The `fitlm` function in MATLAB is used to create a linear regression model from a dataset, allowing users to fit a linear relationship between the dependent and independent variables.
Here’s a code snippet to illustrate its usage:
% Example of using fitlm to create a linear regression model
data = readtable('data.csv'); % Load data from a CSV file
mdl = fitlm(data, 'Response ~ Predictor1 + Predictor2'); % Fit linear model
Understanding Linear Regression
What is Linear Regression?
Linear regression is a statistical method for modeling the relationship between a dependent variable (often called the response variable) and one or more independent variables (predictors). The goal is to find the linear equation that best describes this relationship, allowing for predictions to be made based on new data.
There are two main types of linear regression:
- Simple Linear Regression: Involves a single predictor variable. For example, predicting house prices based on square footage.
- Multiple Linear Regression: Involves two or more predictor variables. For instance, predicting house prices based on both square footage and the number of bedrooms.
Importance of Linear Regression in Data Analysis
Linear regression is foundational in many fields, including:
- Economics: Forecasting economic indicators.
- Biology: Analyzing biological data trends.
- Engineering: Quality control and predictive maintenance.
Understanding key statistical concepts is crucial:
- Predictors are the independent variables.
- Response variables are the dependent quantities that we aim to predict.
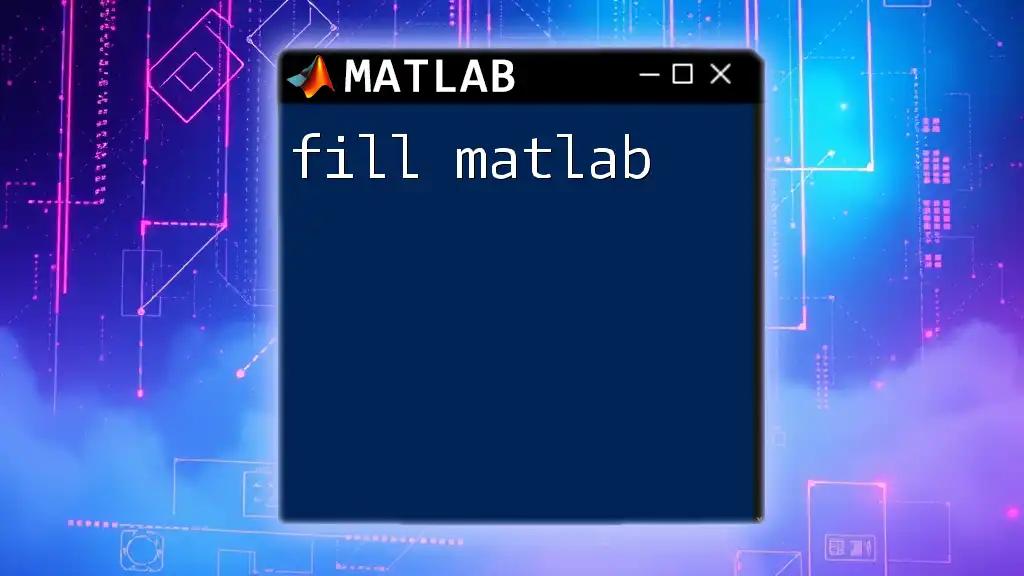
Overview of fitlm Function
Syntax and Basic Usage
The `fitlm` function in MATLAB stands out for its simplicity and effectiveness in fitting linear regression models. The basic syntax is:
mdl = fitlm(X, y)
Here, `X` indicates the matrix containing predictor variables, and `y` is the vector representing the response variable. This command returns a fitted linear model object `mdl` that contains important information about the model.
Inputs and Parameters
When using `fitlm`, the input parameters include:
- Predictor matrix `X`: An n-by-p matrix, where n is the number of observations and p is the number of predictors.
- Response variable `y`: An n-by-1 vector representing the dependent variable.
Other optional parameters include:
- `'VarNames'`, which allows specifying custom variable names, enhancing clarity in output.
- `'Intercept'`, which can be set to include or exclude the intercept.
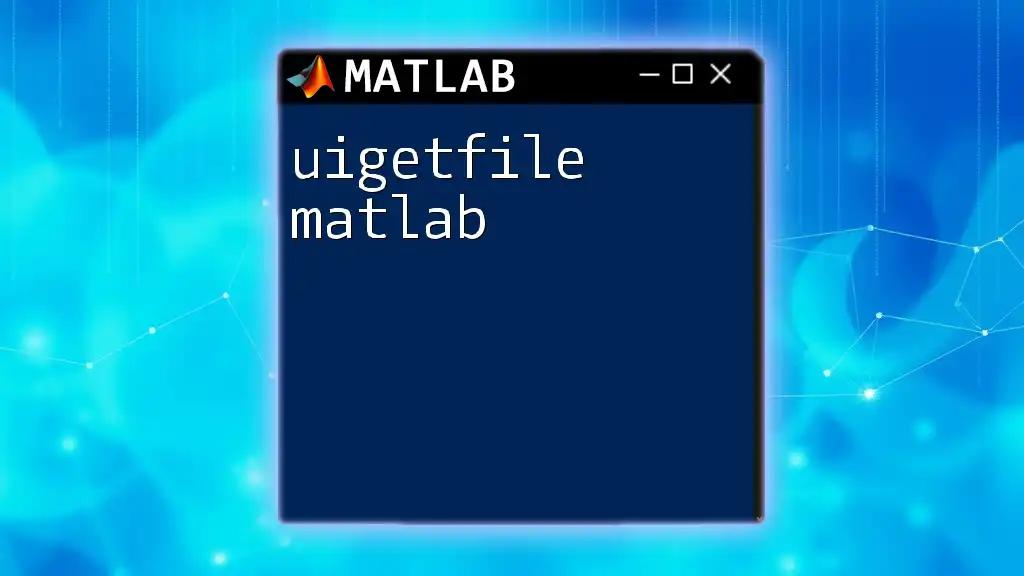
Creating a Linear Model with fitlm
Step-by-Step Example
To better comprehend how to implement `fitlm`, let’s consider a sample dataset. Assume you have the following data:
X = [1 2; 2 3; 3 5; 4 4; 5 6];
y = [1; 2; 3; 4; 5];
This dataset contains two predictors and a single response variable. The first step is to create the linear model using `fitlm`:
mdl = fitlm(X, y);
Fitting a Model
This command fits a linear regression model to the data contained in `X` and `y`. Once the model is fitted, it's essential to interpret the output. The output `mdl` will provide various statistics, including:
- Coefficients of the predictors, informing how much each predictor contributes to the response variable.
- R-squared value, which indicates the proportion of variance explained by the model.

Evaluating the Fit
Summary of Fit Output
When you fit a model using `fitlm`, the summary output will include:
- Coefficients: These values indicate how changes in predictor variables affect the response variable.
- R-squared value: This statistic evaluates the goodness of fit; a higher R-squared value (closer to 1) suggests a well-fitted model.
Understanding these metrics is key to assessing the effectiveness of your model.
Diagnostic Plots
Visualization of Regression
To check how well your model fits the data, generating diagnostic plots is vital. You can visualize residuals and fitted values using:
plot(mdl);
This command provides plots that help in evaluating assumptions of linearity, homoscedasticity, and normality of residuals.
Predictive Accuracy
Assess predictive accuracy by comparing predicted values against actual outcomes. You may want to calculate the Mean Absolute Error (MAE) or Root Mean Square Error (RMSE) for a more robust evaluation.
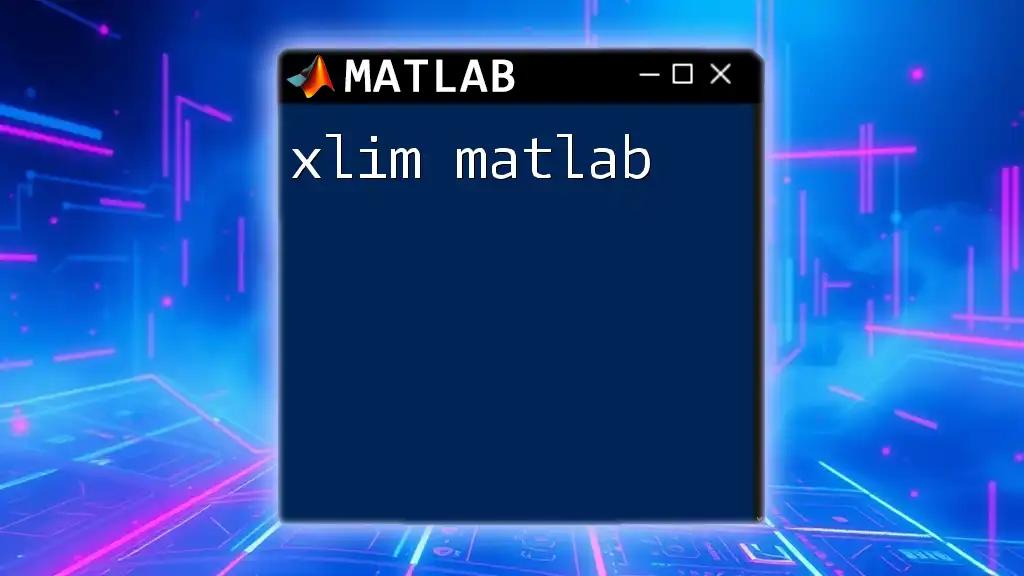
Advanced Features of fitlm
Adding Interaction Terms
In cases where you suspect interaction effects between predictors, `fitlm` allows you to incorporate these directly. Interaction terms can enhance model accuracy. You can include interaction in your model like so:
mdl = fitlm(X, y, 'linear', 'RobustOpts', 'on');
This command extends your regression model to account for interactions.
Categorical Variables
`fitlm` excels in handling categorical data by automatically converting them to dummy variables. For example:
X = table([1; 2; 1; 2], {'A'; 'B'; 'A'; 'B'}, 'VariableNames', {'NumVar', 'CatVar'});
This illustrates how `fitlm` can be used to fit a regression model that includes categorical predictors.
Handling Missing Data
Missing data can skew regression results. `fitlm` provides options to manage missing data effectively. Utilize the following approach to exclude missing rows:
mdl = fitlm(X, y, 'Exclude', 'rows');
This ensures your analysis remains robust and reliable despite missing values.
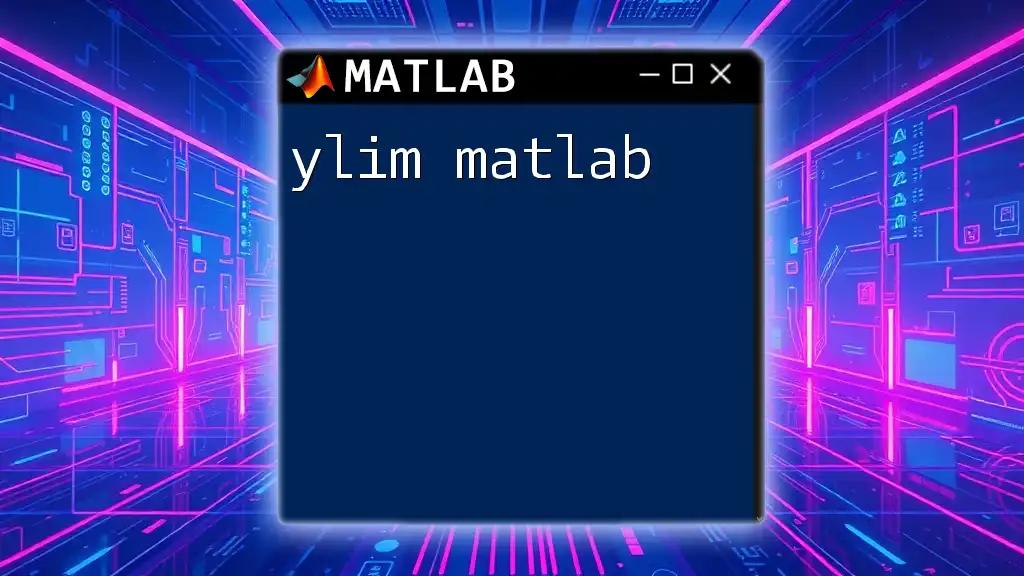
Practical Applications of fitlm
Case Studies
`fitlm` has been applied in various real-world scenarios, such as:
- Healthcare: Modeling patient survival rates based on treatment and demographic factors.
- Finance: Predicting stock prices with variables like market indices, interest rates, and historical prices.
Lessons Learned
Using `fitlm` can lead to numerous insights, such as understanding which predictors significantly impact the response variable, testing hypotheses, and making informed predictions.

Conclusion
This guide to `fitlm` in MATLAB has outlined how to effectively use this essential function for linear regression analysis. By mastering `fitlm`, you can harness the power of statistical modeling to extract meaningful insights from your data.
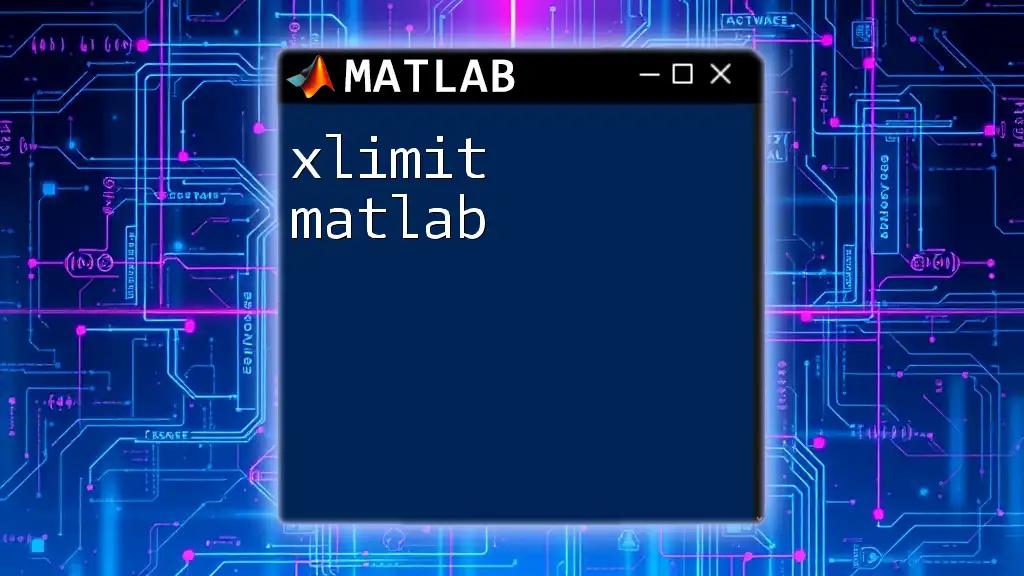
Additional Resources
Further Reading
To deepen your understanding of regression analysis, consider reading academic literature that explores both theoretical and practical aspects of the subject.
MATLAB Documentation Links
For more intricate details and advanced functionalities, refer to the official MATLAB documentation on `fitlm`.

Call to Action
We invite you to practice these concepts and share your experiences with `fitlm` in the comments. Also, consider subscribing for more tips and tutorials to enhance your MATLAB skills!