In MATLAB, the `abs` function is used to compute the absolute value of a number or the magnitude of a complex number.
Here’s a simple code snippet demonstrating the use of the `abs` function:
% Calculate the absolute value
value = -5;
absoluteValue = abs(value);
disp(absoluteValue); % Displays: 5
What is Absolute Value?
Absolute value is a mathematical concept that reflects the distance of a number from zero on the number line, without considering direction. In simpler terms, it transforms negative numbers into positive ones while leaving positive numbers unchanged. For example, the absolute value of -5 is 5, and the absolute value of 3 is still 3.
In programming, using absolute values is important as it can help avoid complications caused by negative numbers, ensuring that computations yield valid and meaningful results.
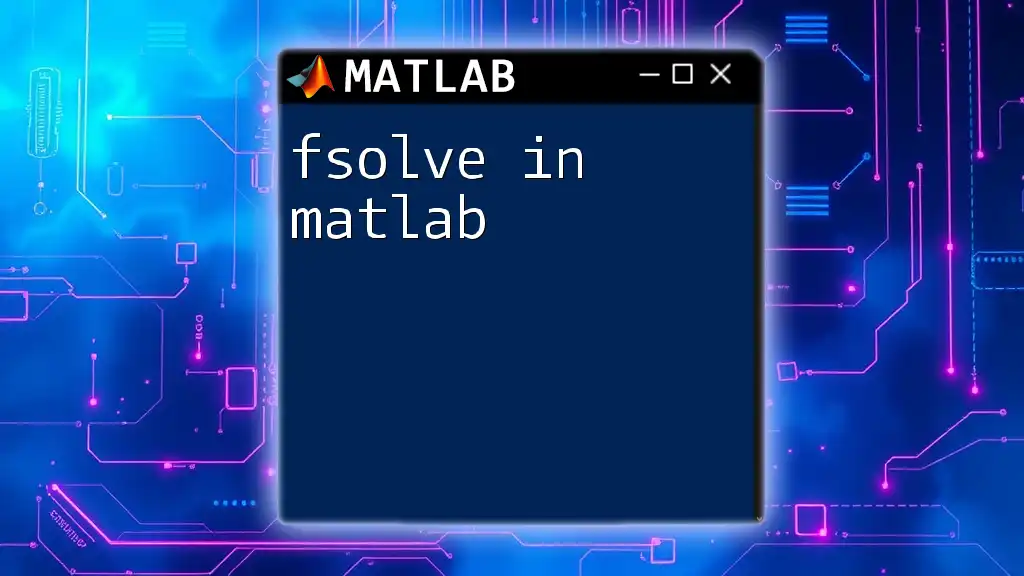
Understanding the abs() Function
What is the abs() Function?
In MATLAB, the `abs()` function is the primary tool to calculate absolute values. Its syntax is straightforward: `B = abs(A)`, where `A` represents the input and `B` is the output containing the absolute values.
One of the significant advantages of using `abs()` is its ability to handle various types of inputs seamlessly, making it versatile for different applications.
Input Types
Scalars and Vectors
The `abs()` function processes scalar and vector inputs effectively. Here are a few examples:
a = -5;
b = abs(a); % Output will be 5
vec = [-1, -2, 3; -4, 5, -6];
mat_abs = abs(vec); % Output: [1, 2, 3; 4, 5, 6]
As demonstrated, when the `abs()` function is applied to a scalar or a vector, it returns a new matrix of the same size, with every element replaced by its absolute value.
Complex Numbers
When working with complex numbers, the `abs()` function calculates the magnitude of the complex number, which is the distance of that number from the origin in the complex plane.
For example:
z = 3 + 4i;
abs_z = abs(z); % Output will be 5 (magnitude)
In this case, the `abs(z)` computes the magnitude using the Pythagorean theorem, resulting in 5, which is calculated as √(3² + 4²).
Matrix Norms
The notion of absolute values is also crucial when computing matrix norms. For example, the L1-norm and L2-norm can be derived using the `abs()` function.
A = [-1, 2; -3, 4];
L1_norm = sum(abs(A), 'all'); % Computes the L1 norm
This example shows how the absolute values of all elements are summed to determine the L1-norm, a useful measure of vector magnitude.
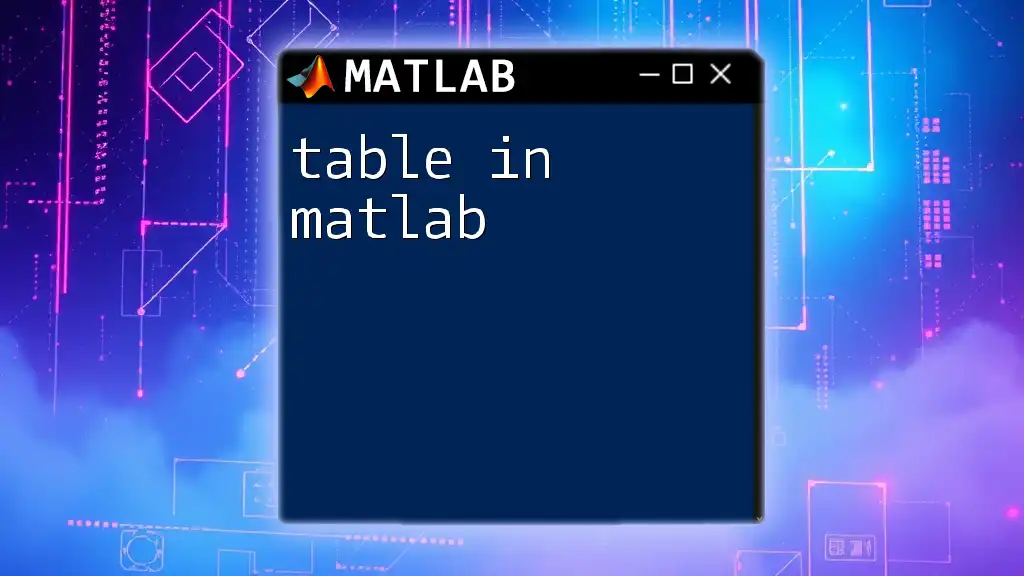
Practical Applications of Absolute Values
Data Analysis
In data analysis, absolute values play a prominent role, particularly in data cleaning processes. They can help eliminate negative values from datasets that may not be meaningful or can mislead interpretations. For instance, if you're dealing with absolute temperature readings, using the `abs()` function ensures you only work with logical responses.
Signal Processing Tools
In signal processing, absolute values are used in filtering and noise reduction. They help in analyzing and visualizing signals by transforming noisy data into a clearer representation. An example of filtering a signal using `abs()` might look like this:
% Simulate a noisy signal
t = linspace(0, 1, 100);
signal = sin(2 * pi * 5 * t) + 0.5 * randn(size(t));
% Apply absolute function to the noisy signal
filtered_signal = abs(signal);
After applying the absolute value, the output represents the absolute amplitude of the noisy signal, making it easier to analyze subsequent patterns or cycles.
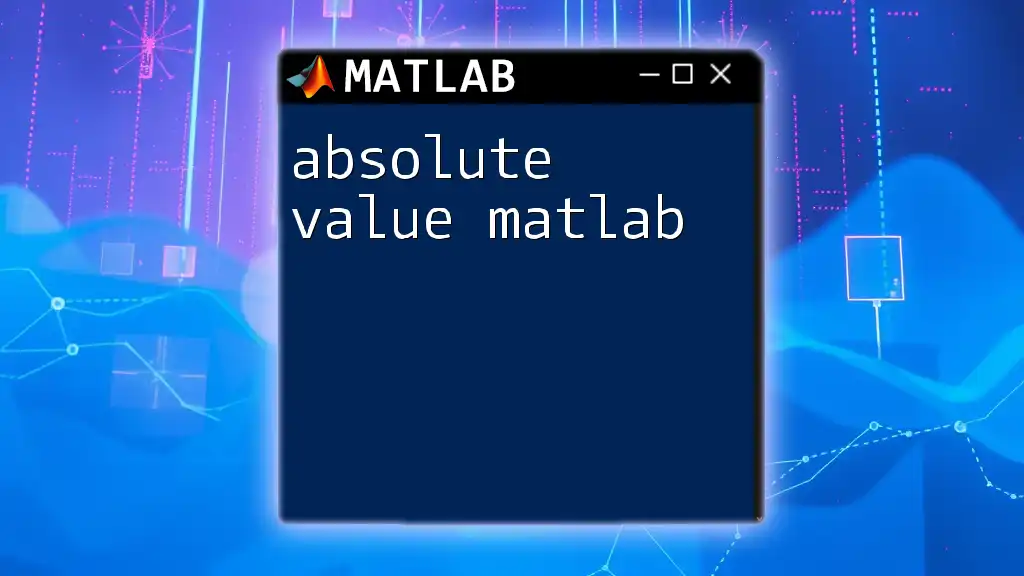
Performance Considerations
Efficiency of the abs() Function
When working with large datasets or matrices, performance can become a significant factor. The `abs()` function is optimized for these operations, allowing for quick calculations even on extensive arrays.
Profiling and Optimization Tips
For optimal performance, especially with large datasets, leverage vectorized operations using `abs()`. This approach avoids loops, thus improving execution time. For example:
large_vector = randn(1, 1e6);
abs_large_vector = abs(large_vector);
This code quickly computes the absolute values for a million elements using vectorization, making it efficient and compact.
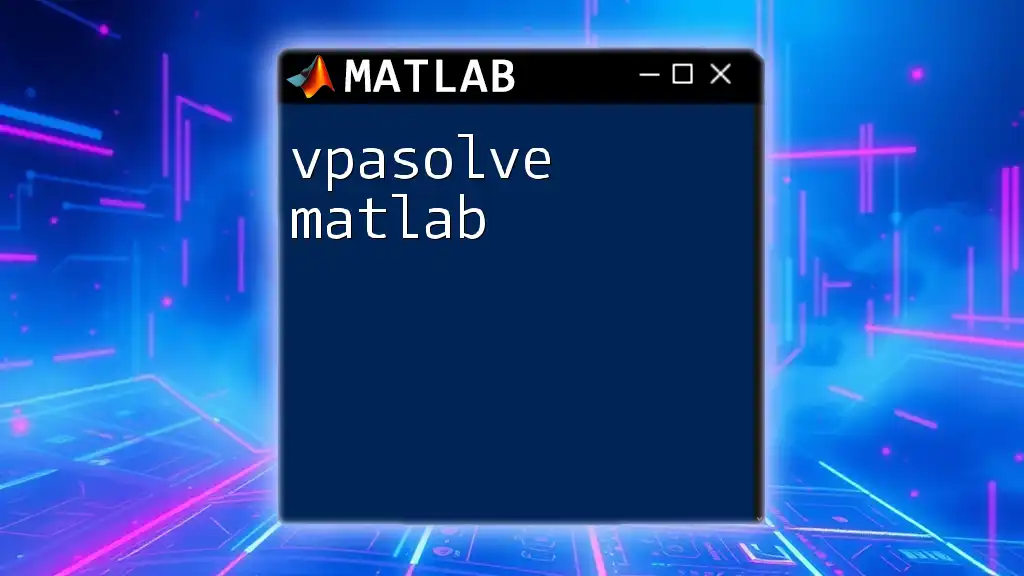
Common Mistakes and Troubleshooting
Mistakes with Complex Inputs
While `abs()` is generally straightforward, common mistakes include using it on non-numerical data types like strings or logic variables. For instance:
wrong_input = abs('a'); % This will produce an error
Debugging Tips
If you encounter unexpected results when using the `abs()` function, double-check the input type and ensure it’s numerical. Utilizing MATLAB’s built-in functions like `isnumeric()` can help diagnose issues early.
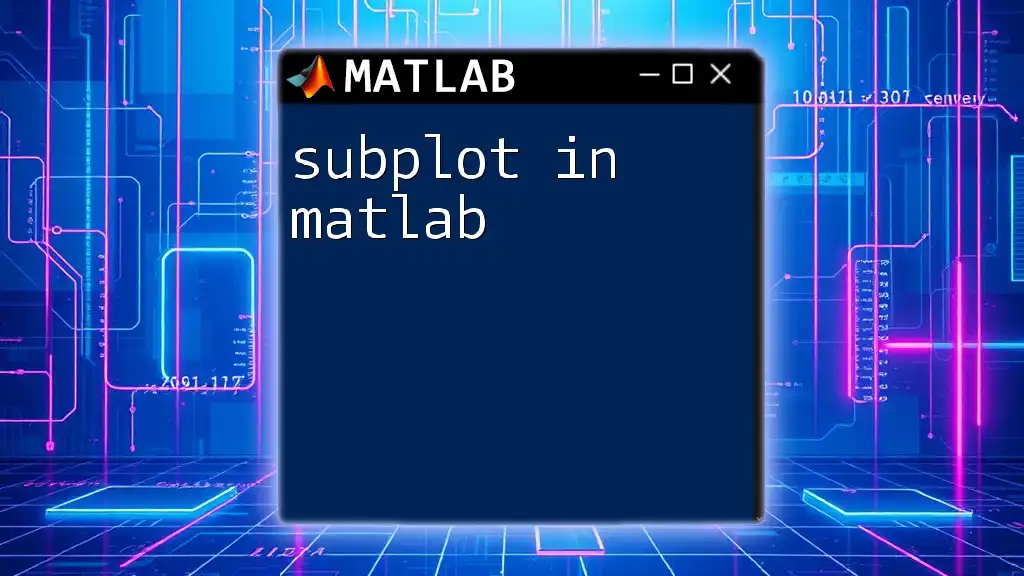
Conclusion
Understanding and utilizing the absolute in MATLAB through the `abs()` function is crucial for anyone working in mathematical computing, data analysis, or programming. It simplifies many operations while ensuring accurate results in complex calculations. To further your understanding, practice using `abs()` in different scenarios and explore its role in larger projects.
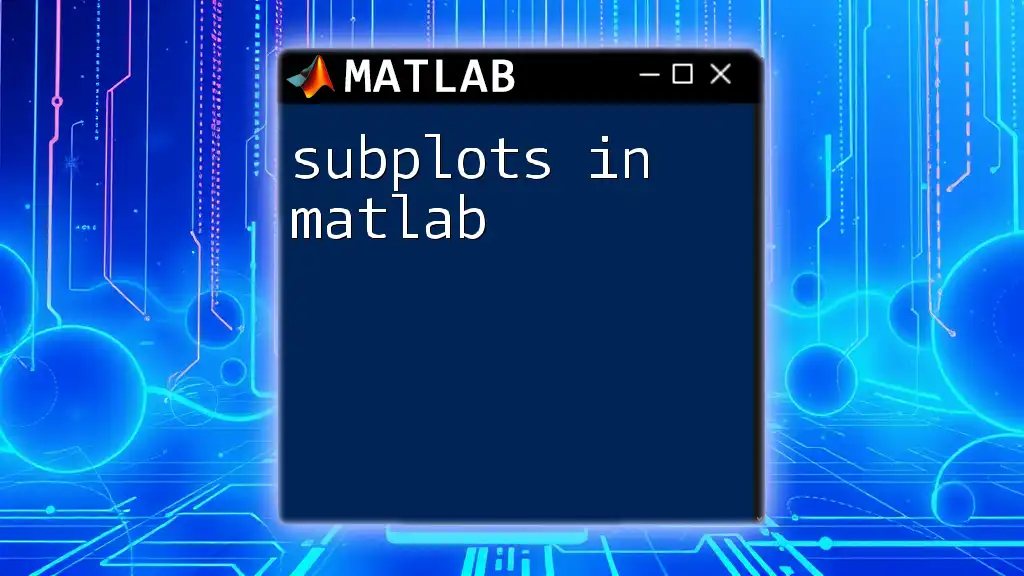
Frequently Asked Questions (FAQs)
What if my input contains NaN values?
The `abs()` function will return NaN for any input values that are NaN, and it's essential to handle these scenarios properly. You can use the `isnan()` function before applying `abs()` to exclude or replace NaN values.
How is `abs()` different from other functions?
Unlike functions like `sign()`, which only return either -1, 0, or 1 based on the input sign, `abs()` focuses on providing the numerical absolute value, ensuring all returned values are non-negative.
By incorporating these key concepts and practices surrounding absolute in MATLAB, you can enhance your coding efficiency and precision while solving problems effectively.