The `readtable` function in MATLAB is used to import data from a spreadsheet or text file into a table format, making it easy to analyze and manipulate the data.
data = readtable('filename.csv');
What is readtable?
The `readtable` function in MATLAB serves a crucial role in data analysis, enabling users to efficiently read and import tabular data from various file formats. This versatile function enables researchers, data scientists, and engineers to quickly integrate datasets into their MATLAB environment, facilitating immediate analysis and visualization.
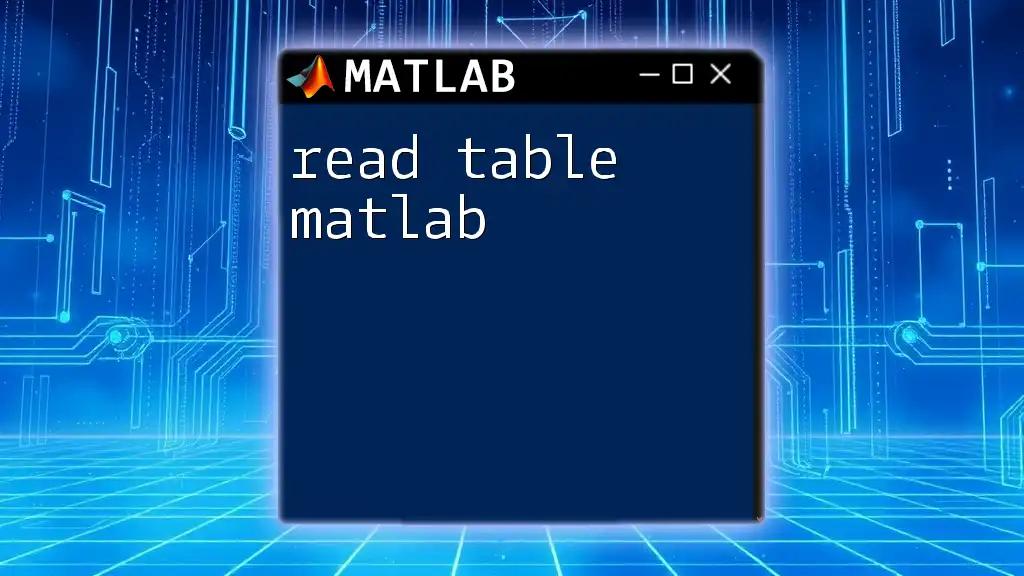
Supported File Formats
`readtable` is remarkably flexible, allowing you to read data from several common file formats. Understanding these formats can help streamline your data import process:
- CSV Files: Comma-Separated Values files are one of the most popular and straightforward formats for data storage. They are easily created and read.
- Excel Files: The function can read Excel spreadsheets, making it convenient for users who often work with this format.
- Text Files: `readtable` can handle text files with fixed-width or delimited formats, allowing for versatile data handling.
- Other Formats: Depending on the version of MATLAB, additional formats may also be supported.
Knowing the capabilities of `readtable` allows for optimal choices regarding the data formats best suited for your analysis.
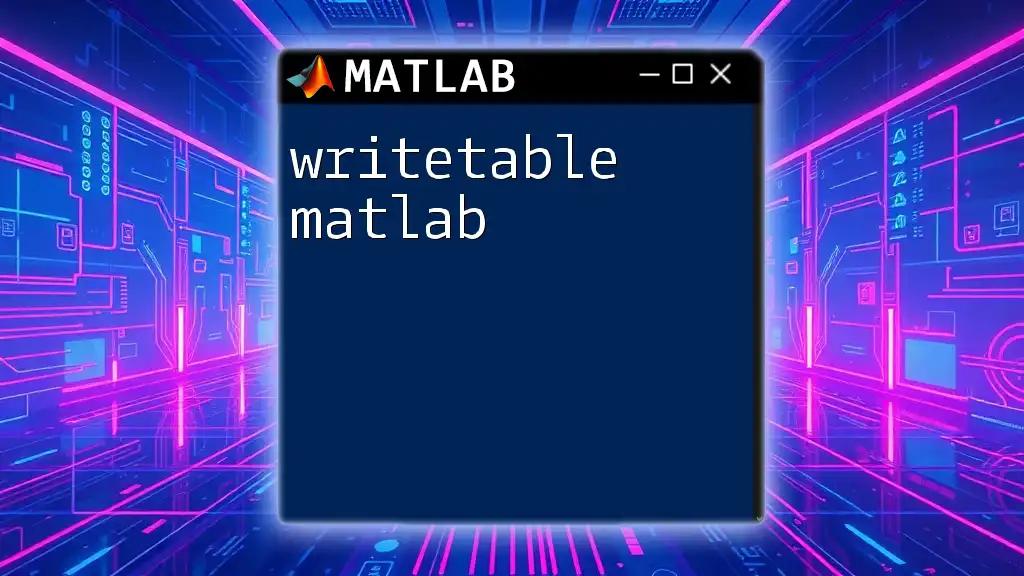
Basic Syntax of readtable
To utilize `readtable`, the basic syntax is as follows:
T = readtable(filename)
In this command:
- filename is the name of the file you want to read. It can include file extensions.
- T is the output table that contains the imported data, structured in a way that is easy to manipulate and analyze.
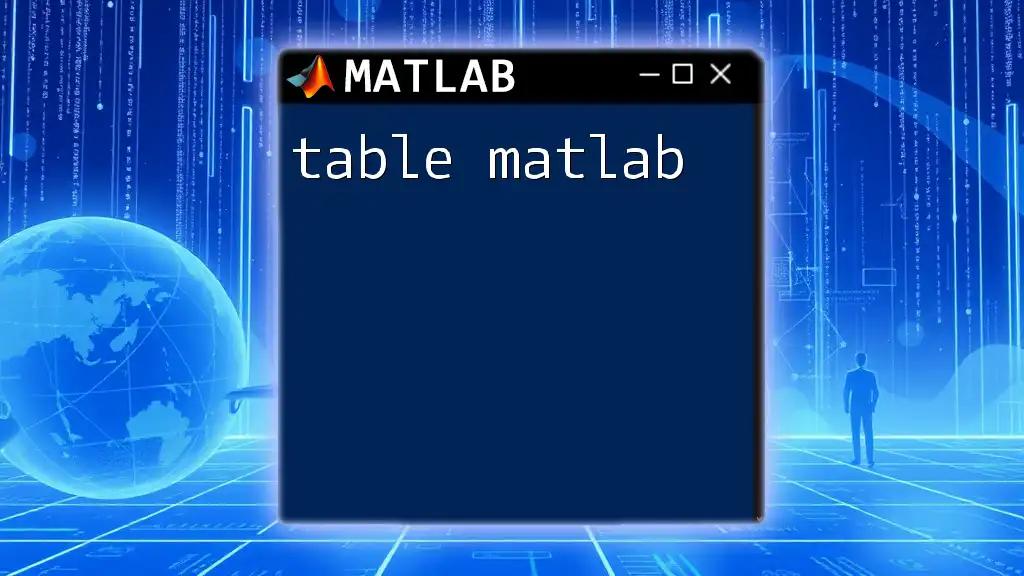
Reading Different File Types
Reading CSV Files
Reading a CSV file is particularly straightforward with `readtable`. For instance, if you have a file called `data.csv`, you would use the command:
T = readtable('data.csv');
This command imports the CSV file into MATLAB as a table, allowing you to access the data through variable names.
CSV files are widely used for data exchanges, making them a common choice for both personal and professional datasets. When working with them, ensure your separator is correct; `readtable` defaults to commas but can be customized.
Reading Excel Files
Excel files are an integral part of many data workflows. To read an Excel file, such as `data.xlsx`, you use:
T = readtable('data.xlsx');
For more advanced scenarios such as specifying which worksheet to read, you can do so with:
T = readtable('data.xlsx', 'Sheet', 'Sheet1');
Specifying the sheet name or even a range enhances your control over the data being imported, ensuring you retrieve the exact information necessary for your analysis.
Reading Text Files
To read data from text files, especially when they have specific delimiter requirements, `readtable` shines. Suppose you have a tab-delimited text file named `data.txt`. The code would look like this:
T = readtable('data.txt', 'Delimiter', '\t');
This flexibility allows you to work with data formatted in various ways, from spaces to tabs or even custom delimiters.
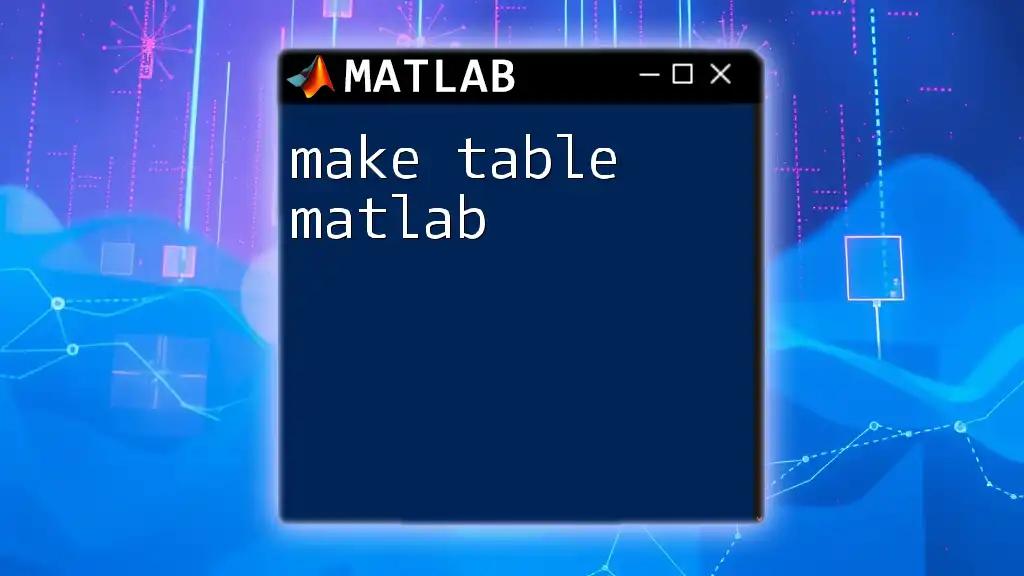
Advanced Options and Customization
Specifying Variable Names
A fundamental feature of `readtable` is the ability to specify variable names. This can enhance readability in your code:
T = readtable('data.csv', 'VariableNames', {'Var1', 'Var2'});
By using this option, you can assign meaningful names to your variables, facilitating easier data manipulation and interpretation later on.
Handling Missing Data
In real-world datasets, missing data is a common issue. With `readtable`, you can handle it effectively by using the 'TreatAsEmpty' option:
T = readtable('data.csv', 'TreatAsEmpty', {'NA', 'NULL'});
This command allows you to specify which strings should be treated as empty values, leading to cleaner data and more accurate analyses.
Selecting and Excluding Variables
If you only need certain columns from a dataset, `readtable` allows you to select specific variables:
T = readtable('data.csv', 'VariableNamingRule', 'preserve', 'ReadVariableNames', true);
By customizing which variables to read, you avoid cluttering your workspace with unnecessary data, making it easier to focus on what truly matters for your analysis.
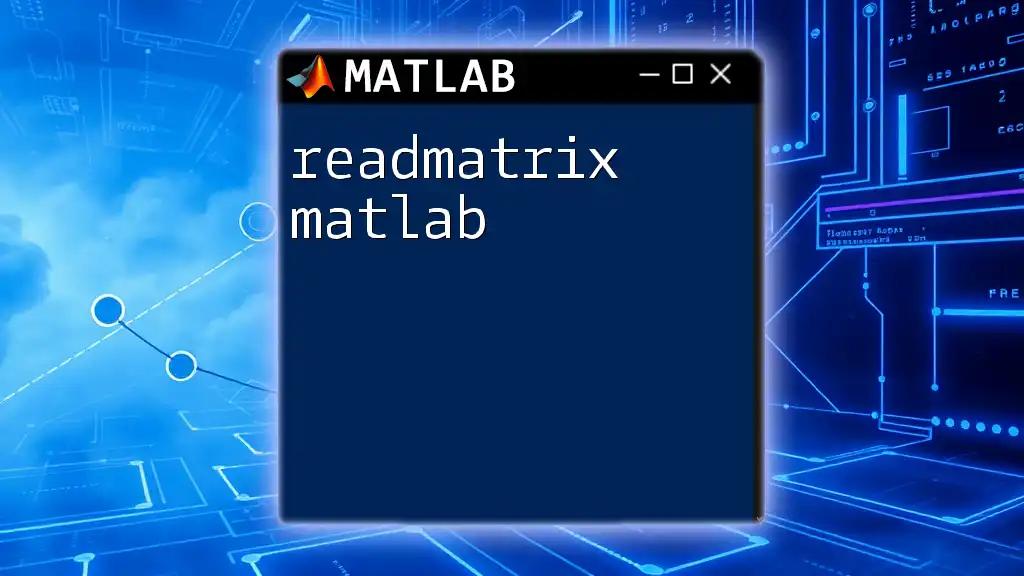
Working with the Imported Table
Exploring the Table
Once the data is imported, it's essential to explore it to understand its structure. You can use several commands to inspect your table:
-
The head() function displays the first few rows of the table, providing a quick overview:
head(T)
-
The summary() function offers a detailed summary, including variable types and counts of missing values:
summary(T)
These functions are invaluable for initial data assessments, helping you identify potential issues or anomalies in your dataset.
Accessing Table Data
Accessing specific data within the table can be done using the column names. For instance, to retrieve data from a variable named `Var1`, you can execute:
data_column = T.Var1; % Accessing a specific variable
This method is intuitive and allows for straightforward data manipulation, making it easier to perform calculations or analysis on specific columns.
Modifying Tables
After exploring the table, you might want to modify it by adding new variables. You can create a new variable based on existing ones:
T.NewVar = T.Var1 + T.Var2; % Creating a new variable
This capability opens the door to complex data manipulations, enabling you to refine your analyses based on newly calculated data.
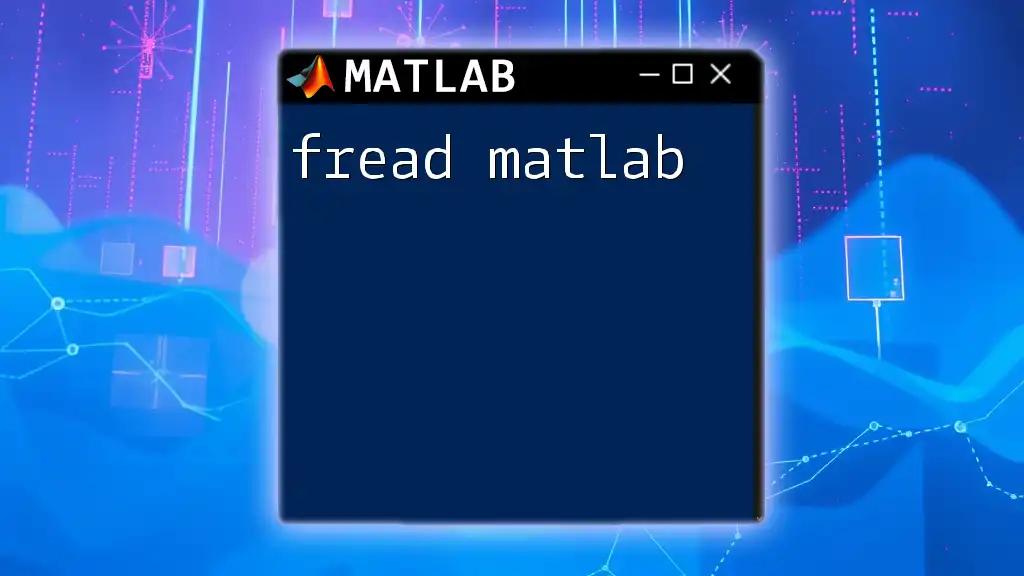
Common Issues and Troubleshooting
While using `readtable`, users may encounter familiar hurdles, such as:
- Incorrect file paths or missing files, leading to file errors.
- Formatting issues, especially with delimiters, resulting in improper data reads.
When facing these challenges, ensure paths are correctly specified, and data formats align with the function's expectations. Checking MATLAB's documentation can also provide guidance on specific errors.
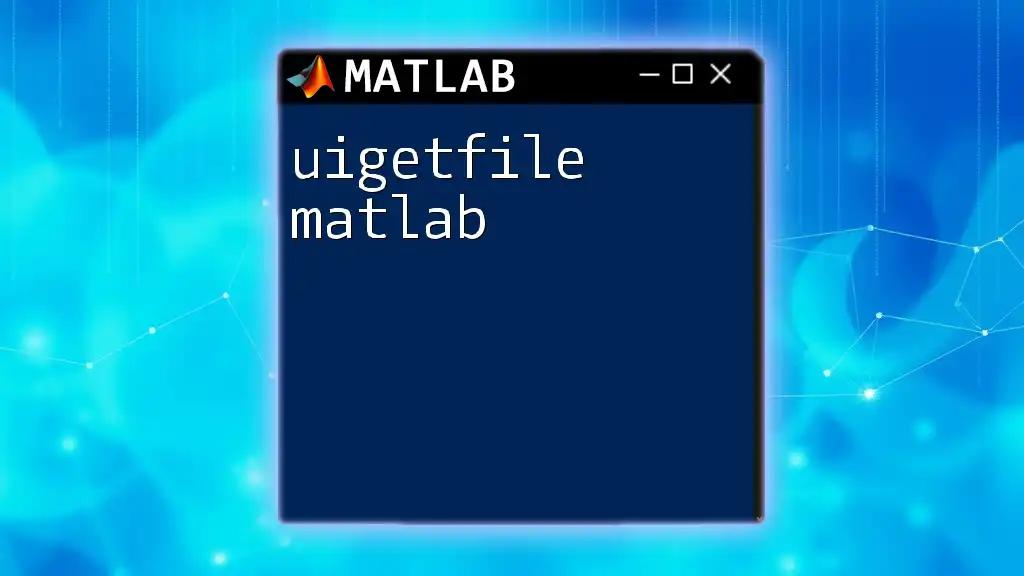
Conclusion
The `readtable` function in MATLAB is an indispensable tool for importing tabular data, simplifying the data acquisition process significantly. By leveraging its capabilities, you can streamline your data analysis tasks and focus on insights rather than data preparation.
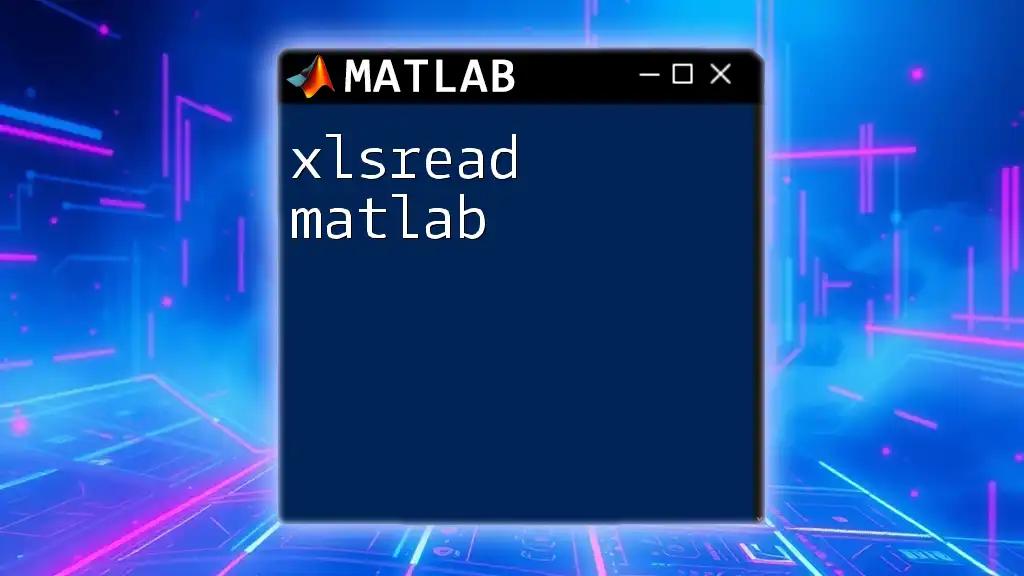
Additional Resources
For further learning, refer to the MATLAB documentation for `readtable` for detailed specifications. You may also explore tutorials or practical exercises to reinforce these concepts and strengthen your MATLAB skills.
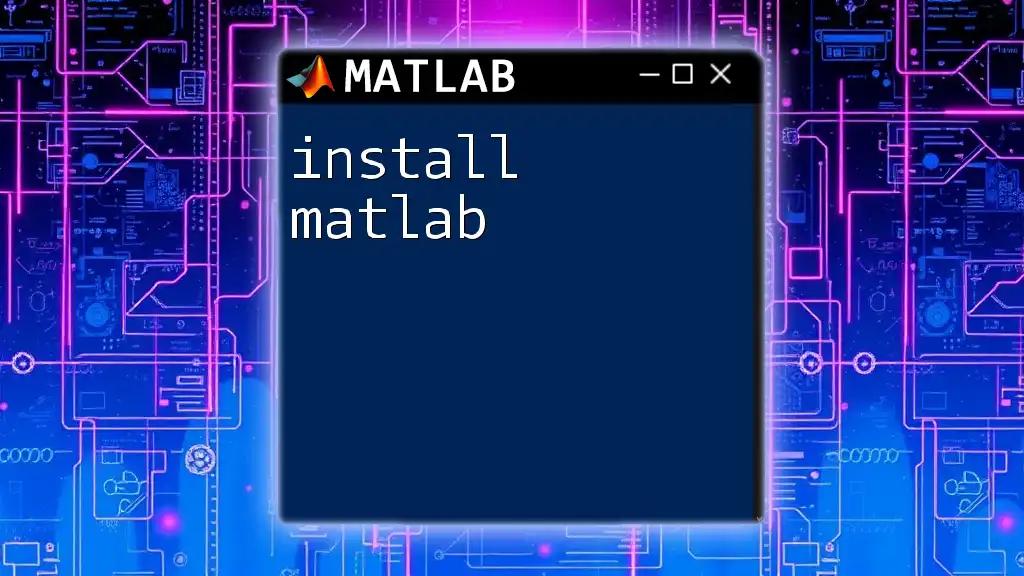
Call to Action
If you're eager to deepen your understanding of MATLAB commands and enhance your data analysis capabilities, consider signing up for our specialized training classes. Additionally, don't miss the opportunity to download our cheat sheet on using `readtable`, which provides quick references and handy tips for mastering this essential function.