In MATLAB, the constant `e` represents the base of the natural logarithm, approximately equal to 2.71828, and it can be utilized in mathematical operations and functions such as exponentiation.
Here’s a code snippet demonstrating its use:
% Calculate e raised to the power of 2
result = exp(2);
disp(result);
Understanding Euler's Number (e)
What is Euler’s Number (e)?
Euler's number, commonly denoted as e, is a fundamental mathematical constant approximately equal to 2.71828. It plays a crucial role in various fields such as calculus, complex analysis, and mathematical modeling. Known as the base of the natural logarithm, e arises naturally in the process of continuous growth or decay, making it essential for understanding real-world phenomena.
The Value of e
As an irrational number, e cannot be expressed exactly as a fraction, and its decimal representation is non-repeating. This characteristic makes it a vital constant in both theoretical mathematics and practical applications across disciplines including finance, biology, and physics.
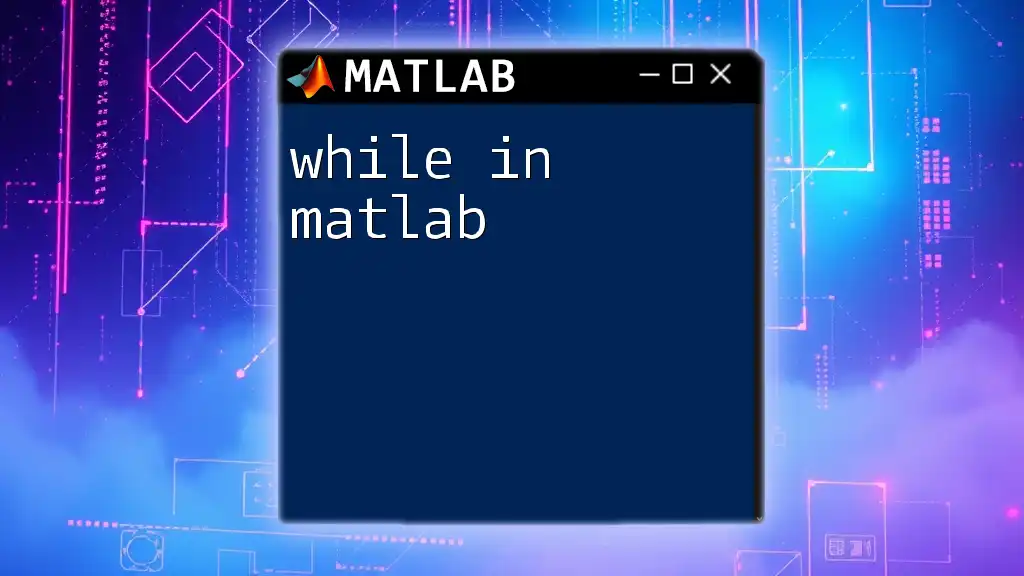
Using e in MATLAB
The Exp Function
One of the most common uses of e in MATLAB is through the `exp` function, which calculates the exponential of each element in an array. The syntax for this function is:
Y = exp(X)
Where X can be a single number, a vector, or a matrix. The `exp` function is straightforward to use and crucial for calculations involving e.
Example Code Snippet:
x = [0, 1, 2, 3];
y = exp(x);
disp(y);
In this example, the output will display the values of e raised to the powers of the elements in x, yielding:
1.0000 2.7183 7.3891 20.0855
This illustrates how e grows exponentially, reflecting its significance in mathematical functions and models.
Calculating Exponential Growth
Exponential Growth Formula
The exponential growth model can be expressed mathematically as: \[ N(t) = N_0 e^{rt} \] In this equation, \( N_0 \) represents the initial quantity, \( r \) is the growth rate, and \( t \) is time. This model is widely used in fields like population dynamics, finance, and pharmacokinetics.
Implementing the Formula in MATLAB
To demonstrate this, you can implement the formula in MATLAB to visualize an exponential growth curve.
Example Code Snippet:
N0 = 100; % Initial quantity
r = 0.05; % Growth rate
t = 0:10; % Time from 0 to 10
N = N0 * exp(r * t);
plot(t, N);
title('Exponential Growth');
xlabel('Time');
ylabel('Population Size');
This code simulates how a population of 100 grows at a rate of 5% over 10 time units. When you run this code, the resulting plot will show an upward curve, reinforcing the concept of exponential growth facilitated by the use of e.
Using e in Mathematical Calculations
Solving Differential Equations
Euler's number is also integral to solving differential equations, particularly first-order equations such as: \[ \frac{dy}{dt} = ky \] Here, k is a constant that represents the rate of growth or decay.
MATLAB Implementation
You can solve this equation in MATLAB by using the following example:
Example Code Snippet:
k = 0.1; % Growth constant
t = 0:0.1:10; % Time vector
y = exp(k*t); % Solution of the differential equation
plot(t, y);
title('Exponential Growth of y(t)');
xlabel('Time');
ylabel('y(t)');
This code snippet captures how a system behaves over time under exponential growth. The resulting plot will depict the continuous increase of y(t) as time progresses, illustrating the powerful effect of e in differential modeling.
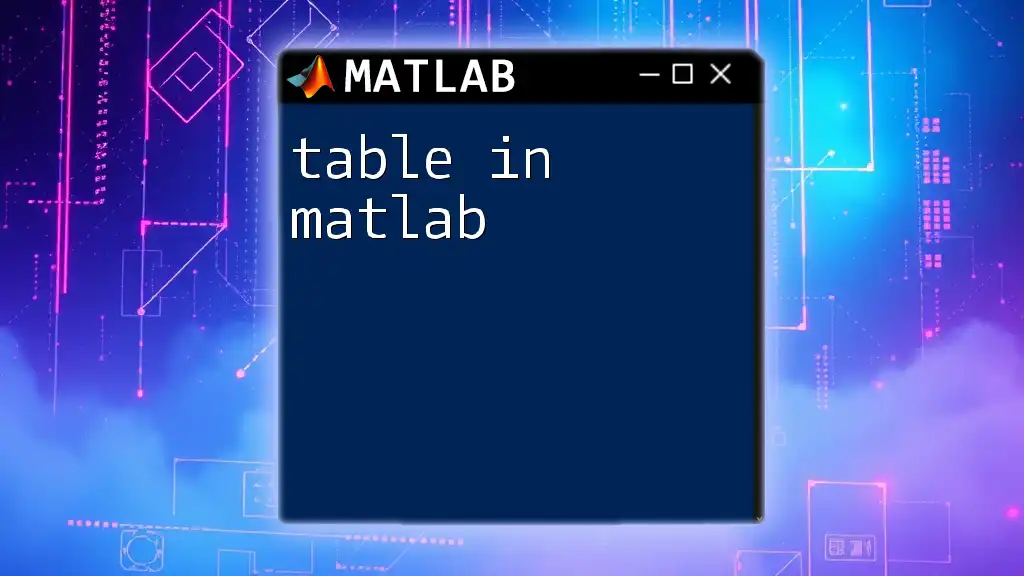
Common Applications of e in MATLAB
Financial Modelling
In finance, the constant e is used extensively to model continuous compounding of interest. The future value computation can be simplified using the formula: \[ A = P e^{rt} \] Where \( P \) represents the initial principal amount, \( r \) the interest rate, and \( t \) the time in years.
Example Code Snippet:
P = 1000; % Principal amount
r = 0.05; % Interest rate
t = 5; % Time in years
A = P * exp(r * t); % Future value calculation
disp(A);
When you run this code, it will output the total amount after 5 years with continuous compounding, illustrating the financial implications of exponential growth.
Natural Phenomena
Euler's number also appears in models describing natural phenomena, such as population decay. For example, a simple decay model can be expressed using the formula: \[ N(t) = N_0 e^{-kt} \] Here, \( k \) is a decay constant. You can implement this in MATLAB to observe how a population decreases over time.
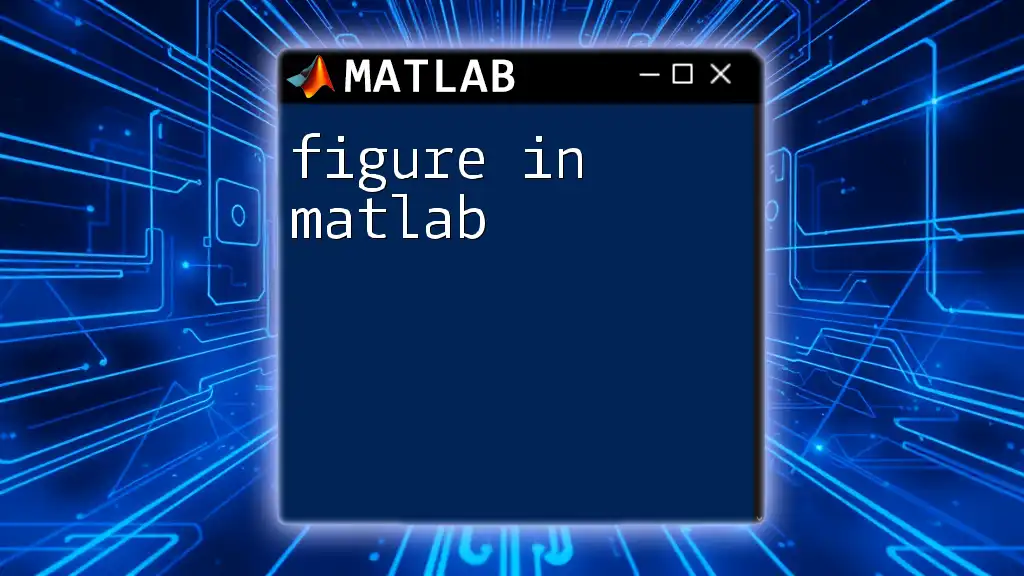
Conclusion
In summary, understanding how to work with e in MATLAB opens the door to a variety of mathematical and engineering applications. From calculating exponential growth to solving differential equations, the utility of e is vast. As you continue to explore the capabilities of MATLAB, you'll find that these concepts can be applied across numerous domains, providing invaluable insight and predictive power.
By utilizing the `exp` function effectively and recognizing the role of Euler's number, you enhance your programming toolkit in MATLAB. Embrace the challenges of computational problems and continue to expand your knowledge of this powerful software.
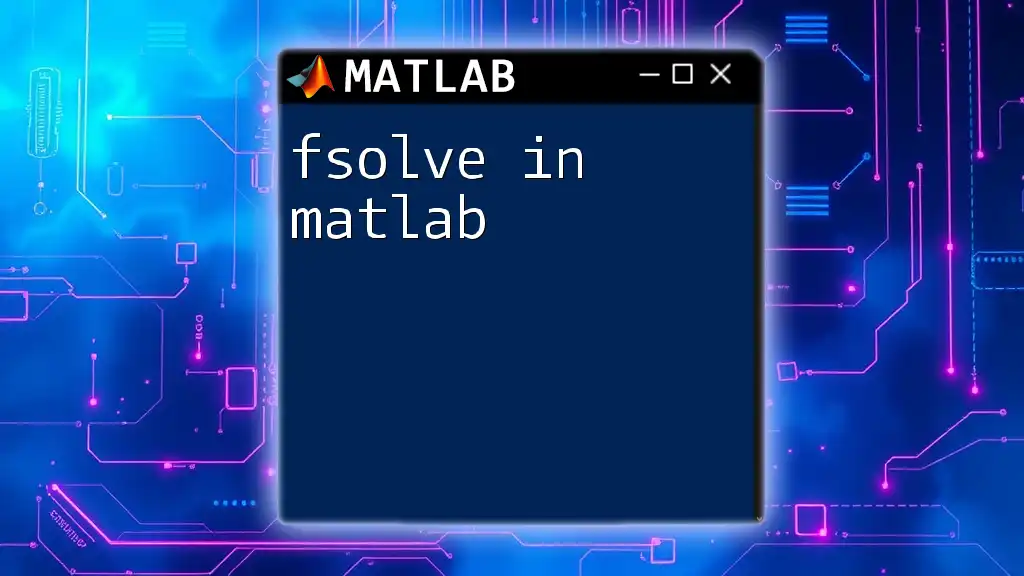
Additional Resources
For those interested in delving deeper into MATLAB functionalities, consider exploring the official documentation on the `exp` function. Participate in an online MATLAB community for support and to access tutorials, offering insights that can enrich your understanding of mathematical concepts in programming.