In MATLAB, the absolute value of a number is computed using the `abs` function, which returns the non-negative value of a given input.
result = abs(-5); % result will be 5
Understanding Absolute Value in MATLAB
Absolute value is a fundamental concept in mathematics, representing the distance of a number from zero on the number line, regardless of its sign. In MATLAB, understanding how to work with absolute values is essential for effective numerical computations.
MATLAB Basics
MATLAB is a powerful programming environment widely used for mathematical computations, data analysis, and visualization. Its strength lies in its ability to handle various numerical data types efficiently. Whether you're working with scalars, vectors, or matrices, knowing how to compute absolute values becomes a valuable skill.
MATLAB Data Types
In MATLAB, you primarily work with three data types relevant to absolute value calculations:
- Scalars: Single numerical values.
- Vectors: One-dimensional arrays that can contain multiple values.
- Matrices: Two-dimensional arrays that consist of rows and columns.
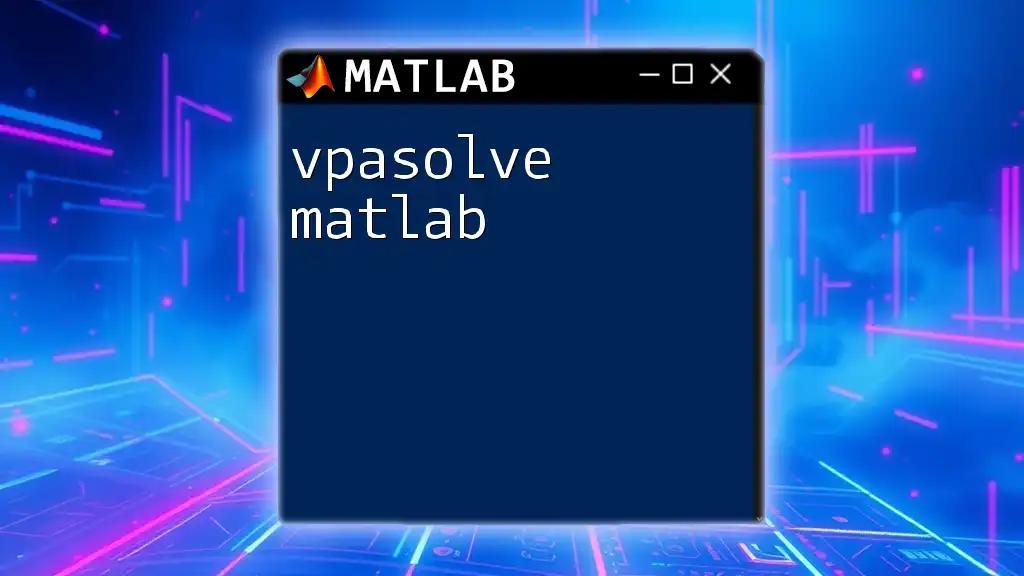
The Absolute Value Function in MATLAB
Using the `abs` Function
MATLAB provides a built-in function called `abs` specifically designed to compute absolute values. Its syntax is straightforward:
abs(x)
Here, `x` can be a scalar, vector, or matrix. The function returns the absolute value of each element in `x`.
Examples of the `abs` Function
Example 1: Absolute Value of a Scalar
Calculating the absolute value of a scalar is as simple as this:
scalar_value = -5;
absolute_value = abs(scalar_value);
disp(absolute_value); % Output: 5
In this example, the negative scalar `-5` is converted into its positive counterpart `5`.
Example 2: Absolute Value of a Vector
You can also apply the `abs` function to a vector:
vector_value = [-1, -2, 3, -4];
absolute_vector = abs(vector_value);
disp(absolute_vector); % Output: [1, 2, 3, 4]
Here, the function processes each element in the vector, resulting in a new vector of positive values.
Example 3: Absolute Value of a Matrix
The `abs` function works seamlessly with matrices:
matrix_value = [-1, 2; -3, 4];
absolute_matrix = abs(matrix_value);
disp(absolute_matrix); % Output: [1, 2; 3, 4]
Each element in the matrix is evaluated, returning a matrix of the absolute values.
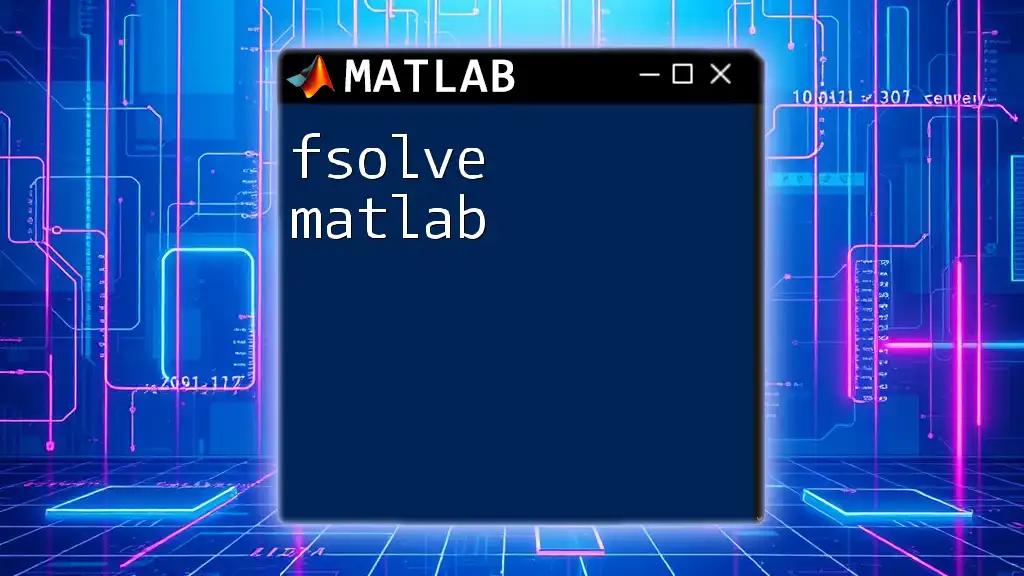
Exploring Advanced Uses of Absolute Value
Working with Complex Numbers
In MATLAB, complex numbers are numbers that have both a real part and an imaginary part. The `abs` function also computes the magnitude of complex numbers, which is defined as:
\[ |z| = \sqrt{a^2 + b^2} \]
where \( z = a + bi \).
Example: Absolute Value of Complex Numbers
For example, using the `abs` function with a complex number:
complex_value = 3 + 4i;
absolute_complex = abs(complex_value);
disp(absolute_complex); % Output: 5
In this case, the magnitude of the complex number is calculated, yielding an absolute value of `5`.
Applying Absolute Value in Mathematical Functions
Absolute values are often critical in optimization problems, especially when measuring distances or deviations.
Example: Absolute Value in Optimization
The following example demonstrates how absolute values can be incorporated into optimization functions:
f = @(x) abs(x - 5); % Define function
x = linspace(-5, 10, 100);
plot(x, f(x)); % Plotting the function
title('Graph of abs(x-5)');
This function measures how far any `x` is from the point `5`, visually represented in the graph.

Performance Considerations
Computational Efficiency
While MATLAB's `abs` function is optimized for performance, it's essential to consider the size of the datasets you're working with. For very large datasets, inefficient use of the function can lead to performance bottlenecks. Profiling your code with MATLAB's built-in performance tools can help identify areas for improvement.
Best Practices
To use the absolute value function effectively, it’s advisable to keep computations simple and avoid unnecessary complexity. Working with vectorized commands, for instance, can enhance performance.
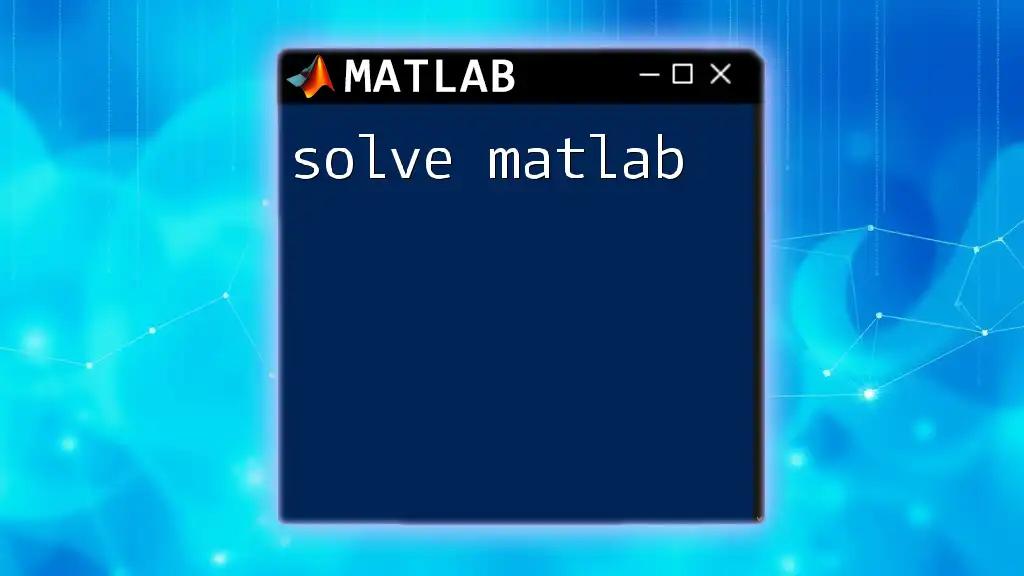
Common Mistakes to Avoid
Misunderstanding the Functionality
A common misconception about the `abs` function is that it only applies to real numbers. In reality, it can seamlessly compute absolute values for complex numbers as well.
Performance Pitfalls
An example of inefficient code is looping through elements to compute absolute values individually, which can significantly slow down your program. Instead, leverage MATLAB's vectorized operations to compute absolute values across entire arrays at once.
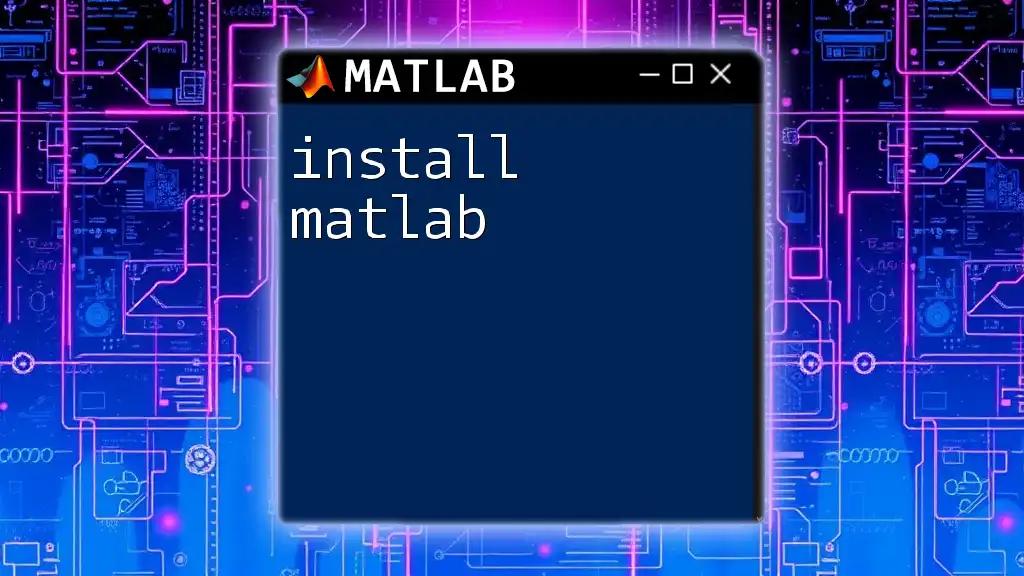
Conclusion
In summary, understanding absolute value in MATLAB is crucial for various mathematical computations. The `abs` function enables quick calculations for scalars, vectors, and matrices – even complex numbers. By focusing on best practices and computational efficiency, you can enhance your MATLAB programming skills and apply them effectively in your projects.
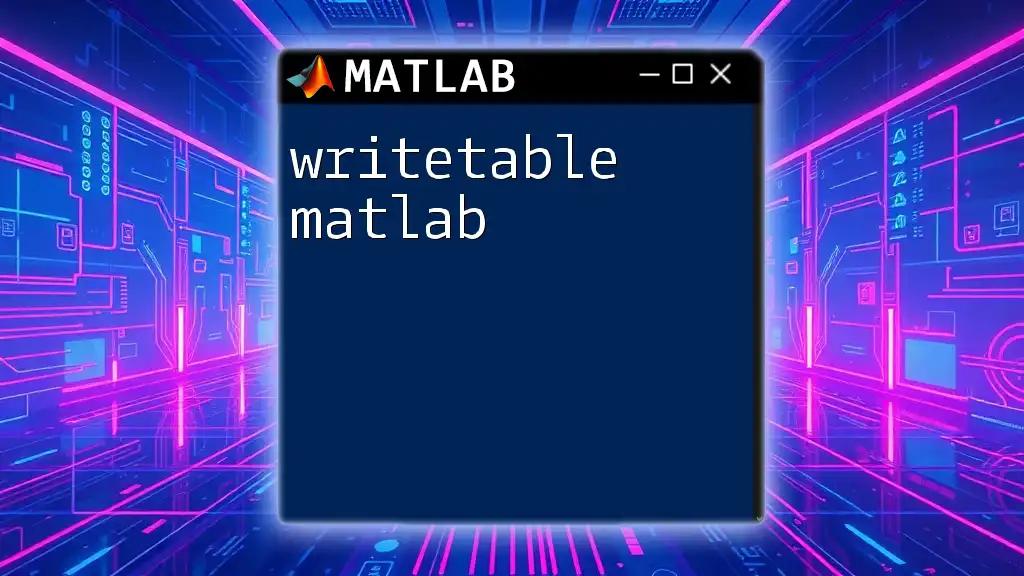
Additional Resources
For more in-depth learning, refer to the official MATLAB documentation on the `abs` function. Exploring related concepts—such as plotting functions and matrix manipulations—can further solidify your understanding and application of absolute values in MATLAB.