"Implementing MATLAB involves using its commands to perform various mathematical computations and data manipulations efficiently, as demonstrated in the following example that creates a simple sine wave plot."
t = 0:0.01:2*pi; % Time vector from 0 to 2*pi
y = sin(t); % Calculate sine values
plot(t, y); % Plot the sine wave
title('Sine Wave'); % Add title to the plot
xlabel('Time (seconds)'); % Label the x-axis
ylabel('Amplitude'); % Label the y-axis
What is MATLAB?
MATLAB, short for Matrix Laboratory, is a high-level programming language and interactive environment used primarily for numerical computation, visualization, and programming. Its versatility makes MATLAB a powerful tool across various fields such as engineering, finance, scientific research, and data analysis. With robust mathematical capabilities and sophisticated graphics, it allows users to prototype, analyze, and create complex algorithms efficiently.
Key Features of MATLAB
Some of the most notable features of MATLAB include:
- Matrix Manipulation: MATLAB's foundation is built on matrices, allowing effortless manipulation and mathematical operations on multidimensional arrays.
- Visualization Tools: It offers a rich set of plotting functions enabling users to create basic plots, 3D visualizations, and animations.
- Algorithm Development: Users can implement algorithms using scripting, making coding intuitive.
- Extensive Libraries: MATLAB provides access to a wide range of built-in functions and user-contributed toolboxes to enhance functionality.
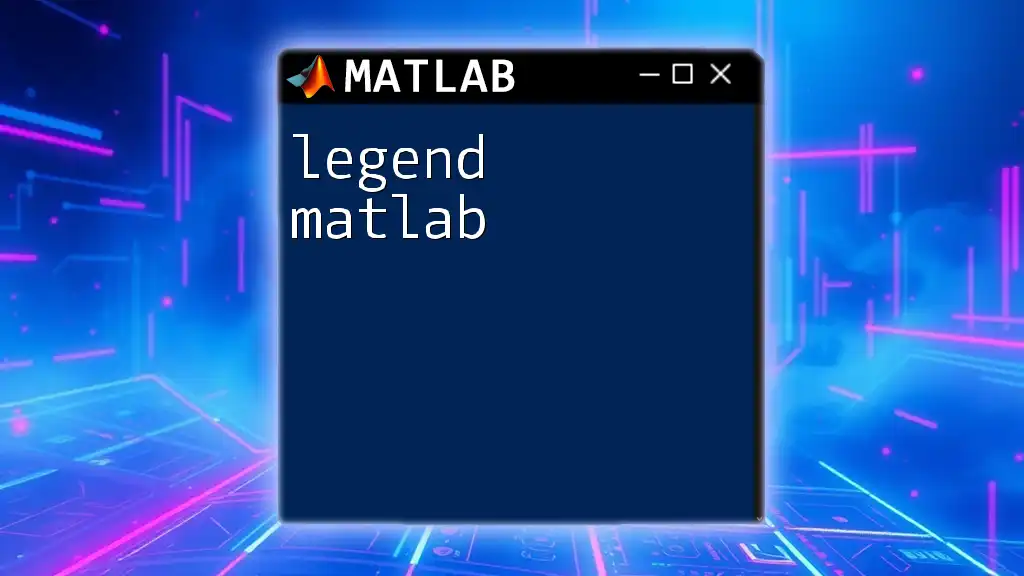
Setting Up MATLAB
Installing MATLAB
To implement MATLAB effectively, the first step is to install it on your system. Visit the official MATLAB website, choose the suitable version, and follow the straightforward steps for installation. Remember to check system requirements to ensure compatibility.
Navigating the MATLAB Environment
Once installed, you will be greeted by a user-friendly interface. The main components include:
- Command Window: This is where you enter commands and see outputs immediately.
- Workspace: Here, you can view all variables currently in memory.
- Editor: Use this section to write and save script files for more complex projects.
- Command History: This keeps a log of previously entered commands, providing a reference for reuse.
Understanding these components will help you quickly adapt and implement MATLAB in your projects.
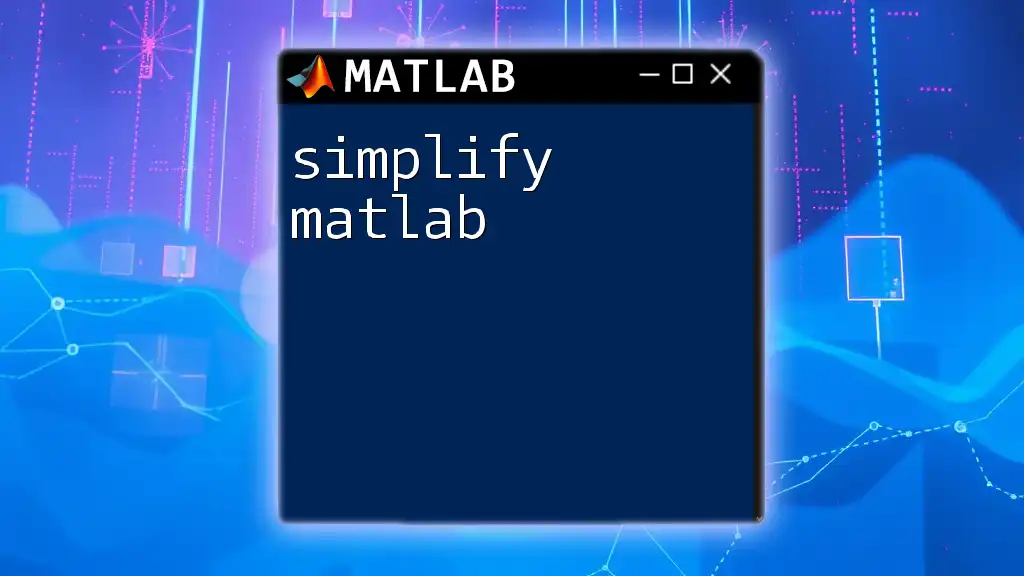
Basic MATLAB Commands
MATLAB Command Syntax
Understanding the syntax is crucial for effective programming in MATLAB. MATLAB commands often follow the structure:
`function_name(arguments)`
For instance, to display a message, you would use:
disp('Hello, MATLAB!')
Variables and Data Types
Declaring variables in MATLAB is straightforward. You can store a variety of data types, including numbers, strings, and matrices. Here’s how you create a few different types of variables:
% Creating variables
a = 10; % Integer
b = [1, 2, 3; 4, 5, 6]; % Matrix (2x3)
c = 'Hello'; % String
Understanding these different data types will aid you in organizing and manipulating data effectively.
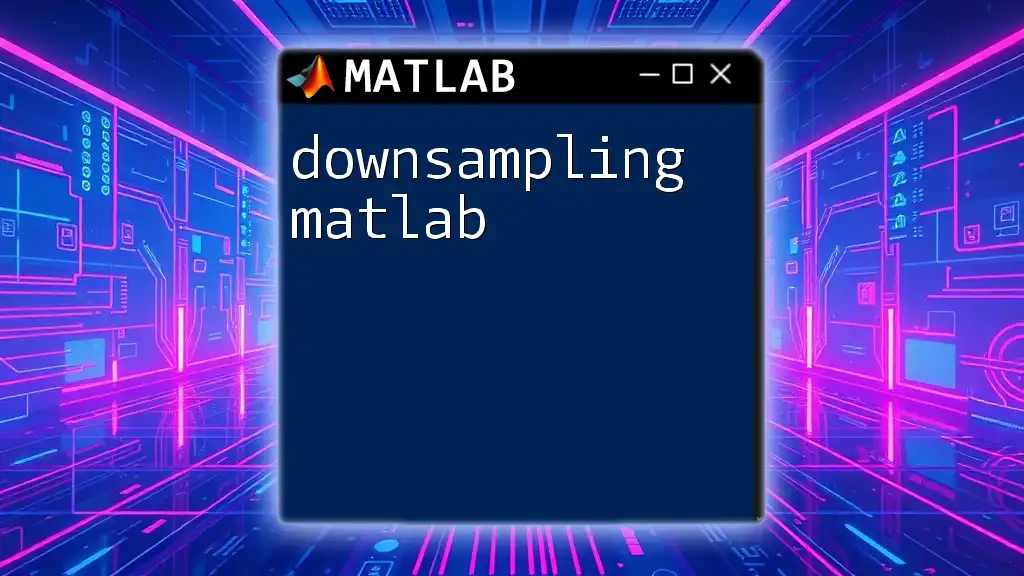
Implementing MATLAB: Practical Examples
Matrix Operations
Matrices are central to MATLAB. Performing basic operations such as addition, subtraction, and multiplication is seamless and efficient.
Here’s a simple example demonstrating matrix addition:
A = [1, 2; 3, 4]; % First matrix
B = [5, 6; 7, 8]; % Second matrix
C = A + B; % Matrix addition
disp(C); % Displays the resulting matrix
Plotting Data
Visualizing data is essential for analysis, and MATLAB excels at this. You can easily create 2D plots with just a few lines of code. Here’s how to plot a sine wave:
x = 0:0.1:10; % Define the x vector
y = sin(x); % Compute y as the sine of x
plot(x, y); % Create a plot
title('Sine Wave');
xlabel('X-axis');
ylabel('Y-axis');
This will generate a clear visual representation of the sine function, demonstrating the relationship between x and y.
Control Flow Structures
MATLAB supports various control flow structures like loops and conditional statements to enhance your programming. For instance, using a `for` loop to print iteration numbers can be valuable for debugging or status tracking:
for i = 1:5
disp(['Iteration: ', num2str(i)]);
end
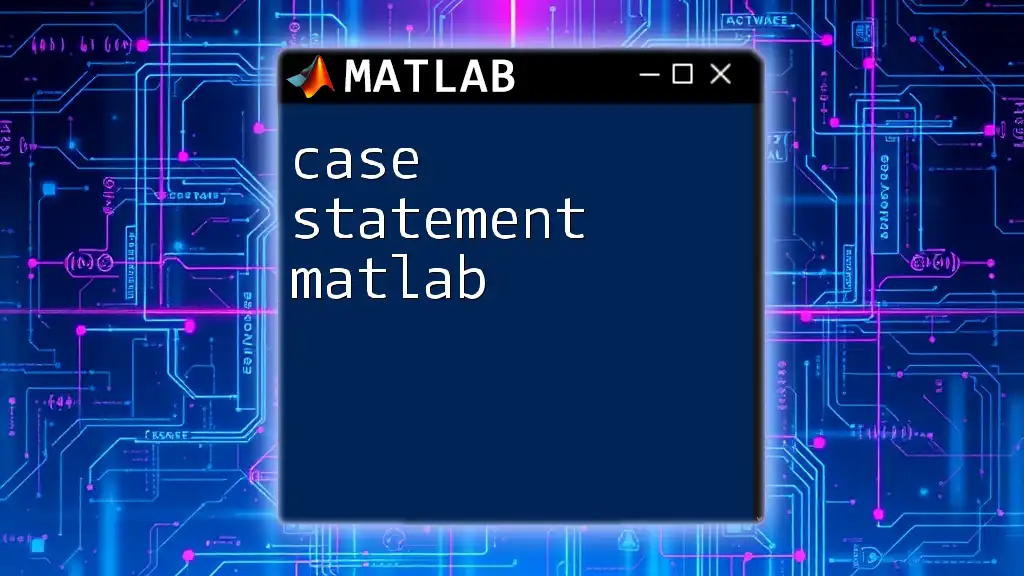
Writing Functions in MATLAB
Creating User-defined Functions
In MATLAB, functions facilitate writing reusable code. You can define your own functions to perform specific tasks. Here's an example function that adds two numbers:
function result = addNumbers(a, b)
result = a + b;
end
Calling this function `addNumbers(5, 10)` will return `15`, demonstrating how functions can simplify complex calculations by breaking them down into manageable parts.
Using Built-in Functions
MATLAB boasts an extensive library of built-in functions that enhance your coding experience. Using functions such as `mean`, `median`, or `sum` can save time and effort. For example, to find the mean of an array:
data = [1, 2, 3, 4, 5];
meanValue = mean(data); % Calculates the average
disp(meanValue);
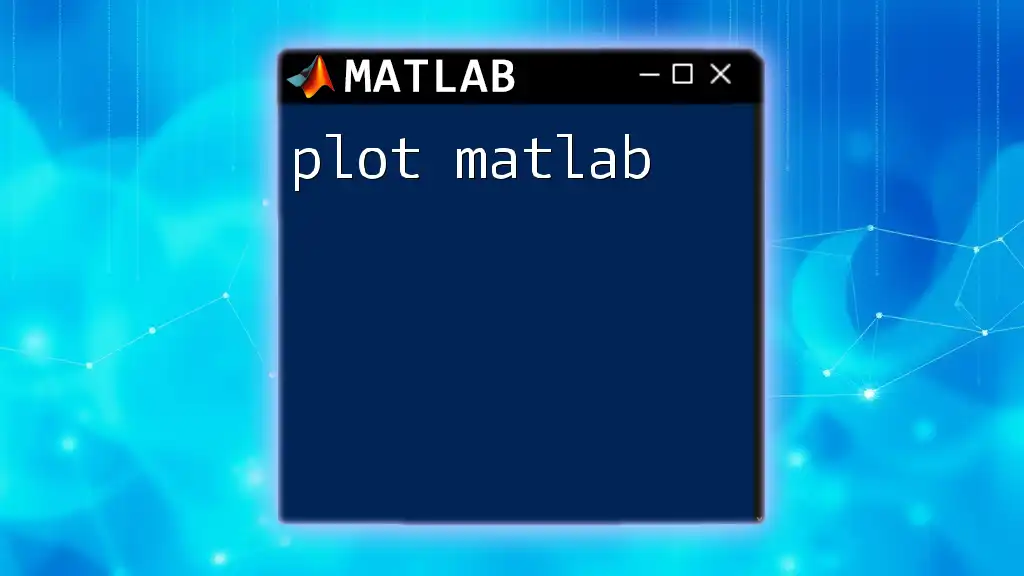
Debugging and Error Handling in MATLAB
Common Errors and Solutions
Debugging is an integral part of programming, and MATLAB provides informative error messages that guide you through resolving issues. Common errors include:
- Syntax Errors: Catch these mistakes before running the code.
- Indexing Errors: Ensure that you're not trying to access elements outside the dimensions of your arrays.
Debugging Tools in MATLAB
MATLAB offers several debugging tools, such as breakpoints to pause the execution of your code, allowing you to check variable values and flow. You can set breakpoints right in the Editor, facilitating a step-by-step analysis of your script.
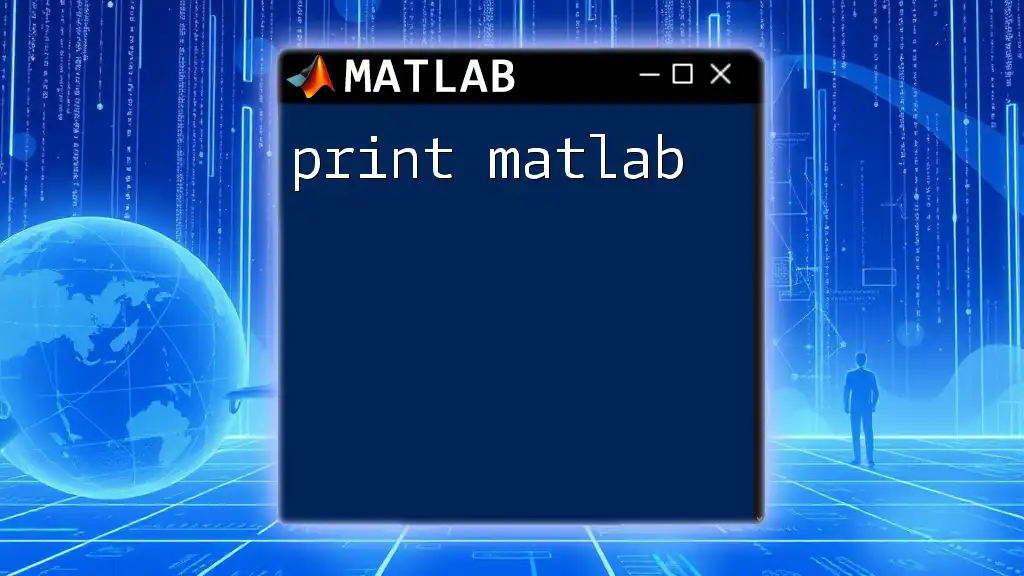
Advanced Topics
Using Toolboxes
MATLAB toolboxes are specialized packages that extend MATLAB's functionality. Toolboxes such as the Image Processing Toolbox or the Statistics and Machine Learning Toolbox provide pre-built functions relevant to specific tasks, saving you time and energy.
Integrating MATLAB with Other Languages
Another advantage of MATLAB is its ability to integrate with other programming languages like Python and C++. This versatility allows users to expand their workflow capabilities and leverage MATLAB’s powerful environment alongside different coding environments.
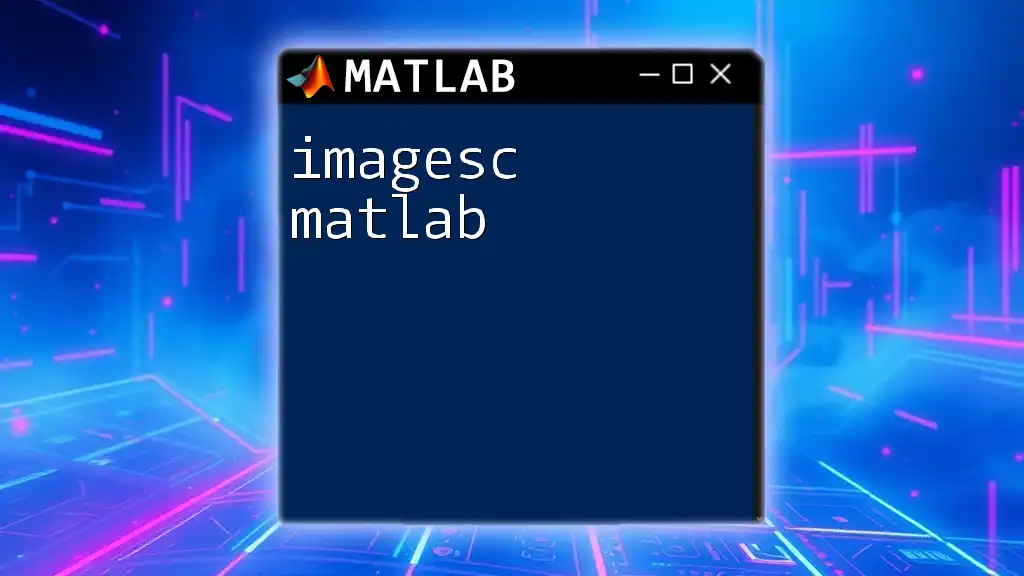
Conclusion
Implementing MATLAB efficiently can significantly improve your productivity and analytical capabilities. With a solid understanding of its fundamental features, variable types, basic commands, and debugging tools, you will be well-equipped to tackle complex problems and enhance your projects. Don’t hesitate to explore more about MATLAB and practice coding to become proficient in this invaluable tool!