In MATLAB, a function is a block of code that performs a specific task and can be reused with different inputs to produce outputs, defined using the `function` keyword.
Here's a simple example of a MATLAB function that adds two numbers:
function result = addNumbers(a, b)
result = a + b;
end
Understanding Function Types in MATLAB
Built-in Functions
MATLAB is renowned for its rich library of built-in functions, which cover a wide range of mathematical operations, data handling tasks, and algorithmic solutions. Familiarizing yourself with these functions is crucial, as they can considerably speed up your programming tasks and improve your code's efficiency.
For example, the `sum()` function computes the total sum of an array. Here's how it works:
total = sum([1, 2, 3, 4]); % Returns 10
Another common function is `mean()`, which calculates the average value of an array:
avg = mean([1, 2, 3, 4]); % Returns 2.5
User-defined Functions
User-defined functions empower you to tailor your code to meet specific needs that aren't covered by built-in functions. They promote code reusability and modular programming, making it easier to manage larger projects.
Creating a Simple User-defined Function
The structure of a user-defined function in MATLAB is straightforward. Below is a fundamental example where we create a function that squares its input.
function output = myFunction(input)
output = input^2; % Squares the input
end
This function can be called with any number, for instance:
result = myFunction(4); % Returns 16
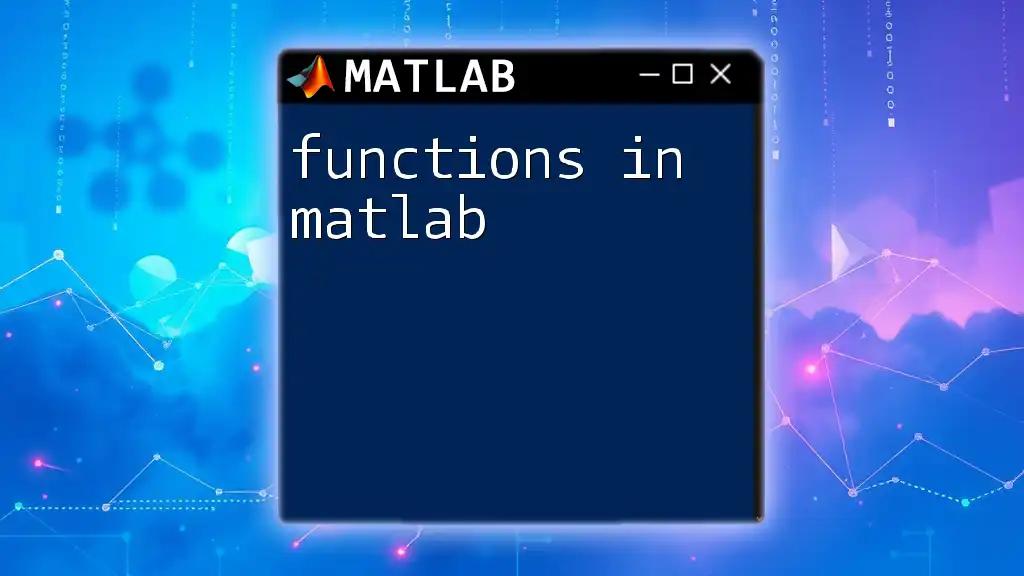
Creating and Using Functions
The Basic Syntax of a Function
The syntax for declaring a function in MATLAB is critical to ensuring its proper operation. Use the following structure to define your functions:
function [outputs] = functionName(inputs)
Steps to Create a Function
Creating a user-defined function in MATLAB involves several key steps:
- Open a new script in MATLAB.
- Write the necessary function code following the established syntax.
- Save the file with the same name as the function (e.g., `myFunction.m`).
Example of a User-defined Function
Let's explore a practical example: computing the factorial of a number recursively.
function f = factorial(n)
if n == 0
f = 1;
else
f = n * factorial(n-1);
end
end
To call this function and calculate the factorial of 5, you would use:
result = factorial(5); % Returns 120
This recursive function illustrates how you can repeat operations until a base case is achieved, showcasing the elegance of functions in MATLAB.
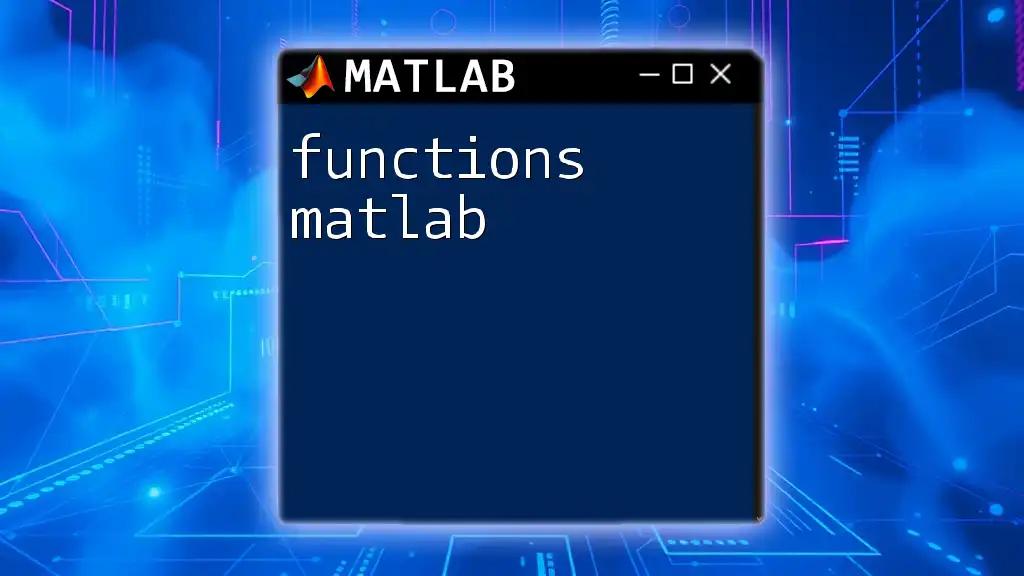
Scope of Variables in Functions
Local vs Global Variables
Understanding variable scope is vital for effective programming in MATLAB. Variables can be local to a function or global, affecting how and where they can be accessed.
Example of Local Variable
In this context, a local variable is only accessible within the function itself:
function x = addTen(y)
x = y + 10; % y is a local variable
end
Example of Global Variable
Global variables can be accessed across different functions, providing a way to share data. Here’s an example:
global z
function demoGlobal()
global z;
z = 5; % Sets global variable z
end
Using global variables should be done with caution as they can lead to code that is hard to debug.

Function Overloading
What is Function Overloading?
Function overloading allows you to define multiple functions with the same name but different input parameters. This increases flexibility and enables the same function name to handle different data types.
Implementation of Function Overloading
Here's an example of how to implement function overloading:
function output = myFunction(x)
if isnumeric(x)
output = x^2; % For numeric input
elseif ischar(x)
output = strcat(x, ' is a string'); % For string input
end
end
This function uses conditional statements to determine the type of input it receives and behaves accordingly.
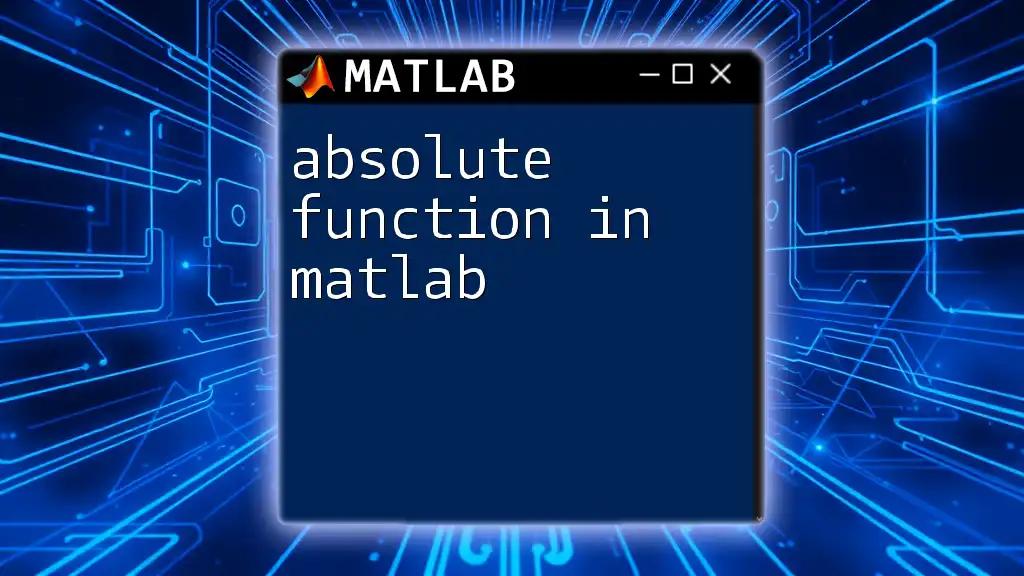
Anonymous Functions
What are Anonymous Functions?
Anonymous functions in MATLAB provide a compact way to define simple functions without creating a separate file. They are especially useful for one-liners or when you need a quick function for a specific context.
Creating and Using Anonymous Functions
To create an anonymous function, use the following syntax:
square = @(x) x^2; % Defines an anonymous function that squares its input
You can then call this function just like any other function:
result = square(5); % Returns 25
Anonymous functions are particularly valuable when used with optimized functions, such as array operations and plotting routines.
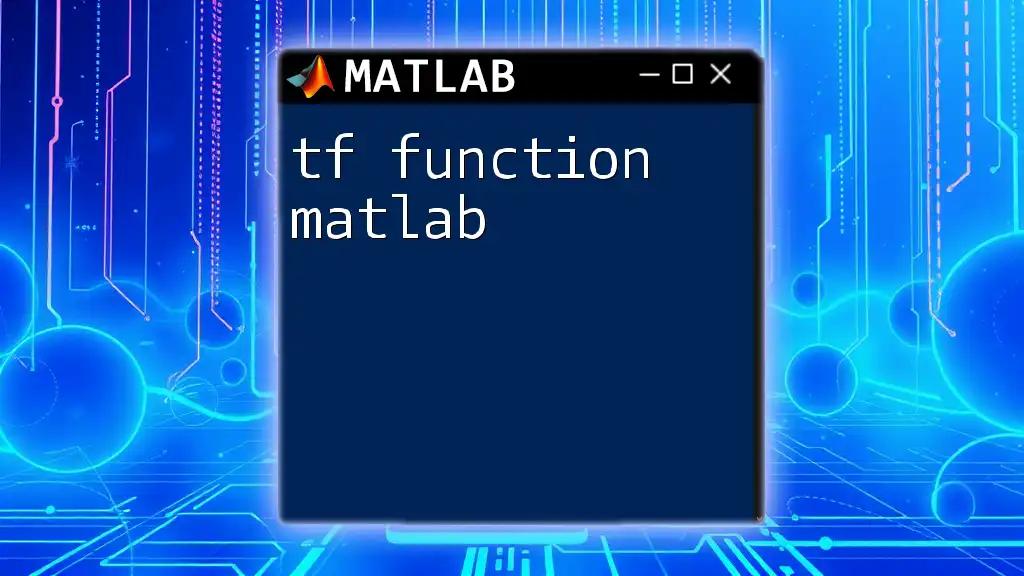
Conclusion
Functions in MATLAB serve as the backbone for creating organized, efficient code. They enhance your programming capability by allowing for modularity and reusability. Understanding the various types of functions—both built-in and user-defined—along with their scopes and behaviors, is essential for any MATLAB programmer.
As you explore MATLAB further, I encourage you to experiment with creating your own functions, delving into both simple and complex scenarios. This practice will deepen your understanding and make you a more proficient coder.
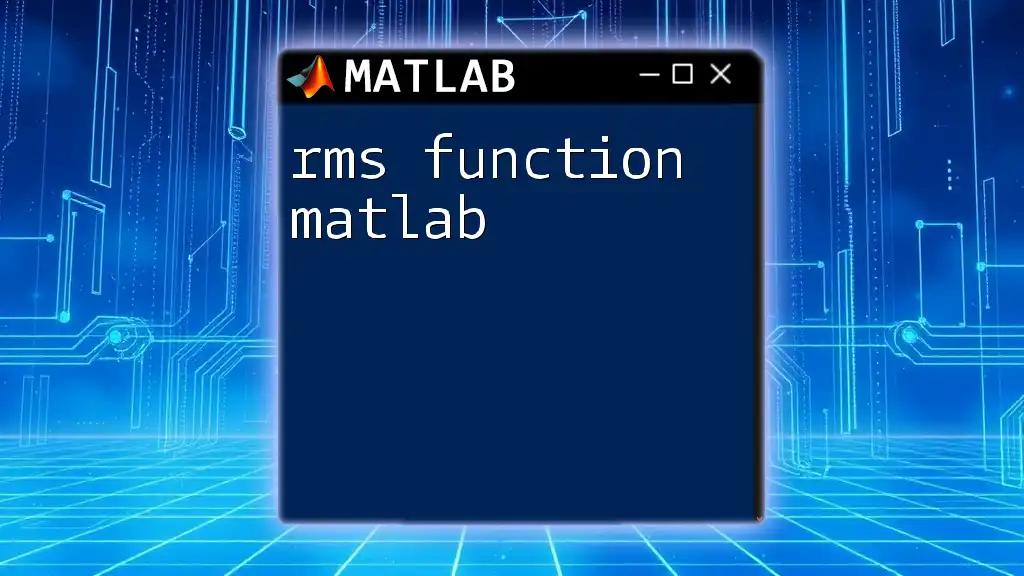
Further Learning Resources
To continue your journey, consider diving into comprehensive resources such as books, online courses, or the extensive MATLAB documentation available online. These will provide nuanced insights into advanced functions and programming techniques.

Call to Action
Have questions about functions in MATLAB? Or perhaps you want to share your own experiences or challenges? Feel free to leave your comments! Together, let’s build a community eager to learn and grow in the realm of MATLAB programming.