A contour plot in MATLAB is a graphical representation of three-dimensional data in two dimensions, where contour lines represent levels of constant value, making it easier to visualize the relationships between variables.
% Example MATLAB code for creating a contour plot
[X, Y, Z] = peaks(30); % Generate sample data
contour(X, Y, Z); % Create a contour plot
title('Contour Plot Example');
xlabel('X-axis');
ylabel('Y-axis');
Understanding the Basics of Contour Plots in MATLAB
What is a Contour Plot?
A contour plot is a graphical representation used to visualize a three-dimensional surface on a two-dimensional plane. It comprises contour lines, which connect points of equal value, typically representing a specific variable across a grid of values. This type of plot provides an intuitive way to examine relationships between multiple variables and helps to identify patterns, peaks, troughs, and regions of interest in the data.
Why Use Contour Plots?
Contour plots offer several advantages for data visualization, making them an effective choice in many scenarios:
- Visual Clarity: They simplify the representation of complex surfaces, allowing easy identification of features such as minima, maxima, and saddle points.
- Comparative Analysis: Contour plots can effectively compare multiple datasets or functions by overlaying different contour plots.
- Data Interpolation: With contours, you can interpolate and estimate values between known data points without needing to resort to 3D plots.
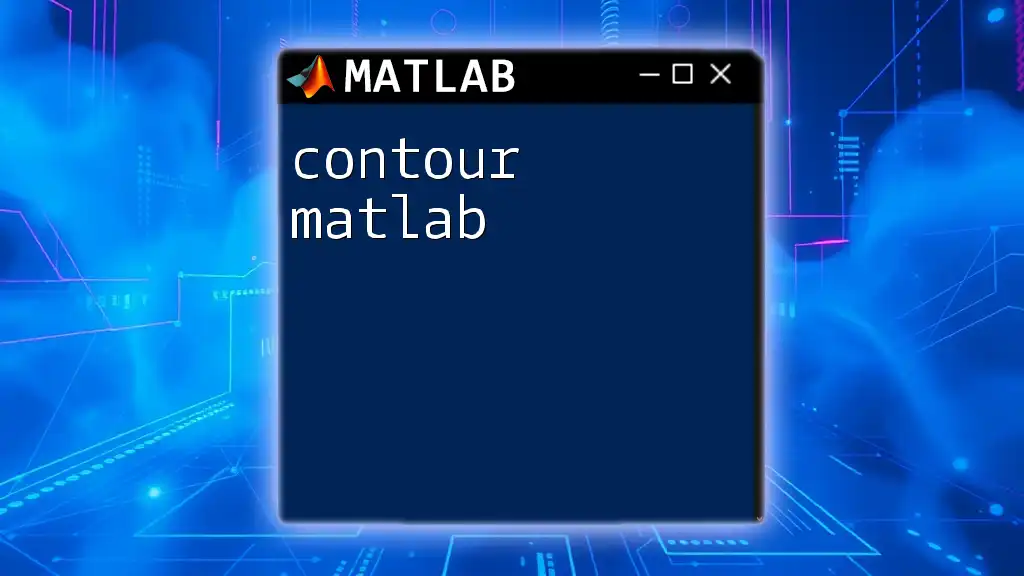
Setting Up Your MATLAB Environment
Downloading MATLAB
Before you can create a contour plot in MATLAB, ensure you have the software installed. You can download MATLAB from the official MathWorks website. After installation, familiarize yourself with the interface, focusing on the Command Window where you’ll execute commands.
MATLAB Basics
Grasping some foundational commands is helpful in creating effective contour plots. Familiarize yourself with commands such as `figure`, which opens a new figure window, and `hold on`, which allows for overlaying multiple plots in the same figure without erasing previous plots.
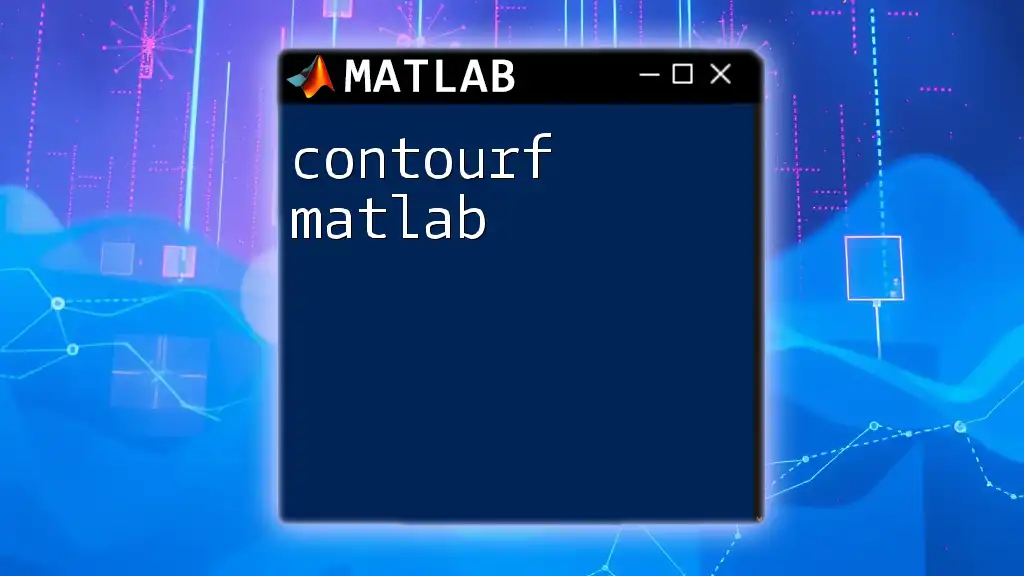
Creating Your First Contour Plot
Simple Example of a Contour Plot
Creating your first contour plot in MATLAB is straightforward. Begin with defining the grid of values over which you want the contour to be plotted. Here’s a simple example:
[X, Y] = meshgrid(-5:0.5:5, -5:0.5:5);
Z = sin(sqrt(X.^2 + Y.^2));
contour(X, Y, Z);
In this example:
- We create a grid of `X` and `Y` values using `meshgrid`, ranging from -5 to 5 with an interval of 0.5.
- The function `Z` computes the sine of the square root of these grid coordinates, producing a set of `z` values for our contours.
- Finally, the `contour` function generates the plot.
Understanding the Output
When the above code is executed, MATLAB produces a contour plot where each contour line represents a different value of the function `Z`. The spacing of the lines can indicate the steepness of the surface; closely spaced lines suggest a rapid change in values, while widely spaced lines indicate a slower change.
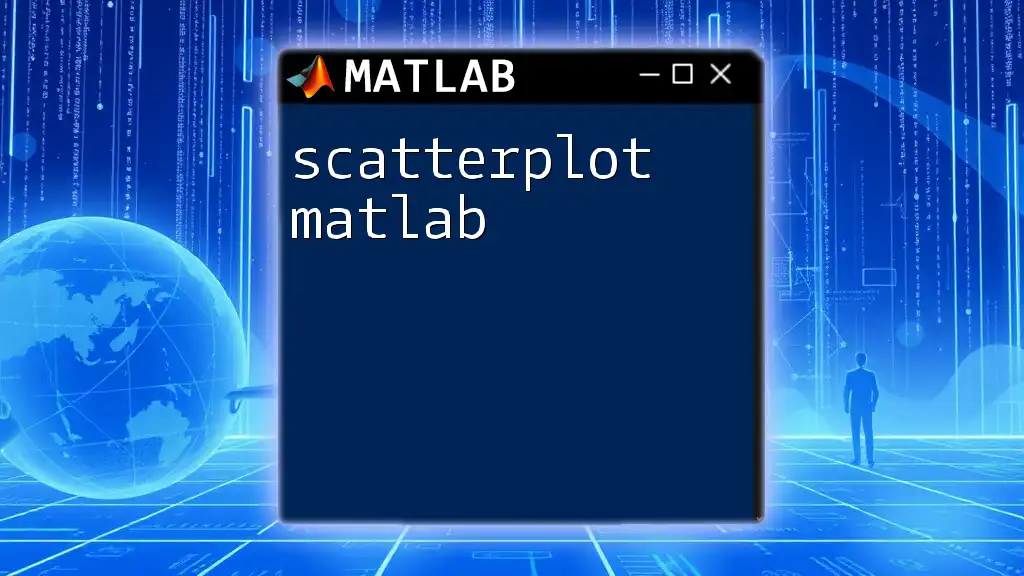
Customizing Your Contour Plot
Color Customization
To enhance the visual appeal and clarity of your contour plot, you can change the colors using the `colormap` function. This allows you to define a color palette that best suits the data being represented. For example, to use the 'jet' colormap, you can add the following line of code:
colormap(jet);
Adding Labels and Titles
Incorporating titles and labels is crucial for making your plot more informative. Use commands such as `title`, `xlabel`, and `ylabel` to provide context to your contours. For example:
title('Contour Plot of Sin Function');
xlabel('X-axis Label');
ylabel('Y-axis Label');
This improvement ensures that viewers understand what the plot represents.
Adjusting Levels in Contour Plots
By specifying levels, you can define how many contour lines to display or to highlight particular ranges of values. To set a specific number of levels, you can modify the `contour` command:
contour(X, Y, Z, 20); % 20 levels
In this case, MATLAB will create 20 equally spaced contour levels based on the `Z` data.
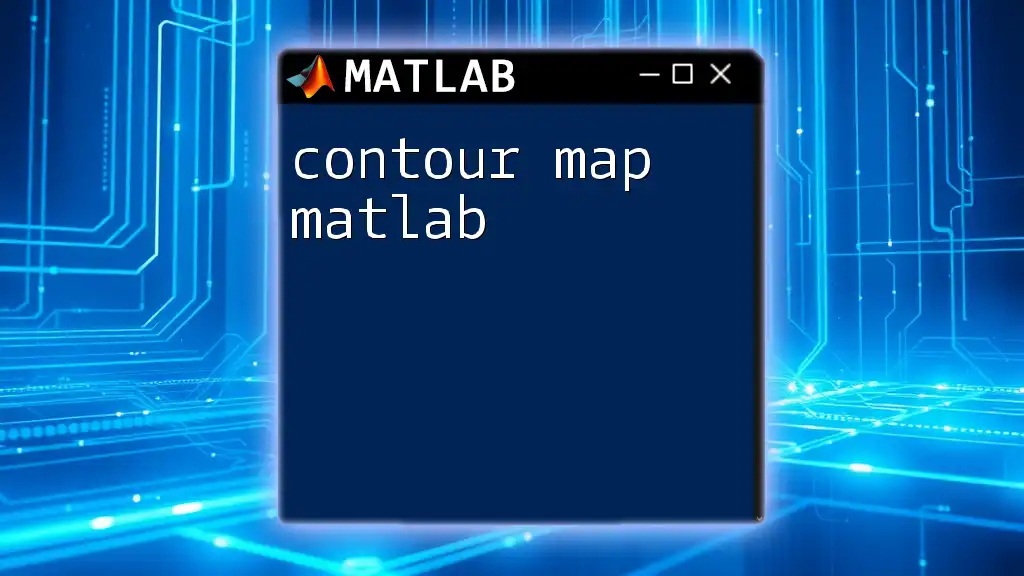
Advanced Features of Contour Plots
Filled Contour Plots
Using filled contour plots can emphasize specific regions of interest. Instead of displaying just the contour lines, filled contours provide color-coded regions, which can be particularly useful for visualizing complex datasets. Use the `contourf` function as shown below:
contourf(X, Y, Z);
colorbar;
The `colorbar` command adds a legend to describe the color scale used in the filled contours.
Overlapping Contours
You can also overlay multiple contour plots to compare different datasets. Here’s how to create overlapping contours:
Z2 = cos(sqrt(X.^2 + Y.^2));
hold on;
contour(X, Y, Z2, 'LineStyle', '--');
hold off;
This example overlays the contour plot of a cosine function on top of the previous sine function plot, showcasing how different datasets can be visualized simultaneously.
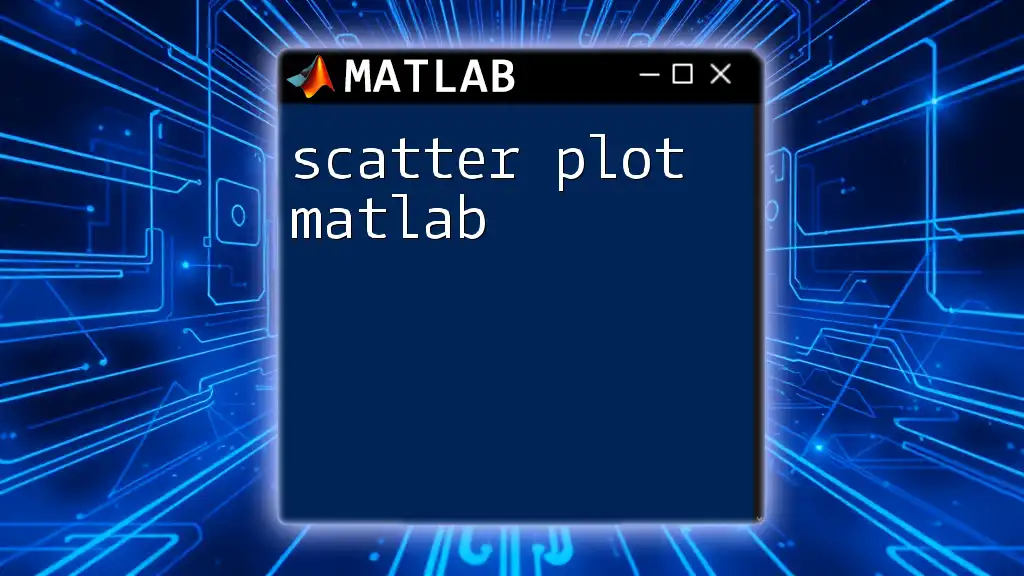
Practical Applications of Contour Plots
Use Cases in Engineering and Science
Contour plots are widely employed across various fields. In meteorology, they can illustrate rainfall distribution; in geography, they are useful for depicting terrain elevation; and in engineering, they can show stress distributions or fluid flow patterns. Their application across these fields underscores their versatility in data analysis and visualization.
Interpreting Contour Plots in Real-World Scenarios
Consider a case study in environmental science where a researcher uses contour plots to visualize air quality data across a city. Various pollutant levels can be displayed effectively, illustrating hotspots of pollution that require attention. Such visual interpretation helps policymakers identify areas in need of intervention.
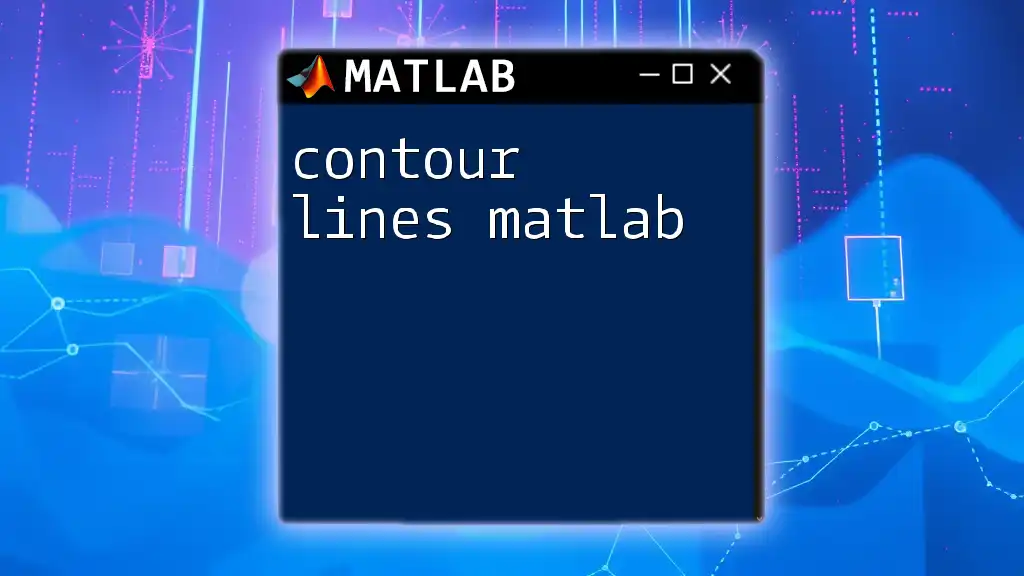
Troubleshooting Common Issues
Common Errors
When working with contour plots, you might encounter errors such as incompatible matrix dimensions. Always ensure that the dimensions of your `X`, `Y`, and `Z` matrices match. A quick solution is to use the `size()` function to verify matrix dimensions before executing the `contour` function.
Optimizing Performance for Large Datasets
Handling large datasets can slow down rendering times. To optimize performance, consider downsampling your data or employing MATLAB’s built-in functions like `griddata` to interpolate data seamlessly while reducing the computational load.
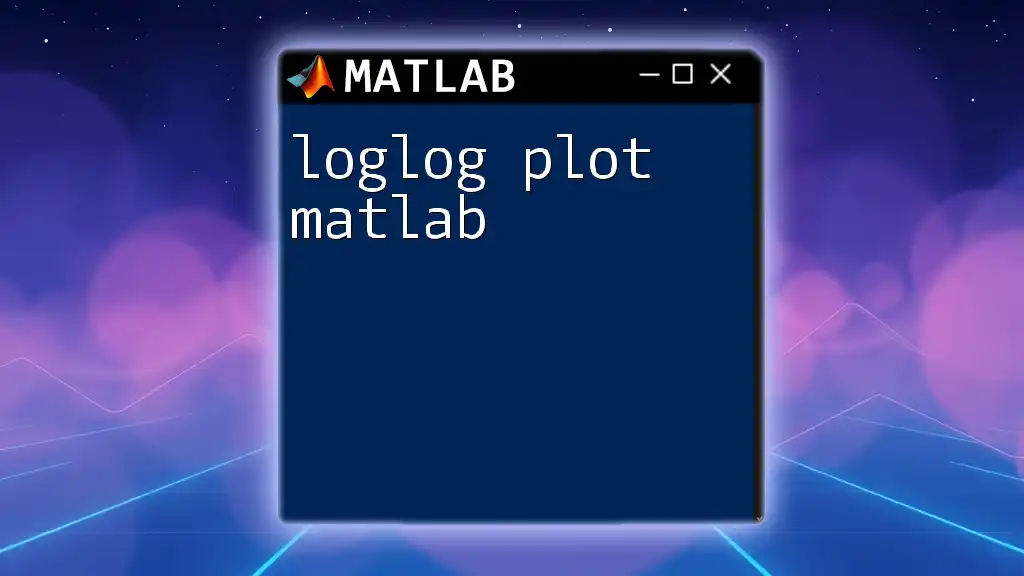
Conclusion
Contour plots are an essential tool in MATLAB for visualizing complex data across multiple dimensions. Their ability to communicate intricate relationships through contour lines makes them invaluable for analysis in various fields. As you dive deeper into using contour plots in MATLAB, don't hesitate to explore their customization and advanced features, enhancing both their functionality and visual appeal.
By experimenting with the examples provided and applying your creativity, you can leverage contour plots to tell compelling stories through your data.
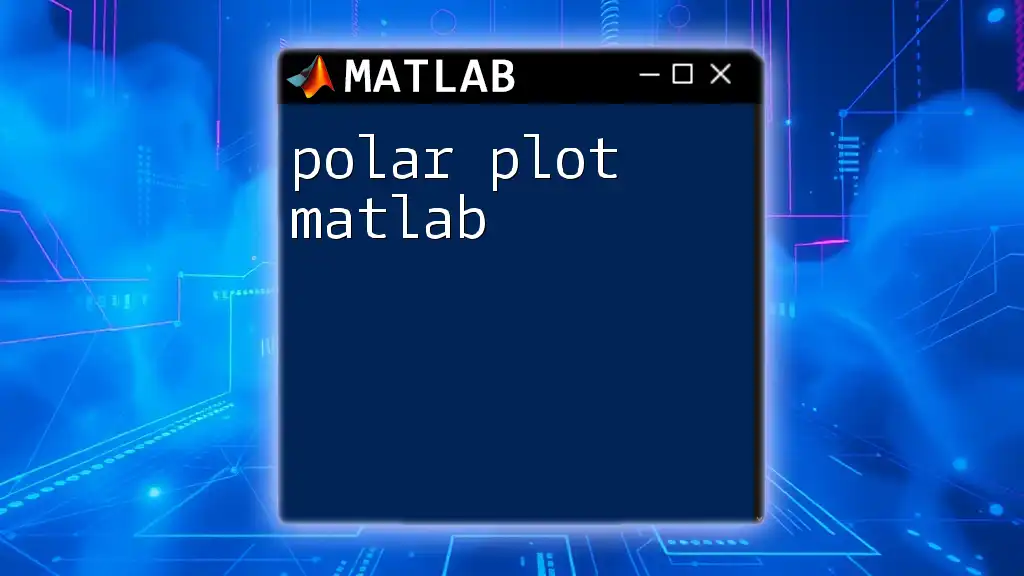
Additional Resources
For further reading and learning material, refer to the MATLAB documentation as it offers extensive details on plotting functions, syntax, and customization options. Engaging with the MATLAB community can also provide additional insights and support as you proceed with your journey in mastering contour plots and other MATLAB functions.