In MATLAB, functions are reusable blocks of code that can take inputs, perform specific operations, and return outputs, allowing users to create modular and organized programs.
Here’s a simple example of a MATLAB function that adds two numbers:
function sum = addNumbers(a, b)
sum = a + b;
end
Why Use Functions in MATLAB
Benefits of Functions
Code Reusability is one of the standout features of functions in MATLAB. By writing a function once, you can call it multiple times throughout your code, which significantly reduces redundancy. This not only saves time but also simplifies maintenance, as any changes made to the function will automatically reflect everywhere it is called.
Modularity refers to breaking your code into smaller, manageable pieces. Functions allow you to compartmentalize complex tasks. Instead of writing an extensive script that performs multiple operations sequentially, you can create functions for each task. This makes it easier to test and debug your code, as you can isolate issues more effectively.
Improved Readability is another important advantage. Well-named functions convey the purpose of the code, making it easier for others (and yourself) to understand what each part does. Instead of a long, convoluted script, a few well-defined function calls can clarify your intentions.
Real-World Applications
Functions in MATLAB have a plethora of applications across disciplines. In engineering, functions can model dynamic systems, making complex calculations easier to manage. In data analysis, they can automate repetitive tasks, such as data cleaning and transformation. In academia, researchers often use functions to simulate experiments or analyze results, illustrating how critical functions are in diverse scenarios.
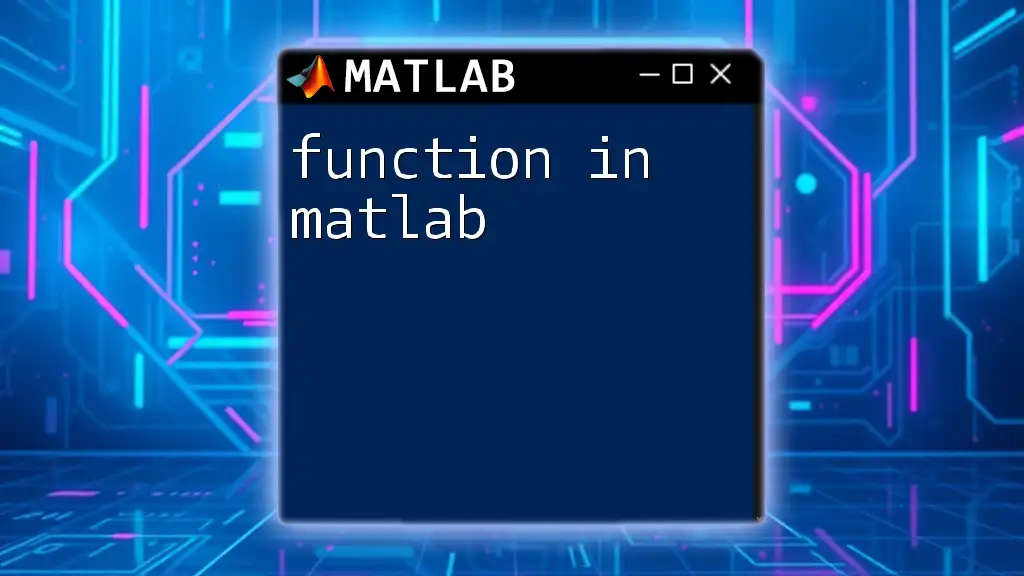
Understanding MATLAB Functions
Definition and Structure
At its core, a function in MATLAB is a reusable block of code. The basic structure of a MATLAB function consists of the following elements:
function [output1, output2] = functionName(input1, input2)
% Function code goes here
end
The function starts with the `function` keyword, followed by the outputs in square brackets. The function name must be unique and is followed by the input arguments in parentheses. The body of the function is where the code is executed, and the `end` keyword concludes the function definition.
Types of Functions
Built-in Functions
MATLAB comes equipped with numerous built-in functions that simplify programming tasks. They tackle common mathematical operations and data analysis needs. For example, the `sum` function computes the total of a set of numbers, which can be invoked as follows:
total = sum(data);
Other commonly used built-in functions include `mean`, which calculates the average of datasets, and `plot`, which visualizes data in graphical form.
User-Defined Functions
In addition to built-in functions, you can create user-defined functions tailored to your specific needs. For instance, consider the following simple addition function:
function result = addTwoNumbers(a, b)
result = a + b;
end
This function takes two inputs `a` and `b`, adds them, and returns the result. You can call this function in the command window or in another script, streamlining operations without duplicating code.
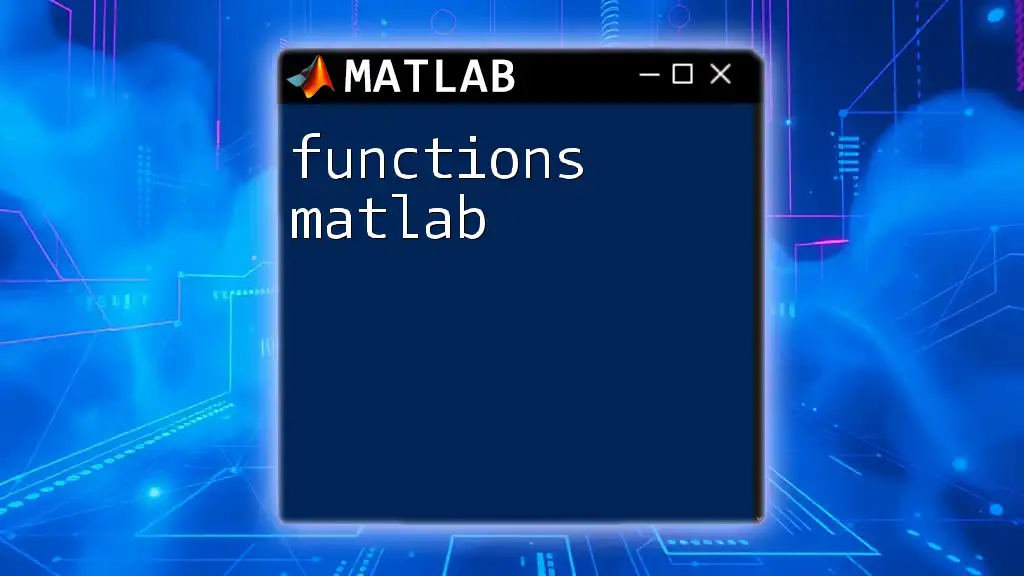
Creating Functions in MATLAB
Step-by-Step Guide
To create an effective function in MATLAB, consider the following steps:
-
Choosing a Function Name: The name should be descriptive enough to indicate what the function does while adhering to MATLAB's naming conventions (e.g., no spaces, starting with a letter).
-
Defining Inputs and Outputs: Identify the required input parameters and corresponding outputs that your function will handle.
-
Writing Function Code: Be concise and clear. Aim for efficiency and avoid redundant computations within the function body.
-
Saving and Calling Functions: Save your function in a `.m` file with the same name as the function. You can call the function from the command window by using the function name followed by its inputs.
Example: Creating a Function to Calculate Area
Let's create a practical function to calculate the area of a rectangle. The following MATLAB code defines a function named `rectangleArea` that takes length and width as inputs.
function area = rectangleArea(length, width)
area = length * width;
end
To use this function, simply type:
areaValue = rectangleArea(5, 3);
This returns `15`, which is the area of a rectangle with those dimensions.
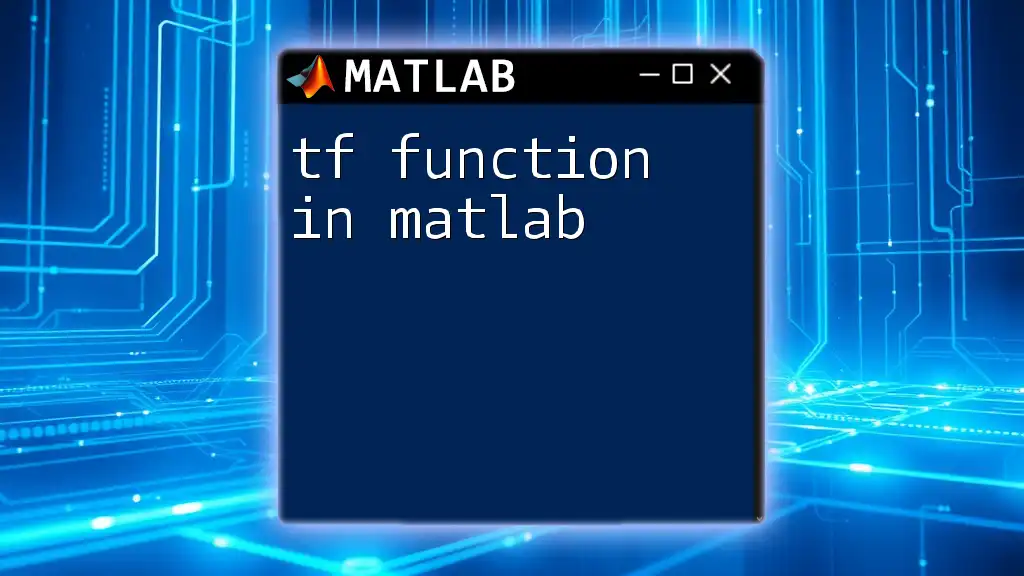
Function Scope and Variable Lifetime
Understanding Scope
Scope pertains to the visibility and lifetime of variables in your functions. There are two main types of variables:
-
Local Variables are defined within a function and can only be accessed there. They do not exist outside the function.
-
Global Variables, on the other hand, are accessible throughout the MATLAB workspace. To declare a global variable, you must use the `global` keyword inside both the base workspace and the function. However, be cautious with globals, as they can lead to unexpected behaviors when overused.
Persistence of Variables
Persistent variables maintain their values between function calls. This allows you to keep track of a value without having to use globals. Here is an example:
function count = persistentCounter()
persistent c;
if isempty(c)
c = 0;
end
c = c + 1;
count = c;
end
In this example, every time `persistentCounter` is called, it increments `c` and retains its value between calls.
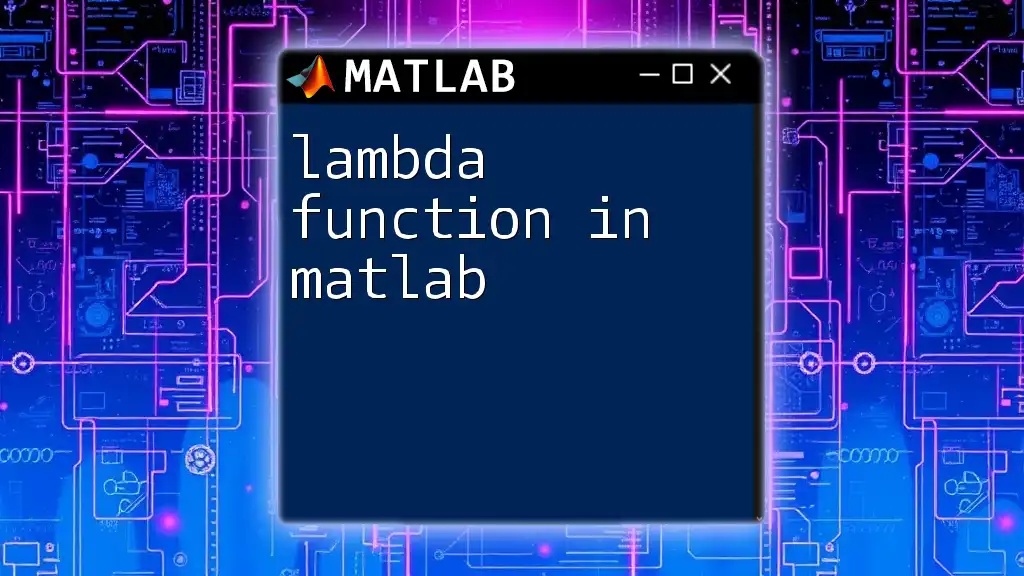
Advanced Functional Techniques
Function Handles
Function handles provide a powerful way to pass functions as arguments or to store functions in variables. They are particularly useful in optimization problems or function plotting. You can create a function handle like this:
f = @sin; % Create a function handle for the sine function
This handle can then be used in various MATLAB functions, allowing for flexible programming.
Anonymous Functions
Anonymous functions are single-line expressions defined without a separate file. This feature can save time in coding when a simple operation is necessary. For example:
square = @(x) x.^2;
result = square(5);
Here, `square` is an anonymous function that takes an input `x` and returns its square.
Nested Functions
Nested functions have their own scope and can access variables from containing functions. You define a nested function inside a parent function. Here’s an example:
function outerFunc()
function innerFunc()
disp('Hello from the inner function!');
end
innerFunc(); % Call inner function
end
`innerFunc` is accessible only within `outerFunc`, providing a great way to encapsulate behavior.
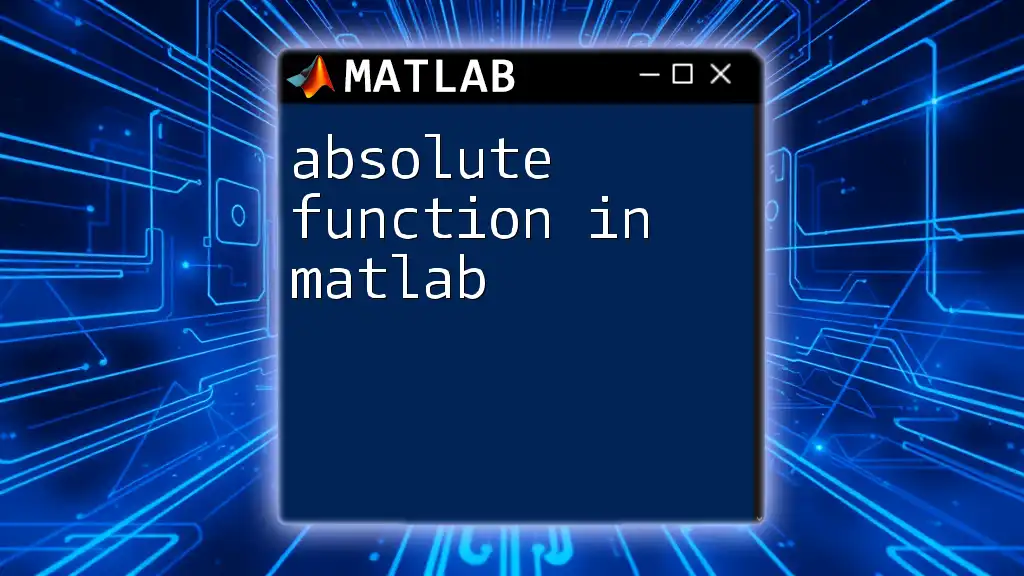
Debugging Functions in MATLAB
Common Errors
Working with functions in MATLAB is not without challenges. Common pitfalls include mismatched input/output arguments and not defining variables properly. Understanding how to structure your functions properly can be key to avoiding these errors.
Using the MATLAB Debugger
MATLAB’s built-in debugger is an invaluable tool for troubleshooting. Here’s how to use it effectively:
-
Setting Breakpoints: You can click on the left of the code window to set breakpoints. This pauses execution at a designated line, allowing you to inspect variables and flow of control.
-
Using Step Through: The step function allows you to monitor how your code executes line by line, which is helpful in understanding and fixing bugs.
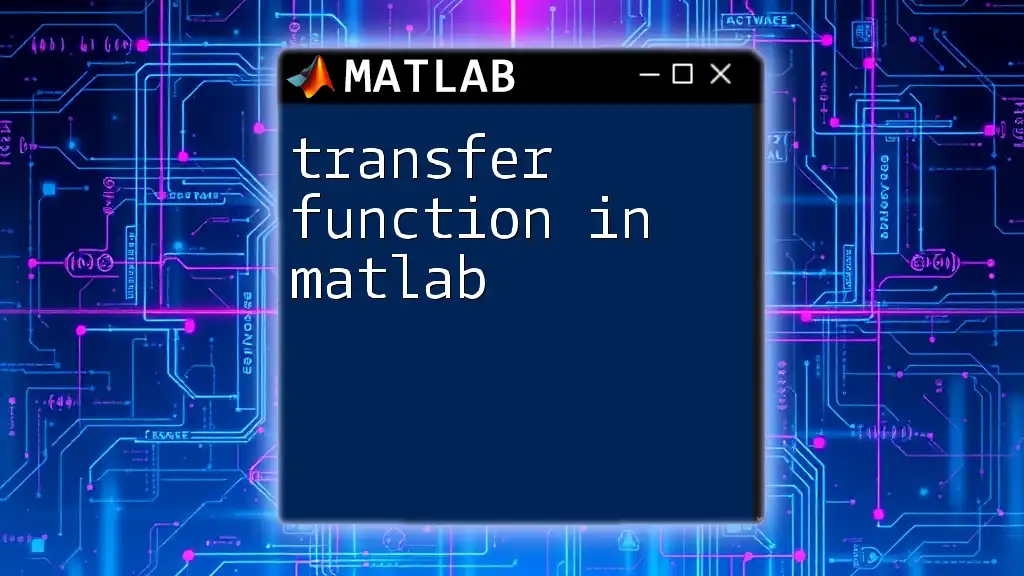
Conclusion
Mastering functions in MATLAB is crucial for writing efficient, readable, and reusable code. As you become more familiar with functions, you will find that they not only streamline your programming workflow but also enhance the comprehensibility of your projects. Functions allow you to encapsulate functionality, making it easier to debug and maintain code over time.
As you embark on your journey of learning, don’t hesitate to experiment with creating and modifying functions. The more you practice, the more intuitive it will become. Keep pushing your boundaries and exploring the power that functions in MATLAB can bring to your computational tasks!
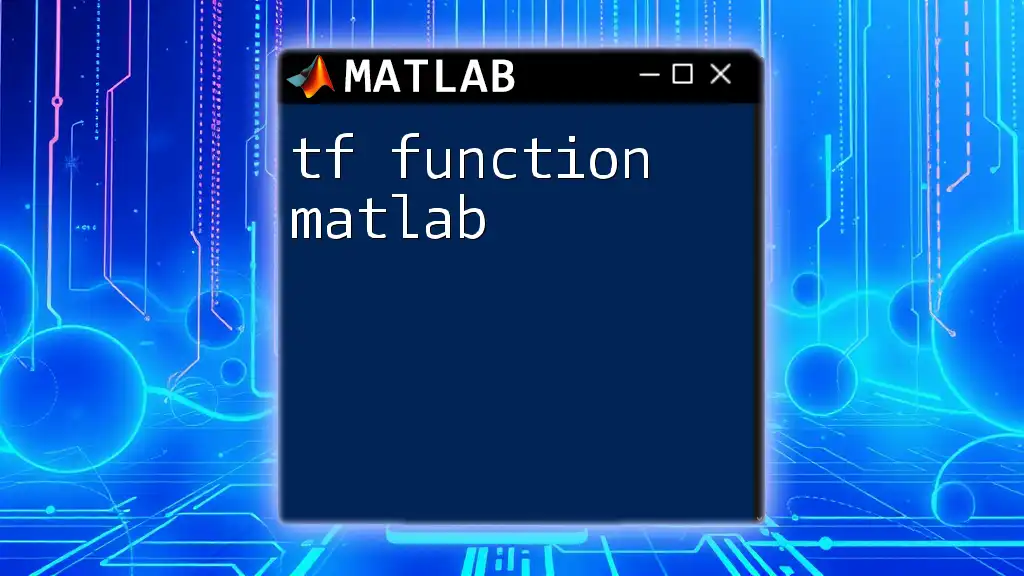
Additional Resources
For further learning, refer to the official MATLAB documentation, tutorials, and user forums. Books and online courses can also provide structured content to boost your knowledge. With continuous learning and practice, you can become proficient in utilizing functions in MATLAB to their fullest potential!