The `flipud` function in MATLAB is used to flip a matrix or array in the up-down direction, effectively reversing the order of its rows.
Here’s a code snippet demonstrating its usage:
A = [1, 2, 3; 4, 5, 6; 7, 8, 9];
B = flipud(A);
In this example, `B` will result in:
B =
7 8 9
4 5 6
1 2 3
Understanding the `flipud` Function
What is `flipud`?
The `flipud` function in MATLAB is used to flip matrices upside down. It essentially reverses the order of the rows in a given matrix, swapping the topmost row with the bottommost row, the second row from the top with the second row from the bottom, and so on. This operation is particularly useful in various data manipulation tasks, especially when working with multidimensional data or images.
Syntax of `flipud`
The basic syntax for using `flipud` is straightforward:
B = flipud(A)
Parameters:
- `A`: This is the input matrix which can be either numeric, logical, or even character data.
- `B`: This represents the output matrix that results from flipping `A` upside down.
How `flipud` Works
Matrix Dimensions: When you apply `flipud` to a matrix, the function works irrespective of the size of the matrix. Whether `A` is a 2D or a column vector, the operation effectively mirrors the matrix along its horizontal centerline.
Consider a matrix A:
A = [1 2 3;
4 5 6;
7 8 9]
After applying `flipud`, the output matrix `B` becomes:
B = [7 8 9;
4 5 6;
1 2 3]
The first row becomes the last, the second remains in the middle, and so on.
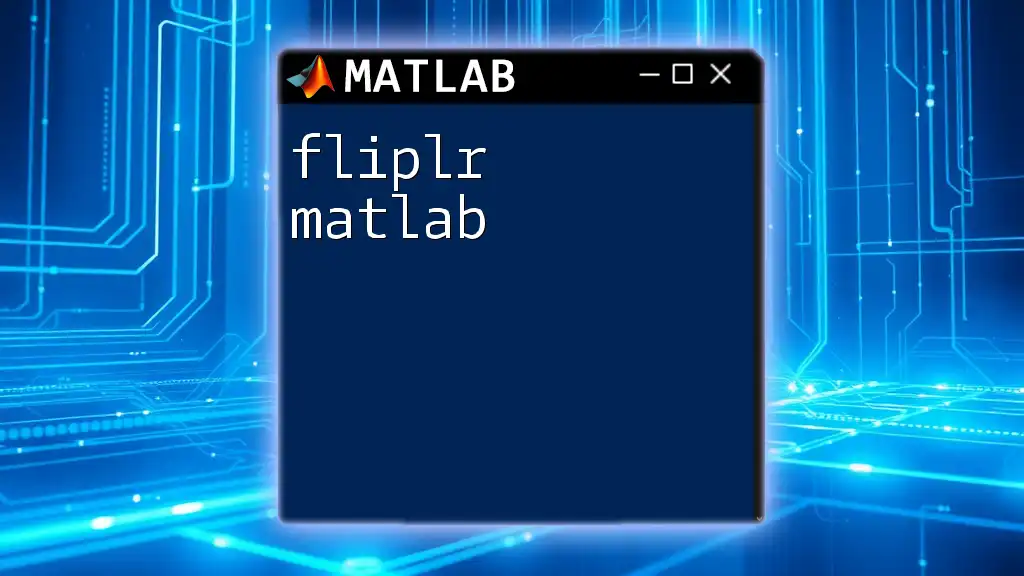
Practical Applications of `flipud`
Data Reversal
Flipping data is often essential in data analysis, especially when interpreting measurements gathered in a specific sequence. For instance, in time-series data, reversing the order might yield better insights or prepare the data for certain types of analysis.
Image Processing
In image processing, the `flipud` function can be utilized to manipulate image matrices. When you read an image into MATLAB, it’s often stored as a matrix where each pixel’s brightness level corresponds to a specific value in the matrix. By using `flipud`, you can create vertical flips of images.
Here’s a practical example:
img = imread('example.jpg'); % Read an image file
flippedImg = flipud(img); % Flip the image upside down
imshow(flippedImg); % Display the flipped image
This operation is particularly useful for data augmentation in machine learning scenarios where you want to increase the diversity of your training datasets.
Signal Processing
In signal processing, `flipud` can be a handy tool for reversing the order of signal samples. This is useful when analyzing waveforms or echoes, as some transformations necessitate analyzing the signal in a reversed format for better feature extraction.
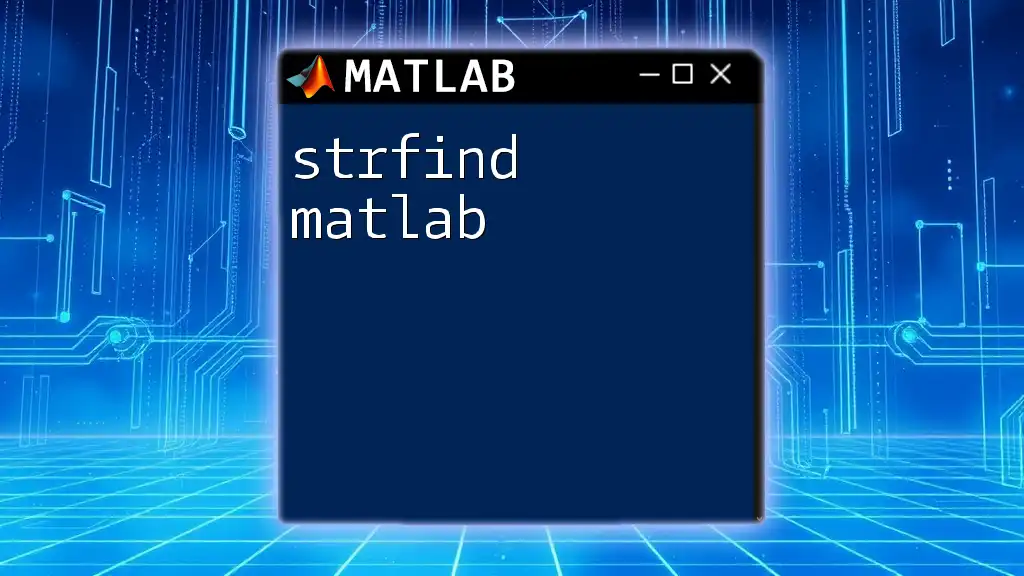
Examples and Code Snippets
Example 1: Flipping a Simple Matrix
Let’s create a simple 2D matrix to illustrate the use of `flipud`.
A = [1 2 3;
4 5 6;
7 8 9];
B = flipud(A);
disp(B);
Expected Output:
7 8 9
4 5 6
1 2 3
This demonstrates how the original matrix's rows are inverted to create a new matrix.
Example 2: Flipping an Empty Matrix
It’s also interesting to see how `flipud` behaves when working with an empty matrix.
C = [];
D = flipud(C);
disp(D); % Output: []
In this case, since there are no elements to flip, the output remains an empty matrix.
Example 3: Flipping a Column Vector
`flipud` can also be applied to column vectors, showcasing its versatility.
vec = [1; 2; 3; 4];
flippedVec = flipud(vec);
disp(flippedVec);
Expected Output:
4
3
2
1
In this example, every element of the vector is rearranged, effectively reversing the order of the data.
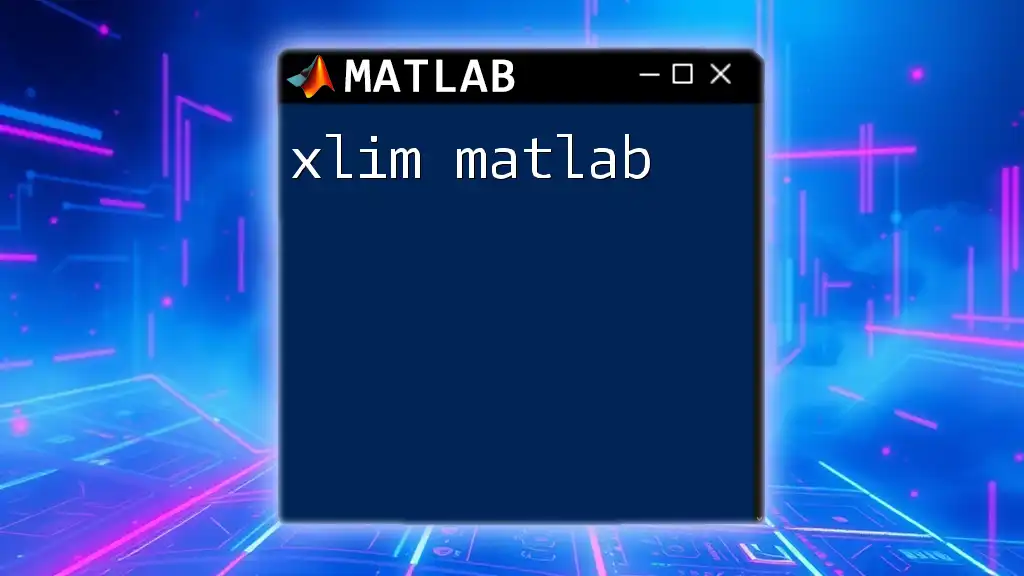
Performance Considerations
Disadvantages of Using `flipud`
While `flipud` is a simple and effective function, it's crucial to be mindful of performance concerns in larger datasets. Flipping very large matrices can be computationally intensive, especially if it’s performed repeatedly in a loop or alongside other time-consuming operations.
Alternatives to `flipud`
In scenarios where `flipud` might be inefficient, consider alternative methods such as logical indexing. For example, you can achieve the same result by creating a reverse index for the rows of a matrix:
B = A(end:-1:1, :);
This approach may be more suitable when you need to perform conditional logic during the flip operation.
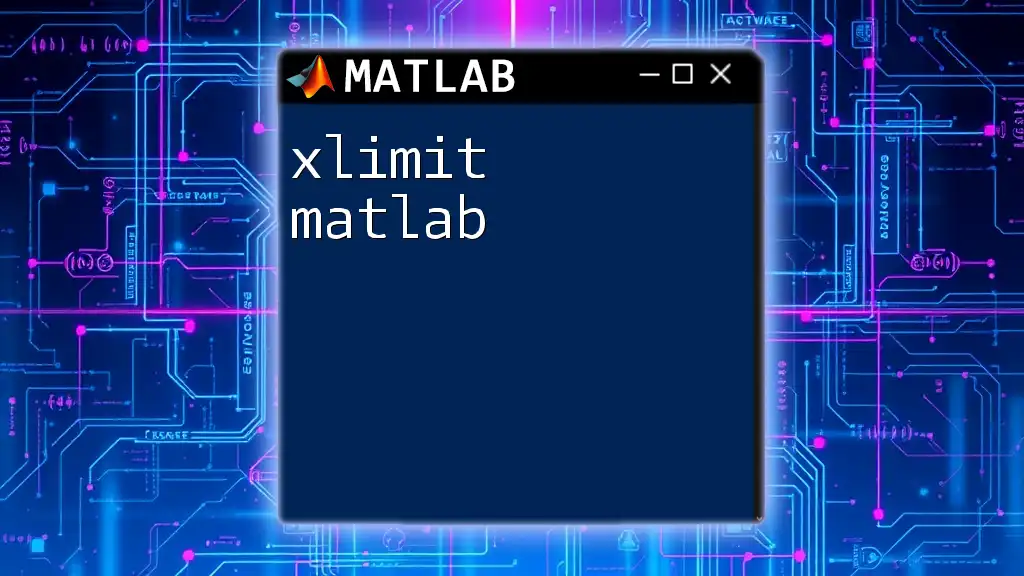
Frequently Asked Questions (FAQ)
Common Issues and Solutions
-
Why isn’t `flipud` working as expected with my data?
Ensure that the input matrix is non-empty and properly formatted. Check for any data type mismatches that might occur. -
Can `flipud` be used on 3D arrays?
No, `flipud` is specifically designed for 2D matrices. To flip along specific dimensions in higher-dimensional data, consider using `flip` with the optional dimension parameter.
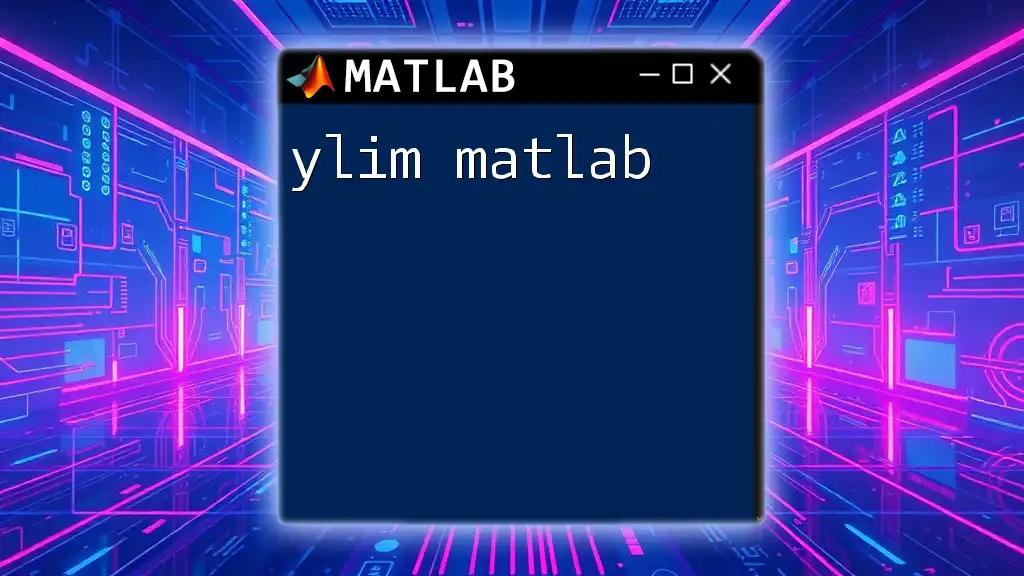
Conclusion
The `flipud` function in MATLAB is an essential tool for matrix manipulation, particularly useful in data analysis and image processing. By understanding its syntax, applications, and limitations, you can effectively incorporate `flipud` into your MATLAB programming practices. As you experiment with this function, you'll discover its importance in various projects and its ease of use.
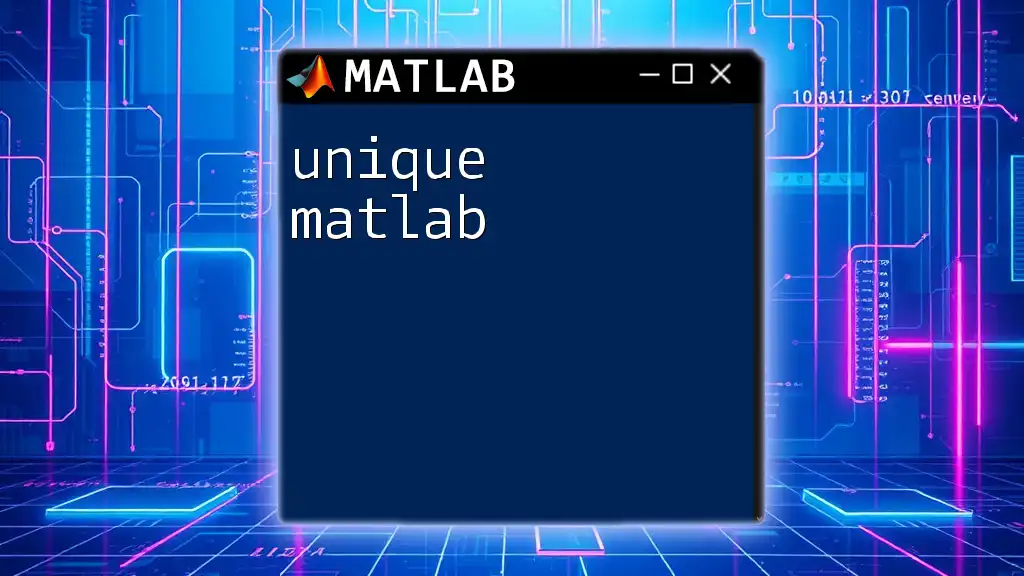
Additional Resources
For more in-depth exploration, refer to the official MATLAB documentation surrounding matrix manipulation and the `flipud` function. Engage with online tutorials and communities for additional techniques and applications.
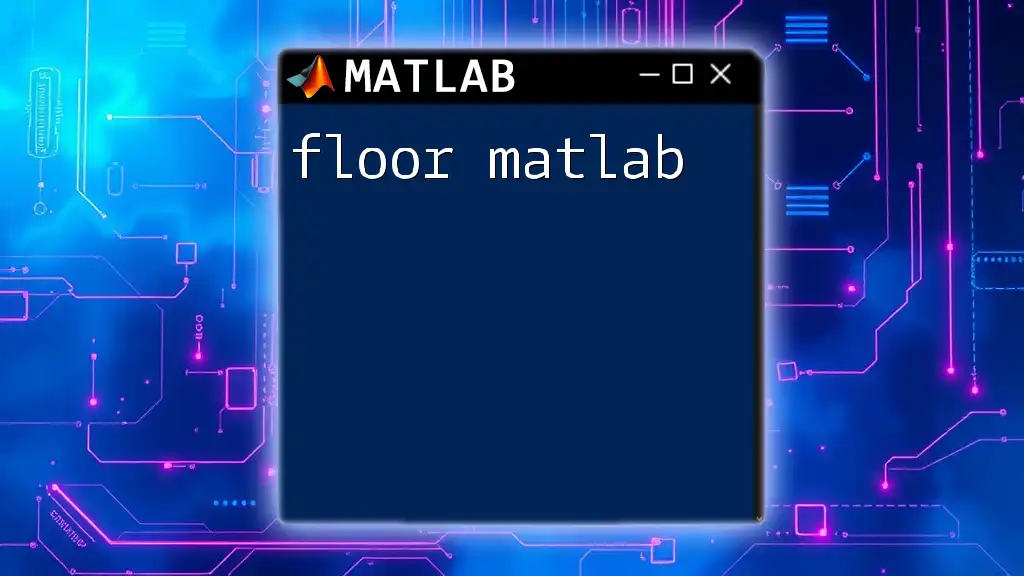
Call to Action
If you found this guide helpful, stay tuned for more quick and concise MATLAB tutorials that will enhance your practical coding skills. Let us know what topics you're interested in exploring next!