The `contains` function in MATLAB is used to determine whether a specific substring is present within a string array or character vector.
str = 'Hello, MATLAB World!';
result = contains(str, 'MATLAB'); % returns true
Understanding the "contains" Function in MATLAB
The `contains` function in MATLAB is a powerful tool for searching and querying strings and arrays for specific substrings. It simplifies the process of identifying whether an item exists within a larger set of text, which can be immensely useful in various programming scenarios—from data analysis to text processing.
Basic Syntax
The basic syntax of the `contains` function is as follows:
C = contains(A, B)
- A: This represents the input array, which can be a string array or a character array.
- B: This is the substring you are searching for.
- C: The output is a logical array that indicates where the substring B is found in A. Each element of C corresponds to the results of the search for each item in A.
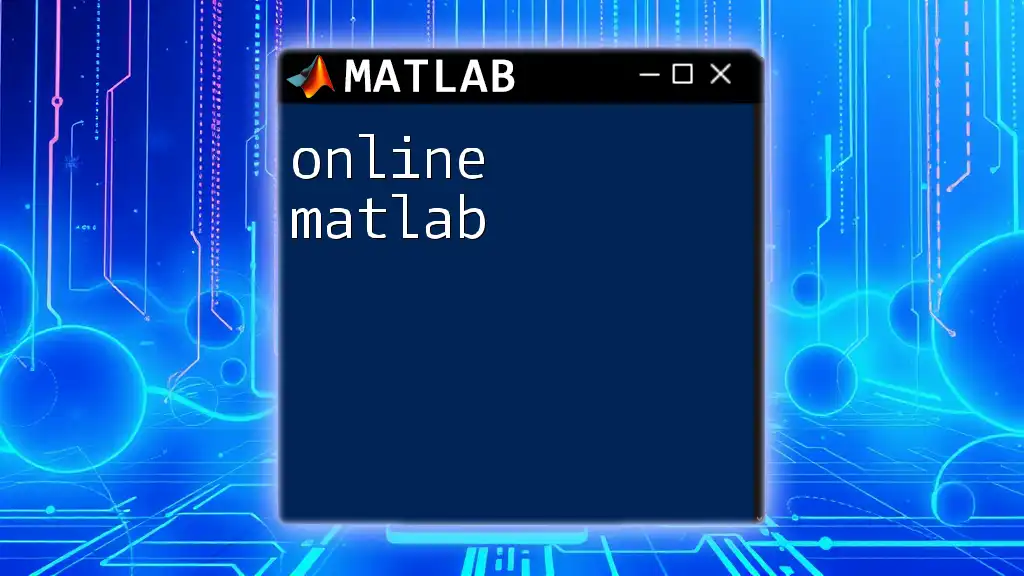
Key Features of the "contains" Function
Case Sensitivity
By default, the `contains` function performs a case-sensitive search. This means that searching for "hello" will not match "Hello" or "HELLO". This characteristic can be customized by using additional options, allowing greater flexibility depending on the requirements of your program.
Support for Cell Arrays and String Arrays
The `contains` function readily accepts both string arrays and cell arrays. This versatility is crucial for users who need to work with diverse data structures. Users can apply the `contains` function to a cell array of strings, and it will return a logical array just as it would for a string array.
Example: Basic Usage of Contains
Consider a basic example to illustrate how to use the `contains` function:
str = "Hello, World!";
result = contains(str, "World");
disp(result); % This will return true
In this snippet, the variable str contains the string "Hello, World!". When we search for the substring "World", the output result will be true, indicating that "World" is indeed contained within the string.
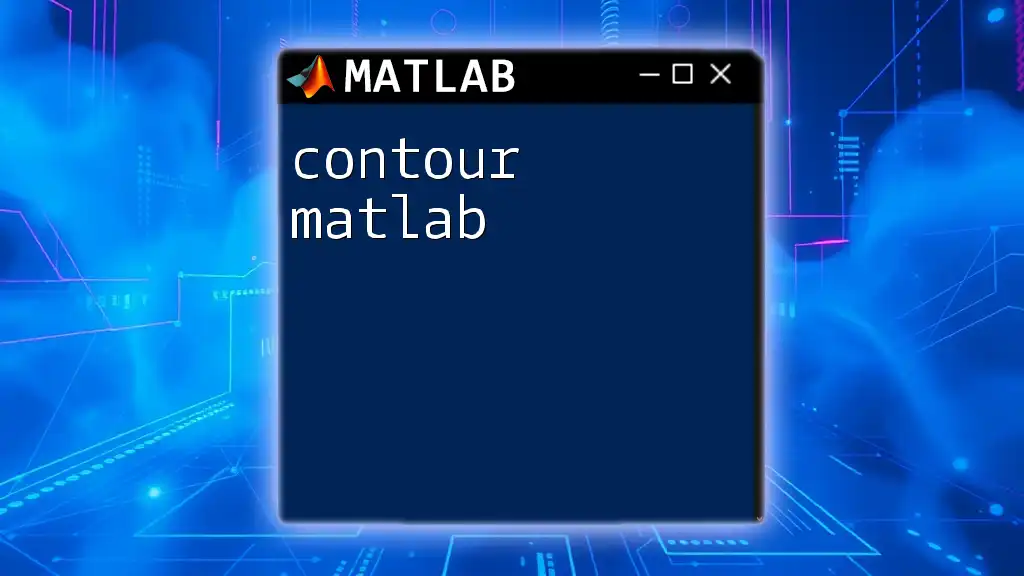
Practical Applications of the "contains" Function
Text Analysis
The `contains` function is particularly advantageous in text analysis, allowing users to swiftly identify the occurrence of specific words or phrases within large bodies of text. This is essential for tasks such as sentiment analysis, keyword extraction, and content categorization.
Filtering Data
Another practical application of the `contains` function is in the filtering of datasets. Users can filter lists or arrays to extract items that contain specific keywords or patterns. This capability is especially useful in data cleaning and pre-processing stages.
Example: Filtering Data
Here’s an example of how to employ the `contains` function to filter data based on a substring:
data = {"MATLAB", "Python", "Java", "C++"};
filteredData = data(contains(data, "MAT"));
disp(filteredData); % This will return "MATLAB"
In this code snippet, data is an array of different programming languages. The `contains` function checks for items that include the substring "MAT", leading to the output filteredData, which returns just "MATLAB". This illustrates how the `contains` function can effectively narrow down lists based on specific criteria.
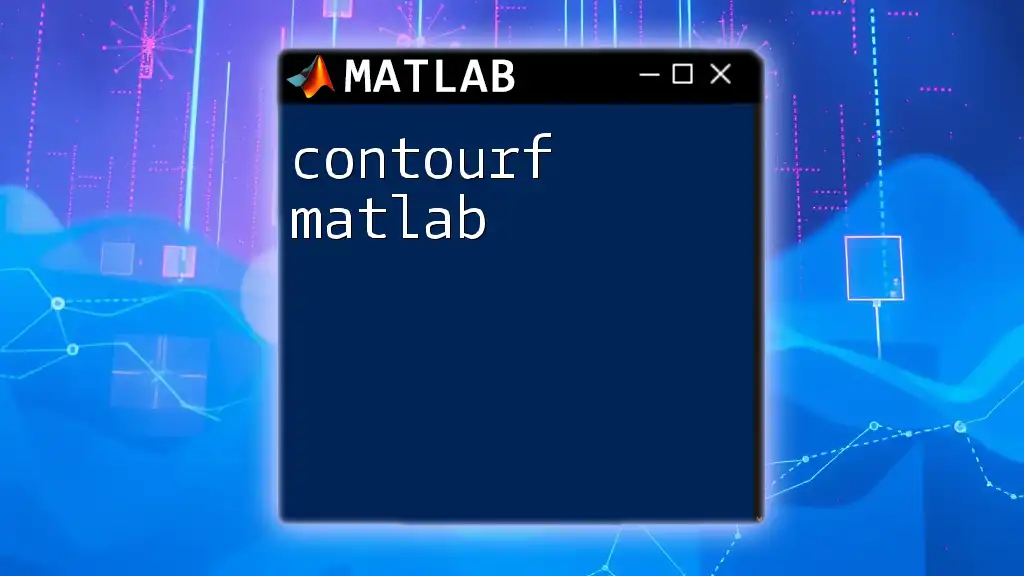
Advanced Usage of the "contains" Function
Using Logical Indexing with Contains
Logical indexing can be combined with the `contains` function to filter arrays based on specific conditions. This powerful pairing enables users to implement more refined data selection strategies in their workflows.
Example: Logical Indexing
Consider the following example that demonstrates how to utilize logical indexing with `contains`:
strings = ["apple", "banana", "grape", "orange"];
logicalIndex = contains(strings, "a");
selectedFruits = strings(logicalIndex);
disp(selectedFruits); % This will return "apple" and "banana"
Here, we have an array strings containing various fruits. By applying `contains` to check which fruit names contain the letter "a", we generate a logical index array that the original array utilizes for filtering. selectedFruits then contains "apple" and "banana", showcasing a practical implementation of the function to refine outputs based on user-defined criteria.
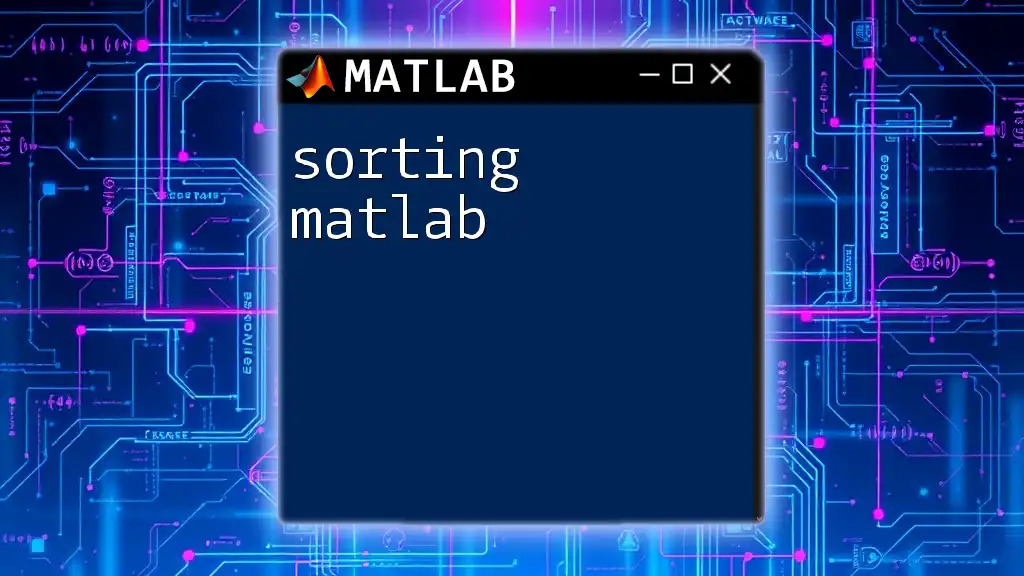
Common Issues and Troubleshooting
Case Sensitivity Problems
Many users initially struggle with case sensitivity, which can lead to unexpected results when searching for substrings. It’s crucial to remember that if the default case-sensitive option does not suit your needs, you can use the 'IgnoreCase' option to perform a case-insensitive search.
Empty Arrays
Another common issue arises when users pass empty arrays to the `contains` function. Understanding how `contains` behaves with such inputs is vital. For instance, if you check whether an empty string contains a non-existing substring, the result will naturally be false.
Example: Handling Edge Cases
result1 = contains("", "test"); % Expect false
result2 = contains([""], ""); % Expect true
In these examples, the first line checks if an empty string contains "test," clearly returning false. Conversely, the second line checks if an empty array contains an empty substring, which returns true. Illustrating these edge cases allows users to better navigate potential pitfalls while coding in MATLAB.
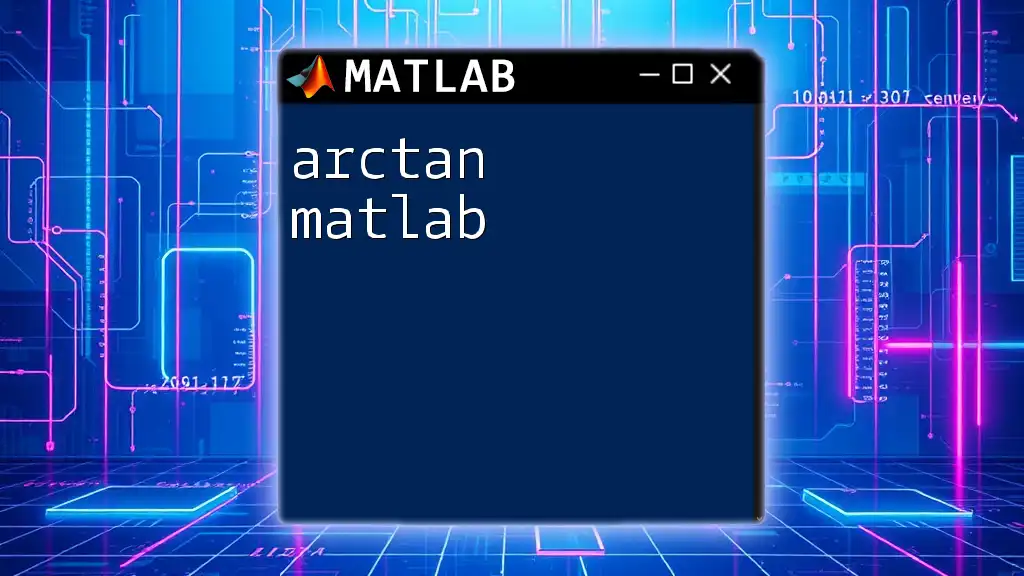
Conclusion
The `contains` function in MATLAB is a versatile and essential tool for anyone working with strings and data filtering. By mastering its syntax and applications, users can streamline text analysis and enhance data management techniques. Its utility extends from straightforward substring searches to more complex logical indexing operations, making it a critical part of any MATLAB user’s toolkit.
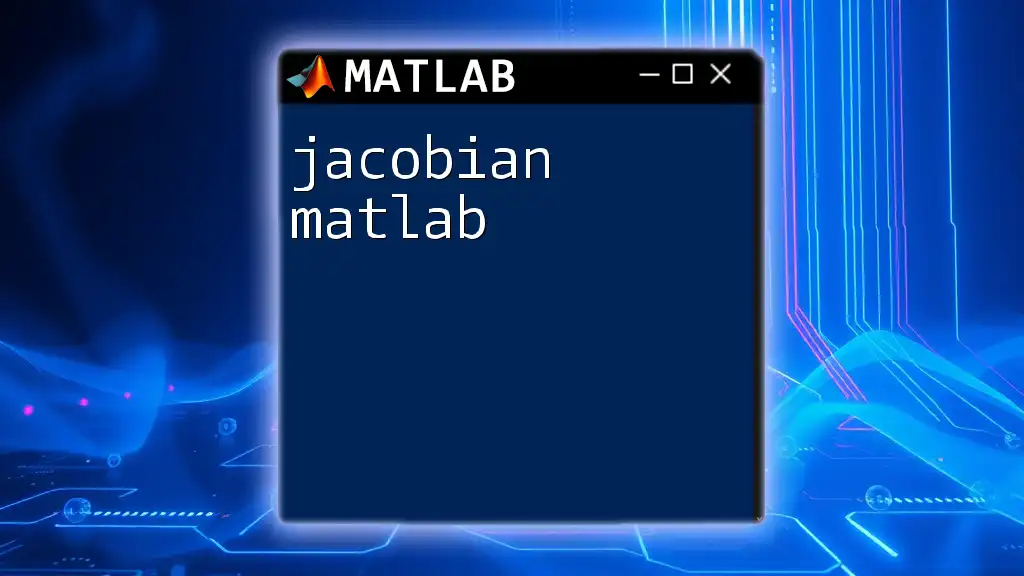
Call to Action
Explore more about MATLAB and sharpen your programming skills by visiting [Your Company Name]. Dive into a world of resources, courses, and tutorials that will help you master commands and functions effectively!
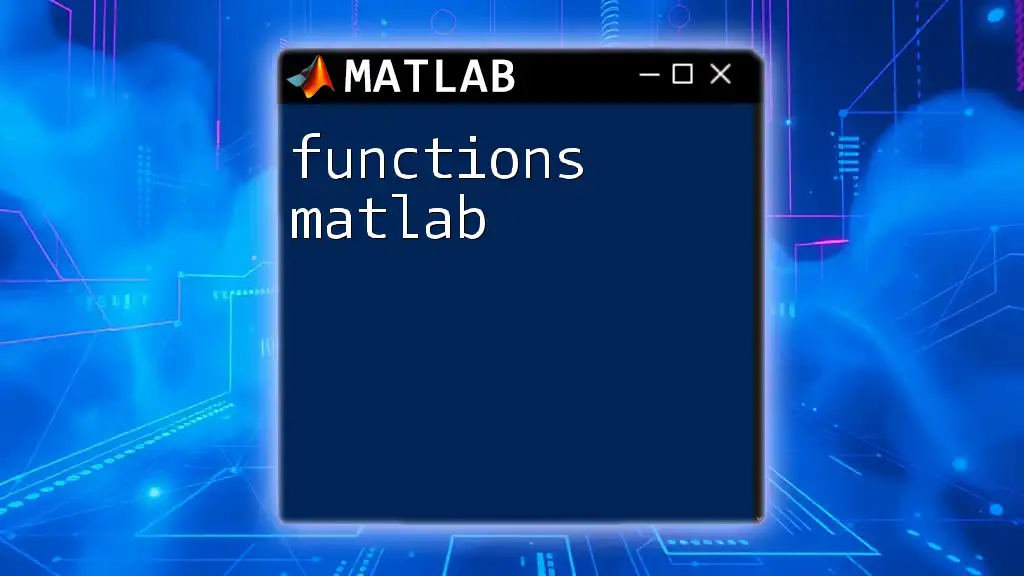
Additional Resources
For further reading, check out the official MATLAB documentation for the `contains` function. Explore related tutorials to deepen your understanding of MATLAB commands and improve your programming proficiency.