The pseudoinverse in MATLAB is computed using the `pinv` function, which provides a least-squares solution to linear systems that may not have a unique solution or may be underdetermined.
Here's an example snippet to compute the pseudoinverse of a matrix `A`:
A = [1, 2; 3, 4; 5, 6];
A_pinv = pinv(A);
What is Pseudoinverse?
The pseudoinverse is a fundamental concept in linear algebra, represented as the matrix that serves as a generalization of the inverse for non-square or rank-deficient matrices. Unlike a traditional inverse that exists only for square matrices with full rank, the pseudoinverse accommodates a broader range of scenarios, making it an essential tool for solving equations in various fields such as data science, signal processing, and control systems.
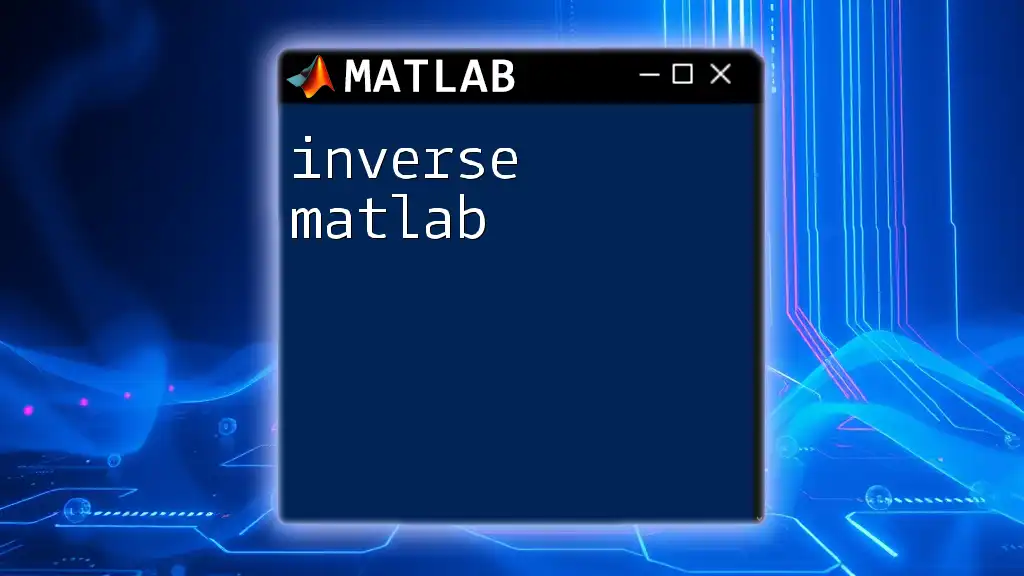
Applications of Pseudoinverse
Overview of Real-World Applications
The pseudoinverse has diverse applications including:
- Signal Processing: Used for filtering and reconstructing signals, particularly in systems with noise.
- Data Science and Machine Learning: Utilized to perform linear regression, especially when the number of observations does not match the number of features.
- Control Systems: Acts in designing control algorithms, where controlling feedback systems can lead to underdetermined states.
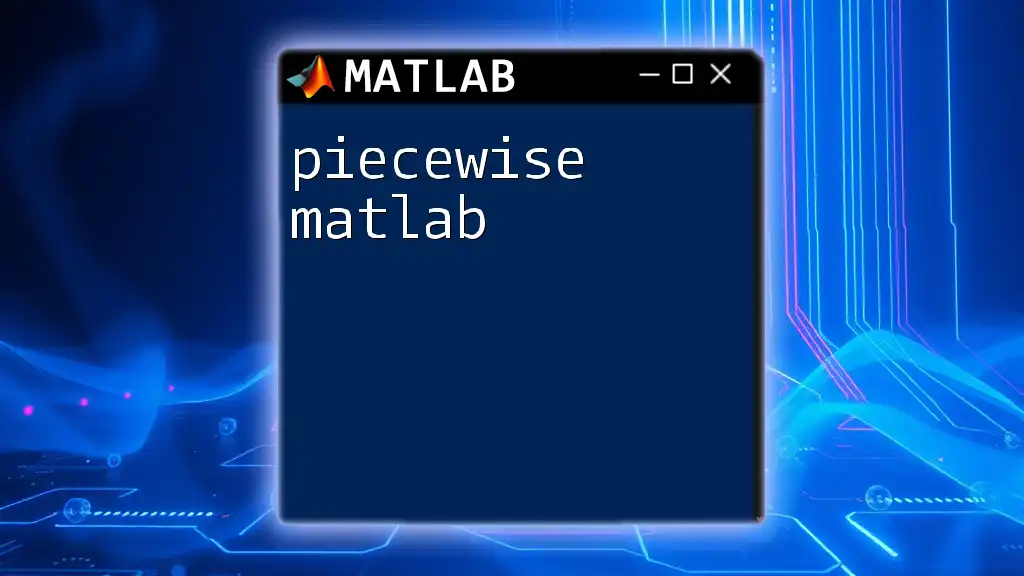
Understanding Pseudoinverse in MATLAB
Basic Terminology
To effectively use the pseudoinverse, it is important to grasp some basic terms:
- Matrix Dimensions: Refers to the number of rows and columns in a matrix.
- Rank: Indicates the maximum number of linearly independent row or column vectors in a matrix.
- Full Rank: A matrix is said to be full rank when its rank is equal to the smaller of the number of rows or columns.
- Rank-Deficient Matrices: These matrices have less than full rank, which can complicate the traditional inverse.
Why Use Pseudoinverse in MATLAB?
Employing the pseudoinverse in MATLAB provides several benefits, notably:
- Solving Linear Systems: The pseudoinverse facilitates solutions for both overdetermined (more equations than unknowns) and underdetermined systems (more unknowns than equations).
- Handling Non-Square Matrices: It allows the MATLAB user to derive solutions even when matrices are not square, extending the applicability of linear algebra.
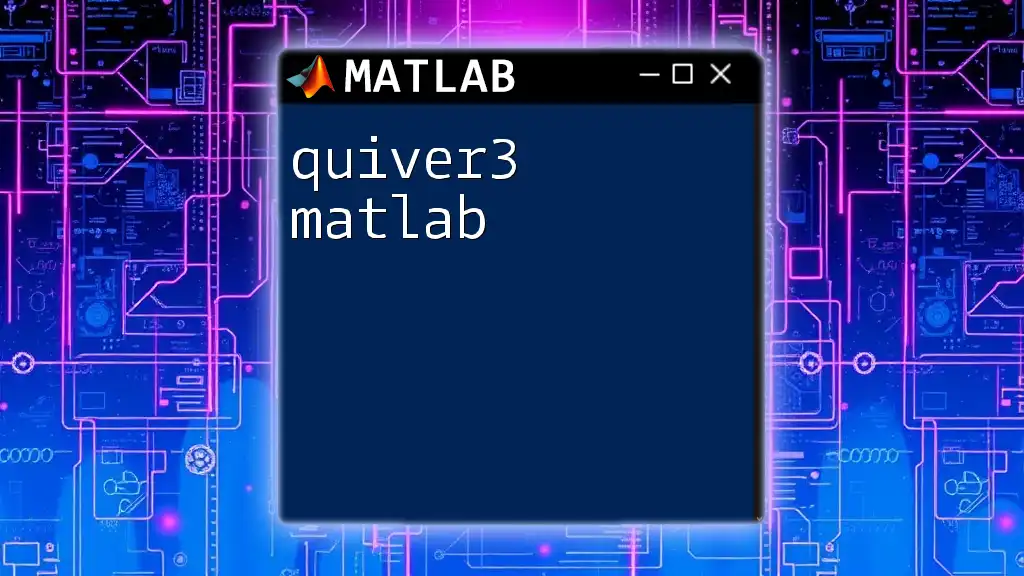
MATLAB Functions for Pseudoinverse
The `pinv` Function
In MATLAB, the `pinv` function is the primary means of calculating the pseudoinverse of a matrix. The syntax is as follows:
B = pinv(A)
Where `A` is the input matrix, and `B` is the resulting pseudoinverse.
Example of Using `pinv`
To better understand how to use `pinv`, consider the following example, where we compute the pseudoinverse of a given matrix:
A = [1, 2; 3, 4; 5, 6];
B = pinv(A);
disp(B);
In this case, `A` is a rectangular matrix with 3 rows and 2 columns, and the output `B` will show the pseudoinverse, which can be used in further calculations.
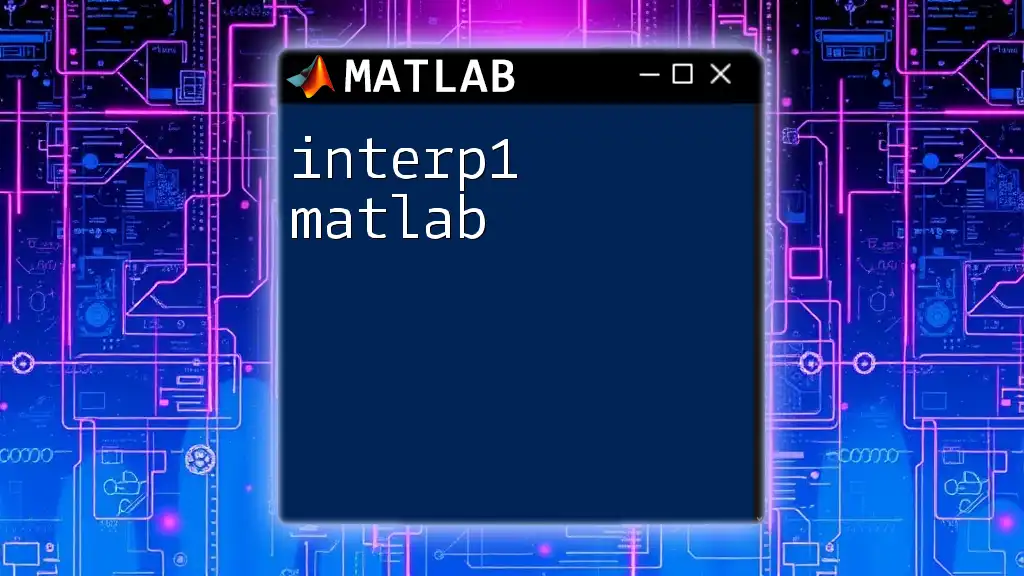
Working with Pseudoinverse: Steps and Examples
Solving Linear Equations
Example: Overdetermined System
In cases where there are more equations than unknowns, the pseudoinverse is particularly useful. Here’s a sample MATLAB code:
A = [1, 1; 2, 2; 3, 4];
b = [1; 2; 3];
x = pinv(A) * b;
disp(x);
In this scenario, `A` is overdetermined. By calculating `x`, you find the least-squares solution that minimizes the squared differences between the observed values and those predicted by the linear model represented by `A`.
Example: Underdetermined System
When the system is underdetermined (more unknowns than equations), the pseudoinverse still provides valuable insights:
A = [1, 0; 0, 1; 1, 1];
b = [1; 2; 3];
x = pinv(A) * b;
disp(x);
This code snippet computes a solution for the underdetermined system represented by `A`, where multiple solutions might exist, and it returns a particular solution.
Pseudoinverse for Non-square Matrices
One of the powers of the pseudoinverse lies in its ability to address non-square matrices. In real-life data analysis, matrices are often not square, and the ability to compute a pseudoinverse allows for meaningful insights, particularly when validating models against data.
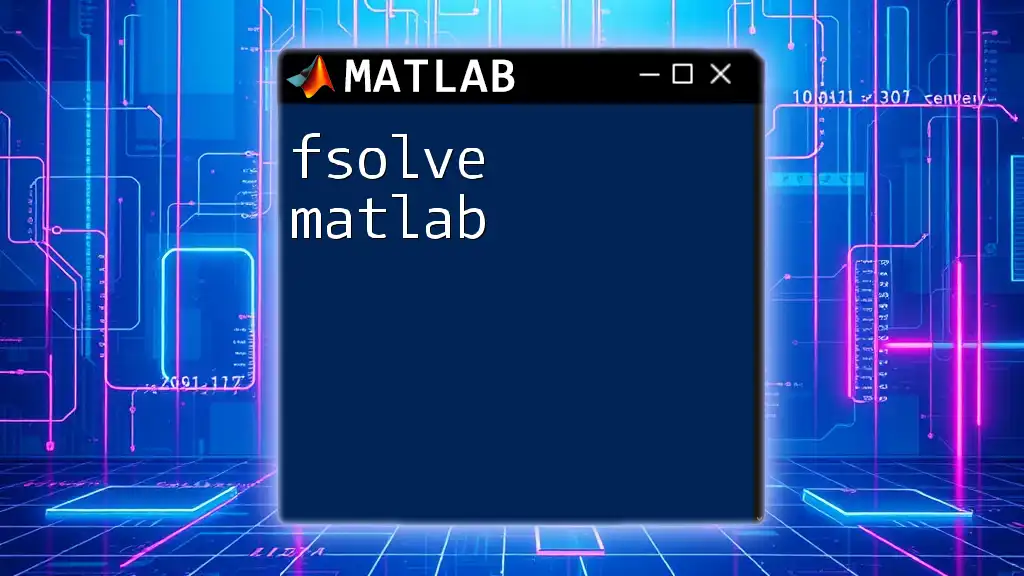
Advanced Uses of Pseudoinverse
Regularization Techniques
Ridge Regression
Regularization techniques such as Ridge Regression leverage the pseudoinverse to mitigate issues of multicollinearity and improve model robustness. The ridge coefficients can be computed with the following MATLAB code:
lambda = 1;
B = pinv(A'*A + lambda*eye(size(A,2)))*A';
Here, `lambda` represents a regularization parameter that controls the extent of the penalty applied to the coefficients. This can yield more stable and interpretable results.
Handling Data with Noise
In practice, data is often plagued with noise, which can distort results significantly. By employing the pseudoinverse, one can robustly fit models that minimize the effects of noise. Testing and comparing results can illustrate the effectiveness of this method, underscoring its value in analytical and predictive contexts.
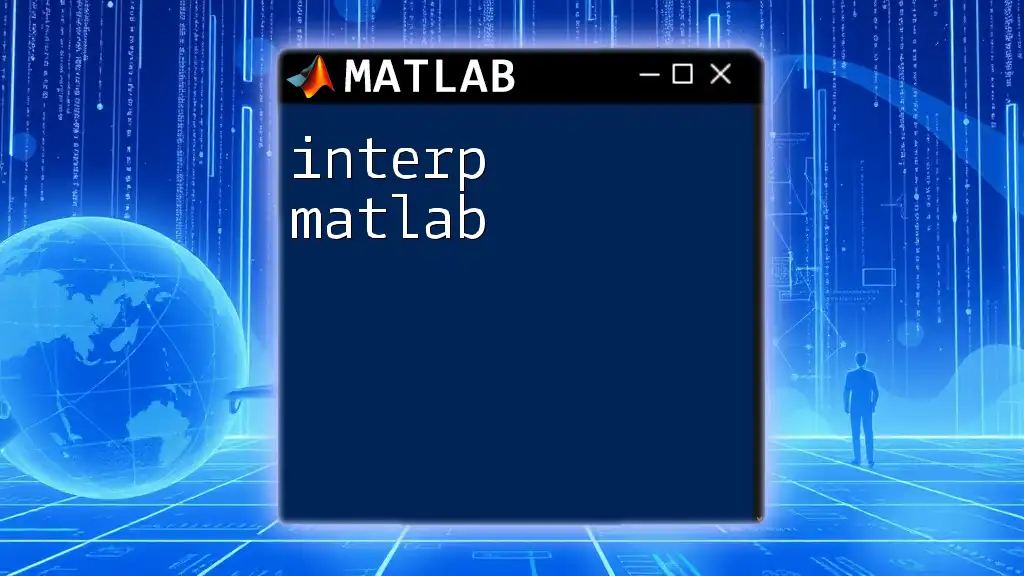
Common Pitfalls and How to Avoid Them
Misinterpretation of Results
An essential step in working with the pseudoinverse is understanding the context of your results. The output may not always correspond directly to the input conditions, particularly with non-square matrices. Therefore, it is vital to analyze residuals and error terms to ensure a proper understanding.
Performance Considerations
Computationally, deriving the pseudoinverse for large matrices can be resource-intensive. It's prudent to consider dimensionality reduction techniques or alternative algorithms to maintain efficiency, particularly in large data settings.
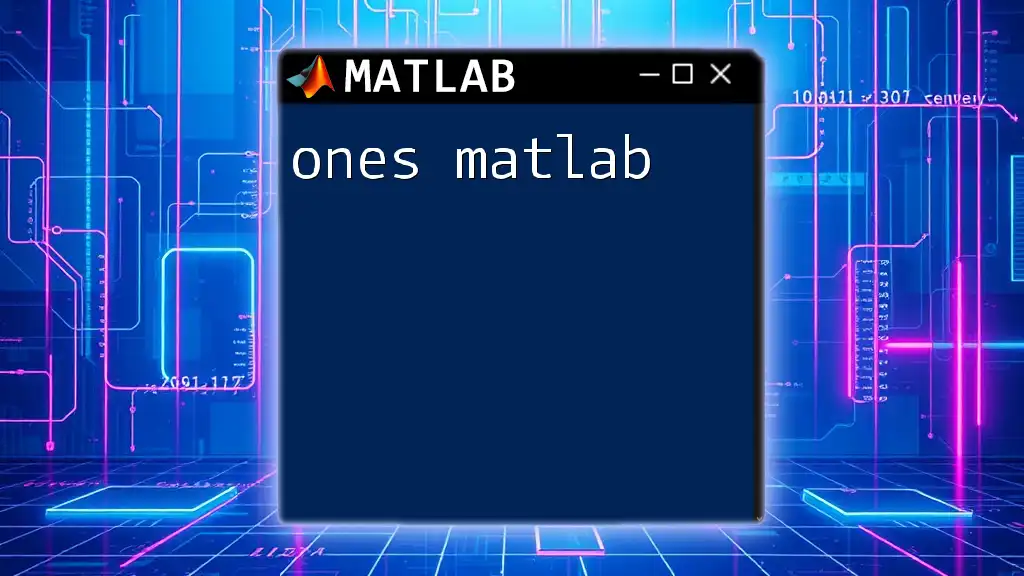
Conclusion
The pseudoinverse is a powerful tool in MATLAB that empowers users to deal with linear algebra challenges effectively. By understanding its applications, utilizing the `pinv` function, and navigating common pitfalls, anyone can elevate their analytical capabilities. The pseudoinverse's ability to address both overdetermined and underdetermined systems, along with its advanced applications in regularization techniques, makes it an invaluable asset across various domains. Users are encouraged to explore related topics and continue practicing with different data scenarios to enhance their MATLAB skills.