A transfer function in MATLAB represents the relationship between the input and output of a linear time-invariant system and can be defined using the `tf` function.
Here's a code snippet to create a transfer function in MATLAB:
num = [1]; % Numerator coefficients
den = [1, 2, 1]; % Denominator coefficients
sys = tf(num, den); % Create transfer function
What is a Transfer Function?
A transfer function is a mathematical representation that describes the relationship between the output and the input of a system in the frequency domain. It essentially encapsulates how a system responds to various inputs, making it a powerful tool in control theory and signal processing.
Mathematical Representation
The transfer function is generally expressed as:
\[ H(s) = \frac{N(s)}{D(s)} \]
where \(N(s)\) is the numerator polynomial and \(D(s)\) is the denominator polynomial. Here, s is a complex frequency variable, and the coefficients of the polynomials represent the dynamics of the system. Understanding the structure of a transfer function is crucial for analyzing the stability and frequency response of control systems.

Understanding Transfer Function in MATLAB
To work effectively with transfer functions in MATLAB, one must become familiar with the Control System Toolbox. This toolbox provides a comprehensive suite of functions specifically designed for control and system analysis, making tasks more efficient and intuitive.

Creating a Transfer Function in MATLAB
Using `tf` function
In MATLAB, transfer functions can be created using the `tf` function. This function allows users to define a transfer function by specifying the coefficients of the numerator and the denominator polynomials.
Syntax of the `tf` function:
sys = tf(numerator, denominator);
For example, to create a simple transfer function \( H(s) = \frac{1}{s^2 + 3s + 2} \), you would enter:
num = [1]; % numerator coefficients
den = [1, 3, 2]; % denominator coefficients
sys = tf(num, den);
Example Explanation
In the example above, we created a transfer function that represents a second-order system. The numerator is a constant \(1\), while the denominator is a quadratic polynomial \(s^2 + 3s + 2\). The behavior of the system can be characterized by analyzing its poles (roots of the denominator) and zeros (roots of the numerator).
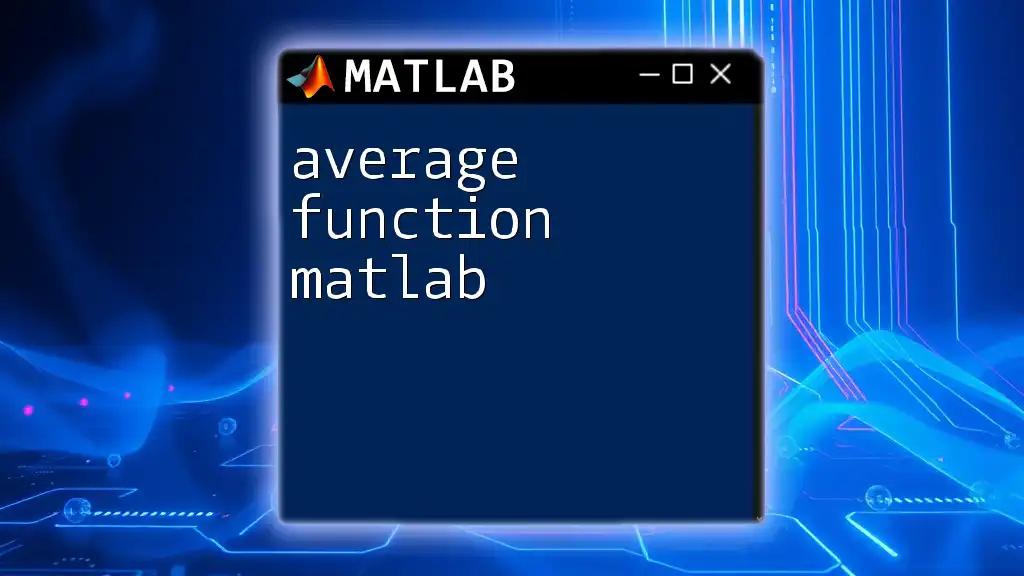
Analyzing Transfer Functions
Step Response
The step response is a time-domain analysis that shows how the system responds to a unit step input. It is vital for gauging system performance characteristics such as overshoot, settling time, and steadiness. To plot the step response of a transfer function, you can use the following command:
step(sys);
grid on;
title('Step Response of the Transfer Function');
This will illustrate the dynamics of the system as it reacts to a sudden change in input.
Impulse Response
Similarly, the impulse response is the output of the system when subjected to an instantaneous input. It provides insights into the system's inherent characteristics. You can visualize this with the impulse function:
impulse(sys);
grid on;
title('Impulse Response of the Transfer Function');
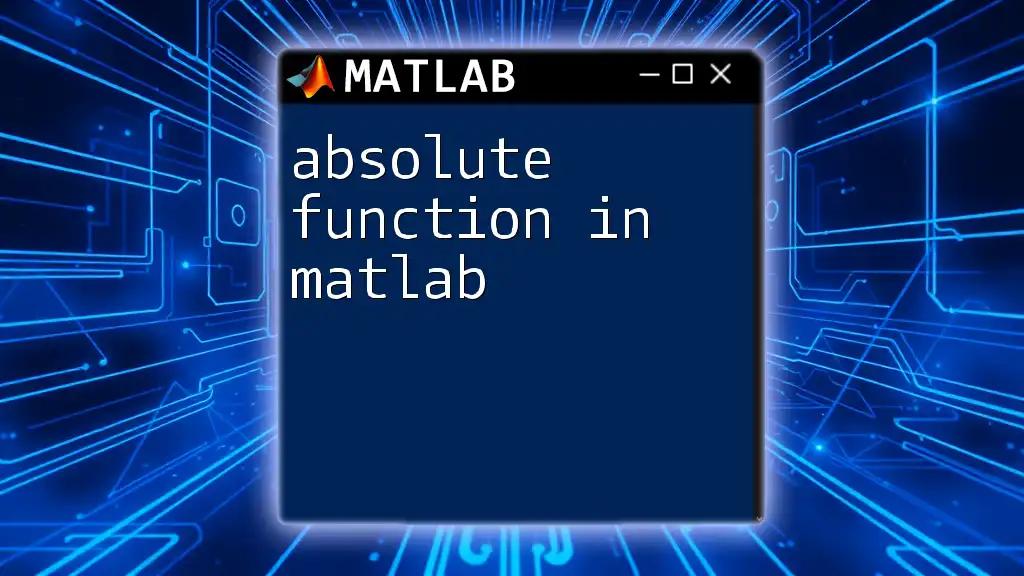
Modifying Transfer Functions
Adding Poles and Zeros
To modify a transfer function by adding poles or zeros, the `pzmap` function can be employed. Poles and zeros significantly affect the behavior of the transfer function and its stability.
To plot the pole-zero map, use the command:
pzmap(sys);
title('Pole-Zero Map of the Transfer Function');
This graphical representation helps you assess system stability and design control strategies accordingly.
Feedback Systems
The feedback system is a crucial concept in control theory that helps stabilize systems. MATLAB makes it easy to implement feedback connections using the `feedback` function. For example, to create a feedback configuration where the output \(sys\) is fed back into the input, use:
feedback_sys = feedback(sys, 1);
step(feedback_sys);
title('Step Response of Feedback System');
This will show how the feedback alters the system dynamics.
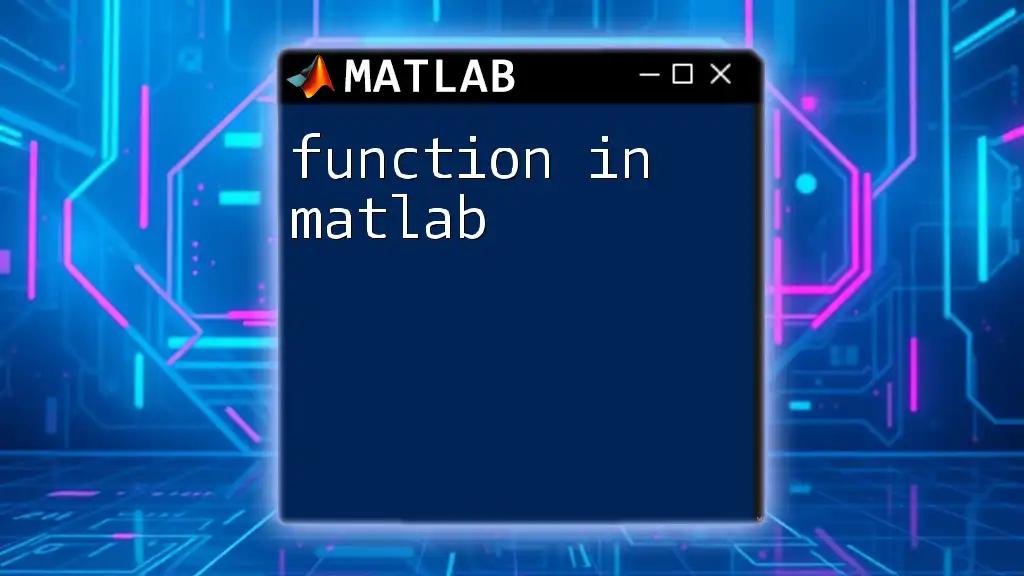
Frequency Response Analysis
Bode Plots
Bode plots are invaluable for analyzing the frequency response of linear time-invariant systems. They provide a graphical representation of a system’s gain and phase across frequencies. To generate a Bode plot, you simply use:
bode(sys);
title('Bode Plot of the Transfer Function');
This powerful visualization aids in assessing how the frequency components influence system behavior.
Nyquist Plots
The Nyquist plot is another critical tool for analyzing stability, especially for feedback systems. It maps the complex frequency response of the system. To create a Nyquist plot in MATLAB, execute:
nyquist(sys);
title('Nyquist Plot of the Transfer Function');
Understanding the Nyquist plot can help ensure that your system is stable under various operating conditions.
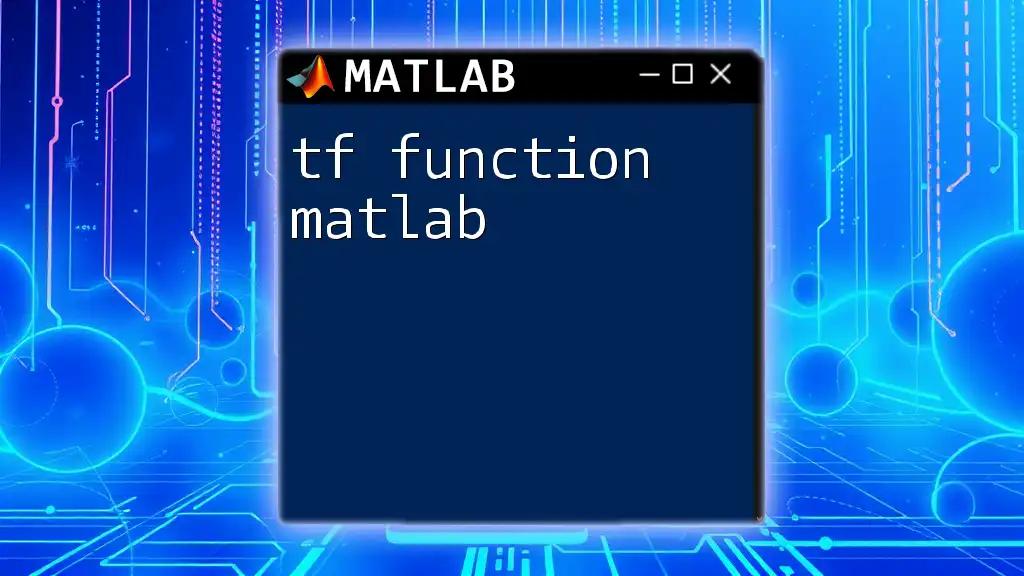
Common Issues and Troubleshooting
Common Errors
When working with transfer functions in MATLAB, one might encounter several typical errors, such as input mismatches or incorrectly defined polynomials. These issues can lead to misleading results. A common error is a dimension mismatch when defining the numerator or denominator vectors.
To resolve such issues, always double-check your vector sizes and confirm they accurately represent the system’s coefficients.
Performance Considerations
When dealing with large or complex systems, performance can become an issue. To maximize efficiency:
- Use vectorization wherever possible to minimize loop-based calculations.
- Preallocate memory for large matrices to avoid dynamic resizing during computations.
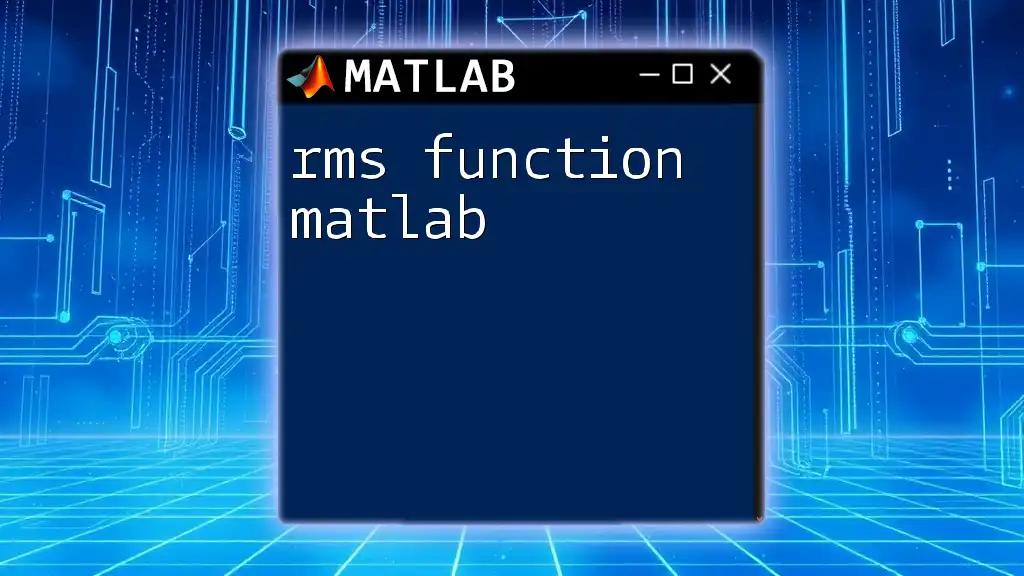
Conclusion
In summary, mastering the concept of the transfer function in MATLAB provides a solid foundation for analyzing and designing control systems. This guide has outlined the essentials of creating, analyzing, and modifying transfer functions, as well as frequency response methods.
As you continue to explore the capabilities of MATLAB, remember that practice is crucial. Engage with examples, experiment with commands, and utilize feedback to refine your understanding of transfer functions and their applications in real-world scenarios.
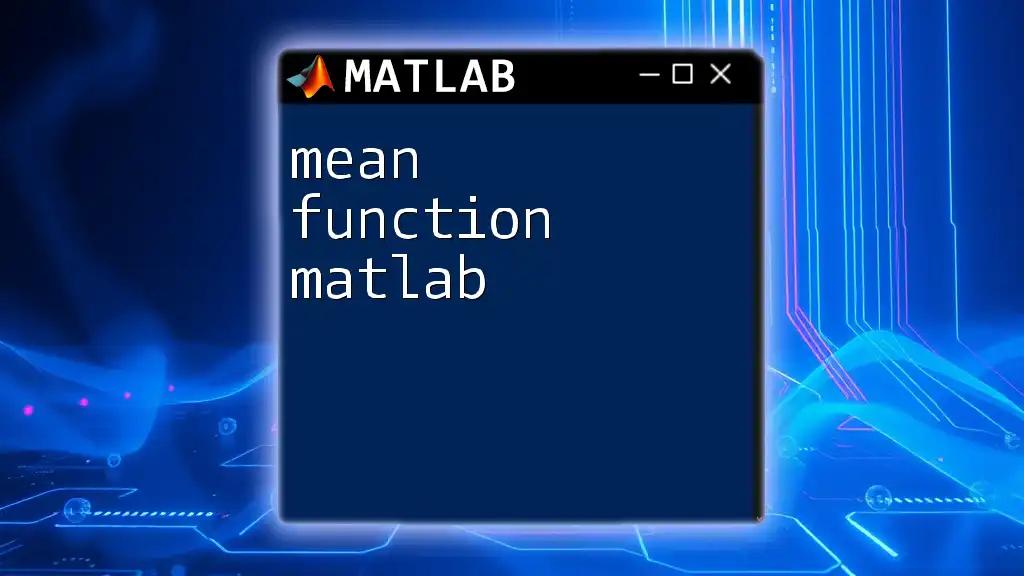
Additional Resources
Consider exploring books and online courses to deepen your understanding of control systems and MATLAB. Furthermore, MATLAB’s documentation provides extensive resources for transfer functions and related analyses, making it an invaluable companion for your learning journey.
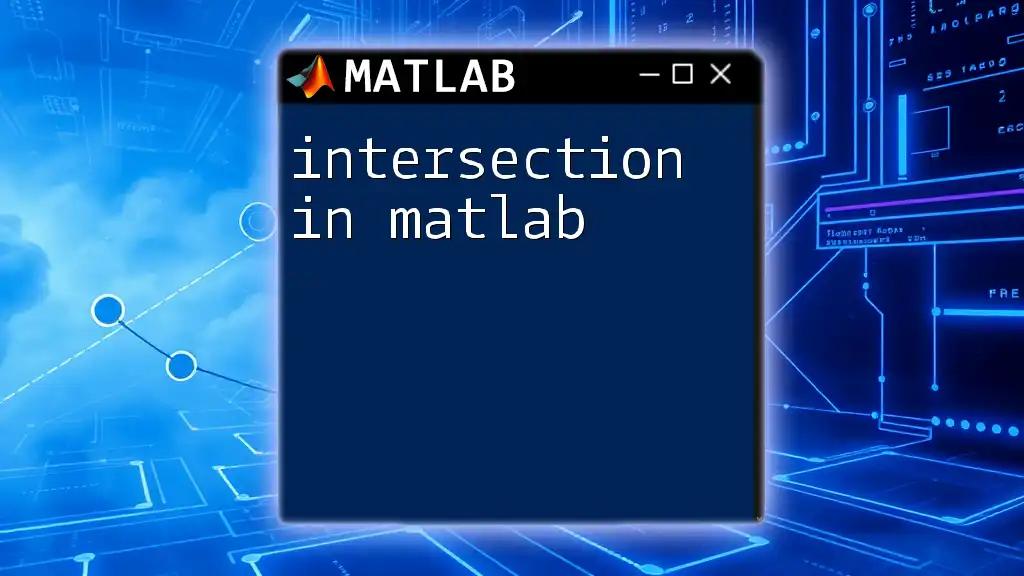
Call to Action
Now is the time to apply what you've learned! Experiment with creating transfer functions in MATLAB and analyze their behaviors. Share your experiences or questions in the comments section to foster a community of learning and exploration.