In MATLAB, an "if statement" allows you to execute a block of code conditionally, depending on whether a specified logical expression evaluates to true.
Here’s a simple example demonstrating an if statement in MATLAB:
x = 5;
if x > 0
disp('x is positive');
end
Understanding If Statements in MATLAB
What is an If Statement?
An if statement is a fundamental component of programming that enables decision-making capabilities. In MATLAB, an if statement examines specific conditions, allowing the program to execute different code segments depending on whether those conditions are met. This functionality is crucial for creating dynamic scripts that respond to varying inputs and states.
Syntax of If Statements
The basic structure of an if statement in MATLAB follows this syntax:
if condition
% Code to execute if condition is true
end
Within the above syntax:
- condition is a logical expression evaluated to either true (1) or false (0).
- The code block inside the if statement executes only when the condition evaluates to true.
- The statement concludes with the `end` keyword, which signifies the termination of the if block.

Types of If Statements in MATLAB
Simple If Statement
The simplest form of an if statement evaluates a single condition.
Example:
x = 10;
if x > 5
disp('x is greater than 5');
end
In this example, the variable `x` is evaluated, and since it is greater than 5, the message "x is greater than 5" is displayed. Thus, if statements enable the program to handle multiple scenarios based on conditional evaluations.
If-Else Statement
Incorporating an else statement enhances the decision-making capabilities of your code by providing an alternative execution path when the primary condition is not met.
Example:
x = 3;
if x > 5
disp('x is greater than 5');
else
disp('x is not greater than 5');
end
Here, since `x` does not satisfy the condition (`x > 5`), the code inside the else block executes, displaying "x is not greater than 5." This structure is valuable for managing binary decisions in your programming logic.
If-Else If-Else Statement
When you need to evaluate multiple conditions, the if-else if-else structure is ideal. It allows you to specify various pathways of code execution.
Example:
x = 0;
if x > 0
disp('x is positive');
elseif x < 0
disp('x is negative');
else
disp('x is zero');
end
In this scenario, the first condition checks if `x` is positive. If that fails, it checks if `x` is negative. Finally, if neither condition holds, it defaults to showing that `x` is zero. This layered approach accommodates more complex decision-making processes in scripting.

Nested If Statements
What are Nested If Statements?
Nested if statements allow programmers to place one or more if statements inside another if statement. This structure is particularly useful when additional checks are needed based on the result of a previous condition.
Example of a Nested If Statement
x = 10;
y = 5;
if x > 5
if y > 3
disp('Both conditions are met');
end
end
In this example, if `x` is greater than 5, the program then checks if `y` is greater than 3. Since both conditions are true, the output will be "Both conditions are met." Nesting increases code flexibility and logic depth, but it’s essential to maintain clear indentation for readability.
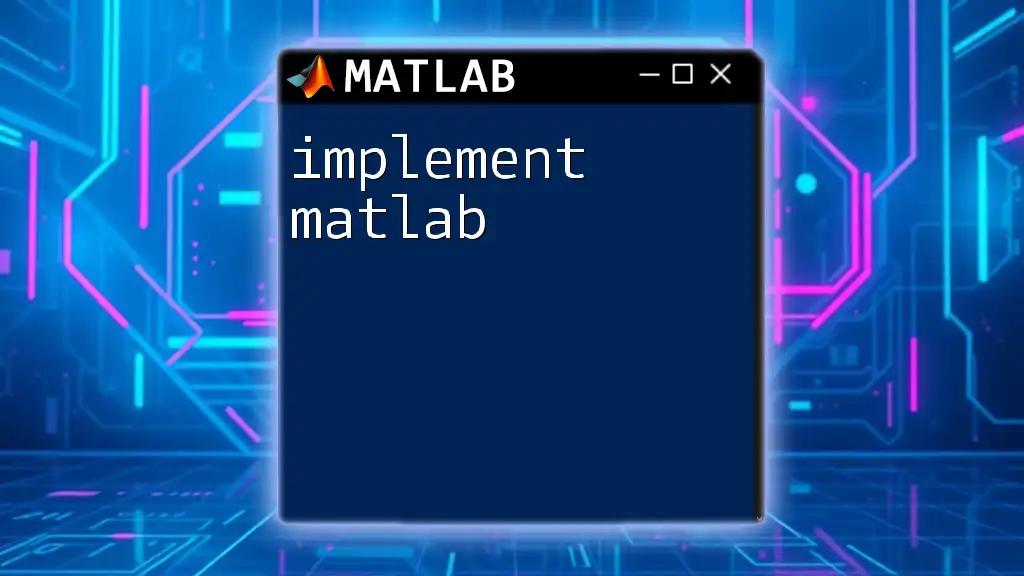
Best Practices for Using If Statements
Keep Conditions Simple
When writing if statements, aim to keep your conditions simple and straightforward. Overly complex logical conditions can lead to confusion and bugs. For instance, preferring a clear check like `if x > 5` instead of `if (x > 5 && y < 10)` promotes better readability.
Use Clear and Descriptive Variable Names
Utilizing clear variable names significantly improves code comprehension. Instead of vague names like `a` or `b`, use meaningful identifiers such as `temperature` or `speed` to enhance understanding. When others (or yourself in the future) read the code, it will be clearer how conditions are evaluated.
Commenting Your Code
Adding comments can be immensely beneficial. Comments clarify the purpose of specific conditions and code blocks, making maintenance and updates more manageable. For example:
% Check if the number is positive
if x > 0
disp('Number is positive');
end
Such annotations help provide context and streamline collaborative work or future debugging.
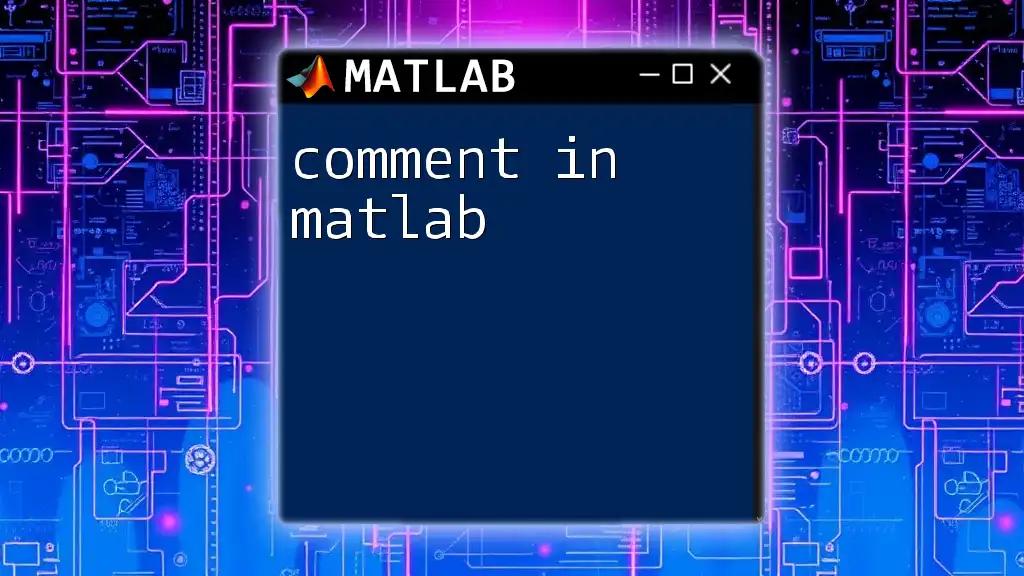
Common Mistakes to Avoid
Forgetting to Terminate with end
A frequent issue among beginners is neglecting the use of the `end` keyword. Omitting it can cause syntax errors or unexpected behavior in the program execution. Always ensure that each if statement block is concluded properly with `end`.
Using Assignment Instead of Comparison
One common mistake is confusing assignment with comparison. For instance, using an assignment operator `=` instead of a comparison operator `==` in conditions:
if x = 10 % This is incorrect
Instead, it should be:
if x == 10 % This is correct
This simple error can lead to logical bugs that are often hard to trace, so pay close attention when writing Conditional statements!
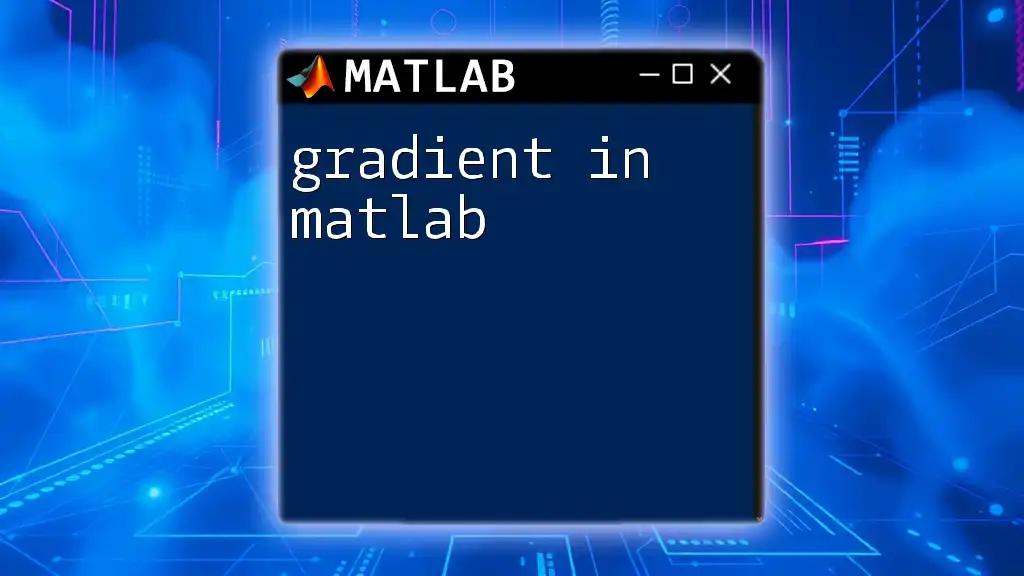
Troubleshooting If Statements in MATLAB
Debugging Tips
When experiencing issues with if statements, MATLAB’s debugging capabilities can be invaluable. You can set breakpoints and inspect variable states before reaching an if statement, allowing you to investigate how conditions are evaluated.
Logical Errors
Identifying logical errors—where the program does not perform as expected due to the condition evaluation—is critical. To debug, print relevant variable values before the if statement to ensure they hold the anticipated values. This strategy should help to clarify why certain branches were executed.
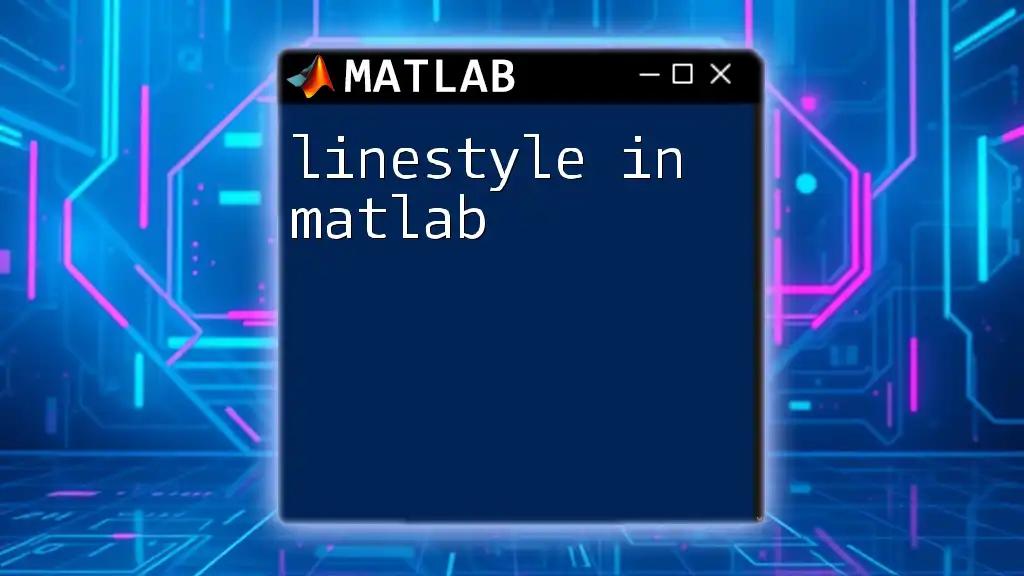
Conclusion
If statements are pivotal to managing control flow in MATLAB programming. They offer developers the ability to create dynamic, responsive scripts that can adapt based on varying inputs and conditions. Mastering the use of if statements can enhance your programming skills significantly.
Call to Action
Join our MATLAB learning community today for more insights, tips, and tricks to elevate your MATLAB programming proficiency!