Eigenvalues in MATLAB can be computed using the `eig` function, which takes a square matrix as input and returns its eigenvalues and eigenvectors. Here's a simple example:
A = [4, 2; 1, 3]; % Define a 2x2 matrix
[eigenvectors, eigenvalues] = eig(A); % Compute eigenvalues and eigenvectors
disp(diag(eigenvalues)); % Display the eigenvalues
Understanding Eigenvalues
What are Eigenvalues?
Eigenvalues are fundamental numbers associated with a square matrix that provide insight into the matrix's properties. Specifically, they arise from the eigenvalue equation \(A \mathbf{v} = \lambda \mathbf{v}\), where \(A\) is a square matrix, \(\mathbf{v}\) is the corresponding eigenvector, and \(\lambda\) is the eigenvalue. In simpler terms, eigenvalues help us understand how a transformation represented by the matrix affects certain vectors (eigenvectors) in a vector space.
Why Use MATLAB for Eigenvalues?
MATLAB excels at performing matrix calculations, making it an ideal choice for users who seek to compute eigenvalues efficiently. Its robust environment allows for quick implementation of linear algebra techniques, enabling both seasoned mathematicians and newcomers to apply concepts seamlessly.
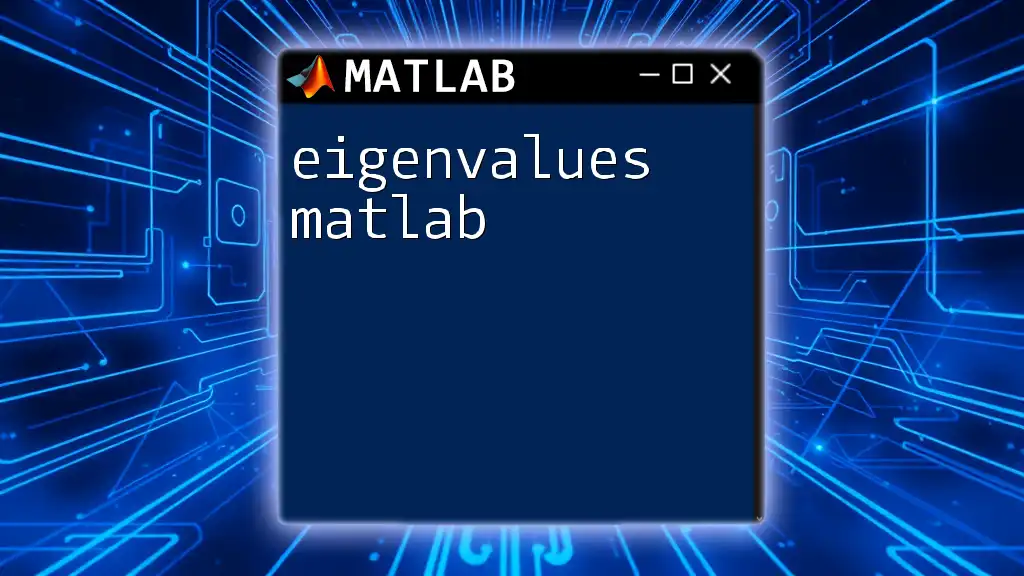
Mathematical Foundation
Eigenvalue Equation
To grasp the concept of eigenvalues, it's essential to understand the eigenvalue equation. Given a square matrix \(A\), if there exists a non-zero vector \(\mathbf{v}\) such that multiplying \(A\) by \(\mathbf{v}\) results in a scalar multiplication of \(\mathbf{v}\) by \(\lambda\), then \(\lambda\) is an eigenvalue of \(A\). The vectors \(\mathbf{v}\) that satisfy this condition are termed eigenvectors.
Spectral Theorem Overview
The spectral theorem states that any symmetric matrix can be diagonalized by an orthogonal matrix, meaning you can express it as \(A = VDV^T\), where \(D\) is a diagonal matrix of eigenvalues, and \(V\) contains the orthogonal eigenvectors. This theorem is pivotal in various applications, from stability analysis to PCA.
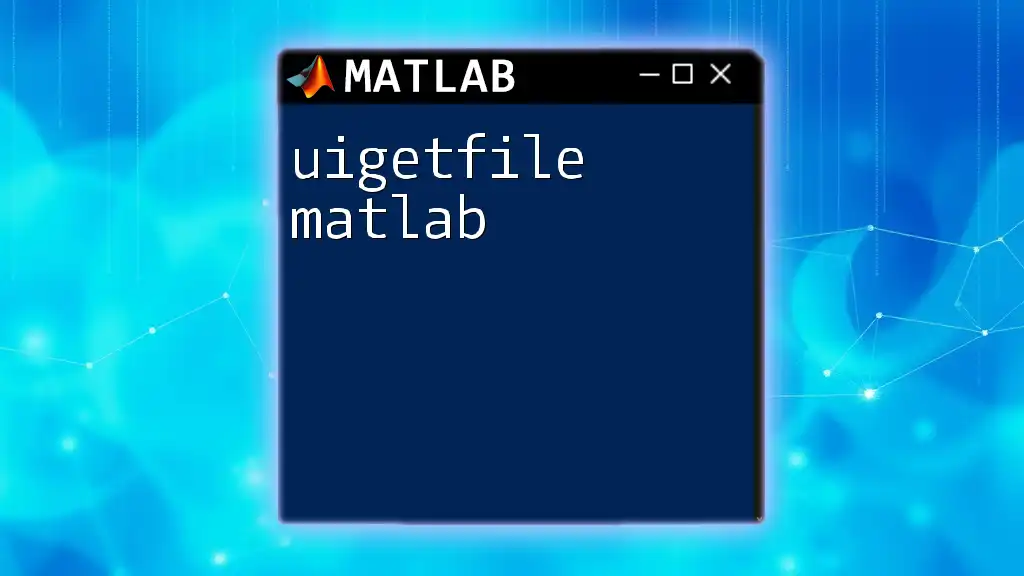
Calculating Eigenvalues in MATLAB
Using `eig` Function
In MATLAB, the primary function used to compute eigenvalues is `eig`. The syntax for this function is straightforward:
[V, D] = eig(A)
where:
- `A` is the input square matrix.
- `V` is the matrix of eigenvectors.
- `D` is a diagonal matrix containing the eigenvalues on its diagonal.
For example, consider the matrix:
A = [4, 2; 1, 3];
[V, D] = eig(A);
disp('Eigenvectors:'); disp(V);
disp('Eigenvalues:'); disp(diag(D));
When you run this code, MATLAB will return the eigenvectors and the eigenvalues of matrix \(A\). The eigenvectors are the columns of matrix \(V\), whereas the eigenvalues can be found along the diagonal of matrix \(D\).
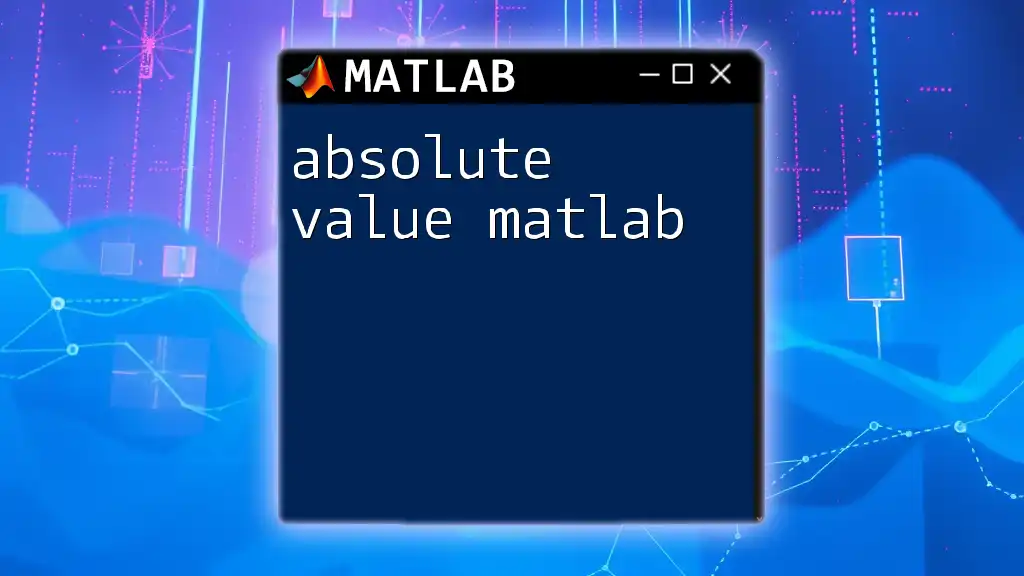
Practical Applications of Eigenvalues
Eigenvalues in Data Analysis
One of the essential applications of eigenvalues is in data analysis, particularly in Principal Component Analysis (PCA). PCA involves transforming data into a set of orthogonal components that maximize variance. It employs the covariance matrix to identify the axes (principal components) along which the variance is maximized.
To compute the eigenvalues of a covariance matrix in MATLAB, you can use the following code snippet:
data = randn(100, 5); % Simulated dataset
covarianceMatrix = cov(data);
[V, D] = eig(covarianceMatrix);
disp('Eigenvalues of the covariance matrix:'); disp(diag(D));
Here, `data` is a matrix containing random numbers. The covariance matrix is calculated using the `cov` function. The eigenvalues you get from `D` reflect the variance explained by each principal component.
Dynamic Systems and Stability Analysis
Eigenvalues play a crucial role in analyzing dynamic systems and stability. In control theory, the stability of a system can be assessed by examining the eigenvalues of its state-space model's system matrix.
For example, if we define a simple state-space model with the system matrix \(A\):
A = [0, 1; -2, -3]; % System matrix
eigenvalues = eig(A);
disp('Eigenvalues of the system:'); disp(eigenvalues);
The eigenvalues derived from this system matrix can indicate whether the system will stabilize or diverge over time. If all eigenvalues have negative real parts, the system is stable.
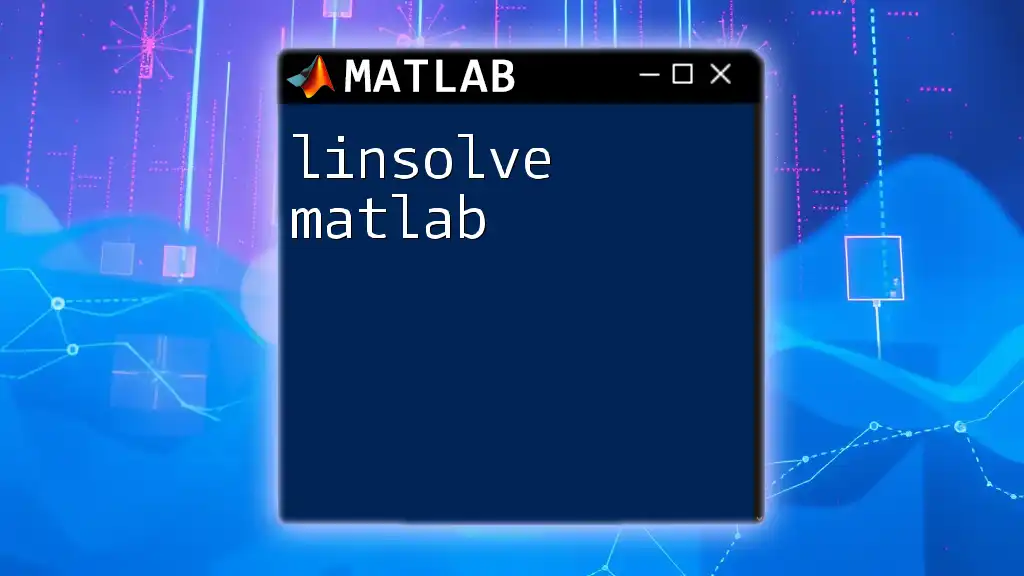
Advanced Eigenvalue Techniques in MATLAB
More on the `eig` Function
In advanced applications, you might only need a specific number of the largest or smallest eigenvalues, especially when dealing with large matrices. Using the `eigs` function instead of `eig` allows for this flexibility:
opts.issym = true;
opts.ichol = 'lower';
[V, D] = eigs(A, k, 'largestabs', opts);
In this code:
- `k` specifies how many eigenvalues to compute.
- The `opts` parameter allows for further optimization, which is useful in large-scale problems, significantly reducing computational time.
Dealing with Complex Eigenvalues
In some instances, you may encounter complex eigenvalues, particularly with matrices that represent rotations or oscillations. To demonstrate how to compute complex eigenvalues, consider the matrix:
A = [0, -1; 1, 0]; % Rotation matrix
[V, D] = eig(A);
disp('Eigenvalues of the rotation matrix:'); disp(diag(D));
Running this will yield eigenvalues in the form \(i\) and \(-i\), indicating that the system represents a pure rotation in the complex plane.
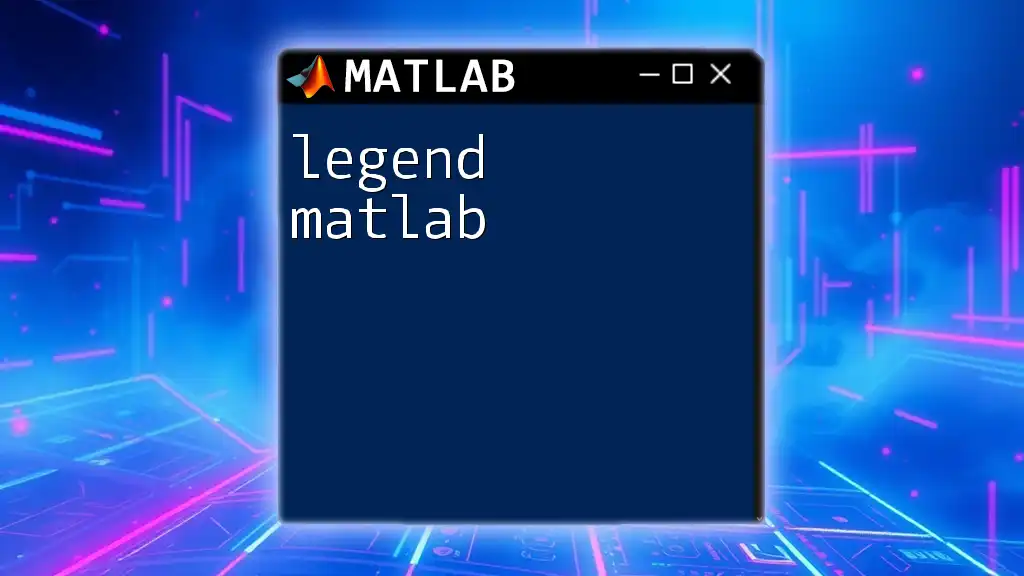
Common Issues and Debugging Tips
Handling Non-Square Matrices
It is crucial to remember that eigenvalues are only defined for square matrices. Attempting to compute eigenvalues for a non-square matrix in MATLAB results in an error. Always ensure the input matrix is square.
Singular and Near-Singular Matrices
When dealing with singular or nearly singular matrices (matrices with eigenvalues very close to zero), you might receive unexpected results. Using `pinv(A)` instead of `inv(A)` can help in such cases, as it calculates the pseudoinverse of the matrix.
Resolving Computational Errors
Common errors while using the `eig` function often arise due to wrongly formatted input (such as invalid matrix dimensions). Always double-check the size and structure of your input matrix to avoid these pitfalls.
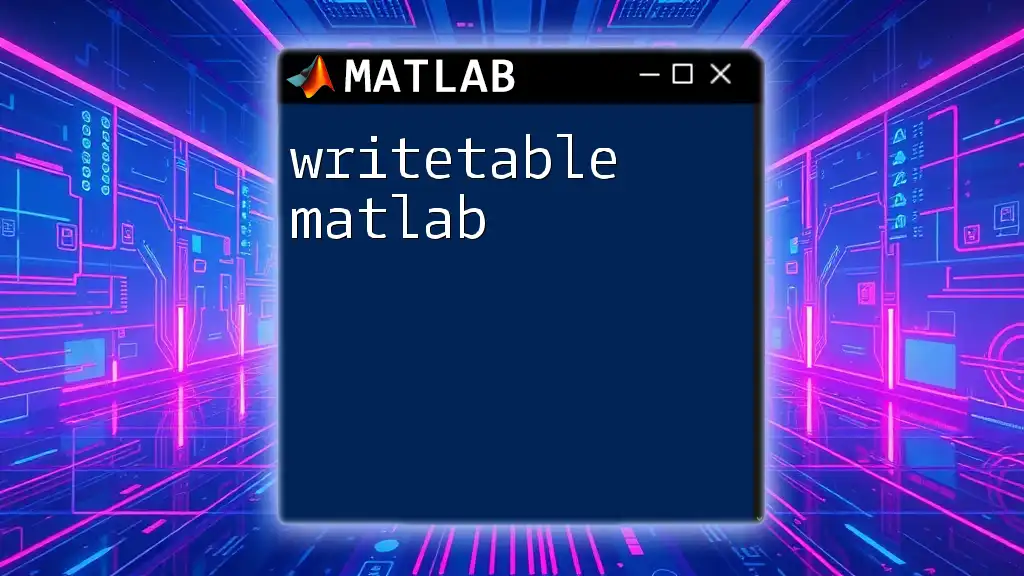
Conclusion
In summary, the concept of eigenvalues is a powerful tool in MATLAB that can radically enhance the analysis of various mathematical problems. By understanding how to utilize the `eig` function effectively, users can investigate a myriad of applications from PCA to stability analysis in dynamic systems. Continued learning and practice with eigenvalues will deepen your analytical capabilities and provide valuable insights into a variety of fields.
FAQs about Eigenvalues in MATLAB
This section will address common questions regarding the computation of eigenvalues, their implications in different applications, and tips for beginners new to MATLAB.
Emphasizing these key points will help reinforce your understanding and mastery of the topic eigenvalue MATLAB.