In MATLAB, you can convert a cell array of strings to a single concatenated string using the `strjoin` function, as shown in the following example:
cellArray = {'Hello', 'World', 'from', 'MATLAB'};
combinedString = strjoin(cellArray, ' ');
What are Cell Arrays?
Definition of Cell Arrays
In MATLAB, a cell array is a versatile data structure that allows for the storage of arrays of varying types and sizes. Unlike regular arrays that can hold only one data type (like integers or strings), cell arrays can hold a mix of different data types. Each element of a cell array can be accessed using curly braces `{}`, which differentiates them from standard arrays that use parentheses `()`.
For example, if you have a cell array that contains numbers, strings, and even another cell array, MATLAB can handle it all without any issues.
When to Use Cell Arrays
Cell arrays are particularly useful when your data contains elements of different types. Suppose you're working with a dataset that includes both text labels and numerical data; a cell array will allow you to keep this data organized and easily accessible. Common applications of cell arrays include:
- Text processing: Storing strings and manipulating them for analysis.
- Data collection: Grouping different types of data, such as labels and measurements, into a single data structure.
- Dynamic data: When the size and type of your data can change frequently, cell arrays provide flexibility.
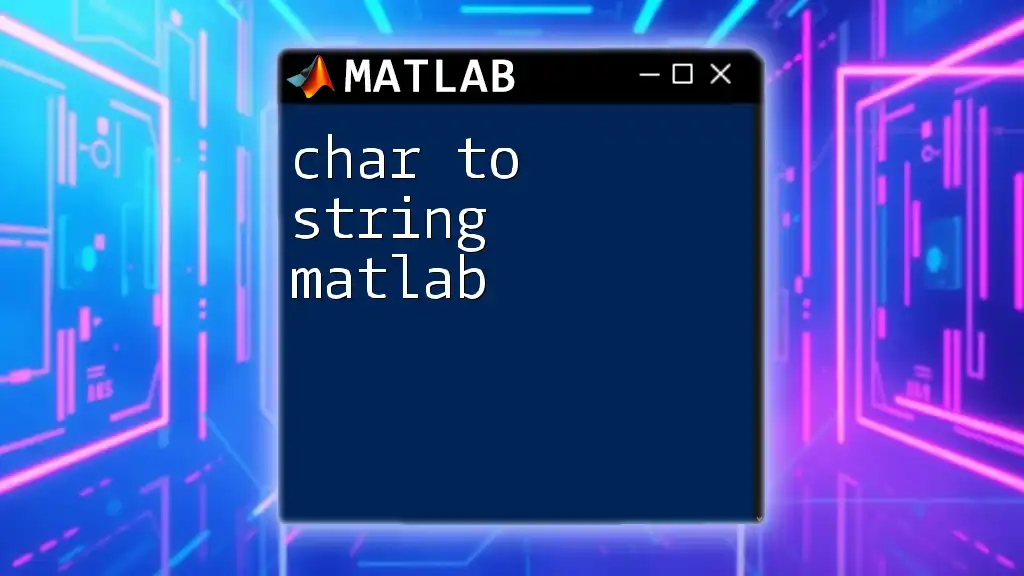
Why Convert Cell Arrays to Strings?
Converting cell arrays to strings is often a necessary step in data processing for various reasons. Some scenarios include:
- Output formatting: When you need to present or log data in a readable format.
- User interfaces: Displaying data dynamically in GUI elements.
- Data export: Preparing data for storage or sharing in text files.
By mastering the conversion process, you can enhance your programming efficiency and ensure clean data output.
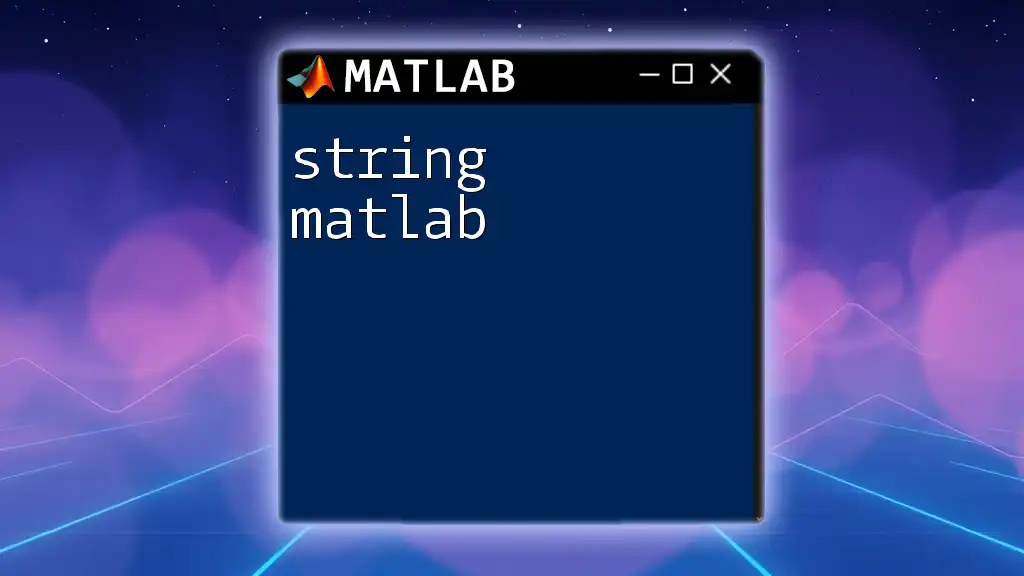
Methods for Conversion from Cell to String
Using `strjoin` Function
Overview of `strjoin`
The `strjoin` function in MATLAB provides a straightforward way to concatenate string elements of a cell array into a single string, using a specified delimiter. Its syntax is simple, making it one of the most efficient methods for transforming your cell arrays.
Example Code Snippet
To illustrate how `strjoin` works, consider the following example:
cellArray = {'Hello', 'World', 'from', 'MATLAB'};
resultString = strjoin(cellArray, ' ');
disp(resultString);
In this example, the output will be "Hello World from MATLAB". Each element from `cellArray` is joined with a space, creating a neatly formatted string.
Using `cellfun` and `char` for Conversion
Explanation of `cellfun`
`cellfun` is a powerful function that allows you to apply a specified function to each element of a cell array. This is particularly beneficial when you need to convert non-string elements to strings before combining them.
Example Code Snippet
Here is how you can use `cellfun` in conjunction with `char` to convert a cell array into a single string:
cellArray = {'Hello', 'World'};
charArray = cellfun(@char, cellArray, 'UniformOutput', false);
resultString = [charArray{:}];
disp(resultString);
This snippet results in "HelloWorld". Each element is converted to a character array and concatenated together. The `UniformOutput` parameter is set to false to allow different data types.
Utilizing `sprintf` for Formatting
Overview of `sprintf`
The `sprintf` function in MATLAB is designed for advanced formatting of strings, providing various options for how data should appear. It excels in situations where you need formatted output, such as controlling decimal places or specifying alignment.
Example Code Snippet
Here’s an example that utilizes `sprintf`:
cellArray = {'Data', 'Analysis', 'in', 'MATLAB'};
resultString = sprintf('%s ', cellArray{:});
disp(strtrim(resultString));
The output for this code will be "Data Analysis in MATLAB". The `'%s '` format specifier tells `sprintf` to treat each element as a string and append a space after each. The `strtrim` function is used to remove any trailing spaces.
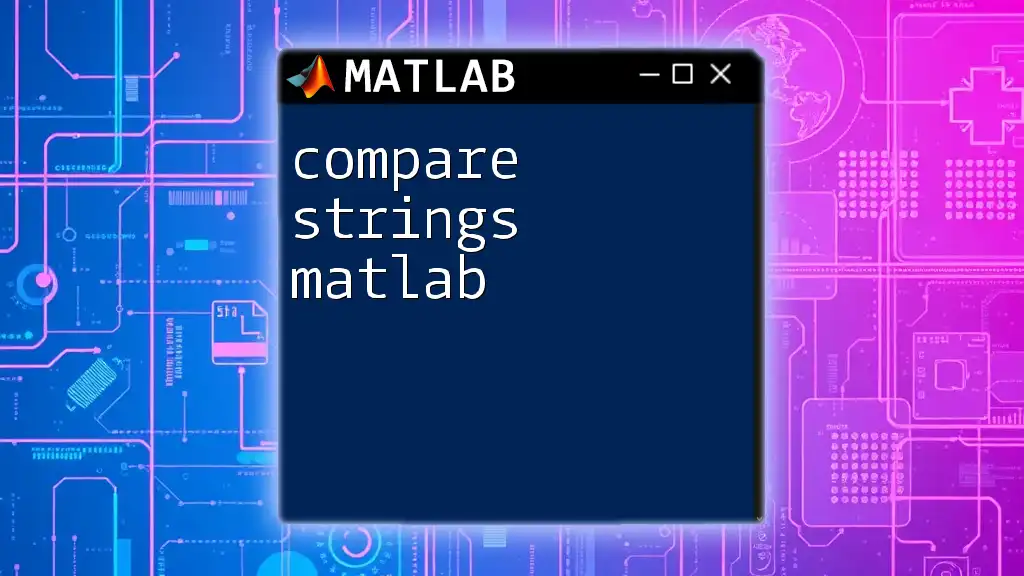
Handling Different Data Types
Mixed Cell Arrays
One of the challenges you may face with cell arrays is dealing with mixed data types. MATLAB allows you to store different types of data in a single cell array, but converting these to strings requires additional care.
Example Code Snippet
Consider the following mixed cell array:
mixedCellArray = {42, 'is', 'the', 'answer'};
resultString = strjoin(cellfun(@num2str, mixedCellArray, 'UniformOutput', false), ' ');
disp(resultString);
The output of this code will be "42 is the answer". Here, `num2str` is used to convert numeric values to strings before joining. This is essential for maintaining data coherency during conversion.
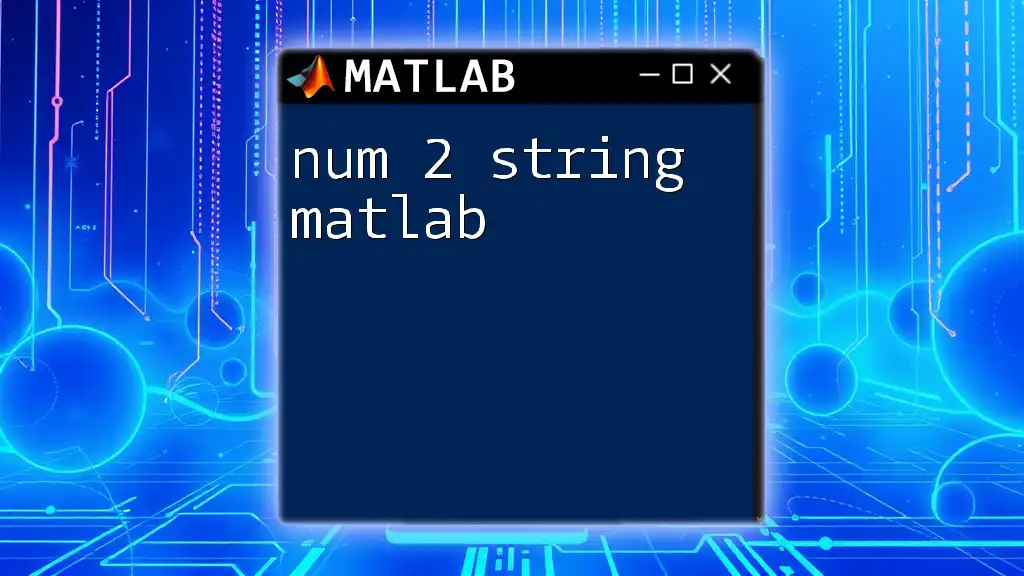
Error Handling and Troubleshooting
Common Errors During Conversion
When converting cell arrays to strings, you may encounter several common errors, such as type mismatches or attempting to join empty cells. Understanding these pitfalls can help you avoid frustration.
For example, if you try to use `strjoin` on a cell array containing numbers without converting them to strings first, you may receive an error similar to:
Error using strjoin (line X)
Input must be a string array or a cell array of strings.
How to Debug
To address these issues, employ some debugging strategies:
- Check Data Types: Use the `class()` function to inspect the data types in your cell array.
- Preview Data: Print sections of your cell array to verify its contents before performing operations.
- Use MATLAB Debugging Tools: Utilize built-in debugging tools like breakpoints to step through your code and inspect variable values at various execution stages.
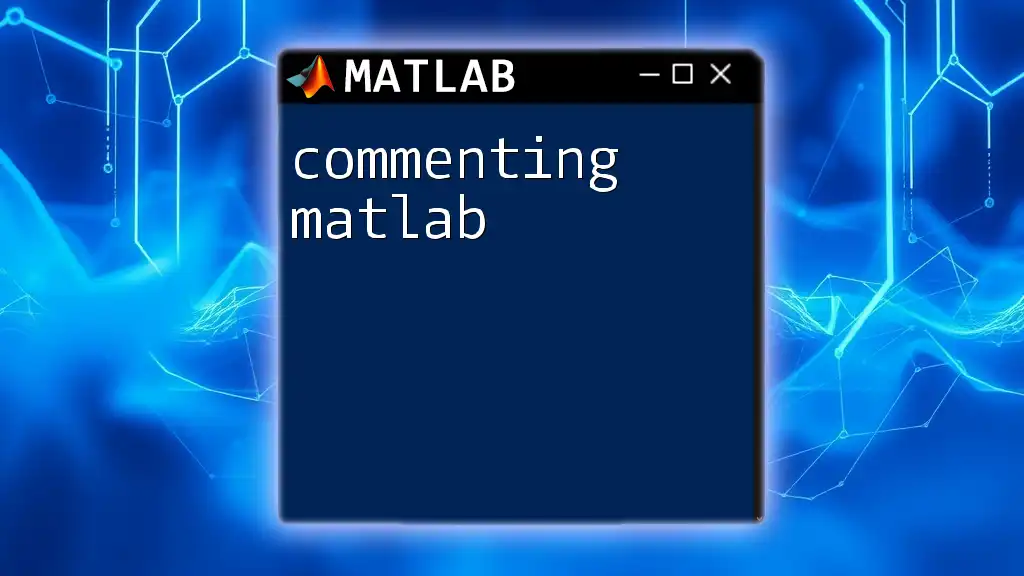
Conclusion
Mastering the conversion from cell to string in MATLAB is a vital skill for anyone working with data in MATLAB programming. Whether you're preparing data for output, user interfaces, or data export, understanding how to manipulate cell arrays will enhance your efficiency. Practice with the examples given, and don’t hesitate to explore further with your own variations.
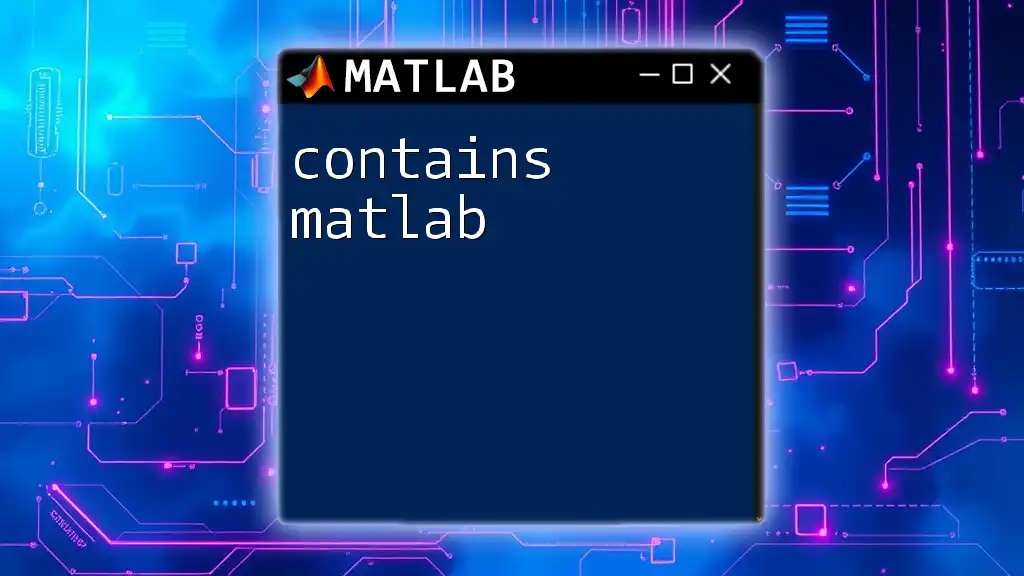
Additional Resources
For further learning, consider checking MATLAB's official documentation specific to string handling and cell arrays. These resources provide in-depth explanations and additional examples that can deepen your understanding of working with strings and cell arrays.
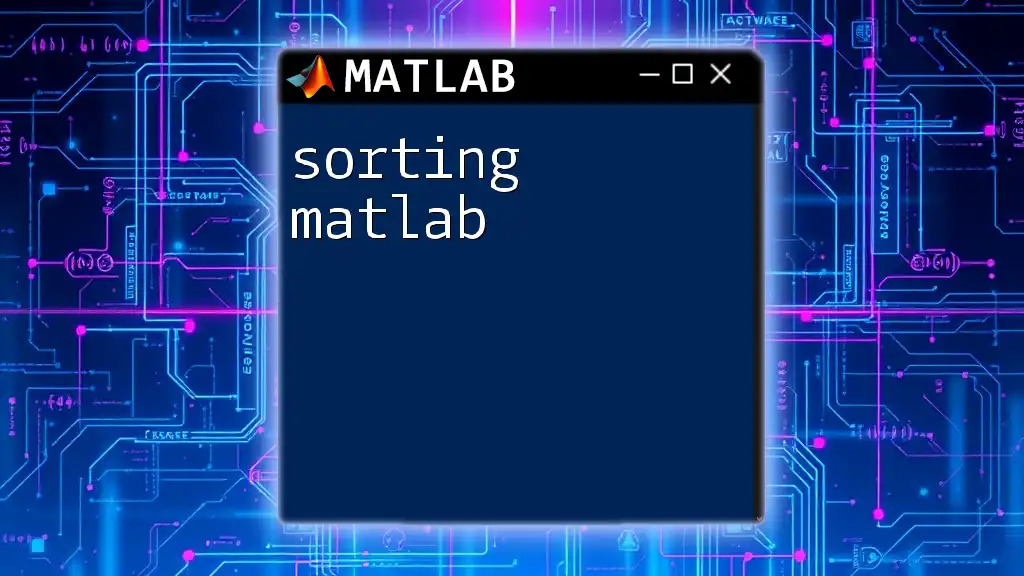
Call to Action
If you're eager to improve your MATLAB skills further, consider signing up for our courses or subscribing to our newsletter for more valuable tips and insights into MATLAB programming. Feel free to reach out for personalized lessons or inquiries!