Cross-correlation in MATLAB can be performed using the `xcorr` function to measure the similarity between a signal and a reference signal, helping to identify delays and patterns within the data. Here's a code snippet demonstrating its use:
% Sample signals
signal = [1, 2, 3, 4, 5];
reference_signal = [2, 3, 4];
% Compute cross-correlation
cross_corr = xcorr(signal, reference_signal);
% Display the result
disp(cross_corr);
Understanding Cross-Correlation
What is Cross-Correlation?
Cross-correlation is a statistical measure used to assess the similarity between two signals as a function of the time-lag applied to one of them. Mathematically, it quantifies the degree to which one signal can predict another, making it a vital tool in various fields, including communications, audio processing, and biomedical engineering. The formula for cross-correlation involves computing the integral of the product of one signal with a time-shifted version of another signal.
The Concepts Behind Cross-Correlation
Cross-correlation closely relates to convolution, where it assesses the overlap of two functions as one slides past the other. However, it diverges from autocorrelation, which measures the similarity of a signal with itself over time. The result of cross-correlation can yield peaks which correspond to points of high similarity, providing insights into the timing and nature of the relationship between the two signals.
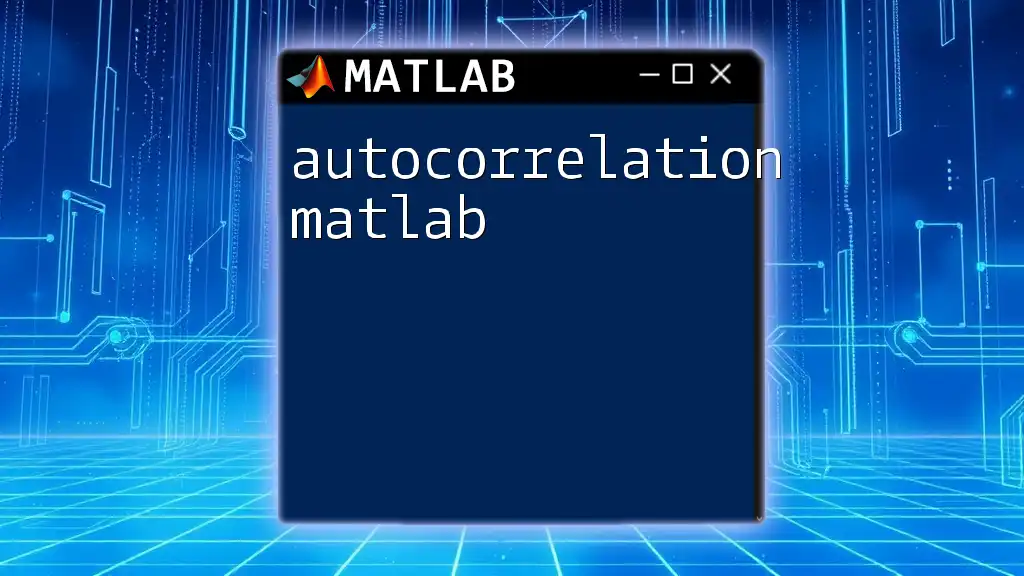
Setting Up Your MATLAB Environment
Installing MATLAB
To begin utilizing MATLAB for signal processing tasks, you need to install the software. Ensure you download the latest version from the official MathWorks website and consider adding the Signal Processing Toolbox, which provides useful functions for advancing your analysis.
MATLAB Basics for Cross-Correlation
Familiarity with the Command Window and the Editor in MATLAB is essential. The Command Window allows you to run commands interactively, while the Editor is suitable for scripting and storing multiple commands for review and execution. Understanding how to define variables and use different data types, such as arrays and matrices, is crucial for working with signals.
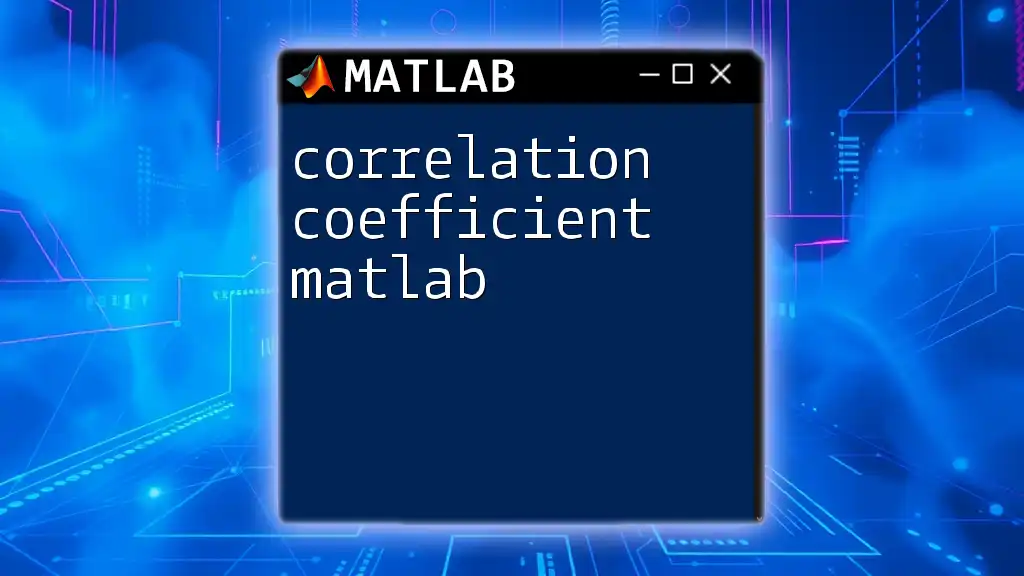
Performing Cross-Correlation in MATLAB
Basic Syntax for Cross-Correlation
The core function for performing cross-correlation in MATLAB is `xcorr`. This function simplifies the process by accepting two primary arguments: the signal and the reference signal.
Example 1: Basic Cross-Correlation
Here’s a simple example to demonstrate how to use the `xcorr` function.
% Example Code
signal = randn(1, 100); % Random signal
reference_signal = randn(1, 100); % Random reference signal
cross_corr = xcorr(signal, reference_signal); % Performing cross-correlation
In this snippet, random signals are generated, and then the cross-correlation is computed. Understanding the output of `xcorr` is crucial, as it will include the cross-correlation values for various lags.
Plotting the Cross-Correlation Result
Visualizing the results is key to interpreting cross-correlation effectively. MATLAB provides built-in plotting functions which are invaluable for this purpose.
% Plotting Code
figure;
plot(cross_corr);
xlabel('Lag');
ylabel('Cross-Correlation');
title('Cross-Correlation between Signal and Reference Signal');
grid on;
This code snippet will create a plot illustrating how the cross-correlation varies with different lags. Understanding the axes is fundamental; the x-axis shows the lag, while the y-axis indicates the correlation strength.
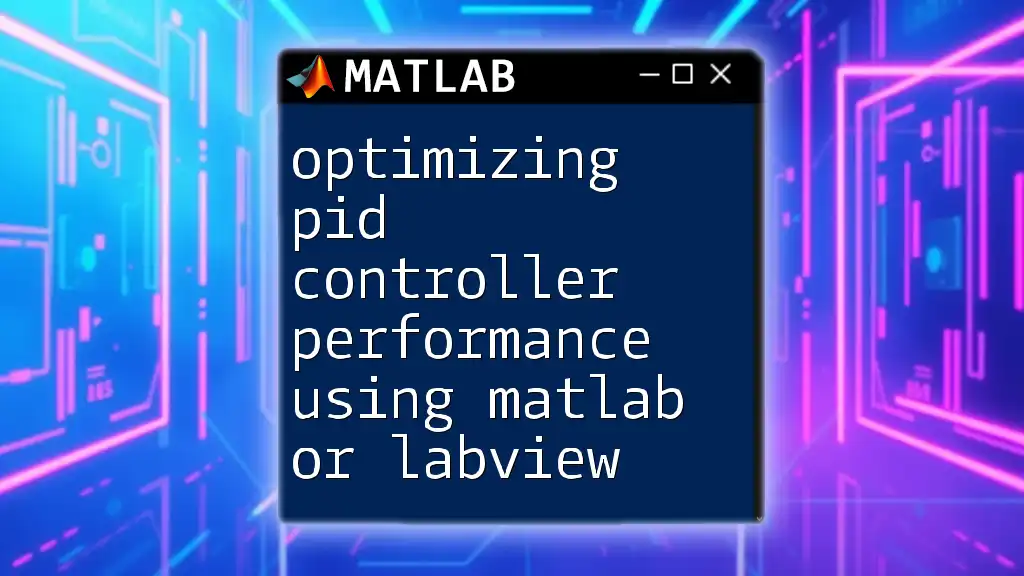
Advanced Cross-Correlation Techniques
Normalizing Cross-Correlation
Normalization is a critical step in analyzing cross-correlated signals, as it allows for meaningful comparative analysis between different signal pairs.
% Normalizing Code
norm_cross_corr = cross_corr / max(abs(cross_corr)); % Normalization
Normalizing the cross-correlation result scales it between -1 and 1, making it easier to interpret peaks and troughs, especially when dealing with signals of varying amplitudes.
Cross-Correlation with Time Lag Handling
Handling time lags allows for a more comprehensive analysis of the signals in question. The `xcorr` function includes options to specify lag handling, providing additional flexibility in the analysis.
% Cross-Correlation with Lags
[cross_corr, lags] = xcorr(signal, reference_signal, 'coeff'); % Coeff normalization
The output includes `lags`, which represents the different lag values corresponding to the cross-correlation outputs. Interpreting these lags is essential to understanding the temporal relationship between the signals.
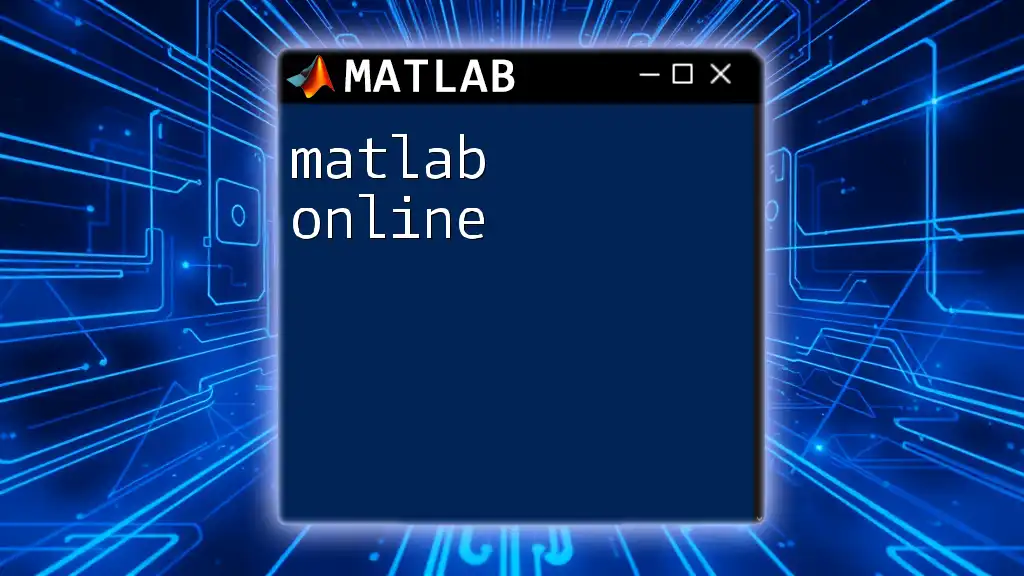
Real-World Applications of Cross-Correlation
Signal Alignment in Audio Processing
In audio processing, cross-correlation can align two audio tracks that may have been recorded separately. By identifying the time shift that maximizes the cross-correlation, one can synchronize audio tracks effectively.
For practical use, consider the following MATLAB snippet that aligns two audio signals:
% Aligning audio signals
[audio1, fs1] = audioread('audio_1.wav');
[audio2, fs2] = audioread('audio_2.wav');
[cross_corr, lags] = xcorr(audio1, audio2);
[maxCorr, index] = max(cross_corr);
lag = lags(index);
This code aligns `audio1` and `audio2` using cross-correlation, enabling seamless playback.
System Identification
Cross-correlation is also instrumental in system identification, a method used to determine the system models based on input-output data. By performing cross-correlation, one can estimate the system's impulse response, which can be pivotal for filtering and control system design.
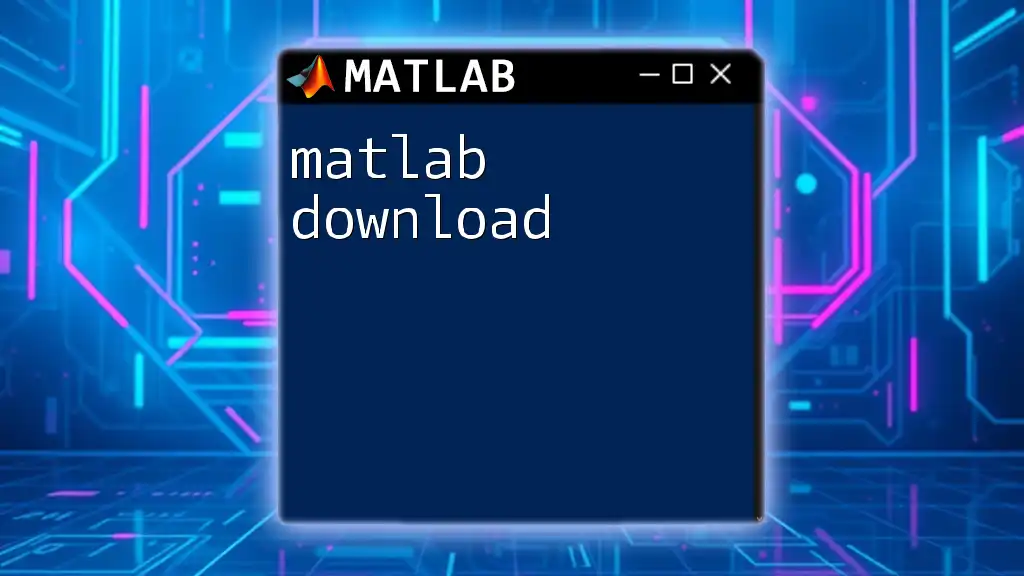
Troubleshooting Common Issues
Handling Noise in Signals
In practical applications, noise can significantly affect the cross-correlation analysis. It’s crucial to preprocess your signals by applying filters (e.g., low-pass filters) to attenuate the noise which might skew the results.
Ensuring Signal Length Compatibility
When performing cross-correlation, ensure that your signals are of compatible lengths. If not, you may end up with misleading results.
% Trimming Code
minLength = min(length(signal), length(reference_signal));
signal = signal(1:minLength);
reference_signal = reference_signal(1:minLength);
Trimming the longer signal makes sure both signals are analyzed over the same duration, ensuring that the results are valid.
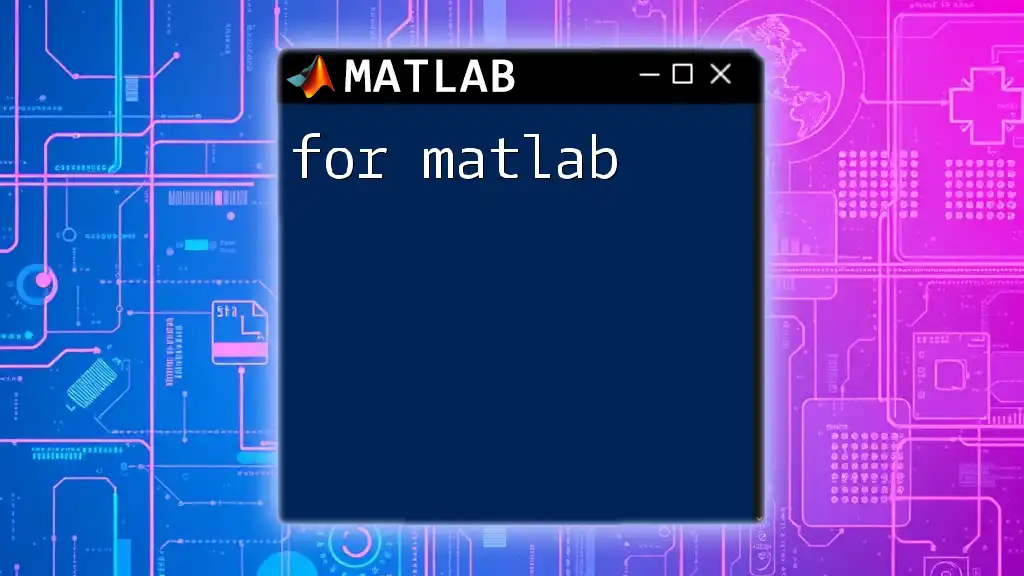
Conclusion
Cross-correlation between signal and reference signal in MATLAB provides powerful insights into the relationships and timings between different signals. By understanding the underlying concepts, utilizing MATLAB's robust functions, and applying best practices in analysis, one can effectively harness cross-correlation for various applications. Practice with the examples provided, and explore further to deepen your comprehension of this essential signal processing technique.
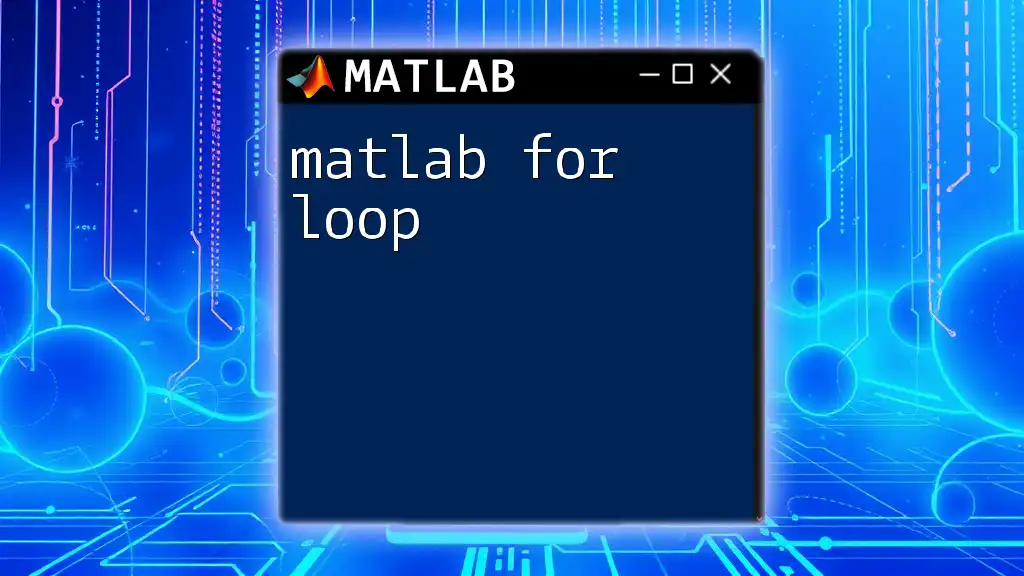
Additional Resources
Recommended Reading and Courses
To further your understanding of signal processing, consider delving into comprehensive texts on the subject or enrolling in online courses dedicated to MATLAB and its application in signal processing.
MATLAB Documentation Links
MATLAB's official documentation provides an invaluable resource for mastering functions like `xcorr` and enhances your capacity to implement them successfully in real-world scenarios.
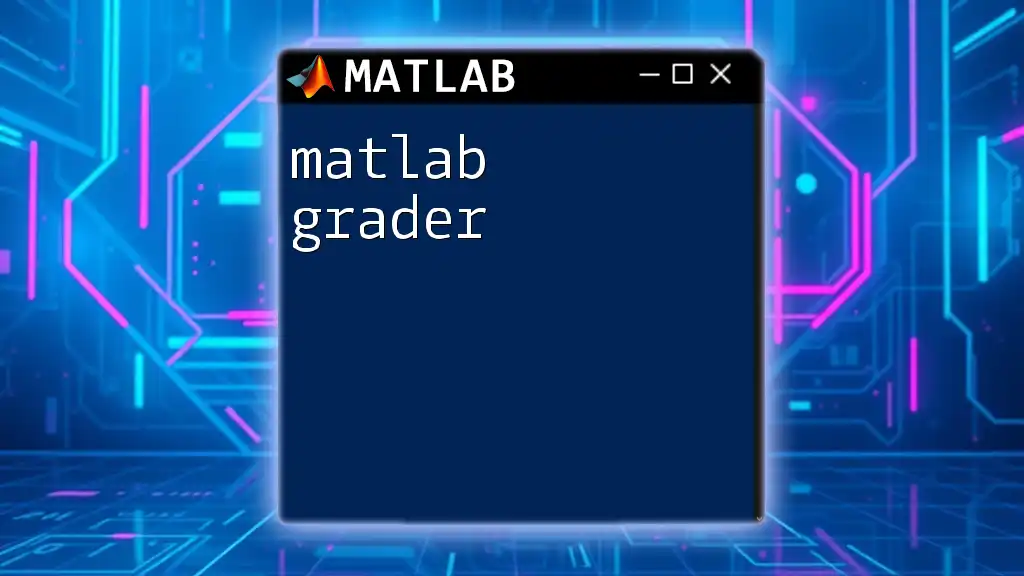
Call to Action
Explore cross-correlation techniques in your projects and experiments. Sign up for more tutorials and insights, and don't hesitate to ask questions or share your experiences with MATLAB's cross-correlation capabilities!