In MATLAB, you can convert a number to a string using the `num2str` function, which allows for easy manipulation and display of numerical data as text.
num = 42; % Example number
str = num2str(num); % Convert number to string
Understanding Data Types in MATLAB
What is a Number in MATLAB?
In MATLAB, a number can be represented using different numerical data types such as double, single, and int. The default data type for numbers in MATLAB is double, which provides a high degree of precision suitable for calculations. Here’s an example of declaring a numeric variable:
x = 5; % A simple double type variable
This variable can be used in mathematical operations like any standard arithmetic.
What is a String in MATLAB?
Strings in MATLAB are sequences of characters stored in either character arrays or as string arrays (introduced in later versions). A character array is created using single quotes, while a string array uses double quotes. Here’s a quick example to illustrate both:
charArray = 'Hello world!'; % A character array
stringArray = "Hello world!"; % A string array
Understanding the difference between these two representations is crucial, especially in scenarios where you may need to manipulate or format string data.
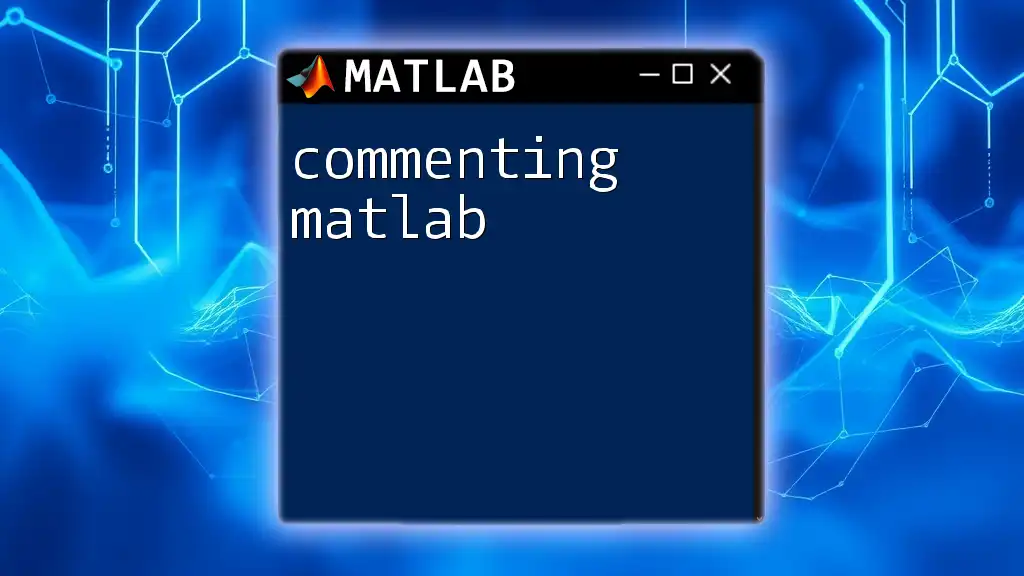
Why Convert Numbers to Strings?
Converting numbers to strings is often necessary for various programming tasks. For instance:
- Formatting Output for Display: When presenting numerical results, strings allow for controlled formatting.
- Creating Dynamic Variable Names: When dealing with loops or generating names dynamically, converting numbers to strings is essential.
- Preparing Data for File Export: Many file formats, like CSV, require strings for proper data representation.
These conversions facilitate better data handling and usability in real-world applications.
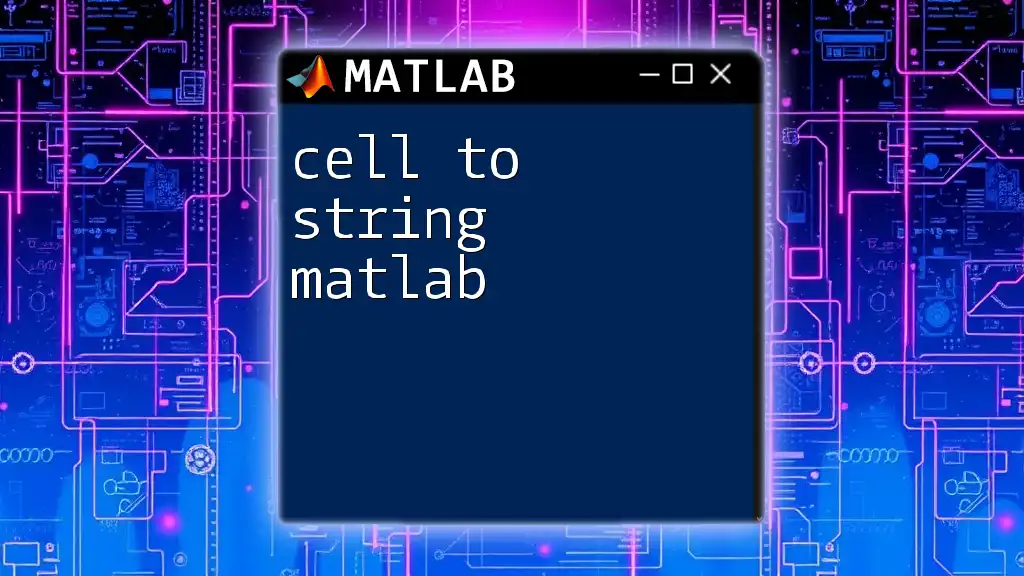
Methods for Converting Numbers to Strings in MATLAB
Using the `num2str` Function
The `num2str` function is a straightforward and widely used method to convert numbers into strings. Its basic syntax is:
str = num2str(X);
Here's a simple example to illustrate how `num2str` works:
x = 5;
str_x = num2str(x); % Output: '5'
You can also convert arrays of numbers into a single string:
array = [1, 2, 3];
str_array = num2str(array); % Output: '1 2 3'
Formatting with `num2str`
The `num2str` function also allows you to control the formatting of the converted strings. For precise formatting, such as specifying the number of decimal places, you can use:
pi_value = pi;
str_pi = num2str(pi_value, '%.2f'); % Output: '3.14'
This is particularly useful in scientific and engineering applications where precision is paramount.
If you need to express large or very small numbers in scientific notation, `num2str` can handle that as well:
large_number = 123456789;
str_large = num2str(large_number, '%.2e'); % Output: '1.23e+08'
Using the `sprintf` Function
The `sprintf` function provides even more formatting flexibility. It allows for formatted output using various placeholders. Here's the general syntax:
str = sprintf(format, A);
Using `sprintf`, you can combine strings and numbers effectively. Here’s an example:
value = 10;
formatted_string = sprintf('The value is %d', value); % Output: 'The value is 10'
This approach is beneficial when you need to create complex strings that incorporate multiple variables.
Using the `compose` Function
Another useful function for converting numbers to strings is `compose`, introduced in more recent versions of MATLAB. It enables the seamless combination of numeric and string data with a simple syntax:
str = compose("Value: %d", A);
Here’s a practical example of using `compose`:
str_value = compose("The number is %d", 42); % Output: 'The number is 42'
This function simplifies the creation of formatted strings, making it easier to concatenate multiple elements.
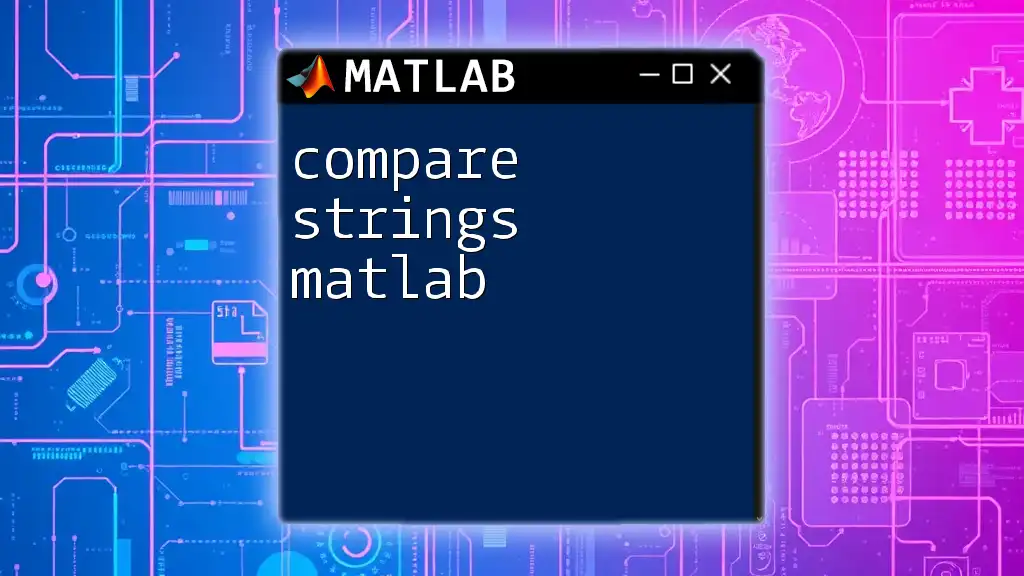
Practical Applications
Displaying Numeric Results
The ability to convert numbers to strings is crucial when displaying results. It helps create more informative outputs. For instance:
result = 100;
disp(["The final score is: " num2str(result)]);
This method enhances user experience by producing clear and descriptive outputs.
File I/O Operations
Another significant application of the `num to string matlab` functionality is in file input/output operations. When exporting numeric data to text files, converting numbers to strings ensures the data is formatted correctly. Here’s an example of writing numeric data to a text file:
numbers = [10, 20, 30];
fid = fopen('output.txt', 'w');
fprintf(fid, '%s\n', num2str(numbers));
fclose(fid);
This ensures that all numeric values are properly written and formatted in the output file.
Dynamic Variable Naming
In situations where dynamic variable names are needed (for example, during loops), converting numbers to strings plays a vital role. Using the `assignin` function, you can dynamically create variable names based on numerical values:
for i = 1:5
assignin('base', ['var' num2str(i)], i*2);
end
This code snippet generates variables `var1`, `var2`, etc., in the workspace, each storing a corresponding numeric value.
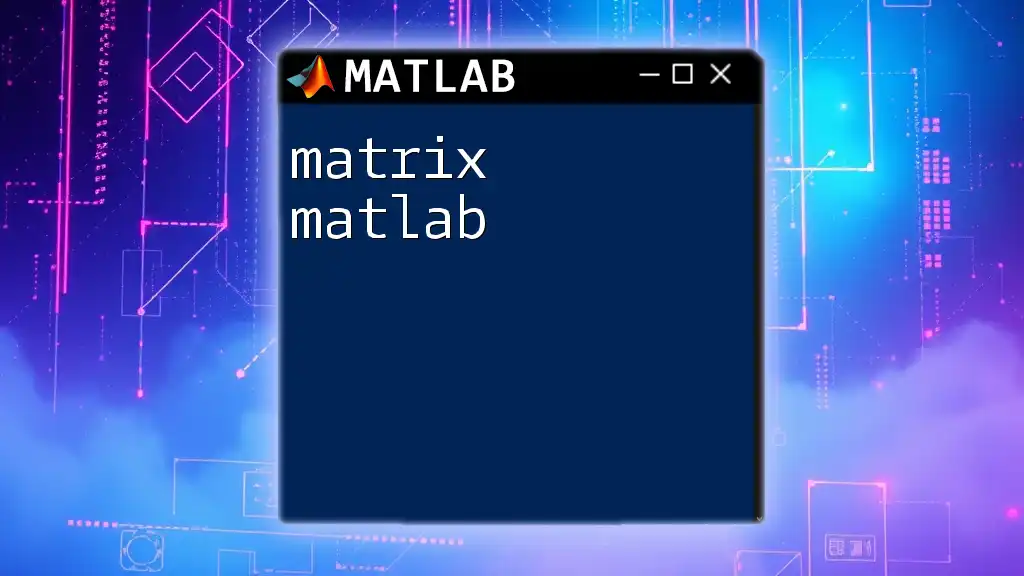
Common Issues and Troubleshooting
Precision Loss in Conversion
When converting large numbers or floating-point numbers, precision loss can occur. It’s vital to be aware of this when formatting strings, especially in applications requiring high accuracy.
Formatting Errors
Formatting strings incorrectly can lead to unexpected outcomes. It's important to use the `whos` command to check variable types and understand how they are being formatted. Always test your formatting strings with sample values to ensure they behave as intended.
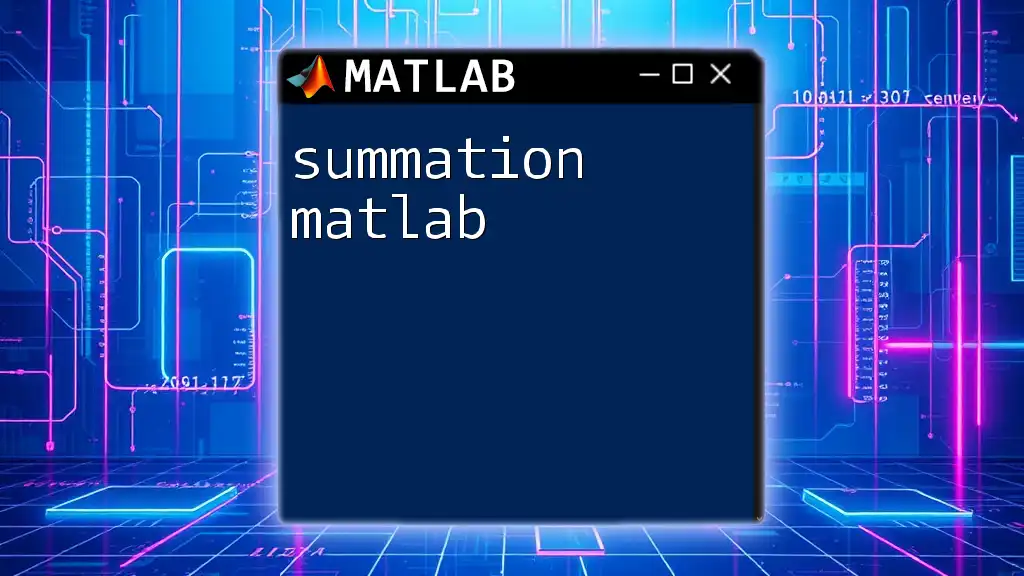
Conclusion
In summary, understanding how to convert numbers to strings using various methods such as `num2str`, `sprintf`, and `compose` is an essential skill in MATLAB programming. These conversions not only enhance the clarity of output but also enable more complex data manipulations and presentations. As you dive deeper into MATLAB, practicing these conversions will significantly improve your coding efficiency and effectiveness.
With the insights and examples provided, you are now equipped to navigate the complexities of data type conversion involving numeric values and strings. Embrace these techniques, and your MATLAB experience will become more powerful and intuitive.