The `imagesc` function in MATLAB displays matrix data as an image with scaled colors, allowing for easy visual interpretation of numerical values.
% Example of using imagesc in MATLAB
data = rand(10); % Create a 10x10 matrix of random values
imagesc(data); % Display the matrix as an image
colorbar; % Add a colorbar to indicate the scale
Understanding the Basics of imagesc in MATLAB
What is imagesc?
The `imagesc` function in MATLAB is primarily designed for visualizing matrices as images. It is an efficient way to display data as colored pixels, where the color reflects the values in the matrix. Unlike other functions such as `imshow` or `image`, `imagesc` automatically scales the matrix values to the range of the current colormap, providing a quick and intuitive representation of numerical data.
Syntax of imagesc
Using the `imagesc` function is straightforward. The general syntax is as follows:
imagesc(C)
Where `C` is the matrix that you would like to visualize. `imagesc` also allows for additional input arguments to customize the display:
- Colormap options: You can choose a specific colormap to better represent your data.
- Axes properties: Additional parameters to modify the axes can also be incorporated in your function call for enhanced clarity.
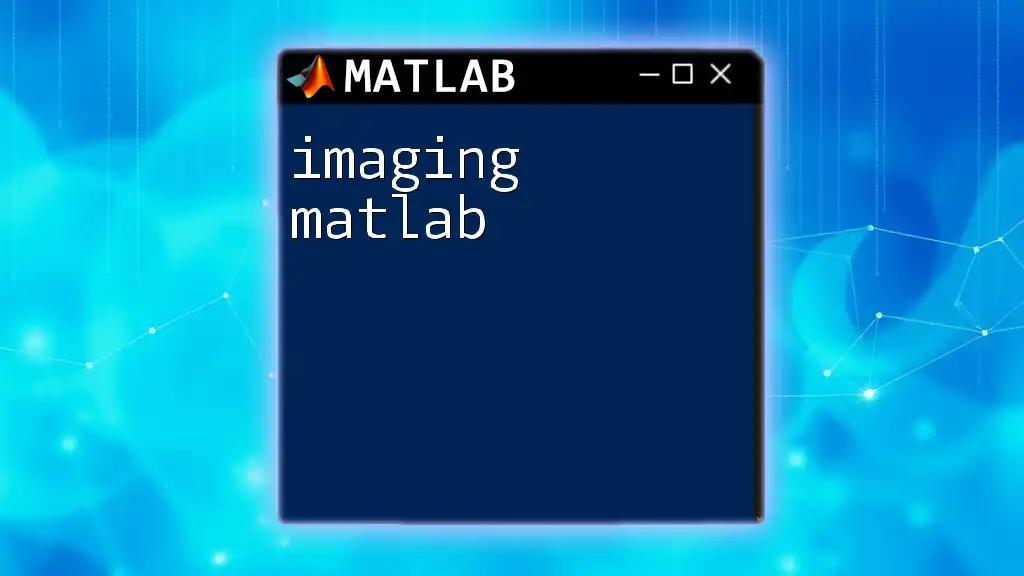
Preparing Data for imagesc
Creating Sample Data
Before using `imagesc`, you need to prepare your data. For demonstration purposes, let’s create a simple 10x10 matrix of random values:
data = rand(10,10);
This command generates a 10x10 matrix filled with random numbers between 0 and 1, which serves as a great sample dataset to visualize.
Data Normalization
In many cases, the values in your input matrix might need to be normalized, especially if they vary widely. Normalization ensures the data is in a consistent range, which makes the visualization more effective. Here's how to normalize the data:
data_normalized = (data - min(data(:))) / (max(data(:)) - min(data(:)));
This line of code rescales the values of `data` to a range between 0 and 1, leveraging the minimum and maximum values.
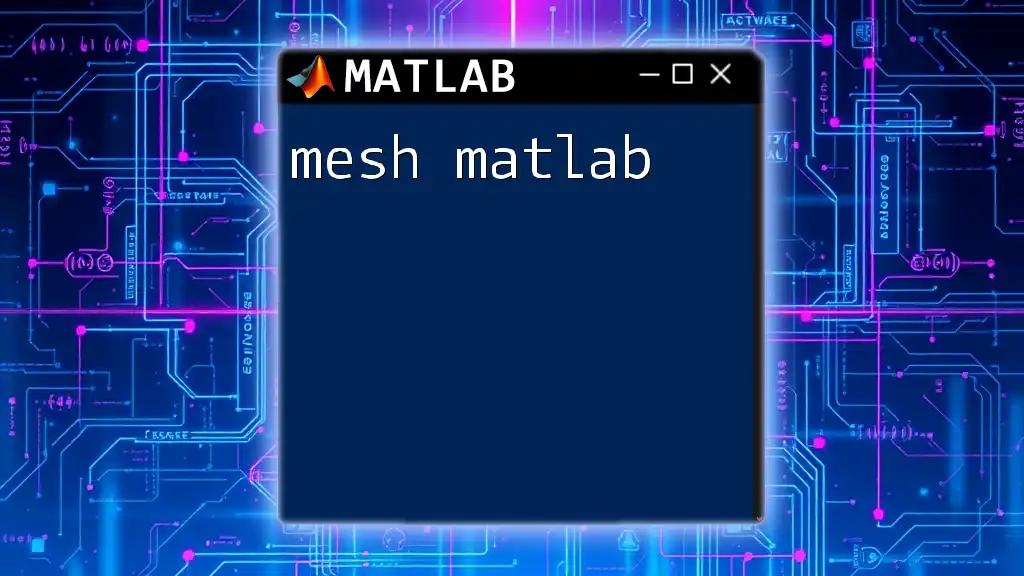
Using imagesc
Basic Usage of imagesc
To visualize the matrix you've prepared, you simply call `imagesc` as follows:
imagesc(data);
colorbar;
The `colorbar` function adds a color scale next to your image, indicating how matrix values correspond to colors on the display. After running this code, you will see a visual representation of your matrix, where different colors represent different values.
Customizing the Display
Adding Colormaps
MATLAB provides a wide range of colormaps to enhance the clarity of your data visualizations. You can change the default colormap by utilizing the `colormap` function. For example, to apply the 'jet' colormap, you would use:
colormap('jet');
This command changes the color scheme to range from blue (low values) to red (high values), allowing for better visual distinctions between varying matrix elements.
Setting Axis Properties
Adding labels and titles enhances the interpretability of your visuals. Below is how you can customize the axes:
xlabel('X-axis label');
ylabel('Y-axis label');
title('Title of the Image');
By assigning descriptive labels, viewers can quickly understand what dimensions the data represents, making your visualization more informative.
Modifying Color Limits
Another useful feature is the ability to manually set the color limits for your visualization using the `caxis()` function. This function defines the mapping of data values to the colormap range. For instance:
caxis([0 1]);
This tells MATLAB to treat the range of your data values between 0 and 1 for color mapping, which is particularly helpful if you want specific emphasis on certain data regions.
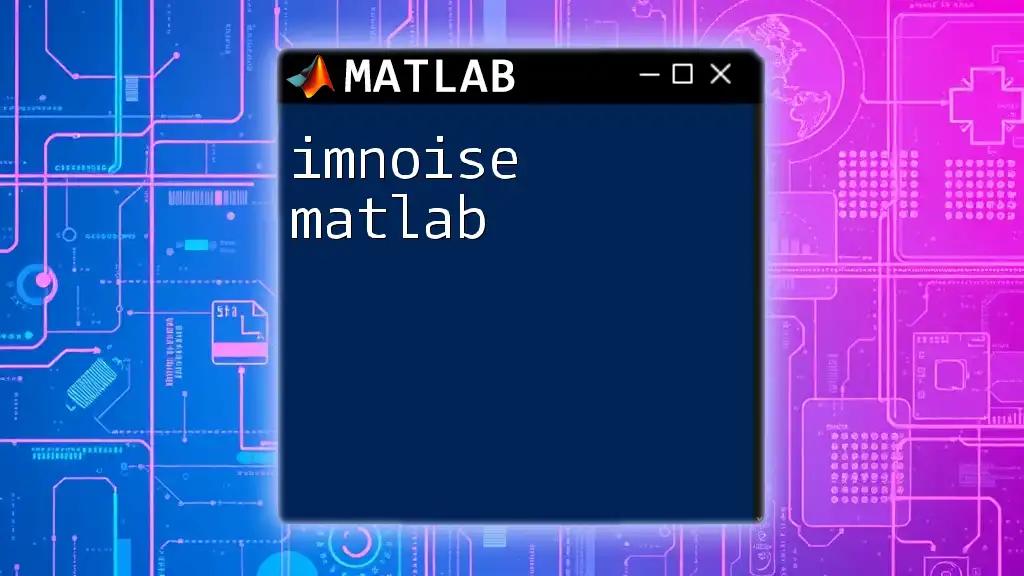
Advanced features of imagesc
Handling Color Maps
In addition to the predefined colormaps, you can create custom colormaps tailored to your specific application. Custom colormaps allow greater flexibility when it comes to highlighting specific data ranges. For example, the following code snippet creates a colormap containing red, green, and blue:
custom_cmap = [1 0 0; 0 1 0; 0 0 1]; % Red, Green, Blue
colormap(custom_cmap);
By designing a colormap that meets your visual requirements, you can effectively communicate the subtleties of your data.
Overlapping Multiple Images
When you want to visualize multiple datasets in a single plot, MATLAB’s `hold on` command facilitates this by layering images on top of each other. Here’s a basic example:
hold on; % Hold current plot
imagesc(data1); % Display first dataset
imagesc(data2, 'AlphaData', 0.5); % Display second dataset with transparency
hold off; % Release plot
In this code, the second image is overlaid with 50% transparency, allowing viewers to see both datasets simultaneously. This approach is particularly advantageous in comparative analysis.
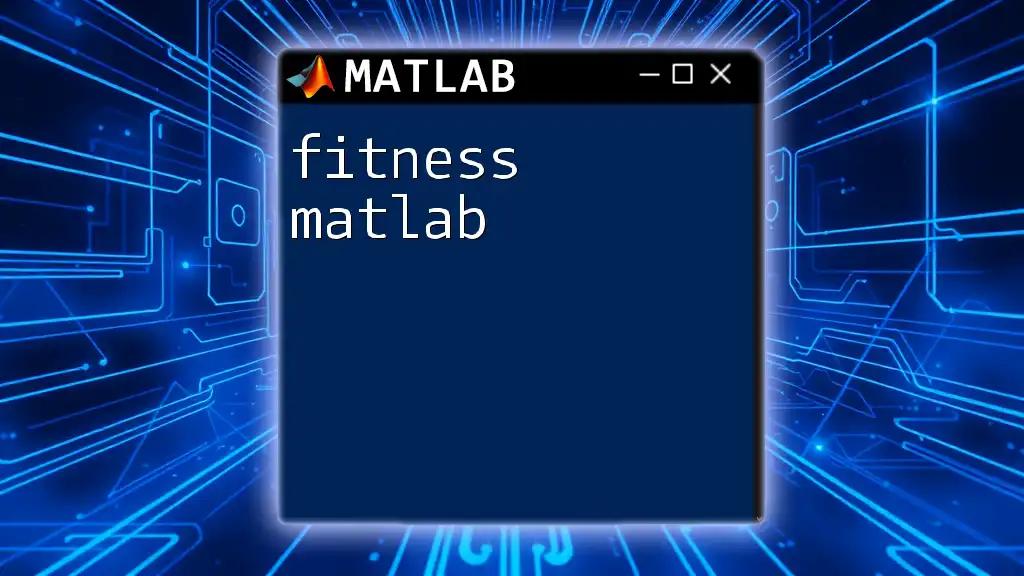
Troubleshooting Common Issues
What to Do When Data Doesn't Display Correctly
Issues with visualizing your data can arise, particularly when the matrix sizes do not match or improper values are present. To ensure your data displays correctly, confirm that the matrix dimensions align with expected outputs. Also, ensure that your data does not contain NaN or infinite values, which can disrupt the visualization process.
Performance Considerations
When working with large datasets, `imagesc` might become slow or unresponsive. If you find the performance lacking, consider using reduced datasets or leveraging other visualization techniques that are better optimized for large-scale data.
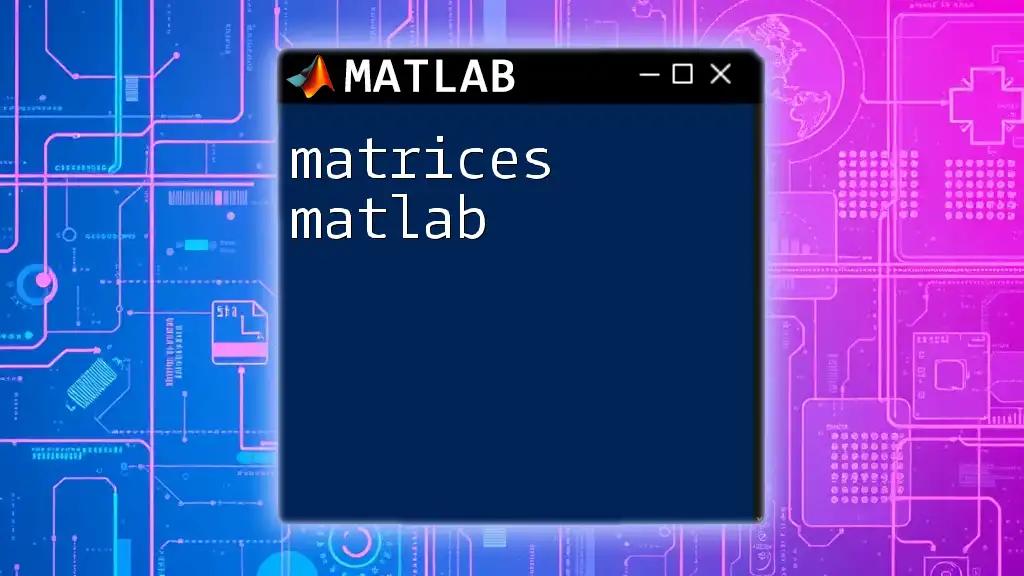
Practical Applications
Case Study: Visualizing Heatmaps
One practical application of `imagesc` is in generating heatmaps. This technique allows for quick visual assessments of data distributions:
data = rand(100); % A larger dataset for heatmap
imagesc(data);
colorbar;
In this case, a 100x100 matrix is visualized. The heatmap easily conveys differences in data density and can be essential in exploratory data analysis.
Integrating imagesc with GUI Applications
If you're developing GUI applications, `imagesc` can seamlessly integrate into uicontrols. This allows users to interact with the visualizations dynamically, offering a more engaging experience.
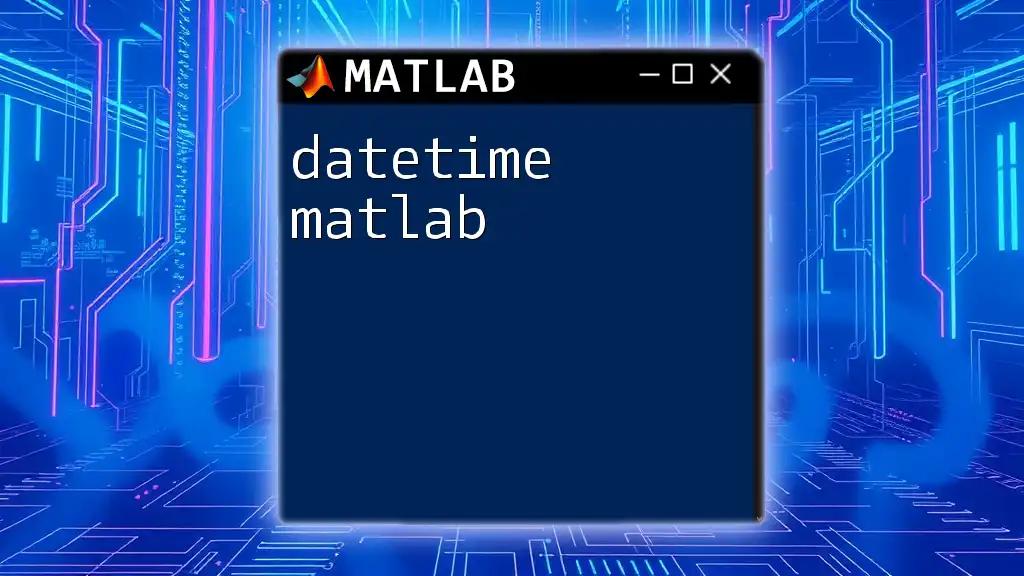
Conclusion
The versatility of `imagesc` in MATLAB cannot be understated. Its ability to turn matrices into vivid visual representations enables users to grasp complex datasets quickly. If you're eager to sharpen your data visualization skills, don't hesitate to experiment with `imagesc` and explore other MATLAB visualization functions to see how they can enhance your data storytelling.
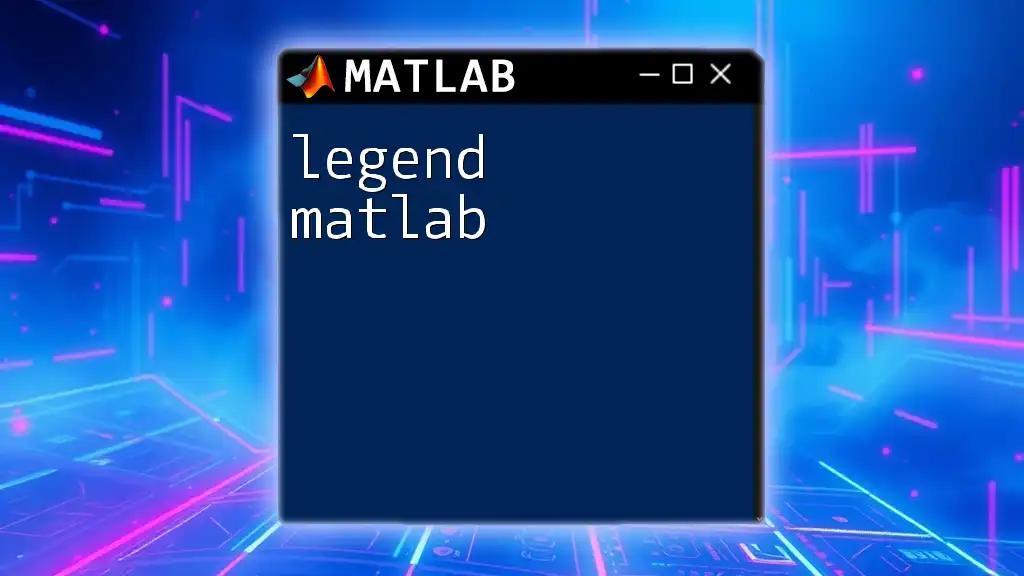
Further Resources
To deepen your understanding, refer to the official MATLAB documentation, where you’ll find extensive resources regarding `imagesc` and other visualization functions. Additionally, exploring learning materials like online tutorials and community forums can offer practical insights and examples tailored to your needs.
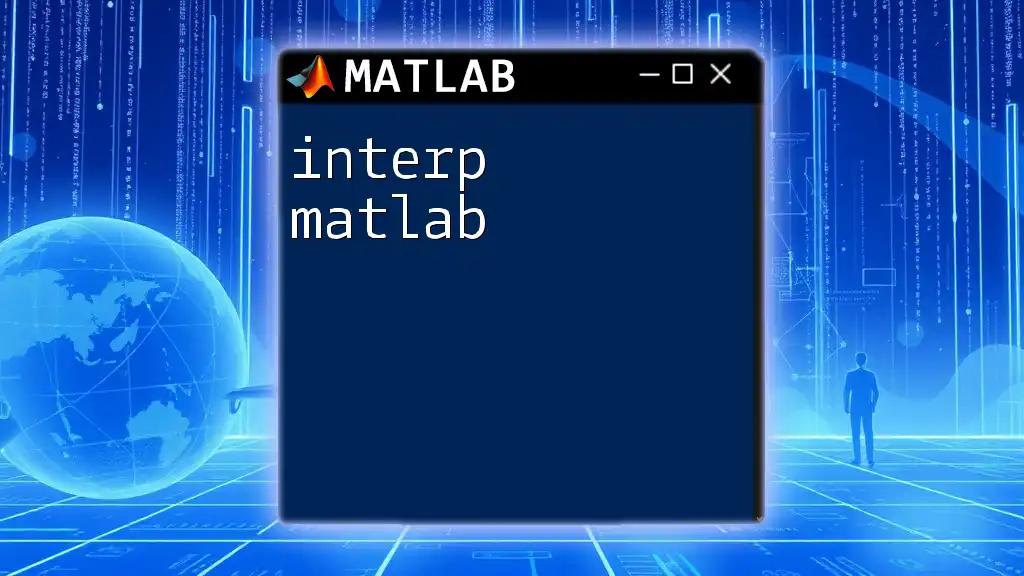
Frequently Asked Questions (FAQs)
Common Questions about imagesc
One common question is about the difference between `imagesc` and other image display functions. Whereas `imagesc` scales your data to the colormap automatically, functions like `imshow` expect image data formatted in specific ways.
Another frequent issue users face concerns adjusting the aspect ratio of displayed images. To maintain the correct proportionality, use the command `axis equal` following the `imagesc` call.
Lastly, many ask whether `imagesc` can work with 3D data. While `imagesc` itself is typically for 2D matrices, you can visualize slices of 3D data by extracting 2D matrices and applying `imagesc` on those slices.