A color plot in MATLAB is a graphical representation of data values using color gradients, allowing for easy visualization of complex datasets.
Here's a simple example of creating a color plot using the `surf` function:
% Create a meshgrid and compute the corresponding Z values
[X, Y] = meshgrid(-5:0.1:5, -5:0.1:5);
Z = sin(sqrt(X.^2 + Y.^2));
% Create the color plot
surf(X, Y, Z);
colorbar; % Add a color bar to indicate the color scale
Understanding Color Maps
What are Color Maps?
Color maps are essential tools that map a range of values to specific colors. They play a critical role in visualizing data, as they can help to illustrate gradients, patterns, and data distributions. In MATLAB, color maps can convey information about magnitude and direction, enabling a more intuitive interpretation of complex data sets.
Common MATLAB color maps include:
- `parula`: A perceptually uniform colormap suitable for most applications.
- `jet`: A classic colormap that transitions through colors, though it's often criticized for misrepresenting data.
- `hsv`: A way to represent hue, saturation, and value, useful for cyclic data.
Creating Color Maps
When existing color maps do not fit your needs, you can create custom color maps using RGB values. This allows for tailored visualizations that can emphasize or downplay specific data elements.
Example: Here's how to define a simple color map utilizing RGB values:
myColorMap = [1 0 0; 0 1 0; 0 0 1]; % Red, Green, Blue
colormap(myColorMap);
This code snippet creates a custom color map with red, green, and blue colors, which can be applied to any plot.
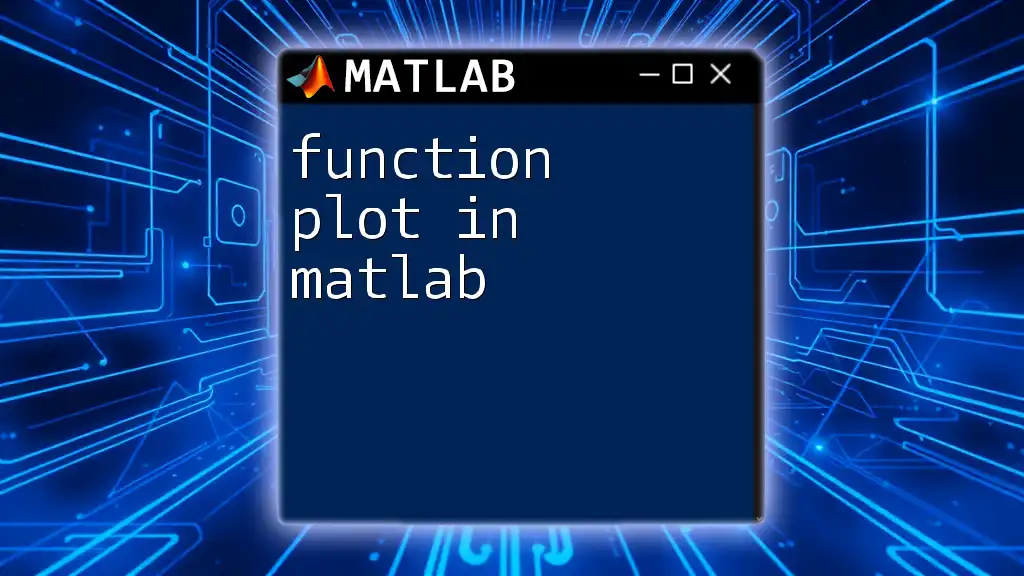
Basic Color Plotting Commands
Using `scatter` for Color-Plotted Data
The `scatter` function in MATLAB provides a straightforward way to create scatter plots that visually represent data with varying colors. This is effective for displaying relationships between numeric variables and can help to highlight clusters or trends.
Example: A simple scatter plot with color coding can be created as follows:
x = rand(1, 100);
y = rand(1, 100);
colors = rand(1, 100); % Random color data
scatter(x, y, 100, colors, 'filled');
colormap('jet');
colorbar; % Show color scale
In the example above, the `rand` function generates random data points where the color intensity is also randomly assigned. The colorbar gives context to the colors being represented.
Using `surf` for Surface Color Plots
The `surf` function is particularly useful for generating three-dimensional surface plots. By assigning colors based on the Z-values, you can create visually compelling representations of topographic data or mathematical functions.
Example: Here is how to create a surface plot with a gradient of colors:
[X, Y] = meshgrid(-5:0.5:5, -5:0.5:5);
Z = X.^2 + Y.^2; % Paraboloid
surf(X, Y, Z);
colormap('hot');
colorbar; % Display color bar
With this code, you create a surface that represents a paraboloid, where the color maps reflect the height of the surface.
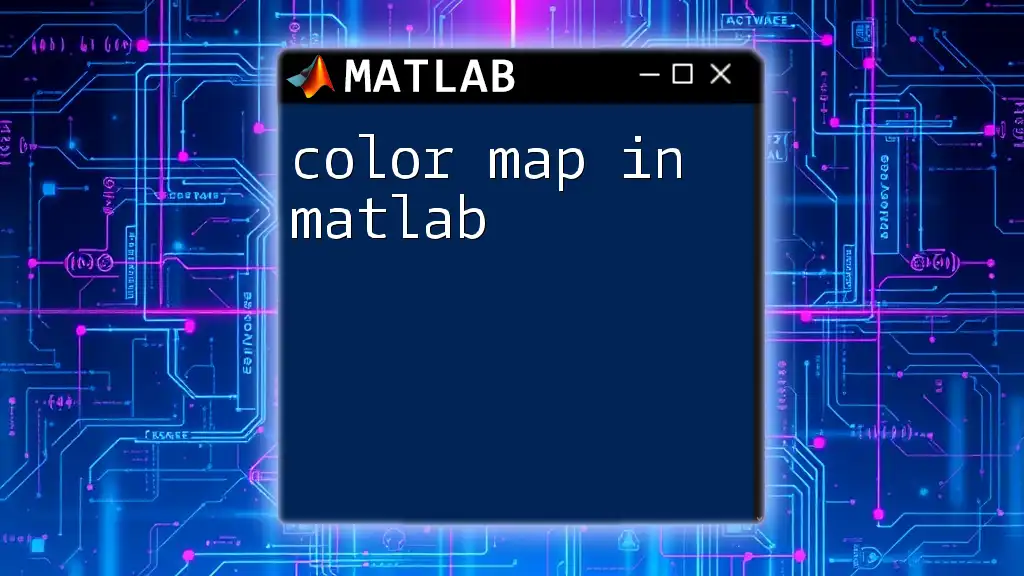
Advanced Color Plot Techniques
Modifying Color Map Properties
To enhance clarity and insight from your plots, modifying color map properties is vital. You can adjust color limits, which will determine how data ranges correlate to color appearances.
Example: Here’s how to use `caxis` to define color limits:
caxis([5, 15]); % Set color limits for better visualization
This command constrains the color mapping to specific numeric limits, improving readability for areas of interest.
Using `pcolor` for Color-Plotted Matrices
The `pcolor` function provides an alternative way to visualize matrices. It uses colored rectangles to represent the values, making it suitable for heatmaps and similar applications.
Example: Here is how to create a color grid using `pcolor`:
Z = peaks(30);
pcolor(Z);
shading interp; % Smoother color transitions
colormap('cool');
The `shading interp` command allows for smoother transitions between colors, enhancing the visual appeal and making it easier to interpret data.
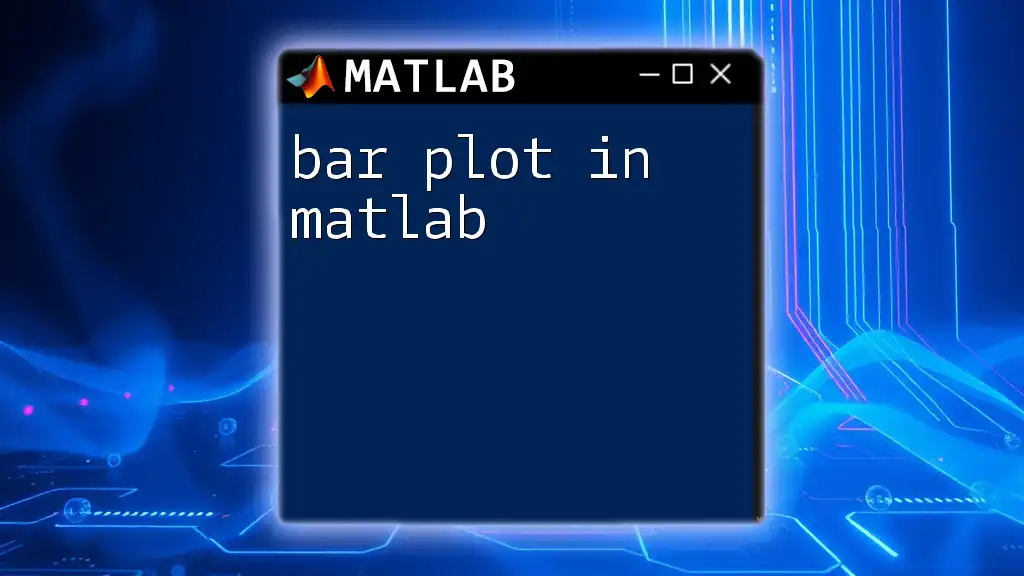
Creating Interactive Color Plots
Adding Data Tips
Enhancing interaction in your plots can improve user experience and understanding. Data tips can be added to color plots, allowing viewers to explore data-driven insights dynamically.
Example: You can easily add data tips to a scatter plot like this:
h = scatter(x, y, 100, colors, 'filled');
datacursormode on; % Enables interactive mode
With `datacursormode on`, users can click on data points to see their values, which makes the plot more interactive and informative.
Utilizing `uicontrol` for Dynamic Color Adjustment
MATLAB's `uicontrol` functionality allows you to create interactive elements, like sliders, for real-time adjustments of color maps or other properties.
Example: While a full implementation requires more setup, a simple slider can adjust the color dynamically:
% Pseudo-code for dynamic adjustment
uicontrol('Style', 'slider', 'Min', 1, 'Max', 10, 'SliderStep', [0.1 0.2], ...
'Callback', @(src, evt) updateColorMap(src.Value));
This functionality enhances user interaction by permitting real-time changes to the color map based on user input.
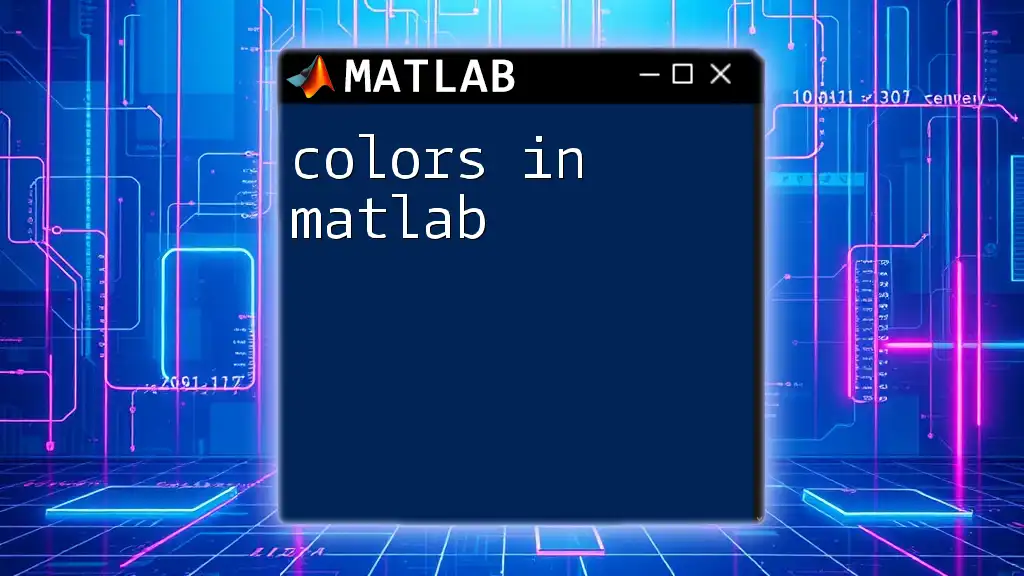
Troubleshooting Common Issues
When creating color plots in MATLAB, users may encounter several common issues, such as poor visibility or misleading representations of data. To avoid these pitfalls, focus on selecting appropriate color maps, ensuring contrast, and using `colorbar` to provide context. Always test your visualizations with real data scenarios to ensure clarity and accessibility for all viewers.
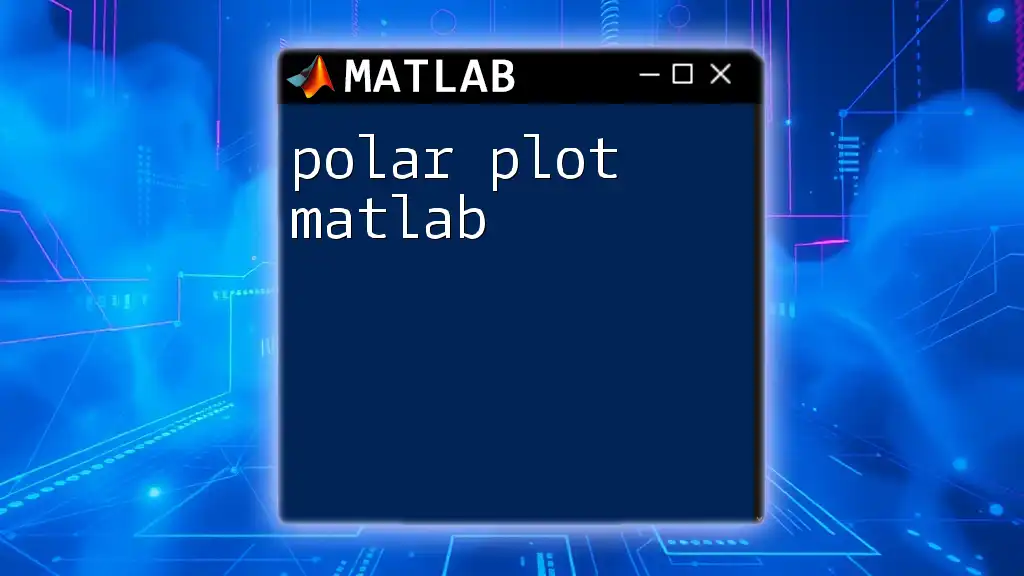
Conclusion
In summary, color plots in MATLAB are powerful tools for data visualization, enabling users to represent complex data intuitively. By understanding and utilizing color maps, functions like `scatter`, `surf`, and `pcolor`, and aiming for interactivity, you can effectively enhance the way data is presented and analyzed. As you continue to explore MATLAB’s capabilities, remember that the right visualization can significantly impact the interpretation and understanding of your data.
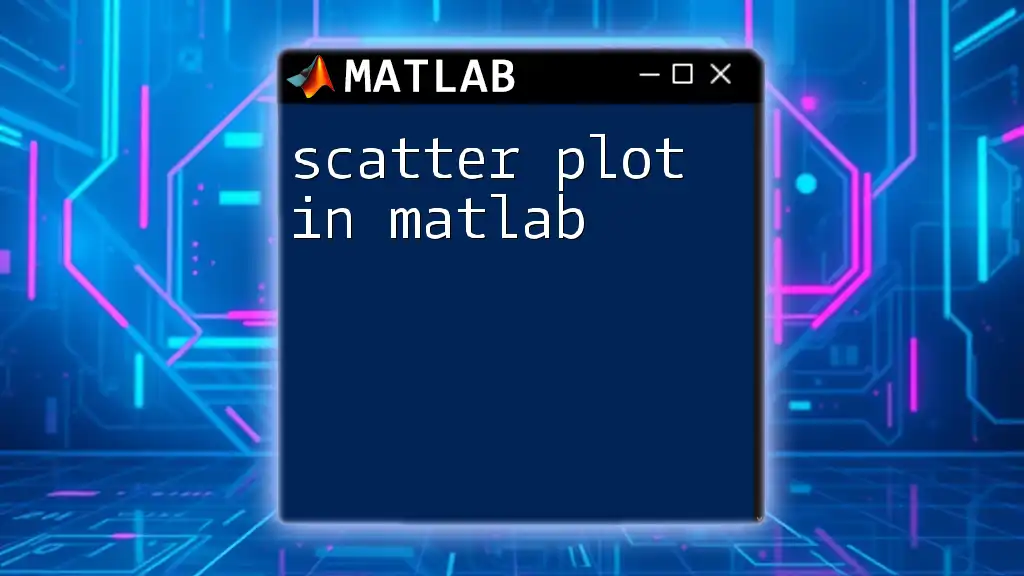
Additional Resources
For further learning, consider exploring MATLAB's official documentation and online forums. These resources will provide valuable insights and community support, enhancing your MATLAB skills and knowledge of plotting techniques.