In MATLAB, you can create a table to organize and display data using the `table` function, allowing for better structure and enhanced readability of datasets.
Here is a code snippet demonstrating how to create a simple table:
% Define sample data
Names = {'Alice'; 'Bob'; 'Charlie'};
Ages = [24; 30; 28];
Scores = [85; 90; 88];
% Create a table
T = table(Names, Ages, Scores);
% Display the table
disp(T);
Understanding Tables in MATLAB
What is a Table?
A table in MATLAB is a data type designed specifically for storing column-oriented or tabular data. Tables can contain different types of data across each column, making them a flexible option for complex datasets.
Tables differ from arrays and cell arrays by allowing for more organized data management, with each column able to hold different data types (e.g., numbers, strings). This coarse-grained separation makes it easier to understand and manipulate data.
Advantages of Using Tables:
- Supports mixed data types across columns.
- Utilizes variable names for easy referencing.
- Provides built-in functions for sorting, filtering, and plotting.
Use Cases for Tables
Tables are particularly beneficial when working on data analysis, visualization, or statistical modeling tasks. They facilitate operations like filtering datasets, running group operations, and preparing data for machine learning algorithms.
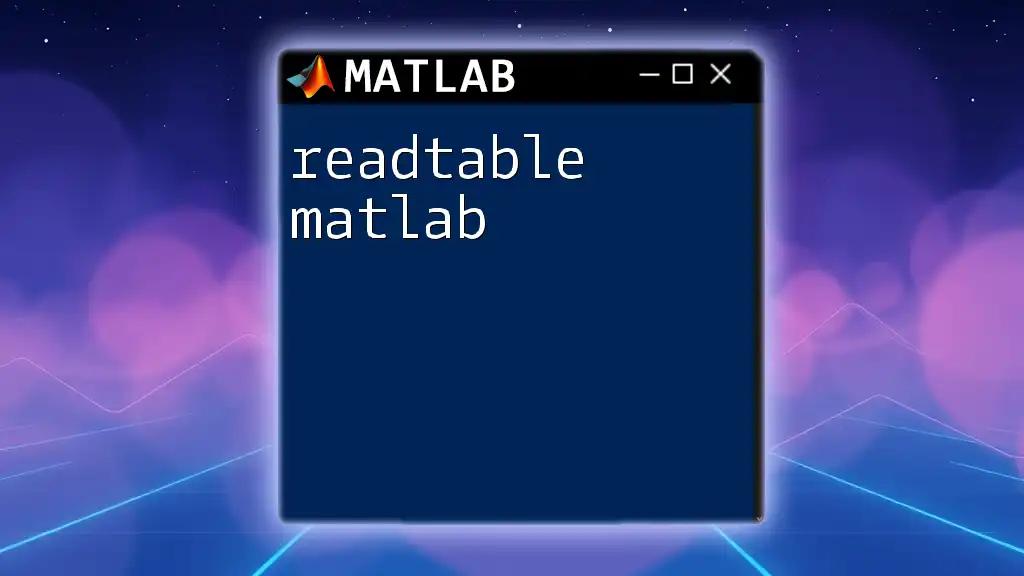
Creating Tables in MATLAB
Using the `table()` Function
To create a table in MATLAB, you will use the `table()` function. This function allows you to combine multiple variables into one table structure.
Basic Syntax:
T = table(var1, var2, ..., PropertyName1,PropertyValue1,...)
Input Parameters:
- `var1, var2, ...`: Column data.
- `PropertyName1, PropertyValue1`: To specify names and types for your columns.
Example:
Names = {'Alice'; 'Bob'; 'Charlie'};
Ages = [25; 30; 35];
tableExample = table(Names, Ages);
This code creates a table named `tableExample` with two columns, "Names" and "Ages", displaying names and their respective ages.
Creating Tables from Existing Data Arrays
You can convert existing arrays into tables using the `table()` function.
Example:
A = [1, 2; 3, 4];
B = ["X", "Y"; "Z", "W"];
newTable = table(A, B);
This creates a table named `newTable` with two columns, where column A holds numerical data and column B holds strings.
Importing Data into Tables
Often, you'll have datasets saved in files. MATLAB makes it easy to import data directly into tables using the `readtable()` function.
Example:
myData = readtable('datafile.csv');
This command reads a CSV file named `datafile.csv` and imports it as a table named `myData`.
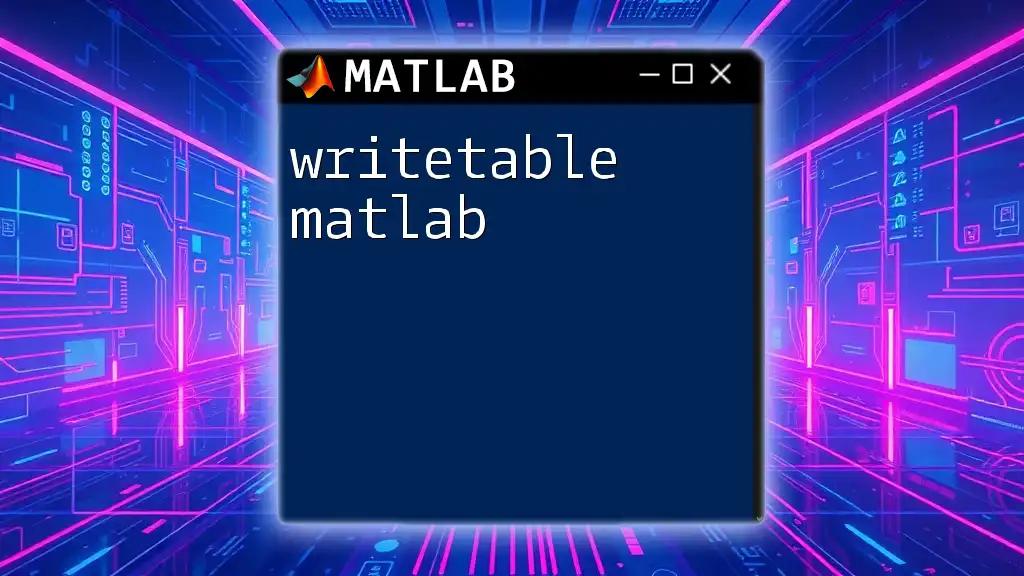
Accessing and Modifying Table Data
Accessing Table Data
Accessing data in a table follows a straightforward indexing approach. You can access rows and columns using parentheses.
Example:
firstRow = tableExample(1, :);
selectedColumn = tableExample.Ages;
Here, `firstRow` contains data from the first row of the table, while `selectedColumn` retrieves all values under the "Ages" column.
Modifying Table Data
Once your data is in a table, you may find that you need to change or add data.
Adding New Rows and Columns: To add a new column, simply assign a new variable name:
Example:
tableExample.NewColumn = [1; 2; 3];
This adds a new column called "NewColumn" to `tableExample`.
Renaming Table Variables: You can rename existing columns using the `Properties.VariableNames` property.
Example:
tableExample.Properties.VariableNames{'NewColumn'} = 'Score';
In this code, we rename "NewColumn" to "Score".
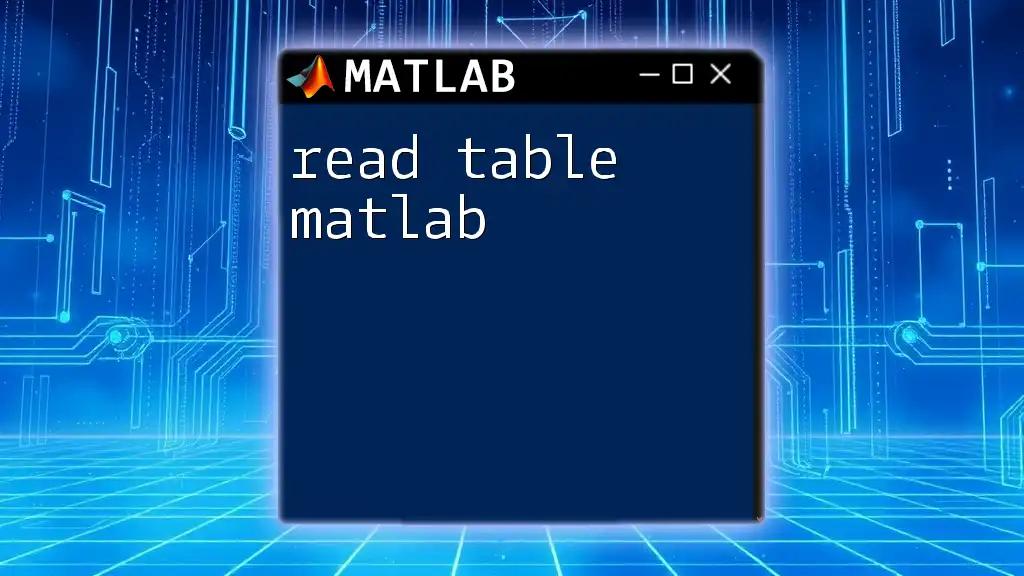
Sorting and Summarizing Table Data
Sorting Tables
Sorting data in tables can be done using the `sortrows()` function. This is particularly handy for understanding trends or organizing data.
Example:
sortedTable = sortrows(tableExample, 'Ages');
This code sorts the table by the "Ages" column in ascending order.
Summarizing Tables
For data aggregation, the `groupsummary()` function is effective. It allows you to group data and compute summary statistics.
Example:
summarizedData = groupsummary(tableExample, 'Ages', 'mean');
This summarizes the table by calculating the mean age, offering quick insights into the data at a glance.
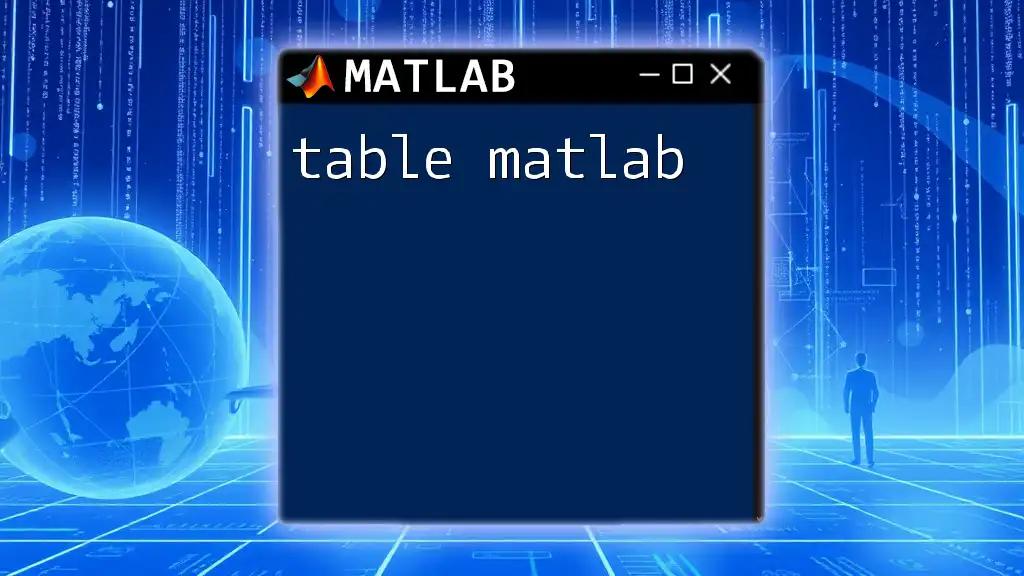
Visualizing Table Data
Creating Plots from Table Data
Once you have your data organized in a table, visualizing it can provide significant insights. You can easily plot data from a table using MATLAB’s built-in plotting functions.
Example of a Scatter Plot:
scatter(tableExample.Ages, tableExample.Score);
xlabel('Ages');
ylabel('Scores');
title('Age vs. Scores Scatter Plot');
This code generates a scatter plot where "Ages" is on the x-axis and "Scores" is on the y-axis, making relationships between variables visually accessible.
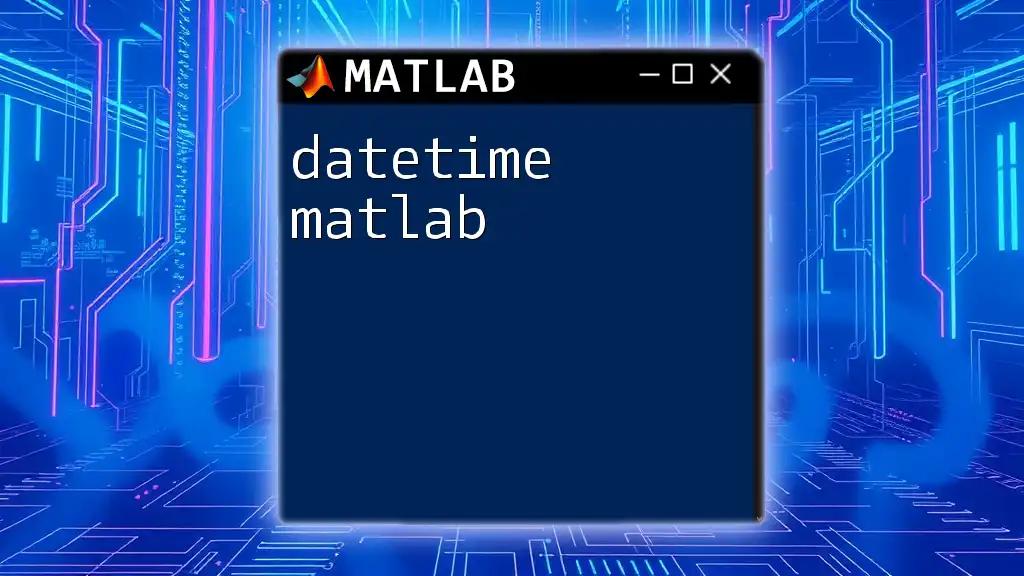
Best Practices for Using Tables in MATLAB
Documentation and Comments
Always document your tables and code thoroughly. Clear comments explaining your table structure and the purpose of your code improve readability and future usability.
Naming Conventions
Use descriptive, consistent naming conventions for your table variables. This practice enhances code understanding and minimizes errors associated with variable misuse.
Performance Considerations
While tables are powerful, be mindful of performance when dealing with very large datasets. For computationally intensive tasks, consider alternate data structures when appropriate.
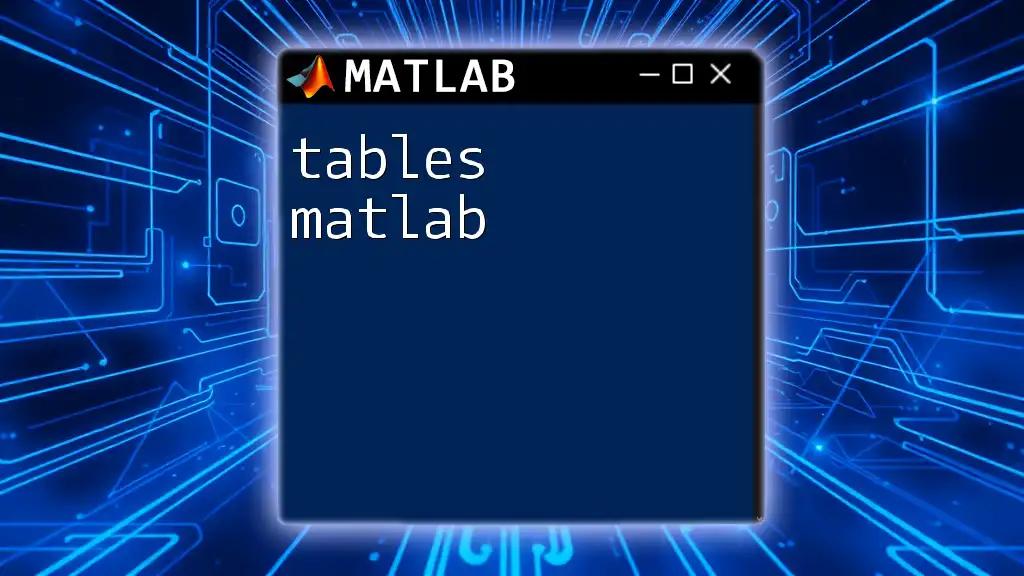
Conclusion
In this guide, we've covered how to create, manipulate, access, and visualize tables in MATLAB thoroughly. By leveraging tables, you can more effectively manage and analyze your data, making your workflow more efficient and organized.
Encourage exploration of various functions and features related to tables, and remain engaged with the MATLAB community for added insights and assistance in your data projects.