correctly even if the size of `A` changes.
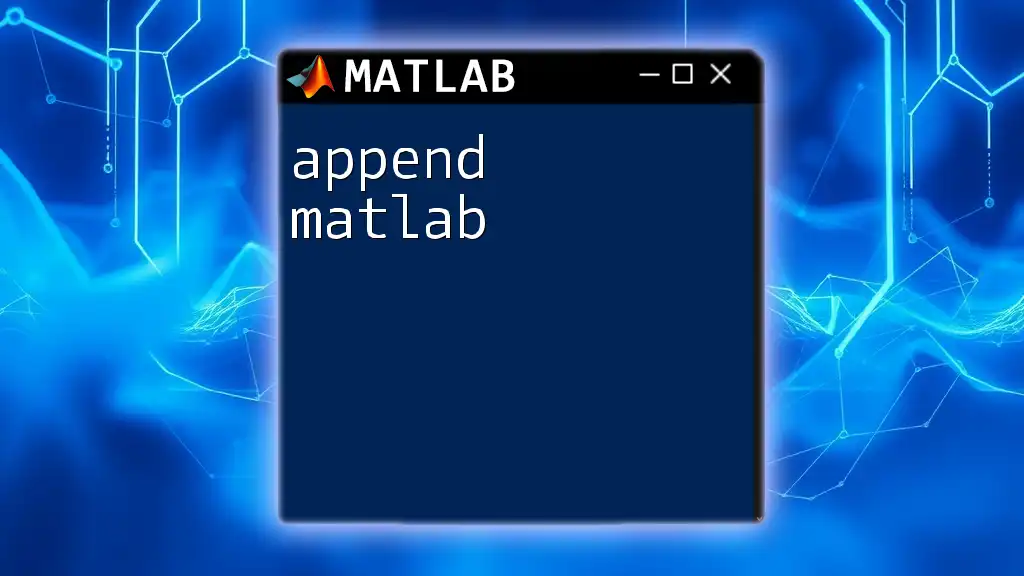
Common Mistakes with `end`
Misunderstanding `end` in Functions
One common mistake is misunderstanding the scope of the `end` keyword, especially in nested functions. If your outer function modifies the dimensions of its inputs, referencing `end` in an inner function may yield unexpected results. To avoid this, always be mindful of the context in which you use `end`.
Using `end` in Cell Arrays Incorrectly
Another frequent error occurs when accessing elements in cell arrays. Remember that MATLAB requires curly braces `{}` to access the contents of a cell. Attempting to use `end` without the proper syntax can lead to confusing errors.
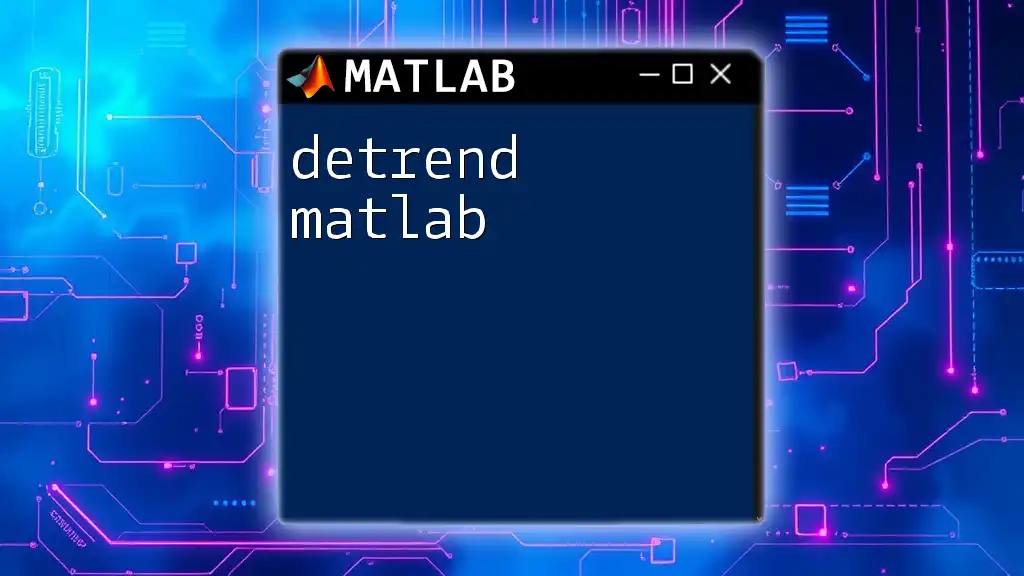
Tips for Effectively Using `end`
Best Practices
To make the most of the `end` keyword, consider these best practices:
- Use it consistently when looping through arrays or matrices to avoid hard-coding indices.
- Ensure clarity by clearly commenting your code, especially when using `end` in complex scripts.
When Not to Use `end`
While `end` can be very useful, there are times it may lead to confusion or errors. For example, it is usually best to avoid using `end` in contexts where the dimensions of arrays may not be clear, such as when dynamically reshaping data. In such cases, explicitly defining dimensions can enhance clarity.
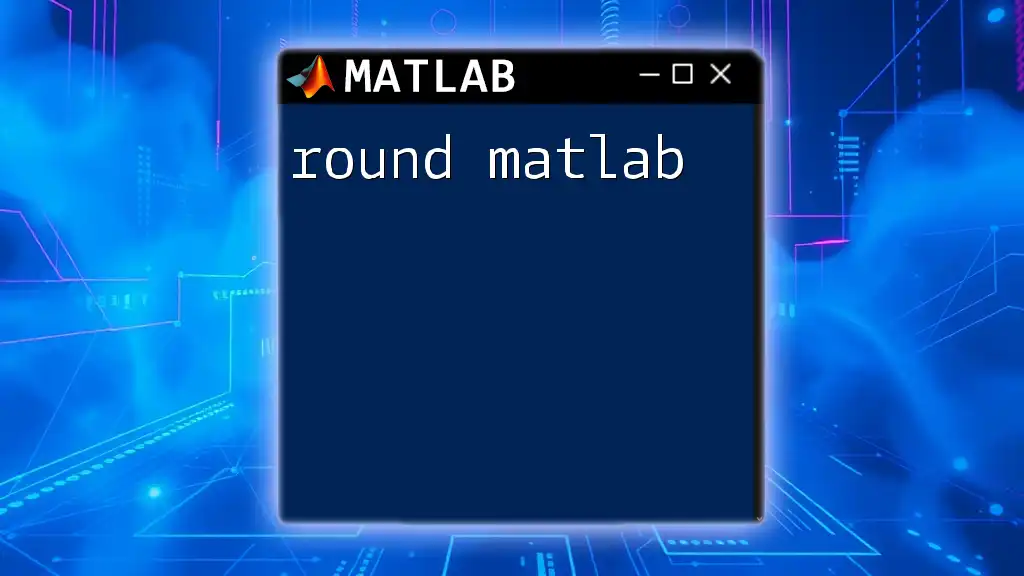
Conclusion
The `end` keyword in MATLAB is a versatile and powerful tool that can significantly enhance programming efficiency and code readability. By mastering how and where to use `end`, you'll find that your scripts become more robust and easier to maintain. Remember to practice using `end` across various contexts to fully leverage its capabilities in your MATLAB coding endeavors.
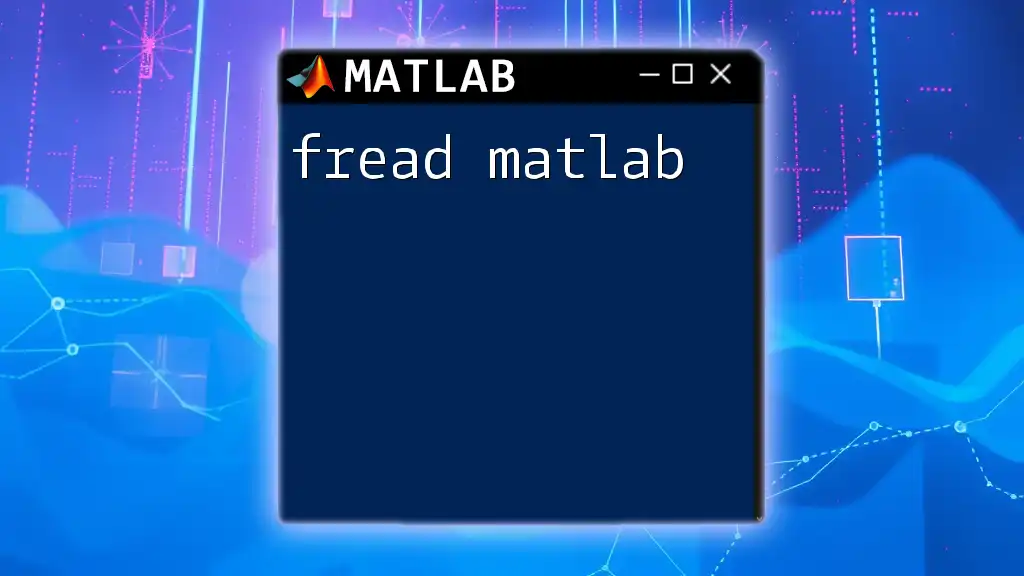
Additional Resources
For those looking to deepen their understanding of the `end` keyword and MATLAB coding in general, consider exploring additional tutorials, official documentation, and forums. Engaging with other MATLAB learners can provide invaluable insights and further solidify your skills.
Call to Action
Feel free to share your thoughts or questions in the comments section. If you're interested in mastering MATLAB commands and techniques more extensively, check out our courses tailored to help you become proficient quickly!