A polar plot in MATLAB is used to create a circular plot that represents data in terms of angles and radius, allowing for better visualization of directional data.
Here’s a simple example of how to create a polar plot in MATLAB:
theta = 0:0.01:2*pi; % Angles from 0 to 2*pi
r = sin(2*theta); % Radius as a function of theta
polarplot(theta, r); % Create the polar plot
title('Polar Plot of r = sin(2\theta)'); % Add title
What is a Polar Plot?
A polar plot allows you to visualize data using polar coordinates rather than Cartesian coordinates. It represents data where each point is defined by a radius and an angle, making it an essential tool for certain types of data, such as circular motion or wave forms. The visualization of data in this format can provide clearer insights into periodic phenomena.
Why Use MATLAB for Polar Plots?
MATLAB (Matrix Laboratory) offers a robust environment for mathematical computing, and it excels at visualizations. The language’s built-in functions enable you to create intricate plots with minimal coding effort. With a simple command, you can represent complex relationships between variables in a visually appealing manner. The efficiency and flexibility of MATLAB make it a popular choice among engineers, scientists, and researchers.
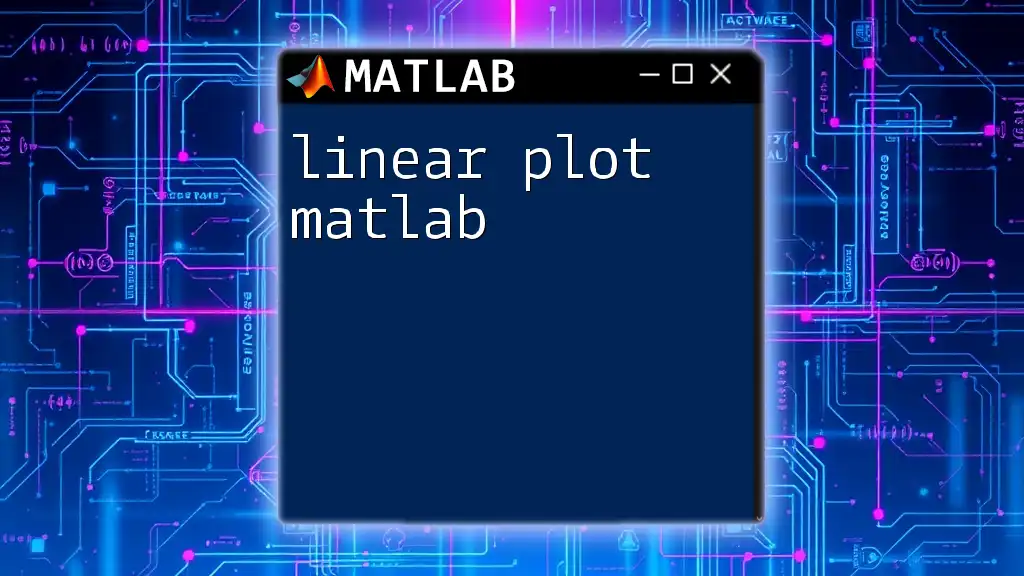
Understanding the Basics of Polar Coordinates
What are Polar Coordinates?
In polar coordinates, a point in a plane is determined by two values: the distance from a reference point (usually the origin) and the angle formed with a reference direction (usually the positive x-axis). This stands in stark contrast to Cartesian coordinates, which use x and y coordinates to describe a point. Understanding polar coordinates is essential for effectively utilizing polar plots in MATLAB.
Syntax of Polar Coordinates in MATLAB
In MATLAB, the basic commands for creating polar plots utilize two main vectors:
- Theta (\( \theta \)): It represents the angle, usually in radians.
- Rho (\( \rho \)): It represents the distance from the origin.
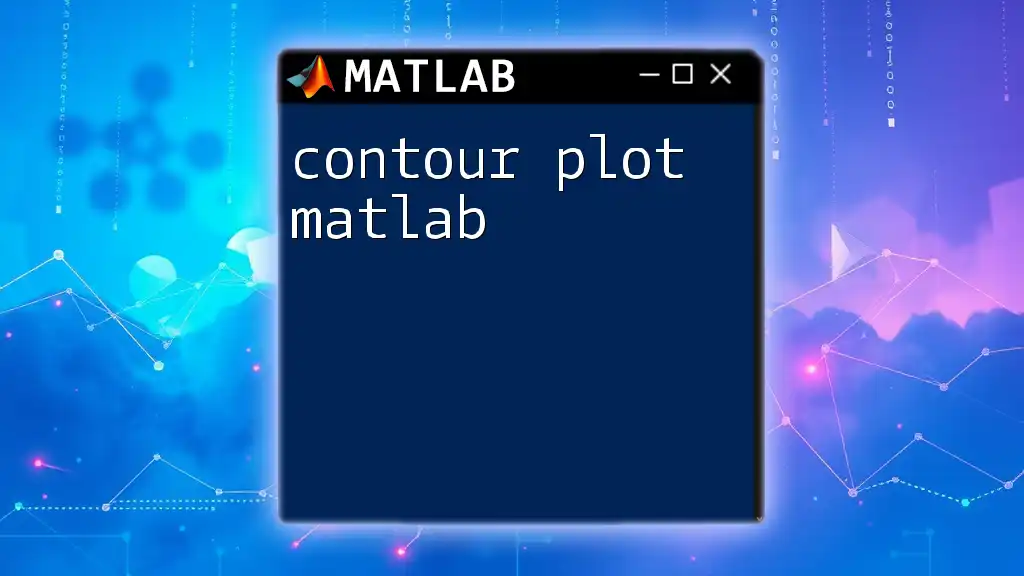
Creating Basic Polar Plots
Using the `polarplot` Function
To create a basic polar plot, you can use the `polarplot` function. This command enables you to visualize data as a function of angles and distances in a polar coordinate system.
Here’s the basic syntax to get started:
theta = linspace(0, 2*pi, 100); % Angles from 0 to 2*pi
rho = sin(theta); % Define radius as a function of theta
polarplot(theta, rho);
In this example:
- `linspace(0, 2*pi, 100)` generates 100 points between 0 and \(2\pi\).
- `sin(theta)` calculates the sine for each angle, producing a wave pattern in the polar plot.
Customizing Your Polar Plot
Adding Titles and Labels
Once you have your polar plotting set up, adding a title or customizing labels can make your plot more informative. You can achieve this easily using:
title('Polar Plot of sin(theta)');
This line adds a descriptive title to your plot, allowing viewers to understand the context quickly.
Changing Line Styles and Colors
MATLAB allows you to customize the appearance of your polar plot extensively. You can modify line styles and colors using additional parameters in the `polarplot` function. For example:
polarplot(theta, rho, 'r--'); % Red dashed line
In this command, `'r--'` specifies that the line should be red and dashed. You can experiment with various color and line style combinations to communicate your data effectively.
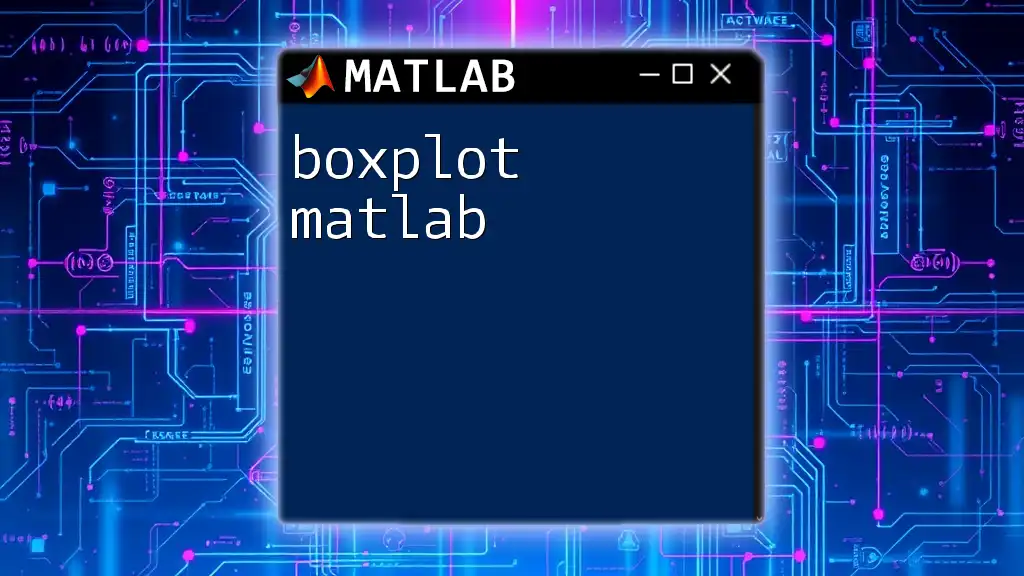
Advanced Techniques with Polar Plots
Overlaying Multiple Polar Plots
You can enhance data visualization by overlaying multiple polar plots on the same figure. This method is particularly useful when comparing different functions or datasets.
To overlay plots, use:
hold on; % Hold the current plot
polarplot(theta, cos(theta), 'b-'); % Blue solid line for cos(theta)
hold off;
Using `hold on` before creating the second plot allows MATLAB to overlay it rather than creating a new figure.
Using Custom Functions
Plotting Parametric Equations
Plotting parametric equations is another powerful feature of polar plots in MATLAB. Particularly for complex shapes like spirals, this option is valuable.
For instance, consider a spiral represented as:
theta = linspace(0, 10*pi, 100);
rho = theta; % Spiral function
polarplot(theta, rho);
This commands will create a spiral where the radius increases with the angle, offering a unique visualization of relationships.
Annotation and Custom Markers
Adding annotations helps explain data directly on the plot, improving clarity. For example, if you want to annotate a particular point, use:
polarplot(theta, rho);
text(pi/2, 1, 'Point (pi/2, 1)', 'HorizontalAlignment', 'left');
This code places a text label near the designated point on your plot, making it easier for viewers to grasp specific information.
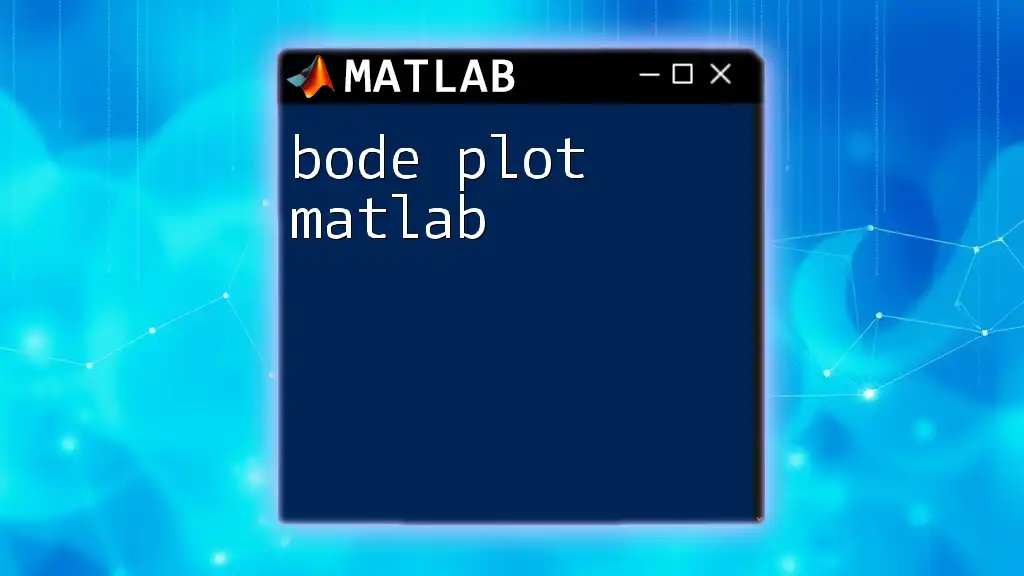
Error Handling and Common Mistakes
Understanding Polar Plot Limitations
While polar plots are powerful, they have limitations. For example, representing data with extreme values in rho can distort the visualization. It is essential to choose the right data and context to use polar plots effectively.
Debugging Common Issues
When using polar plots, users may encounter issues such as dimension mismatches between the theta and rho vectors. Always ensure that both vectors are of the same length to avoid errors. If a polar plot does not display correctly, check the values of theta and rho, and ensure they are correctly defined.
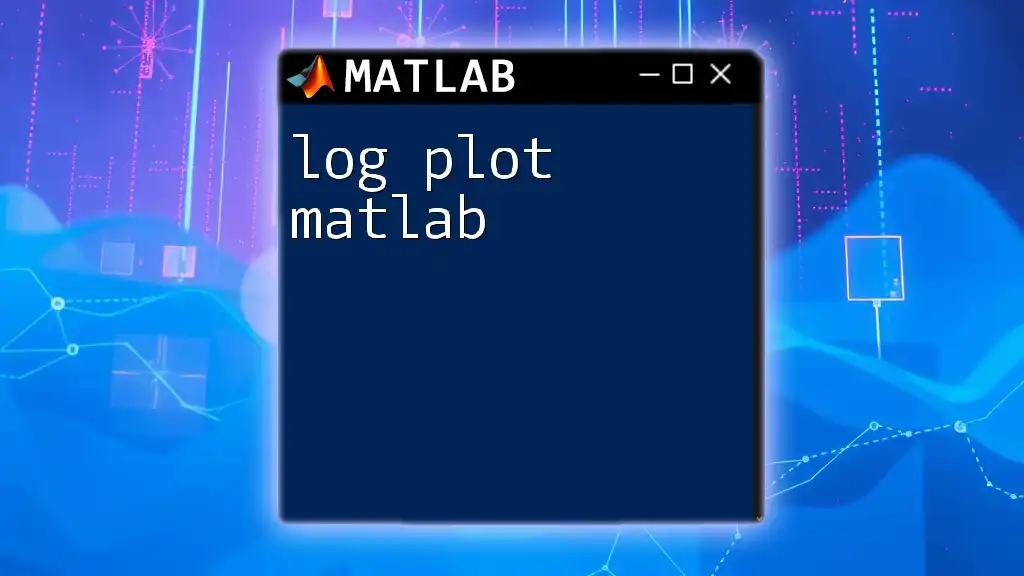
Practical Applications of Polar Plots
Examples in Engineering and Physics
Polar plots find extensive use in engineering and physics, especially for visualizing phenomena that have a circular symmetry. For example, they can illustrate the radiation pattern of antennas or represent the trajectory of rotating bodies.
Case Study: Visualizing Complex Data
Consider using polar plots for a real-world dataset, such as wind directions and speeds. For instance, utilizing the data gathered during a wind study can help visualize prevailing wind directions effectively:
theta = [0, 30, 60, 90, 120, 150, 180, 210, 240, 270, 300, 330]*pi/180; % Wind directions in radians
rho = [6, 7, 5, 8, 6, 5, 4, 7, 5, 6, 7, 8]; % Corresponding wind speeds
polarplot(theta, rho);
This code would depict a polar plot with wind directions, aiding in analyses regarding patterns of wind behavior over time.
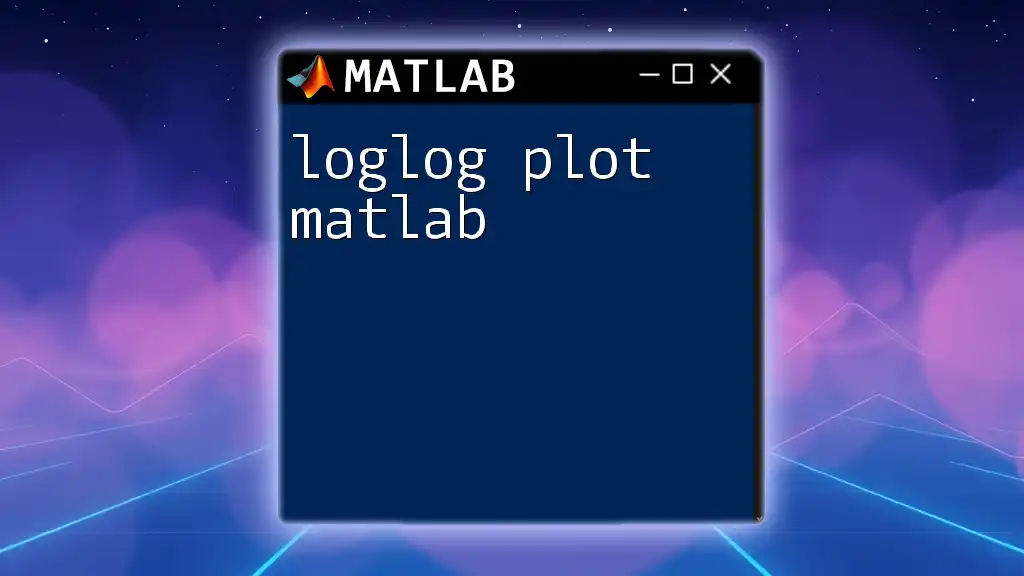
Conclusion
In summary, mastering the creation and customization of polar plots in MATLAB opens up new avenues for data visualization. From simple sine waves to complex parametric equations, you can visualize relationships that might be less clear in Cartesian representations. As you continue your journey in MATLAB, practice creating your plots, experimenting with different datasets, and utilizing advanced features to unlock the full potential of polar graphs.
To further enhance your skills in data visualization, refer to the MATLAB documentation and explore additional resources that provide in-depth learning opportunities. The key to becoming proficient lies in continuous practice and exploration of different visualization techniques.