The `scatter3` function in MATLAB creates a 3D scatter plot using specified x, y, and z coordinates to help visualize data points in three-dimensional space.
Here's a simple code snippet demonstrating its usage:
% Example of using scatter3 in MATLAB
x = rand(1, 100); % Generate random x-coordinates
y = rand(1, 100); % Generate random y-coordinates
z = rand(1, 100); % Generate random z-coordinates
scatter3(x, y, z, 'filled'); % Create a filled 3D scatter plot
xlabel('X-axis');
ylabel('Y-axis');
zlabel('Z-axis');
title('3D Scatter Plot Example');
grid on;
What is Scatter3?
Scatter3 in MATLAB is a powerful command used to create three-dimensional scatter plots. These plots visualize data points in a 3D space, making it easier to observe relationships between three different variables. The ability to represent complex datasets graphically is crucial in various fields, from science to engineering, as it enhances data interpretation and analysis.
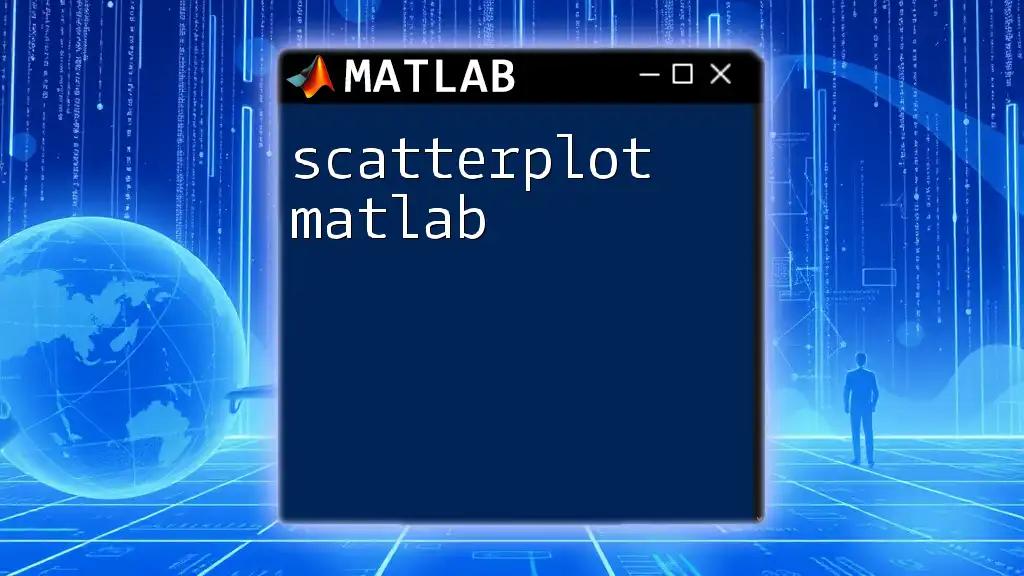
Why Use Scatter3 in MATLAB?
Utilizing scatter3 matlab becomes essential for several reasons:
- Data Visualization: This command allows for a clear presentation of multi-dimensional data, making it easier to spot trends and outliers.
- Interactivity: MATLAB provides interactive features that allow users to rotate, zoom, and pan the 3D plots for a better understanding of the data.
- Versatility: Whether you’re analyzing geographical data, performance metrics, or statistical models, scatter3 can accommodate various types of datasets.
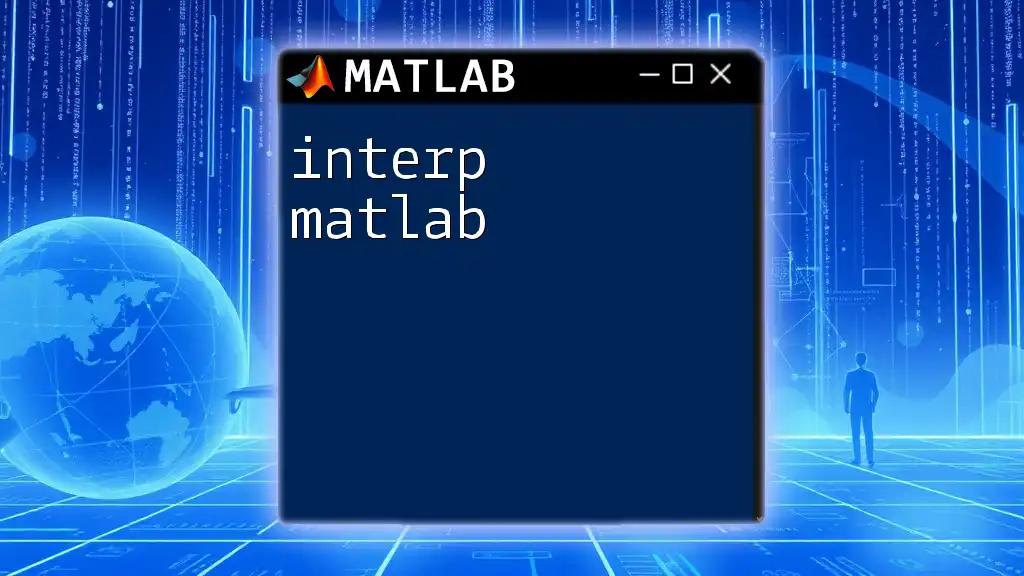
Getting Started with Scatter3
Basic Syntax of Scatter3
Understanding the basic syntax of the scatter3 command is fundamental. The primary structure follows this format:
scatter3(X, Y, Z)
Where:
- X, Y, and Z are vectors containing the coordinates of the data points in three-dimensional space.
- Optional parameters include S (marker sizes) and C (marker colors) for enhanced visualization.
Creating Your First 3D Scatter Plot
Creating your first 3D scatter plot is straightforward. Here’s how to do it step-by-step:
- Generate random datasets for X, Y, and Z coordinates.
- Use the scatter3 command to visualize these points.
For example:
X = rand(1, 100);
Y = rand(1, 100);
Z = rand(1, 100);
scatter3(X, Y, Z, 'filled');
grid on;
title('My First 3D Scatter Plot');
xlabel('X-axis');
ylabel('Y-axis');
zlabel('Z-axis');
In this code snippet, we’ve generated three vectors of random points, created the scatter plot, and labeled the axes. This simple example shows how easy it is to get started with scatter3 matlab.
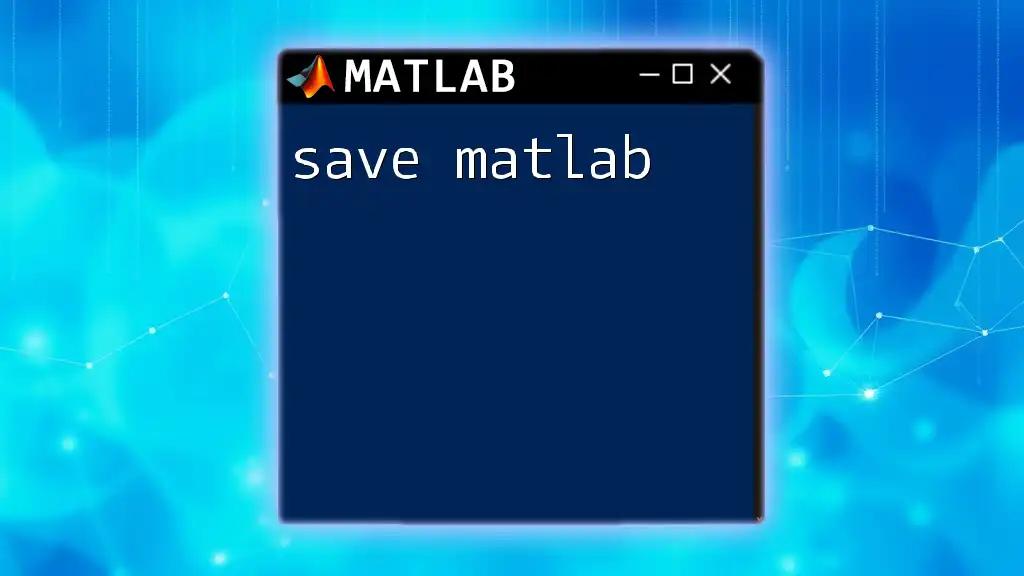
Customizing Your 3D Scatter Plot
Modifying Marker Sizes
One way to enhance your scatter plot is by modifying the marker sizes. The S parameter dictates how large the markers appear on the plot. You might want to represent additional data through marker sizes. For instance:
S = rand(1, 100) * 100; % variable sizes
scatter3(X, Y, Z, S, 'filled');
In this example, each point's size is determined by a random value, allowing for a visually varied display that can represent another variable's significance.
Changing Colors of Markers
Colors can differentiate data points based on a specific variable or category. The C parameter allows for coloring the markers, which can bring out patterns within the data. To apply random colors, consider the following code:
C = rand(1, 100);
scatter3(X, Y, Z, 50, C, 'filled');
colormap(jet); % apply a color map
Here, we’ve generated 100 random colors corresponding to each point. Applying a color map can enhance the visual representation of categorical differences.
Using Different Markers
Scatter3 matlab also allows changing the type of markers used in the visualization. The default marker is an ‘o’ (circle), but you can easily customize it. For example:
scatter3(X, Y, Z, 50, 'r', 'o'); % red circles as markers
You can replace ‘o’ with other options such as ‘*’, ‘x’, or ‘s’ (square), depending on how you wish to represent your data points.

Enhancing the Visual Appeal
Adding Titles and Labels
Ensuring your plot is well-labeled is vital for clarity. Adding titles and axis labels helps communicate what the plot represents. For example, you can apply:
title('3D Scatter Plot Example');
xlabel('X-axis label');
ylabel('Y-axis label');
zlabel('Z-axis label');
This practice enhances the viewer's understanding and provides context for the data visualized with scatter3.
Adjusting View Angles and Grid
The ability to manipulate the view angle of your scatter plot can significantly impact the interpretation of the data. You can adjust your plot’s perspective using the `view` function:
view(45, 30); % sets the view angle
grid on; % enable grid for better visualization
By setting a specific angle, you allow the audience to perceive depth more intuitively, which is particularly useful when dealing with clusters of data.
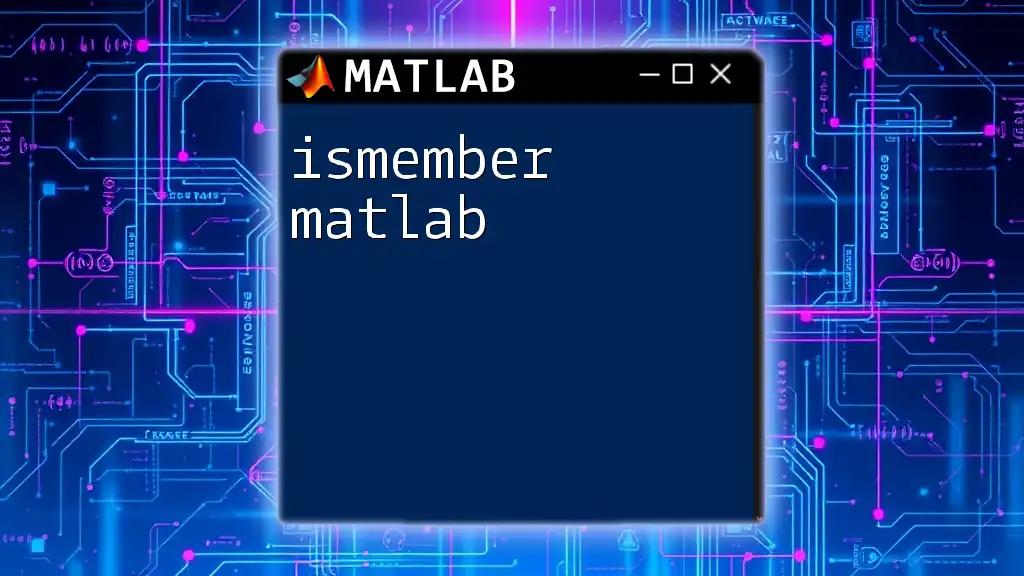
Advanced Features of Scatter3
Incorporating Transparency
To make your plot more visually appealing and to handle overlapping data points, transparency can be crucial. You can set an alpha value to control the marker transparency:
scatter3(X, Y, Z, 50, C, 'filled', 'MarkerEdgeColor', 'k', 'MarkerFaceAlpha', 0.5);
In this example, the markers are colored but appear semi-transparent, allowing for better visualization of overlapping points.
Grouping Data Points
Often, data can be categorized into groups that reveal trends and patterns. You can assign colors based on these categories. Here’s a simple approach:
G = randi([1, 3], 1, 100); % random group assignments
scatter3(X, Y, Z, 50, G, 'filled');
In this scenario, each point is randomly assigned to one of three groups, color-coded based on its affiliation. This way, you can highlight differences or similarities between various data points.
Integrating with Other MATLAB Functions
You can overlay multiple elements in a scatter plot using the `hold on` command. This functionality allows you to integrate different types of visualizations into one plot. For example:
scatter3(X, Y, Z, 50, 'b', 'filled');
hold on;
plot3(X, Y, Z, 'k-'); % add a line over the scatter plot
In this code, the scatter plot is complemented by a connecting line across the points, providing an additional layer of analysis.
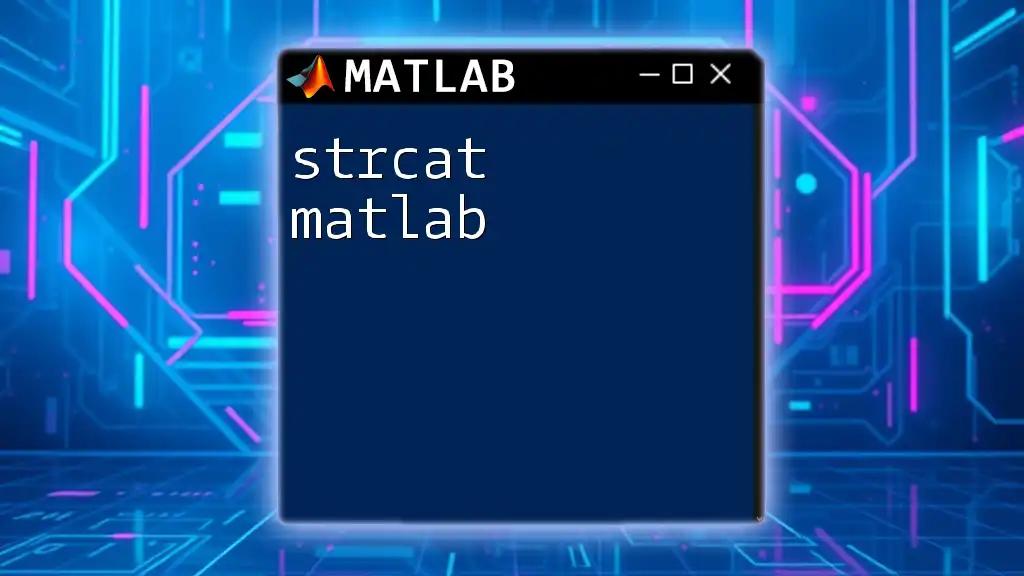
Tips for Effective 3D Scatter Plots
When creating 3D scatter plots, consider these key tips:
-
Choosing the Right Dataset: Ensure that the data you choose to visualize is suitable for a three-dimensional representation. Look for datasets that can effectively convey relationships among three different variables.
-
Common Mistakes to Avoid: Watch out for overcrowded scatter plots, where points overlap significantly. It can mislead viewers about data distribution. Similarly, unclear labels can result in misinterpretation.
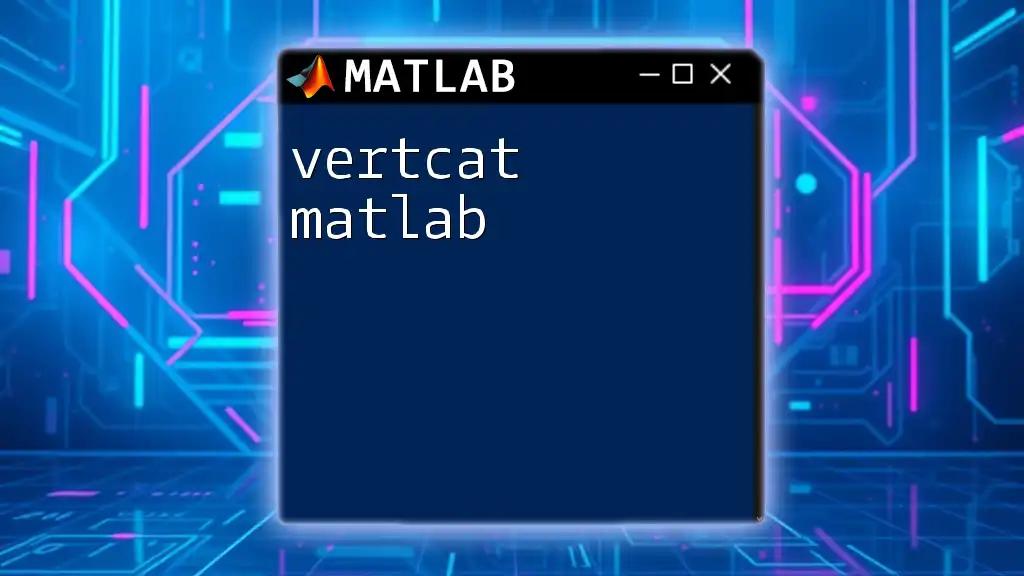
Conclusion
In conclusion, mastering scatter3 matlab opens up a plethora of opportunities for data visualization. By understanding its syntax, customization options, and advanced features, you can effectively communicate your data’s narrative. Remember, practice is key! Regularly experimenting with different datasets and customization techniques will deepen your understanding of this powerful tool.
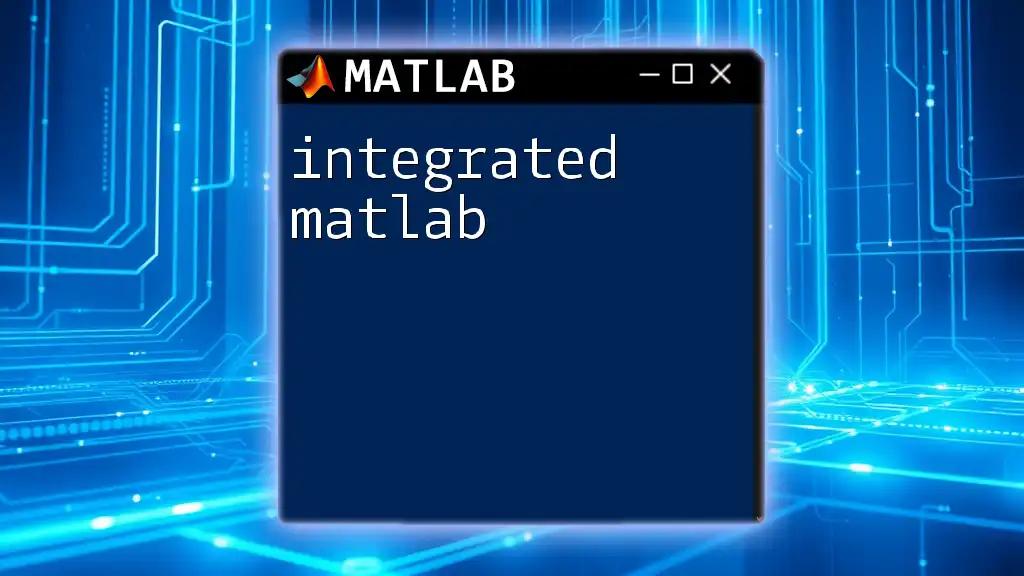
Additional Resources
For further exploration beyond this guide, consider checking out the following resources:
- Online MATLAB Documentation: The official documentation is a great place for in-depth study and advanced usage scenarios.
- Tutorials and Courses on MATLAB Visualization: Many platforms provide structured learning paths on enhancing visualization skills in MATLAB.
- Community Forums and Help: Engaging in forums can provide real-world insights and solutions to common challenges.

Call to Action
Join our community today! Share your experiences, ask questions, and learn from others as you delve into the world of MATLAB and data visualization. Don't miss out on our upcoming workshops focused on mastering MATLAB commands, including scatter3 and beyond!