A linear fit in MATLAB allows you to find the best-fitting straight line for a set of data points using the `polyfit` function to determine the slope and intercept of the line.
Here’s a simple code snippet to demonstrate linear fitting in MATLAB:
% Sample data
x = [1, 2, 3, 4, 5]; % Independent variable
y = [2.2, 2.8, 3.6, 4.5, 5.1]; % Dependent variable
% Perform linear fit
p = polyfit(x, y, 1); % p(1) is the slope, p(2) is the intercept
% Generate fitted line values
y_fit = polyval(p, x);
% Plotting the results
figure;
scatter(x, y, 'filled'); % Original data points
hold on;
plot(x, y_fit, 'r-'); % Fitted line
title('Linear Fit Example');
xlabel('X Values');
ylabel('Y Values');
legend('Data Points', 'Fitted Line');
hold off;
Understanding Linear Fit
What is Linear Fit?
Linear fit, also known as linear regression, is a fundamental statistical method used to model the relationship between two variables by fitting a linear equation to the observed data. The linear model is represented by the equation:
\[ y = mx + b \]
Where:
- y is the dependent variable,
- x is the independent variable,
- m is the slope of the line, and
- b is the y-intercept.
This technique is essential for estimating predictions, identifying trends, and understanding the relationships between variables in various fields, including engineering, finance, and environmental science.
Types of Linear Fit
Simple Linear Fit
Simple linear fit deals with one independent variable and one dependent variable. It seeks to establish a linear relationship between these two variables, providing insights into their correlation.
Multiple Linear Fit
Multiple linear fit extends the simple case to include multiple independent variables. This approach is useful in situations where you need to account for more than one predictor variable to better explain the variation in the dependent variable.
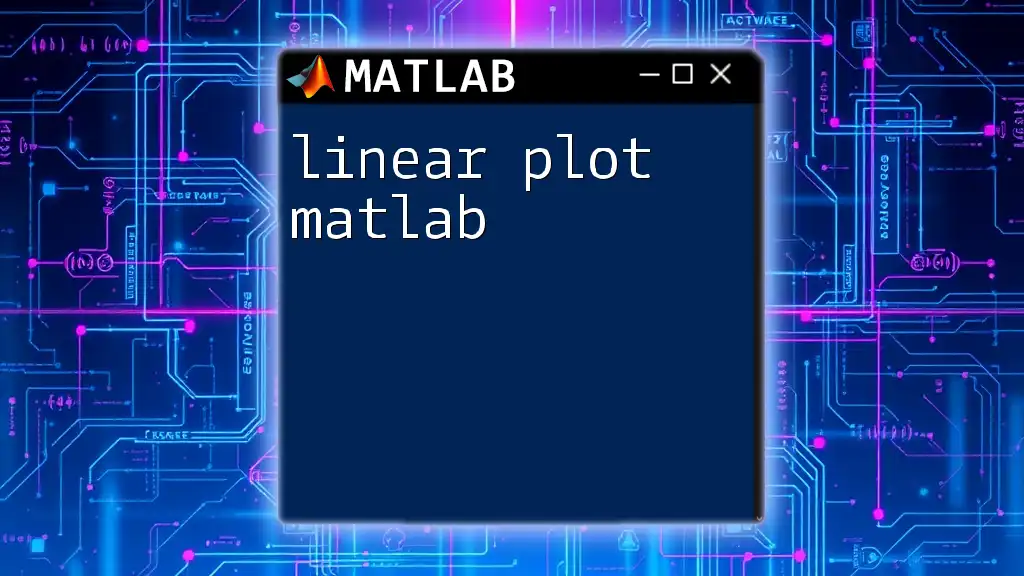
The MATLAB Environment for Linear Fit
Why Use MATLAB for Linear Fitting?
MATLAB is a powerful platform for statistical analysis, providing robust tools and functions for performing linear fitting. It offers rich visualization capabilities, allowing for dynamic representation and interpretation of data. The simplicity and efficiency of MATLAB make it an attractive option for both beginners and experienced analysts.
Key MATLAB Tools for Linear Fit
MATLAB Functions for Linear Fit
Several key functions in MATLAB facilitate linear fitting, including:
- `polyfit`: This function fits a polynomial of specified degree to a set of data points. For linear fitting, you will use a polynomial of degree one.
- `fit`: This flexible function can be used for various types of fitting, including linear and nonlinear scenarios.
- `regress`: This function provides a means of multiple linear regression, returning regression coefficients and additional statistics.
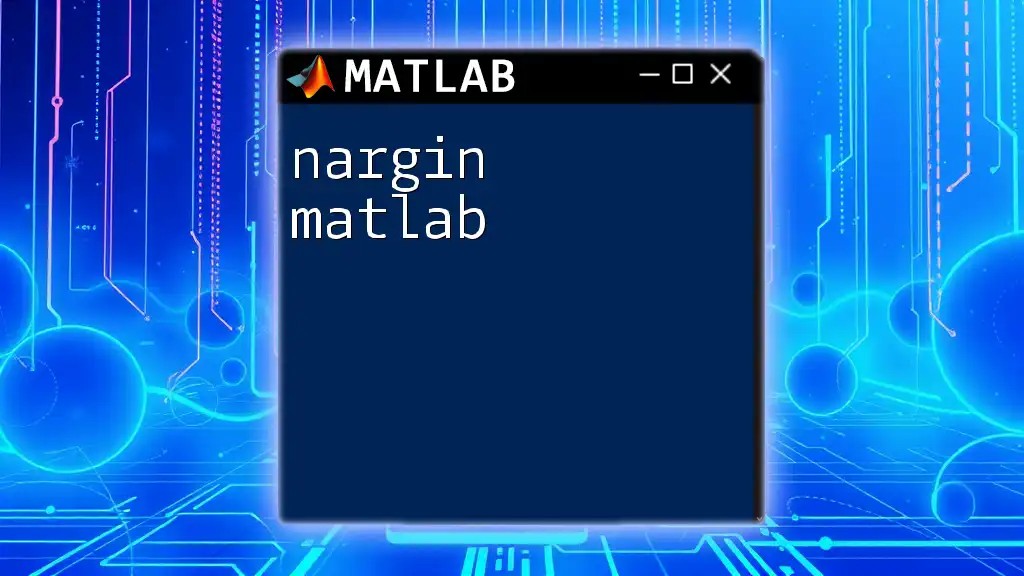
Performing a Simple Linear Fit in MATLAB
Step-by-Step Tutorial
Loading and Preparing Data
Before conducting any analysis, you first need to load your data into MATLAB. Here's an example of how you might import your data from a file:
data = readtable('your_data_file.csv'); % Loading data from a CSV file
x = data.x_column; % Assuming 'x_column' is your independent variable
y = data.y_column; % Assuming 'y_column' is your dependent variable
Data preparation includes ensuring your data is clean and in a suitable format for analysis. Remove any NaN entries or outliers that could skew your fit.
Using `polyfit` for Simple Linear Fit
The `polyfit` function is straightforward to use for simple linear fitting. It requires the vectors of your independent and dependent variables, along with the degree of the polynomial. For linear fitting, use degree 1:
p = polyfit(x, y, 1); % p returns the coefficients for the linear fit
In this case, `p(1)` is the slope (m), and `p(2)` is the intercept (b) of the fitted line.
Evaluating the Fit with `polyval`
Once you have the coefficients from `polyfit`, you can evaluate the fitted line using the `polyval` function:
y_fitted = polyval(p, x); % Evaluating the fit using the coefficients
This gives you the predicted values of y based on your linear model.
Visualizing the Results
Visualization is crucial for understanding the fit's performance. You can create a scatter plot of the original data and overlay the fitted linear line as follows:
figure;
scatter(x, y); % Original data points
hold on;
plot(x, y_fitted, '-r'); % Fitted line in red
title('Simple Linear Fit');
xlabel('x');
ylabel('y');
legend('Data', 'Linear Fit');
hold off;
This plot allows you to visually assess how well the linear model fits your data points.
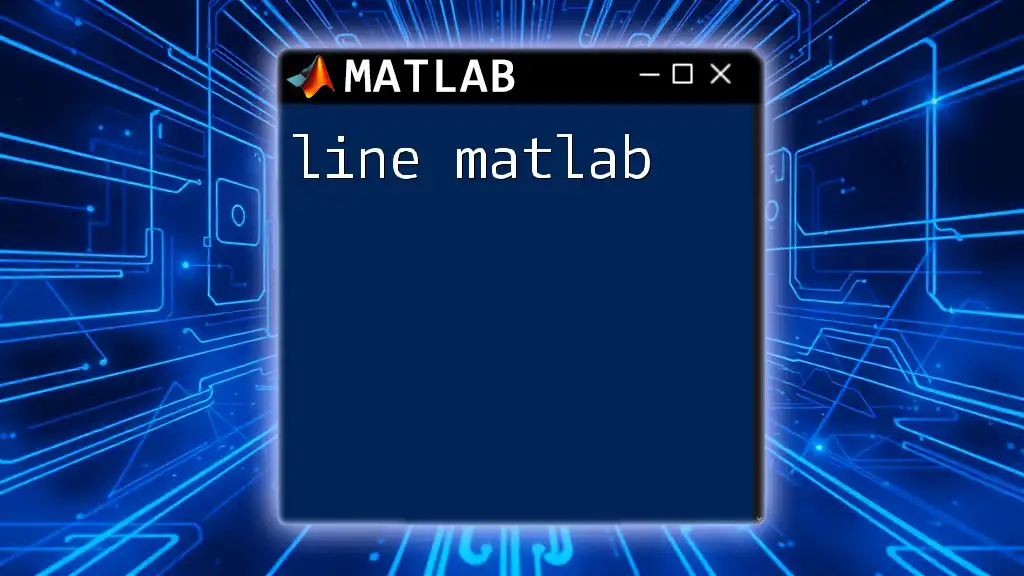
Performing Multiple Linear Fit in MATLAB
When to Use Multiple Linear Fit
Multiple linear fitting is applicable when the dependent variable is influenced by multiple independent variables. This method is particularly useful in fields such as economics and experimental sciences, where many factors interact to affect outcomes.
Step-by-Step Tutorial for Multiple Linear Fit
Example Dataset Preparation
When performing a multiple linear fit, your dataset will contain multiple factors. Here is an example where two independent variables affect the dependent variable:
x1 = [1, 2, 3, 4, 5]; % First predictor
x2 = [10, 20, 30, 40, 50]; % Second predictor
y = [4.5, 5.9, 7.1, 9.0, 10.5]; % Dependent variable
X = [x1', x2']; % Combining predictors into a matrix
Using `regress` for Multiple Linear Fit
To perform multiple linear fitting with these predictors, you can use the `regress` function. Here’s how you can do it:
b = regress(y', [ones(length(y), 1) X]); % Adding an intercept term
The resulting vector `b` contains the estimated coefficients for each predictor and the intercept.
Visualizing Multiple Fit
Visualizing multiple linear fits can be more complex due to the increased dimensionality. However, you can use 3D plots for cases with two independent variables to represent the surface of the fit.
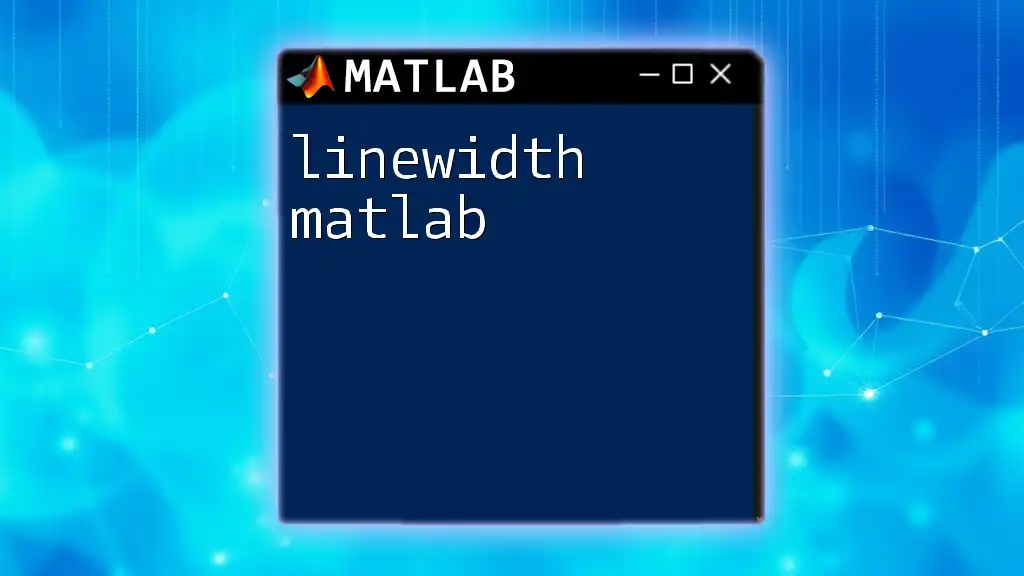
Interpreting the Results
Understanding Output Parameters
Interpreting the results of your linear fit involves analyzing the coefficients you obtained. In a simple fit, the slope (m) indicates the rate of change of the dependent variable for each unit of change in the independent variable. The intercept (b) shows the expected value of y when x is zero.
Diagnostic Plots
Diagnostic plots such as residual plots can help assess the fit. By plotting the residuals (the differences between observed and predicted values), you can determine whether your model's assumptions hold, such as constant variance and normality of errors.
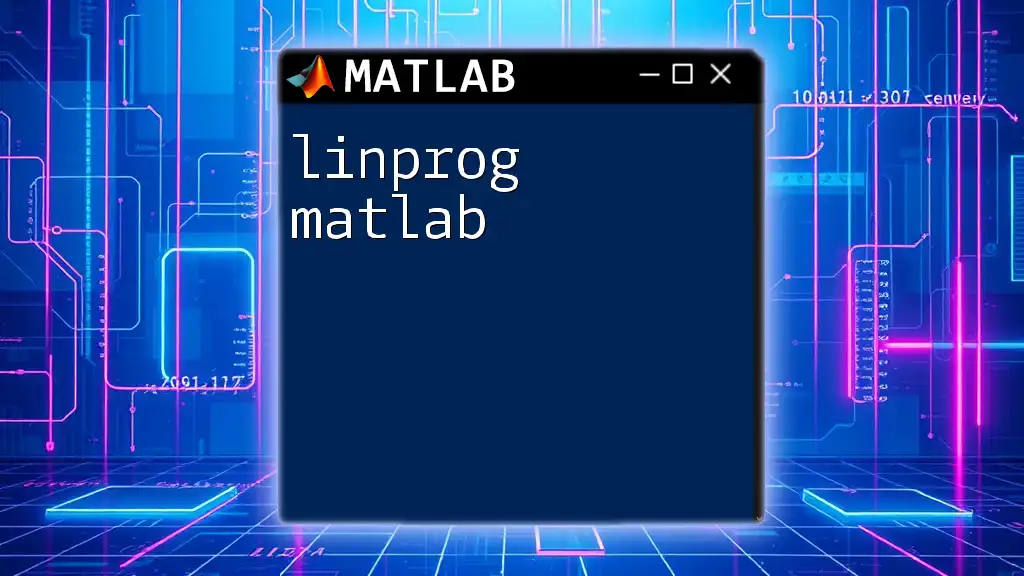
Common Issues and Troubleshooting
Overfitting vs Underfitting
Overfitting occurs when your model is too complex, capturing noise rather than the underlying relationship, while underfitting happens when the model is too simple to capture the trends in the data. Address these issues by using techniques such as cross-validation to determine the model's predictive performance.
Error Messages in MATLAB
MATLAB provides various error messages that can occur during execution. For instance, if your data contains NaN values, the fitting functions will fail. Regularly check and clean your dataset, ensuring it aligns with the function's requirements.
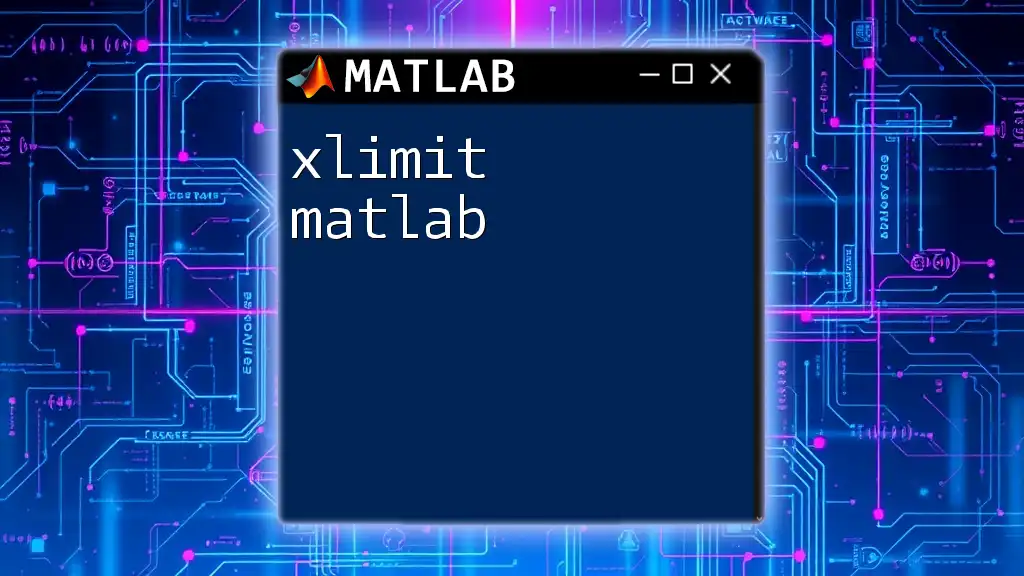
Conclusion
This article has provided you with a comprehensive overview of how to perform linear fitting using MATLAB, covering both simple and multiple linear analyses. By utilizing the functions `polyfit`, `polyval`, and `regress`, you can successfully model your data and draw meaningful conclusions.
As you continue your journey in using the powerful capabilities of MATLAB, don't hesitate to explore its robust documentation, tutorials, and resources available online to deepen your understanding of linear fit in MATLAB and other advanced techniques.