The `imnoise` function in MATLAB is used to add various types of noise, such as Gaussian, salt & pepper, and Poisson noise, to an image for simulation or testing purposes.
Here’s a simple example of how to use `imnoise` to add salt and pepper noise to a grayscale image:
% Read an image
img = imread('image.jpg');
% Convert to grayscale if it's a color image
grayImg = rgb2gray(img);
% Add salt & pepper noise
noisyImg = imnoise(grayImg, 'salt & pepper', 0.02);
% Display the original and noisy images
imshowpair(grayImg, noisyImg, 'montage');
Understanding Image Noise
What is Image Noise?
Image noise refers to the random variations in brightness or color information in images, disrupting the true representation of the scene being captured. It can arise from multiple sources, such as sensor limitations, environmental conditions, or transmission errors.
There are several common types of noise that we encounter in image processing:
-
Gaussian Noise: Often encountered in images, Gaussian noise produces random variations that follow a bell-shaped probability distribution. This type of noise is common in electronic devices.
-
Salt and Pepper Noise: This noise appears as randomly distributed white and black pixels. It typically occurs due to dead pixels on a sensor or erroneous data transmission.
-
Poisson Noise: This type of noise is related to the statistical nature of photon detection, particularly noticeable in low-light environments. Poisson noise follows a Poisson distribution where the variance is equal to the mean.
Impact of Noise on Image Quality
Noise particularly affects the clarity of images, and even a small amount can degrade the quality significantly. It can obscure important features in images, complicating subsequent analysis or processing tasks. For example, in medical imaging, noise could potentially hinder the ability to diagnose conditions accurately. By comparing images with and without noise, one can see notable differences in sharpness and detail.
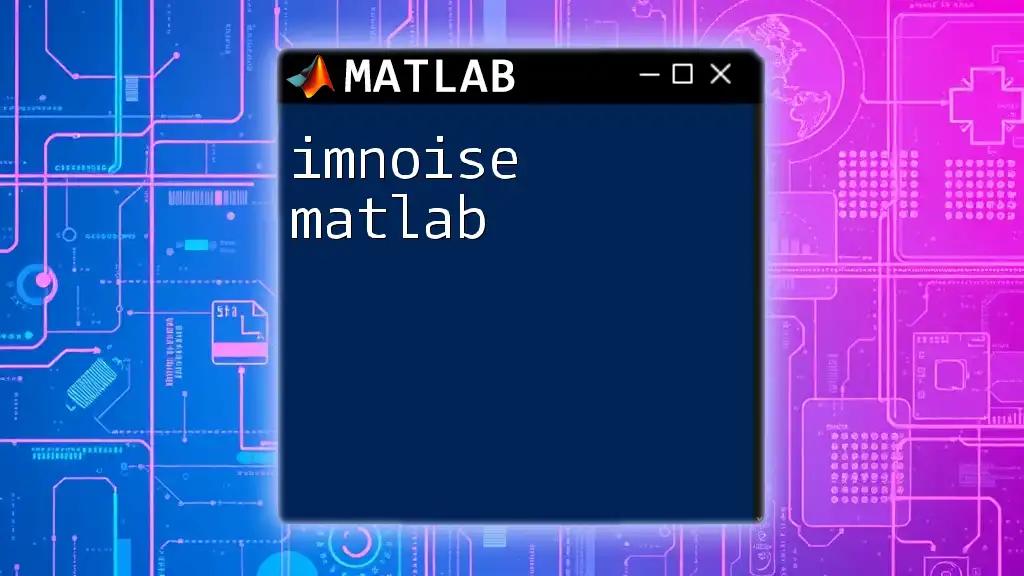
Overview of `imnoise` Function
What is `imnoise`?
The `imnoise` function in MATLAB is a powerful tool designed to add noise to images for the purpose of simulation and testing image processing algorithms. By artificially introducing noise, developers can evaluate the performance of various filtering techniques and other algorithms under realistic conditions.
Syntax of `imnoise`
The general syntax for using the `imnoise` function is as follows:
B = imnoise(A, 'type', parameters)
The parameters in this syntax are crucial to understanding how noise is applied. Here, `A` is the original image, `'type'` specifies the kind of noise you want to add (e.g., 'gaussian', 'salt & pepper', or 'poisson'), and `parameters` vary depending on the noise type.
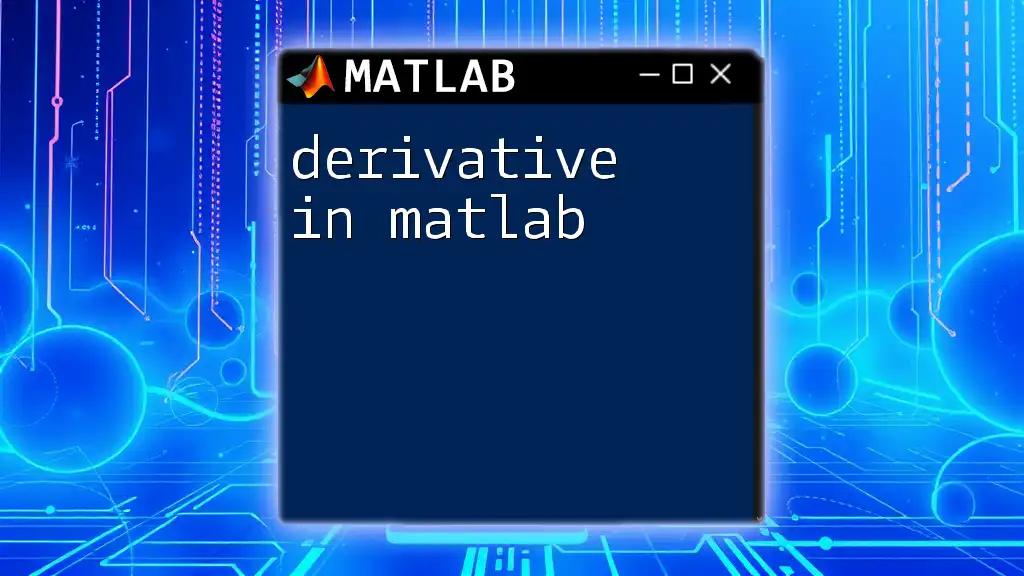
Types of Noise You Can Add with `imnoise`
Adding Gaussian Noise
Gaussian noise can be easily added to an image using the `imnoise` function. This is done by specifying the mean and variance of the noise you wish to introduce.
Here’s how to add Gaussian noise:
A = imread('image.jpg');
B = imnoise(A, 'gaussian', 0, 0.01);
imshow([A B]);
In this example, the parameters `0` and `0.01` indicate that the noise has a mean of 0 and a variance of 0.01. Adjusting these values can affect how pronounced the noise appears in the image.
Adding Salt and Pepper Noise
Salt and pepper noise can also be added easily. It is defined by a parameter, `density`, which determines the proportion of pixels affected by the noise.
To add salt and pepper noise, use the following code:
B = imnoise(A, 'salt & pepper', 0.02);
imshow([A B]);
Here, `0.02` indicates that 2% of the pixels in the image will be modified. You can adjust this value to see how it impacts the image visibly.
Adding Poisson Noise
Adding Poisson noise is straightforward and does not require additional parameters aside from the image itself. Here’s an example:
B = imnoise(A, 'poisson');
imshow([A B]);
This command simulates noise that is statistically consistent with Poisson distribution, often mimicking the behavior of light detection in real-world applications.
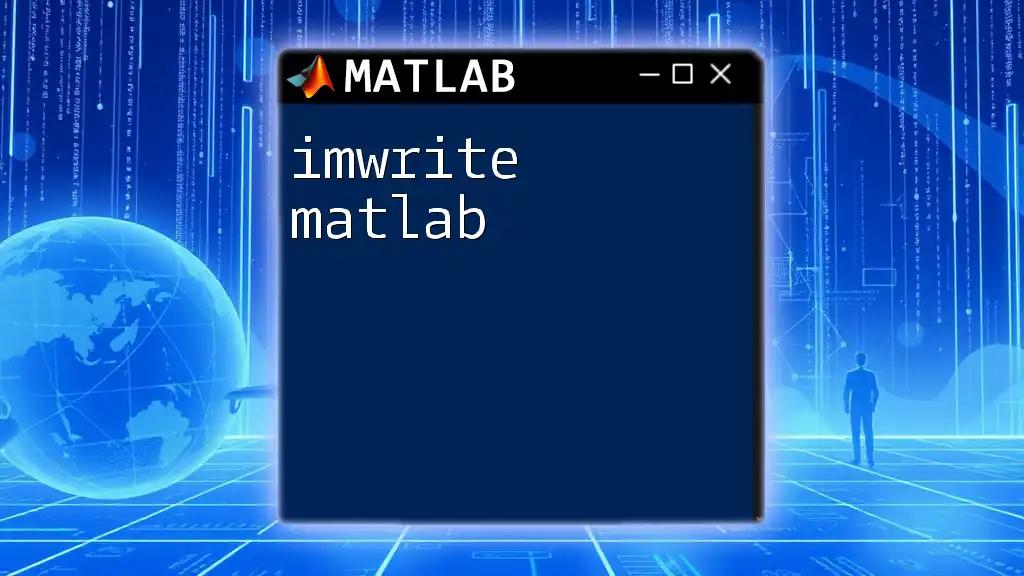
Practical Applications of `imnoise`
Enhancing Image Processing Algorithms
The capability to introduce noise via `imnoise` is invaluable in the realm of image processing, particularly for testing filtering algorithms. For example, when applying a median filter to combat salt and pepper noise, the code looks like this:
B = medfilt2(B);
imshow([A B]);
Here, the median filter helps to remove the noise while preserving edges in the image, which is vital for accurate image interpretation.
Simulating Real-world Conditions
In many applications, particularly in industries like healthcare and remote sensing, accurately simulating noise is critical for developing robust applications. The `imnoise` function allows developers to create various scenarios that mimic real-world conditions, which in turn enables them to evaluate the efficacy of image processing techniques thoroughly.
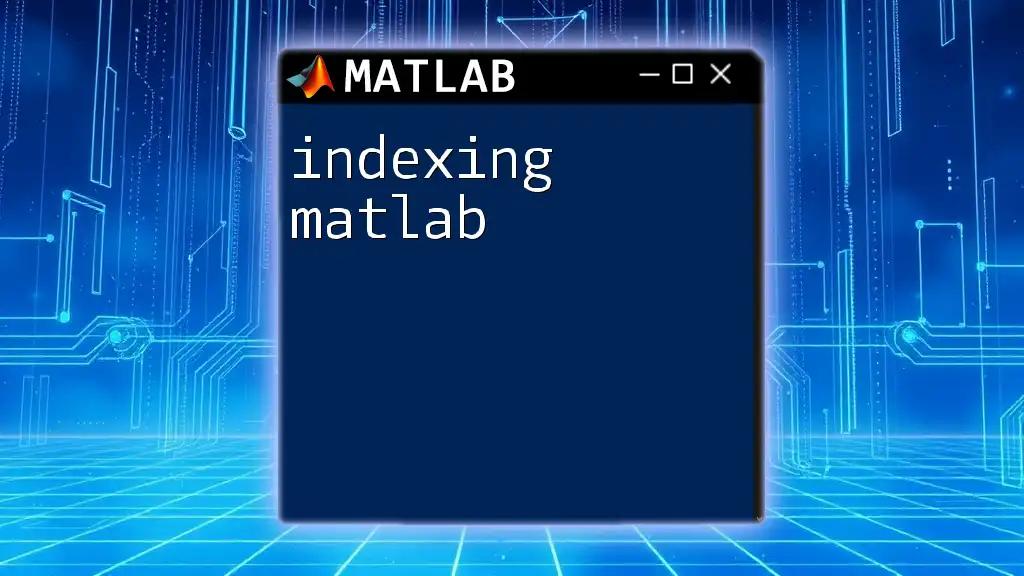
Visualizing the Effects of Noise
Displaying Original and Noisy Images Side by Side
To better understand the impact of added noise, comparing the original and noisy images side by side can be quite effective. Use the following code structure to achieve this:
figure;
subplot(1, 2, 1);
imshow(A);
title('Original Image');
subplot(1, 2, 2);
imshow(B);
title('Image with Added Noise');
This visual representation helps in observing the changes and understanding the type of noise introduced.
Using Histograms to Analyze Noise Impact
Furthermore, analyzing the histograms of the original and noisy images can provide deeper insights into how noise alters pixel intensity distributions:
figure;
subplot(1, 2, 1);
imhist(A);
title('Histogram of Original Image');
subplot(1, 2, 2);
imhist(B);
title('Histogram of Noisy Image');
Such histogram analyses can be crucial for parameter tuning in noise removal algorithms.
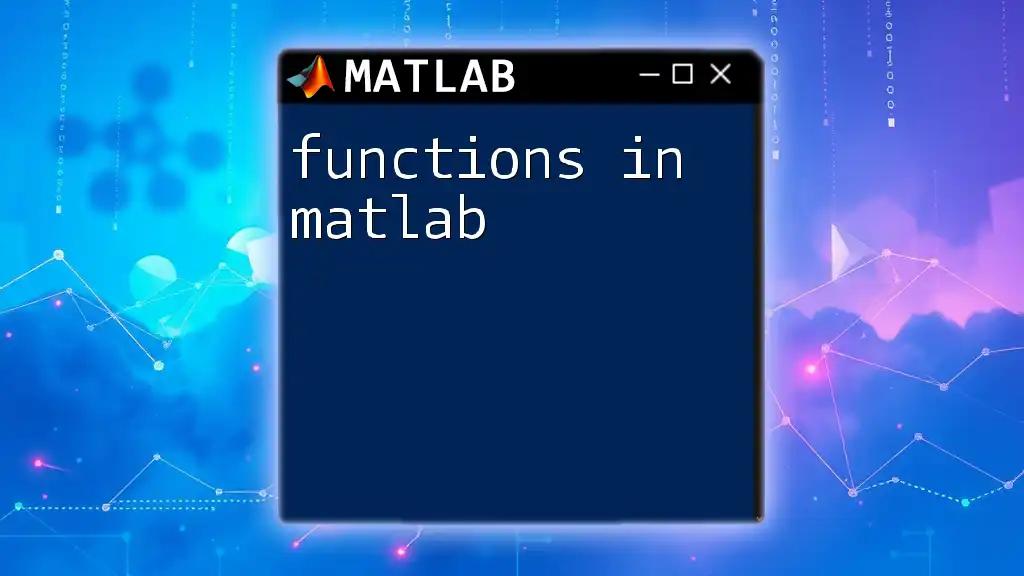
Tips and Best Practices
Choosing Noise Parameters
Choosing the right parameters for different noise types is vital in ensuring your simulations are realistic and applicable. Consider the specific type of noise in your domain—different applications may have different typical noise characteristics.
Dealing with Noise Post-Processing
It is also essential to understand methods for removing noise after it has been added. Techniques such as median filtering, Wiener filtering, or Gaussian smoothing can help mitigate the effects of introduced noise and restore image quality.

Conclusion
The `imnoise` function in MATLAB is a powerful tool for those looking to simulate the effects of noise in image processing. By experimenting with this function, you can gain insights into real-world scenarios and enhance your image processing algorithms.
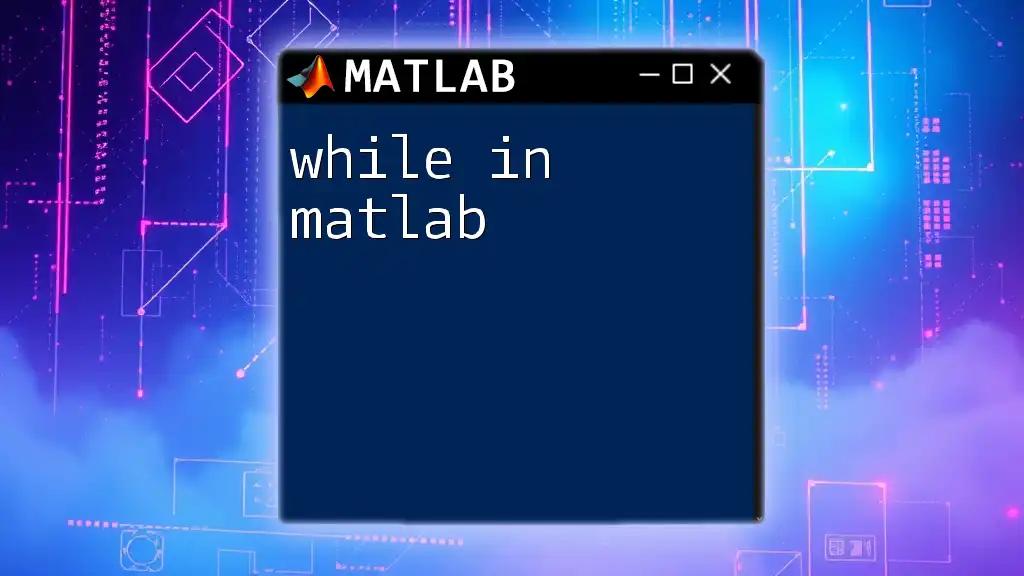
Additional Resources
Official Documentation
For more in-depth information, you can always check MATLAB’s official documentation for the `imnoise` function.
Tutorial Videos
Consider looking for tutorial videos on platforms like YouTube, where experienced developers share visual guides on effectively using `imnoise` in MATLAB.
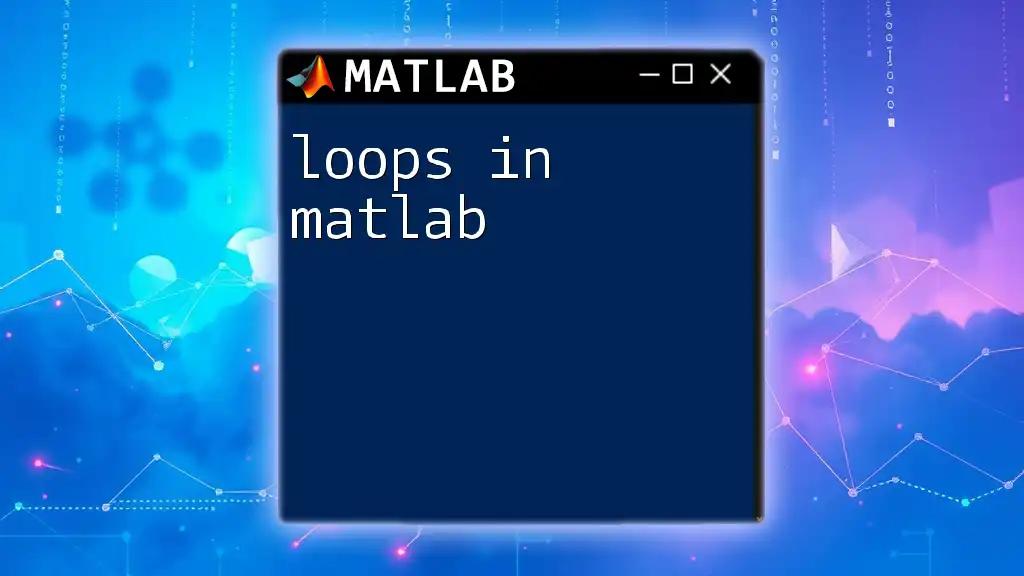
Call to Action
As you dive into the world of image processing, practice with `imnoise` to understand the impact of various kinds of noise fully. Subscribe to our blog for more tips and tricks on MATLAB commands and image processing!