The `circshift` function in MATLAB is used to circularly shift the elements of an array along a specified dimension.
A = [1, 2, 3, 4, 5]; % Original array
B = circshift(A, 2); % Circularly shift elements by 2 positions
% B will be [4, 5, 1, 2, 3]
Understanding the Basics of circshift
What is circshift?
The `circshift` function in MATLAB is an essential command used for circularly shifting the elements of an array. Unlike traditional shifting, where elements may be discarded or need to be padded, circular shifting moves the elements around a defined boundary, effectively wrapping them around. This characteristic makes it highly valuable in various applications, especially when it comes to data manipulation in numerical and signal processing contexts.
Use Cases for circshift
The utility of `circshift` extends to multiple domains, including:
- Data Analysis: It allows for the rearrangement of data without losing any information, making it easy to analyze patterns or trends in time-series data.
- Image Processing: By rotating image matrices, you can manipulate how images are displayed and processed, helping with tasks such as image enhancement and filtering.
- Signal Processing: Circularly shifting signals can simulate effects like delay and echo, which are critical in audio engineering and telecommunications.
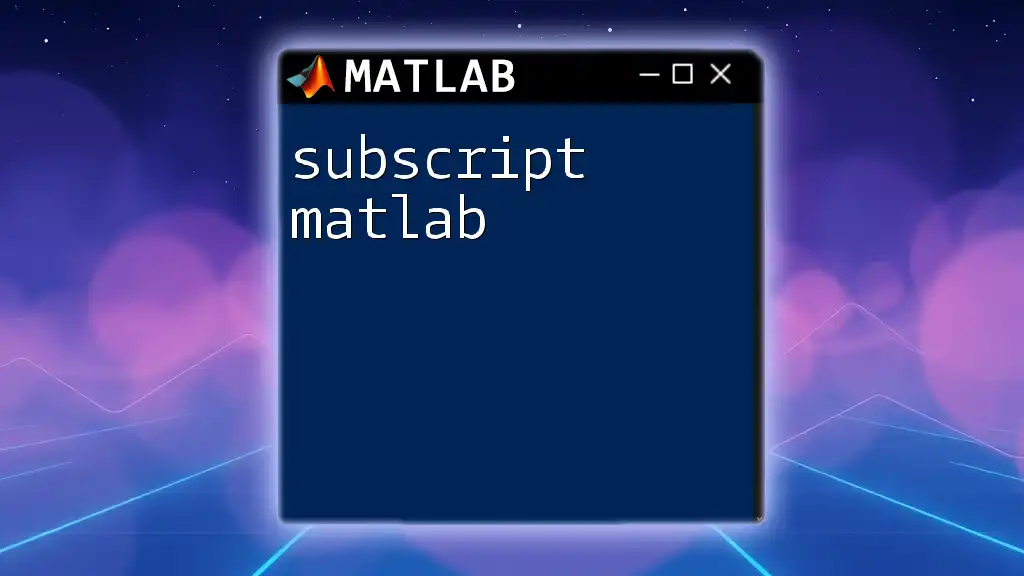
Syntax of circshift
Basic Syntax
The fundamental syntax for the `circshift` function is as follows:
B = circshift(A, K)
Where:
- A represents the input array, which can be a vector, matrix, or multidimensional array.
- K indicates the number of positions to shift. This can be a scalar or an array defining different shifts for different dimensions.
Example with a Simple Array
Here’s a straightforward example illustrating how `circshift` works with a one-dimensional vector:
A = [1, 2, 3, 4, 5];
B = circshift(A, 2); % B becomes [4, 5, 1, 2, 3]
In this case, the elements of array A are shifted two positions to the right, and the process wraps around the end of the array.
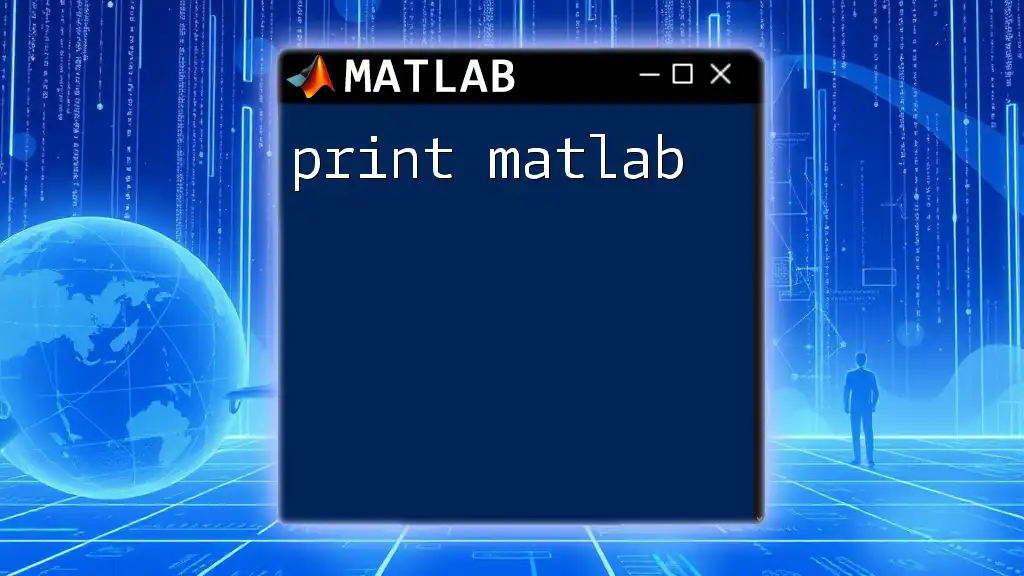
How to Use circshift with Different Dimensions
One-dimensional Arrays
When working with one-dimensional vectors, you can easily modify the order of the elements. For instance:
A = [10, 20, 30, 40, 50];
B = circshift(A, -1); % B is [20, 30, 40, 50, 10]
Here, `circshift` moves each element to the left by one, wrapping the first element around to the end of the array. Positive values shift the elements to the right, while negative values shift them to the left.
Two-dimensional Arrays
In the case of two-dimensional matrices, you can specify how many positions to shift along each dimension:
A = [1, 2, 3;
4, 5, 6;
7, 8, 9];
B = circshift(A, [1, 0]); % B shifts rows down
In this example, the matrix A is shifted down by one row, with the last row wrapping around to the top. The `K` parameter can be a two-element array, where you can separately define shifts for rows and columns (i.e., `K = [m, n]`) where m shifts rows and n shifts columns.
Multi-dimensional Arrays
Though `circshift` is frequently applied to 1D and 2D arrays, it also works seamlessly with N-dimensional arrays. The same syntax applies, providing flexibility and convenience in handling complex datasets.
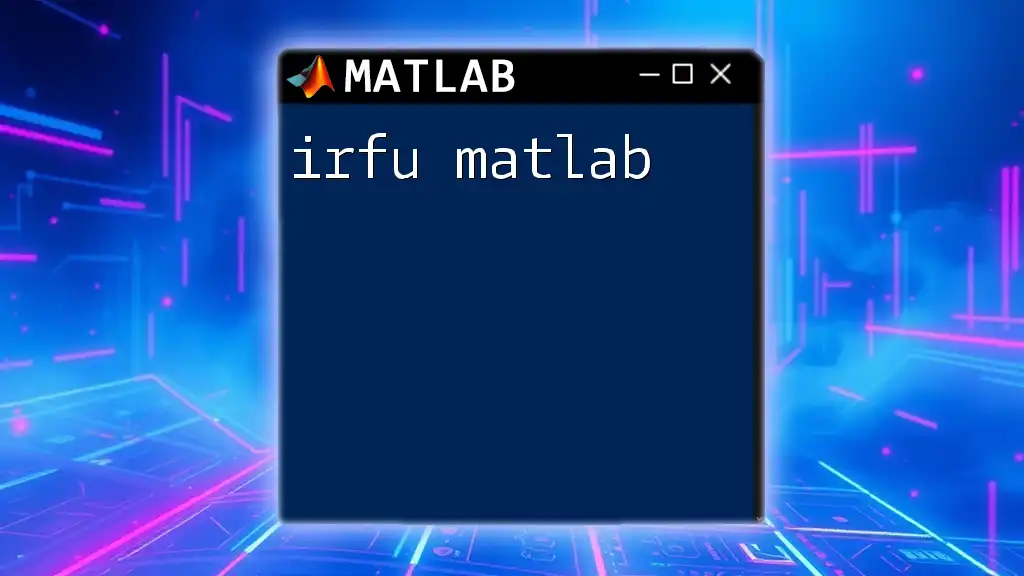
Common Pitfalls and Best Practices
Common Issues
While working with `circshift`, users may encounter several pitfalls, such as attempting to shift arrays of incompatible sizes. It's crucial to ensure that `K` does not exceed the dimensions of the array; otherwise, MATLAB will return an error. One common mistake is to assume that shifts can be made with non-integer values, which is not supported by `circshift`.
Best Practices
To effectively utilize `circshift`:
- Opt for `circshift` when working with time-series data or cyclic phenomena.
- Avoid using `circshift` for operations involving shifts that exceed the array size, as MATLAB will produce an error.
- Be cautious about the dimensions: always ensure that `K` is correctly specified for the array dimensions you are manipulating.
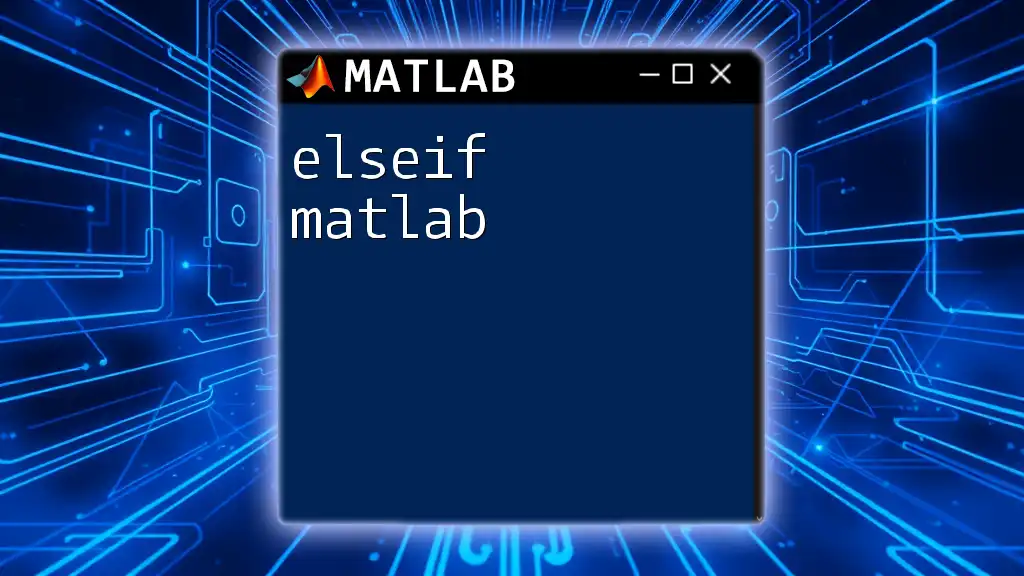
Practical Examples
Example 1: Circular Shifting a Signal
To demonstrate how `circshift` can manipulate a signal array, consider the following example:
t = linspace(0, 1, 20);
signal = sin(2 * pi * 5 * t);
shifted_signal = circshift(signal, 3);
This code simulates a delay of three samples in a sinusoidal signal. It’s important to note how the wrapping effect of circular shifting can introduce interesting characteristics in signals used in audio or image processing algorithms.
Example 2: Rotating an Image
While the implementation of `circshift` for image processing can vary greatly, a basic example might look like this:
img = imread('image.png');
shifted_img = circshift(img, [20, 30]); % shifting image down by 20 pixels and right by 30 pixels
imshow(shifted_img);
In this code, the input image undergoes a vertical and horizontal shift, effectively repositioning it within the display window. Users should ensure proper handling of borders, which might result in empty pixel values.
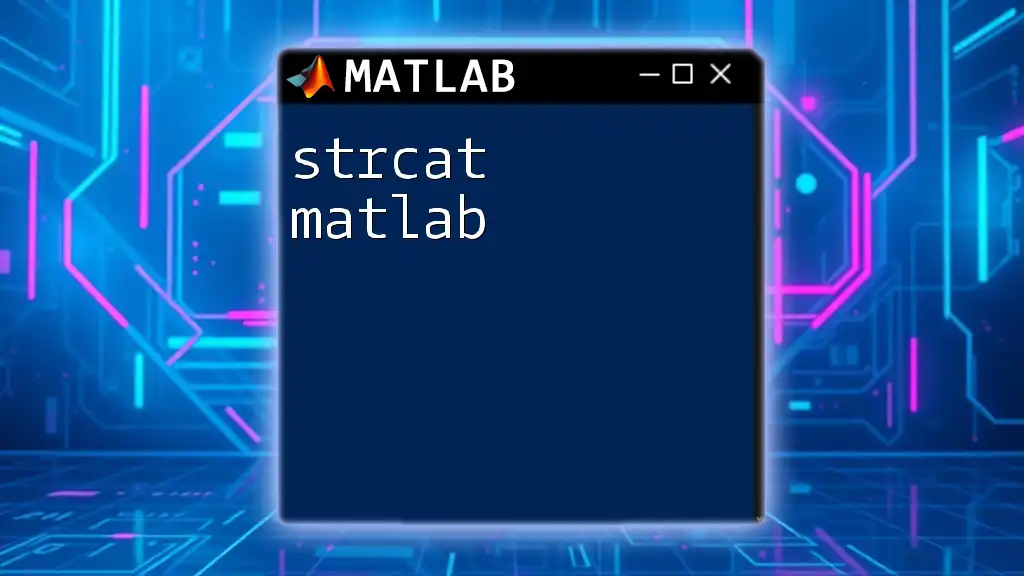
Conclusion
In summary, the `circshift` function in MATLAB is a powerful tool for array manipulation and analysis. Whether you're shifting data in time-series analysis, rotating images in computer vision, or working with signals in telecommunications, `circshift` offers flexibility and efficiency. Practice using this command as it can greatly enhance your project outcomes in various fields of study.
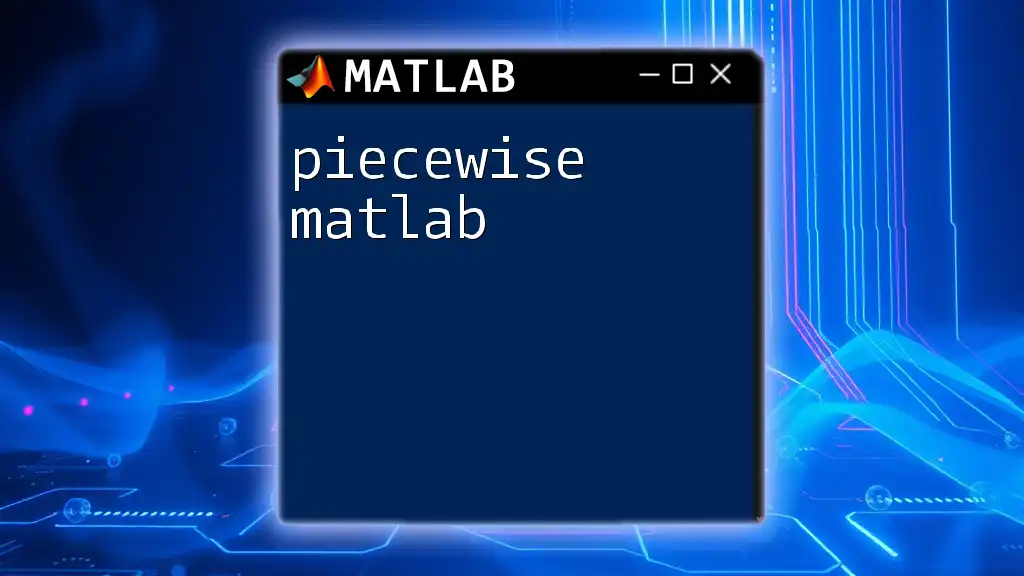
Additional Resources
For further exploration of `circshift`:
- Visit the official MATLAB documentation on `circshift` to get in-depth details.
- Consider registering for tutorials that delve deeper into MATLAB’s extensive functionality, making you a more proficient user.
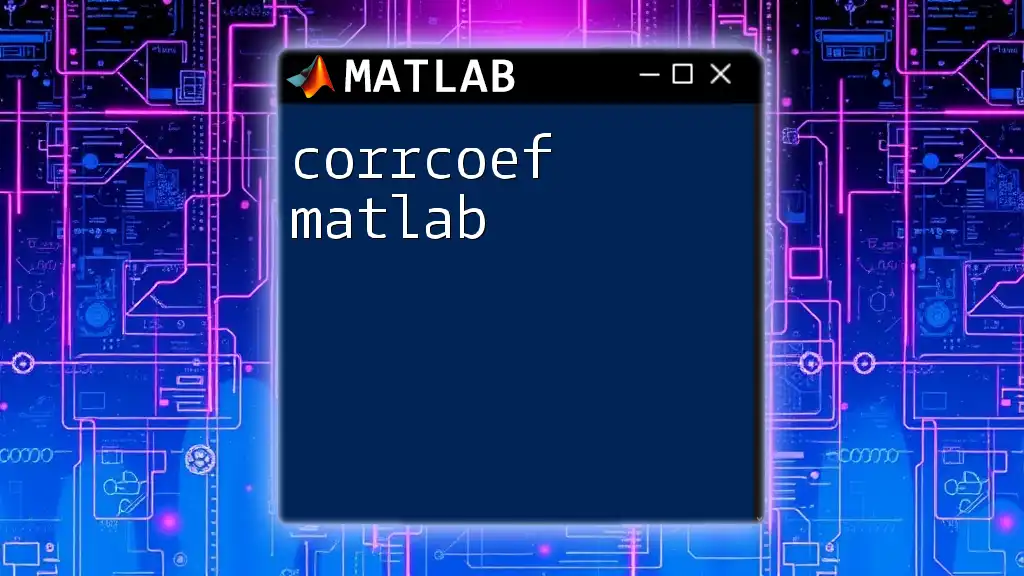
FAQs
-
Can `circshift` be used for complex numbers?
Yes, `circshift` works with arrays of complex numbers without any issues. -
What happens to multidimensional arrays if shift exceeds their size?
If the shift value exceeds the array's dimension, MATLAB will return an error. It is essential to keep track of the array's size. -
Are there alternative methods available for similar operations in MATLAB?
While `circshift` is one of the most straightforward commands available, alternatives like custom circular shifting using indexing can also be explored, especially if you require specific behavior not covered by `circshift`.