In MATLAB, you can convert a character array (char) to a string using the `string` function. Here’s a simple example:
charArray = 'Hello, World!';
str = string(charArray);
Understanding Character Arrays and String Arrays
What are Character Arrays?
In MATLAB, a character array (or char array) is a sequence of characters stored in a matrix format, where each character occupies one element. These arrays are versatile and can be used in various contexts, particularly in text processing and manipulation.
You can create a character array using simple syntax. For example:
charArray = 'Hello, World!';
This command creates a 1-by-13 character array containing the string "Hello, World!". When you work with character arrays, it's essential to remember that they are treated as rows of characters.
What are String Arrays?
String arrays were introduced in MATLAB R2016b as a new data type that allows for more flexibility in handling text data. Unlike character arrays, string arrays are designed for more complex string manipulation and require less fuss.
Here’s how to create a string array:
strArray = "Hello, World!";
This command initializes a string array containing the same text. The key benefit of using string arrays is their ability to hold a collection of strings more naturally and intuitively.
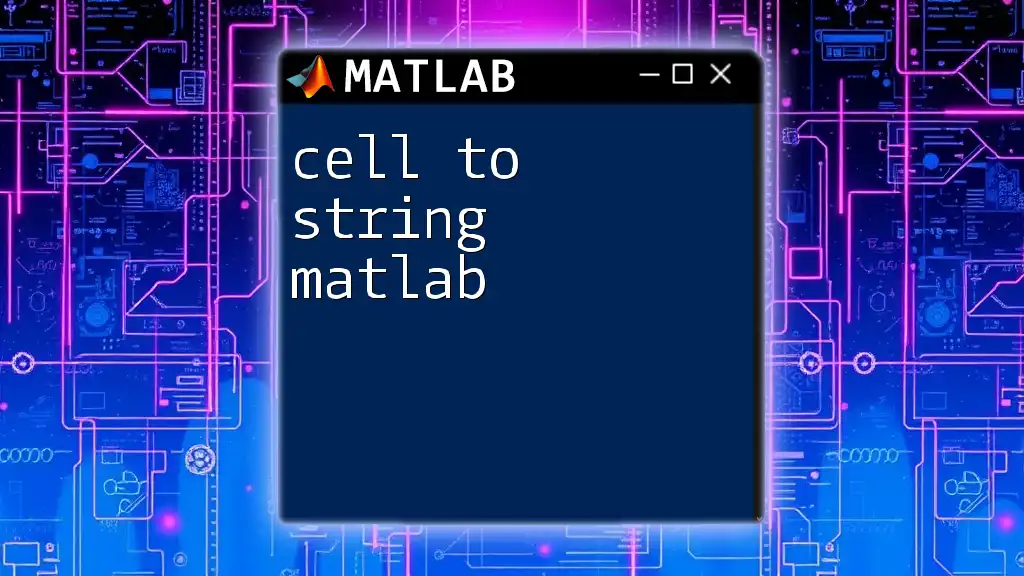
The Conversion Process: Char to String
Why Convert Char to String?
Understanding when to convert character arrays to string arrays is crucial for maximizing efficiency in your MATLAB codes. Some functions and operations explicitly require string inputs, while others are optimized for string types rather than character arrays. For example, functions associated with the string processing toolbox often accept string arrays as inputs.
Methods for Conversion
Using the `string` Function
The primary method to convert from a character array to a string array in MATLAB is through the built-in `string` function. This function takes a character array as an argument and converts it to a string array.
Here’s how to use it:
charArray = 'Hello, World!';
strArray = string(charArray);
Explanation: In this example, the character array `charArray` is converted into a string array called `strArray`. The transformation is straightforward and efficient, allowing you to seamlessly integrate this data into further string-processing tasks.
Implicit Conversion
In some situations, MATLAB allows for implicit conversion from character arrays to string arrays. This auto-conversion can be handy but could also lead to confusion if you're not aware it's happening.
For example:
charArray = 'MATLAB';
strArray = charArray + " is fun!";
Explanation: Here, the operation combines a character array with a string, and MATLAB implicitly converts `charArray` into a string array. This results in `strArray` containing "MATLAB is fun!". Understanding this feature helps prevent unintentional errors, especially when working with mixed data types.
Other Methods and Techniques
Using `cellstr` for Special Cases
When you are working with multidimensional character arrays or need to convert characters to cell arrays, the `cellstr` function becomes invaluable. This function converts a character array into a cell array of strings, which can be particularly useful in various applications.
Here is an example:
charArray = 'Hello, World!';
cellArray = cellstr(charArray);
In this example, `cellArray` will contain strings, but housed within individual cells.
Handling Multidimensional Character Arrays
When dealing with multidimensional character arrays, conversion to string arrays varies slightly. You can still use the `string` function; however, keep in mind that the output will be different.
charMatrix = ['Hello'; 'World'];
strArray = string(charMatrix);
Explanation: This command creates a string array where each row of `charMatrix` becomes a separate string in `strArray`. Ultimately, your string array will be structured correctly and ready for string operations.
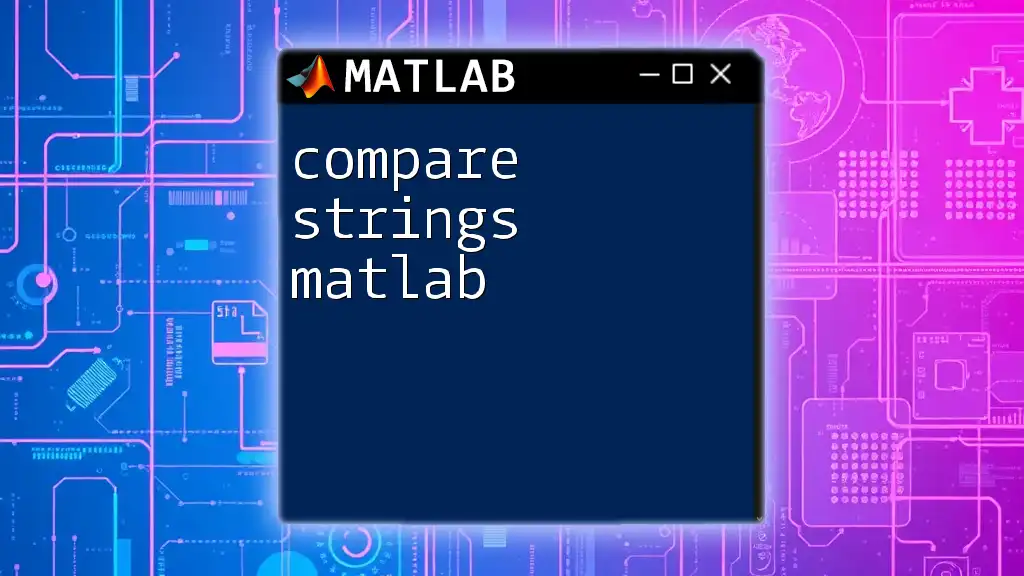
Practical Applications and Examples
Example Use Case 1: Text Processing
Text processing is a common application where converting from a character array to a string array is beneficial. Consider this simple text processing task:
textChar = 'Analyze this sentence.';
textStr = string(textChar);
% Further processing
upperTextStr = upper(textStr); % Convert to uppercase
Explanation: In this example, `textChar` is converted into `textStr`, which is then transformed to uppercase using the `upper` function—a task that typically benefits from leverage on string arrays.
Example Use Case 2: GUI Applications
MATLAB provides built-in functions for GUI applications, many of which require arguments to be string types. A quick example is:
uicontrol('Style', 'text', 'String', string('Welcome!'));
Explanation: This line of code creates a text control in a MATLAB GUI that displays the string "Welcome!". As you can see, using a string type enhances the clarity and functionality of your GUI.
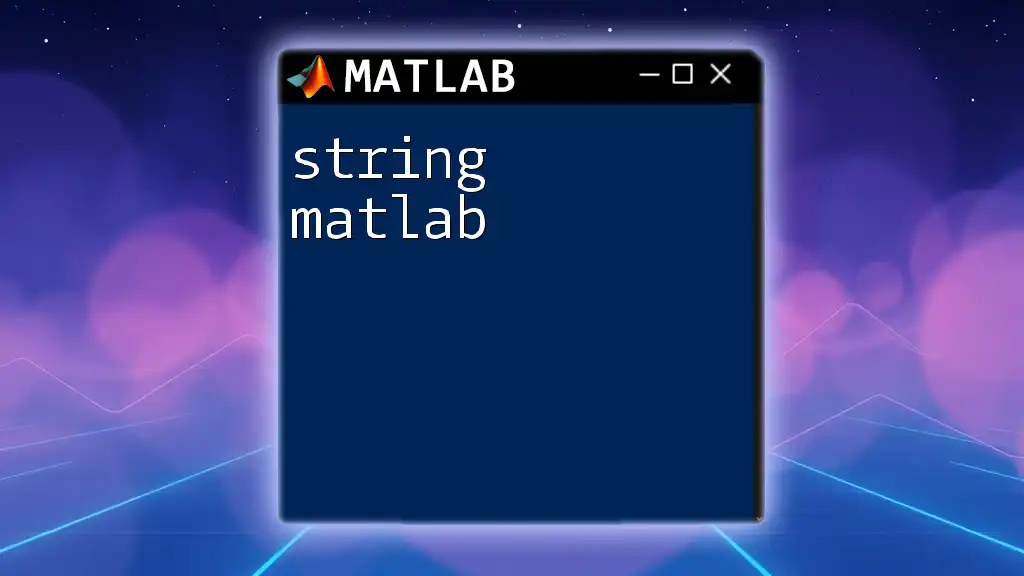
Common Pitfalls and Best Practices
When converting from character arrays to string arrays, users might encounter some pitfalls. Common mistakes include:
-
Forgetting Conversion: Using functions that are string-specific while still working with character arrays can lead to errors. Always remember to convert explicitly if you are unsure.
-
Data Integrity: Be vigilant about maintaining data integrity during conversion; verify that the string outputs align with your expectations, especially during batch processing.
Best Practices:
-
Consistently Use String Types: Wherever possible, adopt string arrays for new projects, as they offer enhanced functionality and simpler syntax.
-
Comment Your Code: Explain conversion steps with comments in your code to maintain clarity, benefiting both you and your team.
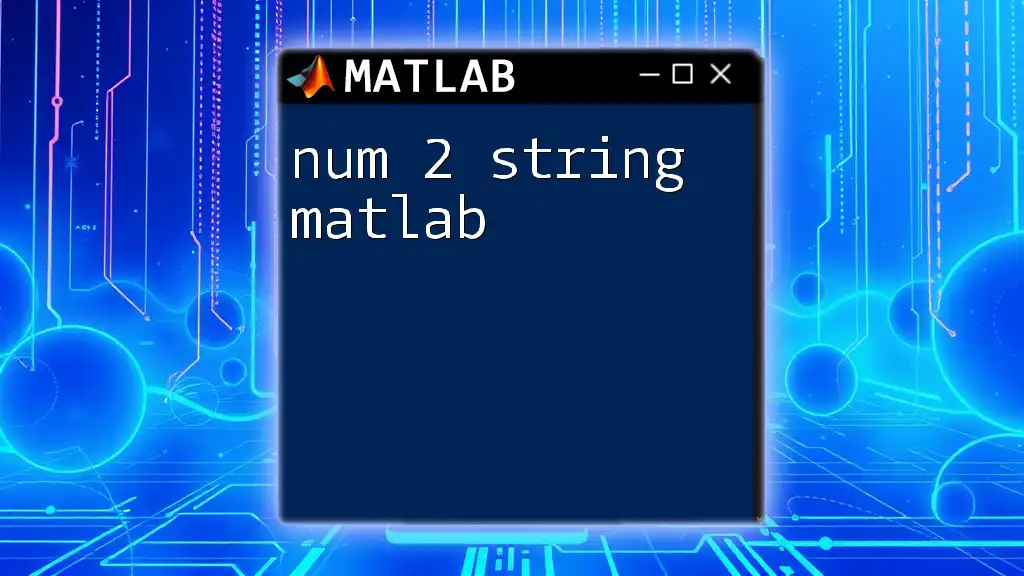
Conclusion
Understanding the intricacies of converting char to string in MATLAB is vital for any MATLAB user looking to enhance their programming efficiency. This comprehensive guide provides insight into the definitions, processes, methods, and practical examples of character and string arrays. By mastering these concepts, you set yourself up for success in handling text-related tasks seamlessly.
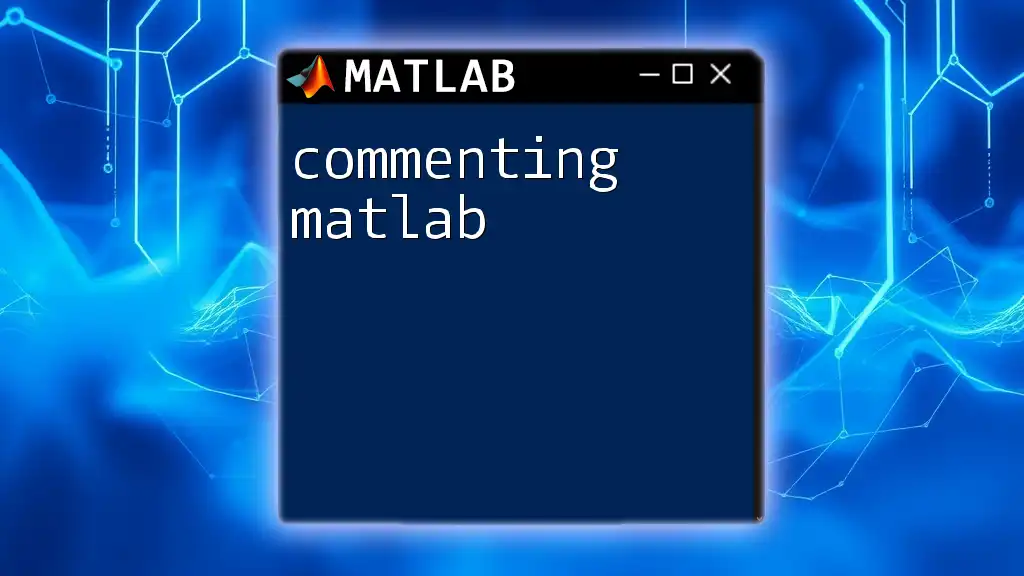
Additional Resources
For further exploration on this topic, consider referring to the official MATLAB documentation, which offers extensive information on string functions and various text manipulation options available in MATLAB.