The `writetable` function in MATLAB is used to write a table to a file, allowing users to easily export their data to formats like `.csv` or `.xlsx`.
Here’s a syntax example using `writetable`:
% Create a sample table
data = table([1; 2; 3], {'A'; 'B'; 'C'}, 'VariableNames', {'Number', 'Letter'});
% Write the table to a CSV file
writetable(data, 'output.csv');
Overview of writetable Function
The `writetable` function in MATLAB provides an efficient way to write tables into text or spreadsheet files. It allows you to store your data in a format that is easily accessible and usable by other software or platforms. This is particularly important for data sharing, reporting, and further analysis when collaborating with others who may not use MATLAB.
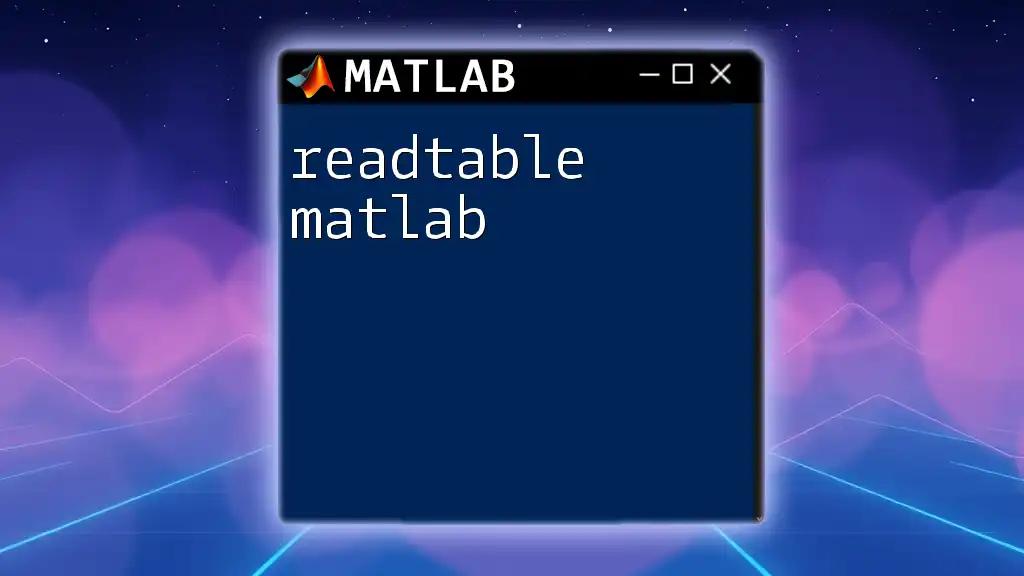
Common Use Cases
You might frequently use `writetable` when you need to:
- Export results from data analysis or experiments.
- Share datasets with colleagues or clients.
- Save processed data for future use.
- Prepare files for reporting, visualization, or further statistical analysis.
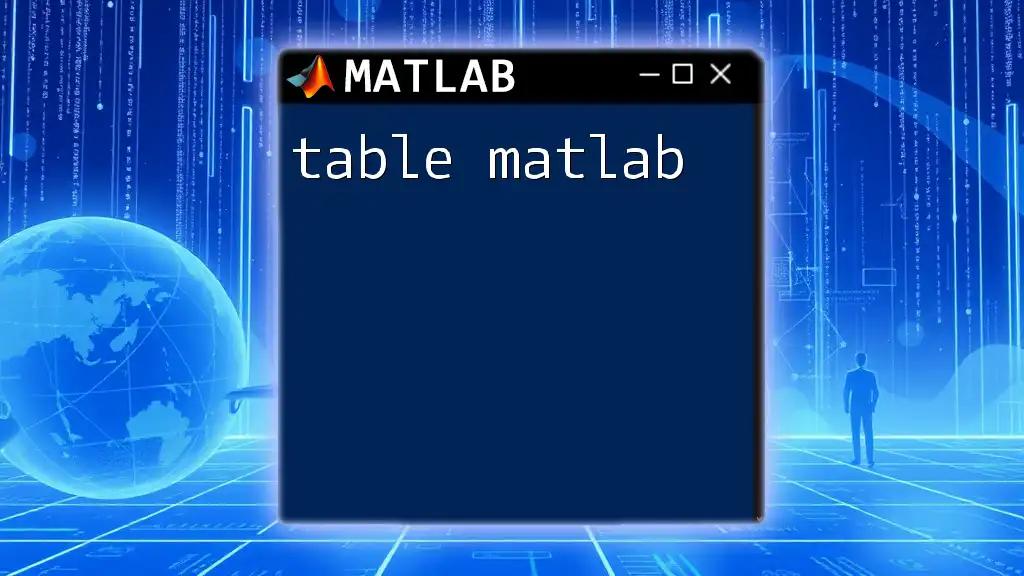
Creating Sample Data for Demonstration
Before learning how to use the `writetable` function, it is essential to create a sample dataset. Here is how you can create a simple table in MATLAB:
data = table([1; 2; 3], ["A"; "B"; "C"], 'VariableNames', {'ID', 'Category'});
In this example, we created a table with two variables: `ID` and `Category`. This table will serve as our sample dataset for demonstrating the `writetable` function.
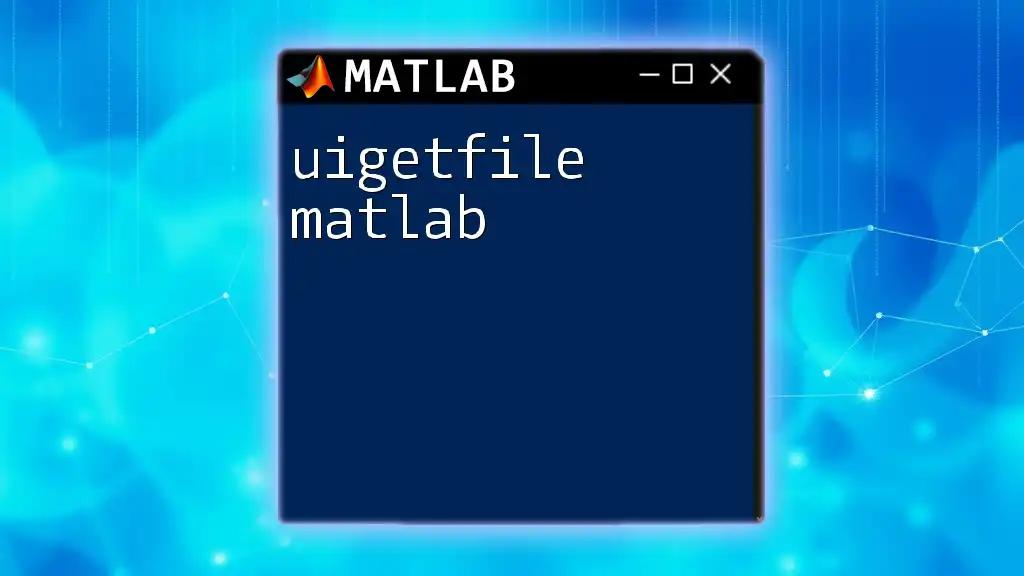
Basic Syntax of writetable
The basic syntax for the `writetable` function is straightforward:
writetable(T, filename)
In this syntax:
- T refers to the table that you want to write.
- filename is the name or path of the file where the table will be saved.
This means that by simply specifying the table and the desired filename, you can efficiently export your data.
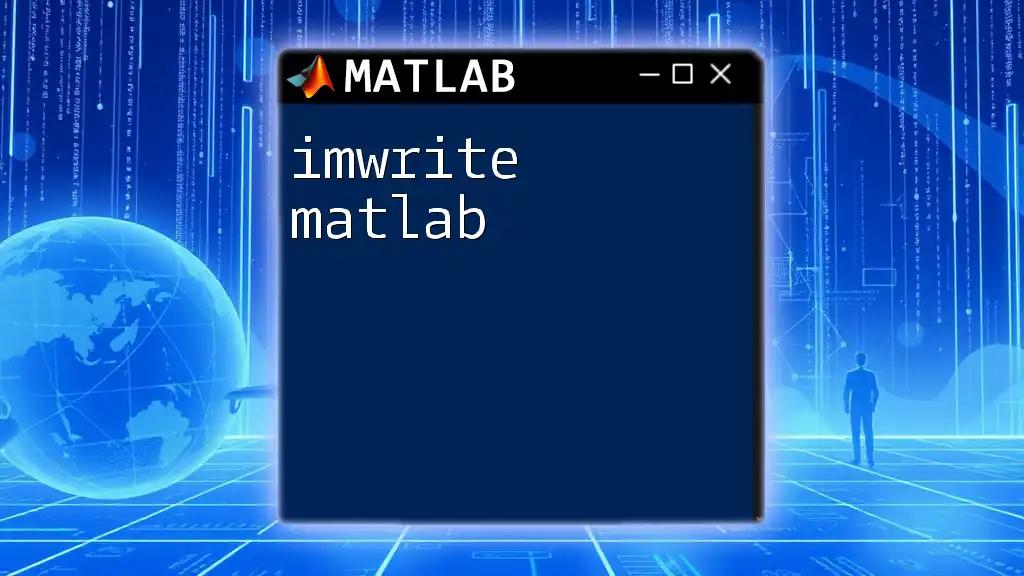
Writing Tables to Files
Writing to a CSV File
One of the most common uses of the `writetable` function is saving tables as CSV files. Here's how to do it:
writetable(data, 'data.csv');
When you run this code, MATLAB will create a file named `data.csv` in the current working directory and write the contents of the `data` table into it. An important feature of the `writetable` function is that it automatically includes the variable names in the first row of the output file, which enhances data readability.
Writing to Excel Files
Saving tables in Excel format is also a popular choice, especially for users who prefer spreadsheet applications. To write a table to an Excel file, you can use the following command:
writetable(data, 'data.xlsx');
This command creates an `Excel` file named `data.xlsx` in your working directory. You can also specify additional options such as the sheet name and range by adding parameters like this:
writetable(data, 'data.xlsx', 'Sheet', 'Sheet1', 'Range', 'A1');
This code snippet specifies that the data should be written to `Sheet1`, starting from cell `A1`.
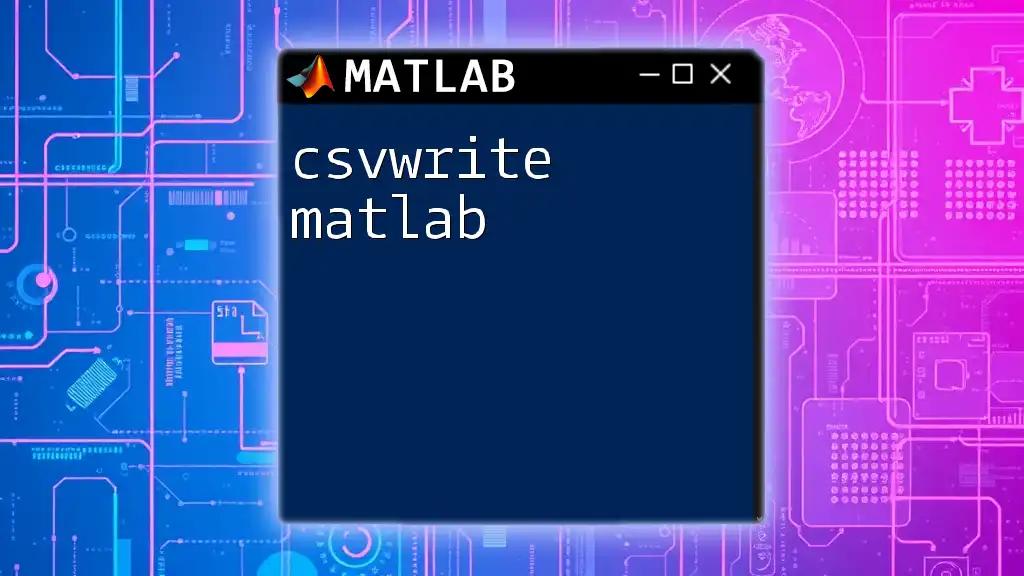
Advanced Options in writetable
Specifying Delimiters
If you need to save your table in a different format, such as a text file with specific delimiters, `writetable` allows you to customize this. For example, to save the table with a tab delimiter, you can use:
writetable(data, 'data.txt', 'Delimiter', '\t');
Using different delimiters can enhance compatibility with other applications that may require specific formats.
Controlling Variable Formatting
When saving your table, you can control whether or not to include variable names. Here’s how you can ensure the variable names are included:
writetable(data, 'formatted_data.csv', 'WriteVariableNames', true);
Conversely, if you prefer not to include variable names, simply set this option to `false`.
Handling Row Names
Flexibility in handling row names is another powerful feature of `writetable`. By default, rows are numbered, but you can specify to include row names (if they exist):
writetable(data, 'data_withRowNames.csv', 'WriteRowNames', true);
This option can be particularly useful when your data has meaningful row names that contribute to data analysis and interpretation.
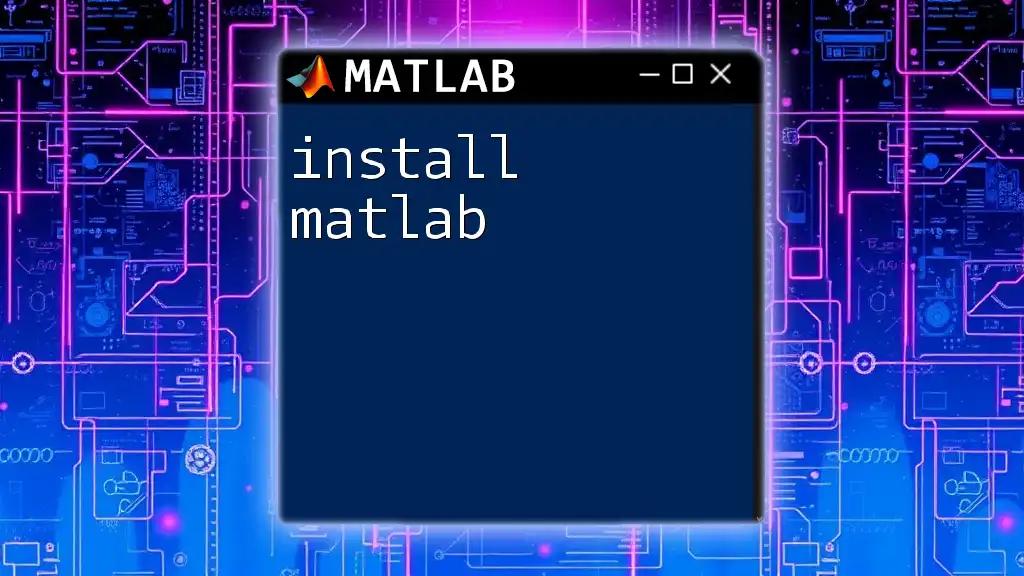
Common Errors When Using writetable
While using the `writetable` function is generally straightforward, users may encounter specific errors. Common issues include:
- Incorrect File Paths: Ensure that your specified filename path exists and is writable.
- Data Types: Ensure that your table contains data types compatible with the output format. For example, cell arrays may not export correctly to CSV.
To troubleshoot these issues, check the MATLAB error messages, which often provide guidance on what went wrong.
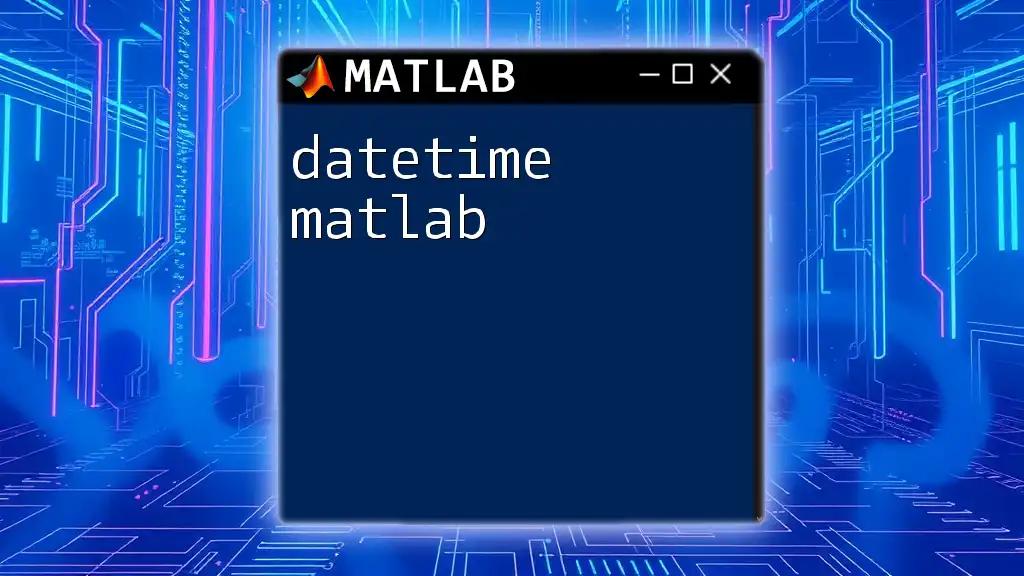
Best Practices for Creating and Saving Tables
To ensure that your data is well-structured and easily exportable, consider these best practices:
- Structure Your Data: Ensure that your data is organized into a table format, with appropriate variable types for each column.
- Use Meaningful Names: Name your tables and variables descriptively, making it easier for others (and yourself) to understand the data context.
- File Naming Conventions: Adopt consistent file naming conventions that include dates or versioning, which will help in file management.
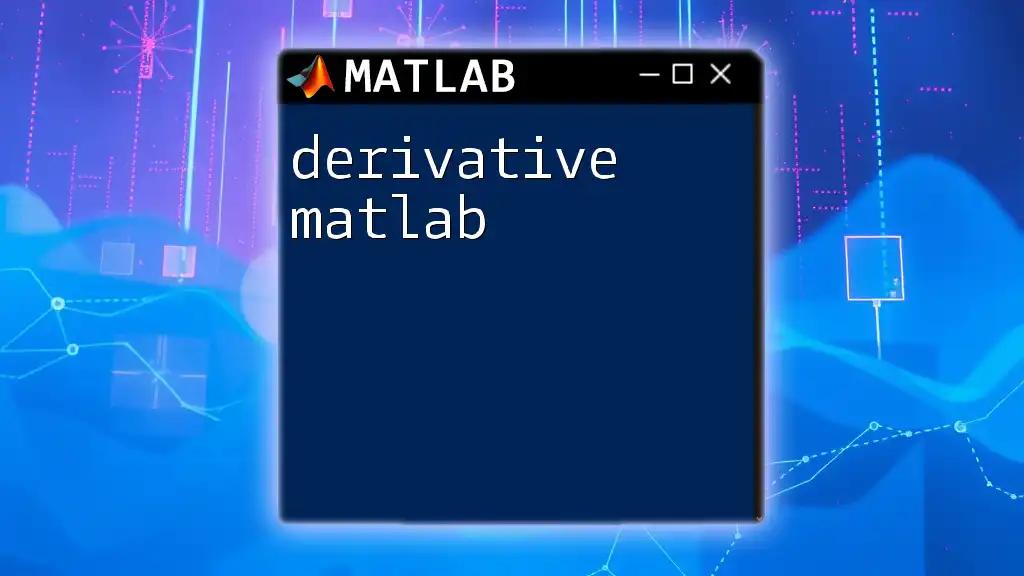
Case Study: Exporting Analysis Results
Consider a scenario where you perform analysis on a dataset and wish to export the results for sharing with a colleague. After generating new insights, your table might look like this:
results = table([1; 2; 3], [0.8; 0.9; 0.95], 'VariableNames', {'Experiment', 'Accuracy'});
To share this with your team, you can export it as follows:
writetable(results, 'experiment_results.csv');
This convenience ensures that your work is open for peer review or additional research collaboration, facilitating a smoother workflow.
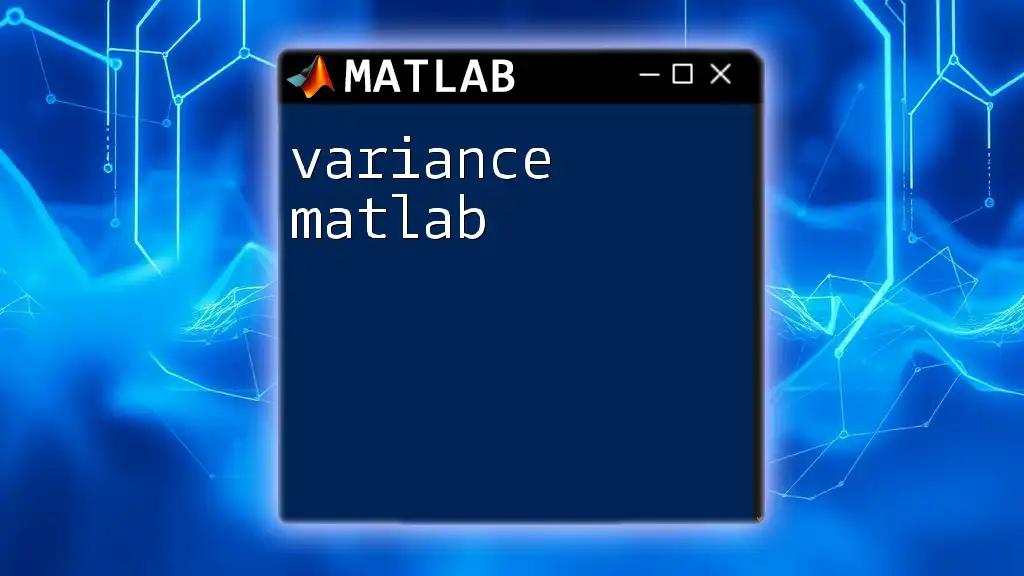
Integrating with Other Functions
The `writetable` function can be seamlessly integrated into more extensive data processing applications. Coupling it with functions like `readtable` enables a smooth transition between data imports and exports, making it easier to manage your datasets efficiently.
For instance, after reading a dataset for analysis:
dataImported = readtable('raw_data.csv');
% Conduct analysis...
writetable(dataImported, 'processed_data.csv');
This integration shows the ease of handling data within MATLAB, promoting a productive workflow.
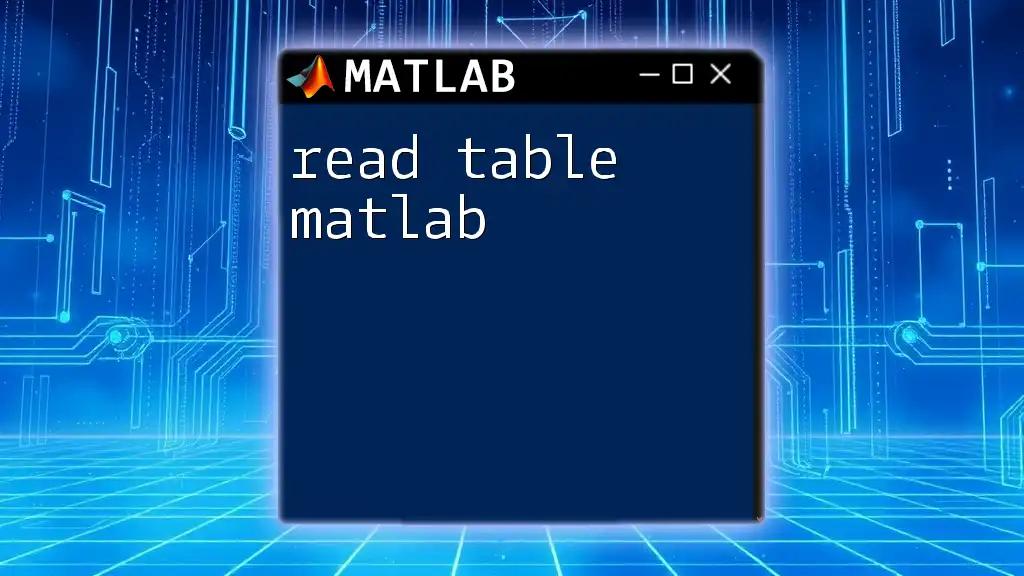
Recap of Key Points
In this guide to writetable in MATLAB, we explored the function's fundamental capabilities for exporting tables. From CSV and Excel formats to advanced options for delimiters and row names, we highlighted practical examples that can enhance data sharing and analysis efficiency.
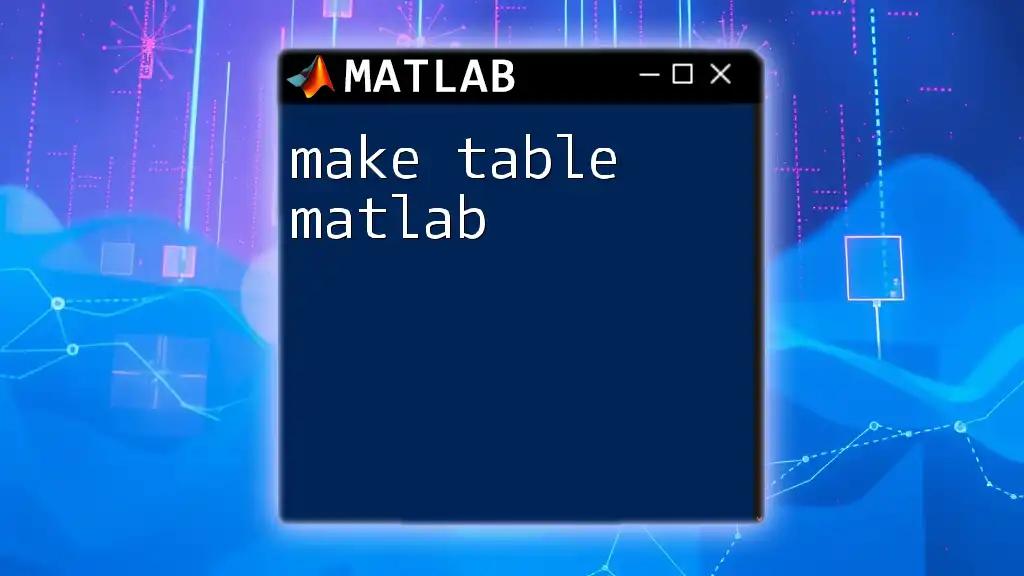
Further Learning Resources
To deepen your understanding of `writetable` and related functions in MATLAB, explore official MATLAB documentation and user forums. Engaging with tutorials or enrolling in courses can also expand your knowledge and skills in data handling.
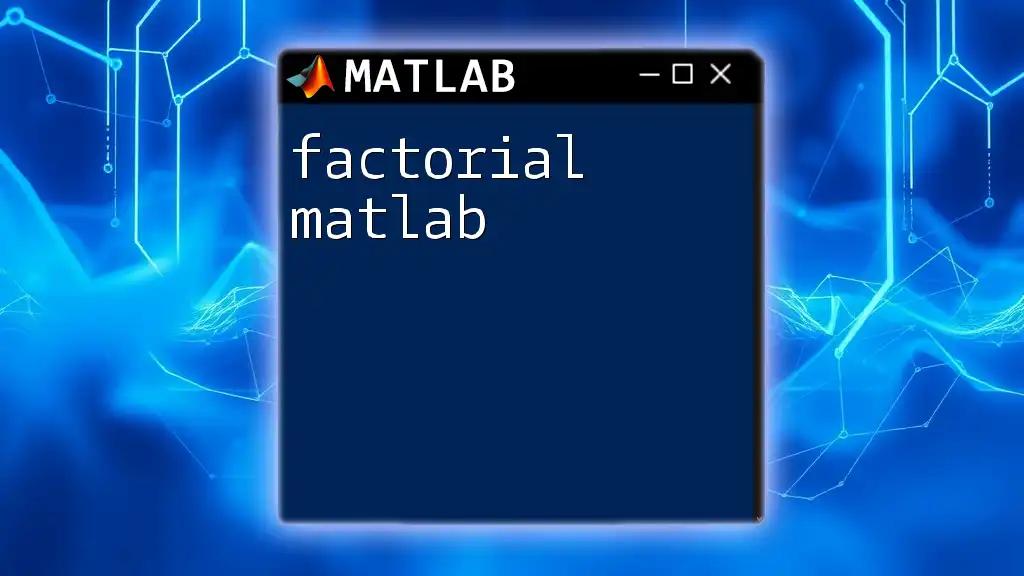
Frequently Asked Questions About writetable
What types of files can I create with writetable?
You can create CSV and Excel files, as well as text files with specified delimiters.
Can I write multiple tables to one file?
Not directly, but you can write multiple tables to different sheets in an Excel file.
How do I handle special characters in my data?
Ensure that you escape special characters or use appropriate text qualifiers to avoid issues during export.
With this comprehensive overview of `writetable`, you are now equipped to efficiently save your tables in MATLAB and share your data insights with others.