The `legend` function in MATLAB creates a legend for plotted data, effectively describing the data series in a figure.
Here’s a simple code snippet to demonstrate its usage:
x = 0:0.1:10; % Create a vector of x values
y1 = sin(x); % Compute sine of x
y2 = cos(x); % Compute cosine of x
plot(x, y1, 'r', x, y2, 'b'); % Plot sine in red and cosine in blue
legend('sin(x)', 'cos(x)'); % Add legend to the plot
What Is a Legend in MATLAB?
In the context of MATLAB plotting, a legend serves as a key that helps to identify different dataset representations in your visualizations. Legends are crucial for ensuring clarity in plots, especially when multiple datasets are displayed simultaneously. They enhance the reader's understanding by providing context to the visual elements, such as lines or markers.
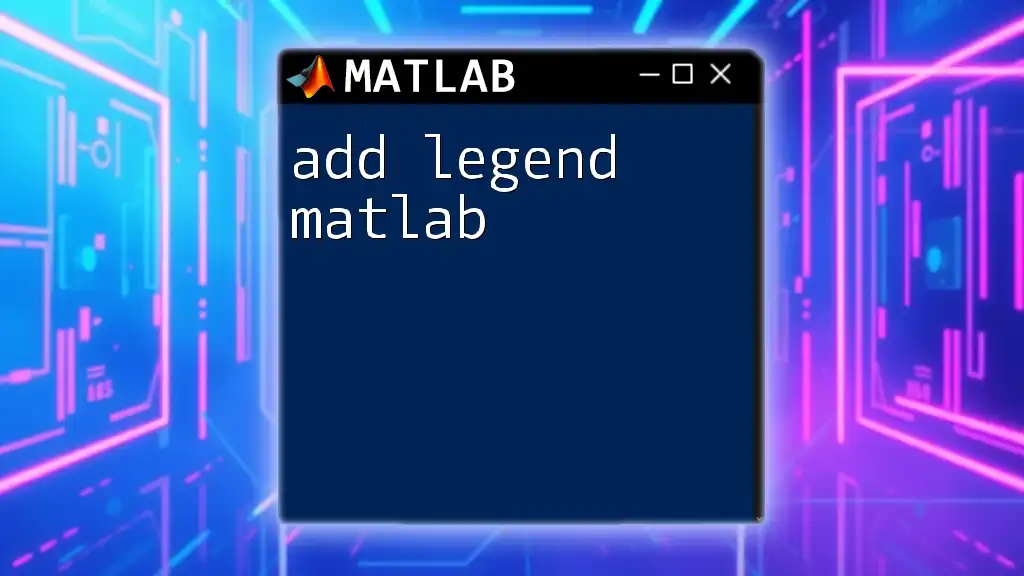
Basic Syntax for Creating a Legend
Understanding the `legend` Function
The `legend` function in MATLAB is straightforward and crucial for adding descriptive labels. The basic syntax follows this form:
Syntax:
legend(string1, string2, ..., 'Location', 'best')
This structure allows you to define labels for your plot components and specify the location of the legend within the plotting area.
Example of Basic Legend Creation
To illustrate the usage, let’s consider an example where we generate sine and cosine curves. The following code creates a simple plot while adding a legend for clarification:
x = 0:0.1:2*pi; % Define the x-values from 0 to 2π
y1 = sin(x); % Calculate sine values
y2 = cos(x); % Calculate cosine values
plot(x, y1, 'r', x, y2, 'b'); % Plot both curves in red and blue
legend('Sine Curve', 'Cosine Curve'); % Adds legend describing the curves
In this example, the legend differentiates between the sine and cosine curves using clear, descriptive labels.
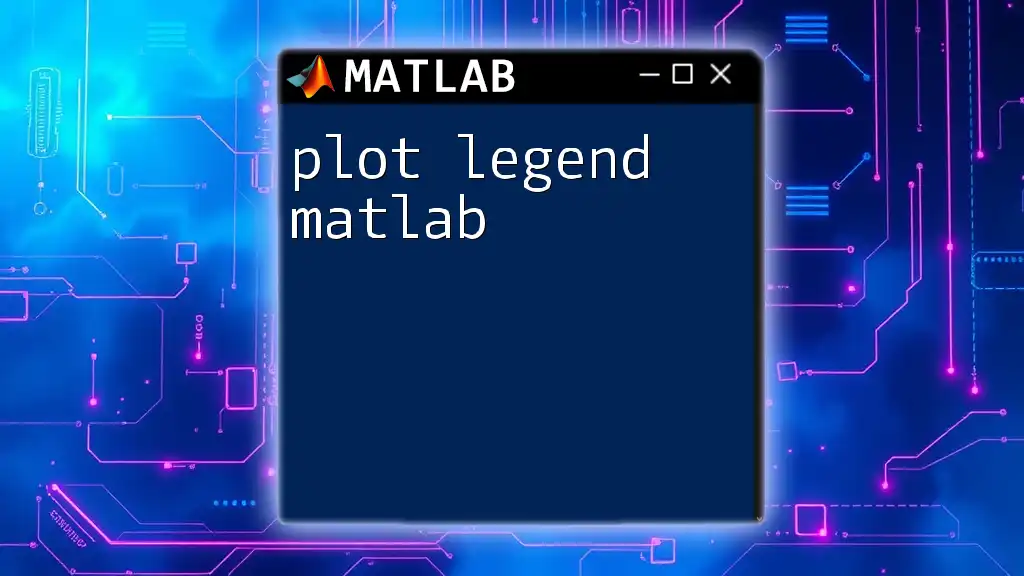
Customizing Legends
Changing Legend Position
Positioning your legend effectively can significantly impact the plot's readability. MATLAB allows various predefined positions such as 'northwest', 'southeast', and 'best'.
Example of Positioning a Legend
Here's how you can customize the legend's position:
plot(x, y1, 'r', x, y2, 'b'); % Generate the plot
legend('Sine Curve', 'Cosine Curve', 'Location', 'northeast'); % Moves the legend to the northeast
This small adjustment can make a big difference, ensuring that the legend does not obstruct the important visual elements of your plot.
Modifying Legend Appearance
Enhancing the appearance of your legend not only improves aesthetics but also facilitates better comprehension.
Font Size and Style
MATLAB allows you to modify the font size and style used in the legend. Here’s how to do that:
lgd = legend('Sine Curve', 'Cosine Curve'); % Generate legend
lgd.FontSize = 12; % Changing font size to 12
This kind of customization ensures that your legend is not only informative but also visually appealing.
Adding Background Color
Another visual enhancement is altering the legend's background color. Here's an example of how to do this:
lgd = legend('Sine Curve', 'Cosine Curve');
lgd.Color = 'yellow'; % Custom background color for the legend
Using background colors can help distinguish the legend from the plot, making it stand out without detracting from the overall visualization.
Adding Multiple Legends
In some cases, you may want to present more than one legend within a single plot. Although unconventional, it is feasible by using additional text boxes.
For instance:
figure; % Create a new figure window
plot(x, y1, 'r', x, y2, 'b'); % Plot the data
lgd1 = legend('Sine Curve', 'Cosine Curve'); % First legend
text(1, 0.5, 'This is an additional note', 'FontSize', 8, 'Color', 'black');
This technique allows you to convey additional context or notes alongside your primary legends.
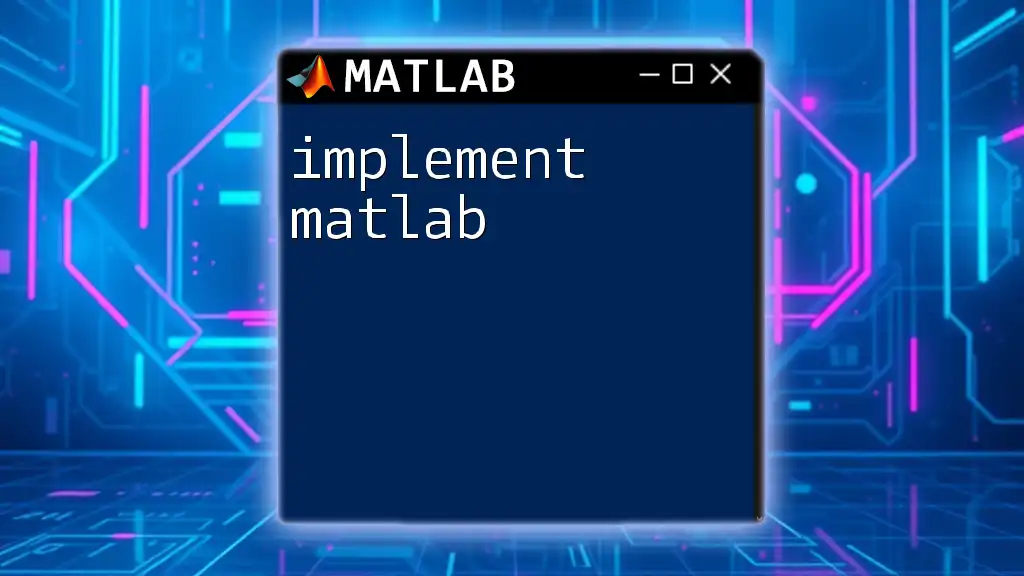
Advanced Legend Features
Legends with Line Styles and Markers
Legends can also communicate information about line styles and markers effectively. This is essential when you have varying styles in your plots.
plot(x, y1, 'r--o', x, y2, 'b:'); % Different styles for sine and cosine
legend('Sine Curve with Dashes and Circles', 'Cosine Curve with Dots'); % Descriptive legend
Clearly identifying differing visual styles helps in avoiding confusion when interpreting the data.
Interactive Legends
In more advanced applications, you may want to create interactive legends. This can lead to dynamic plots where clicking on a legend item can toggle the visibility of associated data sets.
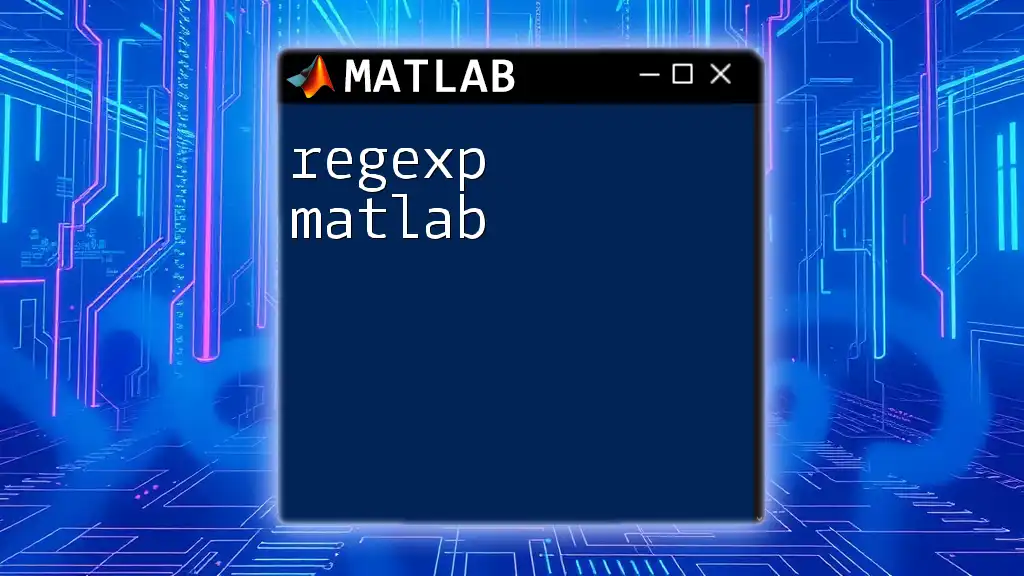
Troubleshooting Common Issues
Legend Not Appearing
Sometimes, users encounter scenarios where the legend does not appear as expected. This often results from improper command sequencing or conflicts within other plot settings. Ensuring the `legend` function is called after the `plot` commands typically resolves this issue.
Overlapping Legends
Overlapping with plot elements can be another common issue. To resolve this, check placement options and resizing attributes:
lgd = legend('Sine Curve', 'Cosine Curve', 'Location', 'bestoutside');
This adjustment moves the legend outside the plot area, ensuring it doesn’t obscure any significant data points.
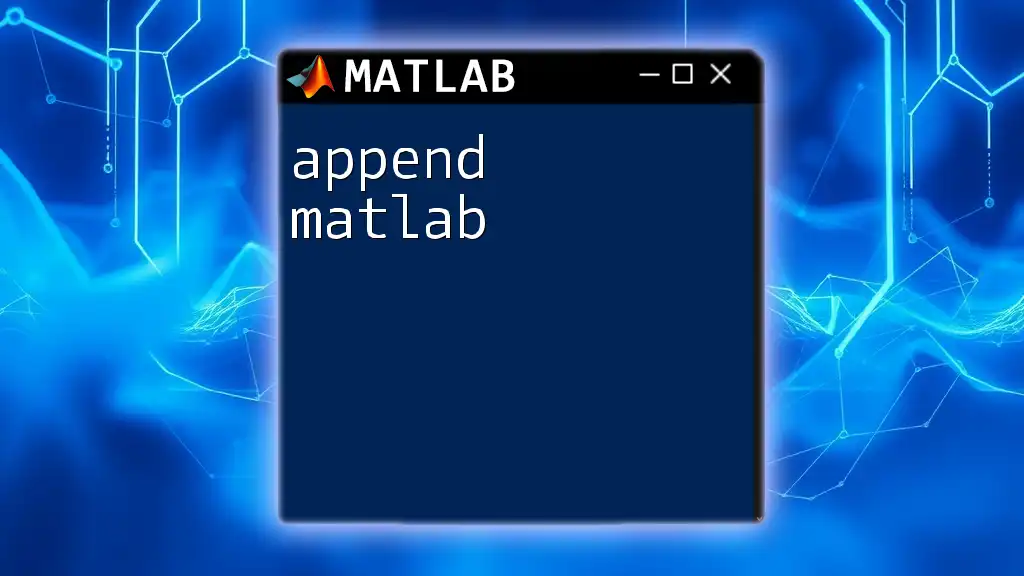
Best Practices for Using Legends
For optimal results when using legends in MATLAB, consider the following best practices:
- Be Descriptive: Choose legend labels that clearly describe the data represented.
- Position Wisely: Place legends in a way that does not interfere with critical data points.
- Limit Information: Avoid overcrowding legends with too many items; keep them concise.
- Consistency: Use consistent terminology across legends for clarity.
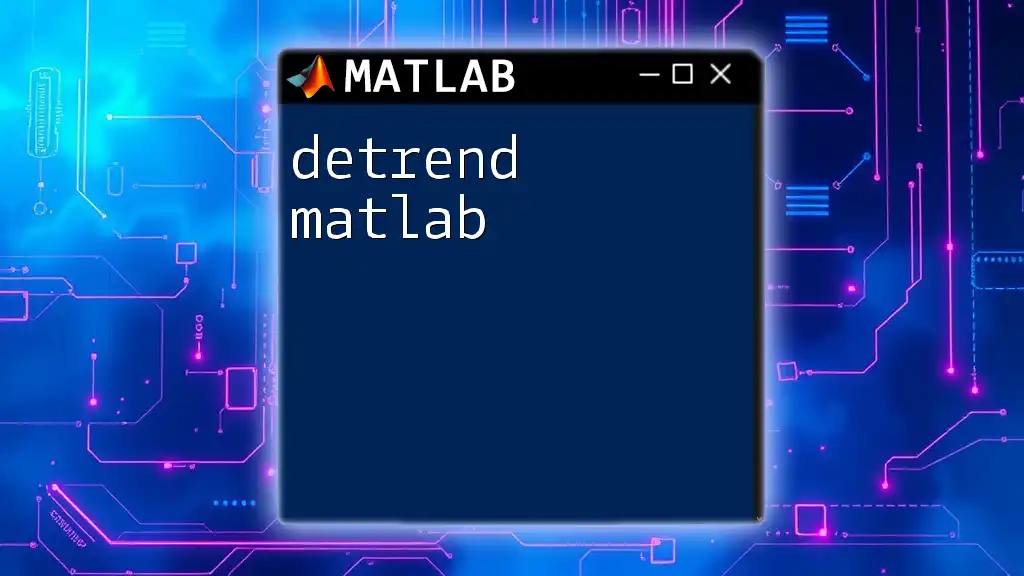
Conclusion
Legends are a pivotal feature in MATLAB plotting, enhancing readability and comprehension of your visual data presentations. With various customization options available, understanding and using legends effectively can greatly improve the clarity of your plots.
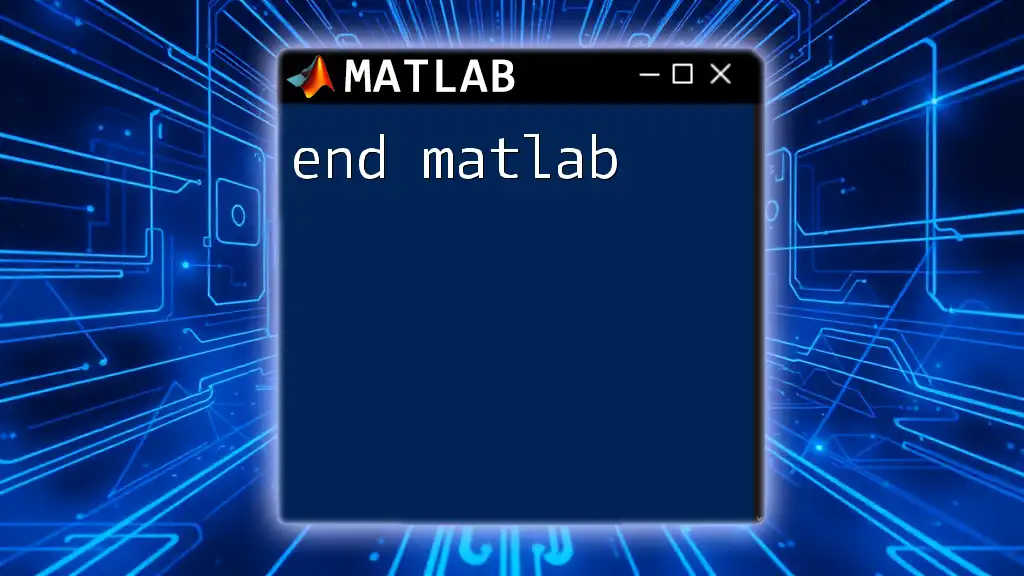
Further Reading and Resources
For more detailed information, explore the official MATLAB documentation on the `legend` function. Additional tutorials can provide further insights into advanced legend usage and tips for mastering MATLAB plotting techniques.
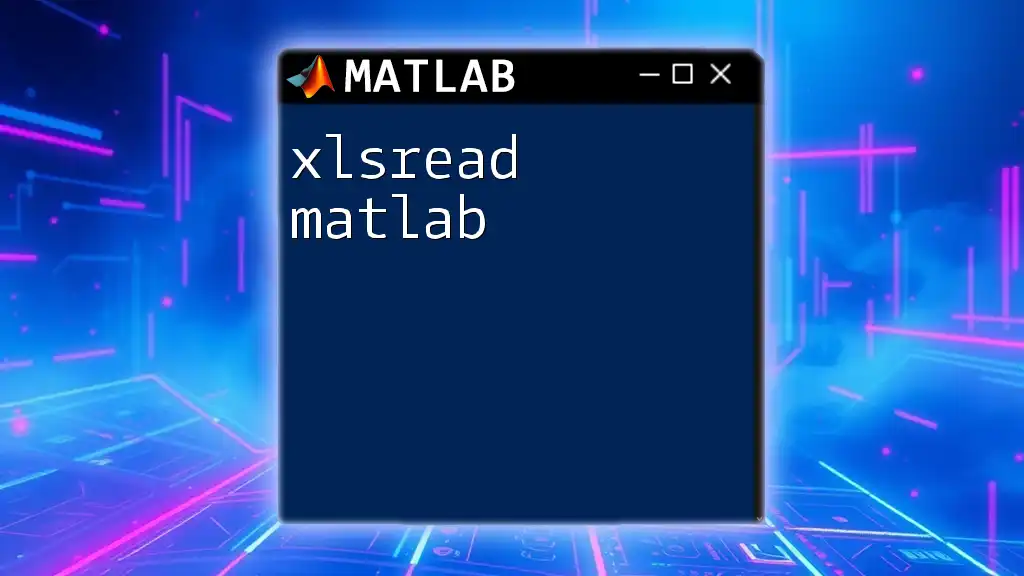
FAQ Section
When integrating legends in your plots, questions often arise regarding functionality and best practices. Familiarizing yourself with common inquiries will further enhance your command over legend usage in MATLAB.