The `case` statement in MATLAB, often utilized within a `switch` block, allows you to execute different sections of code based on the value of a variable, enhancing code readability and efficiency.
Here's a simple example of a `switch-case` statement in MATLAB:
value = 2;
switch value
case 1
disp('Value is one');
case 2
disp('Value is two');
case 3
disp('Value is three');
otherwise
disp('Value is something else');
end
What is a Case Statement?
A case statement is a powerful control structure used in programming to streamline decision-making processes based on specific conditions. In MATLAB, the case statement is implemented through the `switch` construct, allowing developers to execute different blocks of code based on the value of an expression. By organizing multiple potential execution paths based on varying outcomes, case statements enhance code readability and maintainability.
Comparison with Other Control Flow Statements
When considering the best way to manage conditional logic in MATLAB, it's essential to understand the differences between case statements and other control flow options. If-else statements can handle a wide range of conditions, yet they tend to become unwieldy when handling many specific scenarios. A case statement, on the other hand, provides a cleaner and more organized structure, especially when one single variable needs to be evaluated against multiple potential values. For example, if you have to examine the status of different days of the week, using a case statement is often preferable for clarity.
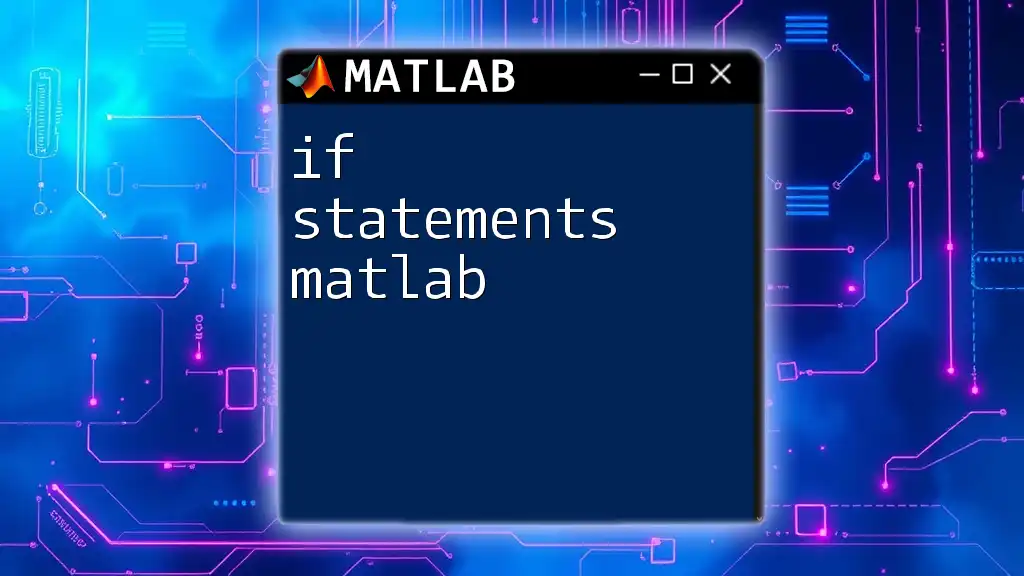
Syntax of the Case Statement in MATLAB
General Structure of the Case Statement
The syntax for a case statement in MATLAB starts with the `switch` keyword, followed by the expression to be evaluated, and includes multiple `case` definitions as well as an optional `otherwise` block. Here’s a general structure of the case statement:
switch expression
case value1
% Code to execute when expression equals value1
case value2
% Code to execute when expression equals value2
otherwise
% Code to execute if no cases are matched
end
Breakdown of Each Component
- switch: This keyword initiates the case statement, indicating the start of the control structure.
- expression: The expression that will be evaluated. The value of this expression is compared against each case.
- case: Each `case` represents a condition to be matched. If the expression matches the case value, the corresponding block of code is executed.
- otherwise: An optional block that executes code when none of the cases match the expression. This acts as a default response.
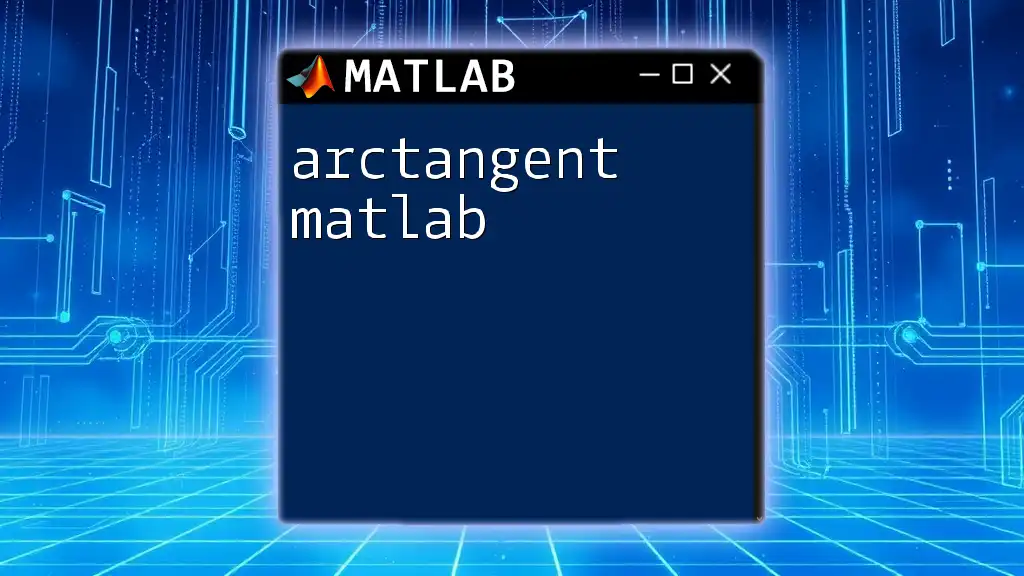
Examples of Case Statements
Simple Example
Consider a basic example where we evaluate a string representing the day of the week. This use case displays how your code can execute different commands based on the day's name:
day = 'Wednesday';
switch day
case 'Monday'
disp('Start of the week');
case 'Wednesday'
disp('Midweek');
case 'Friday'
disp('End of the work week');
otherwise
disp('Just another day');
end
In the above example, since the variable `day` is set to `'Wednesday'`, the output will be "Midweek". This showcases how efficiently a case statement can direct program flow based on variable value.
Example with Numeric Values
Case statements are not limited to strings; they also work beautifully with numeric values. Below is an example that categorizes grades based on score ranges:
grade = 87;
switch true
case grade >= 90
disp('Grade: A');
case grade >= 80
disp('Grade: B');
case grade >= 70
disp('Grade: C');
otherwise
disp('Grade: F');
end
In this example, the output will be "Grade: B" as the expression evaluates the conditions sequentially until it finds a match. This demonstrates the flexibility of the `case statement matlab` for various data types.
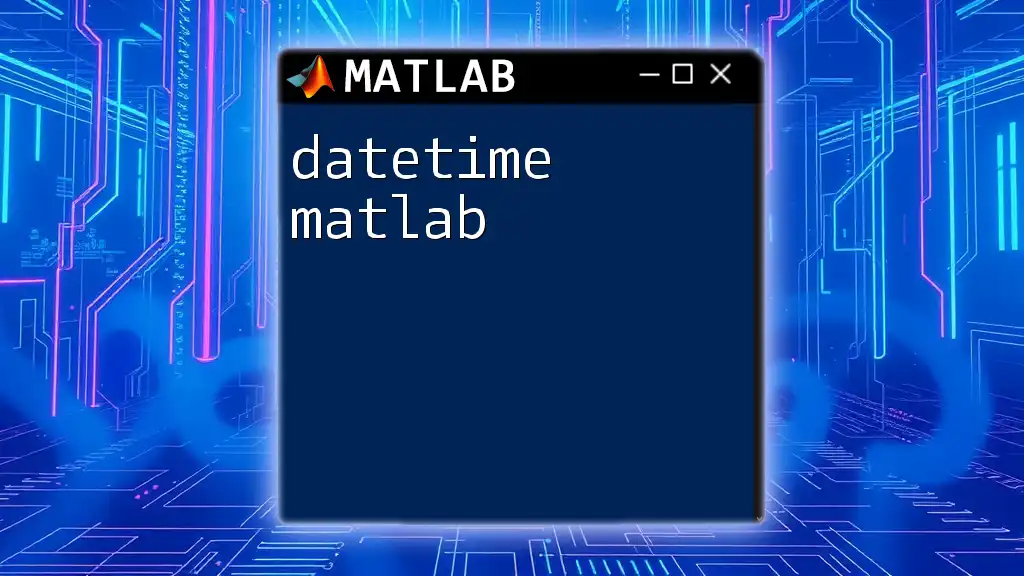
Nested Case Statements
Controlling Complex Logic
When the logic required becomes more intricate, you may need to nest case statements. This enables handling multiple variables efficiently. The following code showcases how to nest case statements:
fruit = 'Apple';
color = 'Red';
switch fruit
case 'Apple'
switch color
case 'Red'
disp('You have a Red Apple');
case 'Green'
disp('You have a Green Apple');
end
case 'Banana'
disp('You have a Banana');
end
Here, the outer `switch` checks the type of fruit, and the inner `switch` further examines the color. This approach allows for detailed control over the logic, providing specific outputs based on combined conditions.
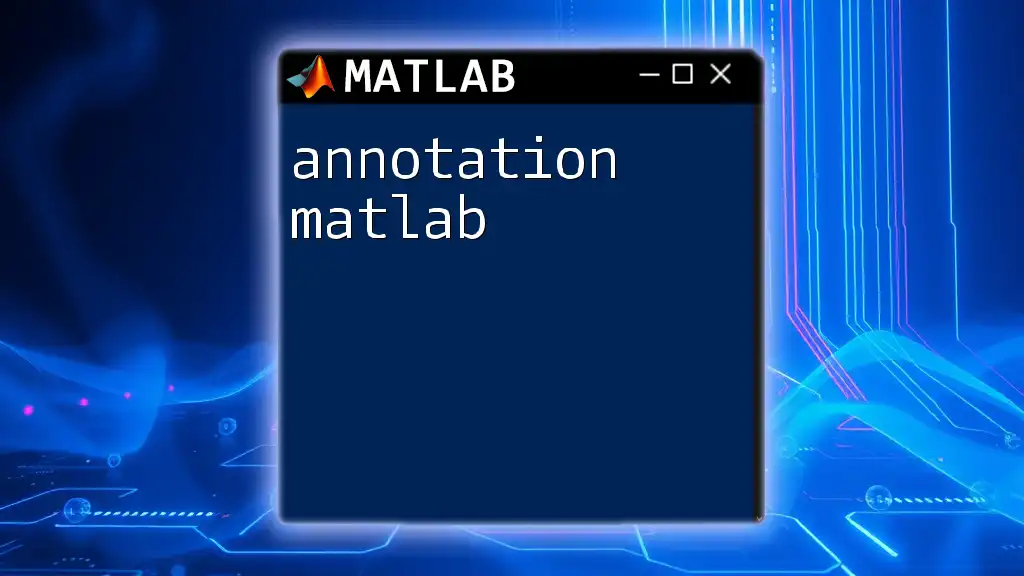
Best Practices for Using Case Statements
Clarity and Readability
To ensure your code is maintainable and easy to follow, clarity should always be a priority. Organize your case statements logically; ensure that each case is well-defined. Use comments judiciously to explain the purpose of each case.
Efficiency Considerations
With efficiency in mind, leveraging a case statement can often reduce the execution time compared to a series of if-else statements—especially when matching against numerous conditions. Evaluate your specific use case to determine which structure best supports your coding goals.
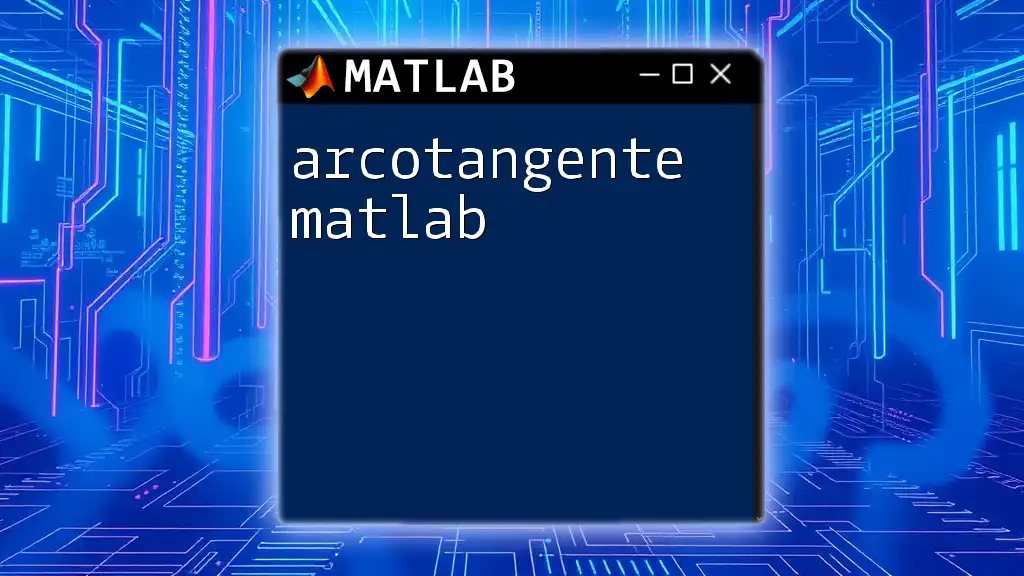
Common Pitfalls to Avoid
Forgetting the Otherwise Clause
One common oversight is neglecting to include the `otherwise` clause. This omission can lead to unexpected behaviors, where the program fails to handle cases that do not match any specified conditions.
Case Sensitivity
MATLAB is case-sensitive when evaluating strings in case statements. Mismatched casing between the case value and the actual data will result in a failure to match. Always be consistent with your capitalization.
Relying on Case Statements for Complex Conditions
Overestimating the capability of case statements for deeply nested and complex conditions can lead to confusion. If you find yourself attempting to handle multifaceted logic through case statements, consider developing a separate function or using other constructs.
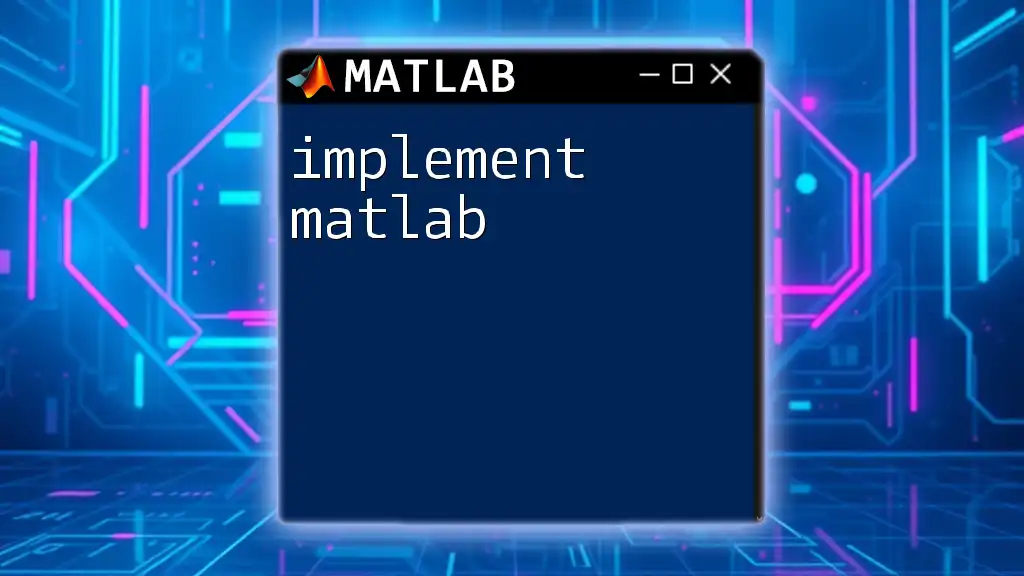
Conclusion
Understanding the mechanics of a case statement in MATLAB opens up a world of possibilities for more organized and efficient coding. By mastering this control structure, you can refine your coding skills, making your scripts easier to read and maintain while increasing their functionality. Practice with varied examples, and don't hesitate to experiment with this valuable tool in your MATLAB projects. Explore additional features of MATLAB to elevate your programming prowess!