In MATLAB, vectors are one-dimensional arrays that can store a sequence of numbers or values, allowing for efficient data manipulation and mathematical operations.
% Creating a row vector
rowVector = [1, 2, 3, 4, 5];
% Creating a column vector
columnVector = [1; 2; 3; 4; 5];
What Are Vectors?
In MATLAB, a vector is defined as a one-dimensional array that can hold either a sequence of numbers or variables. Vectors can be categorized as row vectors, which are horizontal (1 x N), and column vectors, which are vertical (N x 1). Understanding vectors is crucial since they are one of the foundational elements in MATLAB programming, used extensively in mathematical computations, data analysis, and a multitude of engineering applications.
Importance of Vectors in MATLAB
Vectors serve as a fundamental data structure within MATLAB, simple yet powerful. They enable easy manipulation of lists of numbers, which can represent various quantities such as time series data, coefficients in equations, or features in machine learning. By mastering vectors, users can unlock the effective functionality that MATLAB provides for mathematical modeling and complex data analysis.
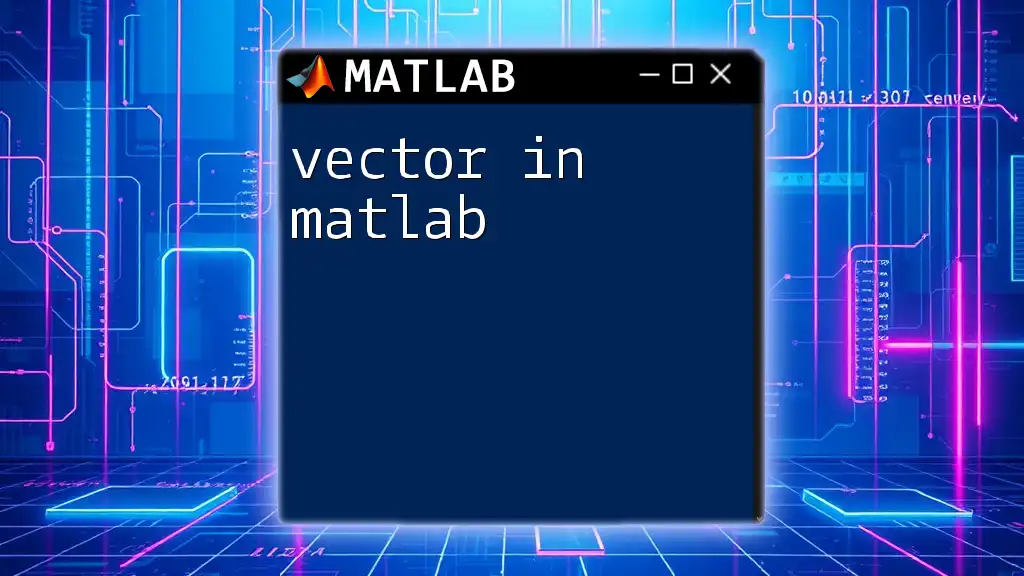
Creating Vectors in MATLAB
Row Vectors and Column Vectors
To create vectors in MATLAB, you can use either of the two formats:
-
A row vector is created using square brackets and separating elements by spaces or commas:
row_vector = [1, 2, 3, 4, 5]; % This creates a row vector
-
A column vector is similarly defined but with semicolons separating the elements:
col_vector = [1; 2; 3; 4; 5]; % This creates a column vector
Using the Colon Operator
The colon operator (`:`) allows for concise vector creation by specifying a starting value, an increment, and an ending value. For example:
vector_seq = 1:10; % Creates a row vector from 1 to 10
This will generate a row vector containing all integers from 1 to 10.
Using the `linspace` Function
When you need a vector containing evenly spaced values, the `linspace` function becomes very useful. It generates a vector based on a specified starting number, ending number, and the number of points required:
linspace_vector = linspace(1, 10, 5); % Creates 5 evenly spaced values between 1 and 10
In this case, the resulting vector would be `[1, 3.25, 5.5, 7.75, 10]`.
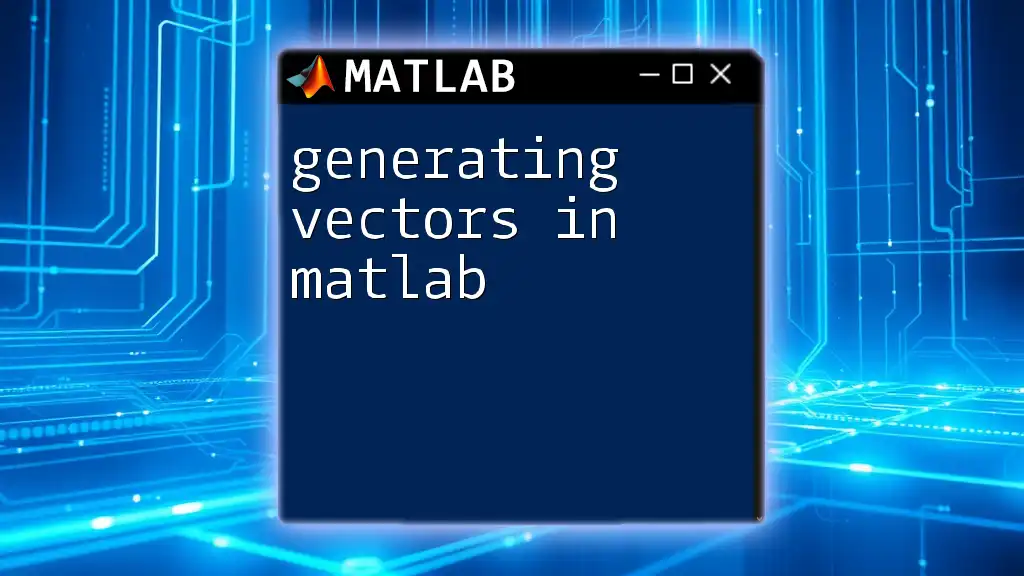
Accessing and Modifying Vectors
Indexing Vectors
MATLAB allows you to access vector elements using indexing, where the first element of a vector is referenced by 1 (not 0). For example, to access the third element of a vector:
element = row_vector(3); % Accesses the 3rd element
Slicing Vectors
You can extract a sub-vector through slicing by specifying a range of indices:
sub_vector = row_vector(2:4); % Extracts elements from index 2 to 4
In this example, `sub_vector` will contain the elements corresponding to the 2nd, 3rd, and 4th indices.
Modifying Elements
Modifying an element in a vector is straightforward. You can reassign values at specific indices:
row_vector(2) = 10; % Changes the 2nd element to 10
This operation will replace the second element of `row_vector` with the value 10.
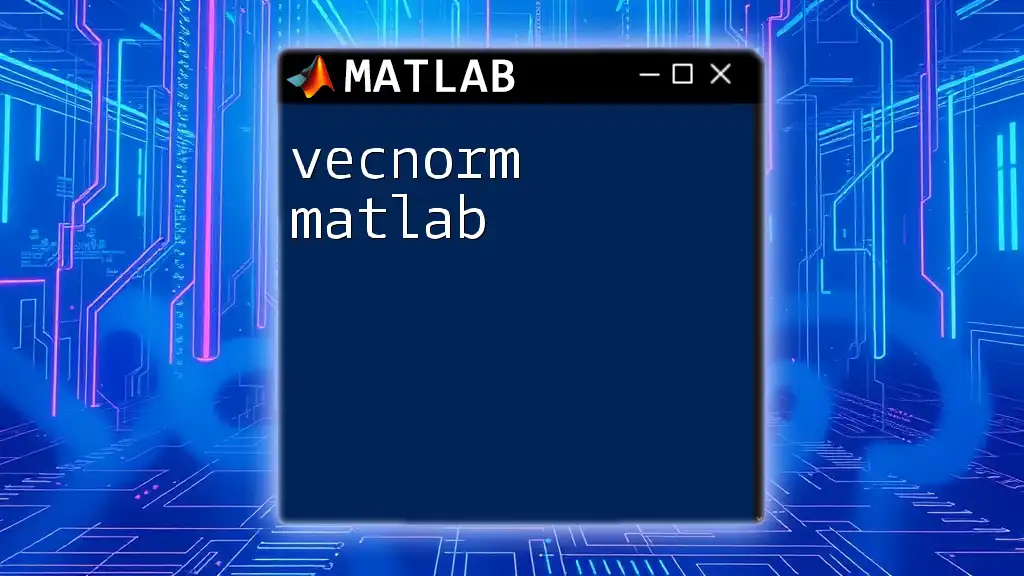
Vector Operations
Basic Arithmetic Operations
MATLAB supports element-wise arithmetic operations, allowing you to perform computations on vectors using simple operators. For example:
vector_a = [1, 2, 3];
vector_b = [4, 5, 6];
sum_vector = vector_a + vector_b; % Element-wise addition
In this scenario, `sum_vector` will yield `5, 7, 9`.
Dot Product and Cross Product
Vectors' relationships can also be assessed through operations such as the dot product and cross product. The dot product quantifies similarity between two vectors, while the cross product provides a vector perpendicular to two vectors in three-dimensional space.
% Dot product
dot_prod = dot(vector_a, vector_b); % Results in the scalar 32
% Cross product (only for 3D vectors)
cross_prod = cross(vector_a, vector_b); % Results in [-3, 6, -3]
Vector Length and Norm
Calculating the length and norm of a vector is essential when assessing its magnitude and performance. Use the following commands:
vec_length = length(vector_a); % Returns the number of elements in vector_a
vec_norm = norm(vector_a); % Computes the Euclidean norm of vector_a
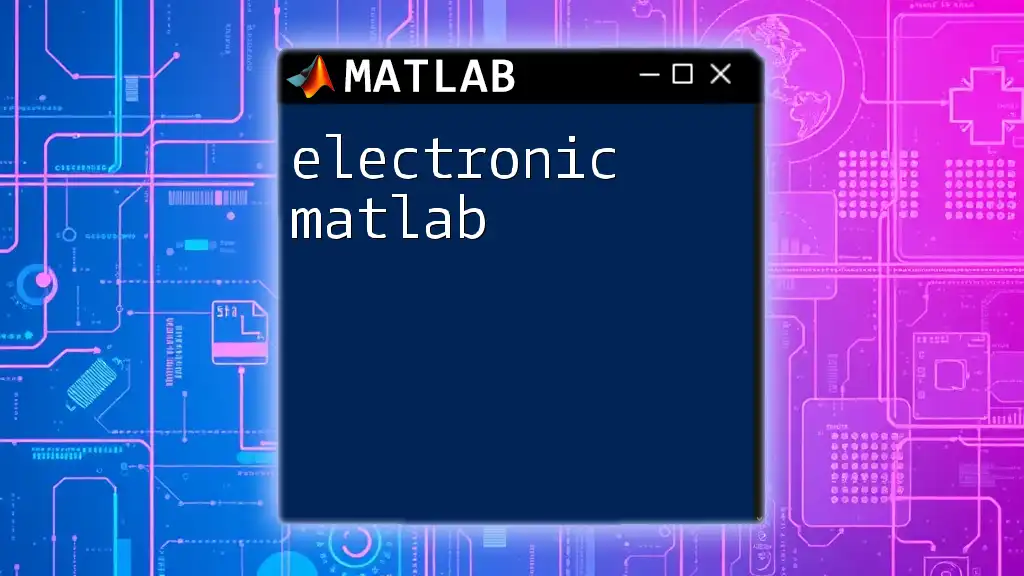
Advanced Vector Techniques
Reshaping Vectors
Using the `reshape` function allows you to reorganize a vector into a different dimensionality. For example, to change a 1D vector with 12 elements into a 3x4 matrix:
reshaped_vector = reshape(1:12, [3, 4]); % Reshapes vector into a 3x4 matrix
Concatenating Vectors
You can create new vectors by joining existing ones. This is called concatenation. Here’s a simple way to concatenate a row and a linearly spaced vector:
concatenated_vector = [row_vector, linspace_vector];
Using Logical Indexing
Logical indexing lets you create filtered vectors based on certain conditions. For instance, to extract elements that are greater than 3, you can use:
filtered_vector = row_vector(row_vector > 3); % Filters for elements greater than 3
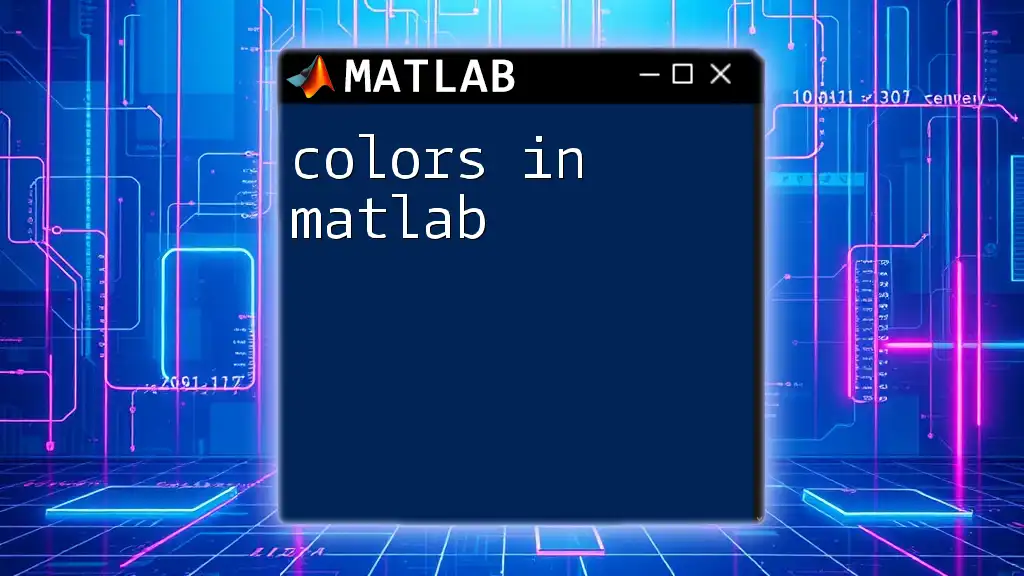
Common Applications of Vectors in MATLAB
Vectors find diverse applications across many domains:
Data Analysis
In data analysis, vectors are pivotal for handling raw data and performing statistical functions such as means, medians, and standard deviations.
Simulation and Modeling
Vectors support simulations in systems engineering and physics, facilitating the representation of variables influencing system behavior.
Machine Learning
In machine learning, vectors represent features for training models, enabling efficient computation and pattern extraction from data sets.
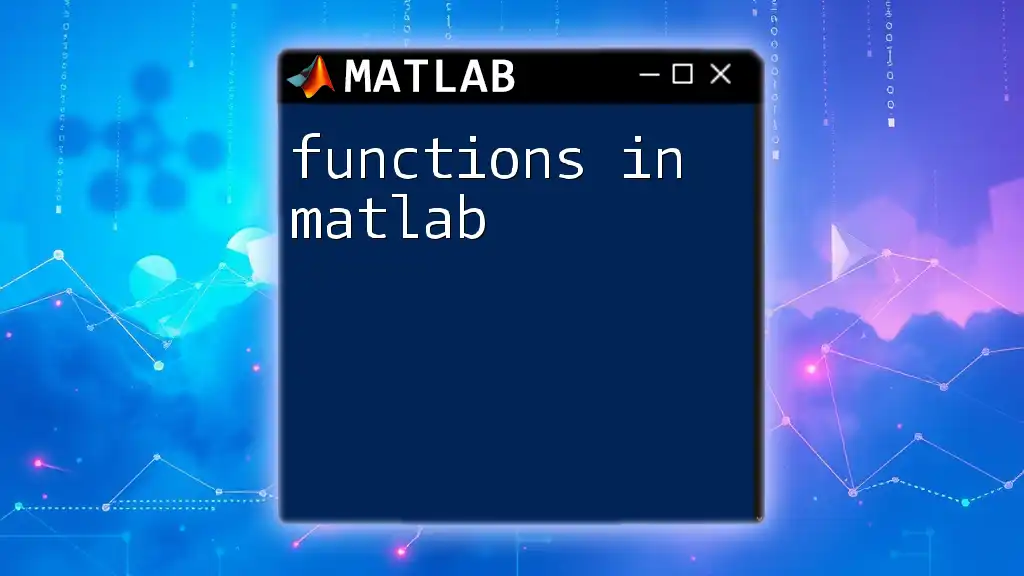
Conclusion
In this comprehensive guide on vectors in MATLAB, we explored their definition, creation methods, manipulation techniques, and various operations. Vectors are indispensable tools for effective programming within MATLAB, particularly useful across data analysis, engineering, and machine learning applications.
To deepen your understanding, practice regularly and apply these concepts to real-world problems. Explore additional resources to enrich your MATLAB skills and join our community for ongoing support tailored to your learning journey!