For loops in MATLAB allow you to execute a set of commands repeatedly for a specified range or array of values, enabling efficient iteration through elements.
Here's a simple example:
for i = 1:5
disp(['Iteration: ', num2str(i)]);
end
Understanding For Loops in MATLAB
What is a For Loop?
In programming, a for loop is used to execute a block of code a specific number of times. In MATLAB, for loops are essential for performing repetitive tasks efficiently, allowing you to iterate through arrays, matrices, and other data structures. This powerful feature can help automate many processes, making your coding more efficient and reducing the potential for human error.
Syntax of a For Loop
The basic syntax of a for loop in MATLAB is straightforward:
for index = start:increment:end
% Code to execute
end
- index represents the loop variable that changes with each iteration of the loop.
- start is the initial value of the loop variable.
- increment (optional) is the step size by which the index variable increases after each iteration.
- end indicates the final value at which the loop will stop executing.
Example of a Basic For Loop
Consider the following simple example:
for i = 1:5
disp(i)
end
In this example, the code inside the loop will execute five times, displaying the values 1 through 5. The loop starts at 1 and increments by 1 until it reaches 5.
Advanced Usage of For Loops
Specifying Increment Values
You can customize the increment value according to your needs. For instance, if you want to increment by 2:
for i = 1:2:10
disp(i)
end
This will output the values 1, 3, 5, 7, and 9. Customizing increments is particularly useful when working with sequences or applying subsets of data.
Nested For Loops
What are Nested For Loops?
Nested for loops are loops within loops. This structure allows you to perform more complex iterations, particularly when dealing with multi-dimensional data.
Example of Nested For Loops
For example, consider the following code:
for i = 1:3
for j = 1:2
fprintf('i: %d, j: %d\n', i, j);
end
end
In this case, the outer loop runs three times while the inner loop runs two times for each iteration of the outer loop. The output will display pairs of `i` and `j`, demonstrating the relationship between the two variables and the structure of the loops.
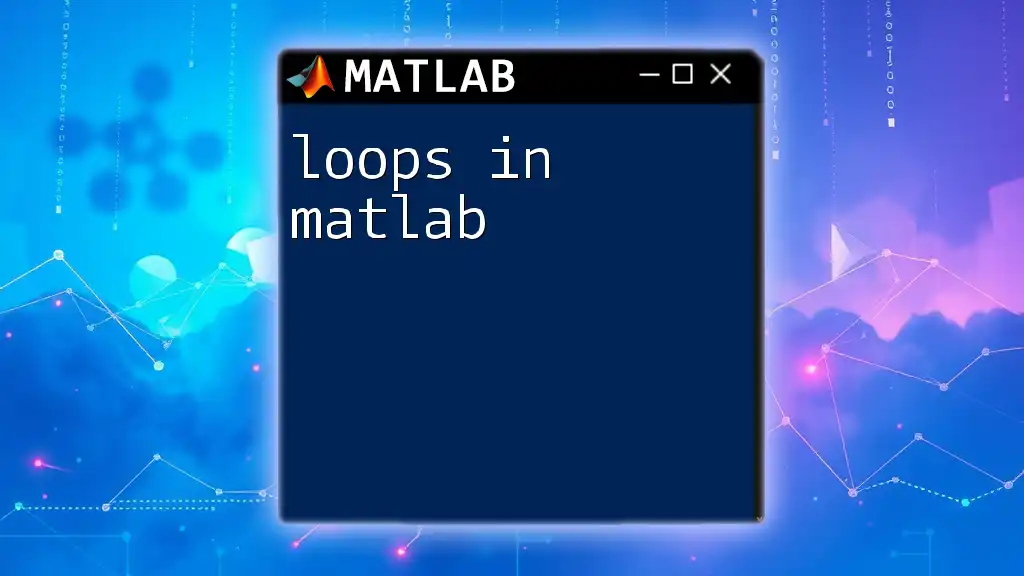
Practical Applications of For Loops
Iterating over Arrays
For loops are particularly useful when you need to manipulate or analyze data stored in arrays.
Example of Array Manipulation
Take a look at the following example that doubles each element in an array:
A = [2, 4, 6, 8];
for i = 1:length(A)
A(i) = A(i) * 2;
end
disp(A);
The output of this operation will be `[4, 8, 12, 16]`. This example illustrates how to iterate over an array's elements and modify them, showcasing the utility of for loops in data processing tasks.
Using For Loops with Functions
Creating custom functions that utilize for loops fosters modularity in your code, making it reusable and easier to debug.
Creating Custom Functions
Here’s an example of a function that calculates the sum of squares of the first `n` integers:
function total = sumOfSquares(n)
total = 0;
for i = 1:n
total = total + i^2;
end
end
By calling `sumOfSquares(3)`, you will receive the output `14`, which accounts for \(1^2 + 2^2 + 3^2\). This modular approach allows the function to be used with different values of `n` easily.
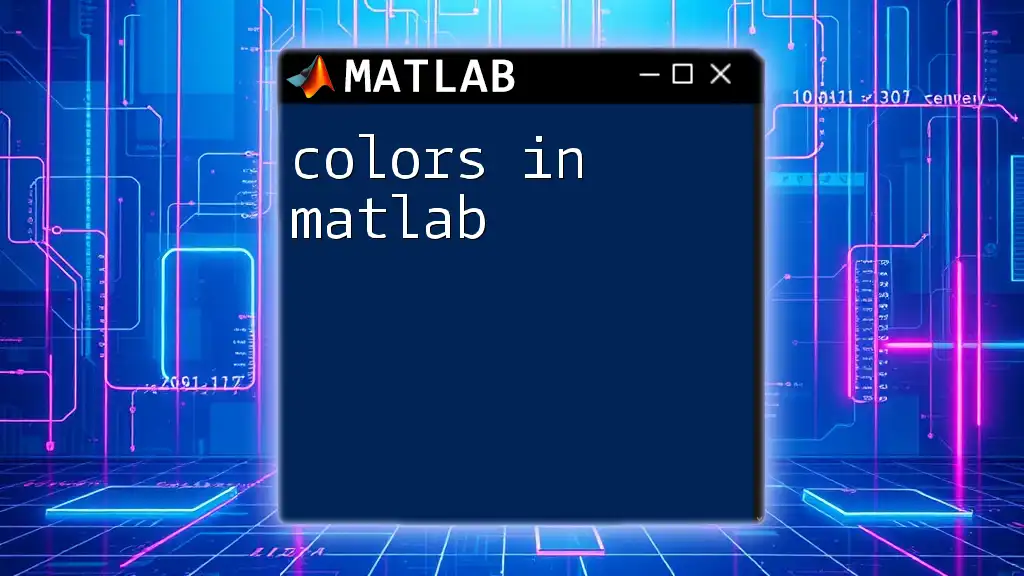
Best Practices with For Loops
Avoiding Common Pitfalls
One common issue users encounter is indexing errors. Remember that MATLAB uses 1-based indexing, meaning the first element of an array has an index of 1, not 0. Additionally, watch for off-by-one errors, where the loop either runs one iteration too few or too many.
Performance Considerations
For loops can significantly impact performance, especially with large datasets. Therefore, consider whether vectorized operations could achieve the same goal more efficiently. MATLAB is optimized for operations on arrays, and vectorization often leads to faster execution times.
Code Readability
The clarity of code is paramount. Use meaningful variable names and include comments to explain complex logic within your loops. Consider the following:
for index = 1:length(dataArray)
% Process each data element
dataArray(index) = dataArray(index) * scalingFactor;
end
Adding comments ensures that anyone reading the code will understand its purpose quickly.
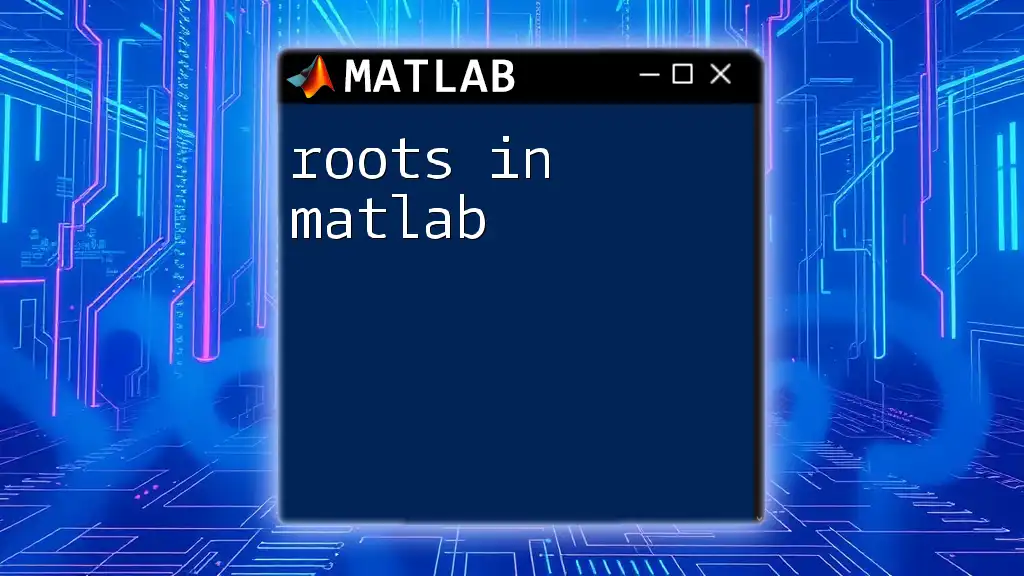
Conclusion
For loops in MATLAB are indispensable for performing repetitive tasks and automating processes. Mastering the concept of for loops can significantly enhance your coding capabilities, particularly when dealing with arrays and complex calculations. By practicing and experimenting with various examples, you can develop a deeper understanding of this powerful feature in MATLAB.
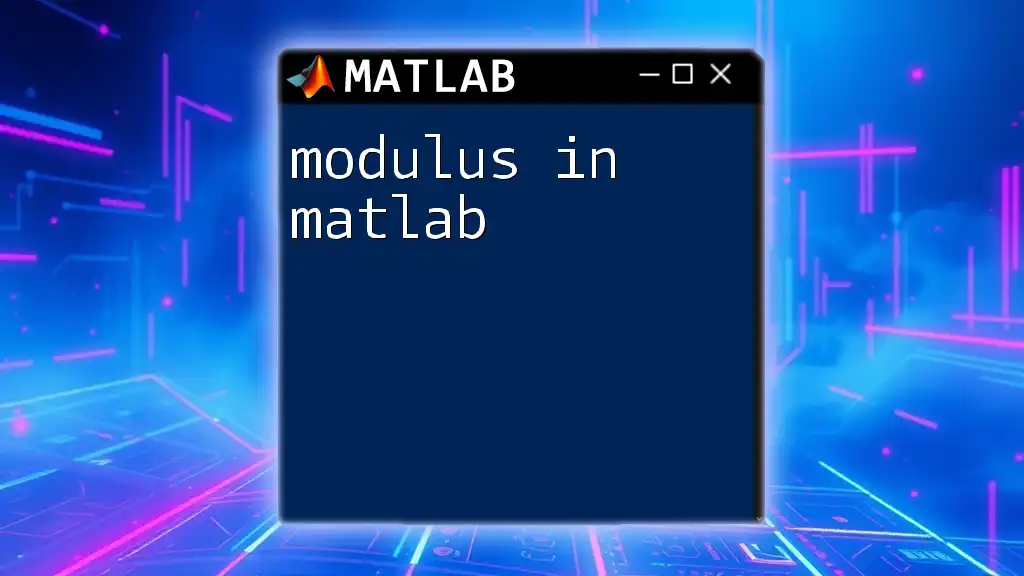
Additional Resources
Further Learning
For those eager to expand their knowledge, consider exploring MATLAB's official documentation, online courses, and tutorials that delve deeper into for loops and other MATLAB functionalities.
Community and Support
Engaging with MATLAB user communities and forums can provide answers to specific problems you encounter, as well as insights from experienced users.
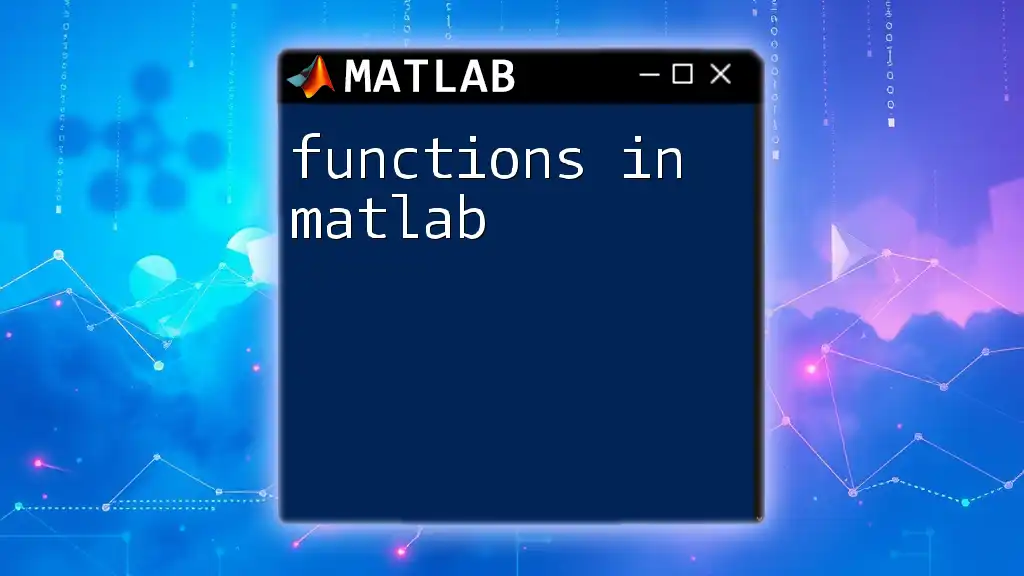
Call to Action
Now that you've learned about for loops in MATLAB, it's time to put that knowledge into practice! Start experimenting with your own for loops and see what you can create!