In MATLAB, you can compare strings using the `strcmp` function, which returns a logical true (1) if the strings are identical and false (0) if they are not.
% Example of comparing two strings in MATLAB
str1 = 'hello';
str2 = 'world';
isEqual = strcmp(str1, str2); % Returns false (0)
Understanding Strings in MATLAB
What are Strings?
In MATLAB, strings are sequences of characters, often used to represent text data. They can be created in different ways: using double quotes for string arrays and single quotes for character arrays. For example, a string array can be created as follows:
strArray = ["Hello", "World"];
In contrast, a character array is defined like this:
charArray = 'Hello World';
Understanding the difference between these two is crucial because they are processed differently in MATLAB. While string arrays are more versatile and come with a richer set of functions, character arrays are traditional and still widely used.
How MATLAB Handles Strings
MATLAB provides a variety of built-in functions specifically designed for effective string manipulation. These functions allow you to work with strings intuitively, taking much of the complexity out of string processing.
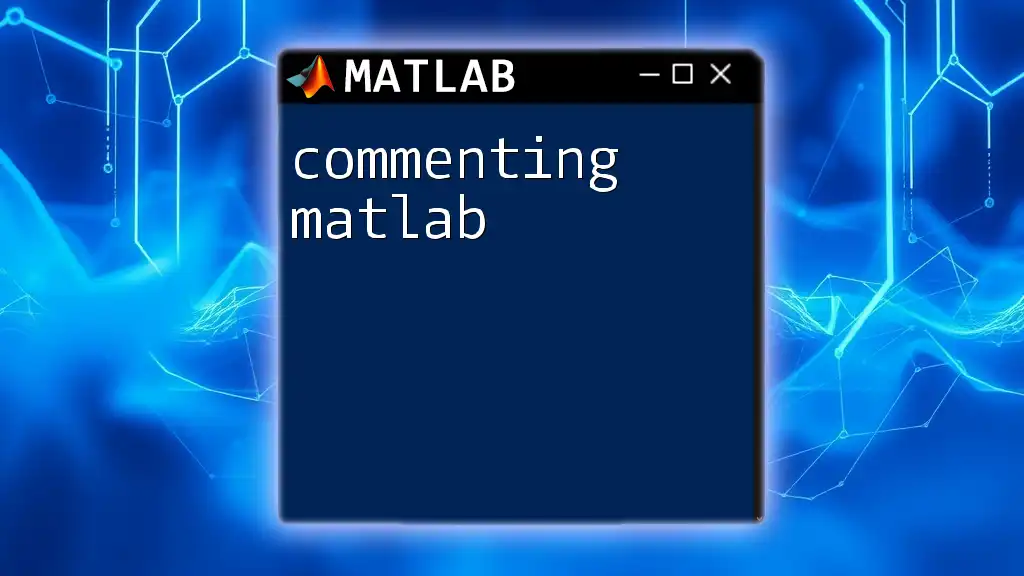
String Comparison in MATLAB
Why Compare Strings?
String comparison is a fundamental operation in many programming scenarios. You might want to verify user input, validate data, or implement search algorithms. In investigating relationships between text data, string comparisons often serve as the backbone of the functionality.
The Basic Functions for String Comparison
strcmp
The `strcmp` function is the most fundamental method for comparing two strings in MATLAB. This function is case-sensitive and returns `true` (1) if the two strings are identical, and `false` (0) otherwise.
Syntax:
strcmp(string1, string2)
Example:
str1 = 'hello';
str2 = 'hello';
result = strcmp(str1, str2); % result is true
In this example, `result` will be `true` because both strings are identical. However, if you change `str2` to `Hello`, `result` will return `false` because of the case difference. Being aware of case sensitivity is essential when performing comparisons.
strcmpi
If you want to ignore case differences in your string comparisons, the `strcmpi` function is your go-to option. This function operates similarly to `strcmp`, but it treats upper and lower case letters as equal.
Syntax:
strcmpi(string1, string2)
Example:
str1 = 'Hello';
str2 = 'hello';
result = strcmpi(str1, str2); % result is true
This time, `result` is `true`, demonstrating that `strcmpi` provides flexibility when the case is not a concern.
Other Useful Comparison Functions
strncmp and strncmpi
For scenarios where you only want to compare a certain number of characters, `strncmp` and `strncmpi` come into play. This is especially useful for partial matches.
Syntax:
strncmp(string1, string2, n)
strncmpi(string1, string2, n)
Example:
str1 = 'hello world';
str2 = 'hello MATLAB';
result = strncmp(str1, str2, 5); % result is true
In this instance, `result` will confirm that the first five characters of both strings are identical. It’s a powerful tool for validating user input or aligning datasets.
Testing for Substrings
contains
Another frequent requirement in string operations is checking for the presence of a substring in a string. The `contains` function fulfills this need effectively.
Syntax:
contains(string, substring)
Example:
str = 'Hello World';
result = contains(str, 'World'); % result is true
The above example yields `true`, indicating that "World" is indeed part of the string. This function is simple yet effective for tasks like extracting keywords or validating input data.
strfind
If you need more detailed information about the location of a substring within a string, `strfind` is the function to use. It returns the starting index of the first occurrence of a substring.
Syntax:
strfind(string, substring)
Example:
str = 'Program in MATLAB';
index = strfind(str, 'MATLAB'); % index returns the position
In this example, `index` will show the starting index where "MATLAB" appears in the string. This can be particularly useful in parsing text or manipulating strings based on their content.
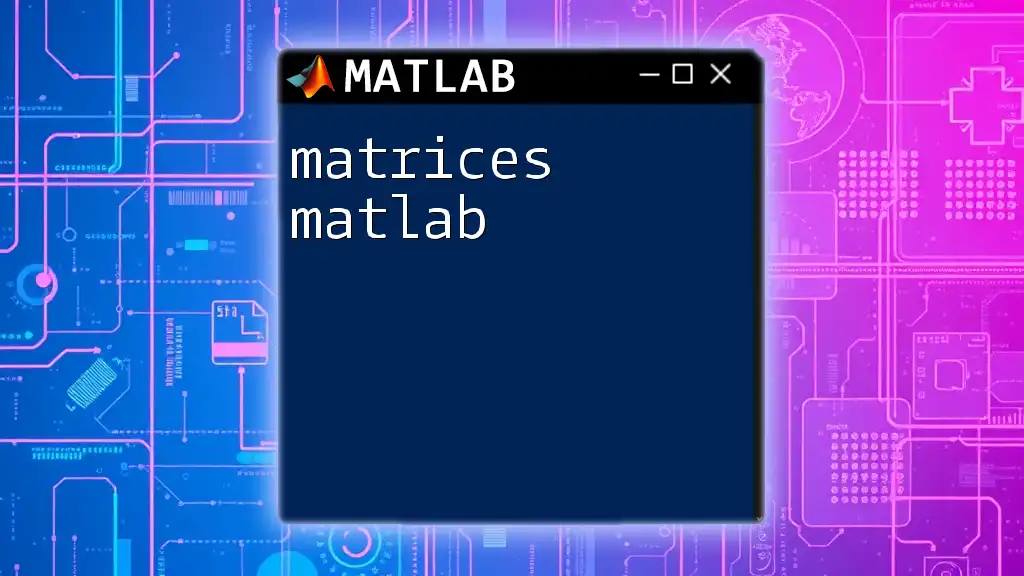
Working with String Arrays
Comparison Operations on String Arrays
When working with string arrays, you can directly compare elements using the `==` operator. This gives you a quick way to check for equality across arrays.
Example:
stringArray1 = ["apple", "banana"];
stringArray2 = ["apple", "orange"];
comparisonResult = stringArray1 == stringArray2; % returns logical array
In this case, `comparisonResult` will be a logical array that indicates which corresponding elements are the same. This functionality is useful for comparing large datasets where efficiency is key.
Combining Comparison Functions with Arrays
You can further enhance string comparison by combining functions. For instance, you might want to compare string arrays conditionally. This opens up a variety of logical operations, allowing for sophisticated data analysis tasks.
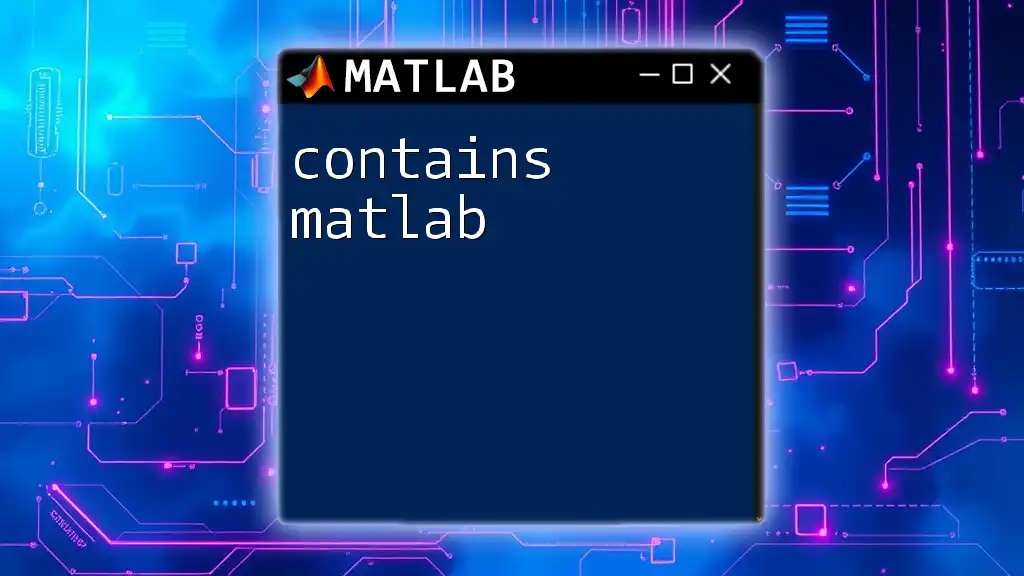
Performance Considerations
Efficiency of String Comparison Functions
As you work with larger datasets, being mindful of performance becomes crucial. Some string comparison functions might be computationally intensive, especially when applied repeatedly or to long strings. To optimize performance, consider pre-filtering data to reduce the number of comparisons needed, and choose functions that suit your specific application scenario.
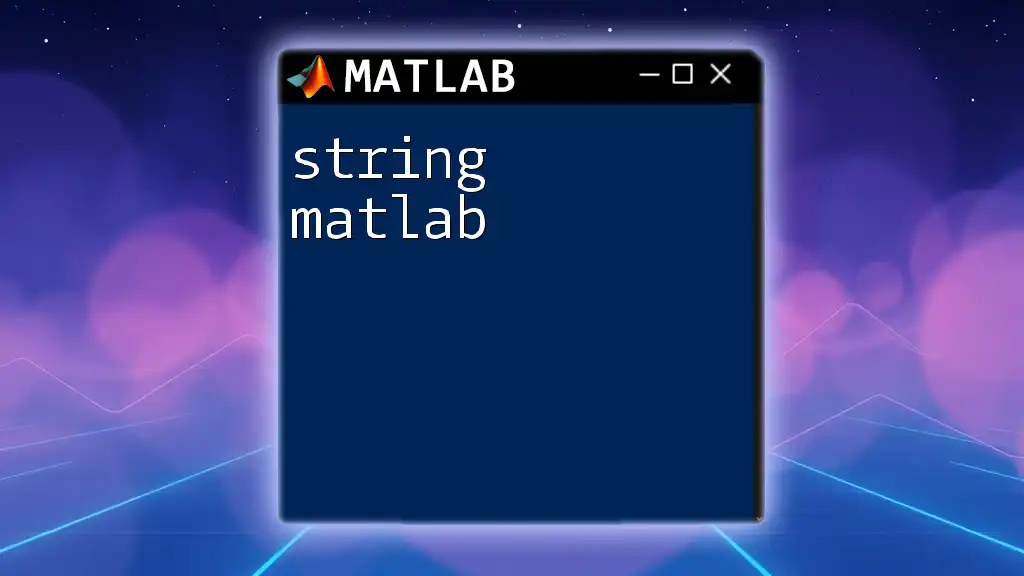
Conclusion
Throughout this guide, we have explored various ways to compare strings in MATLAB. From basic functions like `strcmp` and `strcmpi` to substring searches with `contains` and `strfind`, MATLAB provides a robust toolkit for string manipulation. Understanding how to effectively leverage these functions can greatly enhance your data analysis and programming efficiency.
As you practice with the examples outlined here, remember that mastering these commands will boost your proficiency with MATLAB. Exploring further resources and real-world applications can solidify your grasp on this essential topic.