A log plot in MATLAB is used to create a graph where one or both axes use a logarithmic scale, allowing for better visualization of data that spans several orders of magnitude.
Here’s a simple example of how to create a log plot in MATLAB:
x = 1:100; % Define the data for the x-axis
y = log10(x); % Compute the logarithm (base 10) of the x values
semilogy(x, y); % Create a log plot with logarithmic scale on the y-axis
xlabel('X-axis (linear scale)'); % Label for x-axis
ylabel('Y-axis (log scale)'); % Label for y-axis
title('Log Plot Example'); % Title of the plot
grid on; % Add a grid for better readability
What is a Log Plot?
Definition and Purpose
A log plot is a type of graph that uses a logarithmic scale for one or both axes instead of the traditional linear scale. This transformation allows us to better visualize data that can span several orders of magnitude. Log plots are especially useful for interpreting exponential growth, power laws, and phenomena that involve multiplicative processes.
Applications of Log Plots
Logarithmic scales are applied in various fields, including:
- Engineering: Visualizing frequency response, gain in amplifiers, and system stability.
- Science: Analyzing seismic data, population growth, and P-H diagrams.
- Finance: Presenting stock prices, economic growth rates, and inflation trends.
Log plots make it easier to identify trends, data relationships, and anomalies when dealing with large variations in data.
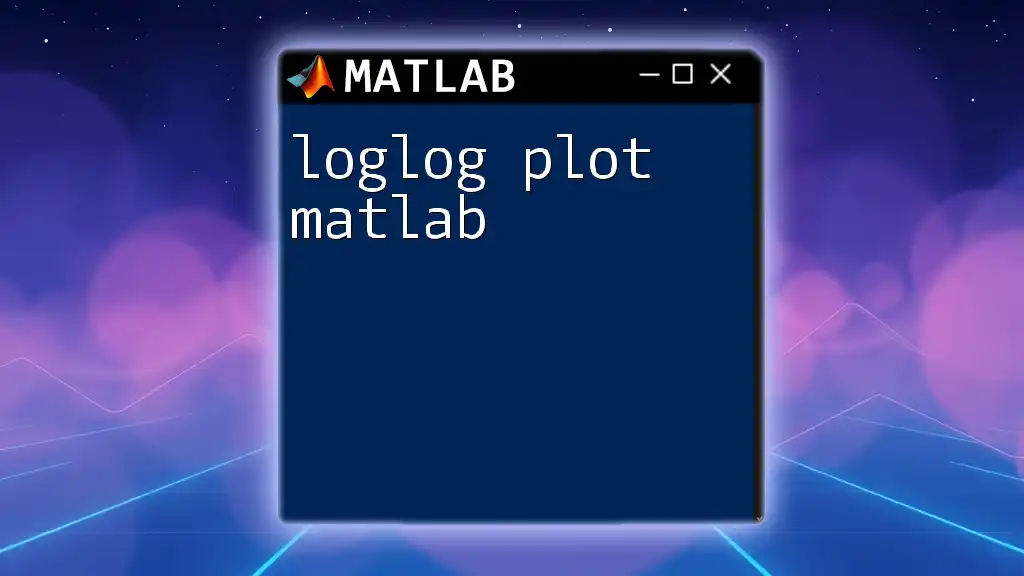
Creating a Log Plot in MATLAB
Basic Syntax for Logarithmic Plots in MATLAB
To create a log plot in MATLAB, you can use the built-in plotting functions such as `plot`, `semilogy`, and `loglog`. Each function serves a particular purpose:
- `semilogy`: Uses a logarithmic scale for the y-axis.
- `semilogx`: Employs a logarithmic scale for the x-axis.
- `loglog`: Implements logarithmic scales for both axes.
Here is a basic syntax example using the `plot` function:
x = 1:10;
y = log(x);
figure;
plot(x, y);
title('Basic Log Plot');
xlabel('X-axis');
ylabel('Log(X)');
grid on;
In this code, we define `x` as a range from 1 to 10 and calculate the natural logarithm of `x` for `y`. The `figure` command creates a new plot, while `plot` renders the graph.
Example: Basic Log Plot
The following code snippet demonstrates how to create a log plot:
x = 1:10;
y = log(x);
figure;
plot(x, y);
title('Basic Log Plot');
xlabel('X-axis');
ylabel('Log(X)');
grid on;
This code plots the logarithm of `x` versus `x`, providing a visual representation of how logarithmic values diminish as `x` increases.
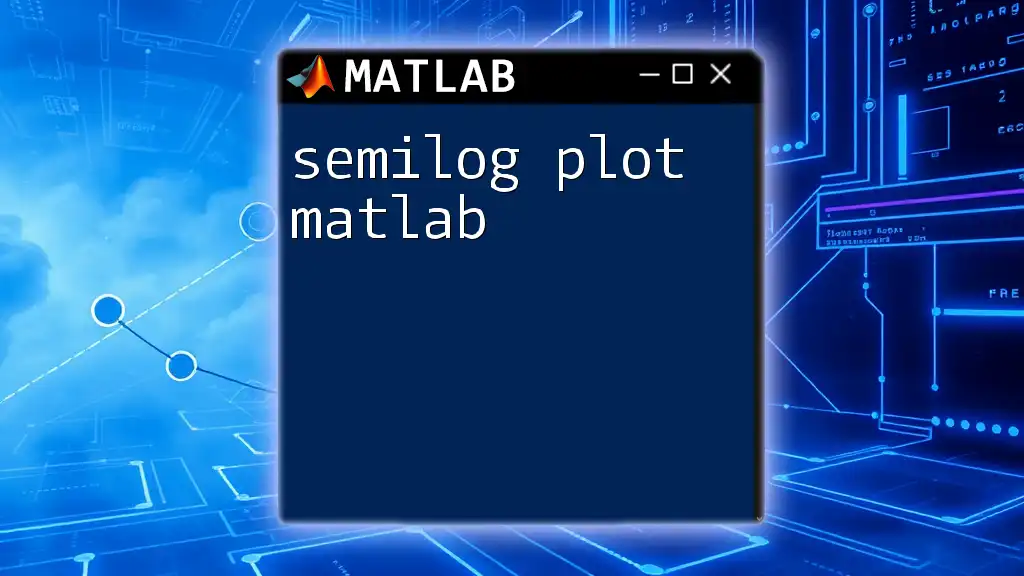
Different Types of Logarithmic Plots
Log-Linear Plots
A log-linear plot features a logarithmic scale on the y-axis while retaining a linear scale on the x-axis, making it ideal for visualizing exponential growth. To create a log-linear plot in MATLAB, we use the `semilogy` function. Here’s an example:
y = exp(x);
figure;
semilogy(x, y);
title('Log-Linear Plot');
xlabel('X-axis');
ylabel('Log(Y)');
grid on;
In this code, we define the function `y` to be the exponential of `x`, which can demonstrate how quickly exponential functions grow.
Log-Log Plots
Log-log plots utilize logarithmic scales for both axes, useful for analyzing data that follows power laws. Here is how to create one using the `loglog` function:
y = x.^2;
figure;
loglog(x, y);
title('Log-Log Plot');
xlabel('Log(X)');
ylabel('Log(Y)');
grid on;
The code above visualizes the quadratic relationship between `x` and `y`, effectively demonstrating how the dimensionless parameters behave across different orders of magnitude.
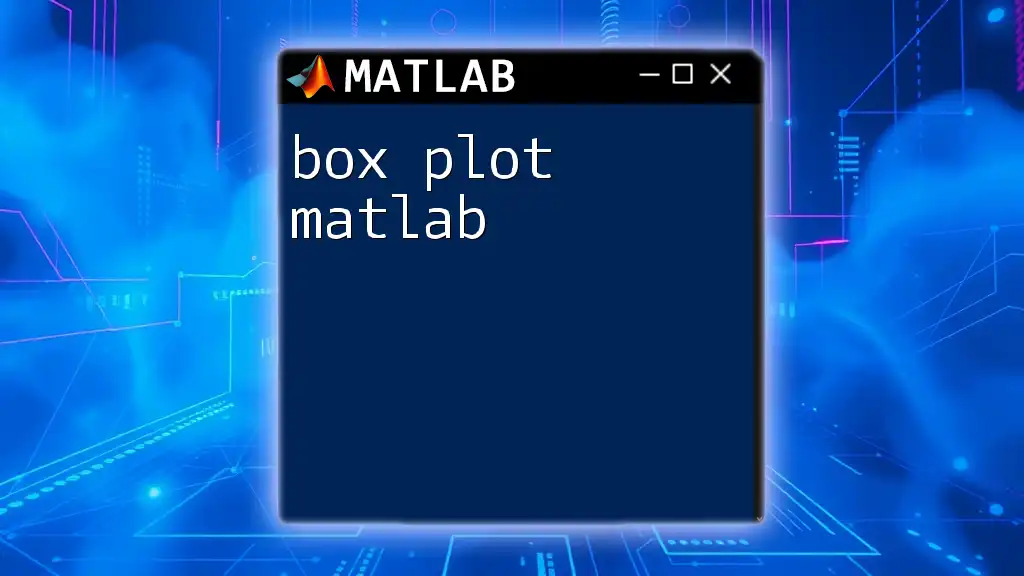
Customizing Log Plots
Enhancing Plot Appearance
MATLAB offers extensive options for customizing the appearance of your plots. You can enhance your graph by adding titles, labels, and displaying a grid. Below is a code snippet showcasing these features:
figure;
loglog(x, y);
title('Customized Log Plot');
xlabel('Log(X)');
ylabel('Log(Y)');
grid on;
In this snippet, we incorporated elements that provide context and clarity to your visualization.
Changing the Axes Scale
Sometimes, you may want to change the scale of the axes after creating a plot. The `set(gca, 'YScale', 'log')` command allows you to accomplish this:
figure;
plot(x, y);
set(gca, 'YScale', 'log');
title('Custom Log Scale on Y-axis');
xlabel('X-axis');
ylabel('Log(Y)');
grid on;
This command enables logarithmic scaling on the y-axis for already plotted data, allowing for easier examination of multiplicative relationships.
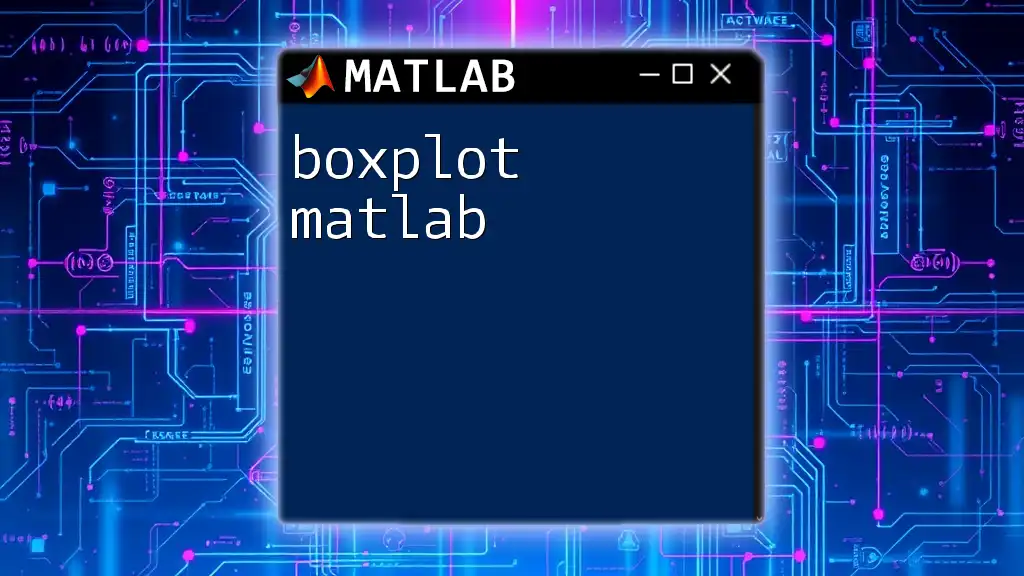
Advanced Log Plot Features
Adding Multiple Data Series
You can overlay multiple datasets on the same log plot, which can be invaluable for comparative analysis. Here’s an example that illustrates this concept:
y2 = exp(x)/10;
figure;
loglog(x, y, 'r', x, y2, 'b--');
legend('y = log(x)', 'y = exp(x)/10');
title('Multiple Data Series on Log Plot');
xlabel('Log(X)');
ylabel('Log(Y)');
grid on;
This code delineates two datasets using different line styles and colors, helping distinguish them on the same plot.
Annotation and Text on Plots
Adding annotations can make specific points or trends within your log plot clearer. You can achieve this with the `text()` function:
figure;
loglog(x, y);
text(2, log(2), 'Point of Interest', 'FontSize', 12, 'Color', 'red');
This piece of code annotates a point on the log plot, emphasizing the significance of that data point.
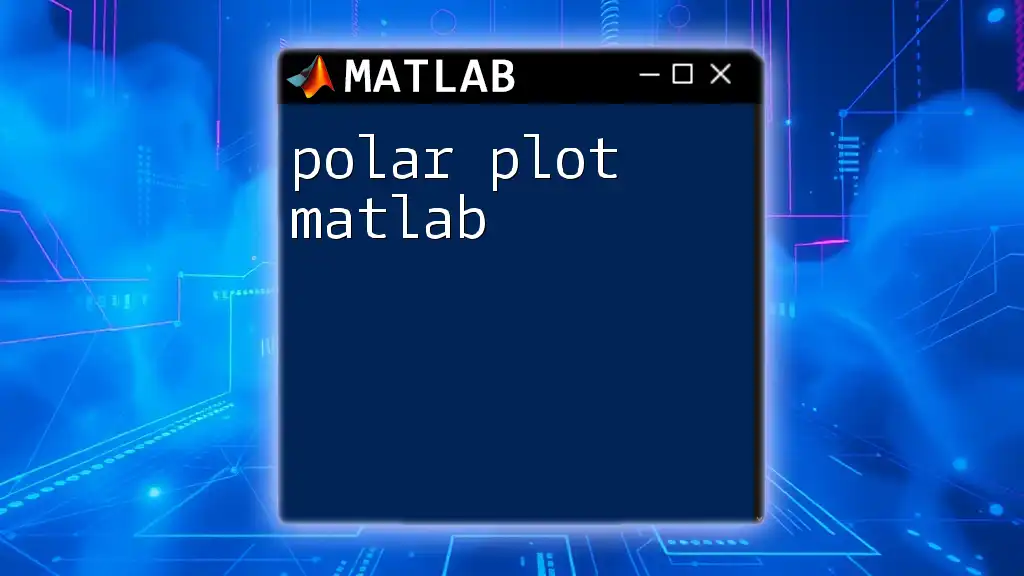
Common Mistakes and Troubleshooting
Typical Errors When Creating Log Plots
When working with log plots, it's imperative to remember that logarithmic scales cannot handle zero or negative values. If you attempt to plot such numbers, MATLAB will return an error. Always ensure that your data adheres to the requirements for logarithmic plotting.
Debugging tips include checking your data values for negatives before plotting and ensuring proper transformations are applied to your data.
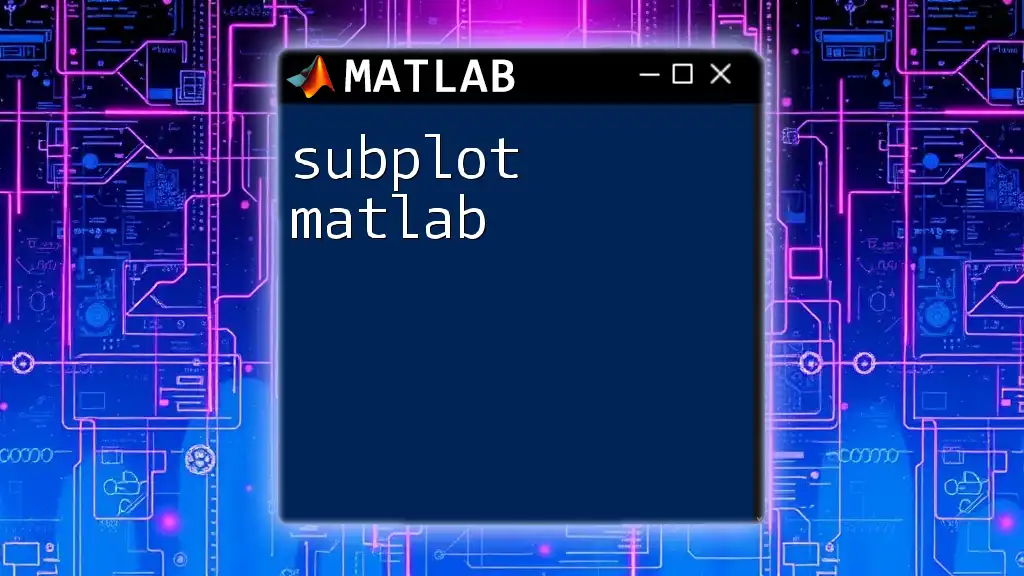
Conclusion
Log plots are indispensable when it comes to analyzing data spanning several orders of magnitude. By fully leveraging MATLAB's built-in functions and customization commands, you can create informative and visually appealing log plots. Through experimentation and practice, you can gain proficiency in using log plots to uncover insights hidden within complex datasets.
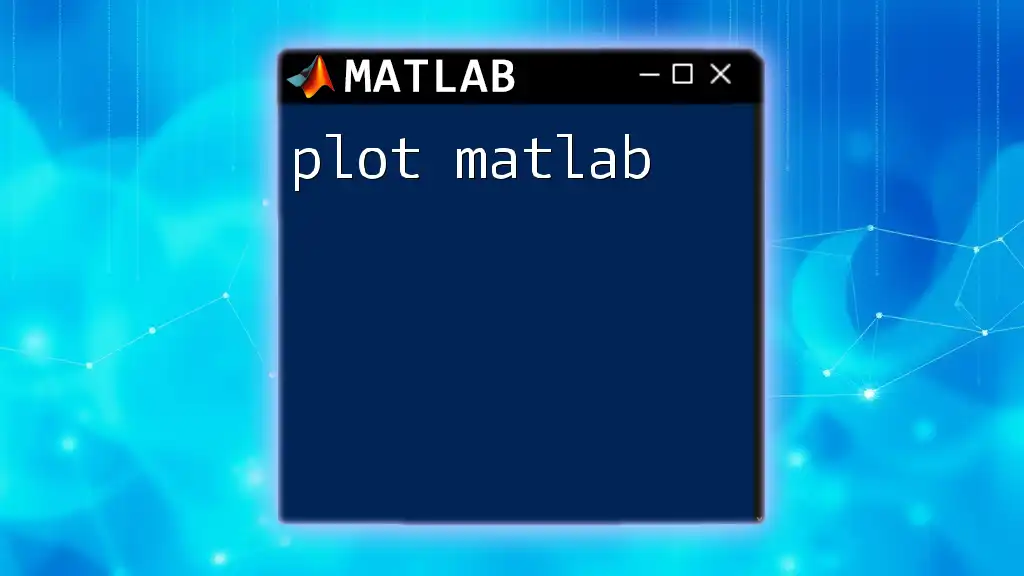
Additional Resources
For further reading, explore the official MATLAB documentation, online tutorials, or specialized courses in data visualization techniques. Engaging with community forums and seeking help from experienced users will also enhance your understanding and application of log plots in MATLAB.